Hands-on machine learning with C++ involves leveraging the language's efficiency and performance to implement foundational algorithms and models directly, enabling quick experimentation and deployment.
Here's a simple example using C++ to implement linear regression:
#include <iostream>
#include <vector>
void linearRegression(const std::vector<double>& x, const std::vector<double>& y, double& slope, double& intercept) {
double n = x.size();
double sum_x = 0, sum_y = 0, sum_xy = 0, sum_x2 = 0;
for (size_t i = 0; i < n; ++i) {
sum_x += x[i];
sum_y += y[i];
sum_xy += x[i] * y[i];
sum_x2 += x[i] * x[i];
}
slope = (n * sum_xy - sum_x * sum_y) / (n * sum_x2 - sum_x * sum_x);
intercept = (sum_y - slope * sum_x) / n;
}
int main() {
std::vector<double> x = {1, 2, 3, 4, 5};
std::vector<double> y = {2, 3, 5, 4, 6};
double slope, intercept;
linearRegression(x, y, slope, intercept);
std::cout << "Slope: " << slope << ", Intercept: " << intercept << std::endl;
return 0;
}
What is Machine Learning?
Machine learning is a subset of artificial intelligence that enables computers to learn from data and make decisions or predictions based on that data without being explicitly programmed for specific tasks. Its importance cannot be overstated as it has transformed industries, enabling advancements in various applications, from healthcare diagnostics to autonomous vehicles and recommendation systems.
Why Use C++ for Machine Learning?
Using C++ for machine learning offers several advantages. Performance is a critical factor—C++ is known for its speed and efficiency, which can significantly reduce training times for large datasets compared to higher-level languages. Additionally, C++ gives developers low-level memory management and control, allowing for optimizations that can be crucial in resource-heavy machine learning applications. Many popular libraries, such as TensorFlow and Dlib, provide C++ APIs, which offer the flexibility to harness the power of C++ while implementing sophisticated machine learning algorithms.
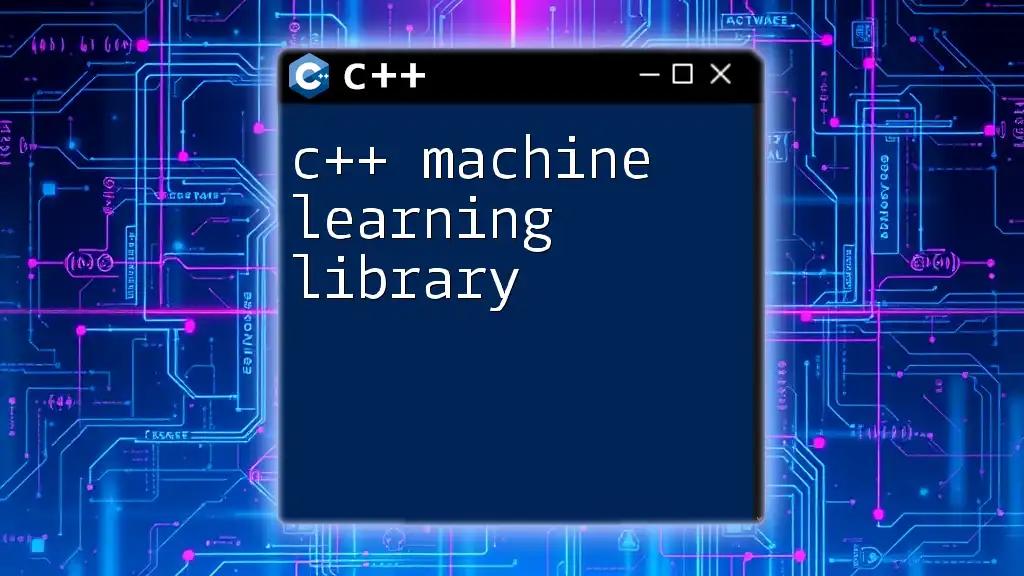
Setting Up Your C++ Environment for Machine Learning
Required Tools and Libraries
To get started with hands-on machine learning with C++, you'll need a few essential tools and libraries:
- C++ compilers: Popular options include GCC and Clang.
- Integrated Development Environments (IDEs): Consider using Visual Studio, CLion, or Code::Blocks for an efficient coding experience.
