In C++, a 128-bit integer can be represented using libraries such as `__int128` in GCC or Clang, allowing for operations on very large integers that exceed the standard 64-bit limit.
#include <iostream>
int main() {
__int128_t largeInt = (__int128_t(1) << 120) - 1; // Example of a 128-bit integer
std::cout << "Large 128-bit integer: " << (largeInt >> 64) << " " << (largeInt & 0xFFFFFFFFFFFFFFFF) << std::endl; // Print high and low parts
return 0;
}
What is a 128-Bit Integer?
A 128-bit integer is a data type capable of representing integers that require a larger bit-width than standard C++ integer types. Compared to traditional types such as 8-bit, 16-bit, 32-bit, and 64-bit integers, a 128-bit integer can handle vastly larger numbers, making it essential in applications that involve high precision and large numerical computations.
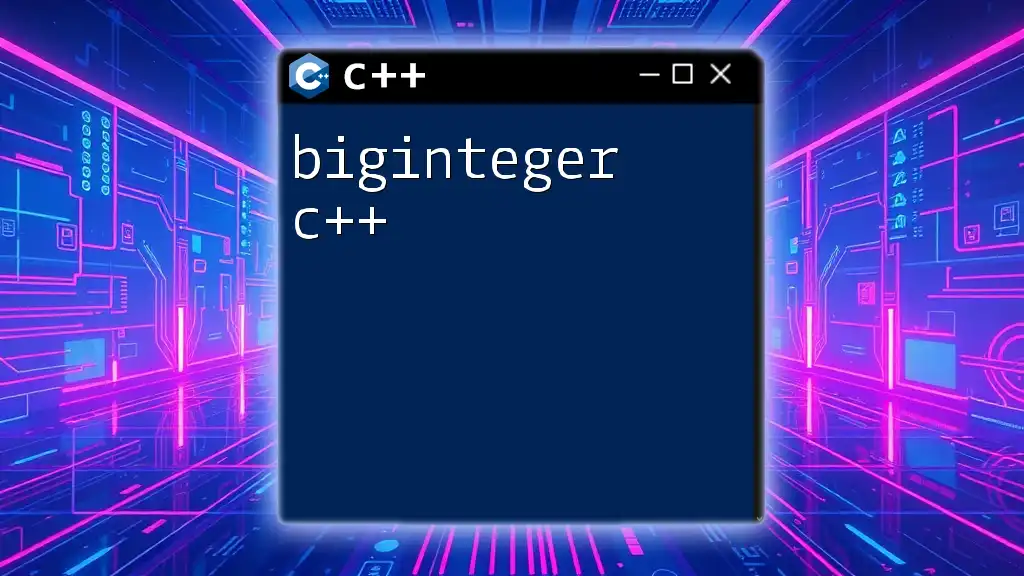
Why Use 128-Bit Integers?
Using a 128-bit integer allows developers to mitigate issues of overflow and underflow associated with smaller data types. These integers are particularly beneficial in situations where vast ranges of numerical values are necessary—such as in cryptography, scientific calculations, and large-scale arithmetic operations. In essence, they offer enhanced reliability for applications that demand accuracy across extensive ranges of values.
