In C++, you can convert an integer to a floating-point number simply by assigning it to a float variable or by using static_cast, which allows for precision in your conversions.
int intValue = 10;
float floatValue = static_cast<float>(intValue); // Converts int to float
Understanding Data Types
What is an int?
In C++, an `int` (short for integer) is a data type used to store whole numbers, which can be either positive or negative. The typical size of an `int` is generally 4 bytes, which allows it to represent a range of values from \(-2,147,483,648\) to \(2,147,483,647\). Given its characteristics, an `int` is commonly utilized for counting, indexing, and performing arithmetic operations.
For example, a simple declaration of an integer may look like this:
int number = 42;
What is a float?
A `float` is a data type in C++ designed for storing floating-point numbers, which are numbers that can contain fractions or decimal points. The size of a float is typically 4 bytes as well, but it has a precision limit — usually around 7 decimal digits. This means that very large or very small numbers may lose precision or experience rounding errors.
Here’s how you might declare a float in your code:
float decimal = 3.14f;
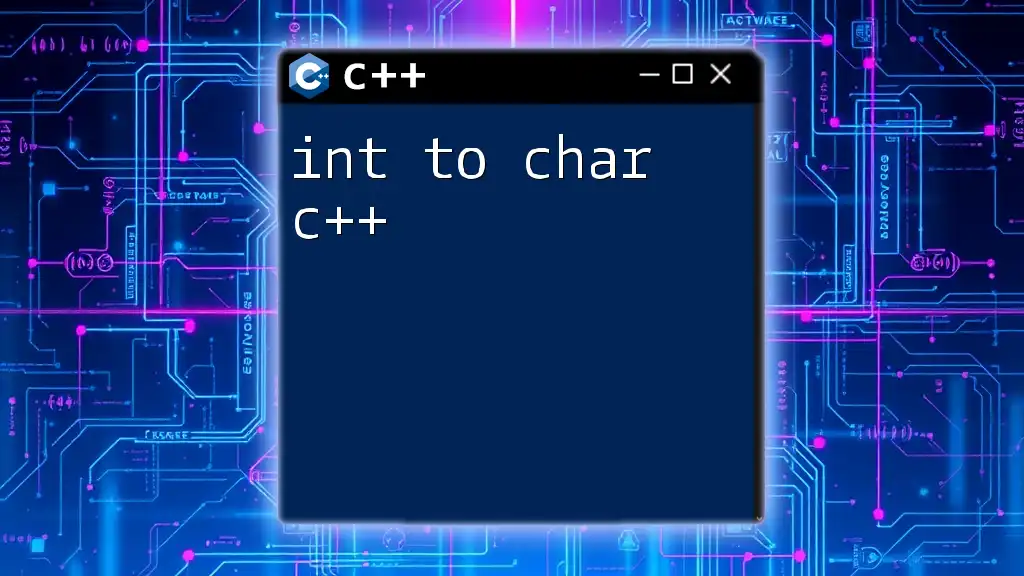
The Need for Conversion
When to Convert int to float
Converting an `int` to a `float` becomes essential particularly in scenarios where fractional results are required. For instance, when averaging a series of numbers, or when performing calculations that involve division, it is crucial to ensure that the result maintains precision rather than defaulting to truncation.
In graphics applications, physics calculations, or any computation requiring decimal representation, converting integers to floats can help achieve more accurate results.
Consequences of Not Converting
If you choose not to convert an integer to floating-point arithmetic, you may encounter several issues—most notably, integer division. When two integers divide in C++, the resulting value is also an integer, leading to truncation.
Consider the following code:
int a = 5, b = 2;
float result = a / b; // Result will be 2 instead of 2.5!
In this case, the division results in `2` because both operands are integers.
