In C++, you can convert a string to an integer using the `std::stoi` function from the `<string>` library. Here's a code snippet that demonstrates this:
#include <iostream>
#include <string>
int main() {
std::string str = "42";
int num = std::stoi(str);
std::cout << num << std::endl; // Outputs: 42
return 0;
}
Understanding String and Integer Types in C++
What is a String in C++?
In C++, a string is typically represented using the `std::string` type provided by the Standard Library. This class encapsulates a dynamic array of characters, allowing for easy manipulation and storage. Strings are used to handle text, making them essential for tasks like user input, reading files, and string manipulation operations.
What is an Integer in C++?
An integer, or `int`, in C++ is a basic data type that represents whole numbers. It is often used for mathematical calculations, looping constructs, and indexing arrays. C++ offers several integer types, such as `int`, `short`, `long`, and `long long`, each with its own range and memory usage.
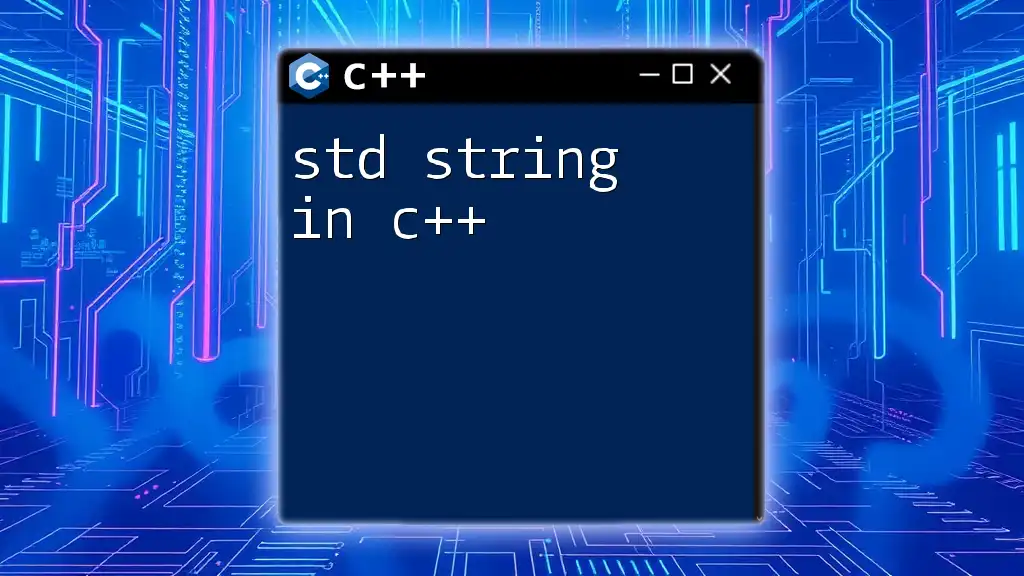
Basic Concept of Type Casting
What is Type Casting?
Type casting refers to the conversion of one data type to another. In C++, this is particularly important for ensuring that variables are handled correctly during compilation and execution. Proper casting helps maintain type safety, which prevents errors that could occur due to mismatched data types.
Casting Overview
-
Implicit Casting: This occurs when the compiler automatically converts one data type to another without explicit instructions. For instance, assigning an `int` to a `float` may not require a cast.
-
Explicit Casting: This occurs when the programmer specifies the conversion. This is often necessary when moving from a larger data type to a smaller one, such as converting a `double` to an `int`.
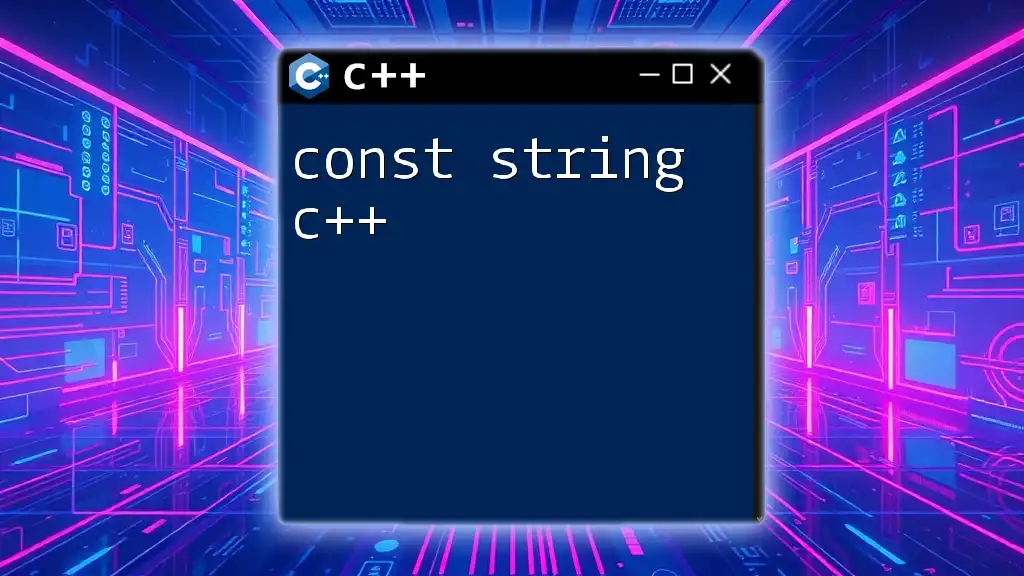
Methods to Convert String to Int in C++
Using `std::stoi` Function
The `std::stoi` function is one of the most straightforward methods for converting a string to an integer. It is part of the C++11 standard and provides a robust way to handle conversions, complete with error checking.