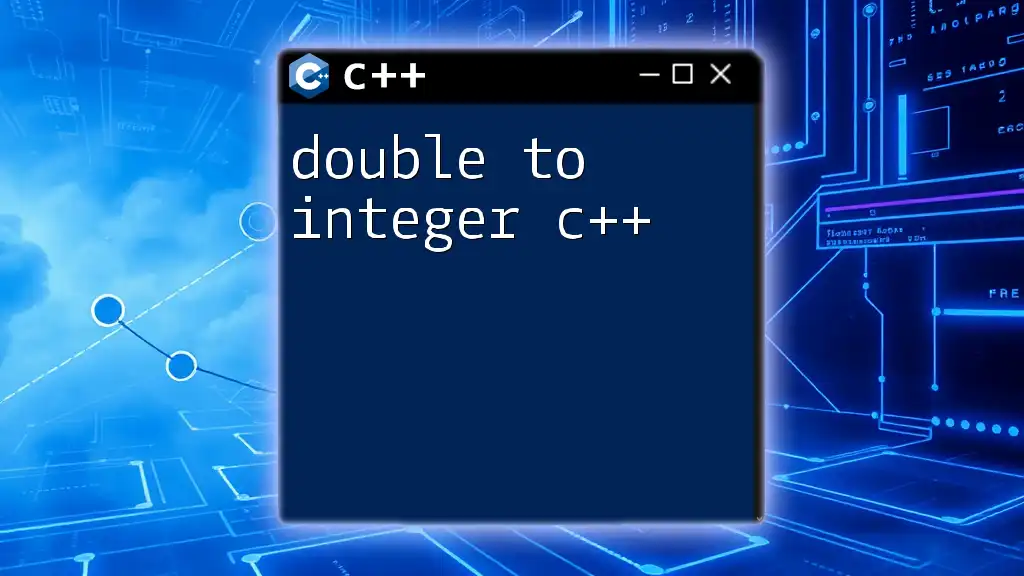
The Basics of Data Types in C++
C++ provides several standard integral data types, which can be summarized as follows:
- char: Typically 1 byte.
- short: Usually 2 bytes.
- int: Generally 4 bytes.
- long: Often 4 or 8 bytes depending on the implementation.
- long long: At least 8 bytes.
Limitations of Standard Integer Types
Each standard type has limitations, particularly when dealing with significant computations. For instance, a 64-bit integer can only represent values up to approximately 18 quintillion. When you exceed this limit, it can lead to overflow, resulting in unpredictable behavior or errors.
For example, attempting to store a value like `12345678901234567890` in a long long variable would cause an overflow, as it exceeds the maximum limit of a 64-bit integer.
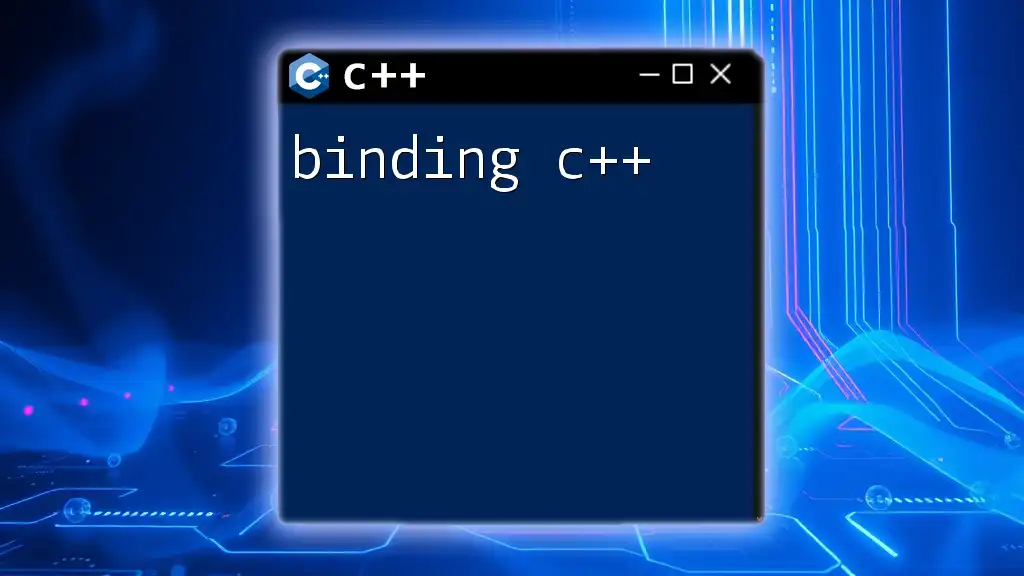
What is `__int128`?
