In C++, the double ampersand `&&` is a logical operator used to perform a logical AND operation, evaluating to true only if both operands are true.
bool a = true;
bool b = false;
bool result = a && b; // result will be false
Understanding Logical Operators
What are Logical Operators in C++?
In C++, logical operators are crucial for making decisions based on Boolean expressions. They allow you to combine multiple expressions and evaluate their truthiness. The three primary logical operators in C++ include:
- Logical AND (`&&`): Yields true if both operands are true.
- Logical OR (`||`): Yields true if at least one operand is true.
- Logical NOT (`!`): Yields true if the operand is false.
Significance of the Double Ampersand
The double ampersand (`&&`) is fundamental in C++ for performing logical AND operations. While the single ampersand (`&`) can also be used for logical operations, it acts more like a bitwise AND, which does not short-circuit. Therefore, understanding the distinction between these operators is crucial for optimal logical evaluations and performance.
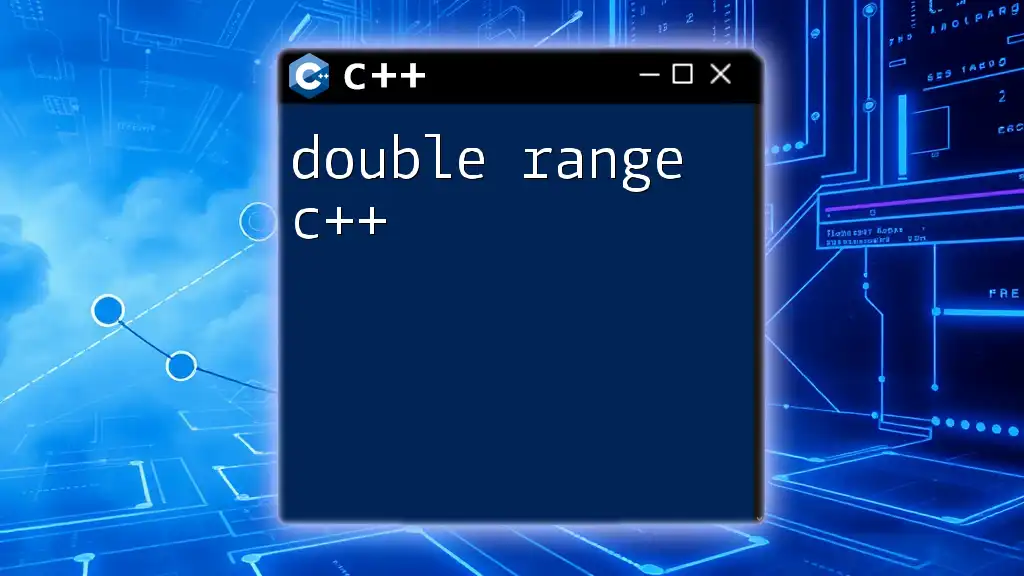
How to Use Double Ampersand in C++
Syntax of the Double Ampersand
The syntax for using the double ampersand in C++ is straightforward. Here’s the basic way to use it:
bool result = (condition1 && condition2);
In this expression, `result` will be true only if both `condition1` and `condition2` are true.
Examples of Using Double Ampersand
Simple Condition Example
Consider the following code snippet:
bool a = true;
bool b = false;
bool result = a && b; // result: false
In this example, since one of the conditions (`b`) is false, `result` evaluates to false. This illustrates a key aspect of the double ampersand: it requires both conditions to be true to return a true value.
Complex Condition Example
Let’s delve into a more complex scenario with multiple conditions:
int x = 5;
int y = 10;
if (x < 10 && y > 5) {
cout << "Both conditions are true!";
}
In this conditional statement, both `x < 10` and `y > 5` must be true for the message to be printed. This demonstrates how the double ampersand can bind multiple expressions together for a clear and concise decision-making process.