- Libraries:
- TensorFlow C++ API: For implementing deep learning models.
- MLPACK: A fast, flexible C++ machine learning library.
- Dlib: Rich in machine learning applications, particularly in computer vision.
Installation Guide
To set up your development environment, follow these steps:
- Install a C++ Compiler: Download and install GCC or Clang based on your operating system.
- Select an IDE: Choose an IDE that suits your preferences, and install it.
- Install Libraries:
- For example, to install TensorFlow's C++ API, follow the instructions on the [official TensorFlow installation guide](https://www.tensorflow.org/install/lang_c).
Once you’ve set up your environment, you’ll be ready to dive into machine learning implementations.
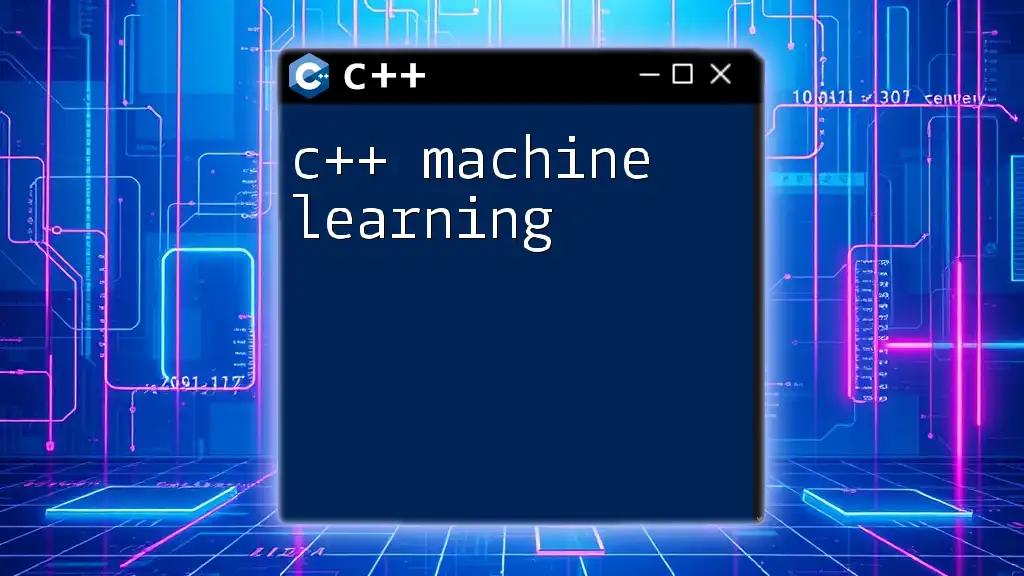
Fundamental Concepts of Machine Learning
Types of Machine Learning
Machine learning can broadly be categorized into three types:
Supervised Learning involves training a model on a labeled dataset, meaning that each training example is paired with an output label. For instance, predicting house prices based on various features (size, location, etc.) can be done using supervised learning techniques.
Here’s a basic implementation of linear regression in C++, which is a popular supervised learning technique:
#include <iostream>
#include <vector>
#include <numeric>
double linearRegression(const std::vector<double>& x, const std::vector<double>& y) {
double n = x.size();
double x_mean = std::accumulate(x.begin(), x.end(), 0.0) / n;
double y_mean = std::accumulate(y.begin(), y.end(), 0.0) / n;
double numerator = 0.0, denominator = 0.0;
for (size_t i = 0; i < n; ++i) {
numerator += (x[i] - x_mean) * (y[i] - y_mean);
denominator += (x[i] - x_mean) * (x[i] - x_mean);
}
return numerator / denominator;
}
int main() {
std::vector<double> x = {1, 2, 3, 4, 5};
std::vector<double> y = {1, 2, 3, 4, 5};
std::cout << "Slope: " << linearRegression(x, y) << std::endl;
}
Unsupervised Learning is different as it deals with datasets that do not have labeled outputs. One common method is clustering, where the algorithm tries to group similar data points together. Below is an example of K-means clustering:
#include <iostream>
#include <vector>
#include <algorithm>
// K-means implementation placeholder
void kMeansClustering(const std::vector<std::vector<double>>& data, int k) {
// Your K-means algorithm implementation would go here.
