Vim is a powerful text editor ideal for C++ development, offering various commands and features that enhance coding efficiency and workflow.
// Example C++ code snippet to demonstrate function declaration and definition
#include <iostream>
void greet() {
std::cout << "Hello, world!" << std::endl;
}
int main() {
greet();
return 0;
}
Getting Started with Vim
Installation of Vim
To start using Vim for C++, the first step is installing the Vim text editor. The installation method varies depending on your operating system:
- Windows: You can download the installer from the official Vim website or use a package manager like `choco` to install it.
- macOS: Use Homebrew by running the command:
brew install vim
- Linux: Most Linux distributions come with Vim pre-installed. If not, you can typically install it using the package manager. For Ubuntu, use:
sudo apt install vim
Basic Vim Commands
Once installed, it is essential to familiarize yourself with some basic Vim commands to navigate and edit files effectively. Here are some fundamental commands:
- Navigation Commands: Use `h`, `j`, `k`, and `l` to navigate left, down, up, and right respectively.
- Inserting Text: You can enter insert mode with `i` (insert before the cursor), `a` (insert after the cursor), or `o` (insert a new line below).
- Saving and Quitting: Save your changes with `:w` and exit Vim with `:q`. If you need to do both simultaneously, just execute `:wq`.
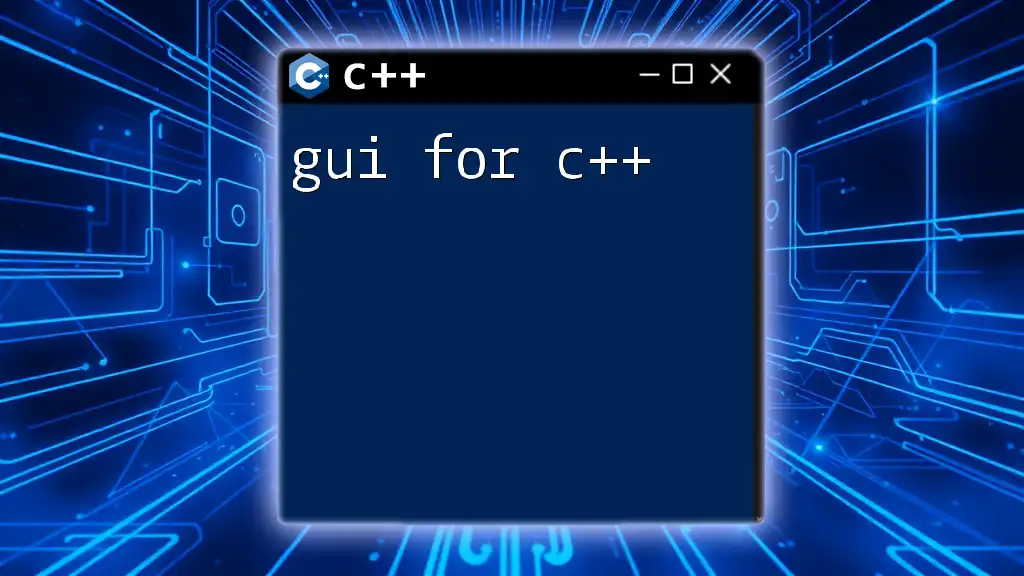
Configuring Vim for C++ Development
Setting Up the .vimrc File
The `.vimrc` file is the configuration file where you can customize Vim's behavior to suit your workflow. For C++ development, consider the following configurations:
syntax on " Enable syntax highlighting
set number " Show line numbers
set tabstop=4 " Set tab width
set shiftwidth=4 " Set indentation
set expandtab " Convert tabs to spaces
This basic configuration improves visibility and maintains code formatting.
Useful Vim Plugins for C++
Enhancing Vim with plugins can significantly speed up your C++ development. Here are a few recommended plugins:
- YouCompleteMe: This auto-completion plugin speeds up coding by suggesting completions as you type.
- ALE (Asynchronous Lint Engine): It provides real-time syntax checking and linting, helping you catch errors early.
- NERDTree: A file system explorer that allows easy navigation and management of your C++ files.
