In C++, you can convert a `float` to a `string` by using the `std::to_string()` function from the `<string>` header. Here's a code snippet demonstrating this:
#include <iostream>
#include <string>
int main() {
float number = 3.14f;
std::string str = std::to_string(number);
std::cout << "The float as a string is: " << str << std::endl;
return 0;
}
Understanding the Float Data Type
A float in C++ is a single-precision floating-point data type designed to handle decimal points and very large or small numbers. It typically occupies 4 bytes of memory, allowing for a fair amount of precision, but not as much as a double, which is a double-precision type. Common usage scenarios for float include mathematical calculations, graphics programming, and scientific computations, thanks to its efficient memory usage.
Key characteristics of the float in C++ include:
- Precision: A float can generally represent numbers accurately up to six decimal places. This is important to note when dealing with calculations that require precision.
- Range: Floats can represent a vast range of values, but with the trade-off of precision, which might lead to rounding errors if not managed properly.
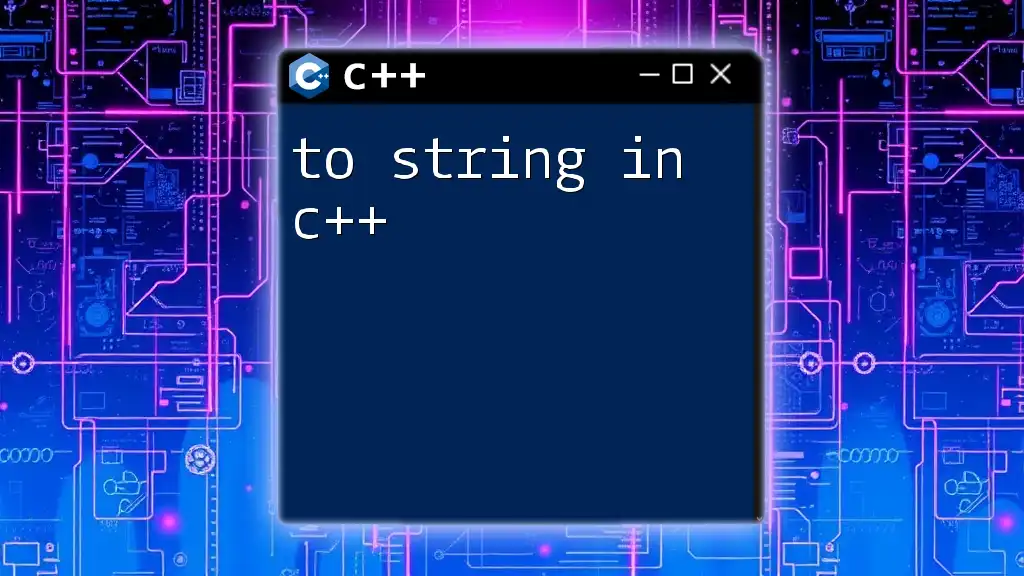
Why Convert Float to String?
Converting floats to strings is essential in many real-world programming applications. Here are a few specific scenarios:
- Formatting Output: When generating user interfaces or reports, displaying numerical values as strings is often necessary.
- Text-Based Interfaces: In command-line applications or logging systems, it's imperative to output numbers in a human-readable format.
Moreover, if you are working with databases, APIs, or file I/O operations, you often need to convert numeric data types like float into strings.
