The `floor` function in C++ returns the largest integer value not greater than the given floating-point number.
#include <iostream>
#include <cmath>
int main() {
double num = 2.7;
double result = floor(num);
std::cout << "The floor of " << num << " is " << result << std::endl;
return 0;
}
What is the Floor Function in C++?
The floor function is a mathematical operation that rounds a given number down to the nearest integer that is less than or equal to the original value. In C++, the floor function plays a key role in scenarios where this rounding behavior is necessary, especially in calculations involving indices, financial computations, and numerical simulations. Understanding this function is crucial, as it helps ensure that your programs yield predictable and accurate results.
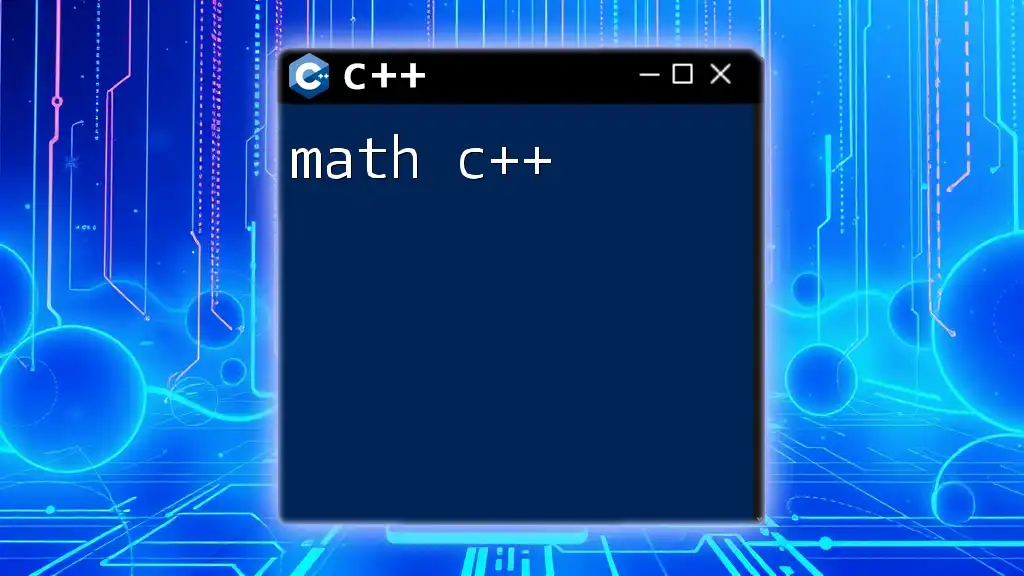
The C++ Floor Function: Overview
In C++, the floor function is part of the <cmath> library, which contains a wealth of mathematical functions for effective number manipulation. You can use the function to handle both positive and negative floating-point numbers, making it versatile for various programming applications.
The syntax for the `floor` function is as follows:
double floor(double x);
Where `x` is the floating-point number you want to round down.
To utilize the `floor` function in your code, make sure to include the necessary header:
#include <cmath>
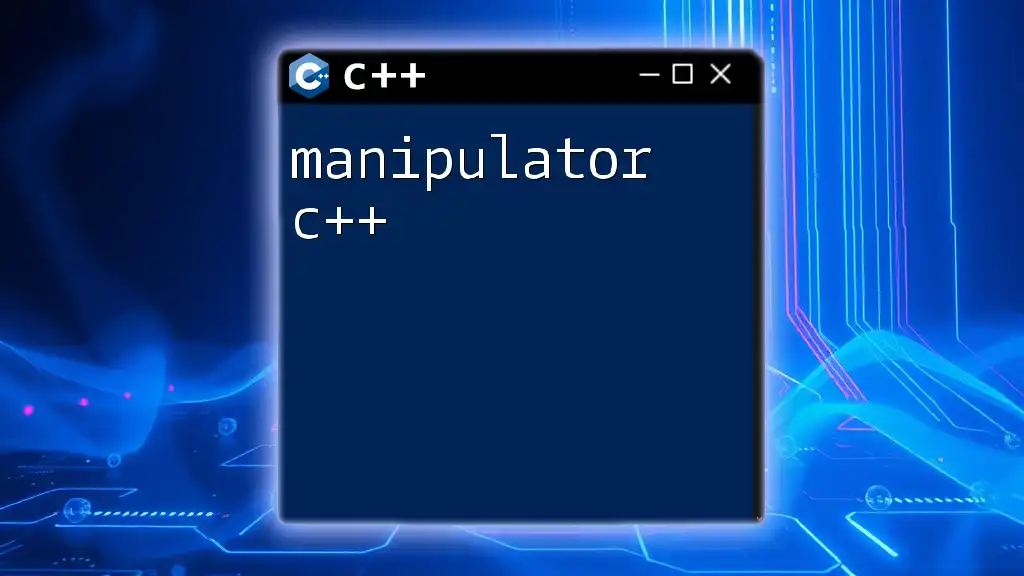
How to Use the Floor Function in C++
Basic Usage of the C++ Floor Function
Let's dive into some basic examples to illustrate how the floor function works in practice. Here’s a simple code snippet demonstrating its use:
#include <iostream>
#include <cmath>
int main() {
double num = 5.7;
std::cout << "The floor of " << num << " is " << std::floor(num) << std::endl;
return 0;
}
This code will output:
The floor of 5.7 is 5
The function effectively truncates the decimal portion of the number, demonstrating its straightforward functionality.