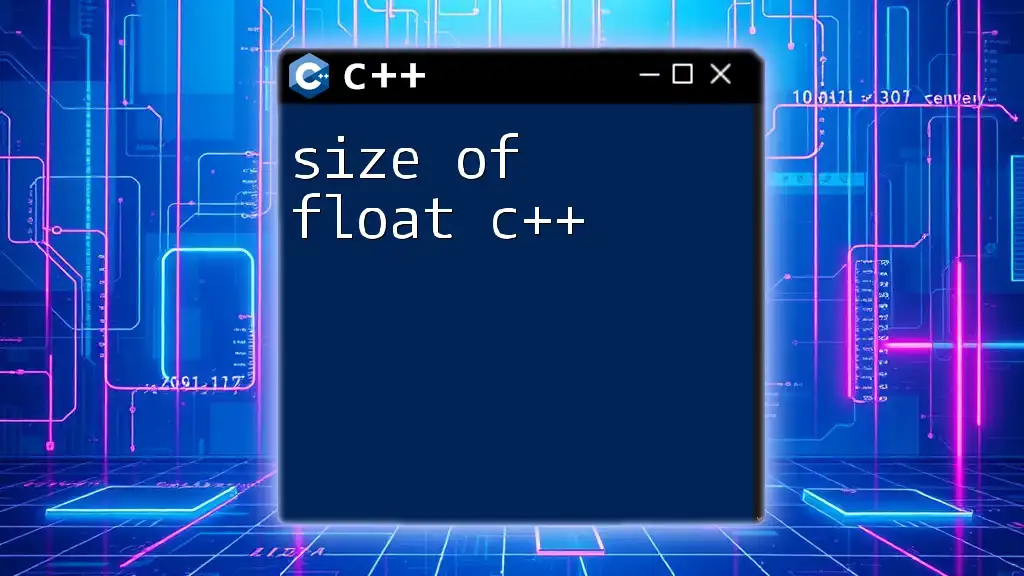
Methods for Converting int to float in C++
Implicit Conversion
C++ performs implicit conversion automatically whenever an integer is assigned to a float variable. The compiler ensures that the conversion retains as much information as possible, even though it is technically introducing a new data type.
Example of implicit conversion:
int num = 10;
float fNum = num; // Implicit conversion
Explicit Conversion (Type Casting)
Sometimes, it is necessary to perform an explicit conversion, or type casting, to clarify your intentions in the code and assure proper type transitions.
Using C-style Cast
C-style casting allows you to convert types using the syntax of `(type)variable`. While straightforward, it lacks the type safety and control provided by modern C++ casting methods.
Example of C-style casting:
int num = 10;
float fNum = (float)num; // C-style cast
Using static_cast
In C++, `static_cast` provides a safer and more expressive syntax for converting between types. It checks for the correctness of the conversion at compile time, making your code more robust.
Example using `static_cast`:
int num = 10;
float fNum = static_cast<float>(num); // Using static_cast
Using Functions
Using std::stof (String Conversion)
While the primary focus here is on converting integers, it's noteworthy that C++ also allows conversion from strings to floats using the `std::stof` function. This can be useful when parsing user inputs or reading numerical data from external sources.
Example of using `std::stof`:
std::string strNum = "10";
float fNum = std::stof(strNum); // Convert string to float
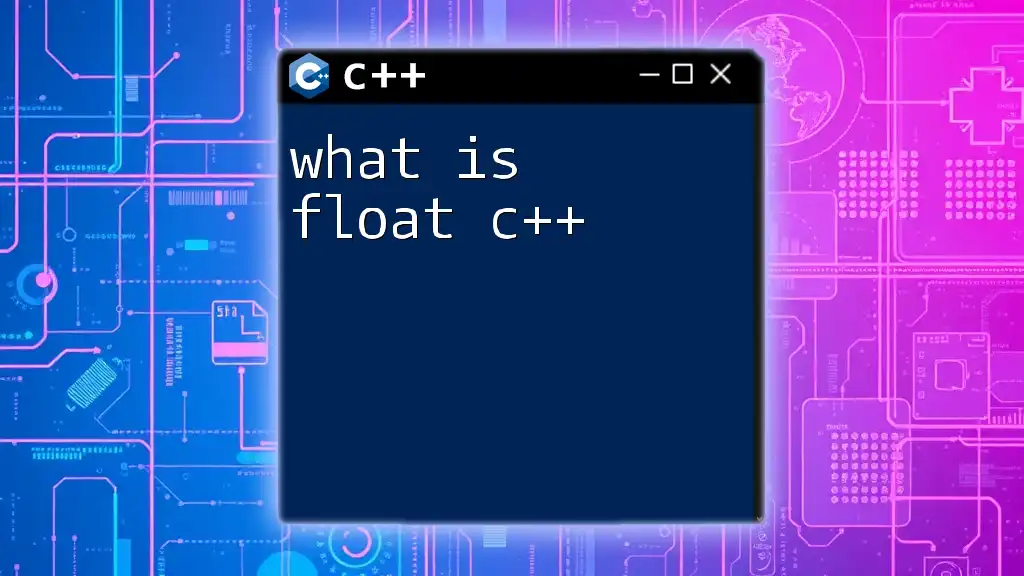
Best Practices for Conversion
Avoiding Precision Loss
Precision is paramount when working with floating-point calculations. When converting to `float`, especially from very large integers, you risk losing values beyond the precision limit of a `float`. To mitigate such risks, assess whether a `double` (which usually provides 15-16 decimal digits of precision) might be more appropriate for your calculations:
int bigInt = 1000000;
float fNum = static_cast<float>(bigInt);
In this case, while the conversion is successful, be cautious about values that exceed float precision.