To install these plugins, consider using a plugin manager like `vim-plug`. Add the following lines to your `.vimrc`:
call plug#begin('~/.vim/plugged')
Plug 'ycm-core/YouCompleteMe'
Plug 'dense-analysis/ale'
Plug 'preservim/nerdtree'
call plug#end()
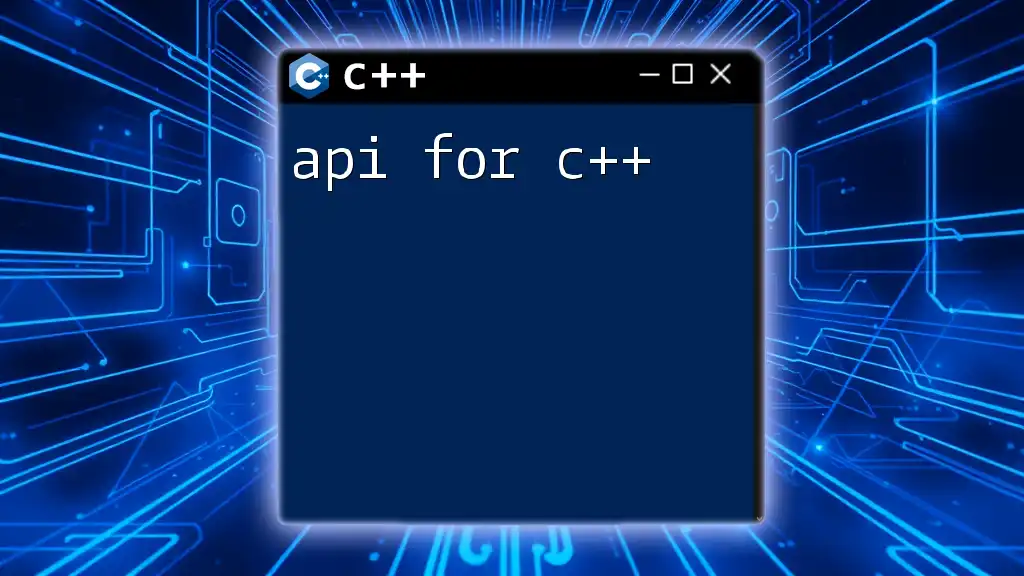
C++ Development in Vim
Compiling and Running C++ Code
One of the advantages of using Vim for C++ is the ability to compile and run code directly from the editor. To compile your C++ file quickly, you can use the command:
:!g++ % -o %<
In this command, `%` represents the current file, and `%<` is the current file name without the extension. After compiling, run your compiled code by typing:
:!./%<
Code Navigation and Management
Syntax Highlighting in Vim
Syntax highlighting in Vim not only beautifies your code but also improves readability and reduces errors. When you enable syntax highlighting, different elements of your C++ code (keywords, comments, variables) will appear in various colors, helping you distinguish quickly between them.
You can manually enable it by adding `syntax on` to your `.vimrc`, although it often comes enabled in newer versions.
Code Folding and Unfolding
Managing large C++ files can be challenging. Code folding allows you to collapse functions or classes to focus on the sections you are currently working on.
To fold code, place the cursor on the line you want to start folding and enter visual mode with `v`, then select the lines. Use the command `zf` followed by a movement command (like `j` to fold down) to create a fold. To unfold it back, you can use the command `zo`.
Code Snippets and Templates
To further streamline your workflow in Vim for C++, you can set up snippets for commonly used code patterns. Using the UltiSnips plugin enhances this feature, allowing you to create snippets that can be quickly inserted without having to type repetitive blocks of code each time.
snippet main
#include <iostream>
using namespace std;
int main() {
$0
return 0;
}
endsnippet
Here, `$0` indicates where the cursor will be placed after activating the snippet, allowing you to continue typing efficiently.
