In C++, you can convert a double value to an integer using static_cast, which truncates the decimal portion of the double when converting.
double myDouble = 3.14;
int myInteger = static_cast<int>(myDouble); // myInteger will be 3
Understanding Doubles and Integers
What is a Double in C++?
A double in C++ is a data type used to represent floating-point numbers, which consist of decimal values. It offers higher precision compared to the `float` data type, typically using 64 bits to store the value. This makes it suitable for applications requiring a large range of values and cumulative calculations, such as scientific computation, graphics programming, and financial applications.
What is an Integer in C++?
An integer is a data type that represents whole numbers without decimals. In C++, the default `int` type is usually 32 bits long, allowing integers to cover a range from approximately −2.1 billion to +2.1 billion. Integers are frequently used for counting, indexing, and controlling program flow (e.g., loops).
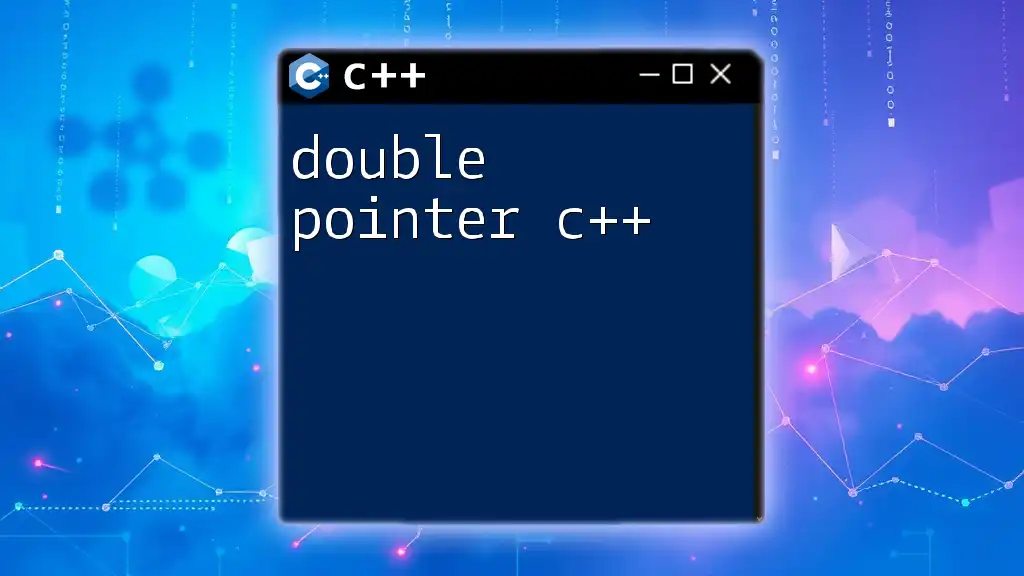
The Need for Conversion: Double to Integer
Why Convert Double to Integer?
In many scenarios, you may find it necessary to convert a double to an integer. Some common reasons include:
- Memory Optimization: In environments where memory is critical, using integers typically consumes less space than using doubles.
- Indexing: Arrays and data structures often require integer indices, making conversion essential.
- Rounding: When numerical precision is less critical, converting doubles to integers can simplify calculations.
Potential Issues with Conversion
While converting a double to an integer can be useful, it's essential to understand potential pitfalls such as precision loss and truncation. When a double value is converted, the decimal part is discarded, leading to a value that may not represent the original double accurately. For example, converting `9.57` directly to an integer will yield `9`, losing the `.57` portion entirely.
