Hands-on embedded programming with C++17 involves utilizing modern language features to efficiently develop software for resource-constrained devices, such as microcontrollers.
Here's a simple example that demonstrates how to set up a basic embedded program using C++17 to toggle an LED:
#include <Arduino.h> // Include the Arduino framework
void setup() {
pinMode(LED_BUILTIN, OUTPUT); // Initialize the built-in LED pin as an output
}
void loop() {
digitalWrite(LED_BUILTIN, HIGH); // Turn the LED on
delay(1000); // Wait for a second
digitalWrite(LED_BUILTIN, LOW); // Turn the LED off
delay(1000); // Wait for a second
}
What is Embedded Programming?
Embedded programming is the specialized form of software development that focuses on creating software for embedded systems—devices that are not traditionally considered computers but instead integrate computing capabilities into other systems. These systems include everything from household appliances like washing machines to sophisticated automotive controls and industrial machinery.
The significance of embedded programming stems from its widespread applications. With the rapid expansion of the Internet of Things (IoT), the demand for efficient, responsive, and reliable embedded software is greater than ever. It combines hardware and software engineering to produce devices that perform specific tasks, often with constraints related to performance, memory, and power consumption.
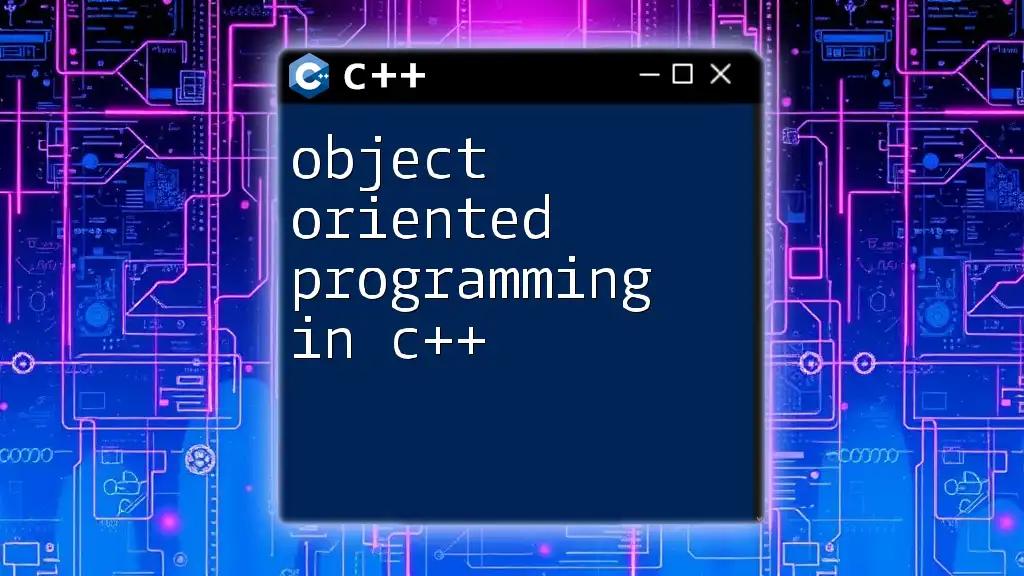
Why C++17 for Embedded Systems?
C++17 presents a robust toolkit for embedded programming, mainly due to its object-oriented and generic programming capabilities, which facilitate the creation of reusable and maintainable code. Key advantages include:
- Performance: C++ allows for low-level memory management while providing high-level abstractions.
- Resource Efficiency: Features like smart pointers enable better resource management, crucial for constrained environments.
- Modern Language Features: C++17 introduces enhancements like `constexpr if` and inline variables, making code cleaner and easier to maintain.
These features collectively make C++17 a strong candidate for modern embedded programming tasks.
