In C++, you can convert an integer to its binary representation using the following code snippet:
#include <iostream>
#include <bitset>
int main() {
int number = 42; // Example integer
std::cout << std::bitset<8>(number) << std::endl; // Converts to binary and prints
return 0;
}
Understanding the Basics of Binary
What is Binary?
Binary is a base-2 numeral system that uses only two symbols: 0 and 1. In the context of computing, binary is fundamental since it directly corresponds to the on/off states of electronic signals. Each binary digit (or bit) represents an increasing power of two, and unlike the decimal system which uses powers of ten, binary is essential for data representation in all digital devices.
How Integer Numbers Work
In programming, integers are whole numbers without a fractional component. They come in various types, such as `int`, `short`, and `long`. In C++, the `int` type is commonly used and can store a range of values depending on the system architecture (e.g., typically -2,147,483,648 to 2,147,483,647 for a 32-bit system). Understanding how these integers relate to their binary representations is vital when performing operations or manipulating data at a low level.
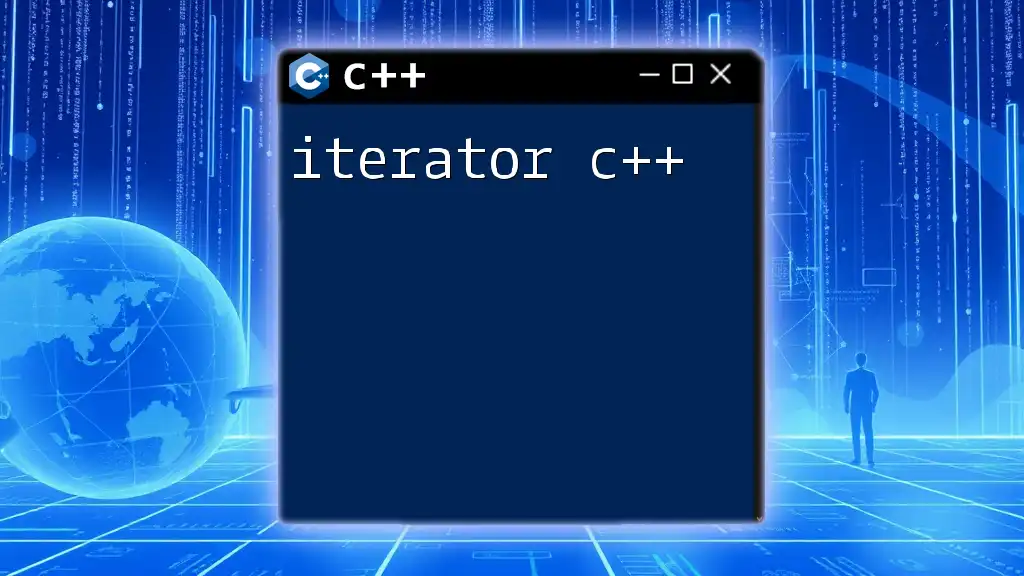
Why Convert Integer to Binary in C++
Common Use Cases
Converting integers to binary is essential for various applications, such as:
- Data Representation: Computers process and store data in binary form; thus, understanding binary representations helps in debugging and optimization.
- Bit Manipulation: Efficient coding practices often involve manipulating bits directly—performing operations like shifting or masking that only make sense in binary.
Real-World Applications
Binary conversions are prevalent in areas such as:
- Networking: Data is often transmitted as binary, so conversions help in understanding protocols and debugging network issues.
- Compression Algorithms: Many algorithms use binary representation for efficiency, reducing storage space and transmission times.
