In C++, you can convert a character to an integer by simply using type casting, as characters are represented by their ASCII values.
Here's a code snippet demonstrating this:
#include <iostream>
int main() {
char ch = 'A'; // Character
int intValue = static_cast<int>(ch); // Convert to integer
std::cout << "The integer value of '" << ch << "' is: " << intValue << std::endl; // Output: 65
return 0;
}
Understanding Characters and Integers in C++
Definition of Characters and Integers
In C++, a character is defined as a single letter, digit, or symbol, represented by the `char` data type. Examples include letters such as 'A', digits like '5', or punctuation like '!'.
Integers, on the other hand, refer to whole numbers, which can be either positive, negative, or zero. C++ offers various integer types, such as `int`, `short`, `long`, and `unsigned int`. The versatility of these data types allows programmers to choose the appropriate size and range for their applications.
ASCII Values
To understand the conversion from character to integer in C++, it's crucial to comprehend ASCII (American Standard Code for Information Interchange). ASCII assigns a unique integer value to each character, significantly aiding in the conversion process.
For instance, the uppercase letter 'A' corresponds to an ASCII value of 65, 'B' corresponds to 66, and so forth. Below is a simple representation of a few common characters and their ASCII values:
- 'A' → 65
- 'B' → 66
- 'C' → 67
- '0' → 48
- '1' → 49

Converting a Character to an Integer
Implicit Conversion
C++ allows for implicit conversion between characters and integers. When performing an operation with a character and an integer, the character can seamlessly convert to its corresponding ASCII value.
For example:
char ch = 'A'; // character
int intValue = ch; // implicit conversion
std::cout << intValue; // Outputs: 65
In this code snippet, the character 'A' is automatically converted to its ASCII value, 65, which is then stored in the integer variable `intValue`.
Explicit Conversion
Using Type Casting
In situations where you want to explicitly specify an integer conversion, type casting is an effective method. Type casting allows you to control how data is transformed from one type to another, ensuring clarity in your code.
For example:
char ch = 'B'; // character
int intValue = static_cast<int>(ch); // explicit conversion
std::cout << intValue; // Outputs: 66
Here, `static_cast<int>(ch)` clearly indicates that you want to convert `ch` to an integer type, resulting in the integer value 66.
Using ASCII Functions
Another method for conversion is using built-in functions to convert characters to their corresponding integer values. You can simply use the `int` keyword for conversion.
For example:
char ch = 'C';
int intValue = int(ch); // Another explicit conversion method
std::cout << intValue; // Outputs: 67
This demonstrates a straightforward and effective approach to convert a character to an integer in C++.

Converting Strings of Characters to Integers
Using `std::stoi()` Function
When working with strings that represent numerical values, C++ provides the `std::stoi()` function from the string library, which converts a string to an integer. This is particularly useful when parsing user input.
For instance:
std::string str = "123";
int intValue = std::stoi(str);
std::cout << intValue; // Outputs: 123
In this code, the string "123" is successfully converted to the integer 123 using `std::stoi()`.
Handling Invalid Inputs
When converting strings to integers, it’s essential to handle potential errors. Error handling is crucial to ensure that your program manages any unexpected input gracefully. The `std::stoi()` function can throw exceptions if the input string is invalid.
Consider the following code:
try {
std::string invalidStr = "abc";
int intValue = std::stoi(invalidStr); // Throws std::invalid_argument
} catch (const std::invalid_argument& e) {
std::cout << "Invalid input for conversion!"; // Error message
}
In this example, when an attempt is made to convert the string "abc", an exception is thrown, which is caught and handled, thus preventing the program from crashing.
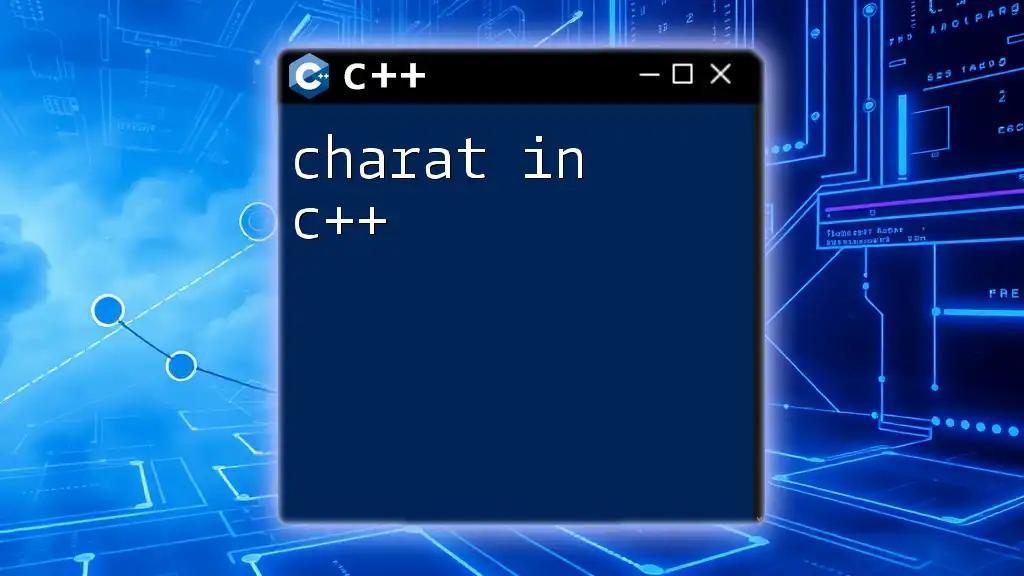
Common Use Cases for Character to Integer Conversions
User Input Handling
Converting characters to integers is frequently utilized for user input handling. When a user inputs numerical data via text, you often receive it in character format. Converting this data to integer format allows for arithmetic operations or validations.
For instance, parsing a user’s age would involve reading the input as a character and converting it to an integer for further computation.
Data Processing
In data processing applications, character to integer conversions play a vital role. You may find algorithms requiring such conversions for sorting, filtering, or aggregating data.
Whether it's processing user inputs in a competitive programming contest or analyzing large datasets, understanding how to handle character to integer conversions efficiently can be a game-changer in achieving the desired results.