Performance Considerations
Frequent conversions between `int` and `float` can impact the performance of your applications, particularly in compute-intensive tasks. While the cost of a single conversion is relatively low, inefficiencies can accumulate in loops or complex operations. It’s best practice to manage types effectively and convert only when necessary to maintain optimal performance.
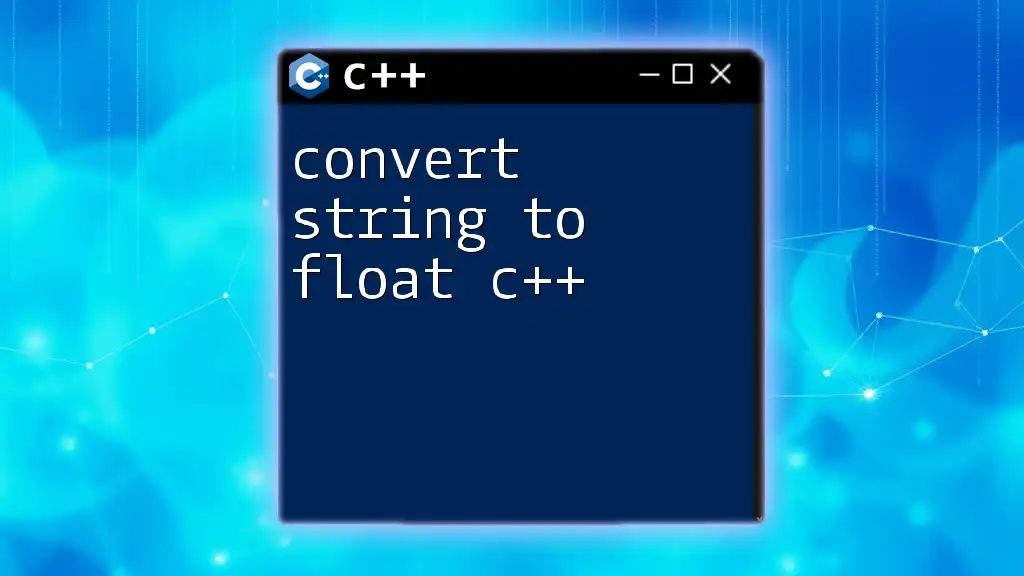
Common Mistakes in Conversion
Forgetting to Maintain Precision
One of the most prevalent mistakes when working with `int` and `float` is forgetting to explicitly handle type conversions, especially in division operations. This often leads to unwanted truncation of decimal values.
For example, to ensure correct decimal results while dividing integers, always perform explicit conversion:
int x = 5, y = 3;
float result = static_cast<float>(x) / static_cast<float>(y); // Correctly obtaining 1.66667
Using Floats in Integer Contexts
Another common error is using floats where integers are required. This can lead to unexpected behavior. Always double-check variable types to ensure you’re using the appropriate type for your operations.