Code Example:
#include <iostream>
#include <string>
int main() {
std::string str = "123";
int number = std::stoi(str);
std::cout << "The integer is: " << number << std::endl;
return 0;
}
In this example, the string "123" is converted into the integer `123`. It is crucial to note that if the conversion fails (for example, if the string contains non-numeric characters), an exception of type `std::invalid_argument` will be thrown. This makes `std::stoi` a robust option for string-to-integer conversion.
Using `std::istringstream`
`std::istringstream` is a class that allows for input from strings. This capability can also be used for string to integer conversion.
Code Example:
#include <iostream>
#include <sstream>
int main() {
std::string str = "456";
std::istringstream iss(str);
int number;
iss >> number;
std::cout << "The integer is: " << number << std::endl;
return 0;
}
Here, the string "456" is read into an `istringstream` object, which allows for extraction into an integer variable. One key advantage of this approach is the ability to chain input extraction, making it suitable for complex parsing tasks.
Using `atoi` Function
The `atoi` function is a C-style method for converting strings to integers. While simpler, it has some drawbacks, such as not throwing exceptions on errors.
Code Example:
#include <iostream>
#include <cstdlib>
int main() {
const char* str = "789";
int number = std::atoi(str);
std::cout << "The integer is: " << number << std::endl;
return 0;
}
The `atoi` function works by converting the C-string (null-terminated) representation directly to an integer. However, it does not offer any error checking, which can lead to potential issues if the input is malformed.
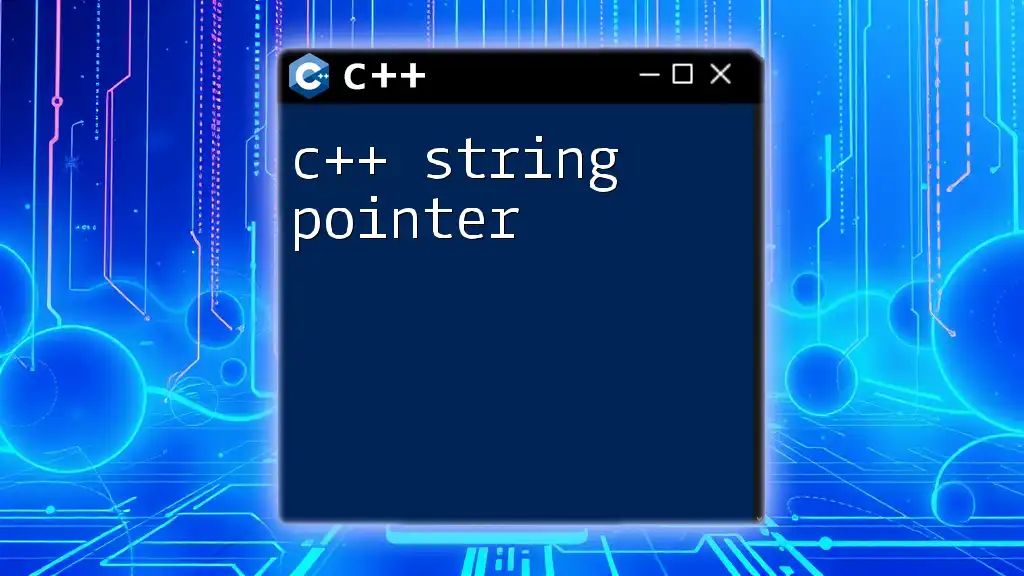
Handling Errors During Conversion
Common Errors When Converting String to Int
When casting strings to integers, there are several common pitfalls:
- Invalid Input Strings: Strings that contain alphabetic or special characters that cannot be converted (e.g., "abc", "4.5").
- Range Errors: Converting strings that represent numbers outside of the range of the target integer type can result in overflow or underflow.
Using Try-Catch for Exception Handling
To mitigate issues during conversion, using a try-catch block around the `std::stoi` conversion is advisable.