The `__int128` data type is a compiler-provided extension (supported by GCC and Clang) that allows for the representation of 128-bit signed integers. This type is platform-dependent and may not be available in all environments. It is worth noting, however, that its usage can drastically reduce the risk of overflow in calculations that involve large numbers.
Using `__int128` in Code
Declaration and Initialization
To utilize the `__int128` type in your C++ programs, you can easily declare it like any other data type. For example:
__int128_t bigNum = 123456789012345678901234567890;
This snippet shows how a large numerical value can be stored in a `__int128` variable, far exceeding the limits of traditional integer types.
Common Operations
Operations on `__int128` follow standard arithmetic rules. Here’s how you can perform basic arithmetic:
__int128_t a = 10;
__int128_t b = 20;
__int128_t sum = a + b; // 30
The same syntax applies for subtraction, multiplication, and division, allowing for seamless integration into existing codebases.
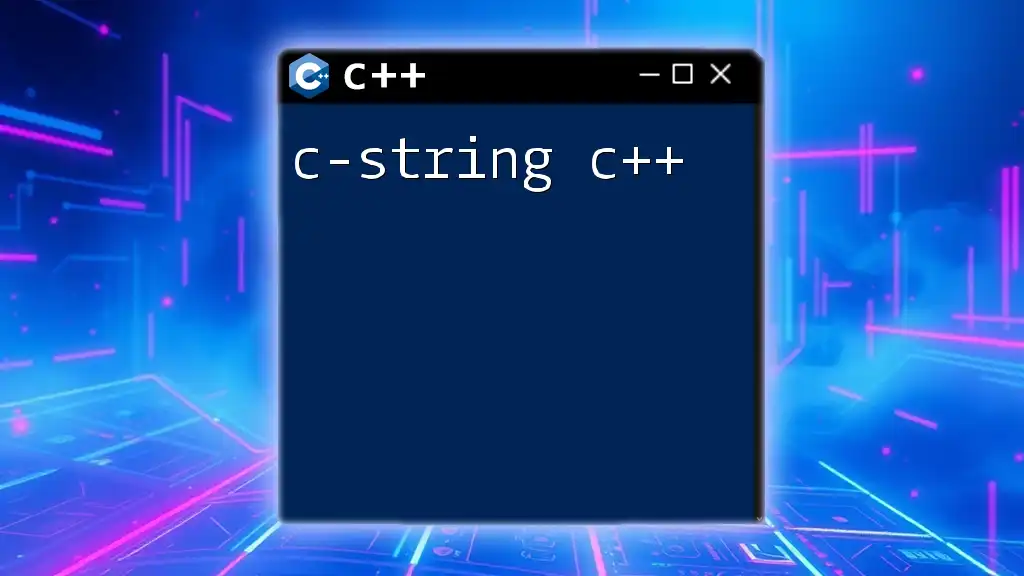
Converting `__int128` to and from Other Types
Converting From `__int128` to Other Integer Types
When converting a `__int128` to a smaller data type, be cautious of potential data loss. You can safely cast it using `static_cast`:
long long smallNum = static_cast<long long>(bigNum);
Converting to String for Output
Notably, printing `__int128` values directly is not supported. You can implement a conversion function to convert a `__int128` to a string:
std::string to_string(__int128_t num) {
std::string result;
// Implement conversion logic here
return result;
}
This function enables the conversion of large integers into a format suitable for output, enhancing usability.
