An API for C++ is a set of tools and protocols that allows developers to interact with software components or services, enabling the integration of functionalities with simplicity and efficiency.
Here's a basic example of using a C++ API to send a GET request using the popular library 'libcurl':
#include <iostream>
#include <curl/curl.h>
int main() {
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
}
return 0;
}
What is an API?
An API, or Application Programming Interface, serves as an intermediary that enables different software applications to communicate with each other. By defining the methods and data formats that applications can use to request and exchange information, APIs facilitate interoperability and integration between disparate systems. In the context of C++, APIs open up numerous possibilities for enhancing the functionality and extending capabilities of your applications.
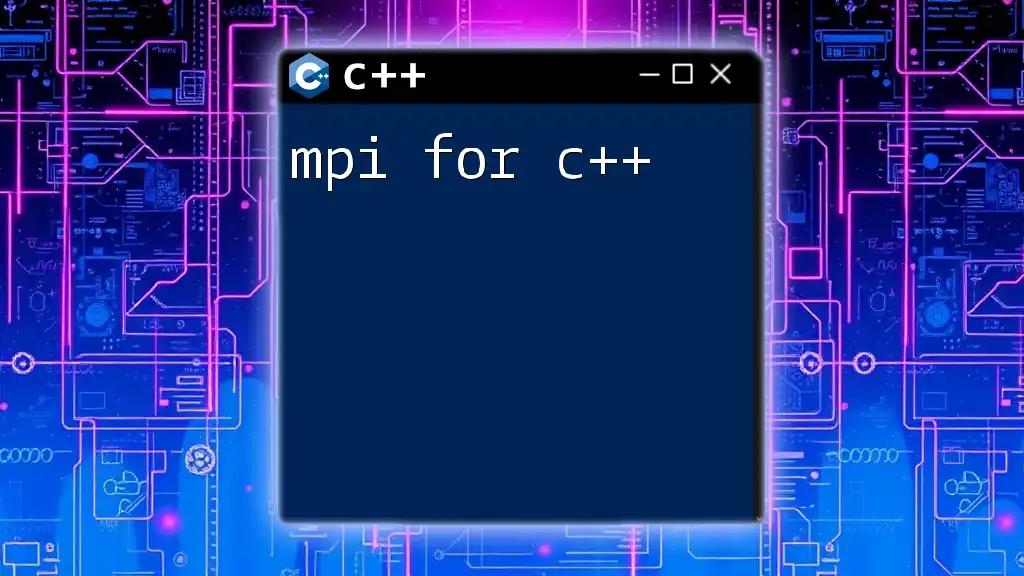
Why Use APIs in C++?
The advantages of utilizing APIs in C++ are substantial:
-
Enhancing functionality and performance: APIs allow developers to leverage existing functionality, optimizing their work without starting from scratch. For example, a graphics application can integrate with a powerful image processing library through its API, thus improving performance and evolving feature sets.
-
Encouraging modular design: APIs promote a modular approach to coding, breaking down complex functionality into simpler, manageable modules. This not only makes development easier but also streamlines maintenance and updates.
-
Facilitating code reusability: By using APIs, developers can reuse code across various projects. This reduces development time and enables teams to focus on more complex and innovative features rather than reinventing the wheel.
