A bitarray in C++ is an efficient way to represent and manipulate a collection of bits, allowing operations like setting, clearing, and checking bit values.
Here’s a simple example of using a bitarray in C++:
#include <iostream>
#include <bitset>
int main() {
std::bitset<8> bits; // Create a bitarray of size 8
bits.set(1); // Set the bit at index 1
bits.set(4); // Set the bit at index 4
std::cout << "Bitarray: " << bits << std::endl; // Output: 00010010
return 0;
}
Definition of Bit Array
A bit array is a data structure that compactly stores bits—binary digits (0s and 1s). Unlike conventional arrays of integers or booleans, which can take up more memory for storage, a bit array uses just one bit for each entry, making it highly memory efficient.
Why Use Bit Arrays?
Using bit arrays can deliver several benefits:
- Memory Efficiency: Storing boolean values in a bit array significantly reduces the memory footprint when compared to using standard data types, as it only requires as much space as needed for the bits.
- Speed of Operations: Operations like setting or clearing bits can be performed in constant time, making bit arrays faster than using primitive data types that require more operations for similar tasks.
- Common Use Cases: Bit arrays are widely used in various fields such as algorithms, data compression, and communication protocols, where managing large datasets efficiently is important.
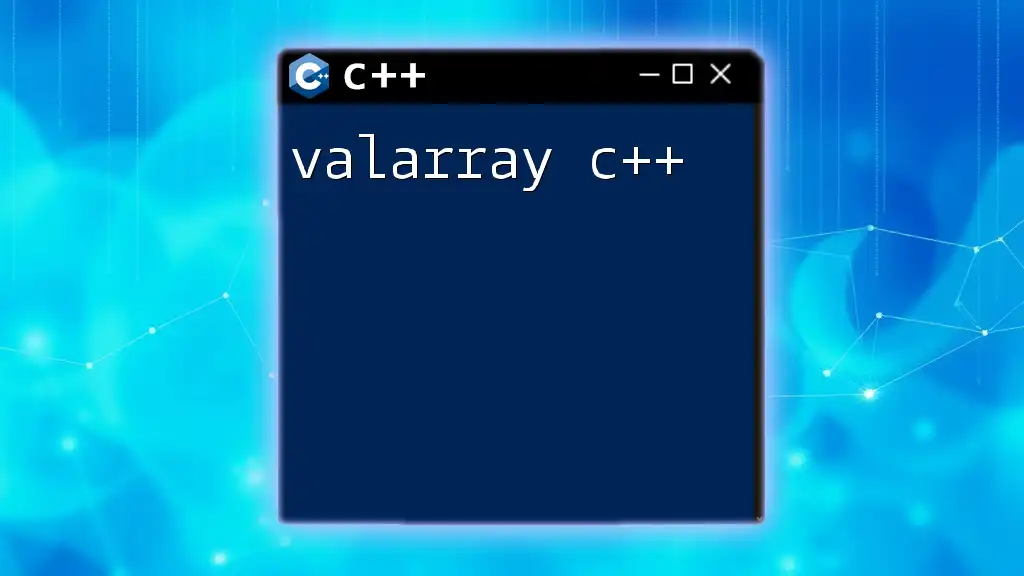
Basic Concepts of Bit Arrays
What are Bits?
A bit is the most basic unit of information in computing and can have a value of either 0 or 1. A collection of bits makes up a binary representation, which is fundamental to how computers process data.
Understanding Bit Manipulation
Bitwise operations are essential when working with bit arrays. These operations enable developers to manipulate individual bits effectively:
- AND (&): Useful in clearing bits.
- OR (|): Essential for setting bits.
- NOT (~): Flips the bits.
- XOR (^): Useful in toggling bits.
These operations can significantly increase both the performance and efficiency of algorithms that need to handle boolean values.
