In C++, you can return a string from a function by specifying the return type as `std::string` and using the `return` statement to send the desired string value back to the caller.
Here's a simple example:
#include <iostream>
#include <string>
std::string greet() {
return "Hello, World!";
}
int main() {
std::string message = greet();
std::cout << message << std::endl;
return 0;
}
Understanding Strings in C++
What is a String in C++?
In C++, a string is essentially a sequence of characters. You can store text with a string and manipulate it in various ways. Unlike other simple data types, C++ provides robust support for strings through the Standard Library.
Strings in C++ can be represented using two primary methods: character arrays and the `std::string` class from the Standard Library. The latter is preferred due to its flexibility and ease of use, allowing you to perform complex operations without worrying about memory management.
The Standard String Class
The `std::string` class offers a wide range of functionalities compared to traditional character arrays. It manages memory automatically, supports dynamic resizing, and provides numerous member functions for string manipulation—such as concatenation, substring extraction, and searching.
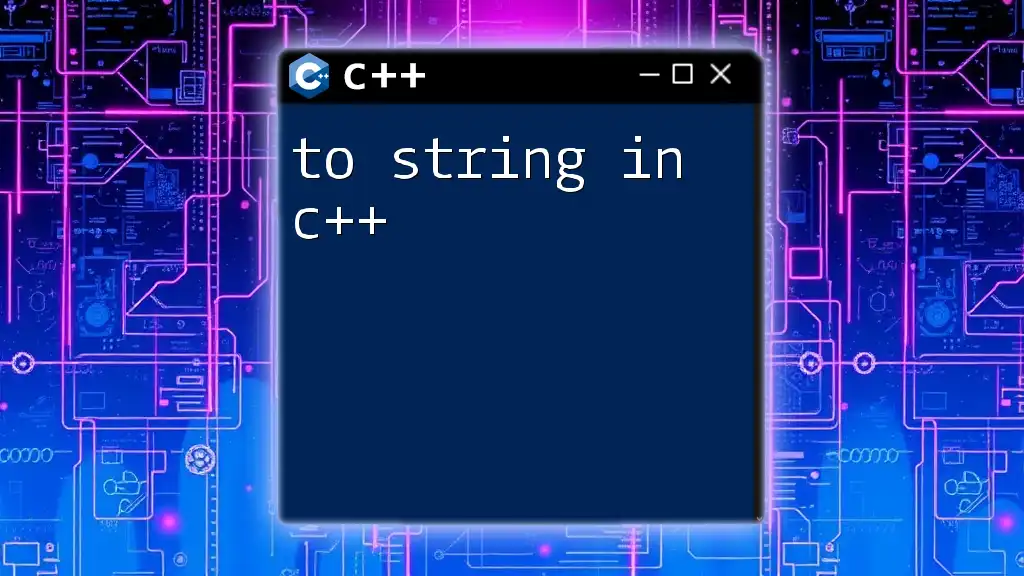
Returning a String in C++
Defining a Function to Return a String
In C++, functions can return strings just like any other data type. The syntax to define a function that returns a string involves specifying `std::string` as the return type.
For example:
std::string greet() {
return "Hello, World!";
}
In this snippet, the `greet` function will return the string "Hello, World!". The importance of the return type in the function signature indicates what will be sent back to the caller, ensuring type safety.
Using std::string as Return Type
Using `std::string` as a return type is typically preferred over character arrays due to its ease of use and built-in memory management. The following example demonstrates a function that personalizes the greeting based on user input:
std::string createGreeting(const std::string& name) {
return "Hello, " + name + "!";
}
In this function, the parameter `name` is passed as a constant reference to avoid unnecessary copying. The function then returns a personalized greeting, allowing you to concatenate strings effectively.