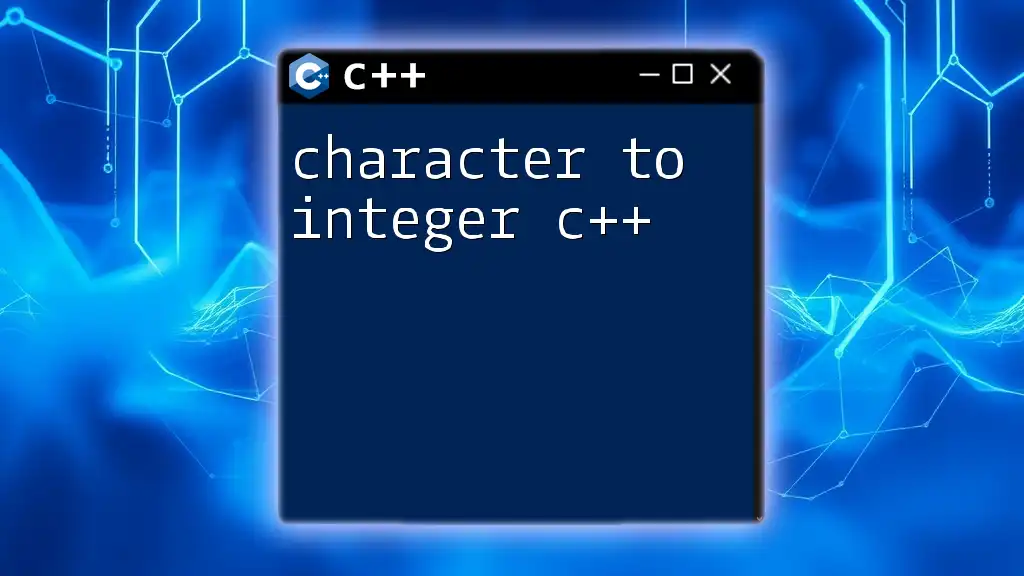
Libraries Supporting 128-Bit Integers
Boost Multiprecision Library
The Boost Multiprecision Library offers a robust alternative for handling large integers. It introduces the `cpp_int` type, capable of representing integers of arbitrary size. Here’s a simple initializer for `cpp_int`:
#include <boost/multiprecision/cpp_int.hpp>
using namespace boost::multiprecision;
cpp_int bigNum = cpp_int("123456789012345678901234567890");
This code demonstrates how easy it is to initialize and use large integers with Boost. The library also provides a wide range of mathematical operations implemented efficiently.
GNU Multiple Precision Arithmetic Library (GMP)
The GNU Multiple Precision Arithmetic Library (GMP) is another powerful library for numerical computations. It facilitates high-precision arithmetic and efficiently manages large integer sizes. You can use GMP to operate on 128-bit integers and beyond, depending on your needs.
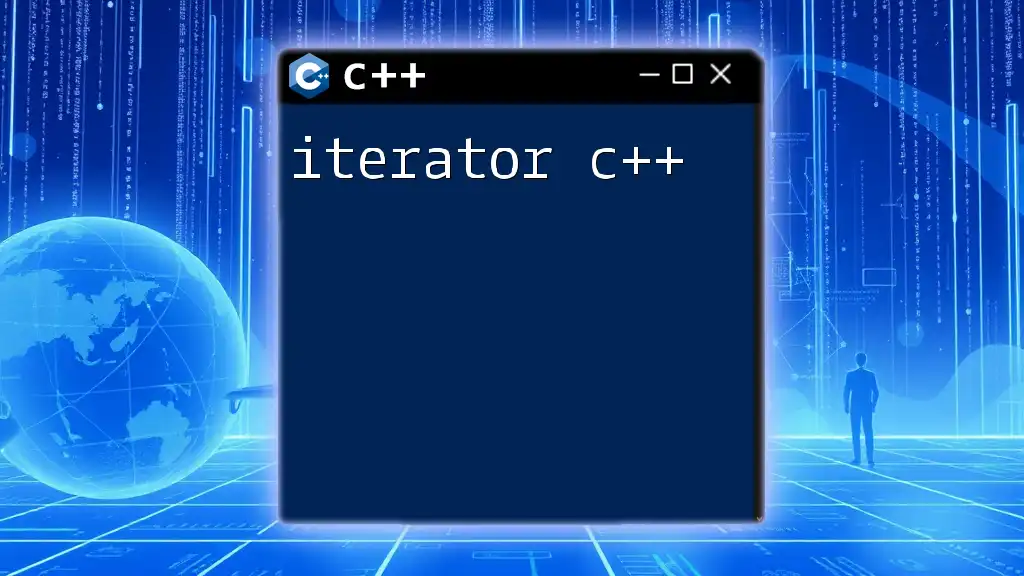
Performance Considerations
While working with 128-bit integers offers precision, it’s essential to understand the performance implications. Using larger integers can arise from the trade-off between computation speed and numerical range. Operations on 128-bit integers may be slower than their 64-bit counterparts due to increased memory use and computational overhead.