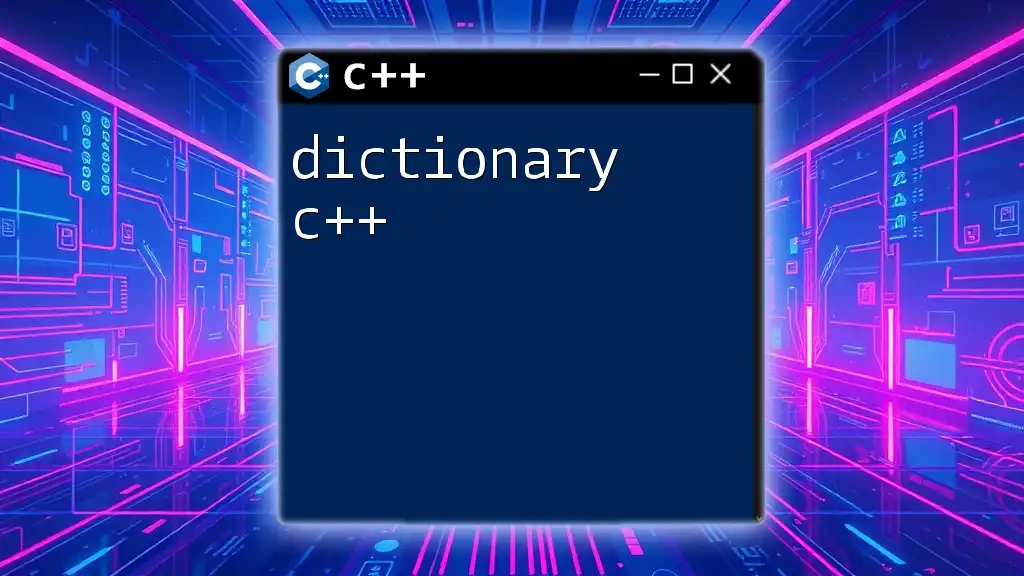
Methods to Convert Integer to Binary in C++
Using the Standard Library
One of the simplest means to convert an integer to binary in C++ is by using the `std::bitset`. This class allows for easy manipulation and representation of binary numbers.
Here’s how you can achieve this:
#include <iostream>
#include <bitset>
int main() {
int number = 42;
std::bitset<32> binary(number);
std::cout << "Binary representation of " << number << " is " << binary << std::endl;
return 0;
}
In this example, by creating a `bitset` of size 32, we can represent the integer 42 in its binary form. The output will clearly show the binary equivalent, padded to represent a full set of bits.
Custom Function for Conversion
If you want to perform the conversion manually, you can create a custom function that converts a decimal integer to binary through basic string manipulation. The logic involves dividing the number by 2 iteratively and capturing the remainder.
Below is a custom implementation:
#include <iostream>
#include <string>
std::string decimalToBinary(int n) {
std::string binary = "";
while (n > 0) {
binary = std::to_string(n % 2) + binary;
n = n / 2;
}
return binary.empty() ? "0" : binary;
}
int main() {
int number = 42;
std::cout << "Binary representation of " << number << " is " << decimalToBinary(number) << std::endl;
return 0;
}
In this function, we build the binary representation by concatenating the remainders. If the number is zero, it returns "0".
Recursive Method for Conversion
For those who appreciate a more elegant approach, a recursive method can be used to convert an integer to binary. This involves calling the function itself until the integer is reduced to zero.
#include <iostream>
void printBinary(int n) {
if (n > 1) {
printBinary(n >> 1);
}
std::cout << (n & 1);
}
int main() {
int number = 42;
std::cout << "Binary representation of " << number << " is ";
printBinary(number);
std::cout << std::endl;
return 0;
}
In this recursive approach, we shift the bits to the right and print the last bit by determining the least significant bit (LSB). This produces the same binary output efficiently.
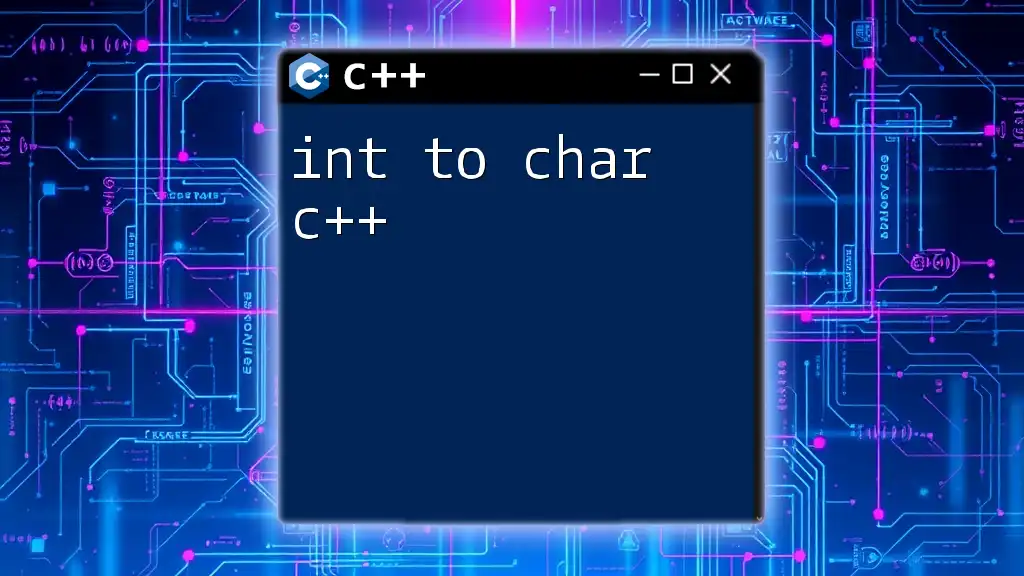
Converting Other Number Types
Converting Long Integers to Binary
When dealing with larger numbers, C++ has various integer types like `long` and `long long`. These types can also be converted to binary using the same methods described. You will need to adjust the size of the `bitset` to accommodate the larger size of the integer.