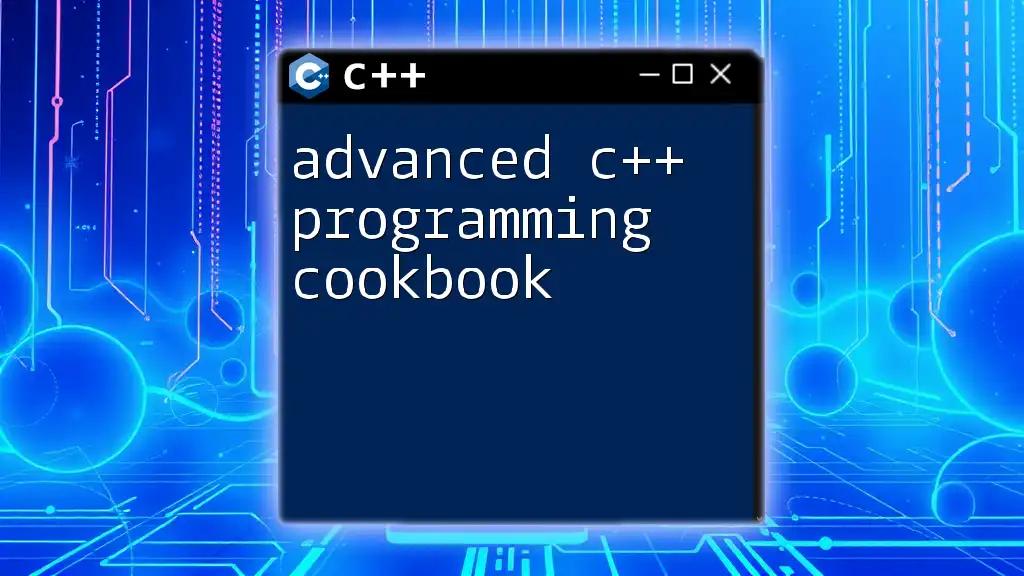
Getting Started with C++17 for Embedded Programming
Setting Up Your Development Environment
Setting up an effective development environment is vital for productive embedded programming. Here are some recommended IDEs and text editors:
- IDE Options: Visual Studio, Eclipse, or CLion.
- Text Editors: VSCode and Sublime Text for lightweight editing.
Cross-compilation tools are essential for executing code on target hardware. Tools like GCC ARM or PlatformIO give you the flexibility to compile your code for various microcontrollers seamlessly. Document the setup processes in detail, ensuring to include necessary configurations and installation steps.
Choosing the Right Hardware
When diving into hands-on embedded programming with C++17, hardware choice plays a pivotal role. Some popular microcontroller options include:
- Arduino: Ideal for beginners and simple projects.
- Raspberry Pi: Greater computational power for more complex applications.
- STM32: Suitable for performance-critical applications.
Factors to consider include computational power, power consumption, community support, and availability of libraries relevant to your project.
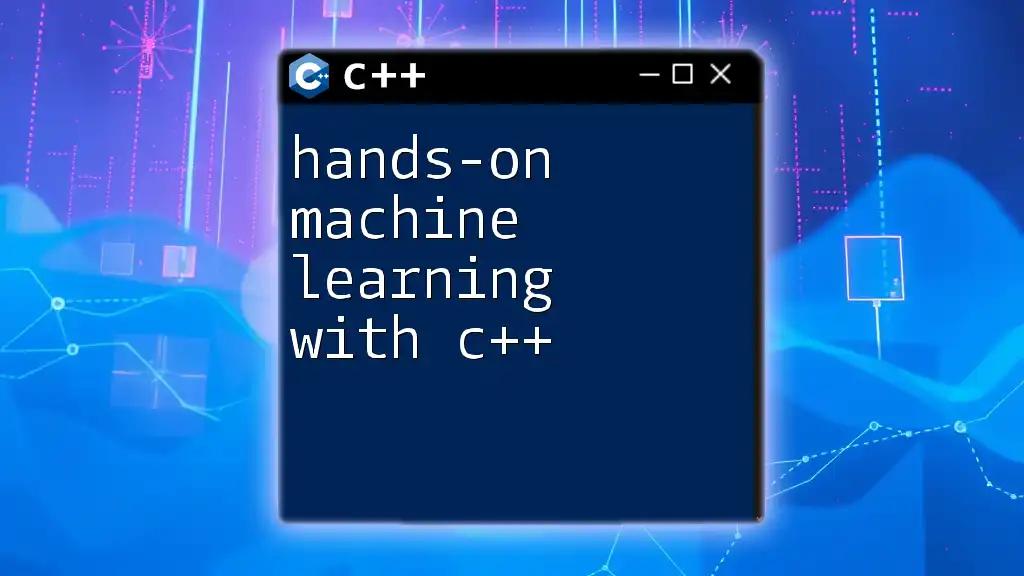
Essential C++17 Features for Embedded Programming
Smart Pointers
Memory management is crucial in embedded programming due to limited resources. C++17 introduces smart pointers like `std::unique_ptr` and `std::shared_ptr` to effectively manage dynamic memory allocation.
