Object-oriented programming in C++ is a paradigm that allows developers to create classes that encapsulate data and functions, fostering code reuse and modularity.
class Circle {
public:
Circle(double r) : radius(r) {}
double area() { return 3.14 * radius * radius; }
private:
double radius;
};
int main() {
Circle myCircle(5.0);
std::cout << "Area: " << myCircle.area() << std::endl;
return 0;
}
Key Concepts of Object-Oriented Programming
Classes and Objects
Defining a Class
In C++, a class serves as a blueprint for creating objects. A class encapsulates data for the object and methods that operate on that data. It consists of attributes (data members) and methods (member functions).
Example: Basic Class Definition
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
In this example, we define a class named `Dog` with a public method `bark()`. When called, this method will print "Woof!" to the console.
Creating Objects
Once a class is defined, you can create objects (instances) of that class. This is done using the class name followed by the object name.
Example: Creating an Object
Dog myDog;
myDog.bark(); // Output: Woof!
Here, `myDog` is an instance of the `Dog` class, and calling its `bark()` method produces the expected output.
Encapsulation
What is Encapsulation?
Encapsulation is a fundamental principle of OOP that involves grouping data and the methods that operate on that data within a single unit, or class. This limits exposure to the internal workings of that class, thereby protecting its state from unintended interference.
Access Modifiers
C++ uses access modifiers to set the visibility of class members. The three main types of access modifiers are public, private, and protected:
- Public members are accessible from outside the class.
- Private members are only accessible within the class.
- Protected members are accessible within the class and by derived classes.
Example: Demonstrating Access Modifiers
class BankAccount {
private:
double balance;
public:
void deposit(double amount) {
balance += amount;
}
double getBalance() {
return balance;
}
};
In this example, `balance` is a private member, meaning it cannot be accessed directly from outside the `BankAccount` class. Instead, the `deposit()` and `getBalance()` methods provide controlled access.
Abstraction
Understanding Abstraction
Abstraction focuses on hiding the complex implementation details and showing only the essential features of an object. In C++, this can be achieved through abstract classes and interfaces.
Abstract Classes and Interfaces
An abstract class is a class that cannot be instantiated and usually contains at least one pure virtual function. This establishes a contract that derived classes must follow.
Example: Creating an Abstract Class
class Shape {
public:
virtual void draw() = 0; // Pure virtual function
};
The `Shape` class serves as an abstract base class that defines a `draw()` function without providing an implementation. Any derived class will have to implement this function.
Inheritance
What is Inheritance?
Inheritance is a mechanism in OOP that allows a new class (derived class) to inherit properties and behaviors (methods) from an existing class (base class). This promotes code reusability and establishes a natural hierarchy.
Types of Inheritance
C++ supports various types of inheritance:
- Single Inheritance: A class inherits from one base class.
- Multiple Inheritance: A class inherits from multiple base classes.
- Hierarchical Inheritance: Multiple classes inherit from one base class.
- Multilevel Inheritance: A class derives from a base class, which itself has a derived class.
Example: Inheriting a Class
class Animal {
public:
void eat() {
std::cout << "Eating..." << std::endl;
}
};
class Cat : public Animal {
public:
void meow() {
std::cout << "Meow!" << std::endl;
}
};
In this example, `Cat` inherits from `Animal`, acquiring its `eat()` method. Thus, an instance of `Cat` can both eat and meow.
Polymorphism
Understanding Polymorphism
Polymorphism allows methods to do different things based on the object it is acting upon, even though they share the same name. This feature is incredibly powerful in OOP, particularly when used with inheritance.
Compile-Time vs Run-Time Polymorphism
- Compile-Time Polymorphism is achieved through method overloading and operator overloading. It occurs when the function to be invoked is determined during compile time.
- Run-Time Polymorphism is achieved through method overriding. It occurs when the function to be invoked is determined at runtime through a virtual function.
Example: Achieving Polymorphism with Function Overloading
class Print {
public:
void display(int i) {
std::cout << "Integer: " << i << std::endl;
}
void display(double d) {
std::cout << "Double: " << d << std::endl;
}
};
Here, the `Print` class has two `display()` methods, each of which accepts different parameter types. The correct method is resolved at compile time based on the argument passed.