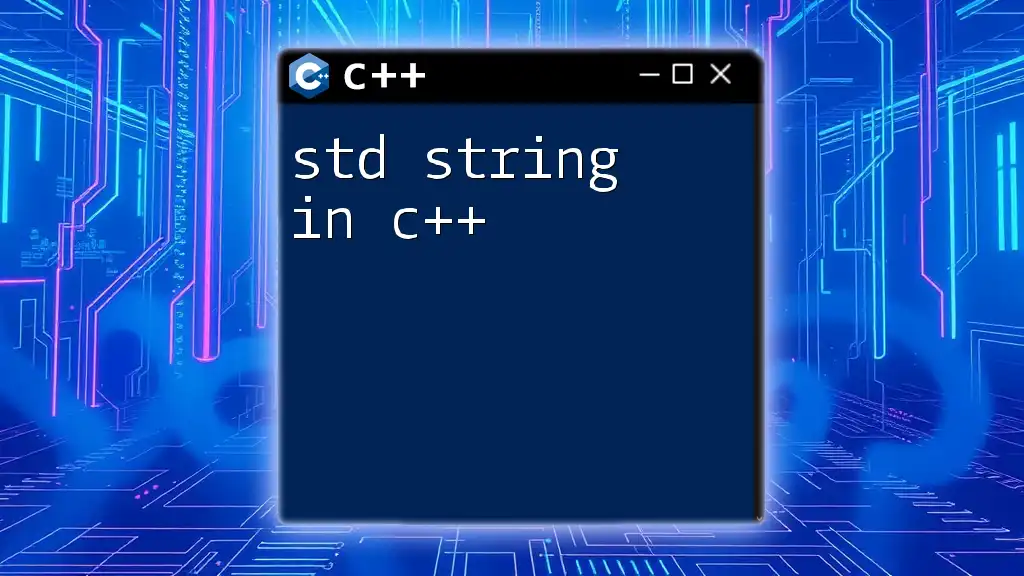
Memory Management and Returning Strings
Returning by Value vs. Reference
When designing functions that return strings, you have to consider whether to return by value or by reference.
-
Returning by Value: This method creates a copy of the string and returns it. It is generally safe and simple but can incur a performance cost, especially with large strings.
-
Returning by Reference: This allows the function to return a reference to an existing string. While this can enhance performance, it carries the risk of returning a reference to a local variable that goes out of scope, leading to undefined behavior.
Here’s an example of returning by reference:
std::string& modifyString(std::string& str) {
str += " modified";
return str;
}
This approach allows the original string passed to the function to be modified directly, which can be efficient.
Returning a String by Value
If you do not need to modify the original string, returning by value is straightforward and ensures that the returned string object is safe to use. Here’s how to do it:
std::string appendSuffix(const std::string& original) {
return original + "_suffix";
}
In this case, the `appendSuffix` function creates a new string with "_suffix" added to the original, demonstrating how simple and effective returning by value can be.
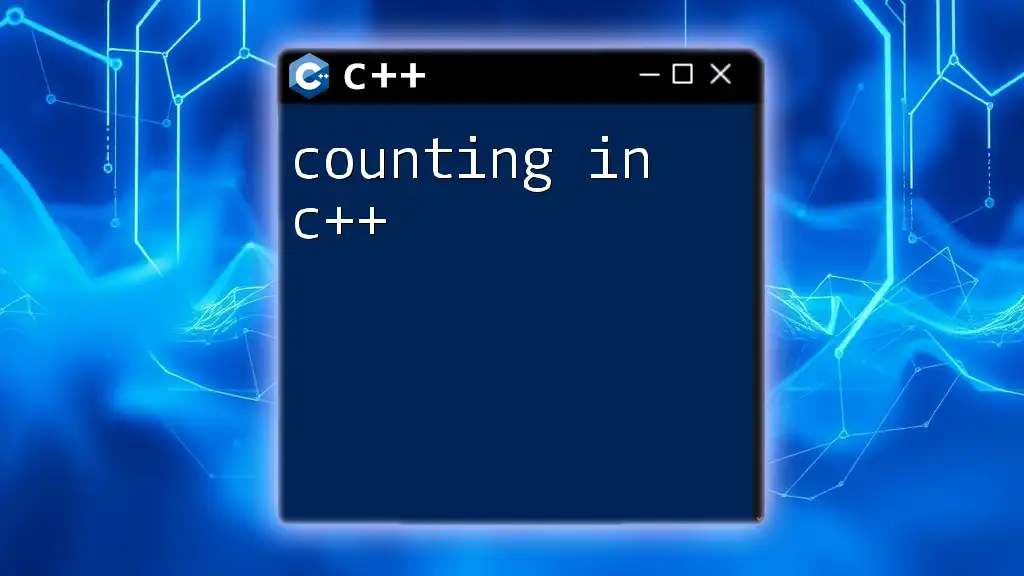
Common Mistakes and How to Avoid Them
Forgetting to Return a String
One common mistake in C++ is omitting a return statement. If a function that is expected to return a string lacks a return statement, it will lead to undefined behavior.
