A character array in C++ is a sequence of characters stored in contiguous memory locations, typically used to handle strings efficiently.
#include <iostream>
int main() {
char greeting[] = "Hello, World!";
std::cout << greeting << std::endl;
return 0;
}
Understanding Character Arrays
What is a Character Array?
A character array in C++ is a basic data structure that holds a sequence of characters. This makes it a fundamental aspect of string manipulation in C++. Unlike regular arrays, a character array is specifically designed to store characters, making it easier to handle text data. Understanding character arrays is essential for effective programming in C++, especially when dealing with legacy code or systems that utilize C-style strings.
Syntax of Character Arrays in C++
The syntax for declaring a character array in C++ is straightforward. You define it by specifying the type `char`, followed by the name of the array and its size in square brackets. For example:
char myArray[10];
In this case, `myArray` can hold up to 10 characters. One important point to note is the necessity of the null-terminator (`'\0'`). This special character signals the end of the character array. Thus, if you initialize a character array with a string, you must consider its length plus one for the null-terminator.
Types of Character Arrays in C++
Static Character Arrays
Static character arrays have a fixed size, determined at compile-time. Here's a simple example:
char staticArray[5] = {'H', 'e', 'l', 'l', 'o'};
In this case, `staticArray` holds 5 characters, but be mindful that unless you explicitly add a null-terminator, this won't be considered a valid C-style string.
Dynamic Character Arrays
Dynamic character arrays are created using the `new` keyword, allowing for greater flexibility at runtime. Here's how you can declare a dynamic character array:
char* dynamicArray = new char[5];
After employing a dynamic array, it’s crucial to release the allocated memory using `delete[]` when it is no longer needed to prevent memory leakage.
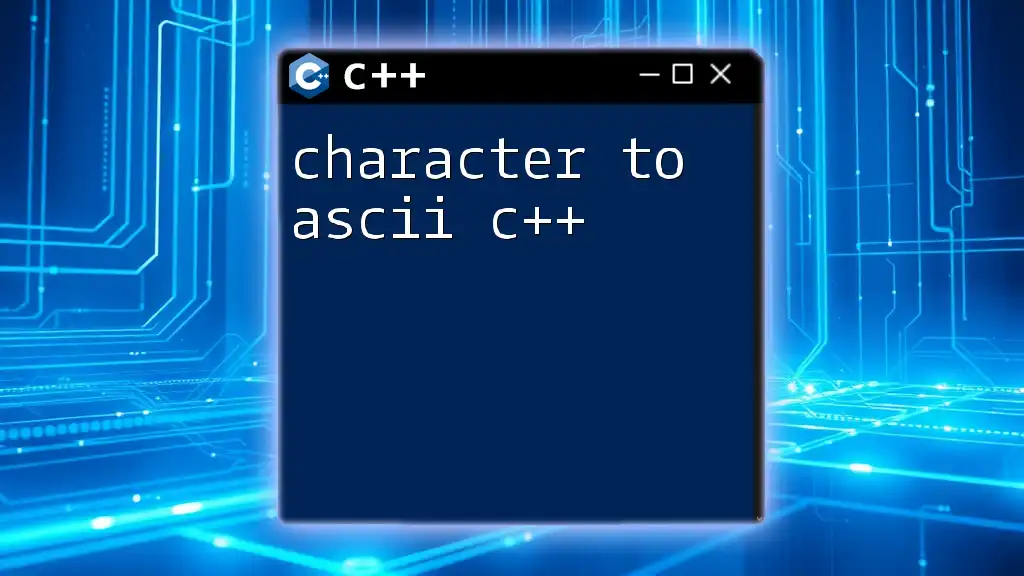
Working with Character Arrays
Initializing Character Arrays
Character arrays can be initialized in several ways. One common method is using an initializer list:
char welcomeMessage[] = "Welcome!";
In this example, `welcomeMessage` is automatically sized to 8, including the null-terminator.
Accessing Elements in Character Arrays
You can access individual elements in a character array using indexing, similar to other arrays. For example:
char sentence[] = "Hello";
char firstCharacter = sentence[0]; // 'H'
Keep in mind that array indices begin at zero!