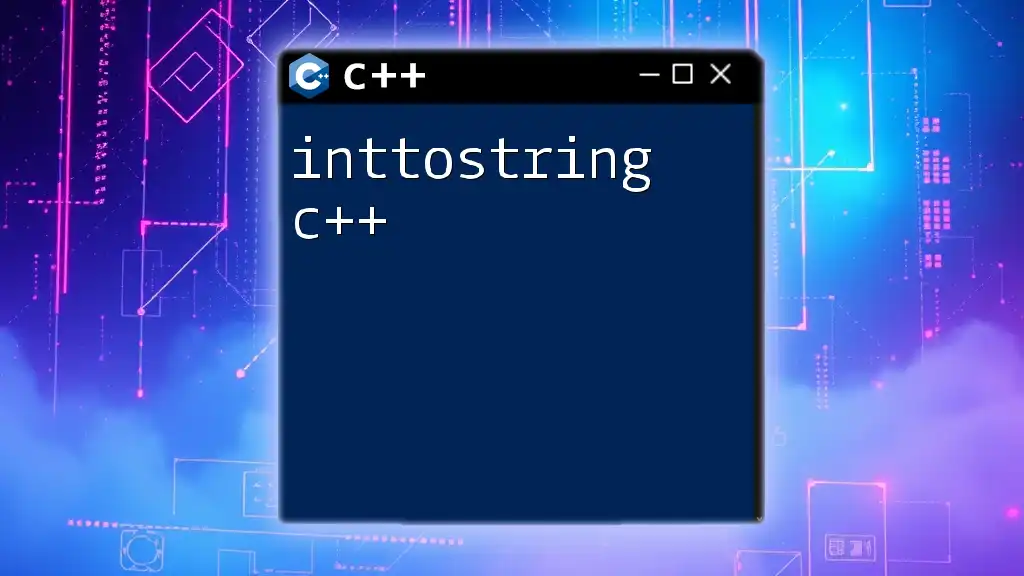
Methods for Converting Float to String in C++
Using std::to_string
The simplest way to convert a float to a string in C++ is by using the `std::to_string` method, which is available in the `<string>` header. This function takes a float as an argument and returns a string representation.
Here’s the basic syntax and example of how to use it:
float number = 3.14;
std::string str = std::to_string(number);
However, keep in mind that `std::to_string` may not offer precise control over the string's formatting or precision. The conversion could result in more decimal places than expected, which might not always be desirable depending on your application’s requirements.
Using std::ostringstream
If you need more control over the formatting, std::ostringstream from the `<sstream>` header allows for a more flexible conversion. This method enables you to manipulate the string format, including deciding on the number of decimal places.
Here’s how you can use `std::ostringstream` effectively:
#include <sstream>
float number = 3.14159;
std::ostringstream oss;
oss << number; // Insert the float into the stream
std::string str = oss.str(); // Convert stream to string
Using std::ostringstream also allows you to format the float output, making it a favored method among C++ developers looking for clarity and precision in their output.
Using sprintf (C-style)
For those accustomed to the C programming language, the `sprintf` function offers a classic approach to converting floats to strings. Although it may require more caution regarding buffer overflow issues, it remains popular due to its formatting capabilities. Here's an example of how you could use it:
char buffer[50]; // Allocate sufficient buffer size
float number = 2.71828;
sprintf(buffer, "%.2f", number); // Format to 2 decimal places
std::string str(buffer); // Convert char array to string
While this method allows you to control the formatting easily, it is vital to be cautious about the buffer size to avoid overflow errors.
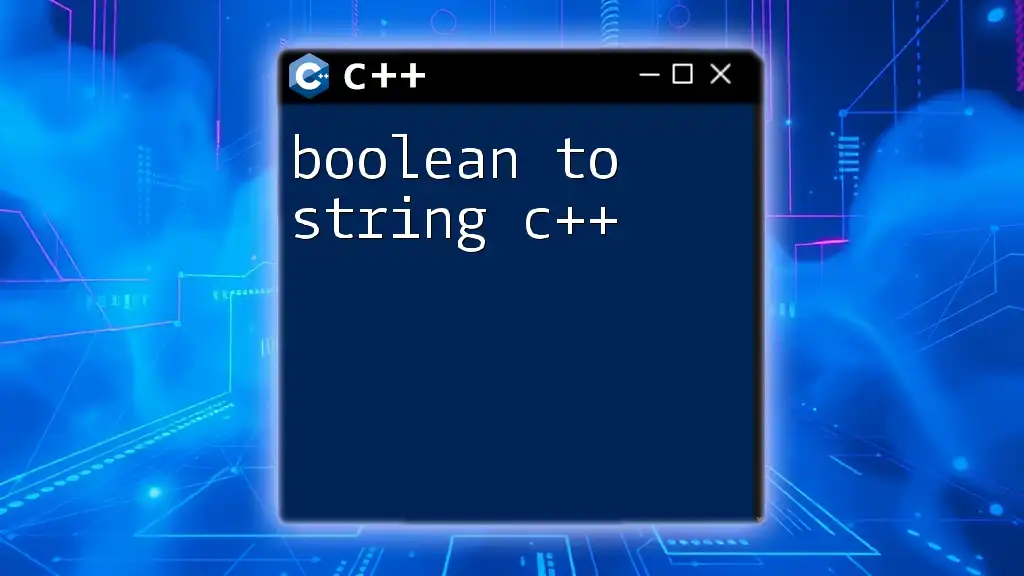
Handling Precision and Format
Precision management is a crucial aspect of converting floats to strings. Often, applications only require a specific number of decimal places. This can be effectively controlled both with `std::ostringstream` and `sprintf`.
