To clear an array in C++, you can set all of its elements to zero using a simple loop or the `std::fill` algorithm. Here's an example using a loop:
#include <iostream>
int main() {
const int size = 5;
int arr[size] = {1, 2, 3, 4, 5};
// Clearing the array
for(int i = 0; i < size; i++) {
arr[i] = 0;
}
// Output the cleared array
for(int i : arr) {
std::cout << i << " "; // Output will be: 0 0 0 0 0
}
return 0;
}
What is an Array in C++?
In C++, an array is a collection of elements that are stored in contiguous memory locations. Arrays can hold multiple values of the same type, which allows easy access and organization of data.
Types of Arrays
Arrays in C++ can be categorized into two main types: static arrays and dynamic arrays.
-
Static Arrays: These are arrays where the size is fixed at compile time. Once declared, the size cannot be altered during runtime. For example:
int arr[5]; // An array of five integers
-
Dynamic Arrays: These are created at runtime using pointers and can be resized. This type of array requires careful memory management. You can create dynamic arrays using the `new` keyword:
int* arr = new int[5]; // Dynamic array of five integers
Key Characteristics of Arrays in C++
- Size: The size of an array must be known at compile time for static arrays and can be determined at runtime for dynamic arrays.
- Type: All elements in an array must be of the same data type.
- Indexing: Arrays are zero-indexed, which means the first element is accessed with index 0.
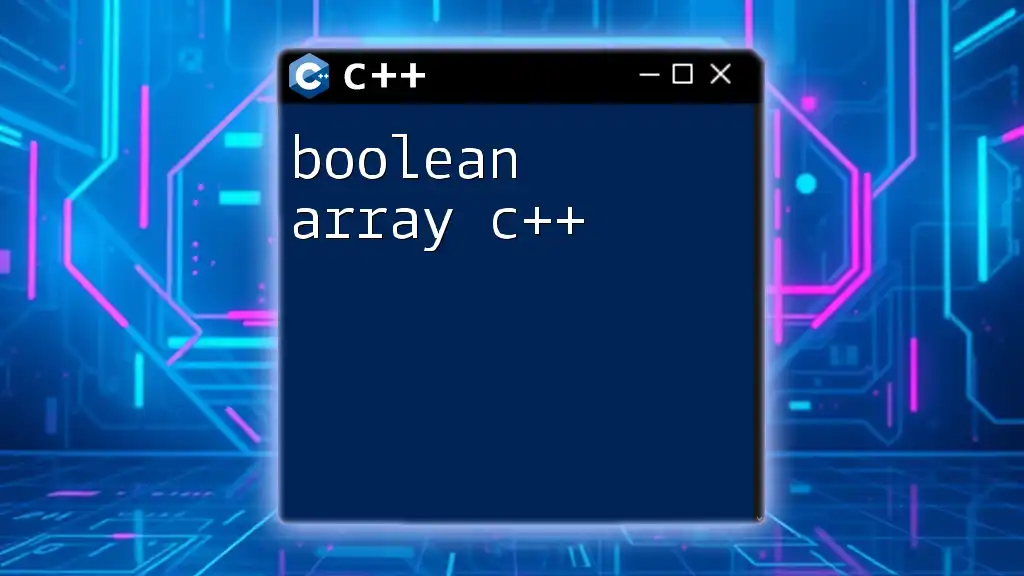
Understanding the Need to Clear an Array
Clearing an array in C++ is essential for effective memory management. Using uninitialized or stale data can lead to unpredictable behavior in a program. Here are some crucial reasons to clear an array:
- Memory Management: By clearing arrays, you release any resources allocated to them, thus preventing memory leaks.
- Data Integrity: Ensuring that old or irrelevant data doesn't interfere with new calculations or logic enhances the reliability of your programs.
Use Cases: Situations like game development, data processing, and real-time systems heavily rely on clearing arrays to ensure optimal performance and accurate results.