Benchmarking Different Integer Types
When considering performance, it's advisable to benchmark different integer types for specific applications. Performance might vary dramatically based on the operation types and the specific libraries used.
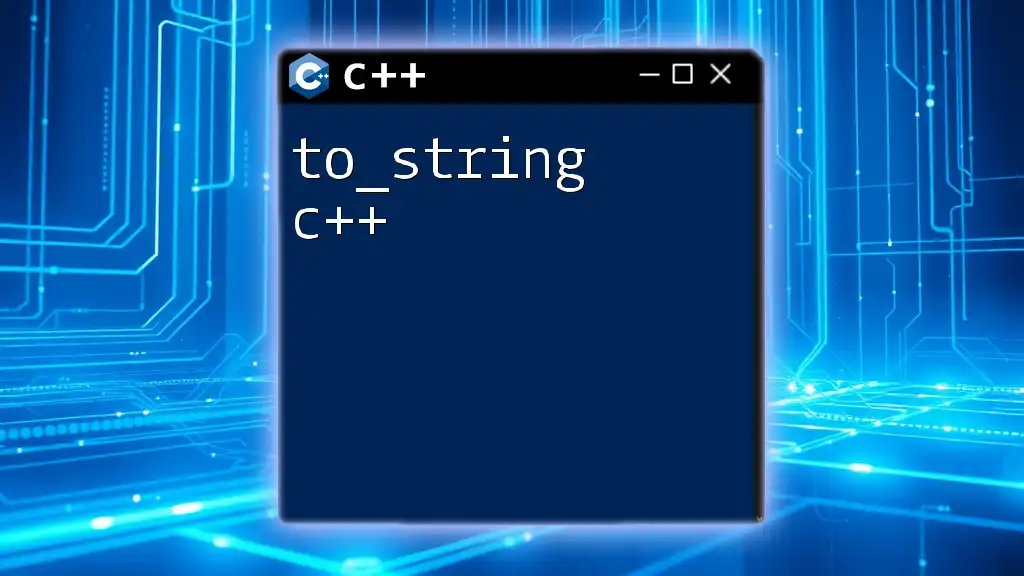
Use Cases for 128-Bit Integers in C++
Cryptography Applications
In cryptography, having the ability to manage large integers is crucial, as many cryptographic algorithms rely on large prime numbers and modular arithmetic with high precision. Utilizing 128-bit integers ensures the integrity of cryptographic processes, protecting data against attacks.
Scientific Computing
Scientific calculations often require precision when dealing with enormous numbers, especially in fields like astrophysics or large-scale simulations. 128-bit integers provide the capability to handle these computations without the risk of overflow.
Financial Calculations
Accurate calculations are paramount in financial applications. 128-bit integers offer the necessary precision to manage large amounts, ensuring that calculations involving large transactions or interest figures remain accurate.
