In C++, the `if` statement allows you to execute a block of code conditionally based on whether a specified expression evaluates to true or false.
Here's a simple example:
#include <iostream>
int main() {
int number = 10;
if (number > 5) {
std::cout << "Number is greater than 5." << std::endl;
}
return 0;
}
What is an `if` Statement?
An `if` statement is a fundamental component of C++ that allows you to implement conditional logic in your programs. It provides a way to execute certain blocks of code only if specific conditions are met, thereby enabling dynamic decision-making in your applications. This construct is essential for developing responsive applications that behave differently based on user input or other runtime conditions.
Basic Syntax of `if` Statements
The basic syntax of an `if` statement is straightforward:
if (condition) {
// code to execute if condition is true
}
- Condition: This is a boolean expression that resolves to true or false.
- The block of code inside the curly braces `{}` executes only if the condition evaluates to true.
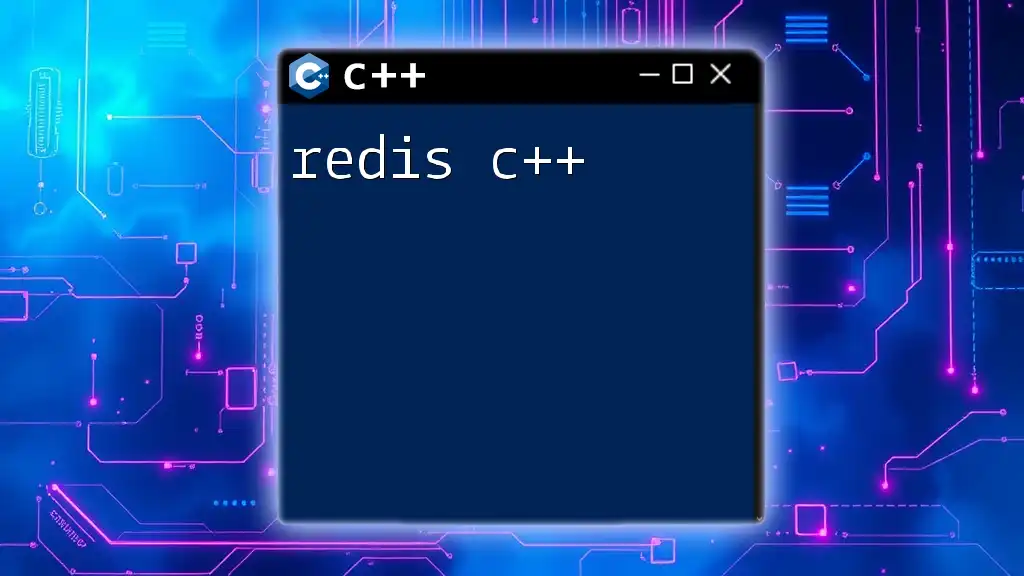
Understanding the Condition
What Makes a Valid Condition?
A valid condition in an `if` statement must be a boolean expression. Boolean expressions can involve comparisons and logical operations.
Comparative Operators in C++
C++ offers various comparative operators to evaluate conditions:
- `==`: checks if two values are equal.
- `!=`: checks if two values are not equal.
- `<`: checks if the left value is less than the right value.
- `>`: checks if the left value is greater than the right value.
- `<=`: checks if the left value is less than or equal to the right value.
- `>=`: checks if the left value is greater than or equal to the right value.