For example:
std::string faultyFunction() {
// Missing return statement
}
In this case, the absence of a return statement could confuse the compiler, and depending on the context, the behavior might lead to crashes or incorrect outputs.
Returning Local Variables
Another significant pitfall is returning a pointer to a local variable within a function. Since local variables go out of scope once the function exits, any references to them will be invalid.
For instance:
std::string* getLocalString() {
std::string temp = "Hello";
return &temp; // Dangerous: temp is out of scope
}
This function attempts to return a pointer to `temp`, which will no longer exist after the function returns. This code can lead to crashes or corrupt data, so it must be avoided.
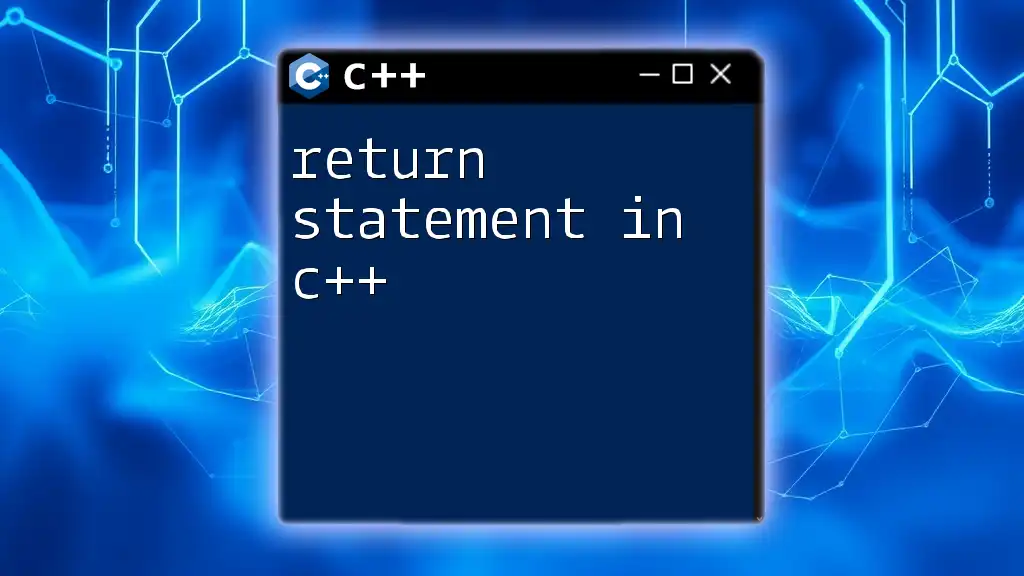
Advanced Techniques
Returning Strings in Classes
When working with classes, member functions can also return strings. This allows you to encapsulate string data within classes effectively.
For example:
class Person {
public:
std::string getName() const {
return name;
}
private:
std::string name;
};
In this code snippet, the `getName` member function returns the value of the `name` variable, enabling access to the class's data in a safe manner.
Utilizing String Streams
C++ also offers a powerful feature called string streams, encapsulated in the `std::ostringstream` class. This class can be used to build complex strings in a convenient way.
For instance:
std::string formatMessage(const std::string& input) {
std::ostringstream oss;
oss << "Formatted: " << input;
return oss.str();
}
In this example, `std::ostringstream` constructs a formatted string from the input. This technique is particularly useful when you want to combine multiple data types into a string seamlessly.