Converting Negative Integers
Negative integers require special handling, often using the two's complement method. This approach flips the bits of the absolute value and adds one. Here’s a basic rendition:
#include <iostream>
#include <bitset>
std::bitset<32> toTwoComplement(int n) {
return std::bitset<32>(n);
}
int main() {
int number = -42; // Example of negative integer
std::cout << "Binary representation of " << number << " using two's complement is " << toTwoComplement(number) << std::endl;
return 0;
}
In this example, the `bitset` constructor will handle the conversion automatically, displaying the negative number in two's complement form properly.
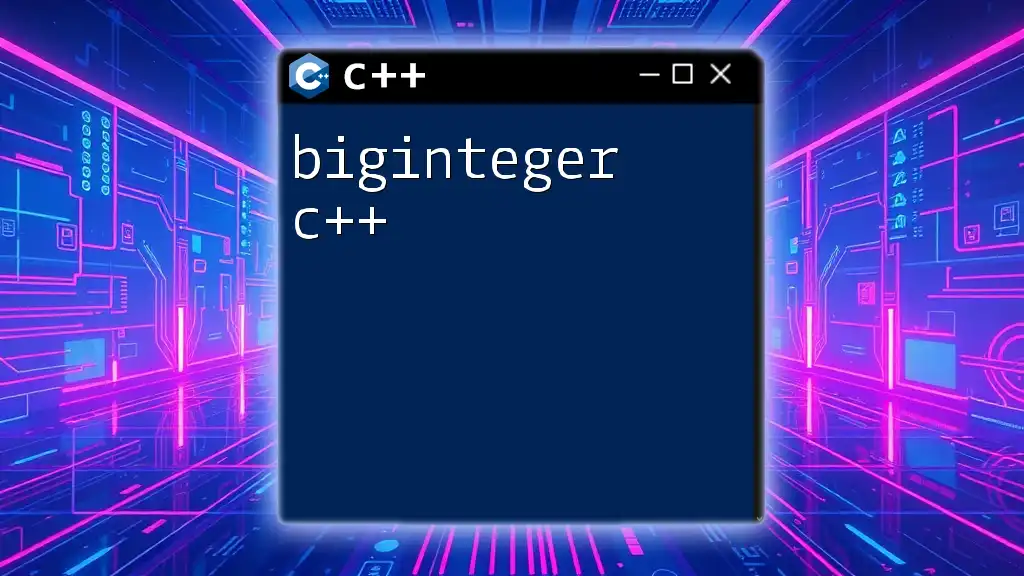
Practical Applications of Integer to Binary Conversion
Binary Operations and Bit Manipulation
Understanding binary representation enables programmers to execute operations like AND, OR, and NOT effectively. These operations are crucial in low-level programming, graphics processing, and other optimization tasks.
Use in Data Structures
Binary representation is vital in data structures such as Trees and Graphs, where it is often necessary to work with binary trees or to implement algorithms that require bit-level manipulation.