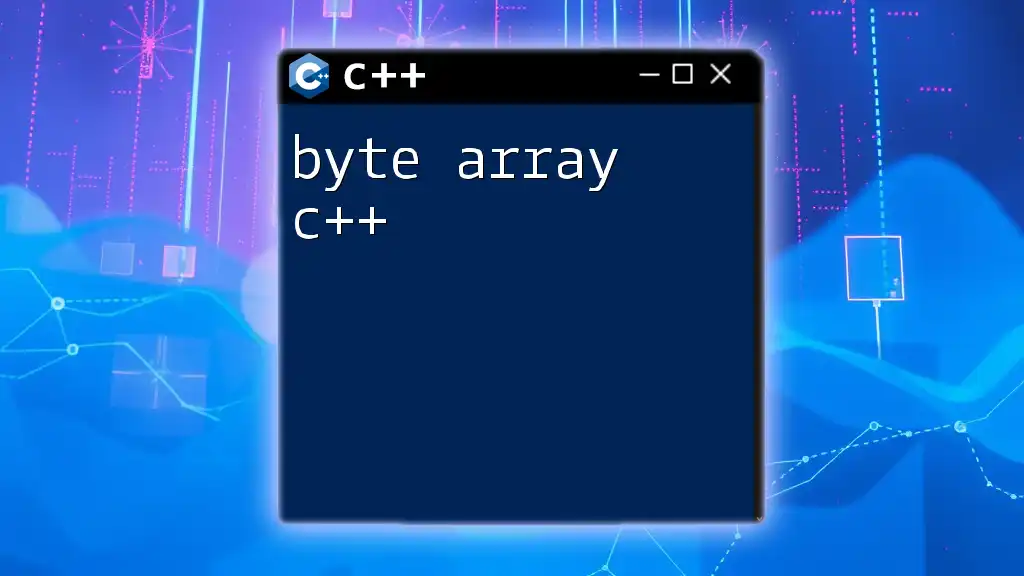
Implementing Bit Array in C++
Choosing the Right Data Structure
When implementing a bit array in C++, there are several options. One of the most straightforward is to use `std::vector<bool>`. This specialized vector compresses storage, enabling efficient management of bits.
Using `std::vector<bool>`
Though `std::vector<bool>` provides a compact form of boolean storage, there can be concerns regarding the performance and behavior of the underlying implementation. While it is easy to use, its behavior is sometimes counterintuitive, particularly when it comes to passing references to bits.
Custom Bit Array Class
Creating your own BitArray class can provide flexibility and optimized performance. Below is a simple implementation:
class BitArray {
private:
std::vector<bool> array;
size_t size;
public:
BitArray(size_t n) : size(n), array(n) {}
void set(size_t index) {
array[index] = true;
}
void clear(size_t index) {
array[index] = false;
}
bool get(size_t index) const {
return array[index];
}
size_t getSize() const {
return size;
}
};
This class encapsulates the bit array's behavior and provides methods for setting, clearing, and getting values.
Example of Bit Array Usage
Basic Operations
Here’s how a simple operation with the BitArray class might look:
BitArray bits(10);
bits.set(3); // Set the 3rd bit
bool value = bits.get(3); // returns true
In this example, we created a bit array of size 10 and set the 3rd bit. When we retrieve the value using the `get` method, we receive `true`, indicating that the bit has indeed been set.
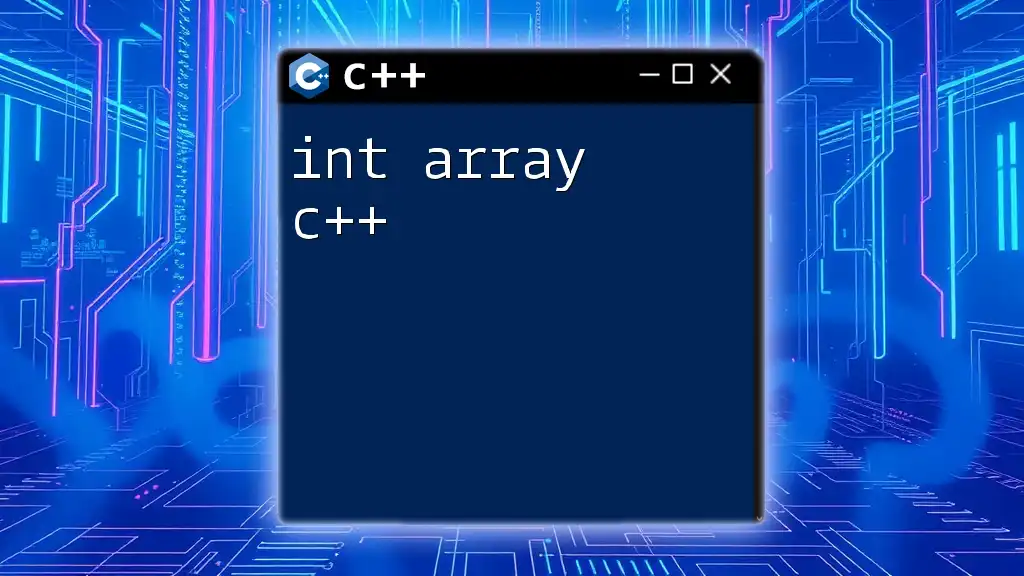
Advanced Bit Array Techniques
Compression Techniques
Bit packing involves storing bits more densely to save space. One common technique is to store multiple bits in a single byte or an integer. Here’s a simple illustration using bitwise operations to pack bits:
class PackedBitArray {
private:
std::vector<uint8_t> packedArray;
size_t size;
public:
PackedBitArray(size_t n) : size(n), packedArray((n + 7) / 8, 0) {}
void set(size_t index) {
packedArray[index / 8] |= (1 << (index % 8));
}
void clear(size_t index) {
packedArray[index / 8] &= ~(1 << (index % 8));
}
bool get(size_t index) const {
return (packedArray[index / 8] & (1 << (index % 8))) != 0;
}
};
In this example, each byte can hold 8 bits, demonstrating a more space-efficient way to manage a bit array.