}
int main() {
std::vector<std::vector<double>> data = {{1.0, 2.0}, {3.0, 4.0}, {1.5, 1.5}};
int num_clusters = 2;
kMeansClustering(data, num_clusters);
}
Reinforcement Learning focuses on training agents to make decisions by taking actions within an environment to maximize cumulative rewards. It’s often used in robotics and game-playing AI.
Key Concepts in Machine Learning
Understanding machine learning involves grasping certain fundamental concepts:
- Datasets: The foundation of any machine learning project. Datasets often consist of features (input variables) and labels (outputs).
- Features and Labels: Features can be various attributes used for prediction, while labels are what you want to predict or classify.
- Model Training: The process of feeding data to algorithms to enable them to learn patterns.
- Testing and Validation: Evaluating model performance using separate testing datasets ensures that the model generalizes well to new data.
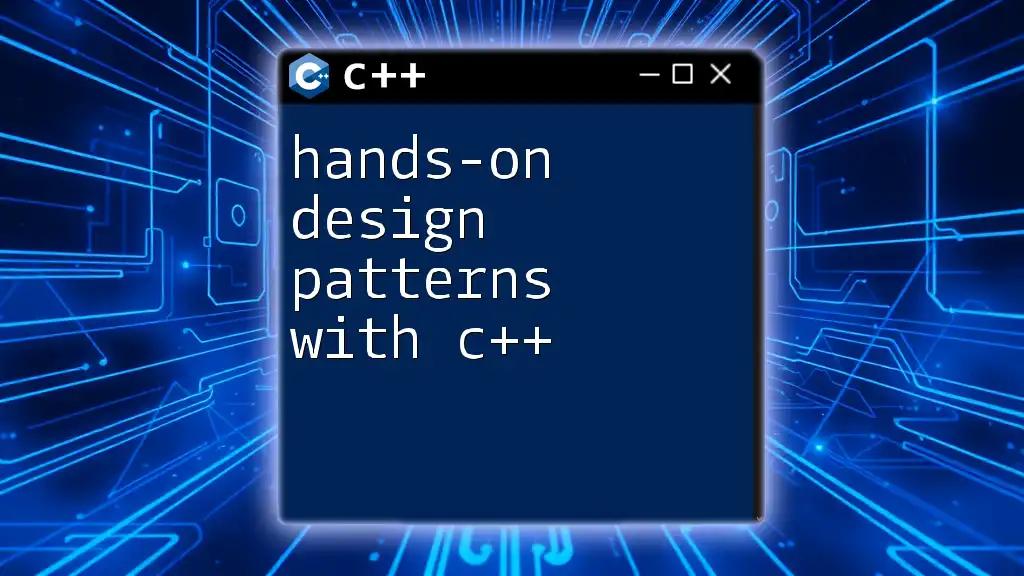
Implementing Machine Learning Algorithms in C++
Linear Regression Example
As mentioned earlier, linear regression is a fundamental algorithm in supervised learning. Here is a detailed implementation that demonstrates how to create a simple linear regression model from scratch:
#include <iostream>
#include <vector>
#include <numeric>
double linearRegression(const std::vector<double>& x, const std::vector<double>& y) {
double n = x.size();
double x_mean = std::accumulate(x.begin(), x.end(), 0.0) / n;
double y_mean = std::accumulate(y.begin(), y.end(), 0.0) / n;
double numerator = 0.0, denominator = 0.0;
for (size_t i = 0; i < n; ++i) {
numerator += (x[i] - x_mean) * (y[i] - y_mean);
denominator += (x[i] - x_mean) * (x[i] - x_mean);
}
return numerator / denominator; // Return the slope of the line
}
int main() {
std::vector<double> x = {1, 2, 3, 4, 5}; // Features
std::vector<double> y = {1, 3, 3, 2, 5}; // Labels
std::cout << "Slope: " << linearRegression(x, y) << std::endl; // Output the slope of the regression line
}
Walkthrough: This code computes the slope of the best-fit line for the given data points. By following the steps within the `linearRegression` function, you’ll gain insight into the calculations involved behind linear regression.