Example: Utilizing `std::unique_ptr` for LED control minimizes memory leaks.
#include <memory>
class LED {
public:
LED(int pin) : pin(pin) {
// Initialize pin
}
void on() {
// Turn the LED on
}
void off() {
// Turn the LED off
}
private:
int pin;
};
int main() {
std::unique_ptr<LED> led = std::make_unique<LED>(13);
led->on();
// Automatically cleaned up when led goes out of scope
}
Structured Bindings
C++17 simplifies working with tuples and pairs through structured bindings. This feature allows for the unpacking of multiple return values from the same function.
Code Snippet:
#include <tuple>
std::tuple<int, int> getCoordinates() {
return {10, 20};
}
int main() {
auto [x, y] = getCoordinates();
// x = 10, y = 20
}
Constexpr and Compile-time Computation
With constexpr capabilities, you can perform computations at compile-time, saving valuable run-time resources.
Example: Create a compile-time evaluated constant for configuration.
constexpr int maxConnections = 10;
void setup() {
// Use maxConnections in setup
}
Inline Variables
In C++17, you can define inline variables in header files, making them accessible across multiple translation units without creating multiple definitions.
Example:
// config.h
inline int maxValue = 100;
// In some .cpp file
#include "config.h"
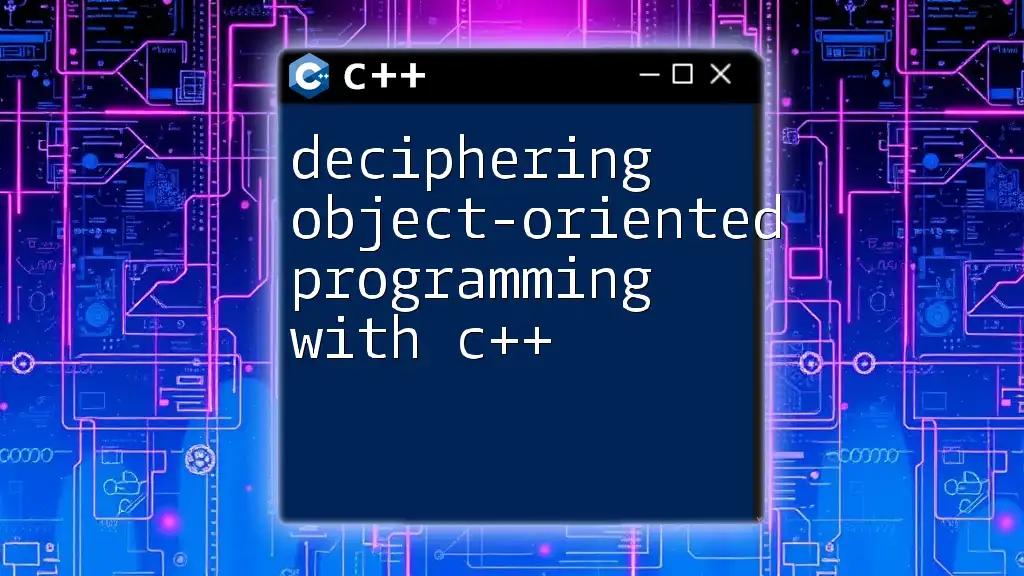
Building Your First Embedded Application
Setting Up Your Project Structure
Organizing the project effectively is crucial in embedded development. Here’s a recommended structure:
/MyEmbeddedProject
/src
/include
/lib
/tests
Following consistent naming conventions ensures clarity and maintainability.
Writing Your First Program: Blink an LED
Your first project can be as simple as an LED blink program.
Step 1: Hardware Setup
Connect an LED to your chosen microcontroller.
Step 2: Write the Code
#include <iostream>
#include "LED.h" // Hypothetical LED library
int main() {
LED led(13); // Pin 13
while (true) {
led.on();
delay(1000);
led.off();
delay(1000);
}
}
This program continuously blinks the LED, reinforcing your understanding of basic control structures in embedded programming.
Debugging Embedded Code
Debugging embedded systems can be challenging due to limited visibility into the hardware’s operation. Common techniques include:
- Using GDB: The GNU Debugger can be utilized to step through code running on target hardware.
