A GUI (Graphical User Interface) for C++ allows developers to create visually appealing applications with interactive elements, enhancing the user experience.
Here's a simple example using the popular Qt framework:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, World!");
button.resize(200, 100);
button.show();
return app.exec();
}
Why Use C++ for GUI Development?
When it comes to graphical user interface (GUI) development, C++ offers several compelling advantages that make it a preferred choice for many developers. Let's explore some of these benefits in detail.
Performance
C++ is renowned for its high performance and efficiency, making it ideal for applications that require real-time graphics and responsiveness. This performance advantage is particularly crucial in applications that require significant computation or rendering, such as games and graphics design software. The ability to write close-to-the-metal code means you can achieve higher frame rates and smoother UI interactions.
Cross-Platform Compatibility
Another significant benefit of using C++ for GUI development is its ability to create cross-platform applications. With libraries like Qt and wxWidgets, developers can write code once and deploy it across different operating systems without needing to change functionalities or appearances. This saves time and resources, allowing developers to reach broader audiences.
Rich Core Libraries
C++ boasts a rich set of core libraries that enhance GUI development capabilities. Frameworks such as Qt and wxWidgets come with built-in widgets, layout managers, and even advanced functionalities like animations and 3D graphics support, enabling developers to create feature-rich applications more efficiently.
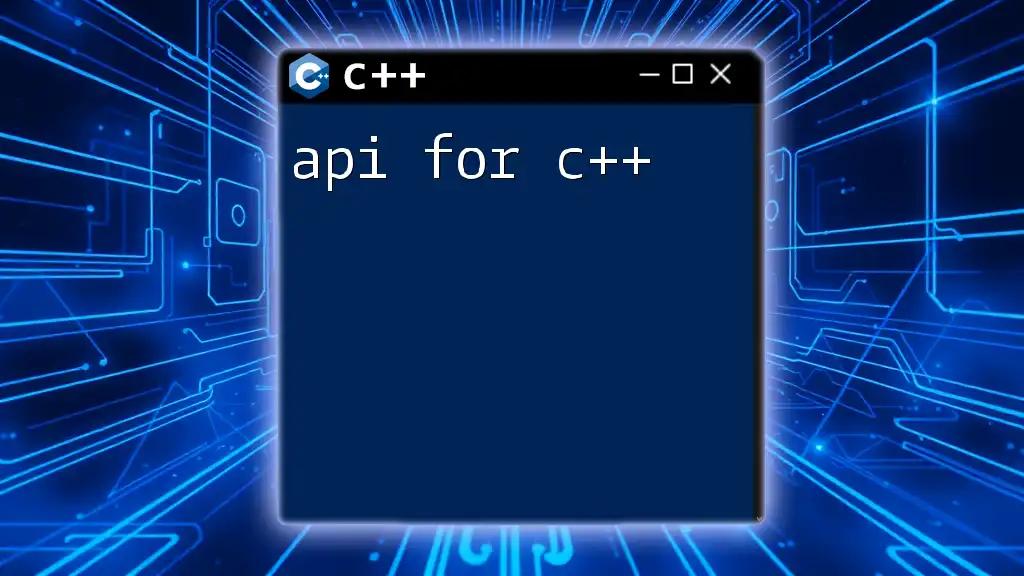
Overview of C++ GUI Libraries
There are several powerful libraries available for creating GUIs in C++. Here, we’ll discuss some of the most popular options, highlighting their unique features.
Qt
Qt is one of the most widely used frameworks for C++ GUI development. It is cross-platform, highly portable, and has a rich set of features for building complex interfaces.
Example of a simple window creation using Qt:
#include <QApplication>
#include <QWidget>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWidget window;
window.resize(400, 300);
window.setWindowTitle("Simple Qt Window");
window.show();
return app.exec();
}
This example demonstrates how to create a basic window using Qt. The `QApplication` class manages the GUI application's control flow and main settings, while `QWidget` represents a basic window.
wxWidgets
wxWidgets is another popular library that allows developers to create applications for multiple platforms. It gives a native look and feel on each platform while maintaining a common API.