Here’s an example demonstrating comparison operators:
int a = 5;
int b = 10;
if (a < b) {
// This block executes because 5 is less than 10
std::cout << "a is less than b";
}
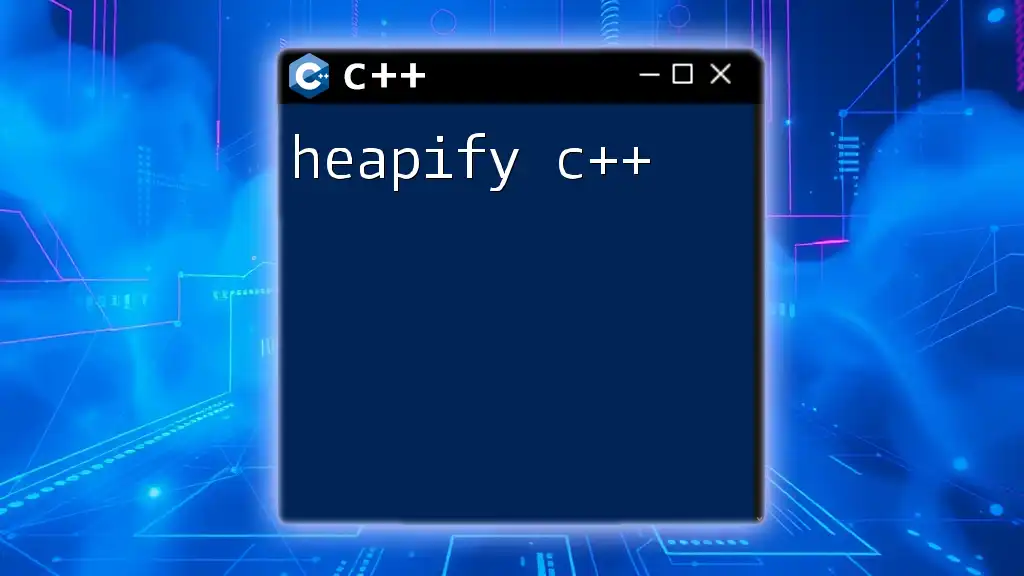
Using `if` Statements in Practice
Single `if` Statement
The single `if` statement checks a condition and executes a block of code if it is true. Here’s a simple example:
int age = 20;
if (age >= 18) {
std::cout << "You are an adult.";
}
`if-else` Statements
An `if-else` statement allows you to define an alternative block of code that runs when the condition is false. Here’s how it works:
int age = 16;
if (age >= 18) {
std::cout << "You are an adult.";
} else {
std::cout << "You are a minor.";
}
Use `if-else` when you need to execute different branches based on the condition evaluated.
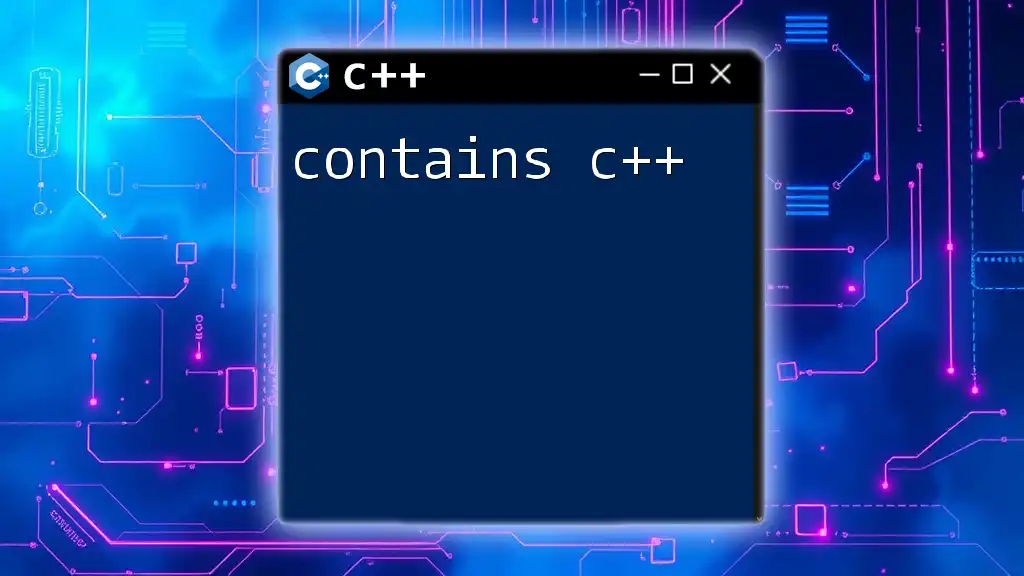
Complex Conditional Logic
Chaining Conditions with `else if`
Sometimes, you may have multiple conditions to check. The `else if` construct allows for this chaining:
int score = 85;
if (score >= 90) {
std::cout << "Grade: A";
} else if (score >= 80) {
std::cout << "Grade: B";
} else {
std::cout << "Grade: C or below";
}
Nested `if` Statements
Nested `if` statements can be used when you need one condition to be checked only if another condition is true. For example:
int x = 10;
if (x > 5) {
std::cout << "x is greater than 5.";
if (x > 8) {
std::cout << "x is also greater than 8.";
}
}
While powerful, nested `if` statements` can make code harder to read, so it’s essential to manage their structure carefully.