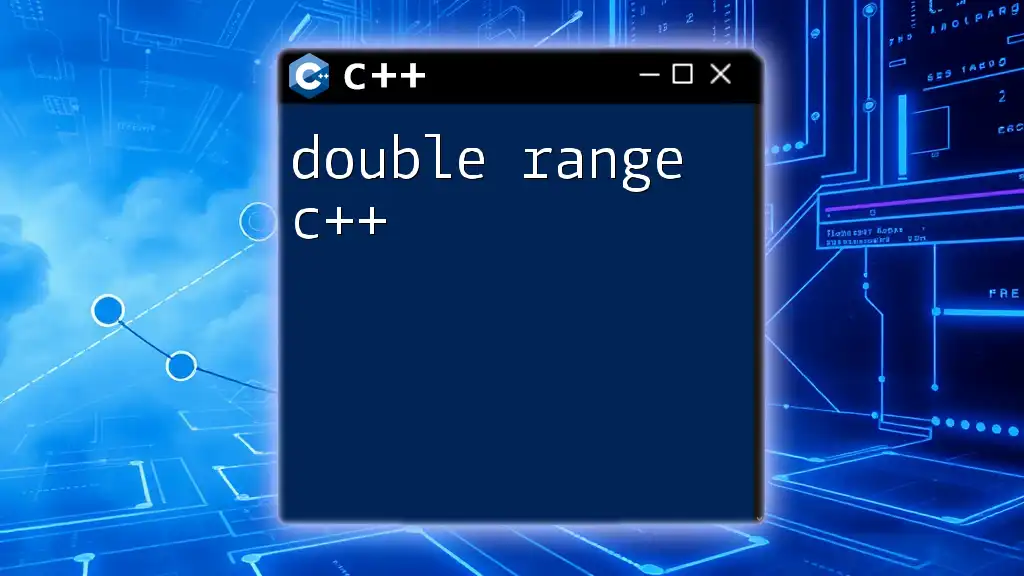
Methods to Convert Double to Integer in C++
Using `static_cast`
Explanation of `static_cast`
In C++, `static_cast` provides a safe and explicit way to convert data types, ensuring type safety during the conversion. It is widely regarded as the preferred method for type casting due to its clarity and intent.
Code Example:
double num = 9.57;
int intNum = static_cast<int>(num);
// Output: intNum = 9
Explanation of Example
In this example, the variable `num` is assigned the value `9.57`. When we convert it to an integer using `static_cast`, we retain only the whole number portion, resulting in `9`. The rest of the decimal is discarded, demonstrating how truncation occurs during conversion.
Using C-style Casting
Explanation of C-style Casting
C-style casting is another method that offers a more straightforward approach but is less safe than `static_cast`. It involves placing the target type in parentheses before the variable being converted.
Code Example:
double num = 9.57;
int intNum = (int)num;
// Output: intNum = 9
Explanation of Example
This code achieves the same result as the previous example. Although this method is simpler, it doesn't provide the clarity and safety offered by `static_cast`. Thus, it's generally recommended to use `static_cast` for better readability.
Using the `floor()` Function
Overview of the `floor()` Function
The `floor()` function, provided in the `<cmath>` library, rounds down a double value to the nearest whole number. This method is beneficial when always wanting to round down without affecting the integer result.
Code Example:
#include <cmath>
double num = 9.57;
int intNum = static_cast<int>(floor(num));
// Output: intNum = 9
Explanation of Example
Here, `floor(num)` rounds the value of `9.57` down to `9`, which is then converted to an integer. This method ensures that you round down rather than simply truncating, which is useful in specific scenarios, such as indexing and maintaining lower bounds in calculations.
Using the `round()` Function
Overview of the `round()` Function
The `round()` function rounds a double value to the nearest integer—if the decimal part is `0.5` or higher, it rounds up; otherwise, it rounds down.
Code Example:
#include <cmath>
double num = 9.57;
int intNum = static_cast<int>(round(num));
// Output: intNum = 10
Explanation of Example
In this case, `round(num)` rounds up the value to `10` because the decimal portion `.57` is greater than `0.5`. This function is particularly useful when you want to maintain mathematical correctness for values that fall in the middle of two integers.
Using the `ceil()` Function
Overview of the `ceil()` Function
The `ceil()` function always rounds up to the nearest whole number, regardless of the decimal portion. This is advantageous in scenarios where you need to ensure that the result is not less than the original double value.
Code Example:
#include <cmath>
double num = 9.57;
int intNum = static_cast<int>(ceil(num));
// Output: intNum = 10
Explanation of Example
In this example, using `ceil()` on `9.57` results in `10`, providing a guarantee that the outcome will never be less than the original double value. This is particularly useful in certain algorithmic applications, such as calculating the total number of items needed based on non-integer results.