Best Practices for Converting Character to Integer
When working with character to integer conversions, it's essential to adopt best practices to enhance code clarity and reliability.
-
Choose the Right Conversion Method: Decide between implicit and explicit conversion based on the context. While implicit conversion is automatic and convenient, explicit conversion is safer and reduces potential misunderstandings in your code.
-
Input Validation: Always validate user input before attempting to convert to ensure your program can gracefully handle any unexpected situations. This includes using exception handling for string conversions.
By following these practices, you can write more robust and understandable code when performing character to integer conversions.
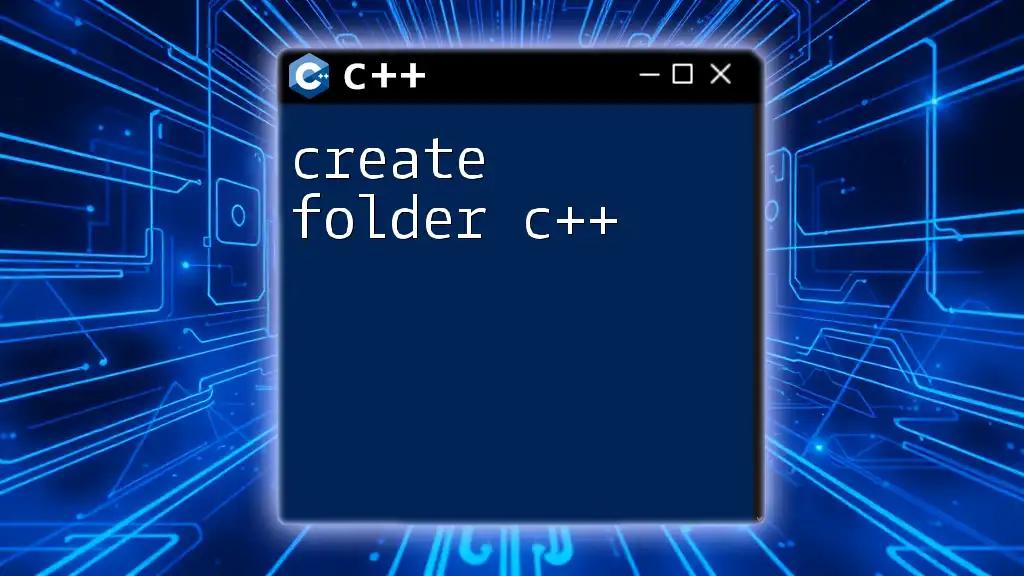
Conclusion
Understanding how to convert characters to integers in C++ is a fundamental skill for any aspiring programmer. Whether you are handling user inputs or processing data, mastering this capability can enhance your programming efficacy significantly. By applying the various methods and best practices discussed in this guide, you’ll be well-equipped to handle conversions seamlessly in your C++ projects.

Additional Resources
For further exploration of character to integer conversions and C++ programming, consider consulting the official C++ documentation and other related literature. Engaging with online courses or tutorials can also solidify your understanding and application of these concepts in real-world scenarios.