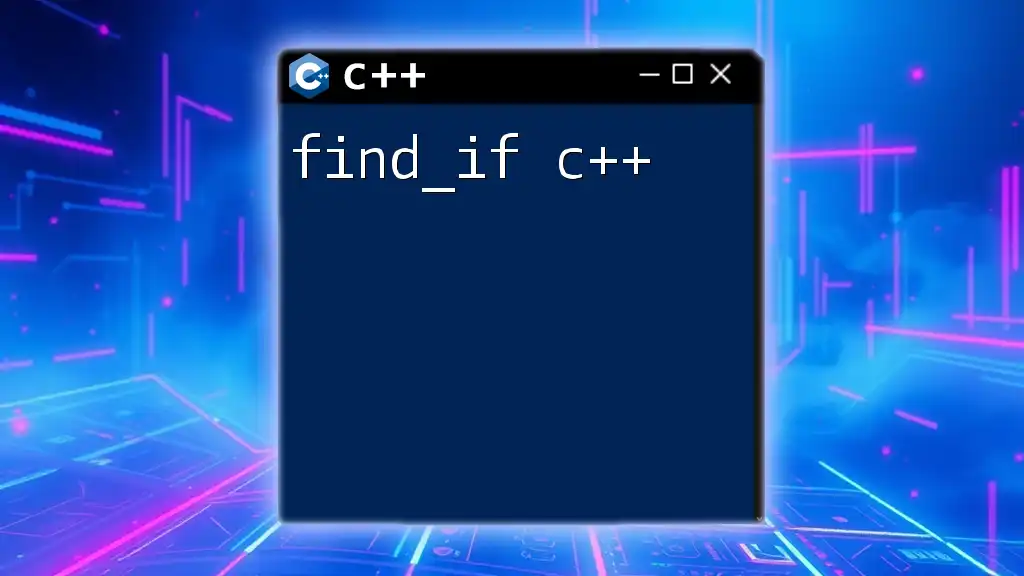
Logical Operators with `if` Statements
Using Logical Operators
Logical operators allow you to combine multiple conditions within an `if` statement. The primary logical operators include:
- `&&` (AND): True if both conditions are true.
- `||` (OR): True if at least one condition is true.
- `!` (NOT): Reverses the boolean value.
For example:
int a = 5;
int b = 10;
if (a < 10 && b > 5) {
std::cout << "Both conditions are true.";
}
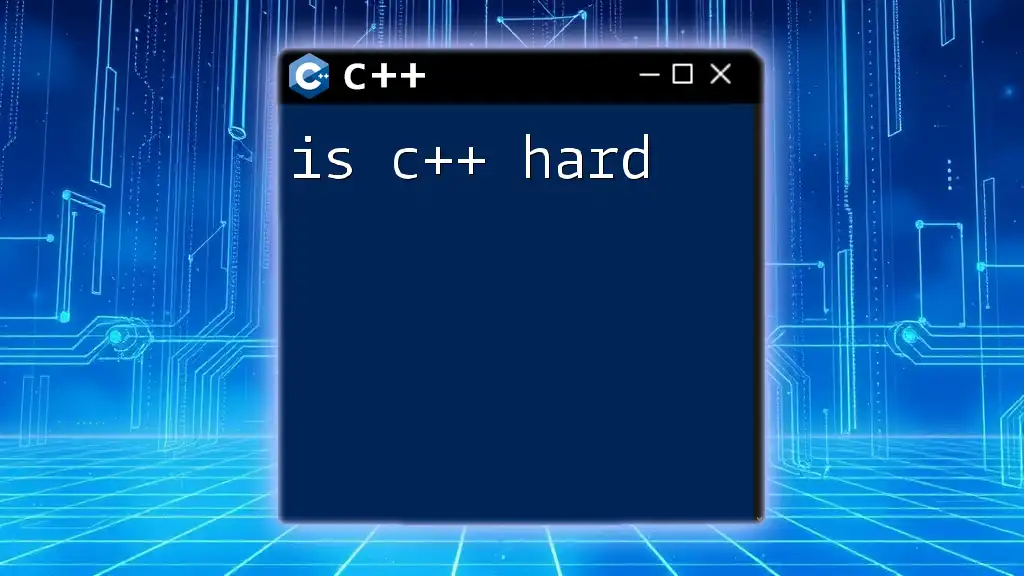
Common Pitfalls and Best Practices
Avoiding Logical Mistakes
A frequent error in C++ is confusing the assignment operator `=` with the equality operator `==`. This can lead to misleading results in condition checking. Always double-check that you're using the correct operator. Here’s an example that illustrates the mistake:
int x = 10;
if (x = 5) { // Mistake: should be x == 5
std::cout << "This will always execute!";
}
Writing Clear and Maintainable Code
To maintain readability and clarity in your code, consider the following best practices:
- Use meaningful variable names and include comments to clarify complex logic.