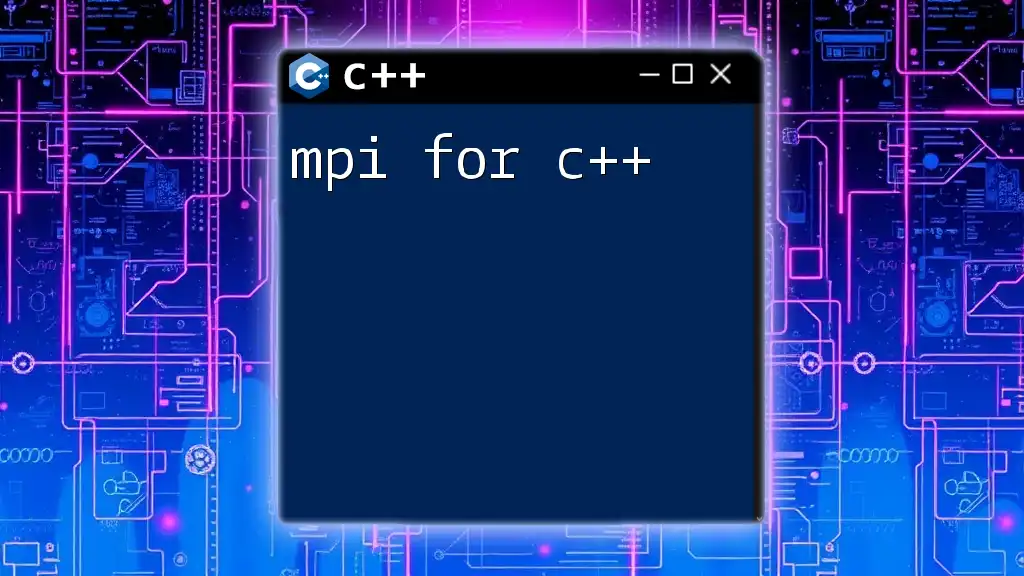
Debugging and Troubleshooting C++ Code in Vim
Integrating GDB with Vim
Debugging your C++ code within Vim can be done effectively by integrating GDB. After compiling, start a GDB session directly from Vim:
:!gdb %<
You can set breakpoints with the command `b main`, run your program using `run`, and navigate using GDB commands like `step`, `next`, and `print` for variable inspection. This integration allows you to leverage Vim's powerful navigation while debugging.
Common C++ Errors and Solutions
While coding in C++, you're likely to encounter compilation and runtime errors. Common issues might include:
- Syntax errors (e.g., forgetting a semicolon or curly brace)
- Type mismatches (assigning different data types)
- Logical errors leading to unexpected behavior
When debugging, always read the compiler's error messages closely; they often point directly to the line in your code that needs attention. Being meticulous will save you time and frustration.
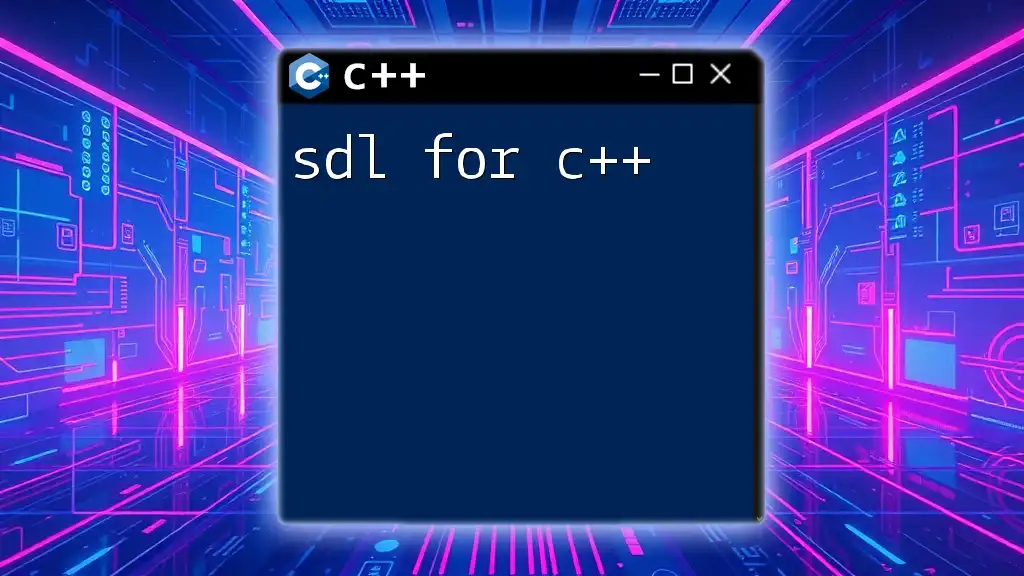
Enhancing Your Vim Workflow for C++
Custom Key Mappings
Custom key mappings allow you to streamline operations that you perform frequently. To enhance your C++ workflow in Vim, you might want to create custom mappings for running code or opening files. Add the following lines to your `.vimrc`:
nnoremap <leader>r :!g++ % -o %< && ./%<<CR>
This command maps `<leader>r` to compile and run the currently opened C++ file in one swift action.