Working with Negative Numbers
One interesting aspect of the `floor` function is how it operates with negative numbers. Unlike simple rounding, where you might expect a different result, the floor function will return the next lowest integer. For instance:
double negativeNum = -3.4;
std::cout << "The floor of " << negativeNum << " is " << std::floor(negativeNum) << std::endl;
This code will produce:
The floor of -3.4 is -4
Here, the floor function rounds down to -4, highlighting its unique behavior with negative values.
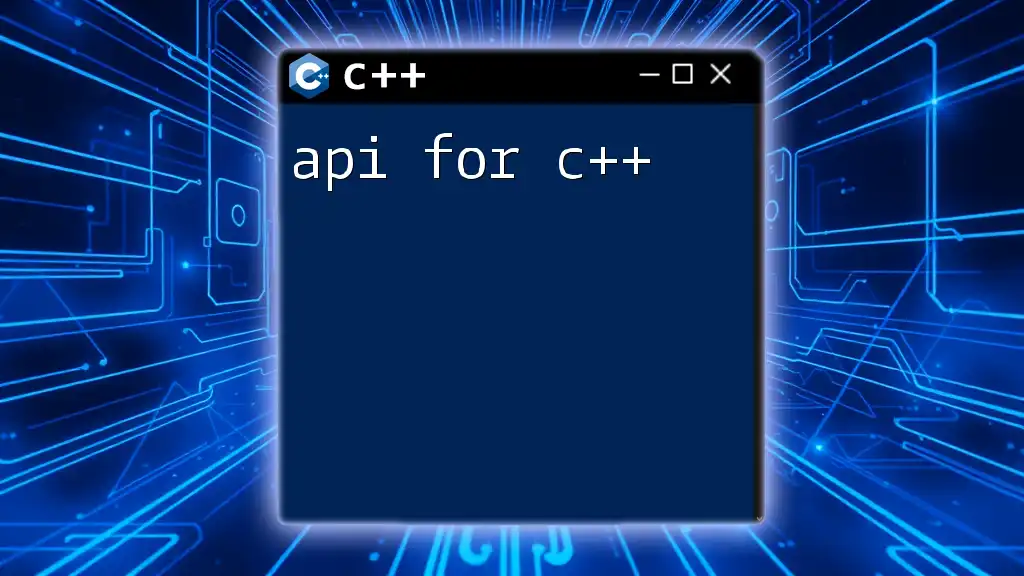
Practical Applications of the Floor Function
Rounding Down in Financial Calculations
In programming, especially in finance, it is common to deal with metrics that require rounding down. For instance, when calculating discounts or tax deductions, it might be necessary to floor the final results to avoid fractions of a cent.
Consider the following example:
double price = 9.99;
double discount = 0.20;
double finalPrice = std::floor(price * (1 - discount));
std::cout << "Final price: " << finalPrice << std::endl;
This will output:
Final price: 7
By flooring the result, we ensure that the final output adheres to standard pricing policies in many businesses.
Array Indexing and Looping
The floor function is also invaluable in situations where you need to calculate array indices, especially when dealing with fractional numbers produced by calculations. By flooring a value before using it as an index, you can eliminate potential out-of-bound errors.
Here’s an example:
double valueToIndex = 3.9;
int index = static_cast<int>(std::floor(valueToIndex));
std::cout << "Array index is: " << index << std::endl;
This code will produce:
Array index is: 3
Utilizing the `floor` function here assures that we retrieve the correct and expected index.
