Yes, C++ is an object-oriented programming language that allows the creation of classes and objects to encapsulate data and functions.
Here’s a simple code snippet demonstrating a class in C++:
class Dog {
public:
void bark() {
std::cout << "Woof! Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
Understanding Object-Oriented Programming (OOP)
Definition of OOP
Object-Oriented Programming (OOP) is a programming paradigm characterized by the concept of “objects,” which can contain data in the form of fields (often known as attributes or properties) and code in the form of procedures (often known as methods). The purpose of OOP is to increase the flexibility and maintainability of code by structuring software in a way that models real-world entities and their interactions.
Core Principles of OOP
Understanding OOP principles is essential to grasp why C++ is considered an object-oriented language. Here are the four core principles:
Encapsulation
Encapsulation is the bundling of data with the methods that operate on that data. This principle restricts direct access to some of the object’s components, which can prevent the accidental modification of data.
Here is a simple example in C++:
class BankAccount {
private:
double balance; // Private attribute
public:
BankAccount(double initialBalance) : balance(initialBalance) {}
void deposit(double amount) {
balance += amount; // Method to modify private data
}
double getBalance() {
return balance; // Method to access private data
}
};
Inheritance
Inheritance is a mechanism that allows one class (derived class) to acquire the properties and methods of another class (base class). This promotes code reusability.
For example:
class Animal {
public:
void eat() {
std::cout << "Eating..." << std::endl;
}
};
class Dog : public Animal { // Dog inherits from Animal
public:
void bark() {
std::cout << "Barking..." << std::endl;
}
};
Polymorphism
Polymorphism allows methods to do different things based on the object it is acting upon. It can be achieved through function overloading and function overriding.
An example:
class Shape {
public:
virtual void draw() {
std::cout << "Drawing a shape." << std::endl;
}
};
class Circle : public Shape {
public:
void draw() override { // Override base class method
std::cout << "Drawing a circle." << std::endl;
}
};
Abstraction
Abstraction is the concept of hiding complex realities while exposing only the necessary parts. It helps in reducing programming complexity.
You might use abstract classes in C++ like this:
class AbstractEmployee {
public:
virtual void askForPromotion() = 0; // Pure virtual function
};
class Employee : public AbstractEmployee { // Inherited class
public:
void askForPromotion() override {
std::cout << "Promoted!" << std::endl;
}
};
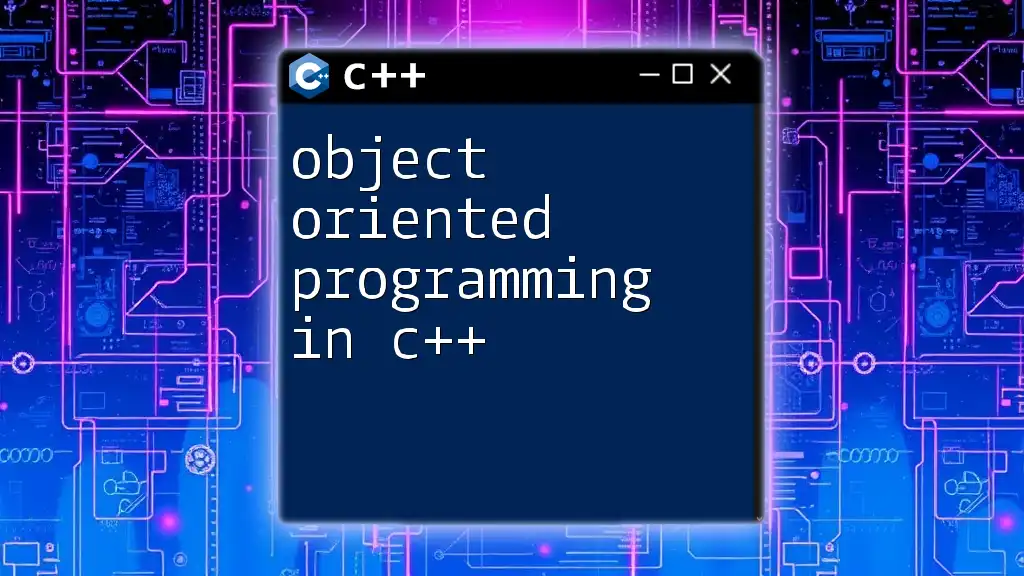
Is C++ an Object-Oriented Language?