Example of Exception Handling with `std::stoi`:
#include <iostream>
#include <string>
int main() {
std::string str = "abc"; // Invalid string
try {
int number = std::stoi(str);
std::cout << "The integer is: " << number << std::endl;
} catch (const std::invalid_argument& e) {
std::cerr << "Invalid input: " << e.what() << std::endl;
} catch (const std::out_of_range& e) {
std::cerr << "Number out of range: " << e.what() << std::endl;
}
return 0;
}
In this example, if the string "abc" is passed to `std::stoi`, it will throw an `invalid_argument` exception, which is caught and appropriately handled, ensuring that your program does not crash.
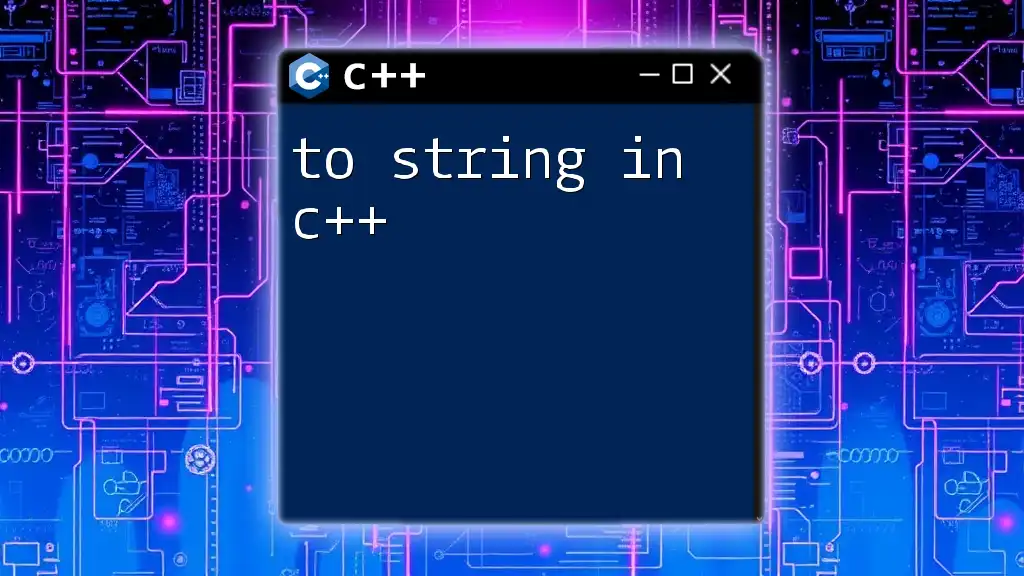
Performance Considerations
Performance of Different Methods
When selecting a method to cast a string to an int in C++, performance can vary:
- `std::stoi` is generally efficient and preferred for modern C++.
- `std::istringstream` can be slightly slower due to the overhead of creating a stream.
- `atoi` is faster than `std::stoi`, but lacks safety features, making it less suitable for production code.
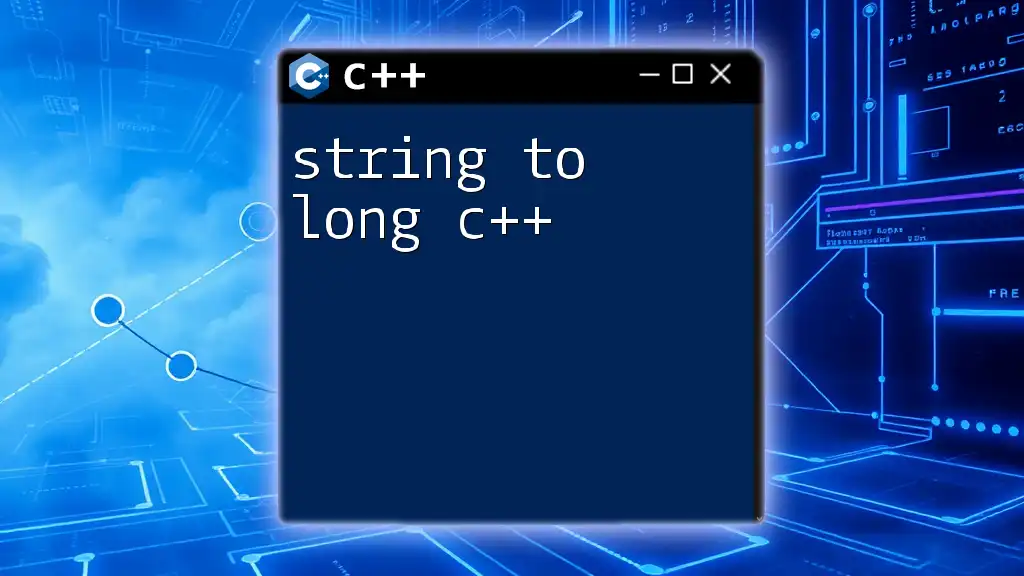
Best Practices for Converting Strings to Integers
Tips for Successful Conversion
- Validate Inputs: Always check if the string you want to convert is well-formed. Remove any unwanted characters beforehand if necessary.