Here’s an example demonstrating a basic wxWidgets application:
#include <wx/wx.h>
class MyApp : public wxApp {
public:
virtual bool OnInit();
};
class MyFrame : public wxFrame {
public:
MyFrame() : wxFrame(NULL, wxID_ANY, "Simple wxWidgets Window", wxDefaultPosition, wxSize(400, 300)) {}
};
bool MyApp::OnInit() {
MyFrame *frame = new MyFrame();
frame->Show(true);
return true;
}
wxIMPLEMENT_APP(MyApp);
In this snippet, we define a simple application that creates a robust window using wxWidgets.
GTK+
GTK+ is primarily used for creating applications on the GNOME desktop. Its focus on high-performance graphical applications makes it a strong contender for C++ GUI development, especially in Linux environments.
Here’s how you can create a simple button using GTK+:
#include <gtk/gtk.h>
static void on_button_clicked(GtkWidget *widget, gpointer data) {
g_print("Button clicked!\n");
}
int main(int argc, char *argv[]) {
gtk_init(&argc, &argv);
GtkWidget *window = gtk_window_new(GTK_WINDOW_TOPLEVEL);
GtkWidget *button = gtk_button_new_with_label("Click Me");
g_signal_connect(button, "clicked", G_CALLBACK(on_button_clicked), NULL);
gtk_container_add(GTK_CONTAINER(window), button);
gtk_widget_show_all(window);
gtk_signal_connect(window, "destroy", G_CALLBACK(gtk_main_quit), NULL);
gtk_main();
return 0;
}
In this example, a GTK+ window is created with a button that, when clicked, prints a message to the console.
FLTK
FLTK (Fast, Light Toolkit) is well-known for its lightweight nature and ease of use. It's an excellent choice for projects where performance and a small footprint are critical.
Here’s a straightforward example of a GUI application using FLTK:
#include <FL/Fl.H>
#include <FL/Fl_Window.H>
#include <FL/Fl_Button.H>
void button_callback(Fl_Widget *w, void *) {
Fl::success("Button clicked!");
}
int main() {
Fl_Window *window = new Fl_Window(400, 300, "Simple FLTK Window");
Fl_Button *button = new Fl_Button(160, 120, 80, 40, "Click Me");
button->callback(button_callback);
window->end();
window->show();
return Fl::run();
}
In this snippet, we create a simple FLTK window with a button that triggers a callback function when clicked, displaying a success message.
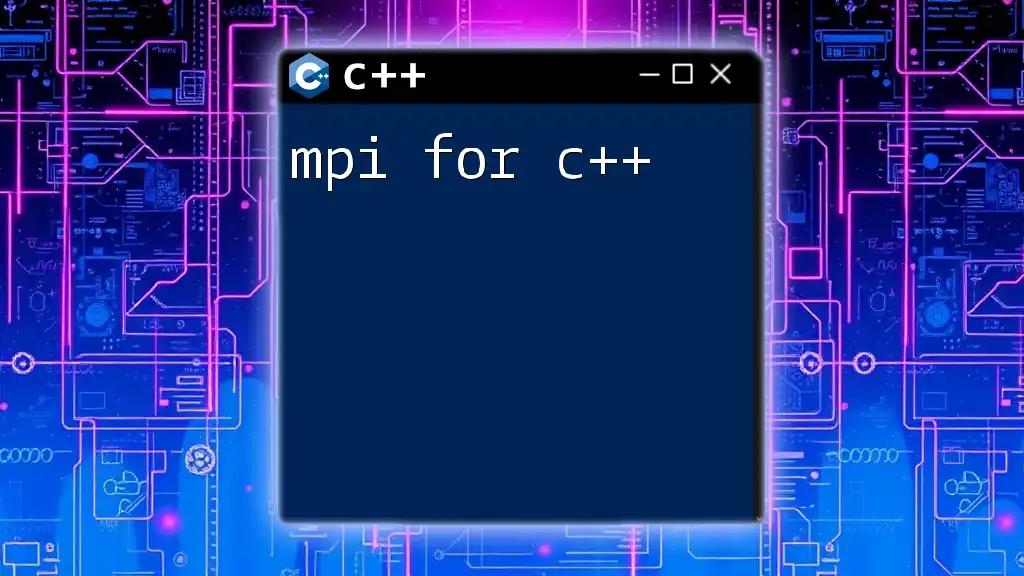
Setting Up Your C++ GUI Environment
Installing Necessary Libraries
To start developing GUIs with C++, you need to install the appropriate libraries. Here are some steps for popular libraries:
- Qt: Download and install the Qt Creator IDE, which includes the Qt framework.