Common Operations on Character Arrays
Finding Length of Character Array
To determine the length of a character array, you can use the `strlen()` function, which returns the number of characters excluding the null-terminator.
int length = strlen(welcomeMessage);
Here, `length` would store the value `7` for "Welcome!".
Copying Character Arrays
Copying one character array to another is smoothly handled using the `strcpy()` function.
char source[] = "Source";
char destination[10];
strcpy(destination, source);
After this operation, `destination` will hold the same value as `source`.
Concatenating Character Arrays
You can concatenate two character arrays using the `strcat()` function. For example:
char str1[20] = "Hello ";
char str2[] = "World!";
strcat(str1, str2);
This concatenation will result in `str1` holding "Hello World!".
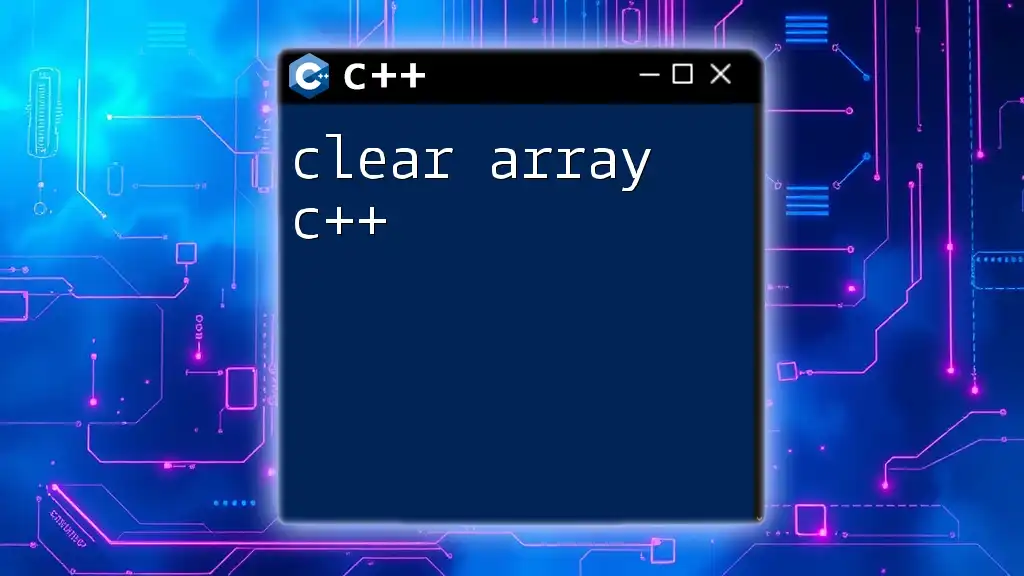
Advanced Character Array Techniques
Character Arrays as Strings
Understanding that character arrays are often used as C-style strings is crucial. These arrays are null-terminated; without the null character, functions expecting a C-style string may read unexpected memory locations, which can lead to undefined behavior.
Multi-dimensional Character Arrays
C++ allows for multi-dimensional character arrays. This is particularly useful for tasks like storing a list of strings.
char colors[3][10] = { "Red", "Green", "Blue" };
In this example, `colors` is a 2D array holding three colors. Each color can occupy up to 9 characters, plus the null-terminator.