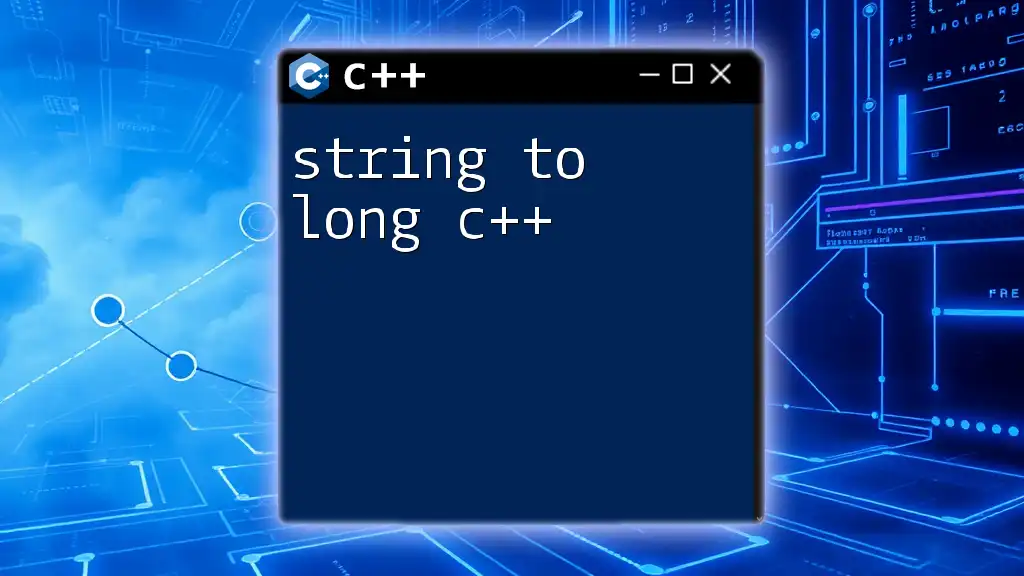
Conclusion
Understanding how to efficiently convert int to float in C++ is a critical skill in any programmer's toolkit. Whether employing implicit conversions or explicit casting techniques, knowing when and how to convert these data types can dramatically improve accuracy and performance in your applications. Always be mindful of potential pitfalls, including precision loss and the impact on performance metrics.
Arming yourself with this knowledge will empower you to write cleaner and more effective code, ultimately enhancing the functionality of your programs. Practice is key—experiment with the different methods discussed here to solidify your understanding and application of these concepts in real-world coding scenarios.
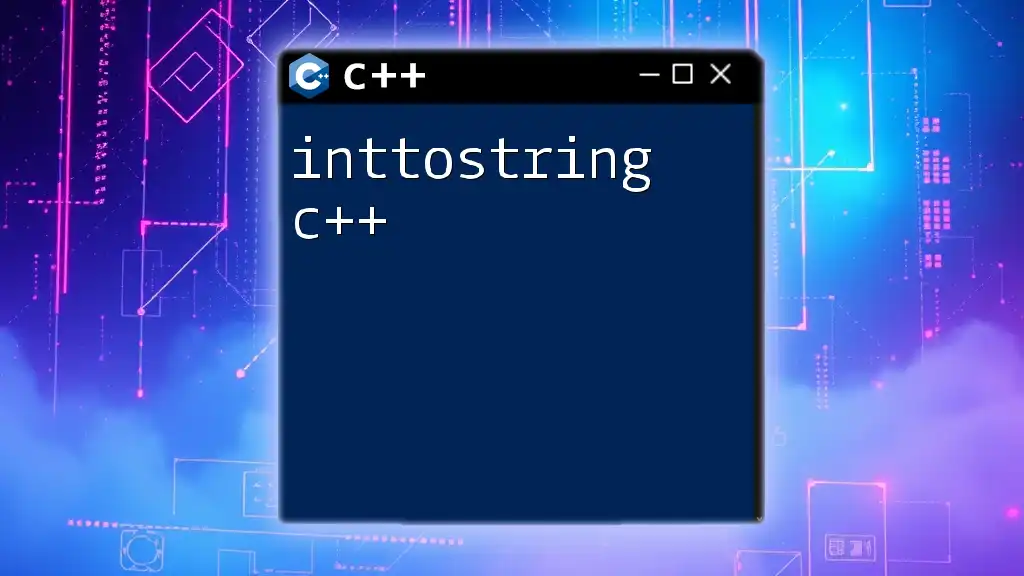
Additional Resources
For further reading, consider exploring the official C++ documentation or engaging with interactive coding platforms that emphasize type handling and conversion. Developing your understanding of these topics will not only make you a more effective programmer but also enhance your confidence in tackling diverse programming challenges.