Unary operators in C++ are operators that operate on a single operand to perform various operations such as incrementing, decrementing, or negating the value.
Here is a code snippet that demonstrates the use of the unary increment operator:
#include <iostream>
int main() {
int number = 5;
std::cout << "Original number: " << number << std::endl;
// Unary increment operator
++number;
std::cout << "After increment: " << number << std::endl;
return 0;
}
Types of Unary Operators
Arithmetic Unary Operators
Unary operators primarily include arithmetic operators like unary plus (`+`) and unary minus (`-`). The unary plus operator doesn't change the value of its operand, while the unary minus operator negates the value.
For example:
int a = 5;
int b = -a; // b will be -5
In this snippet, `b` now holds the negative value of `a`. Unary operators are straightforward but play a crucial role in mathematical computations.
Increment and Decrement Operators
Another set of unary operators are the increment (`++`) and decrement (`--`) operators. These operators modify the value of a variable by one and can do so in two flavors: pre-increment and post-increment.
- Pre-increment (`++a`) increases the value before it’s used in an expression.
- Post-increment (`a++`) increases the value after it’s been used in the expression.
Consider the following code:
int a = 5;
int b = ++a; // Pre-increment: a is 6, b is 6
int c = a--; // Post-decrement: a is 5, c is 6
In this example, when `b` is assigned `++a`, `a` is incremented before its value is used. However, with `c`, `a` is decremented after its value is assigned to `c`. Understanding these nuances ensures that you avoid common pitfalls and errors in your programs.
Logical Unary Operators
The logical NOT operator (`!`) is a unary operator that inverses the boolean value of its operand. This is particularly useful in conditional statements.
For instance:
bool flag = true;
bool result = !flag; // result will be false
In this case, `result` is `false` because it negates the `true` value stored in `flag`. Logical operators like this are essential in control flow and decision-making in code.
Bitwise Unary Operators
Unary operators also include bitwise operations, with the bitwise NOT operator (`~`) allowing you to invert all bits of its operand. This can be particularly useful when working with integers and bit manipulation.
Here’s an example:
int x = 5; // binary: 0101
int y = ~x; // unary bitwise NOT: y becomes -6 (binary: 1010)
In this case, `~x` inverts the bits of `x`, resulting in `y` being `-6`. Understanding bitwise operations can significantly enhance your ability to perform low-level data handling and manipulation.
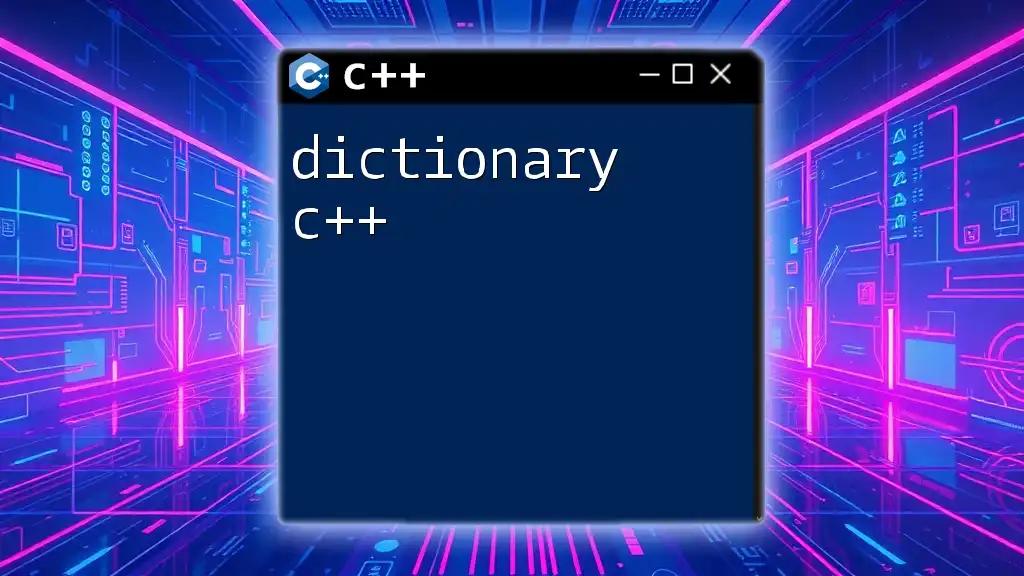
How Unary Operators Affect Program Flow
Operator Overloading for Unary Operators
In C++, you can also overload unary operators, allowing them to work with user-defined types. This concept can lead to very intuitive code that utilizes custom classes seamlessly.