Character Arrays and Functions
When passing character arrays to functions, use the syntax like so:
void printMessage(char message[]) {
cout << message << endl;
}
This function accepts a character array, and you can invoke it with:
printMessage(welcomeMessage);
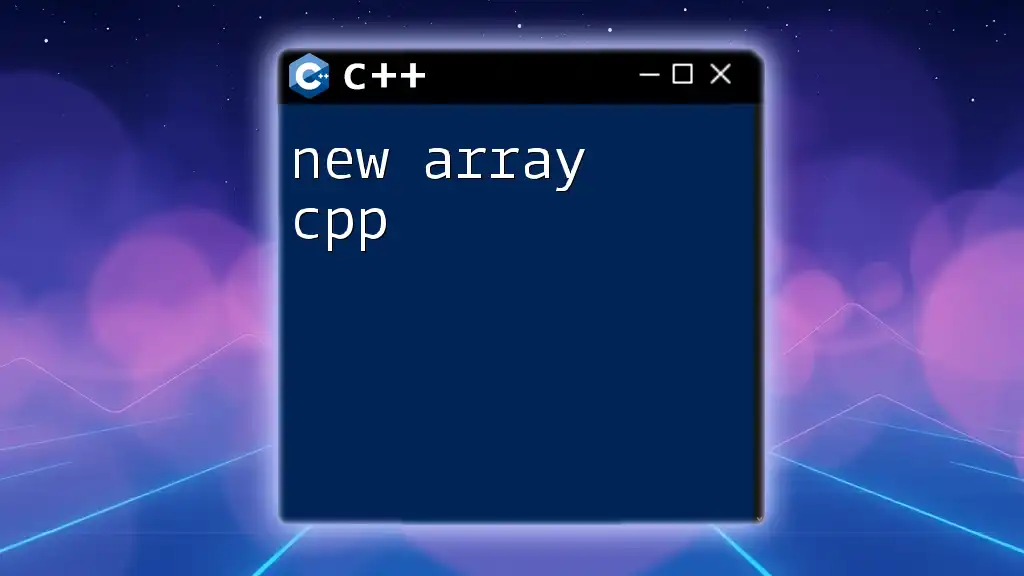
Common Mistakes with Character Arrays
Forgetting the Null-Terminator
A very common mistake with character arrays is neglecting to include the null-terminator. This oversight can result in unexpected behavior, as functions like `strcpy()` and `strlen()` will not know where the string ends.
Buffer Overflow Risks
Buffer overflows are a significant risk when using character arrays. If you write past the bounds of an array, it can corrupt the memory and potentially lead to security vulnerabilities or crashes. Always ensure that you allocate enough space and stay within the defined limits.
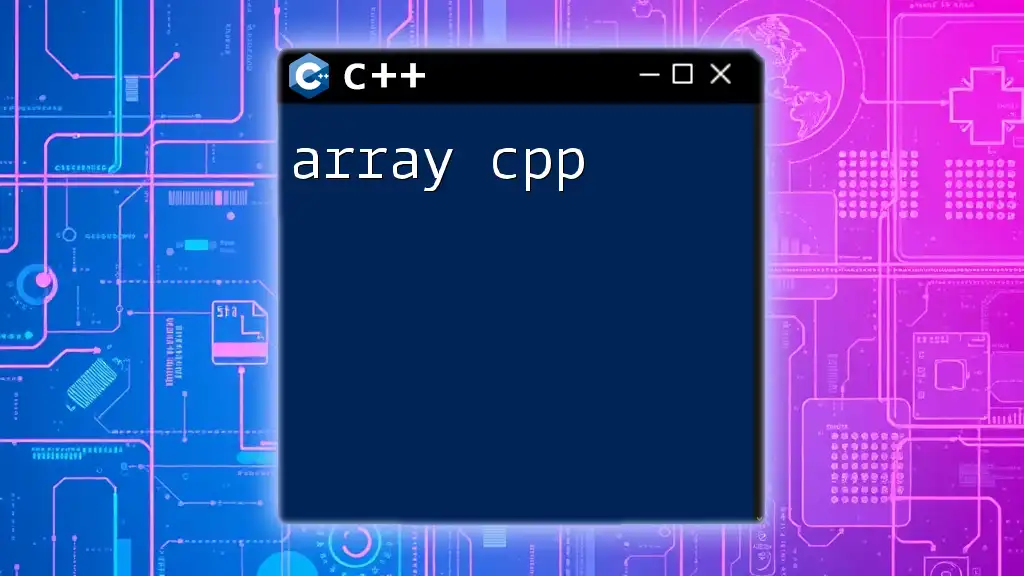
Conclusion
Mastering character arrays in CPP is a foundational skill in programming. By understanding their structure, implementation, and common operations, you can work effectively with text data in C++. Focus on avoiding typical pitfalls like forgetting null-terminators and managing memory correctly if using dynamic arrays.