- Use Exception Handling: Employ try-catch blocks with `std::stoi` to handle potential errors gracefully.
- Understand Method Selection: Choose the conversion method based on performance needs and whether error handling is a priority.
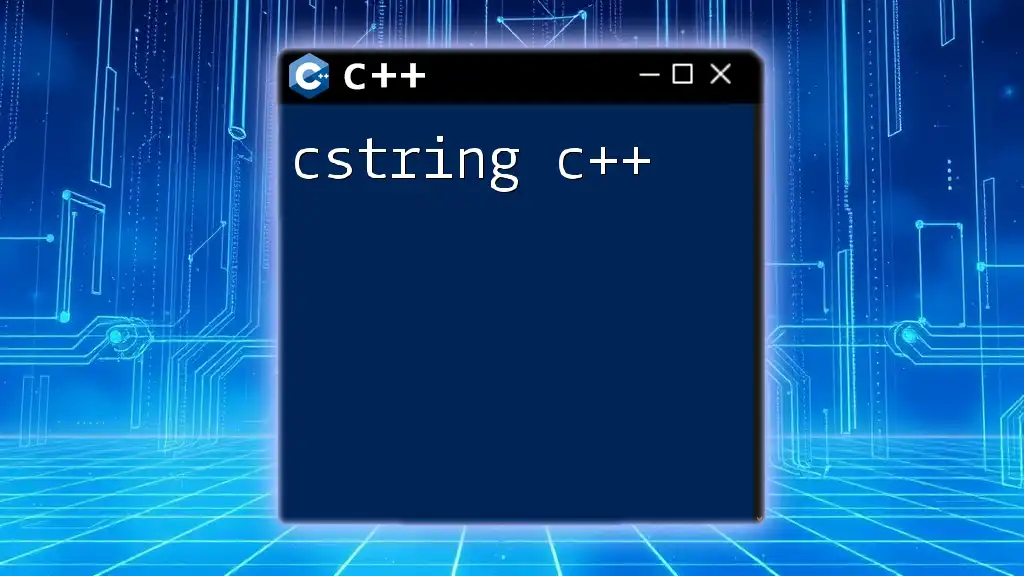
Conclusion
In mastering how to cast string to int in C++, knowing the right methods and error handling practices is vital. By utilizing functions like `std::stoi`, `std::istringstream`, and `atoi`, you can effectively convert strings to integers while minimizing the risk of runtime errors. The approach you choose should not only depend on ease of implementation but also on the robustness and performance needs of your application. Embrace the nuances of string-integer conversion to enhance your C++ programming skills.
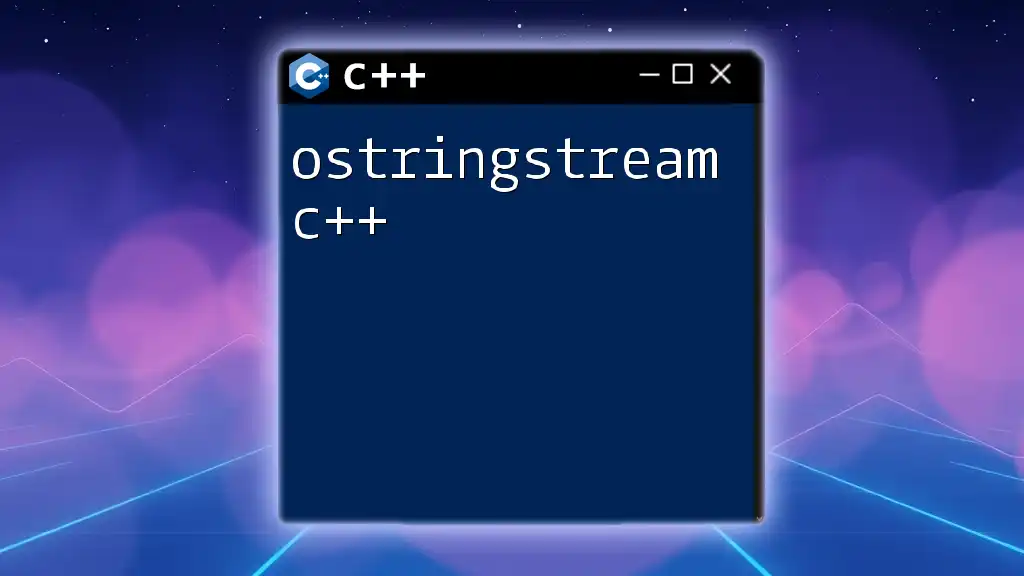
Additional Resources
For further learning, consider exploring official documentation and online courses focused on C++ programming. Understanding the best practices and underlying principles will help you become proficient in successfully converting strings to integers and handling conversions in complex applications.