For example, let’s look at a class that overloads the unary minus operator:
class Number {
public:
int value;
Number(int v) : value(v) {}
Number operator-() {
return Number(-value);
}
};
Number num(10);
Number negNum = -num; // negNum.value is -10
In this example, when the unary minus operator is applied to an instance of `Number`, it returns a new `Number` object with the negated value. Operator overloading is a powerful feature that enhances the expressiveness of your code.
Practical Examples and Use Cases
Unary operators come into play in various programming situations. For instance, increment and decrement operators are frequently used in loops, where concise and effective updates to loop counters can simplify code:
for (int i = 0; i < 10; ++i) {
std::cout << i << std::endl;
}
By using unary operators, the above example efficiently iterates through numbers without the verbosity of manually adjusting the counter.
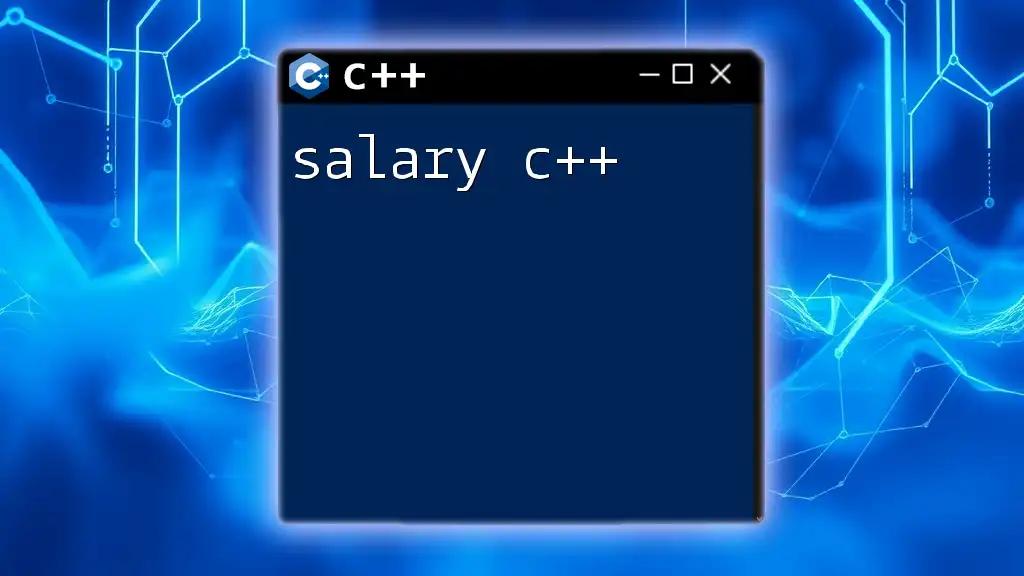
Common Mistakes and Misunderstandings
Mistakes with Increment and Decrement Operators
A common pitfall when working with increment and decrement operators arises in complex expressions. It’s essential to be aware of the timing of these operators:
int a = 5;
int b = (a++ + 5); // b is 10, and a is now 6
In this case, `a++` evaluates to 5 at the time `b` is calculated but is incremented immediately after. This behavior can lead to unexpected results if not properly understood.
Confusion between Unary and Binary Operators
Understanding the distinction between unary and binary operators is crucial. Unary operators operate on a single operand, while binary operators require two.
For example, in:
int a = 3;
int b = a + 2; // Binary operation
int c = -a; // Unary operation
Recognizing these differences and their contexts can significantly reduce programming errors, making your code cleaner and more efficient.