Clipboard Management
Managing the clipboard within Vim can be simple yet powerful. By using `"*y` to yank (copy) and `"*p` to paste, you can seamlessly transfer text between Vim and other applications, thus enhancing your productivity.
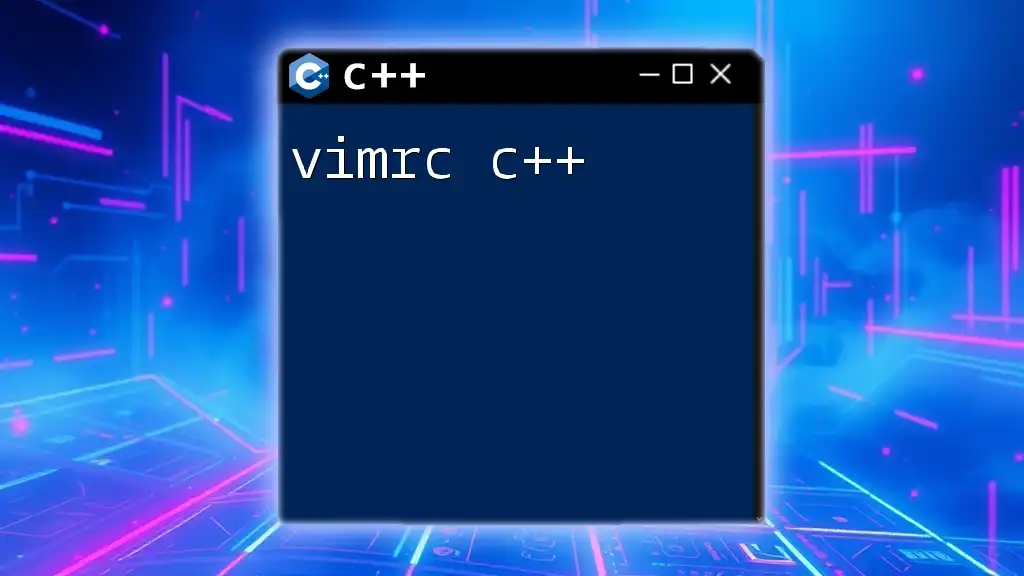
Conclusion
Using Vim for C++ offers numerous benefits, from efficient coding practices to advanced debugging capabilities. With the right configuration and tools, you can transform your development experience, making it both enjoyable and productive. Dive deeper into Vim's capabilities and personalize your environment to become a more proficient C++ programmer.
