In C++, you can convert a binary string to an integer by using the `std::stoi` function with a specified base of 2.
#include <iostream>
#include <string>
int main() {
std::string binaryStr = "1011"; // Example binary string
int integerValue = std::stoi(binaryStr, nullptr, 2); // Convert binary string to integer
std::cout << "The integer value is: " << integerValue << std::endl; // Output: 11
return 0;
}
What is a Binary String?
A binary string is a sequence of characters consisting solely of the digits 0 and 1. In programming, binary strings are commonly used to represent binary numbers, which are fundamental for computer science and digital electronics. They often serve as a representation of data that can be processed by computers.

Why Convert Binary Strings to Integers?
Converting binary strings to integers is essential in various scenarios, such as computing, data manipulation, and algorithm design. When performing numerical operations, integers are easier and more efficient to work with than strings. For example, calculations, bitwise operations, and logical comparisons benefit greatly from numerical representation. The ability to convert binary strings to integers allows developers to handle data more effectively and optimize performance.

Understanding Binary Representation
What is Binary?
The binary number system is a base-2 numeral system that uses only two symbols: 0 and 1. Each binary digit (bit) represents an increasing power of 2, akin to how each digit in the decimal system represents an increasing power of 10. For instance, the binary number `1101` is calculated in decimal as follows:
- \( 1 \times 2^3 + 1 \times 2^2 + 0 \times 2^1 + 1 \times 2^0 = 8 + 4 + 0 + 1 = 13 \)
Significance of Binary in Computing
Computers fundamentally operate on binary. All data processing, storage, and transmission occur using binary representation. By understanding binary, programmers can optimize algorithms and manipulate data structures more effectively.

Overview of Converting Binary Strings to Integers in C++
When it comes to C++, there are various methods available to convert binary strings to integers. The most common methods involve either manual conversion using loops or utilizing built-in functions.

Method 1: Manual Conversion Using Loops
Concept Breakdown
The manual conversion method involves iterating through each character in the binary string, updating the resultant integer accordingly. To convert a binary string to an integer, start from the leftmost bit and calculate its contribution to the total value based on its position (power of 2).
Key considerations for manual conversion include handling invalid binary strings and leading zeros. A check for valid input, ensuring only '0' or '1' characters are present, is also critical.
Code Example: Manual Conversion
Here’s a simple implementation of binary string conversion using a for loop:
#include <iostream>
#include <string>
int binaryStringToInt(const std::string& binaryStr) {
int result = 0;
for (char bit : binaryStr) {
result = result * 2 + (bit - '0');
}
return result;
}
int main() {
std::string binaryStr = "1101";
std::cout << "The integer value is: " << binaryStringToInt(binaryStr) << std::endl;
return 0;
}
Explanation of Code
- In `binaryStringToInt`, the function initializes the result to 0.
- It iterates through each character in the string binaryStr.
- For each bit, the result is multiplied by 2 (shifting left in binary representation) and added to its integer equivalent (bit - '0').
- The output in `main` demonstrates how to implement the function and what results it produces: in this case, converting `1101` results in the integer value 13.
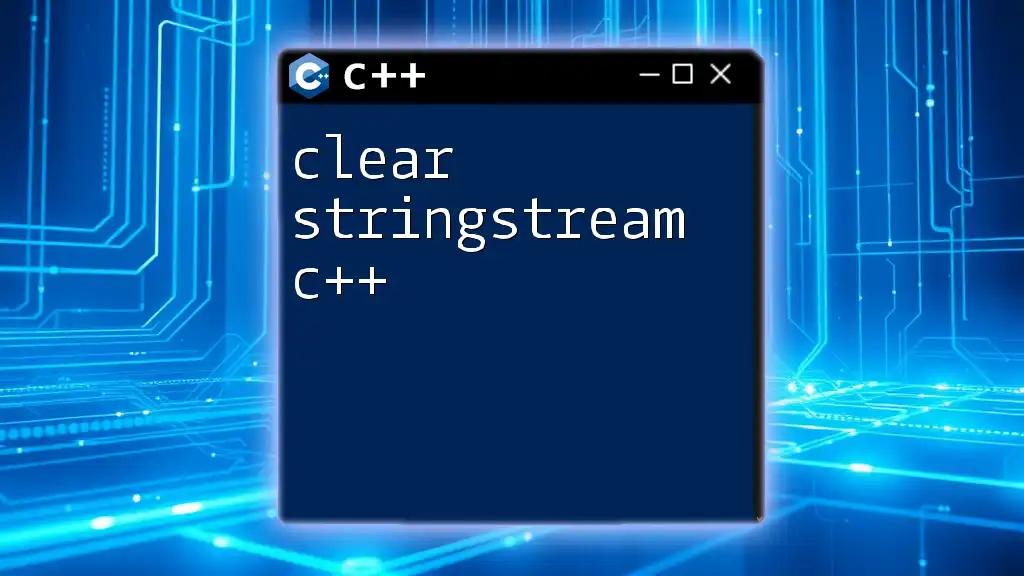
Method 2: Using Built-in Functions
The `std::stoi` Function
C++ provides a convenient built-in function, `std::stoi`, which can convert strings to integers with specified bases. By using base 2, you can transform binary strings directly into integers with minimal code.
Code Example: Using `std::stoi`
Here’s how to accomplish the conversion using `std::stoi`:
#include <iostream>
#include <string>
int main() {
std::string binaryStr = "1010";
int result = std::stoi(binaryStr, nullptr, 2);
std::cout << "The integer value is: " << result << std::endl;
return 0;
}
Explanation of Code
- In this example, `std::stoi` takes three parameters: the string to convert, a pointer that is not used (set as `nullptr`), and the base 2 to specify that the string is in binary format.
- When executing the above code with the binary string `1010`, the output will be 10, reflecting its correct integer representation.