Decision Trees
Decision trees are a popular choice for both classification and regression tasks. They work by splitting the dataset into subsets based on attribute values. Here’s a simplified pseudo-implementation concept:
#include <iostream>
#include <vector>
class DecisionTree {
public:
void fit(const std::vector<std::vector<double>>& data, const std::vector<int>& labels) {
// Your decision tree logic goes here
}
int predict(const std::vector<double>& input) {
// Your prediction logic would go here, returning a class label
return 0; // Placeholder
}
};
int main() {
std::vector<std::vector<double>> data = {{1.0, 2.0}, {1.5, 1.8}, {5.0, 8.0}};
std::vector<int> labels = {0, 0, 1}; // Class labels
DecisionTree tree;
tree.fit(data, labels);
std::cout << "Predicted class: " << tree.predict({1.5, 2.0}) << std::endl;
}
Walkthrough: In this example, the `DecisionTree` class provides a structured framework to implement basic decision tree logic. The `fit` function would enable training on the dataset, while the `predict` function allows for inference from new data.
Neural Networks
Neural networks can model complex patterns and are effectively implemented for tasks like recognition and classification. Here is an introductory implementation:
#include <iostream>
#include <vector>
class SimpleNeuralNetwork {
public:
SimpleNeuralNetwork(int input_size, int hidden_size) {
// Initializing weights and biases
}
void train(const std::vector<std::vector<double>>& inputs, const std::vector<double>& targets) {
// Training logic goes here
}
double predict(const std::vector<double>& input) {
// Prediction logic goes here
return 0.0; // Placeholder return
}
};
int main() {
SimpleNeuralNetwork nn(2, 2); // Example sizes
std::vector<std::vector<double>> inputs = {{0.0, 0.0}, {1.0, 1.0}};
std::vector<double> targets = {0.0, 1.0};
nn.train(inputs, targets);
std::cout << "Prediction: " << nn.predict({0.5, 0.5}) << std::endl;
}
Walkthrough: This example creates a simple neural network class with methods for training and prediction. The implementation gives an idea of how neural networks can be structured in C++, but it requires elaboration on the training algorithm and weight updates.
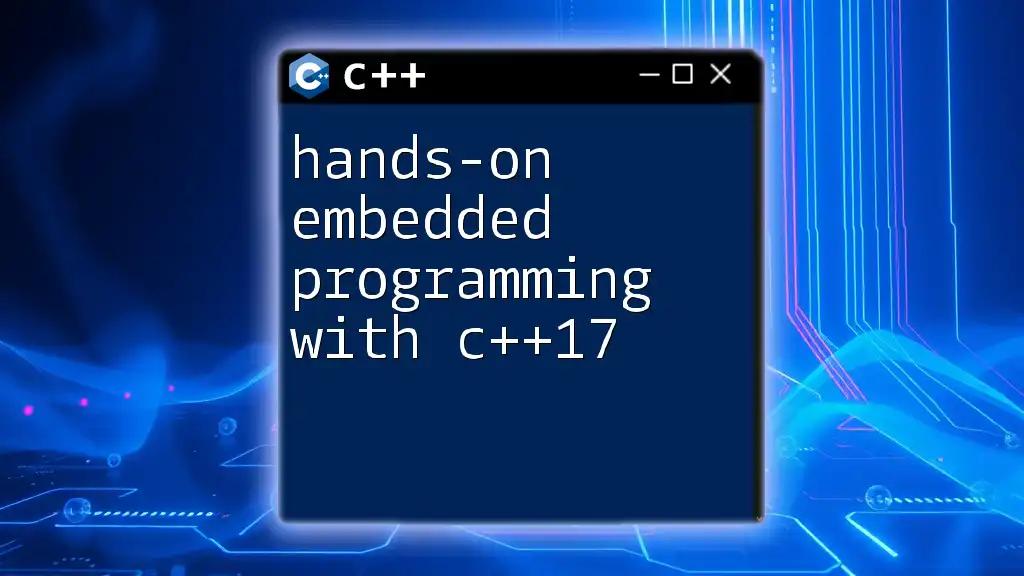
Evaluating Machine Learning Models
Performance Metrics
Performance evaluation is crucial in assessing how well your machine learning model performs. Common metrics include:
- Accuracy: The ratio of correctly predicted instances to the total instances.
- Precision: The ratio of true positives to the total predicted positives.
- Recall: The ratio of true positives to the total actual positives.