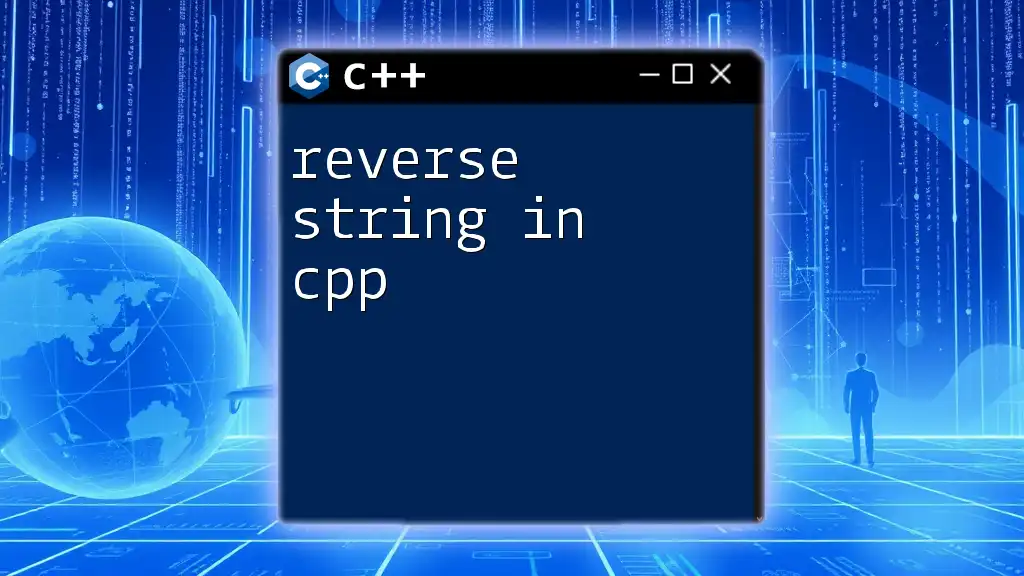
Conclusion
Returning strings in C++ is not only straightforward but also crucial for effective program design. Understanding how to manage string functions—whether by value or reference—alongside common pitfalls is essential for writing robust and maintainable code. By practicing the examples provided in this article, you can develop a solid foundation for working with strings in C++.
Consider dive deeper into C++ to discover more advanced string manipulation techniques. With practice and exploration, you'll master the art of returning strings!
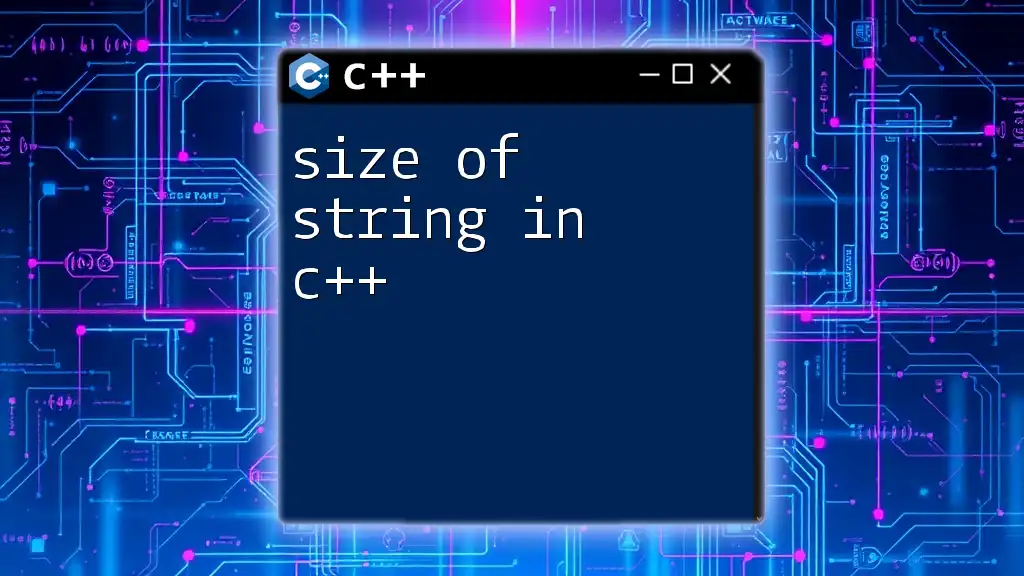
Further Reading and Resources
To enrich your knowledge further, consult the official documentation for `std::string`, explore C++ best practices, and take advantage of additional resources like video tutorials or hands-on coding exercises. Happy coding!