For `std::ostringstream`, you can use std::fixed and std::setprecision from the `<iomanip>` library to specify how many decimal places you want. Here’s an example:
#include <iomanip>
#include <sstream>
float number = 3.14159;
std::ostringstream oss;
oss << std::fixed << std::setprecision(2) << number; // Set to 2 decimal places
std::string str = oss.str();
This snippet will render the float as `3.14`, ensuring that the output matches your formatting requirements.
When dealing with extremely large or small float values, you may also encounter scientific notation. It might often be necessary to convert these scientific forms into standard string formats, and std::ostringstream allows for straightforward management of this.
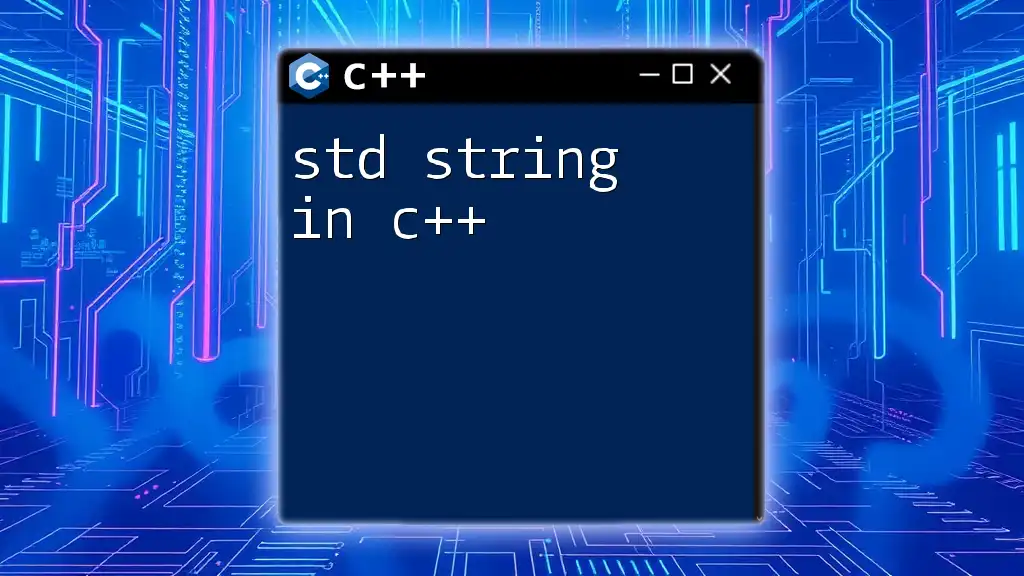
Conclusion
In summary, converting a float to string in C++ can be achieved in various ways, each with its advantages and disadvantages. The std::to_string method is quick and straightforward but lacks detailed control over formatting, while std::ostringstream provides robust formatting options, and sprintf allows for traditional C-style formatting.
When choosing the best method for conversion, consider the context of your application, including the need for precision and formatting options. Experimenting with various methods in personal projects will enhance your skills and confidence in handling data type conversions in C++.