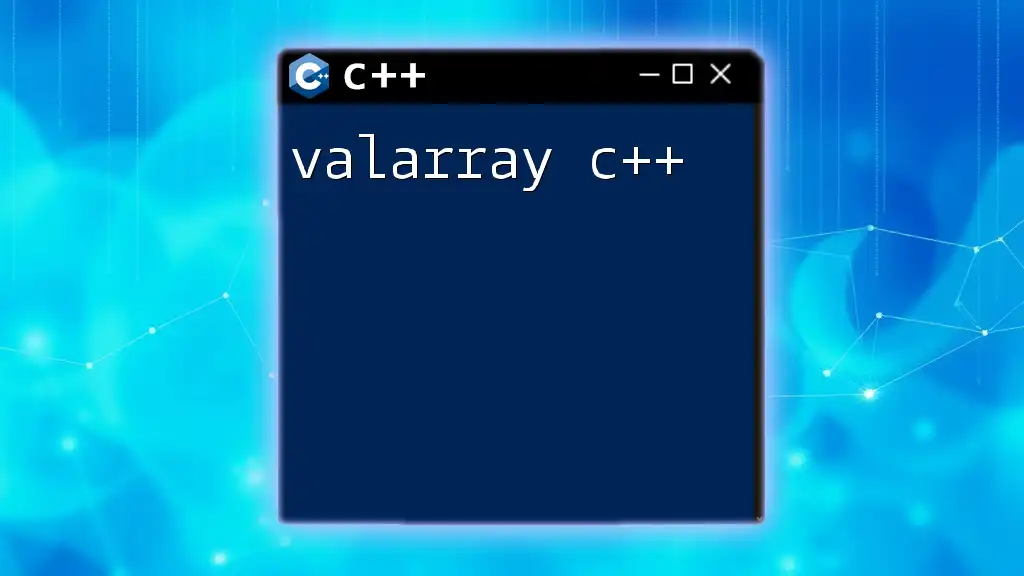
Methods to Clear an Array in C++
Clearing a Static Array
Static arrays are straightforward to manage since their size is known at compile time. To clear the contents of a static array, you can explicitly assign a value to each element (commonly 0). Here's how you can do it:
int arr[5] = {1, 2, 3, 4, 5};
for(int i = 0; i < 5; i++) {
arr[i] = 0; // Clearing array elements
}
This code iterates over each element in the array, setting its value to 0, effectively clearing the array.
Clearing a Dynamic Array
Dynamic arrays require careful memory management, particularly when it comes to clearing them. To clear a dynamic array, you should deallocate the memory allocated to it using the `delete[]` operator. Here's an example:
int* arr = new int[5]{1, 2, 3, 4, 5};
delete[] arr; // Deallocate memory
arr = nullptr; // Prevent dangling pointer
This code allocates memory for a dynamic array, then clears it using `delete[]`, which frees up the memory. It's good practice to set the pointer to `nullptr` to avoid dangling pointers that can lead to undefined behavior.
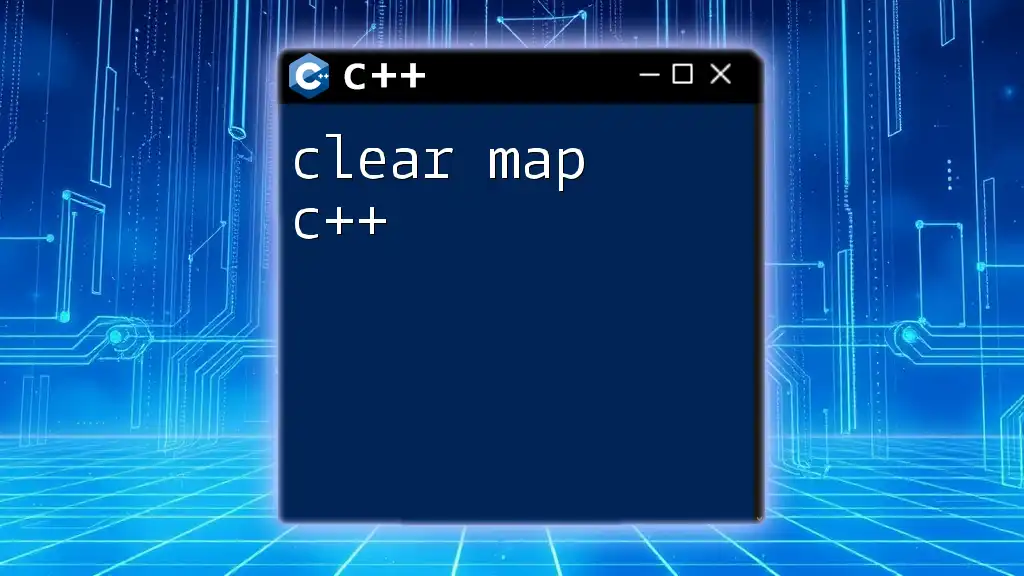
Best Practices for Clearing Arrays
Choosing the Right Method
Understanding when to use static versus dynamic arrays is crucial. Static arrays are faster and easier to use, but if you need flexibility in size during runtime, dynamic arrays are the better option.
Performance Considerations
When dealing with large datasets, consider the performance implications. Using simple loops or standard library functions might perform better depending on use cases. Avoid excessive memory allocation and deallocation which can degrade performance.
Using Standard Library Functions
The C++ Standard Library offers functions that simplify many tasks, including clearing arrays. One such function is `std::fill`, which can be used to set all elements to a specified value. This approach can be more efficient and easier to read:
#include <algorithm>
int arr[5] = {1, 2, 3, 4, 5};
std::fill(arr, arr + 5, 0); // Clearing using std::fill
This snippet uses `std::fill` to set all elements to 0, offering concise and clear syntax.