C++ was designed with object-oriented programming in mind, making it an exemplary language for OOP. However, it is essential to understand how it integrates OOP concepts into its design.
C++ vs. Other OOP Languages
While C++ is often compared to other popular OOP languages such as Java, Python, and C#, it offers unique advantages like greater control over system resources and memory management. This multi-paradigm nature means that C++ supports not only OOP but also procedural and generic programming, granting developers more flexibility.
Features that Support OOP in C++
Some of the key features in C++ further highlight that C++ is object-oriented, namely:
Classes and Objects
Classes are blueprints for creating objects. In C++, you can define a class and instantiate an object, as shown below:
class Car {
public:
std::string brand;
std::string model;
Car(std::string b, std::string m) : brand(b), model(m) {}
};
Car myCar("Toyota", "Corolla");
Access Specifiers
C++ provides three access specifiers to control access levels to class members: private, public, and protected. This feature is pivotal in encapsulating data.
class Student {
private:
int age;
public:
void setAge(int a) {
age = a; // Access via public method
}
int getAge() {
return age;
}
};
Constructors and Destructors
While creating an object, constructors are called for initialization, and destructors manage resource cleanup when an object goes out of scope. Here’s an example:
class Book {
public:
Book() {
std::cout << "A book is created." << std::endl;
}
~Book() {
std::cout << "A book is destroyed." << std::endl;
}
};
Operator Overloading
C++ permits operators to be redefined to work with user-defined types, enhancing the language's expressive power.
class Complex {
public:
double real, imag;
Complex operator + (const Complex& other) {
return Complex{real + other.real, imag + other.imag};
}
};
Complex c1{1.0, 2.0}, c2{2.0, 3.0};
Complex result = c1 + c2; // Adds two complex numbers
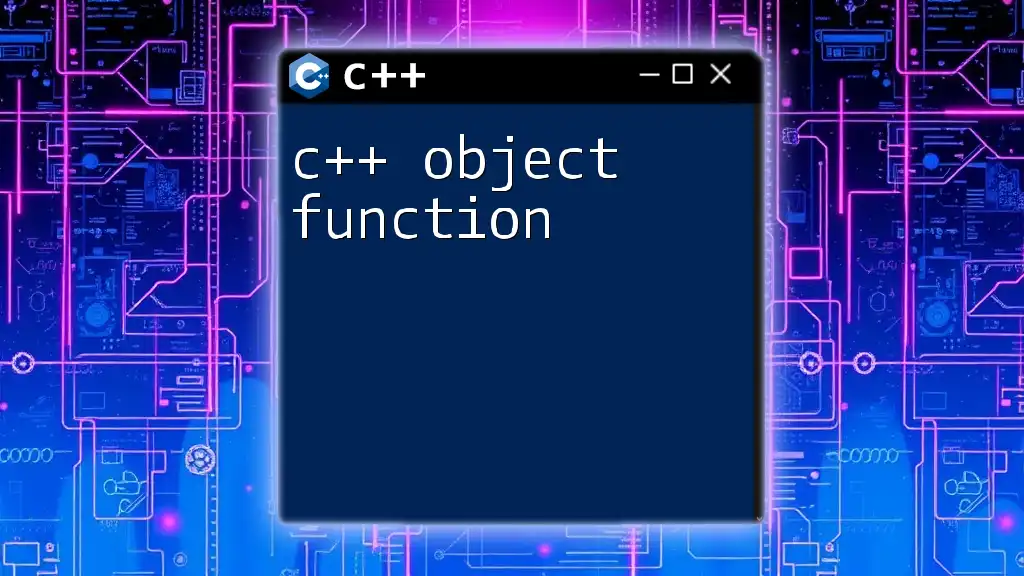
Practical Use Cases of OOP in C++
Real-World Applications
OOP is heavily utilized in real-world applications across various domains:
- Game Development: OOP facilitates the design of intricate game systems, enabling developers to create diverse types of characters through inheritance and polymorphism.