- wxWidgets: Download the wxWidgets package from the official site and configure it in your IDE.
- GTK+: Depending on your OS, you can install GTK+ through package managers (e.g., `apt` for Ubuntu).
- FLTK: Download FLTK from its official site and follow the build instructions.
Configuring Your IDE for GUI Development
Most modern IDEs support these libraries. Here are some configuration tips:
- For Qt Creator, simply create a new project and select Qt Widgets Application.
- In Visual Studio, configure include and library paths via Project Properties.
- For Code::Blocks, ensure to set the search directories under compiler settings for your chosen library.
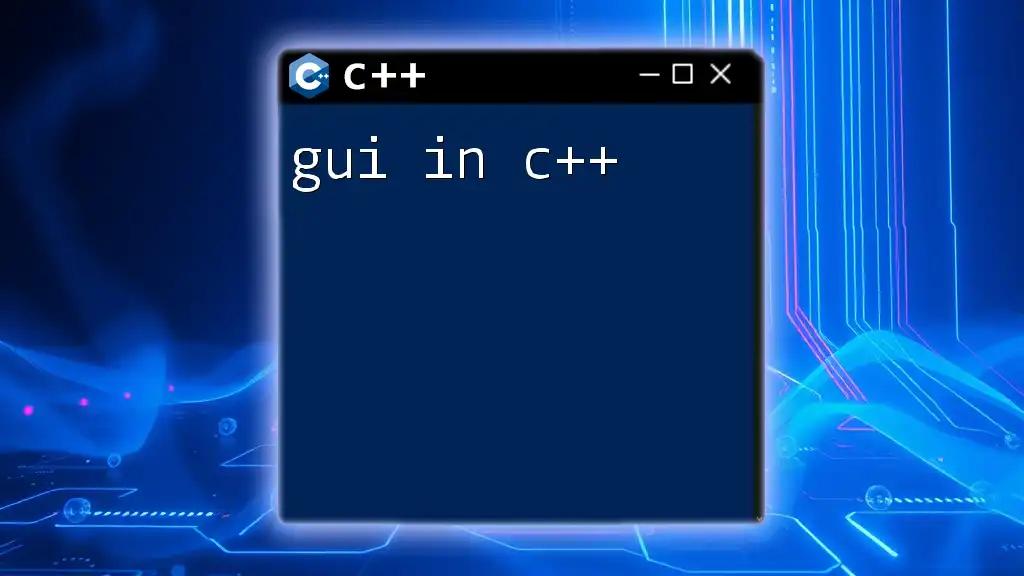
Creating Your First C++ GUI Application
Basic Window Example
Let's create a simple GUI application using Qt, where we display just a window. First, you'll need a basic setup:
#include <QApplication>
#include <QMainWindow>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QMainWindow window;
window.setWindowTitle("My First GUI Application");
window.resize(300, 200);
window.show();
return app.exec();
}
In the above example, we create a `QMainWindow` object that serves as the main window with a set title and size.
Adding Widgets and Handling Events
To create interactive applications, you need to implement various widgets and handle user inputs. Below is an example of adding a button to our Qt application:
#include <QApplication>
#include <QPushButton>
#include <QMessageBox>
void handleButton() {
QMessageBox::information(NULL, "Hello", "Button was clicked!");
}
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Click Me");
QObject::connect(&button, &QPushButton::clicked, handleButton);
button.resize(200, 100);
button.show();
return app.exec();
}
In this enhanced example, we implement a QPushButton. When the button is clicked, it triggers a message box displaying a customized message.