- Keep conditions simple and avoid unnecessary nesting.
- Use consistent indentation to clearly show the structure of your conditionals.
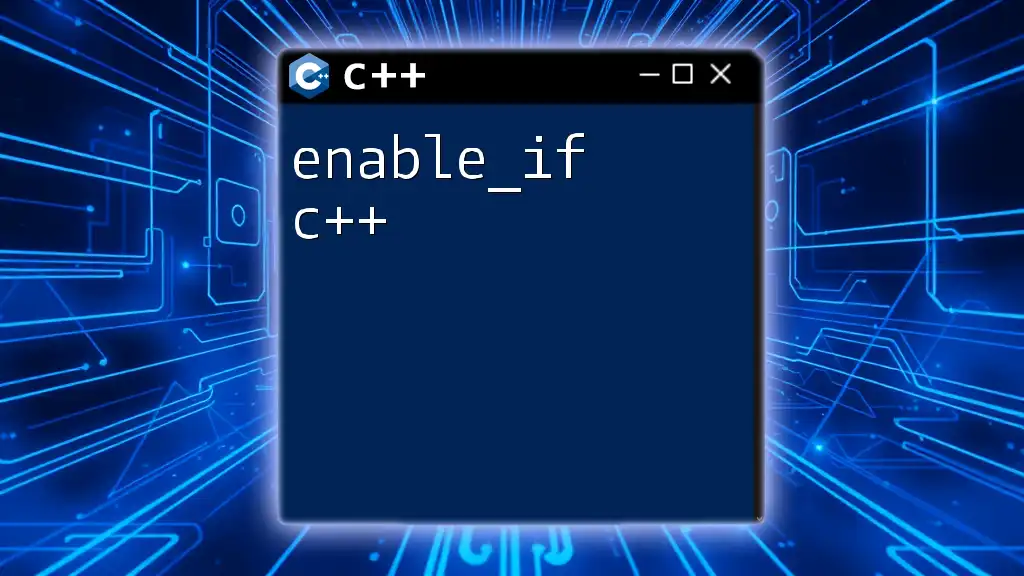
Real-world Applications of `if` Statements
Examples from Day-to-Day Programming
`If` statements can be used in numerous real-world applications such as:
- User Input Validation: Checking if a user enters valid data.
- Conditional Messages: Providing feedback based on user actions.
For instance:
std::string userInput;
std::cout << "Enter your age: ";
std::cin >> userInput;
if (std::stoi(userInput) >= 18) {
std::cout << "Access granted.";
} else {
std::cout << "Access denied.";
}
Understanding Control Flow in Larger Applications
Within larger applications, `if` statements serve as decision points that steer the flow of execution based on varying inputs. Mastery of `if` statements is essential as they are foundational to understanding how to create dynamic and interactive programs.