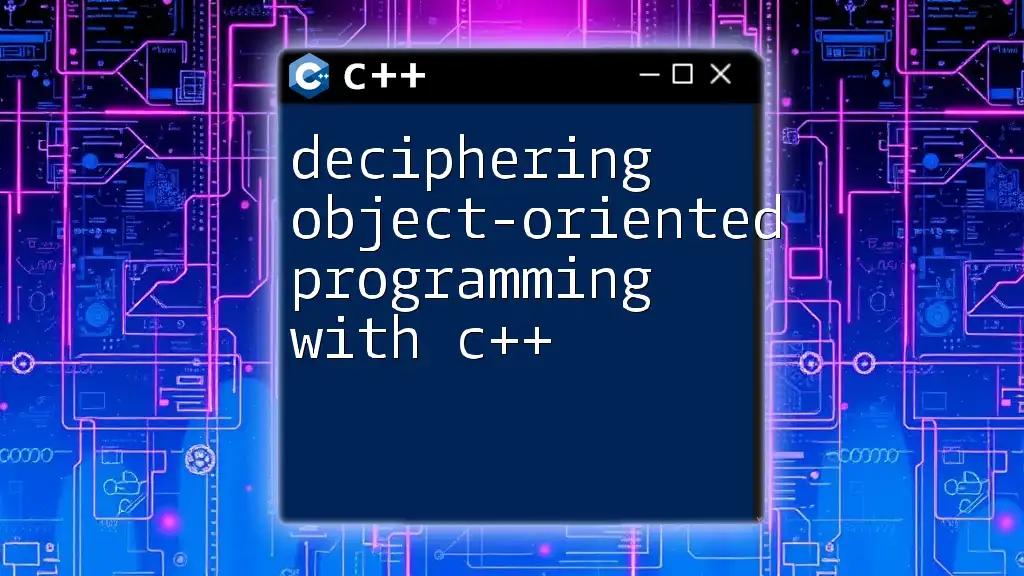
C++ OOP Features
Constructors and Destructors
What are Constructors?
Constructors are special member functions that initialize objects of a class. They have the same name as the class and do not return any value.
Destructors in C++
Destructors are also special member functions that are called when an object goes out of scope or is explicitly deleted. They help perform necessary clean-up tasks.
Example: Using Constructors and Destructors
class Car {
public:
Car() {
std::cout << "Car created." << std::endl;
}
~Car() {
std::cout << "Car destroyed." << std::endl;
}
};
In this example, when an object of type `Car` is created, the constructor outputs "Car created." When the object is destroyed, the destructor displays "Car destroyed."
Operator Overloading
Understanding Operator Overloading
Operator overloading allows you to redefine the functionality of operators (like +, -, *, etc.) for user-defined types. This makes your custom classes easier to use, mimicking the behavior of built-in types.
Examples of Operator Overloading
Example: Overloading the '+' Operator
class Complex {
public:
double real;
double imag;
Complex operator + (const Complex &obj) {
Complex temp;
temp.real = real + obj.real;
temp.imag = imag + obj.imag;
return temp;
}
};
In this example, we've overloaded the `+` operator to add two `Complex` numbers. This allows intuitive use of the `+` operator with instances of `Complex`.