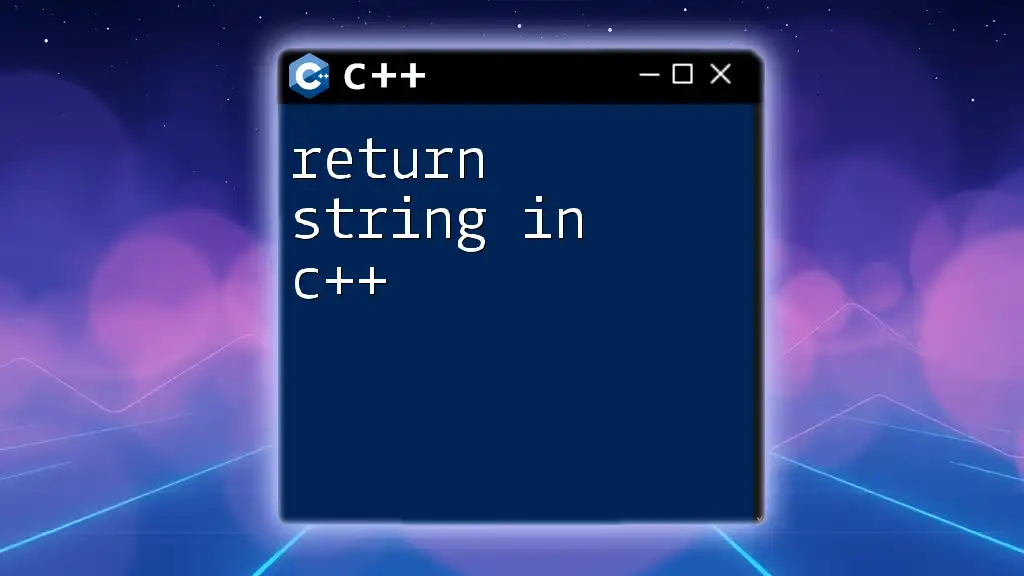
Further Resources
For additional learning, consider exploring the following resources to deepen your understanding of C++ data types and their manipulation:
- C++ Documentation on Data Types
- Programming Forums and Communities (such as Stack Overflow)
- Recommended books on C++ programming fundamentals
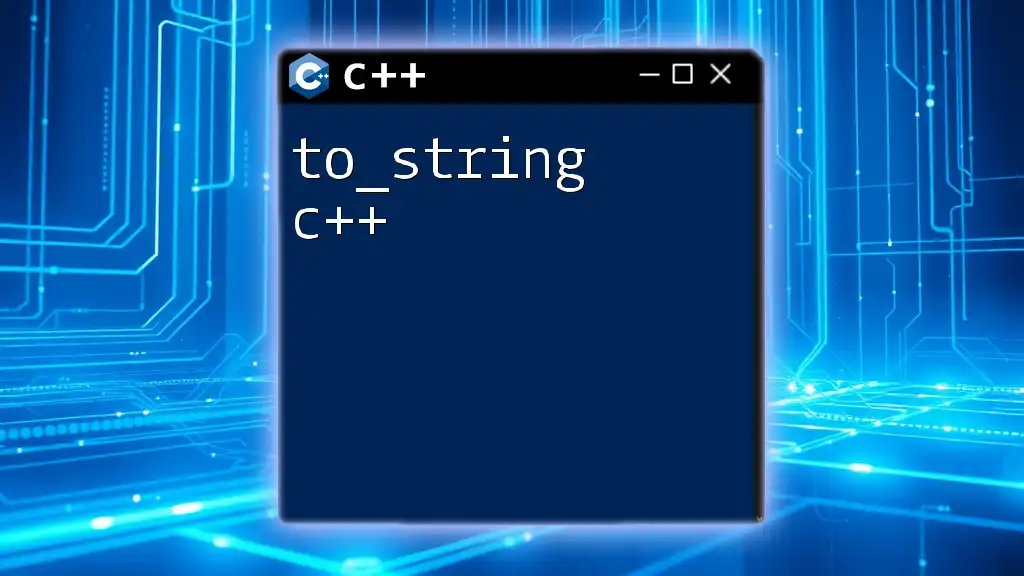
Call to Action
Feel free to leave comments with your questions or share your own experiences with float to string conversion in C++. Don’t forget to subscribe for more quick C++ tutorials and tips!