Layout Management
Creating an attractive GUI often requires organizing widgets efficiently. Layout managers help automatically position your GUI elements.
Here’s an example where we use a vertical layout to position buttons:
#include <QApplication>
#include <QPushButton>
#include <QVBoxLayout>
#include <QWidget>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWidget window;
QVBoxLayout *layout = new QVBoxLayout;
layout->addWidget(new QPushButton("Button 1"));
layout->addWidget(new QPushButton("Button 2"));
window.setLayout(layout);
window.setWindowTitle("Vertical Layout Example");
window.show();
return app.exec();
}
In this implementation, `QVBoxLayout` manages the positioning of two buttons vertically within the main window.
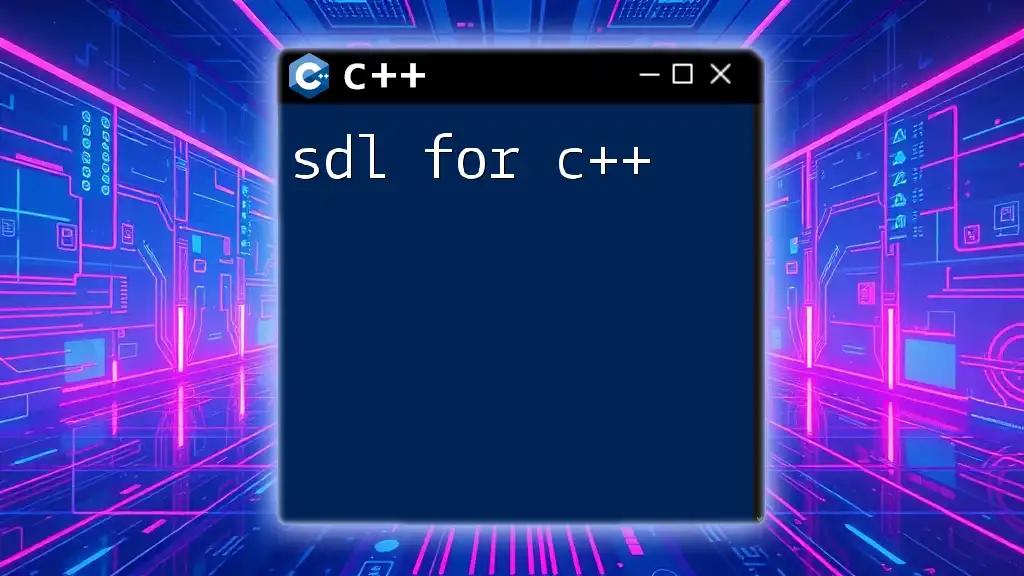
Advanced GUI Concepts
Custom Widgets
Custom widgets provide unique functionalities tailored for specific applications. You can subclass a widget to extend its behavior. Here’s a simple example where a custom widget displays a message:
#include <QWidget>
#include <QPainter>
class MessageWidget : public QWidget {
protected:
void paintEvent(QPaintEvent *) override {
QPainter painter(this);
painter.drawText(rect(), Qt::AlignCenter, "Custom Widget");
}
};
In this example, we create a `MessageWidget` class that overrides the `paintEvent` to display centered text.
The Model-View-Controller (MVC) Design Pattern
The MVC design pattern helps separate the application logic from the user interface. This pattern promotes code organization, making your application easier to maintain.
In a typical Qt model-view scenario, you can use `QAbstractListModel` for the data, `QListView` for the view, and interact with controllers through signals and slots. This architecture enhances the ability to modify your data without impacting the UI directly.
Multithreading in GUI Applications
To keep your GUI responsive, particularly during lengthy operations, multithreading is crucial. C++ threads can be integrated for executing background tasks, allowing the UI to remain interactive.