Handling Various Scenarios
Validating Input Strings
It is crucial to validate the input string before conversion. Only characters 0 and 1 should be present. An example validation function could look like this:
bool isValidBinary(const std::string& str) {
for (char c : str) {
if (c != '0' && c != '1') {
return false;
}
}
return true;
}
This function iterates through each character and checks if they fall within the valid set of binary digits.
Handling Edge Cases
When working with binary strings, several edge cases must be handled to avoid errors:
- Empty Strings: An empty string should be managed gracefully by either returning 0 or throwing an exception.
- Strings with Illegal Characters: Input strings that contain characters other than 0 and 1 must be rejected or handle exceptions explicitly.

Performance Considerations
Complexity Analysis
When converting binary strings to integers, both the manual method and `std::stoi` have their respective complexities:
- Manual Conversion: Time complexity is \(O(n)\), where n is the length of the string. This is due to the need to iterate through each character.
- Built-in Method (`std::stoi`): Similarly has \(O(n)\) time complexity; however, it is optimized and offers better performance with standard libraries.
Best Use Cases
For operations involving simple conversion or one-off transformations, using `std::stoi` is recommended due to its simplicity and efficiency. On the other hand, manual conversion is beneficial when additional logic (like validation or special computation) needs to be incorporated.
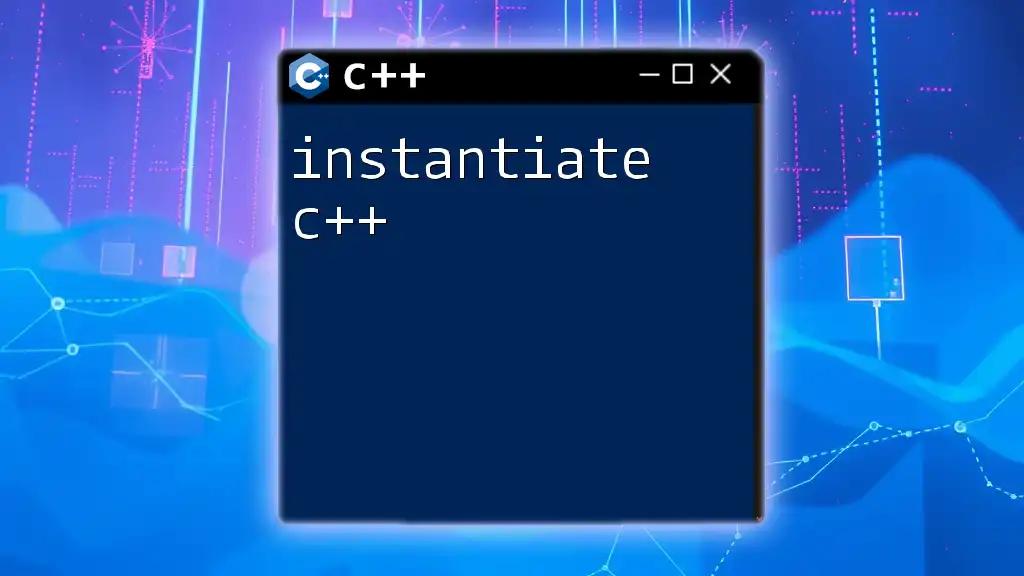
Conclusion
Converting a binary string to an integer in C++ is straightforward and essential for efficient data manipulation. Whether using a manual approach or the built-in `std::stoi` function, developers have the tools to handle binary data effectively. Understanding these methods not only eases programming tasks but also encourages improved performance and data handling practices.

Additional Resources
For further exploration, consider checking out:
- GitHub repositories related to C++ utilities and algorithms.
- Online compilers for quick testing and experimentation with code snippets.
- C++ documentation for a deeper dive into standard libraries and functions.
FAQs Related to Binary Conversion in C++
-
Can I convert a binary string with letters or symbols? No, only the characters 0 and 1 are valid in a binary string.
-
What happens if I programmatically convert an invalid binary string? It may result in undefined behavior or exceptions, depending on how you handle input validation.
By mastering the art of converting binary strings to integers, you can elevate your programming skills and optimize your applications for better performance in C++.