- Software Engineering: It promotes scalable and maintainable software development. By using classes and objects, large systems can be broken down into manageable pieces.
- GUI Applications: User interface design greatly benefits from OOP principles, allowing the creation of modular components that can be reused and extended.
Common Misconceptions About C++ and OOP
A prevalent misconception is that C++ is not a purely object-oriented language because it allows procedural programming. While it does support this paradigm, the presence of OOP features solidifies its position as an OOP language. Concrete examples show how developers can effectively use OOP while harnessing the procedural aspects when needed, making C++ versatile.
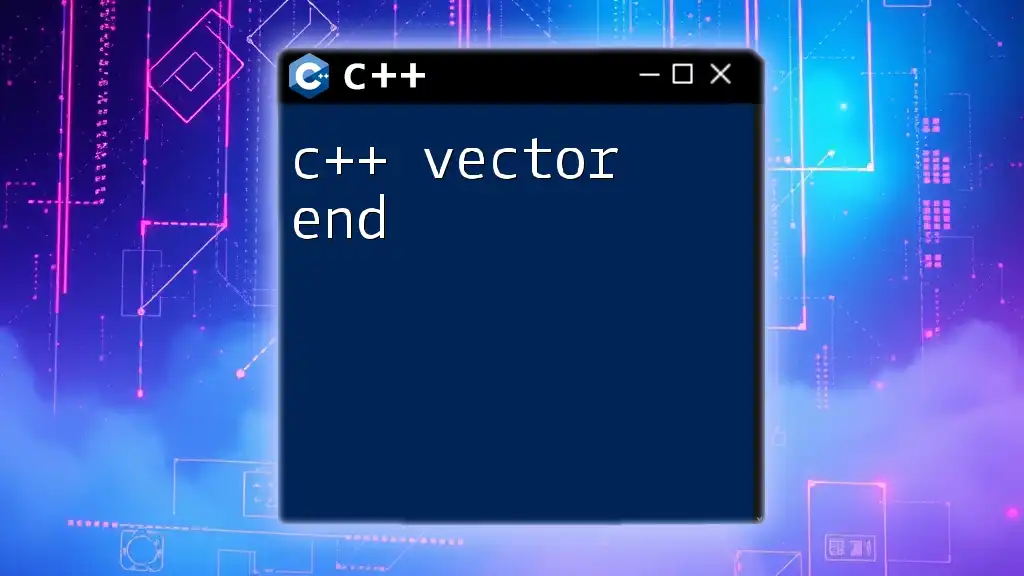
Challenges and Criticism of OOP in C++
Complexity of OOP Concepts
For beginners, OOP concepts can be difficult to grasp, leading to increased complexity. It takes time and experience to understand and implement these principles effectively.
Performance Considerations
While the advantages of OOP are clear, certain trade-offs exist. Object-oriented designs may introduce overhead in terms of memory and processing, particularly in performance-critical applications. For example, in some scenarios, procedural programming might provide better efficiency.