- Serial Debugging Tools: Output log messages to a serial port for runtime diagnostics.
Develop a debugging workflow by setting breakpoints, inspecting variable states, and tracking down issues as you build your programs.
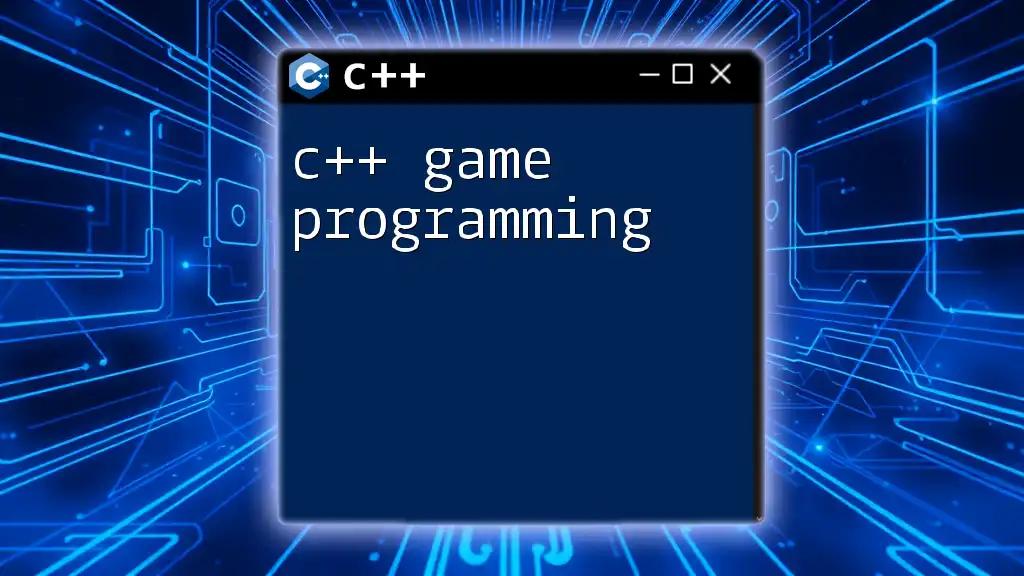
Advanced C++17 Techniques in Embedded Programming
Type Traits and SFINAE
C++17's type traits and SFINAE (Substitution Failure Is Not An Error) allow for selective function overloads based on types, enhancing code flexibility.
Example: Implement a function that behaves differently based on whether the input is an integer or a floating-point.
#include <type_traits>
#include <iostream>
template<typename T>
typename std::enable_if<std::is_integral<T>::value>::type foo(T value) {
std::cout << "Integer: " << value << std::endl;
}
template<typename T>
typename std::enable_if<std::is_floating_point<T>::value>::type foo(T value) {
std::cout << "Float: " << value << std::endl;
}
Multithreading with C++17
C++17 introduces standard multithreading features, allowing you to manage concurrent operations effectively.
Example Code:
#include <thread>
void processSensorData() {
// Read and process sensor data
}
int main() {
std::thread sensorThread(processSensorData);
sensorThread.join(); // Wait for the thread to finish
}
This enables more responsive applications, essential for real-time embedded systems where multiple tasks often occur simultaneously.
Using Lambdas for Callback Functions
Lambda functions can simplify the writing of callback functions, especially in cases where event-driven programming is necessary.