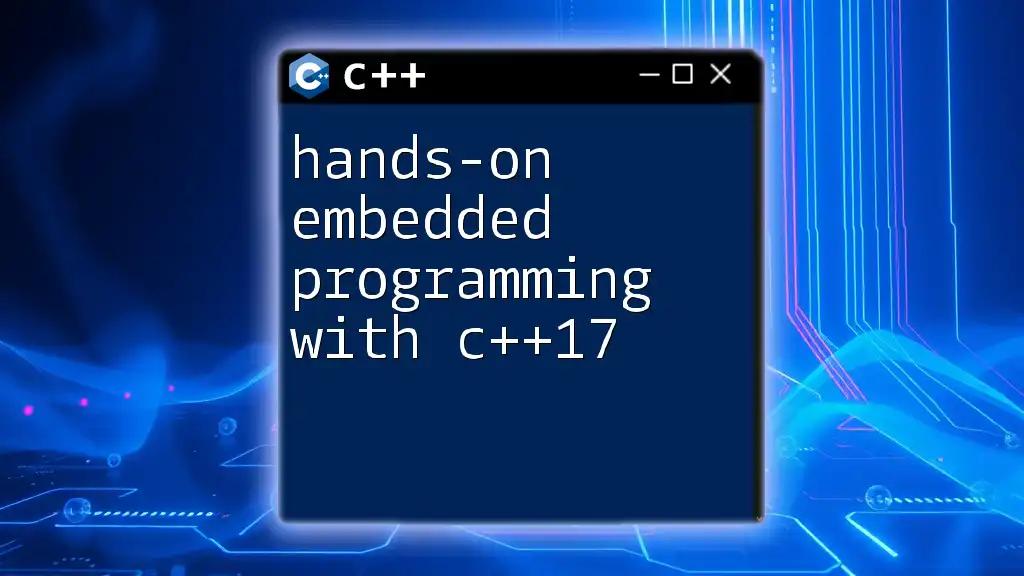
Benefits of Object-Oriented Programming in C++
Code Reusability
One of the most significant advantages of OOP is code reusability. You can reuse existing classes to create new ones, which reduces redundancy and errors while increasing efficiency.
Improved Software Maintainability
OOP organizes code into modular structures, making it easier to manage and update. Changes can frequently be made to a class without affecting other parts of the program, which leads to enhanced maintainability.
Enhanced Collaboration
By promoting a clear structure through encapsulation and abstraction, multiple developers can work on different components of a project simultaneously without interfering with one another's work.
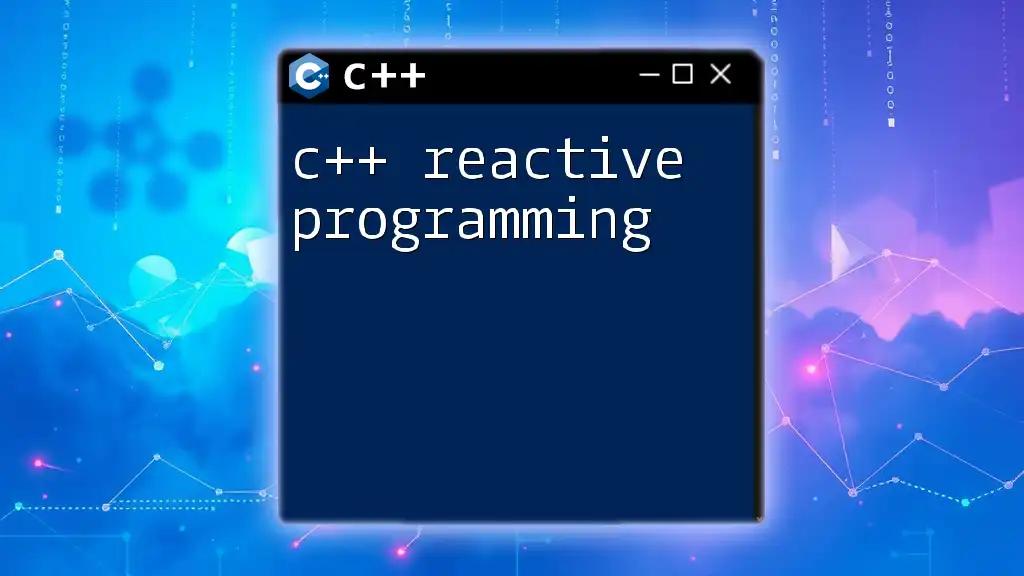
Common OOP Mistakes to Avoid
- Not Using Constructors Properly: Constructors are essential for initializing objects, and neglecting them can lead to uninitialized data members.
- Neglecting Access Modifiers: Overexposing class members can lead to unintended modifications, breaking encapsulation.
- Creating Too Many Classes: While encapsulation is vital, creating too many classes can lead to complexity and code bloat.