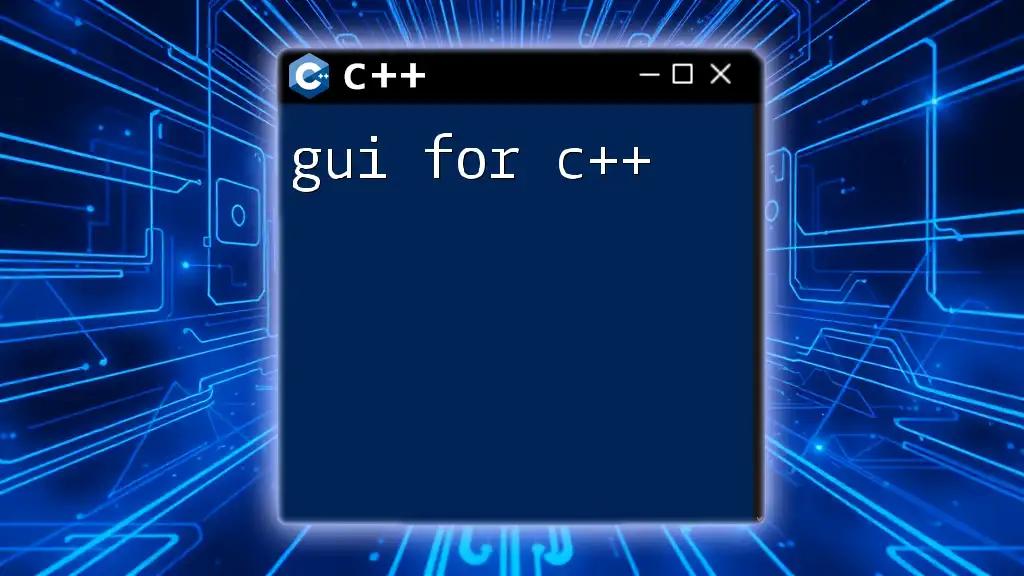
Types of APIs for C++
Standard APIs
C++ offers a rich set of standard libraries that encompass varying APIs. The Standard Template Library (STL) is particularly noteworthy, as it provides a collection of algorithms and data structures essential for efficient programming.
- Common STL components:
- Vectors: Dynamic arrays that can grow in size.
- Lists: Doubly linked lists that provide efficient insertions and deletions.
- Maps: Associative containers that store elements in key-value pairs.
Third-Party APIs
Third-party APIs extend the functionality of C++ applications by providing external libraries. By integrating these libraries, developers can harness complex functionalities with ease.
- Popular third-party APIs:
- Boost: A widely-used library that offers a variety of features ranging from smart pointers to multi-threading capabilities.
- Qt: Comprehensive libraries for developing GUI applications, making it easier to create cross-platform interfaces.
- OpenCV: An extensive library mainly used for computer vision tasks, providing numerous algorithms and image-processing functions.
Web APIs
Web APIs have gained immense popularity for their ability to communicate over HTTP, enabling applications to interact with online services.
-
Introduction to RESTful APIs: Representational State Transfer (REST) APIs use standard HTTP methods for CRUD (Create, Read, Update, Delete) operations, making them a preferred choice for web services.
-
How to interact with web services in C++: By using HTTP client libraries, C++ developers can easily send requests and handle responses from web APIs, allowing for seamless integration of online resources.
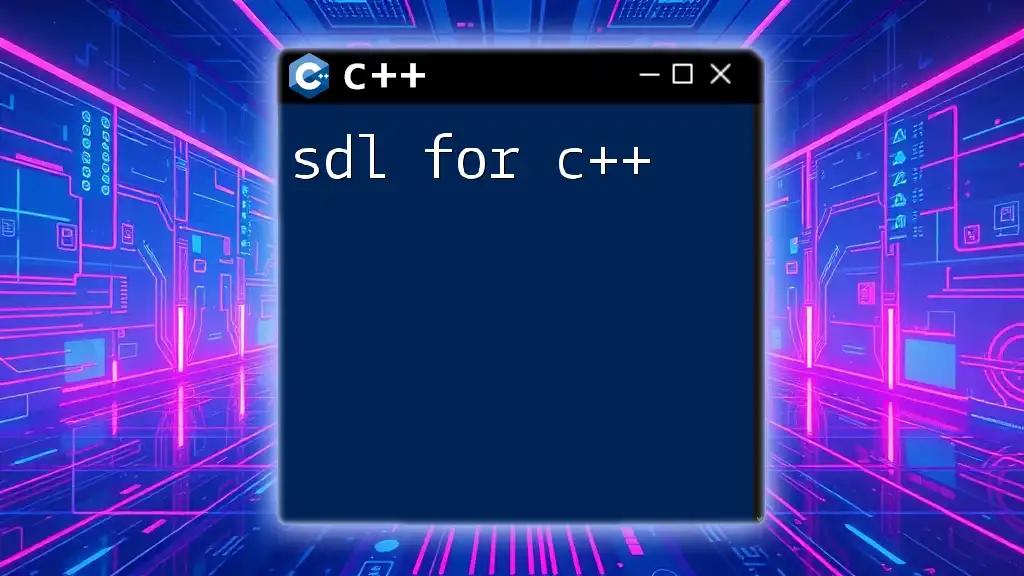
Building a Simple API in C++
Setting up the Development Environment
Before developing your API, it's essential to set up your development environment properly. Required tools may include a modern C++ compiler, a build system like CMake, and specific libraries such as cpprestsdk for handling HTTP requests.