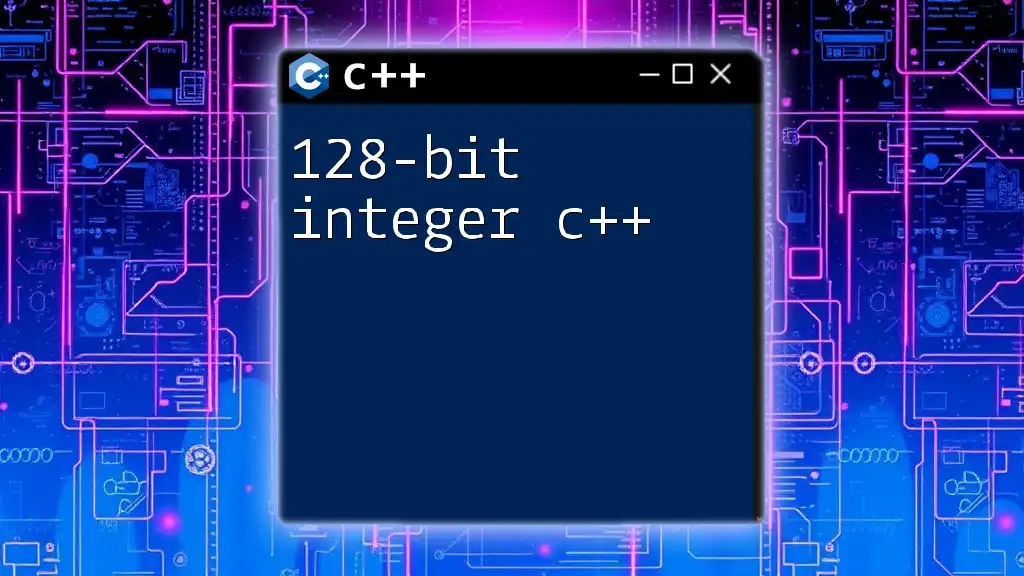
Best Practices and Considerations
Choosing the Right Conversion Method
To choose the right conversion approach when converting a double to an integer, consider the following factors:
- Precision Needs: If retaining the decimal precision is critical, consider using rounding functions.
- Performance: For performance-sensitive scenarios, `static_cast` is often the best option due to its clearer intent.
- Desired Outcome: Decide whether you want truncation (use `static_cast`), rounding down (`floor()`), rounding to the nearest integer (`round()`), or always rounding up (`ceil()`).
Handling Errors and Exceptions
When performing conversions, it’s important to handle any potential errors gracefully. Here are some strategies:
- Check Validity: Ensure that the double value is within a range that can be accurately represented by the integer type.
- Avoiding Overflows: Be mindful of situations where a double value might exceed the maximum range of an integer type, which can lead to undefined behavior.
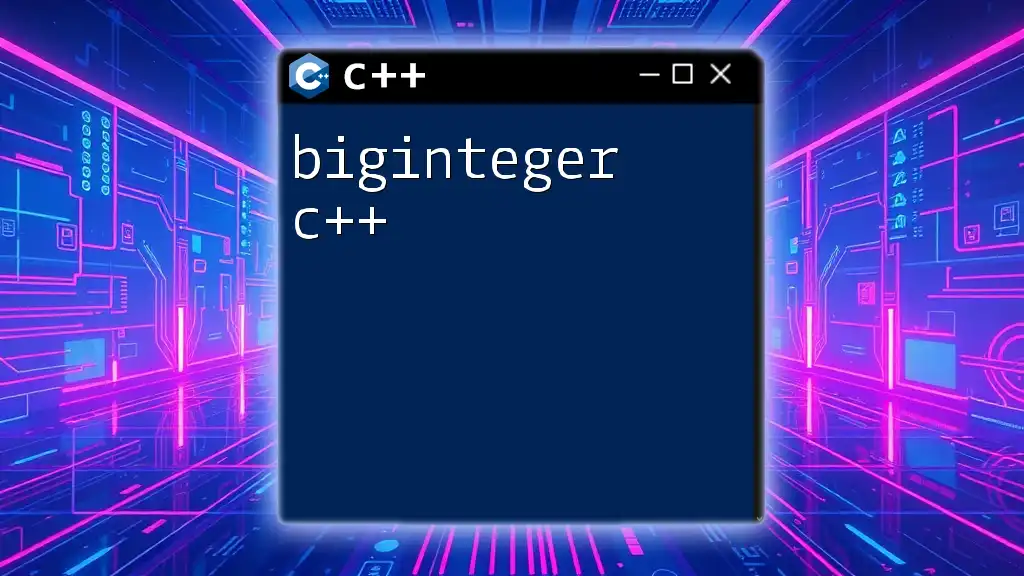
Conclusion
Converting double to integer in C++ is a fundamental concept that every programmer should master. By understanding the methods and implications of conversion, you can make informed decisions that enhance program accuracy and efficiency. Whether you choose `static_cast`, C-style casting, or functions like `floor()`, `round()`, and `ceil()`, the key is to align your choice with the needs of your application.