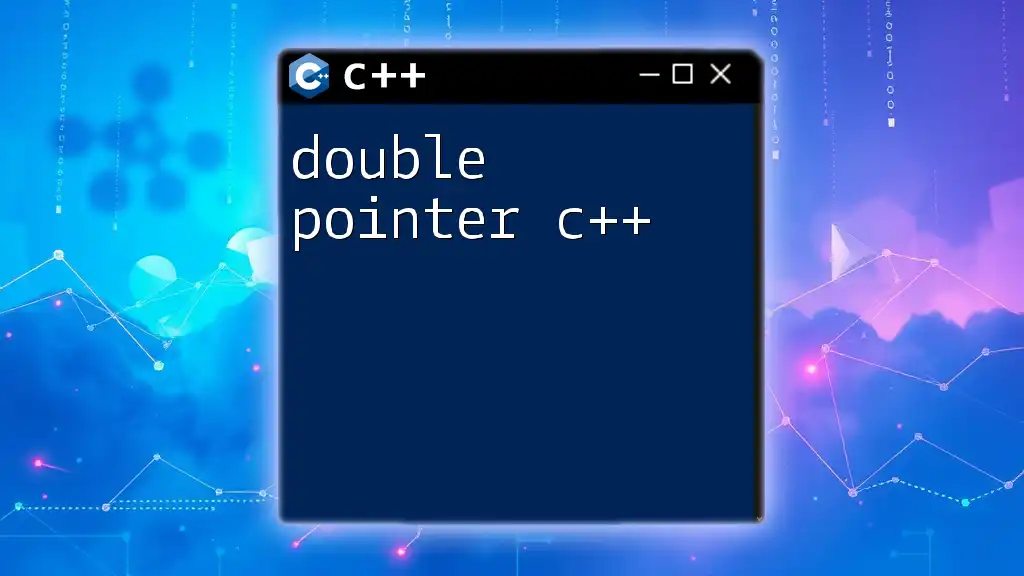
Short-Circuit Evaluation
What is Short-Circuit Evaluation?
Short-circuit evaluation is a unique feature of the double ampersand operator. This means that if the first condition evaluates to false, C++ will not evaluate the second condition. This is beneficial for improving efficiency and can prevent unnecessary computations or even runtime errors that might occur if the second condition is dependent on the first.
Examples of Short-Circuiting with Double Ampersand
Consider a function that checks for a valid age:
bool isValid(int age) {
return (age > 0 && age < 150);
}
In this example, if `age` is less than or equal to zero, the second condition `age < 150` will not be evaluated. Hence, short-circuiting averts unnecessary checks, enhancing performance and ensuring that only relevant conditions are assessed.
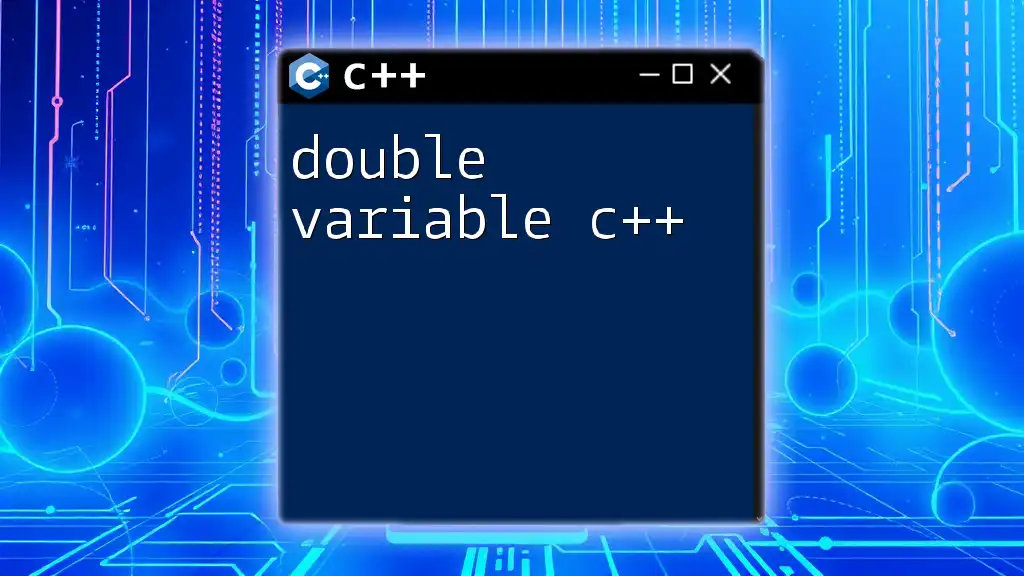
Common Pitfalls and Best Practices
Common Mistakes with Double Ampersand
When working with the double ampersand, it’s easy to encounter some common pitfalls:
-
Neglecting Parentheses: In more complex expressions, omitting parentheses can lead to unexpected results. For instance, consider the expression `a && b || c`. Without proper parentheses, the order of evaluation might not lead to the intended outcome.