Creating a Basic API
When creating a basic API in C++, organization and structure are vital. A common approach involves setting up a web server that listens for HTTP requests and responds accordingly.
As an example, let’s build a simple REST API using C++:
#include <iostream>
#include <cpprest/http_listener.h>
using namespace web;
using namespace web::http;
using namespace web::http::experimental::listener;
void handle_get(http_request request) {
json::value response_data;
response_data[U("message")] = json::value::string(U("Hello, World!"));
request.reply(status_codes::OK, response_data);
}
int main() {
uri_builder uri(U("http://localhost:8080/api"));
auto addr = uri.to_uri().to_string();
http_listener listener(addr);
listener.support(methods::GET, handle_get);
listener.open()
.then([&listener](){ std::wcout << L"Starting to listen at: " << listener.uri().to_string() << std::endl; })
.wait();
std::string line;
std::getline(std::cin, line);
return 0;
}
In this example, we set up an HTTP listener on `localhost:8080/api` and define a simple GET endpoint that responds with a JSON message.
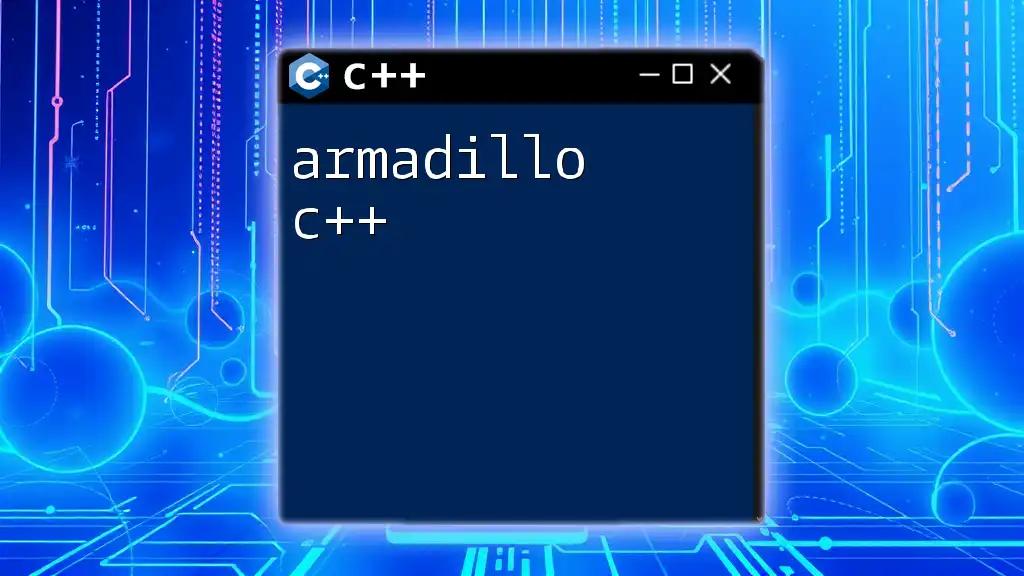
Consuming APIs in C++
Understanding HTTP Methods
When consuming APIs, understanding HTTP methods is crucial. Each method serves a specific purpose:
- GET: Retrieve data from the server.
- POST: Send data to the server to create a new resource.
- PUT: Update an existing resource on the server.
- DELETE: Remove a resource from the server.