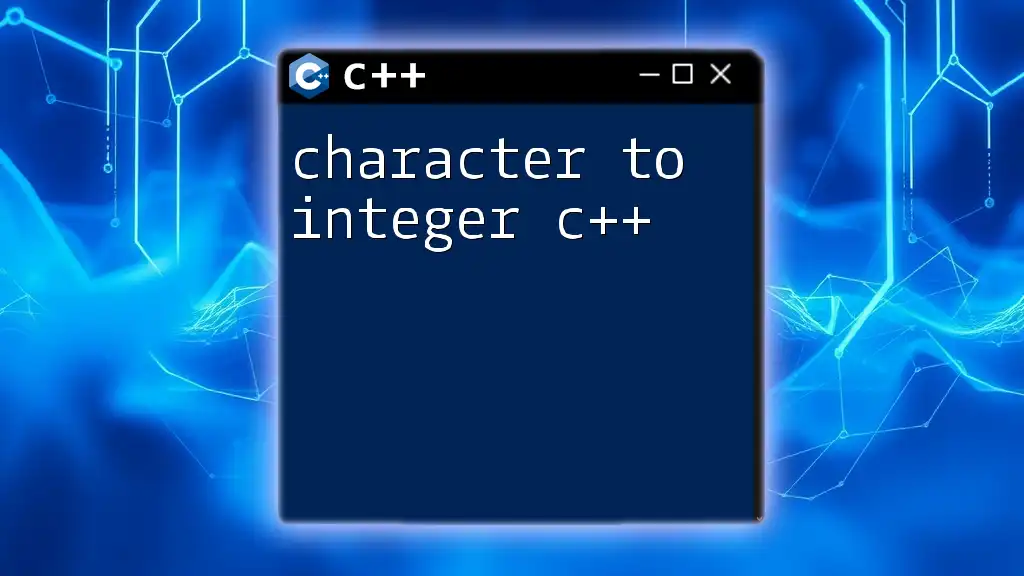
Additional Resources
For a deeper understanding, consider exploring the official C++ documentation or foundational texts that delve into data types and conversions. Books on C++ programming can also provide additional context and examples to reinforce your learning.
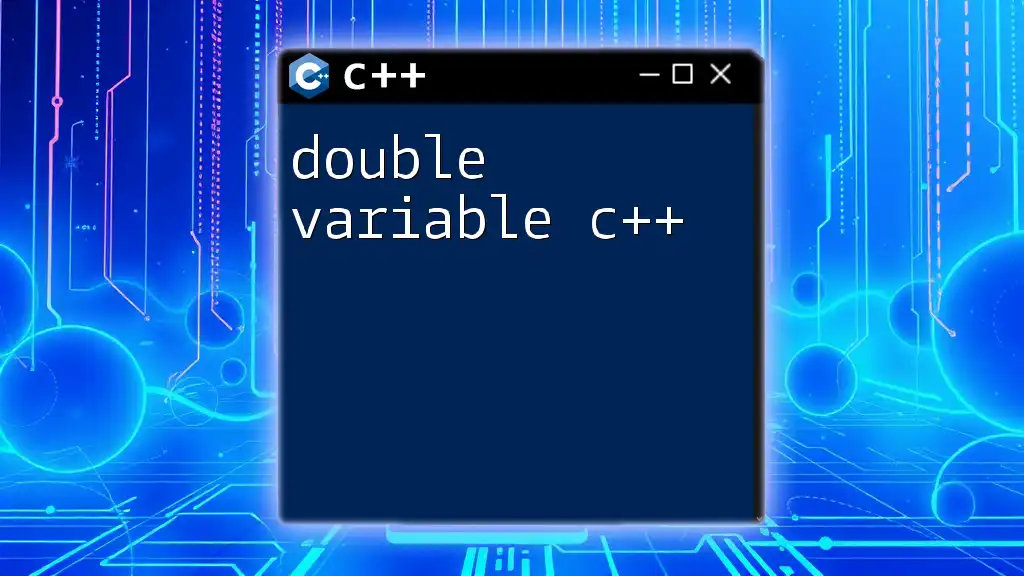
Call to Action
Now that you have a thorough understanding of converting doubles to integers, try practicing with the provided examples! Follow us for more tips and tricks on mastering C++ commands and improving your programming skills.