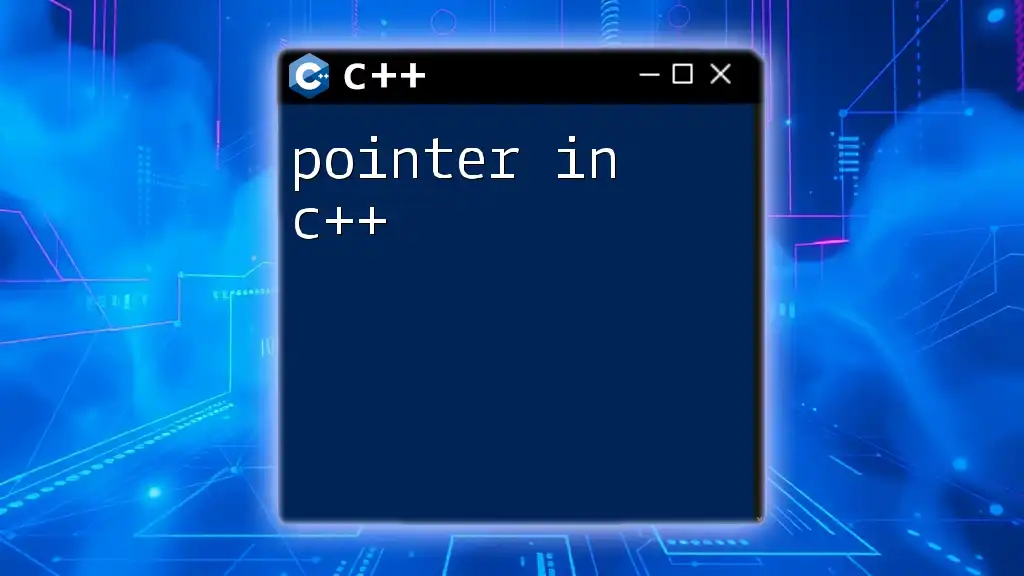
Conclusion
Converting integers to binary in C++ is not just a programming exercise; it forms the foundation of many important concepts in computing. Understanding this conversion enables better problem-solving skills, optimization techniques, and insights into how data is processed at the fundamental level. The ability to convert numbers efficiently also opens avenues toward more advanced topics in computer science.
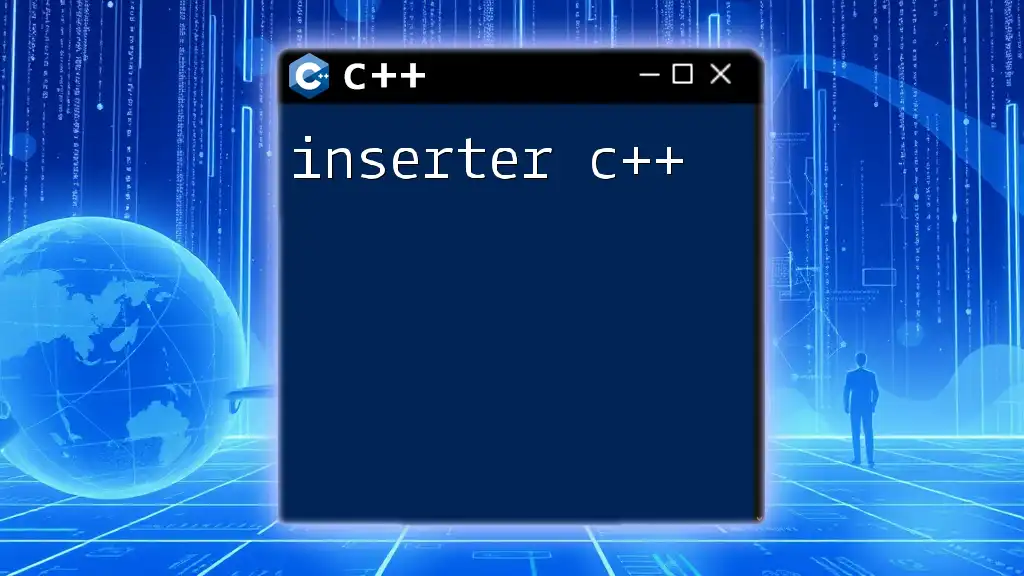
FAQs
What is the fastest way to convert integers to binary in C++?
Using `std::bitset` is generally one of the most efficient and easiest ways to convert integers to binary, especially for those less familiar with manual encodings.
Can I convert binary back to decimal in C++?
Yes, you can easily convert binary strings back to decimal using functions such as `std::stoi`. For example, you can leverage base-2 conversion as follows:
int decimalNumber = std::stoi(binaryString, nullptr, 2);
What are the potential errors during conversion?
Common errors include assumptions about integer size or mishandling negative numbers. It's crucial to implement proper checks and use appropriate types to avoid overflows or incorrect representations.
Encouragement to practice conversion methods frequently will enhance proficiency and confidence in handling similar numerical tasks.