Making HTTP Requests
C++ developers can utilize libraries such as cpprestsdk to make HTTP requests easily. Here’s how to send a GET request to our earlier API:
#include <cpprest/http_client.h>
using namespace web::http;
using namespace web::http::client;
void get_request() {
http_client client(U("http://localhost:8080/api"));
client.request(methods::GET).then([](http_response response) {
if (response.status_code() == status_codes::OK) {
json::value response_data = response.extract_json().get();
std::wcout << response_data[U("message")].serialize() << std::endl;
}
}).wait();
}
In this code snippet, we create an HTTP client that sends a GET request to our API and prints out the received message.
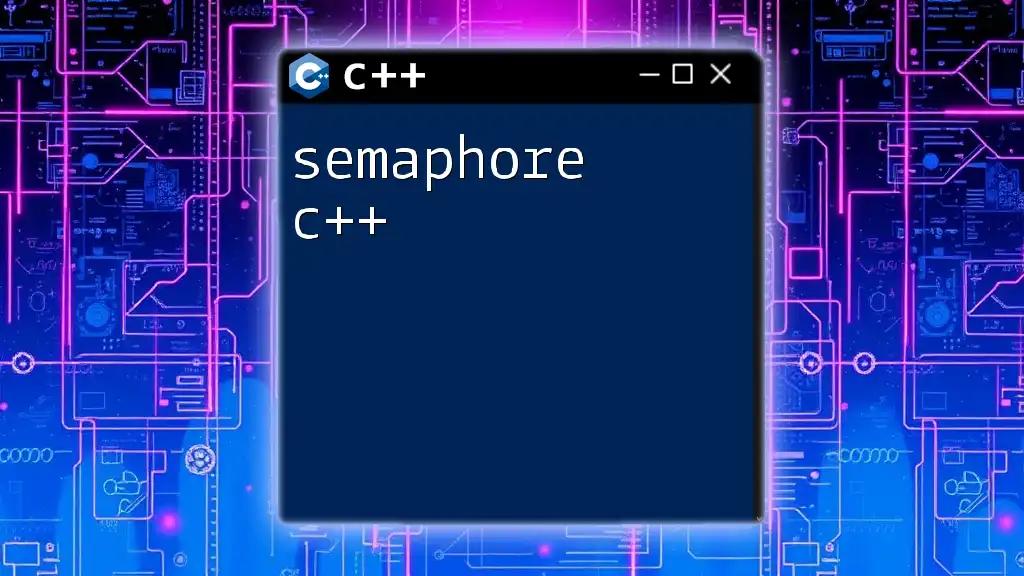
Best Practices for API Development in C++
Designing an Efficient API
A well-designed API is key to developer satisfaction and application usability. Important considerations include:
- Clear and concise endpoints: Make sure your endpoint structures are intuitive and easily understandable.
- Versioning strategies: Implement versioning in your API to allow for improvements without breaking existing functionality for users.
Error Handling and Debugging
Effective error handling can prevent user frustration. Familiarize yourself with common HTTP status codes, such as:
- 200 OK: The request was successful.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: The server encountered an unexpected condition.
Security Considerations
Implementing security measures is critical for any API:
- Authentication: Use methods like OAuth or API keys to ensure that only authorized users have access.
- Data validation: Validate input data rigorously to prevent vulnerabilities such as SQL injection.