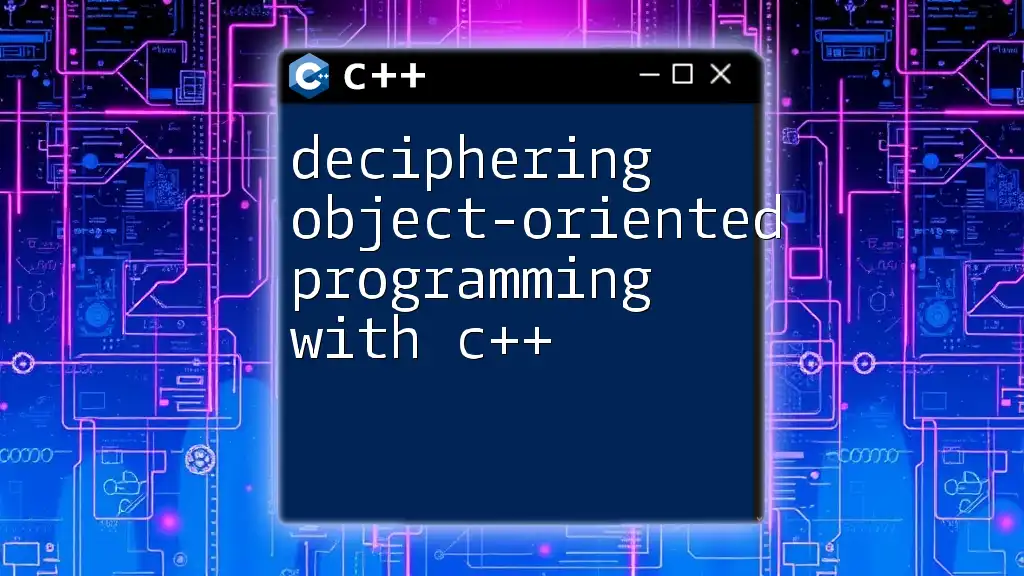
Conclusion
In summary, C++ is fundamentally an object-oriented programming language equipped with robust features that support OOP principles like encapsulation, inheritance, polymorphism, and abstraction. By leveraging these principles, developers can create scalable, reusable, and maintainable code. Mastering these concepts enhances your programming capability and empowers you to tackle complex problems effectively. As you continue on your programming journey, remember the significance of embracing OOP principles in C++.
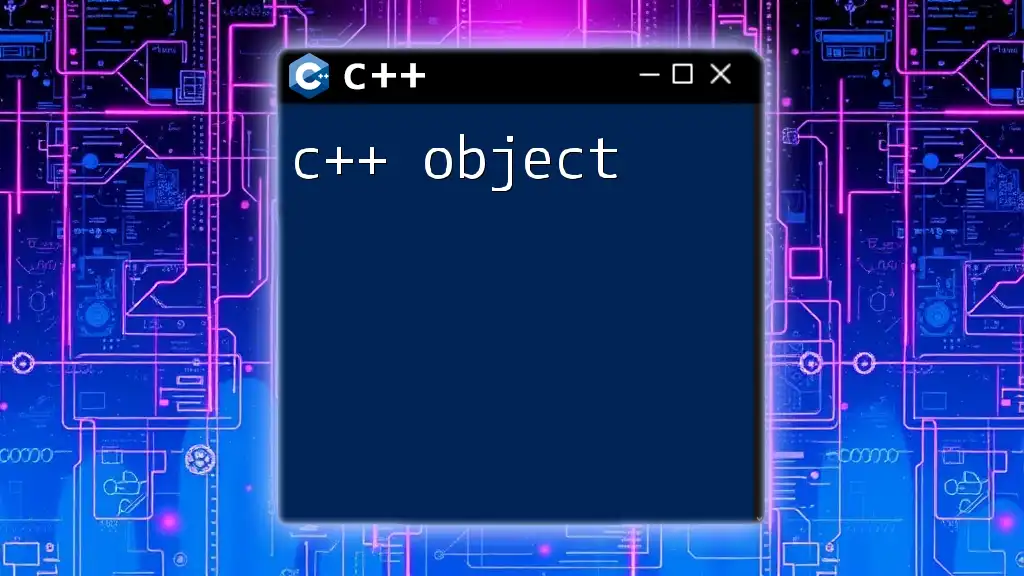
Call to Action
If you’re eager to deepen your understanding of C++ and object-oriented programming, consider enrolling in our upcoming courses designed to enhance your skills in a quick, short, and concise manner. Your path to mastering C++ commands and OOP principles begins here!