-
Misunderstanding Evaluation Order: It's vital to understand how C++ evaluates conditions; left to right, honoring the operator precedence.
Best Practices
To avoid mistakes, adhere to the following best practices:
-
Always Use Parentheses: When dealing with complex logical expressions, enclosing conditions in parentheses ensures clarity and correctness.
-
Understand Short-Circuiting: Recognizing the implications of short-circuit behavior can protect your code from executing unnecessary operations and improve performance.
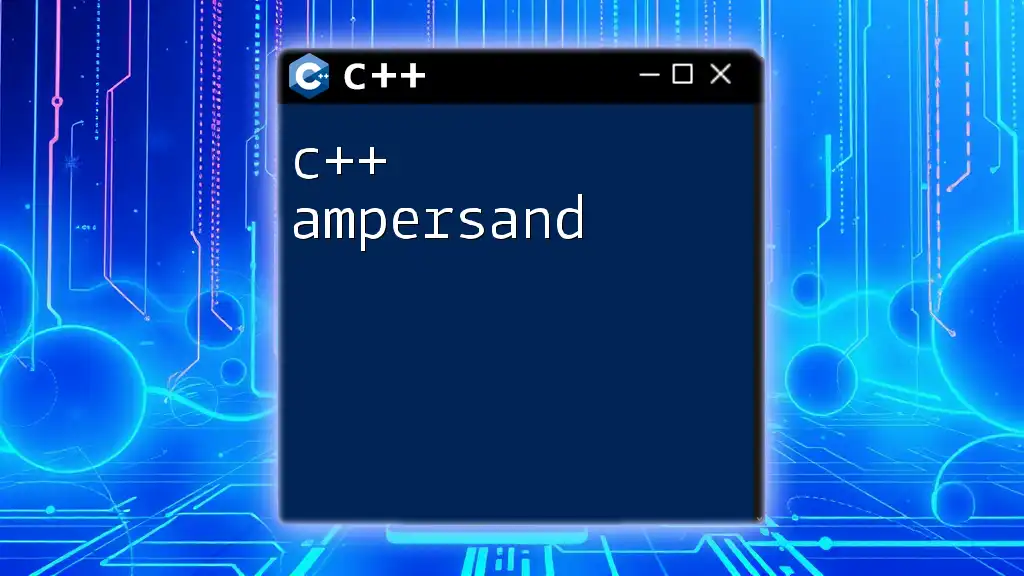
Conclusion
The double ampersand in C++ is a vital component of logical operations. It allows programmers to combine conditions effectively and succinctly, ensuring clear and efficient decision-making in their code. By practicing the use of the double ampersand, understanding its behavior, and adhering to best practices, you can harness its full potential in your C++ programming endeavors.