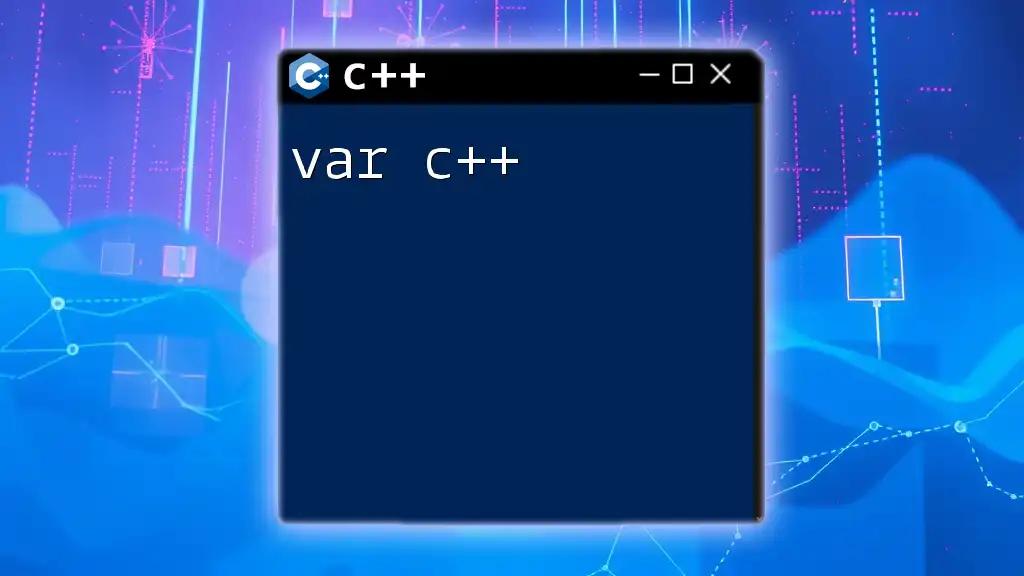
Best Practices for Using Unary Operators
Code Readability
Maintaining code readability is essential, even when using unary operators. While they can make code concise, clarity should take precedence. For example, rather than overusing pre-increment in complex expressions, opt for clearer expressions that convey intent.
Performance Considerations
It's important to understand how unary operators impact performance. In tight loops or performance-critical code, using unary operators can be more efficient than their longer equivalents, but as a general best practice, focus on the clarity of your code first.
for (int i = 0; i < n; ++i) {
// Your code here
}
Using `++i` or `i++` can be mere micro-optimizations. Ensure any use of unary operators genuinely benefits performance rather than complicating the code.

Conclusion
Unary operators are an essential aspect of C++ programming, providing functionality that enhances code efficiency and clarity. Mastering unary operators not only aids in better programming practices but also contributes significantly to the elegance of your code. Continue practicing and applying these concepts in your projects, ensuring that you understand their implications and contexts.
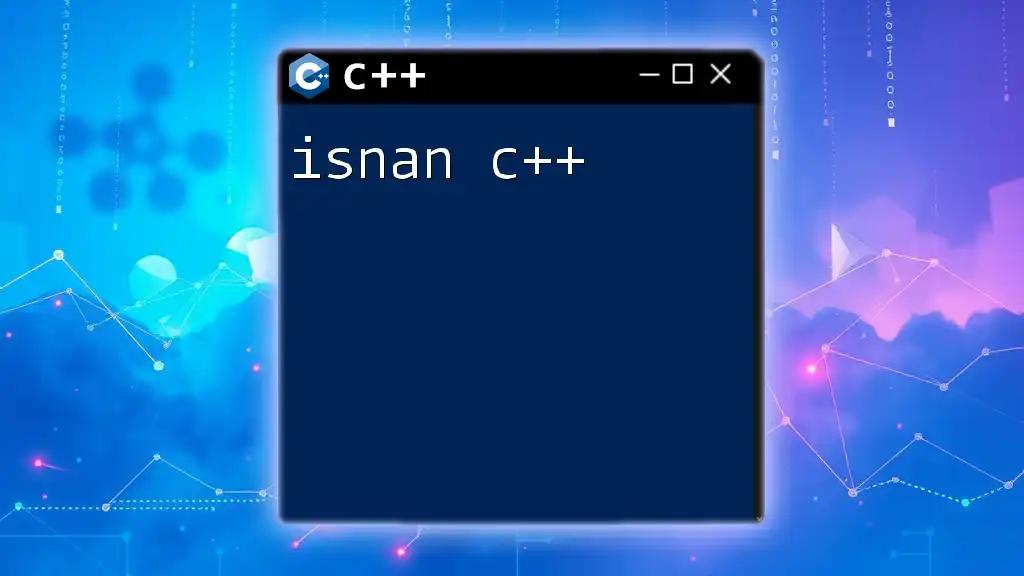
Additional Resources
For further reading on unary operators in C++, consider exploring various programming books, documentation, and tutorials that delve into operator overloading, control structures, and the intricacies of C++. Online platforms like Codecademy, Coursera, and documentation sites like cppreference.com also provide valuable insights and exercises to enhance your skills.