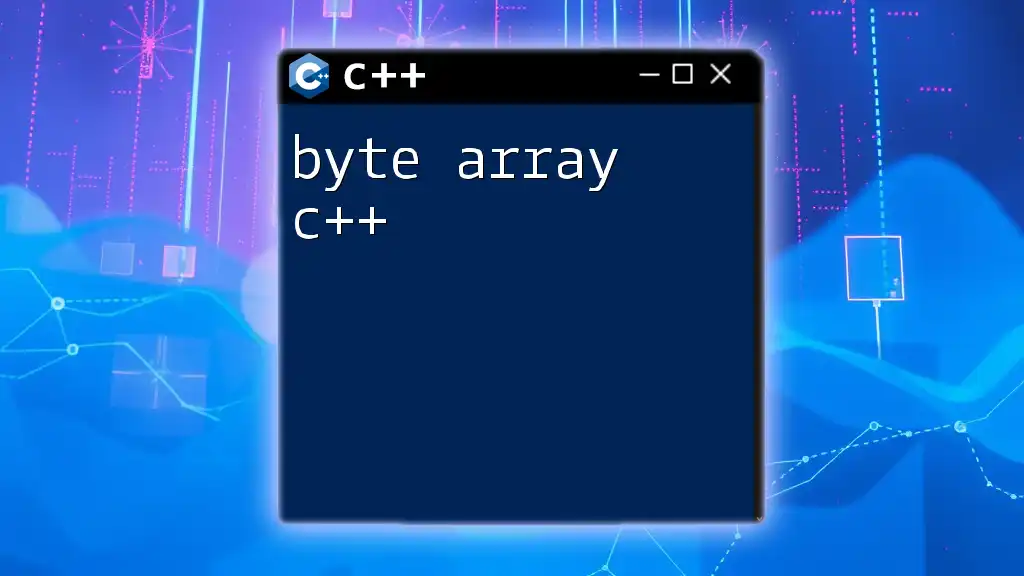
Common Mistakes When Clearing Arrays
Even seasoned programmers can make mistakes when managing arrays. Here are common pitfalls to avoid:
- Memory Leaks: Failing to deallocate dynamic arrays properly may lead to memory leaks, consuming resources unnecessarily.
- Using Uninitialized Data: If static arrays are not cleared, they may contain garbage values from previous computations.
Example Mistakes: Forgetting to delete a dynamically allocated array can lead to applications consuming more memory over time, resulting in reduced performance or crashes.
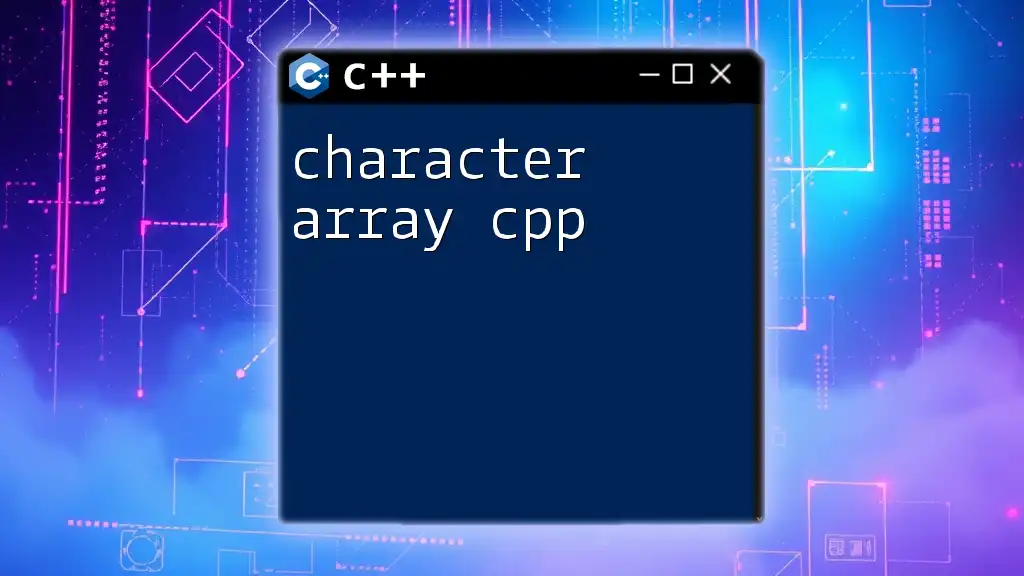
Conclusion
Clearing an array in C++ is a fundamental skill that enhances both performance and data integrity. Whether you are working with static or dynamic arrays, understanding how to effectively manage and clear them will significantly improve your programming efficacy. By following best practices and learning from common mistakes, you will be better positioned to write robust C++ applications.
For further learning, explore the intricacies of C++ arrays through additional resources, including books, tutorials, and video courses that cover arrays in detail. Keep practicing to streamline your array management skills and ensure your programs are efficient and reliable.