Iterating Over a Bit Array
You can iterate through the bits in your class as such:
for(size_t i = 0; i < bits.getSize(); ++i) {
std::cout << bits.get(i) << " ";
}
This approach allows you to easily visualize or process each bit within the array, crucial for debugging or performing operations based on bit values.
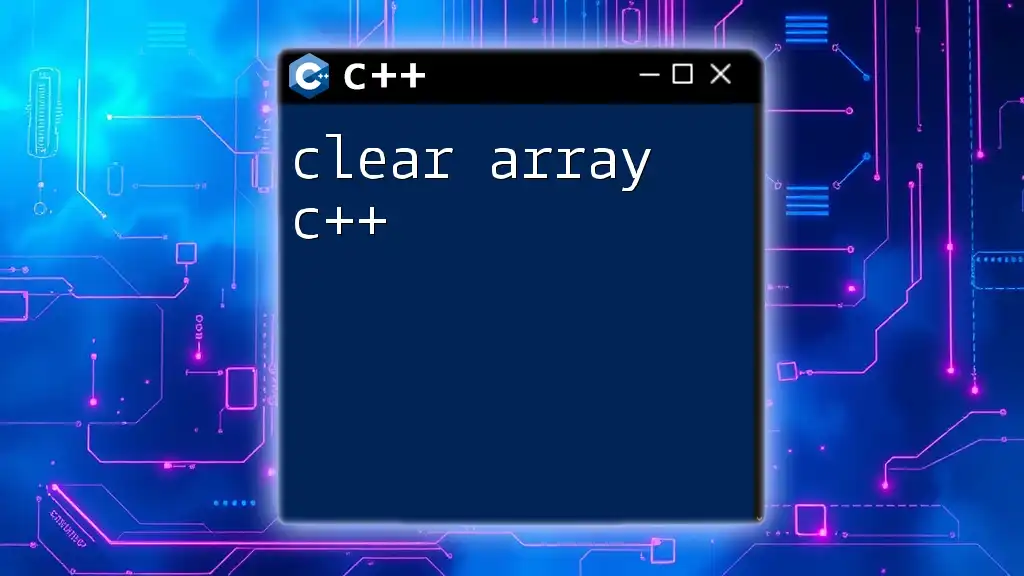
Performance Considerations
Time Complexity of Bit Operations
In the context of operations on a bit array, the time complexity for setting, clearing, and getting bits is O(1), meaning these operations run in constant time regardless of the size of the bit array. This efficiency is a significant advantage over other data structures that cannot guarantee such performance.
Memory Management and Optimization
For developers focused on efficiency, it’s important to consider how bits are stored. Using native `uint8_t` or other integer types for storage can save memory. Moreover, optimizing storage alongside bitwise manipulation can yield greater performance, especially for large datasets.