For instance, you can use `QThread` in Qt for this purpose:
#include <QApplication>
#include <QPushButton>
#include <QThread>
#include <QMessageBox>
class Worker : public QObject {
Q_OBJECT
public slots:
void doWork() {
// Simulate a long operation
QThread::sleep(3);
emit workDone();
}
signals:
void workDone();
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Start Long Operation");
QThread workerThread;
Worker worker;
button.connect(&button, &QPushButton::clicked, [&]() {
workerThread.start();
QMetaObject::invokeMethod(&worker, "doWork", Qt::QueuedConnection);
});
QObject::connect(&worker, &Worker::workDone, [&]() {
QMessageBox::information(nullptr, "Done", "Operation completed!");
workerThread.quit();
});
button.show();
return app.exec();
}
In this example, clicking the button starts a worker thread that simulates a long operation, keeping the GUI responsive. Once the task is complete, a message box is displayed.
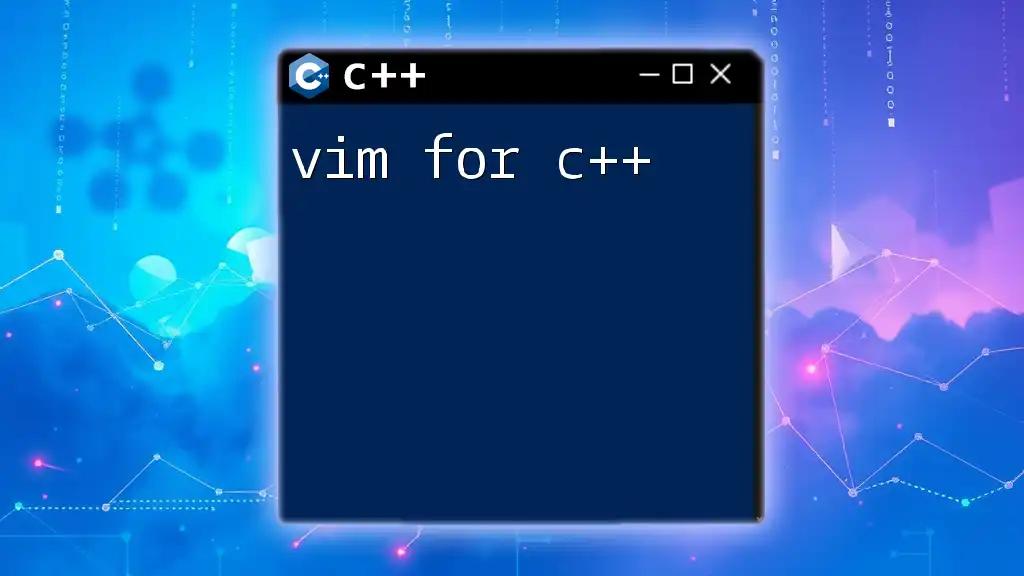
Testing and Debugging C++ GUIs
Testing a graphical application can be challenging due to its interactive nature. Here's how to tackle this:
- Unit Testing: Use frameworks like Google Test for non-UI logic.
- Functional Testing: Tools like Sikuli allow automation of GUIs based on images.
- Debugging Tools: Leverage integrated debugging tools within your IDE, such as breakpoints and watch variables, specifically for GUI interaction points like button clicks and load times.