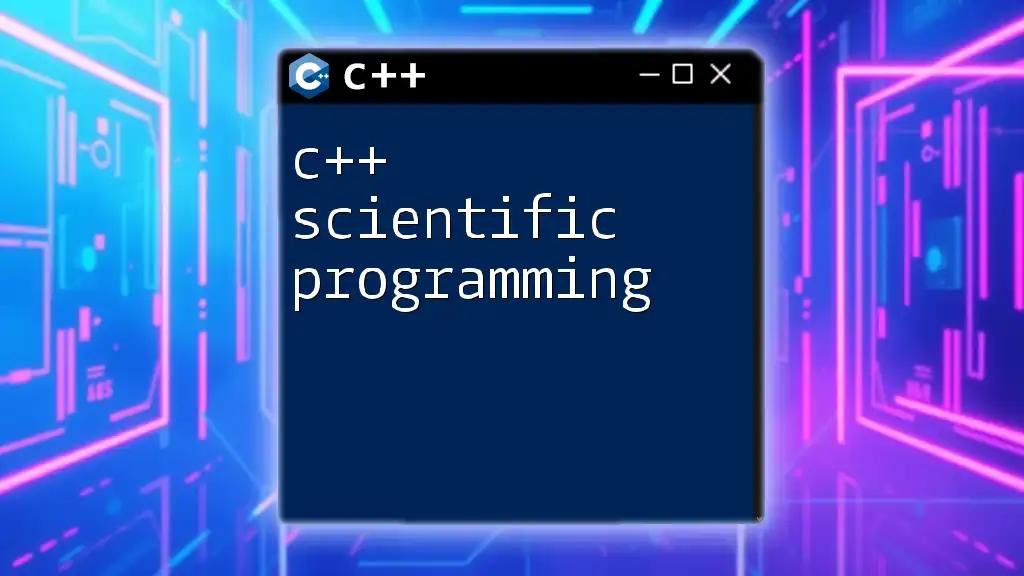
Conclusion
In conclusion, understanding object-oriented programming in C++ is essential for any budding C++ developer. By grasping the concepts of classes, encapsulation, abstraction, inheritance, and polymorphism, you are equipped to write modular, maintainable, and efficient code. Practice these concepts in your projects to see their true benefits in action.
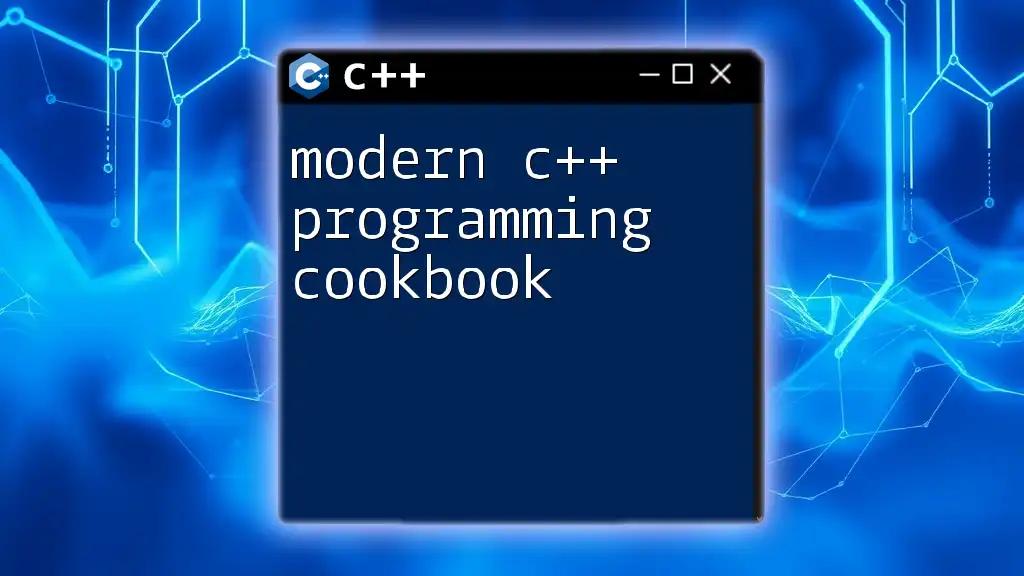
Call to Action
Join our community for upcoming workshops where you can deepen your understanding of C++, and follow us on social media to stay connected and updated on the latest in programming education!