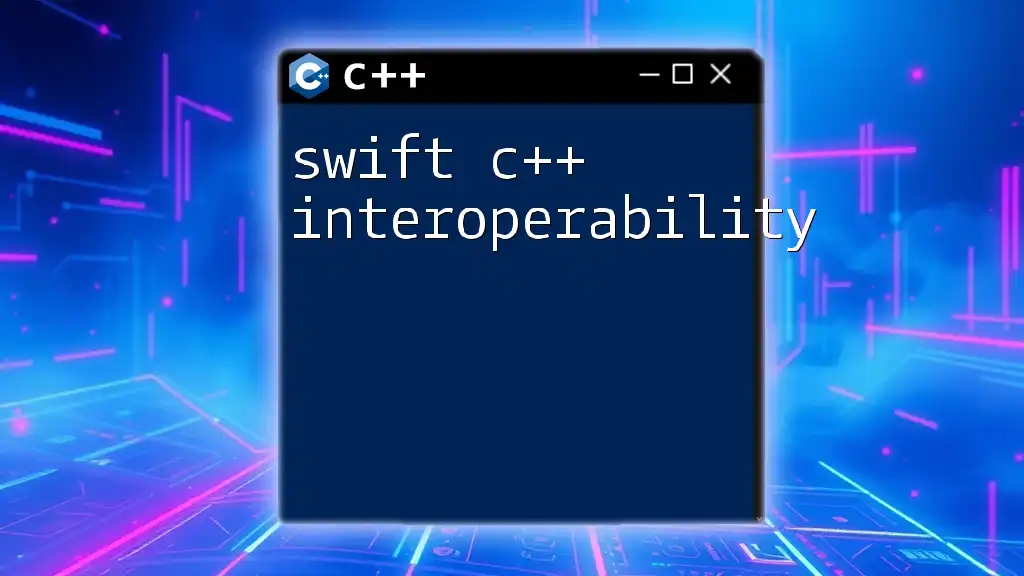
Conclusion
In this comprehensive guide, we have explored the pivotal role of `if` statements in C++, including their syntax, various forms, and practical applications. Understanding how to use `if` statements effectively will empower you to develop more responsive and intelligent applications.
Encouragement to Experiment
Don’t hesitate to experiment with your own conditions and code samples. The best way to learn `if` statements is through practice. Dive into your projects, play with the code, and see what creative solutions you can implement using this powerful feature of C++.
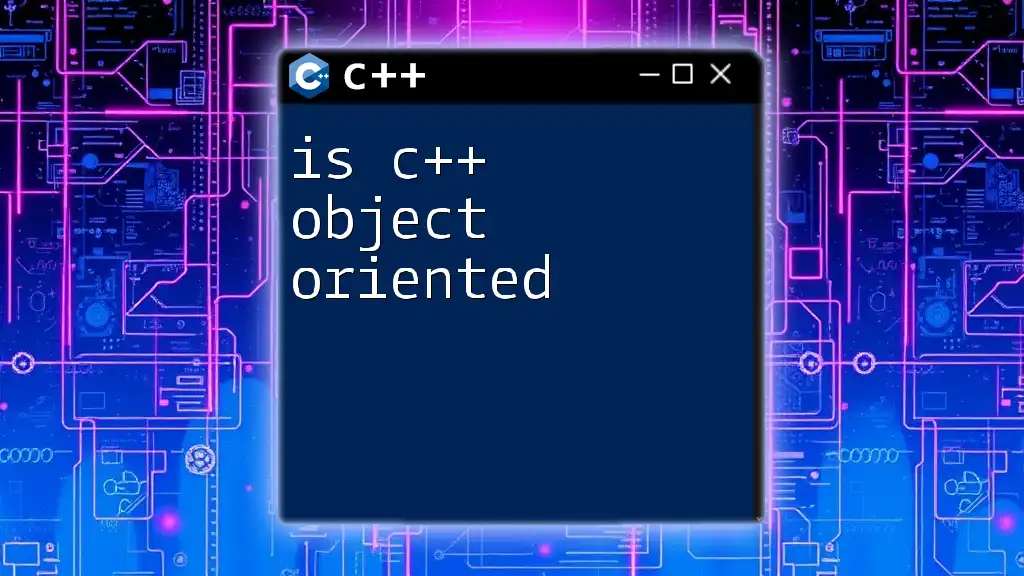
Additional Resources
To further enhance your understanding of `if` statements and C++ programming, consider checking out the following:
- Books: Look for introductory texts on C++ programming.
- Websites: Explore resources like C++ documentation and online coding platforms for practice exercises.
- Community and Forums: Engage with the programming community on platforms like Stack Overflow, where you can ask questions and share knowledge with fellow learners.