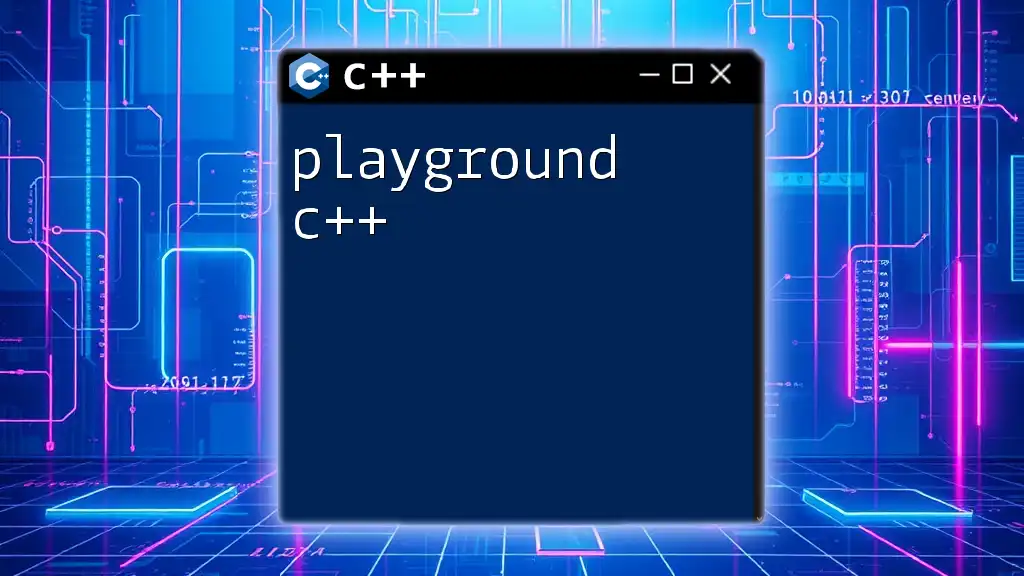
Additional Resources
Exploring resources such as textbooks, online courses, and official C++ documentation can significantly enhance your learning journey. These materials provide deeper insights into not just the double ampersand but also other critical aspects of C++ programming.
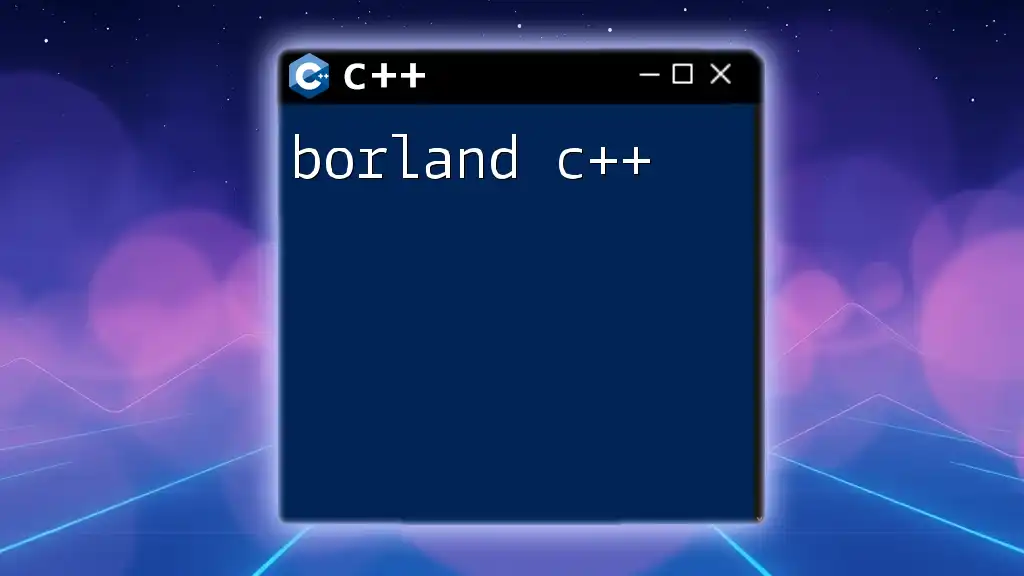
FAQs
What is the difference between `&&` and `&`?
The main difference lies in their operation: `&&` performs short-circuiting and is used for logical evaluation, while `&` performs a bitwise AND operation without short-circuiting, evaluating both operands irrespective of their truthiness.
Can I combine multiple `&&` operators?
Yes, you can combine multiple `&&` operators to create complex logical conditions. Just remember to group them using parentheses for clarity.
How does short-circuiting affect my program's performance?
Short-circuiting can enhance performance by preventing unnecessary evaluations. In scenarios where certain conditions can lead to costly computations or can cause errors, short-circuiting ensures that only the necessary evaluations are made, streamlining execution.