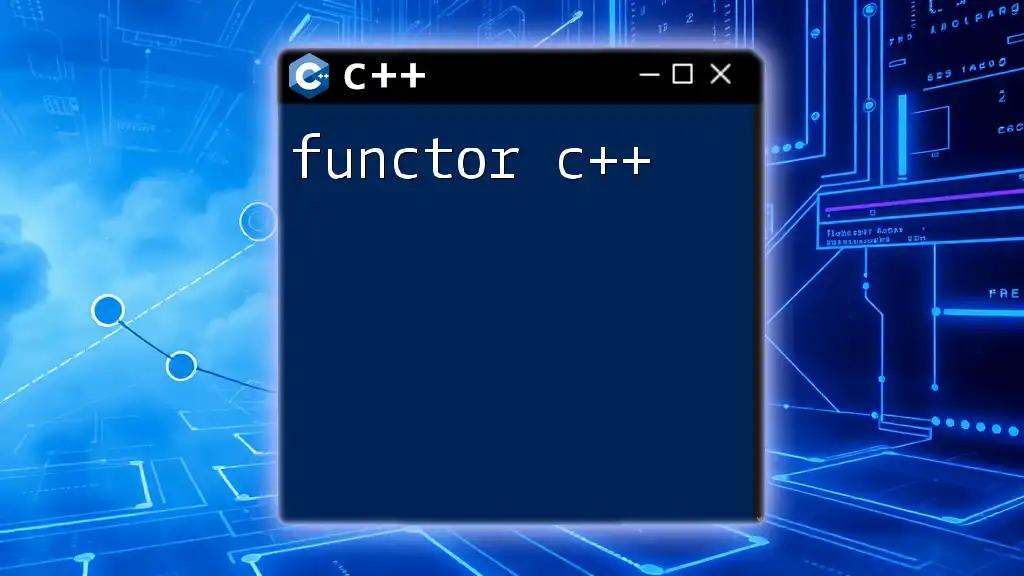
Resources and Further Learning
To enhance your knowledge of C++ GUI development, consider exploring these resources:
- Qt Documentation: The official Qt documentation provides comprehensive guides and examples.
- wxWidgets Documentation: Access wxWidgets tutorials and reference materials here.
- Books: Title recommendations include "C++ GUI Programming with Qt 5" and "Programming with wxWidgets."
- Online Courses: Platforms like Udemy and Coursera often have specialized courses on C++ GUI development.
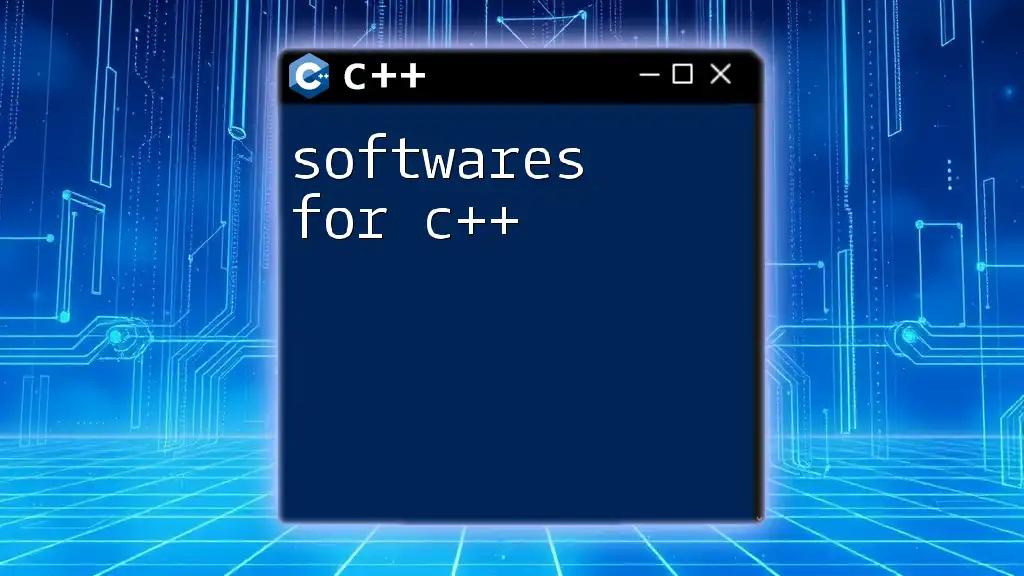
Conclusion
In conclusion, developing a GUI for C++ can be both rewarding and challenging. C++ offers significant performance advantages, cross-platform capabilities, and a variety of powerful libraries to assist in the development process. Whether you choose Qt, wxWidgets, GTK+, or FLTK, understanding the nuances of GUI design and management will allow you to create dynamic and engaging applications. Don't hesitate to explore these libraries and begin your journey in C++ GUI development today!