Code Snippet:
#include <iostream>
#include <functional>
void registerCallback(const std::function<void()>& callback) {
// Invoke the callback when an event occurs
callback();
}
int main() {
registerCallback([]() {
std::cout << "Event occurred!" << std::endl;
});
return 0;
}
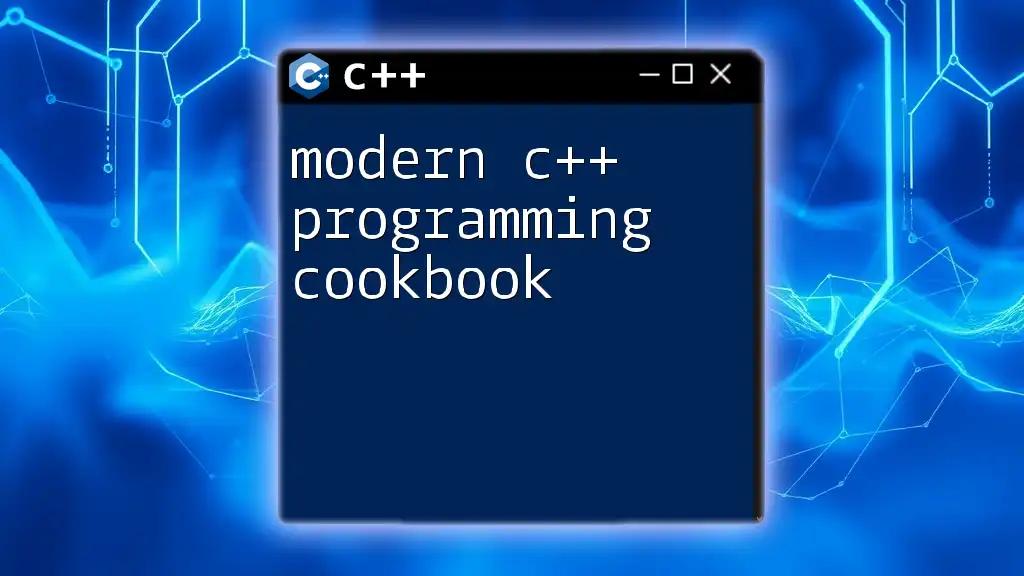
Resource Management and Constraints
Memory Management in Embedded Systems
Efficient memory management is crucial in embedded systems, where resources may be severely constrained. Techniques such as stack allocation and avoiding frequent dynamic memory allocation can help minimize memory footprints.
Power Management Techniques
Power conservation is critical for battery-operated embedded systems. Leverage C++ features to create sleep modes and timer-based wake-up calls to optimize power usage.
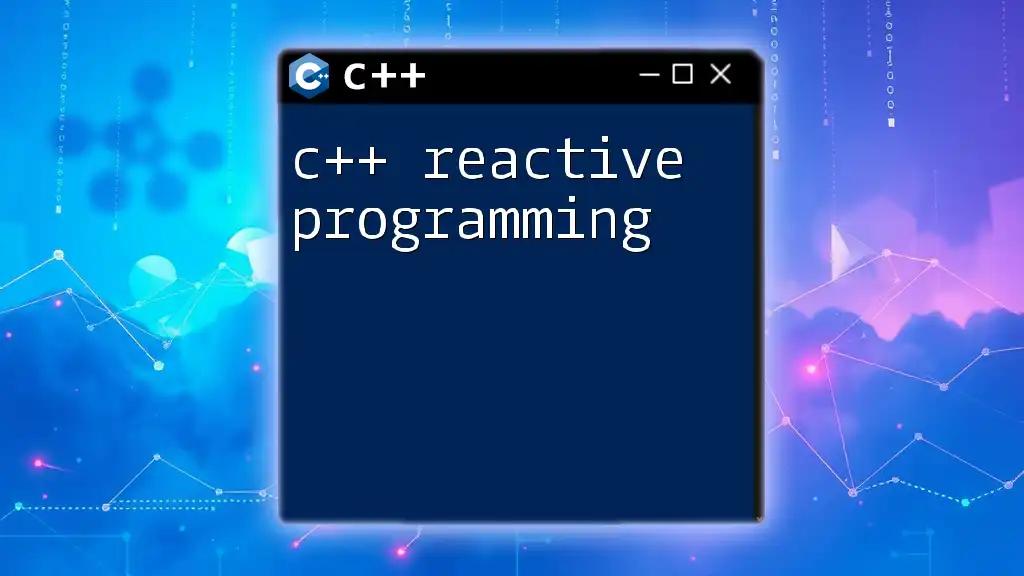
Real-world Applications
Hands-on embedded programming with C++17 has seen successful implementations across various domains. Products like smart thermostats, wearable devices, and automotive systems leverage these advancements for enhanced functionality.
Lessons Learned: Teams using C++17 reported improved maintainability and reduced time spent debugging legacy code, showcasing the advantages of modern language features in embedded systems.

Conclusion
The future of embedded programming with C++ is promising, particularly with the ongoing evolution of standards and technology. As the industry leans more into IoT, the need for efficient, reliable, and responsive embedded systems will grow, making skills in C++17 increasingly valuable.
Additional Resources
Aspiring embedded programmers can deepen their understanding through online courses, community forums, webinars, and valuable literature. Continuing education and engagement with the community will help you stay ahead in the rapidly advancing field of embedded programming.