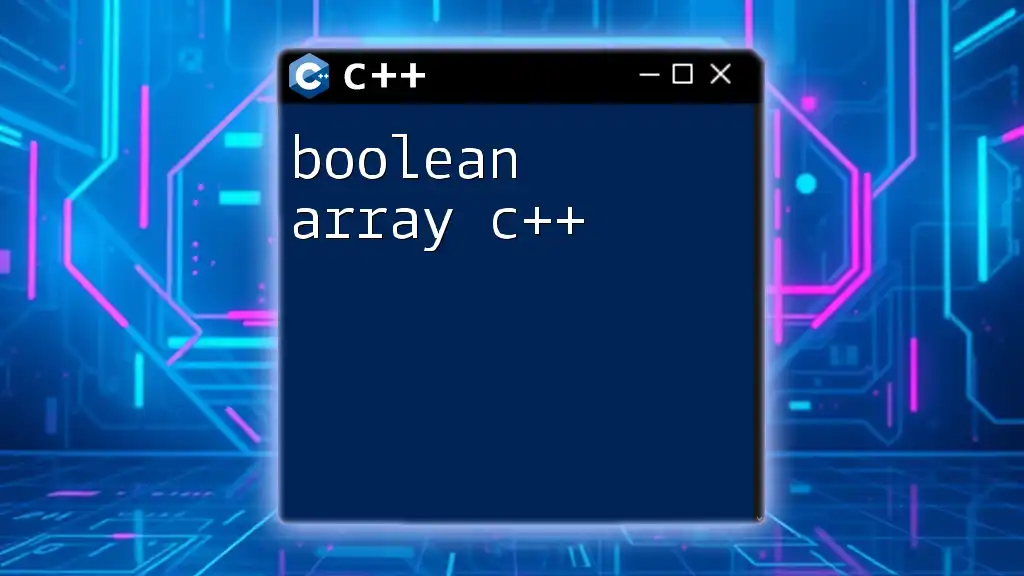
Real-world Applications of Bit Arrays
Use Cases in Computer Science
Bit arrays excel in several scenarios:
- Set Representation: They can represent large sets efficiently, where the presence or absence of each element corresponds to a bit.
- Network Operations: In protocols where flags need to be set for transmission, using bit arrays can reduce overhead significantly.
- Algorithms: They are widely used in search algorithms, such as bloom filters, where the existence of an item in a set is represented by bits.
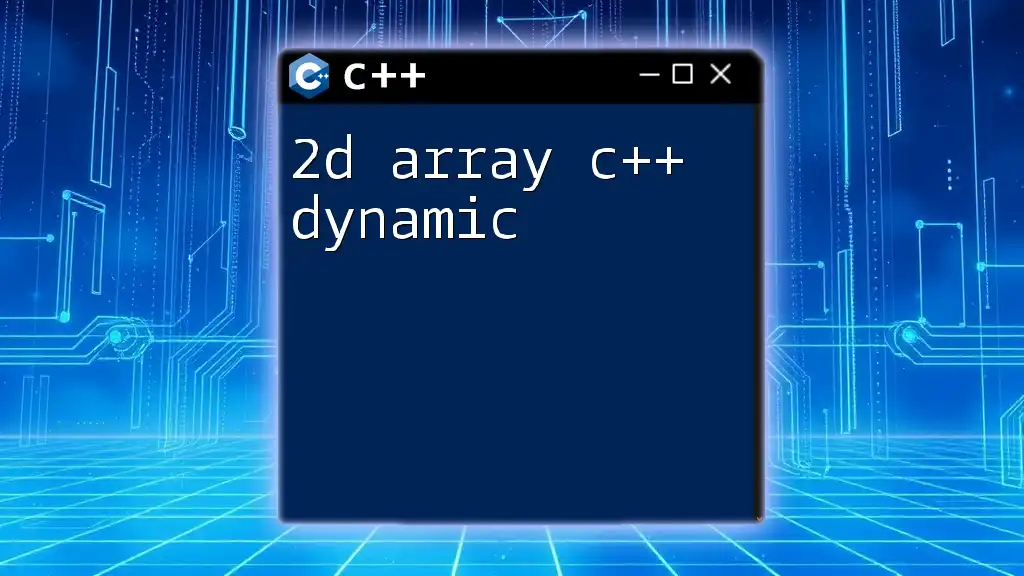
Conclusion
In summary, bitarray in C++ provides a powerful mechanism for managing binary data efficiently. By understanding how to create, manipulate, and optimize bit arrays, you will harness the full power of bit manipulation in your C++ applications. Whether you are dealing with large datasets, implementing algorithms, or just keen on efficient coding, mastering bit arrays is an invaluable skill. Don’t hesitate to experiment and create your own implementations to deepen your understanding of this essential data structure.