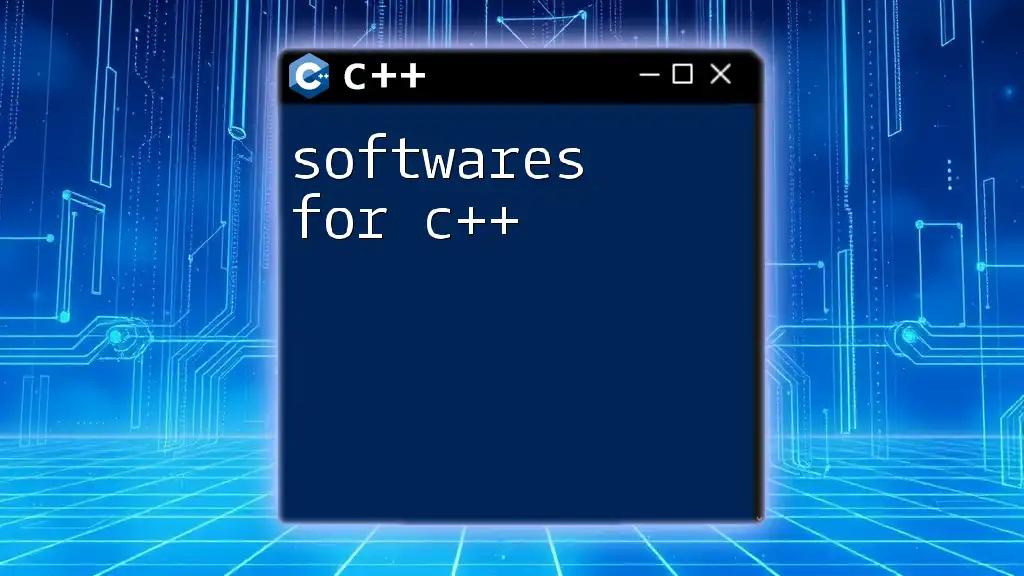
Tools for API Development in C++
API Testing Tools
Testing your API is essential to ensure it behaves as expected. Postman is a popular API testing tool that allows you to send requests and analyze responses easily. Automated testing frameworks can also help streamline this process.
Documentation Tools
Documentation is vital for users and developers interacting with your API. Generators like Swagger and Doxygen are invaluable for creating comprehensive, user-friendly API documentation.

Resources for Learning More About APIs in C++
Developers seeking to expand their knowledge of APIs in C++ can turn to a range of resources:
Books and Online Courses
There are numerous books and courses that cover C++ and API development in depth. Reading materials can provide a wealth of information that may not be readily available in online articles.
Community and Support
Engagement in developer communities, such as Stack Overflow or GitHub, can provide valuable insights. Asking questions, sharing knowledge, and contributing to open-source projects can significantly enhance your experience and expertise.
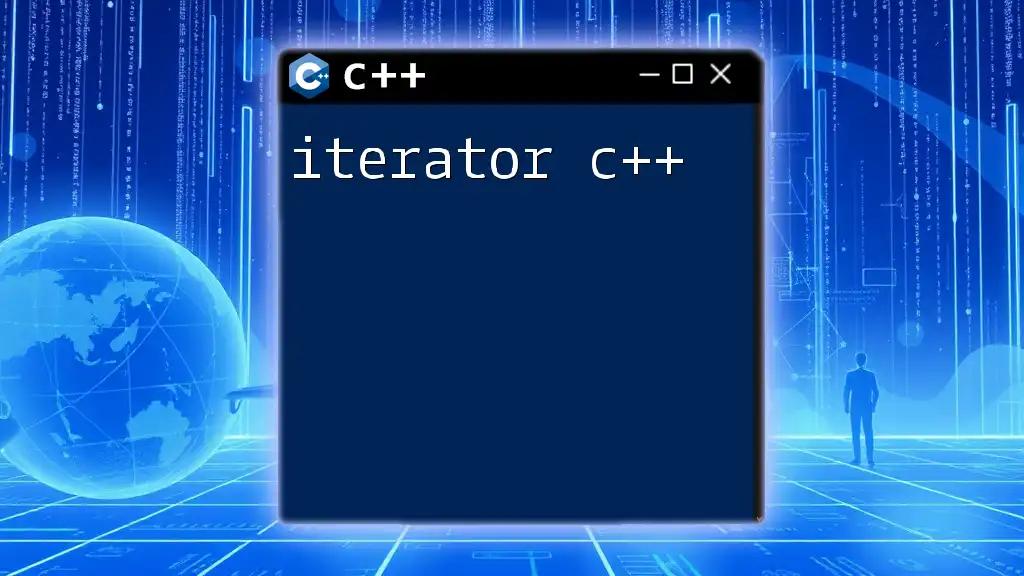
Conclusion
Understanding and utilizing APIs in C++ is essential for modern software development. By building efficient, modular, and secure APIs, developers can create powerful applications that leverage existing technology to provide an enhanced user experience. As you embark on your journey in API development, remember to practice what you've learned, engage with the community, and continually refine your understanding.