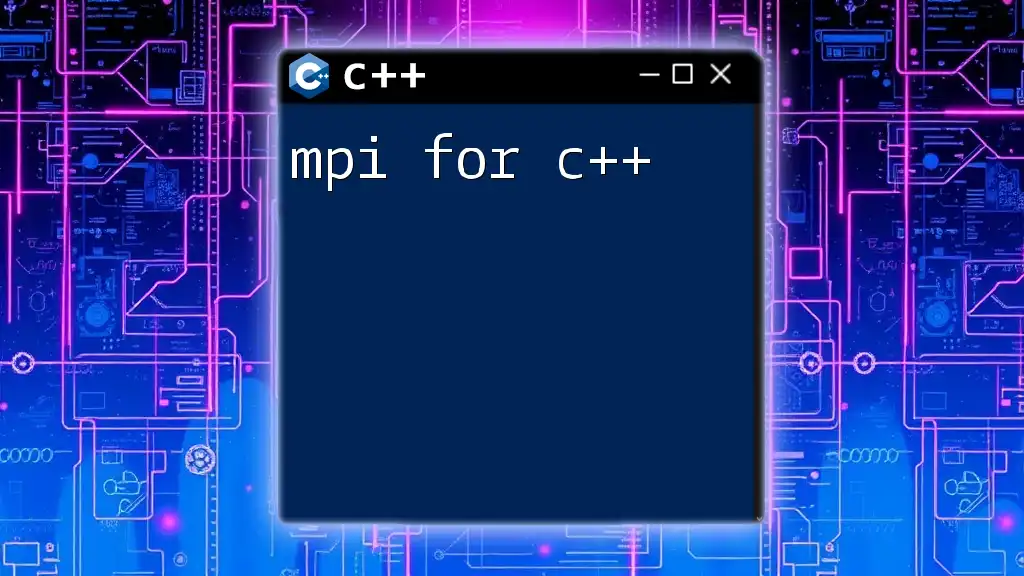
Common Mistakes When Using the Floor Function
Misunderstanding Floor vs. Round
A frequent source of confusion is the difference between the `floor` function and the `round` function. While `floor` always rounds down, `round` will round to the nearest integer, potentially increasing the value if the decimal part is 0.5 or higher.
Here’s a comparison:
double mistakenValue = 2.5;
std::cout << "Mistaken round: " << std::round(mistakenValue) << std::endl;
std::cout << "Correct floor: " << std::floor(mistakenValue) << std::endl;
This will yield:
Mistaken round: 3
Correct floor: 2
Understanding this distinction is fundamental when implementing mathematical functions in your C++ programs.
Type Conversions
Another common mistake relates to type conversions when storing the result of the floor function. Since the floor function returns a `double`, if you are storing that in an integer variable, it’s important to be aware of how implicit conversions work. It can lead to confusion or even data loss in certain scenarios. Here’s an illustration:
double value = 5.9;
int flooredValue = std::floor(value); // Implicit conversion
std::cout << "Floored value: " << flooredValue << std::endl;
The output will be:
Floored value: 5
This example shows how flooring the value and assigning it to an integer is perfectly valid, but knowing how implicit type conversion can affect your results is critical.
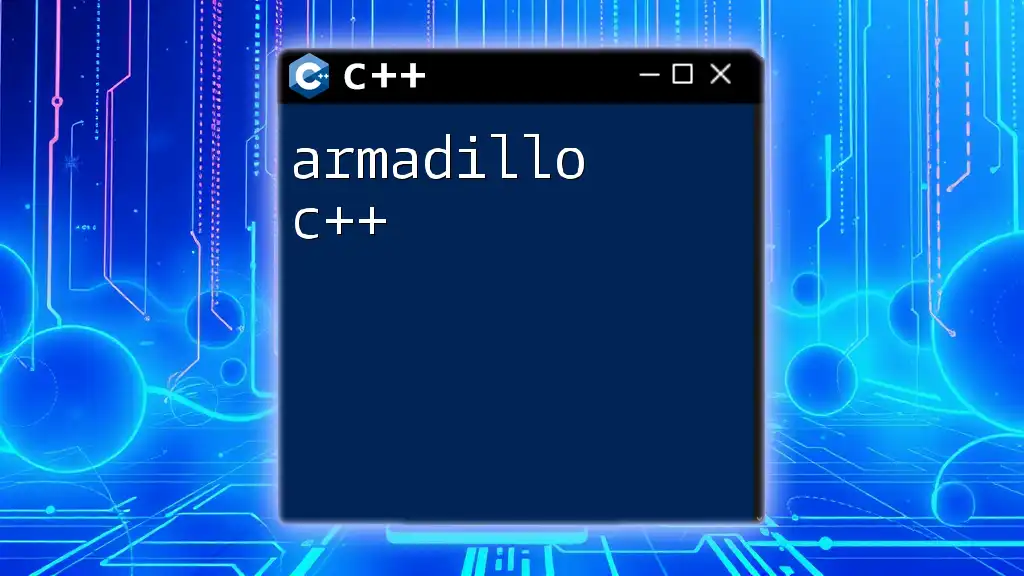
Conclusion
The math floor c++ function is a powerful tool that, when understood and applied correctly, facilitates accurate mathematical computations within your programs. From financial calculations to manipulating array indices, the importance of flooring operations cannot be understated.