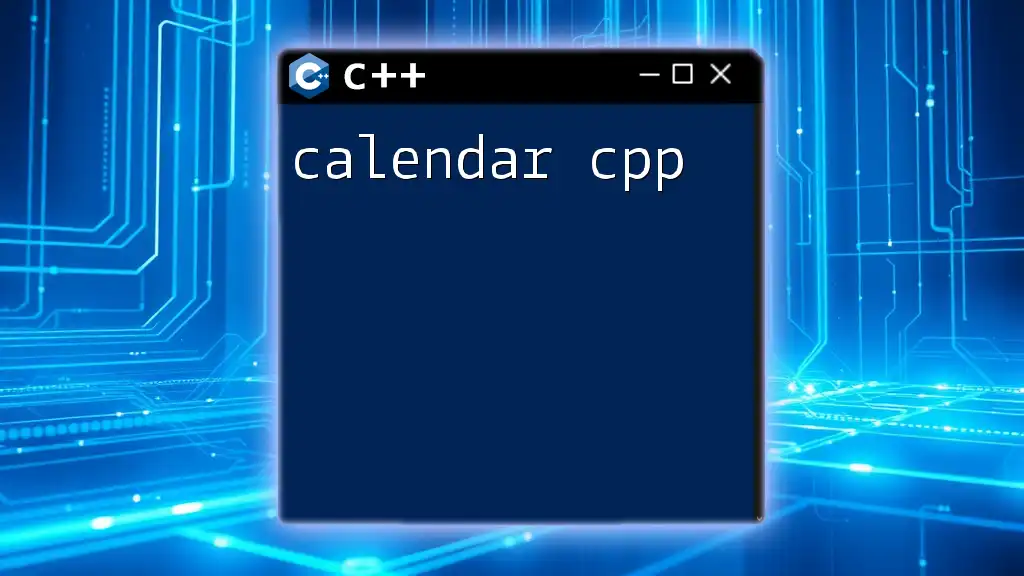
Additional Resources
For further learning about character arrays, consider books on C++ programming, online courses focusing on data structures, and forums or communities dedicated to C++ programming.
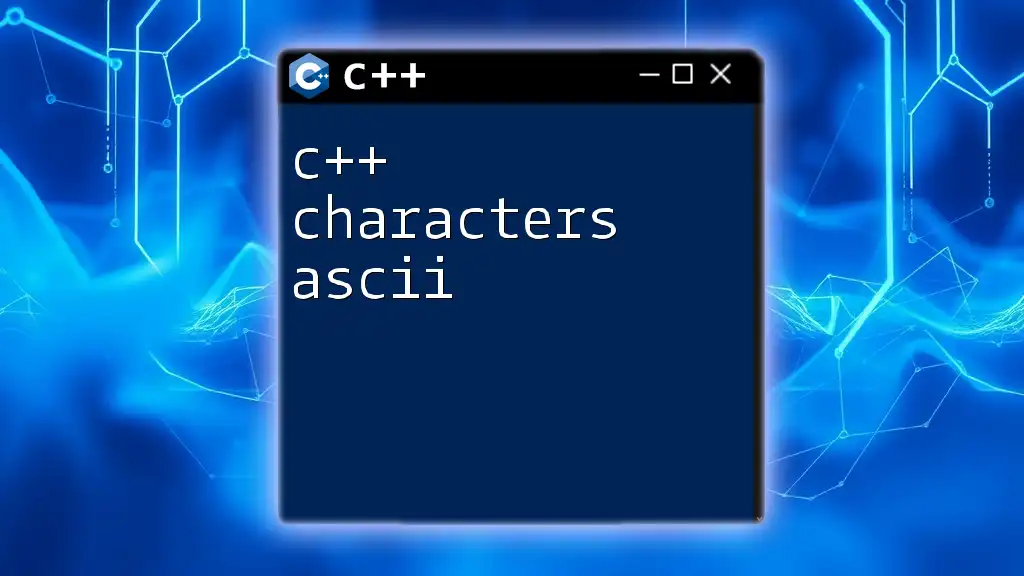
FAQs on Character Arrays in C++
While working with character arrays, you may have questions like, "What is the difference between char arrays and std::string?" or "How to convert a string to a character array?" Exploring these inquiries can deepen your understanding of strings and character manipulation in C++.