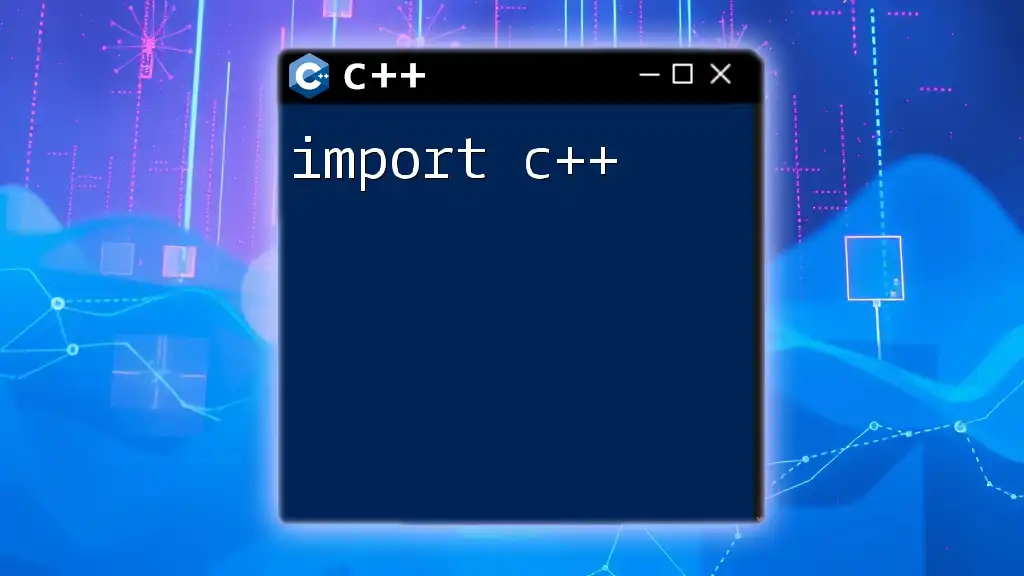
Additional Resources
To further expand your knowledge of Vim and C++, consider exploring books, online courses, or tutorials dedicated to mastering these tools. Engaging with community forums can also provide valuable insights and support from fellow Vim users.
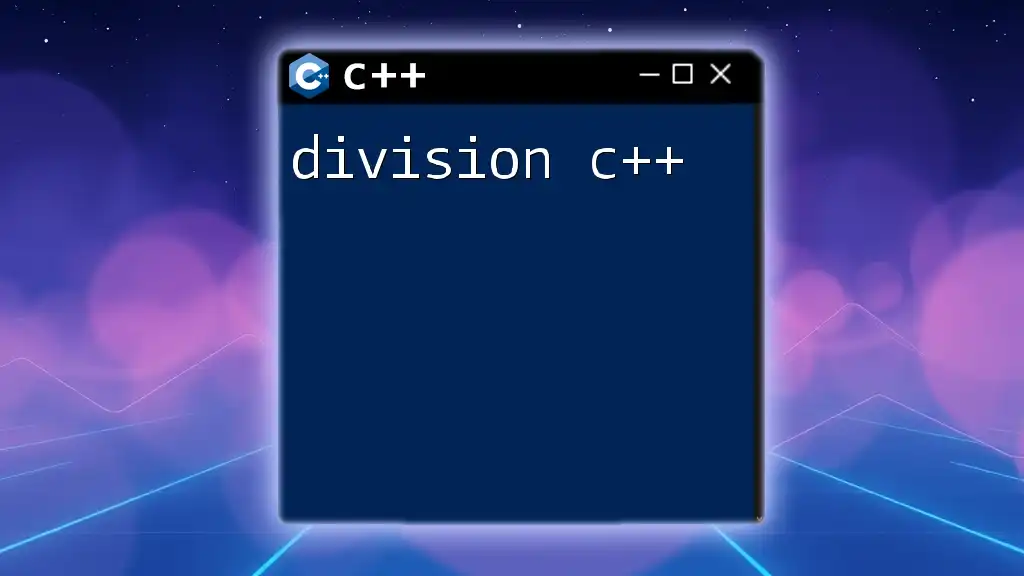
FAQs about Vim for C++
If you have questions about using Vim for C++, you’re not alone! Here are some frequently asked questions that may help clear up common misunderstandings:
-
What are the advantages of using Vim over other editors? Vim is lightweight, highly customizable, and boasts an efficient workflow that many developers prefer.
-
Can I use Vim with other programming languages? Absolutely! Vim supports a wide range of programming languages with the right configuration and plugins.
-
Is Vim difficult to learn? While it has a steep learning curve, mastering Vim can lead to increased productivity in the long run. There are many resources available to aid beginners in their journey.
Investing time into learning Vim for C++ will undoubtedly enhance your coding experience and efficiency. Happy coding!