By experimenting and implementing what you have learned about the floor function, you can enhance your programming skills and ensure your code works reliably across various scenarios.
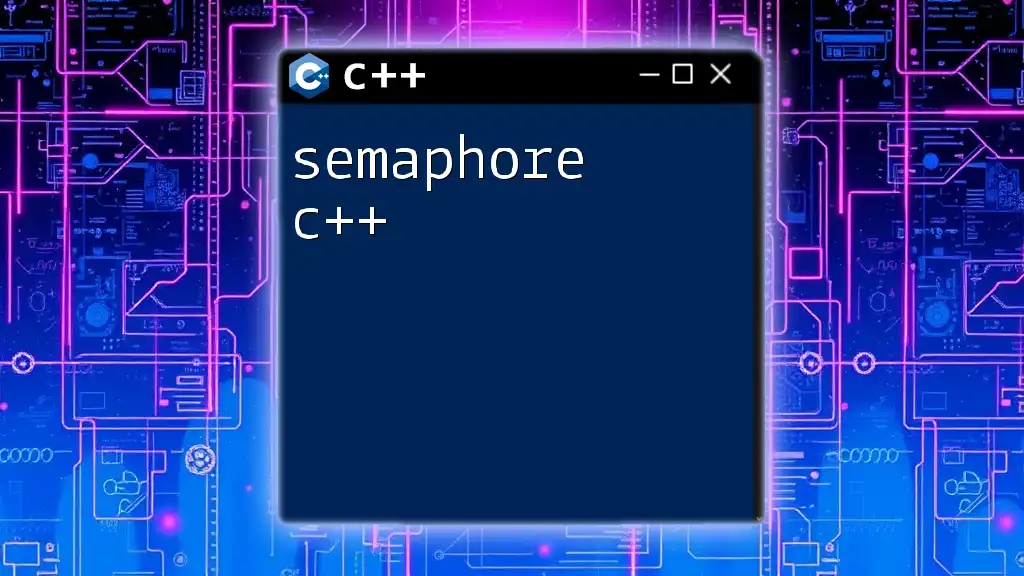
Additional Resources
For further reading and exploration, consider looking into the following topics:
- C++ documentation on mathematical functions
- Tutorials on floating-point arithmetic in C++
- Online courses focused on numerical methods in programming
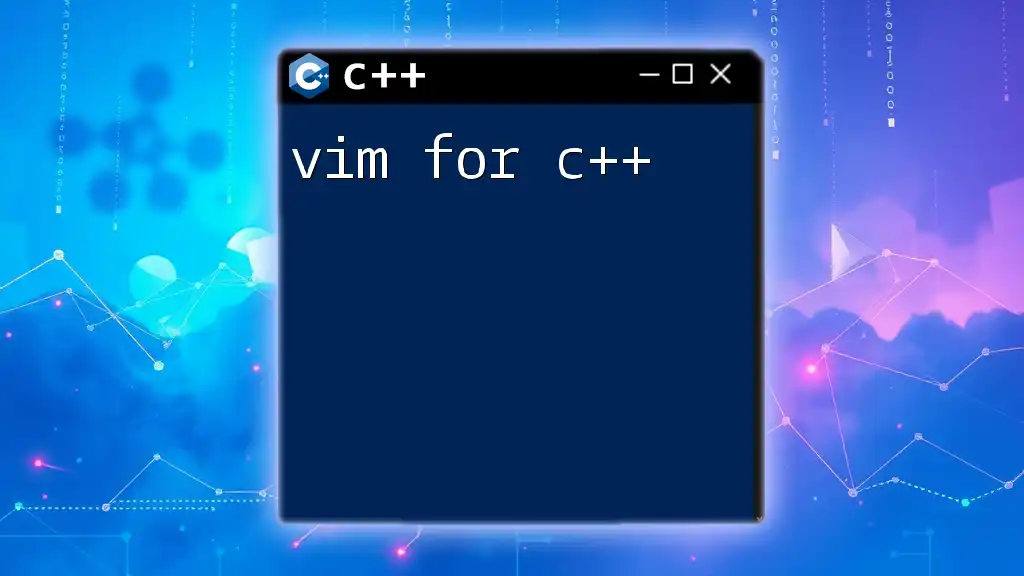
Call to Action
Now it’s your turn! Experiment with the `floor` function in your projects and discover how it can improve your work. Share your experiences, code snippets, or any questions you might have in the comments section below!