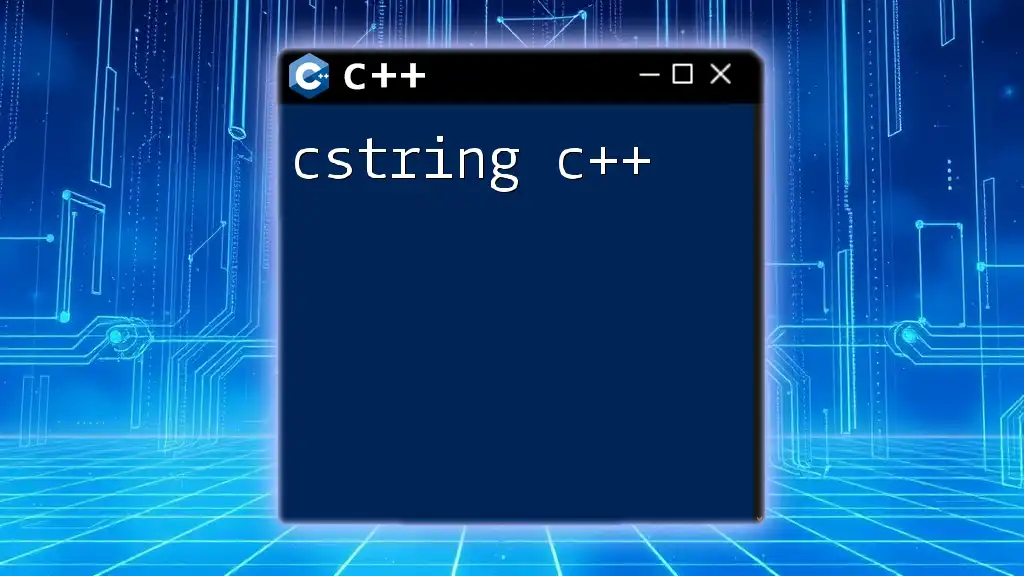
Conclusion
In conclusion, 128-bit integers in C++—specifically through the use of `__int128_t` or libraries like Boost or GMP—greatly enhance the ability to perform big integer arithmetic with high precision. These integers mitigate the risk of overflow common with traditional integer types and open the door to a world of advanced computations in various domains. Experimenting with 128-bit integers is highly encouraged for anyone seeking to expand their programming toolkit.
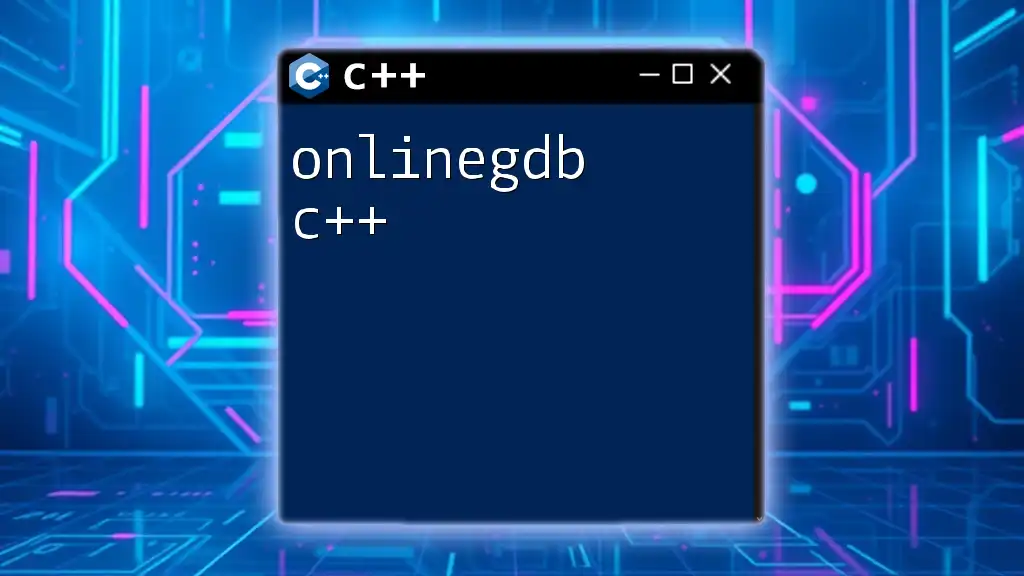
Additional Resources
For those who wish to continue exploring this topic, I recommend delving into the documentation of the Boost Multiprecision and GMP libraries, alongside further reading on integer arithmetic in C++. These resources provide in-depth insights that will bolster your understanding of 128-bit integer C++ and its wide-ranging applications.