To calculate these metrics, your implementation can include:
#include <iostream>
#include <vector>
double calculateAccuracy(const std::vector<int>& true_labels, const std::vector<int>& predicted_labels) {
int correct_count = 0;
for (size_t i = 0; i < true_labels.size(); ++i) {
if (true_labels[i] == predicted_labels[i]) correct_count++;
}
return static_cast<double>(correct_count) / true_labels.size();
}
Cross-Validation Techniques
Cross-validation is a technique for assessing how a predictive model performs in practice. The purpose is to partition the dataset into subsets, train the model on some of the subsets, and test it on the others. One effective method is k-fold cross-validation.
Example implementation can be outlined as follows:
#include <iostream>
#include <vector>
void kFoldCrossValidation(const std::vector<std::vector<double>>& data, int k) {
int fold_size = data.size() / k;
for (int i = 0; i < k; ++i) {
// Split your training and validation sets based on the current fold.
}
}
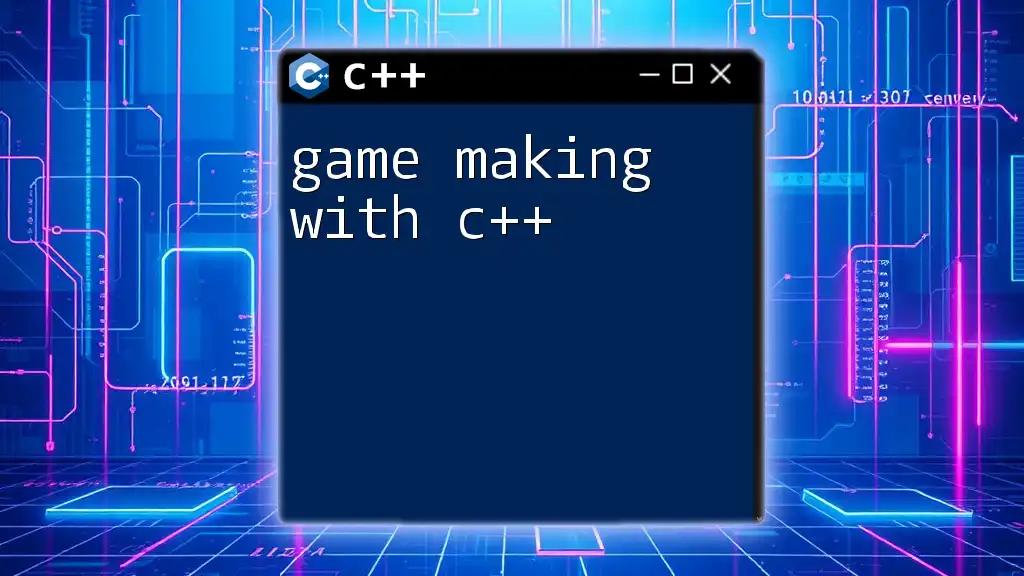
Advanced Topics in Machine Learning with C++
Feature Engineering
Feature engineering is the process of using domain knowledge to extract features that enhance the performance of machine learning algorithms. Examples of techniques include:
- Normalization: Scaling features to a common range.
- Encoding categorical variables: Transforming categorical features into numerical values.
#include <iostream>
#include <vector>
void normalizeFeatures(std::vector<double>& features) {
double max_value = *std::max_element(features.begin(), features.end());
for (auto& feature : features) {
feature /= max_value; // Simple normalization
}
}
Hyperparameter Tuning
Hyperparameter tuning involves optimizing the parameters that are not learned through training. Strategies include:
- Grid Search: Testing combinations of hyperparameters.
- Random Search: Randomly searching a subset of hyperparameter space for optimal values.
Here’s a basic placeholder for implementing grid search:
void gridSearch(const std::vector<int>& hyperparameters) {
// Loop through hyperparameters and evaluate model performance.
}
RNNs and LSTMs
Recurrent Neural Networks (RNNs) and Long Short-Term Memory (LSTM) networks are extensively used for sequential data, such as time series or natural language processing. Understanding and implementing these models requires a deeper domain knowledge, but the general structure is as follows:
#include <iostream>
#include <vector>
class RNN {
public:
void train(const std::vector<std::vector<double>>& sequences) {
// Training logic for RNNs goes here
}
double predict(const std::vector<double>& input) {
// Prediction logic for an input sequence
return 0.0; // Placeholder
}
};
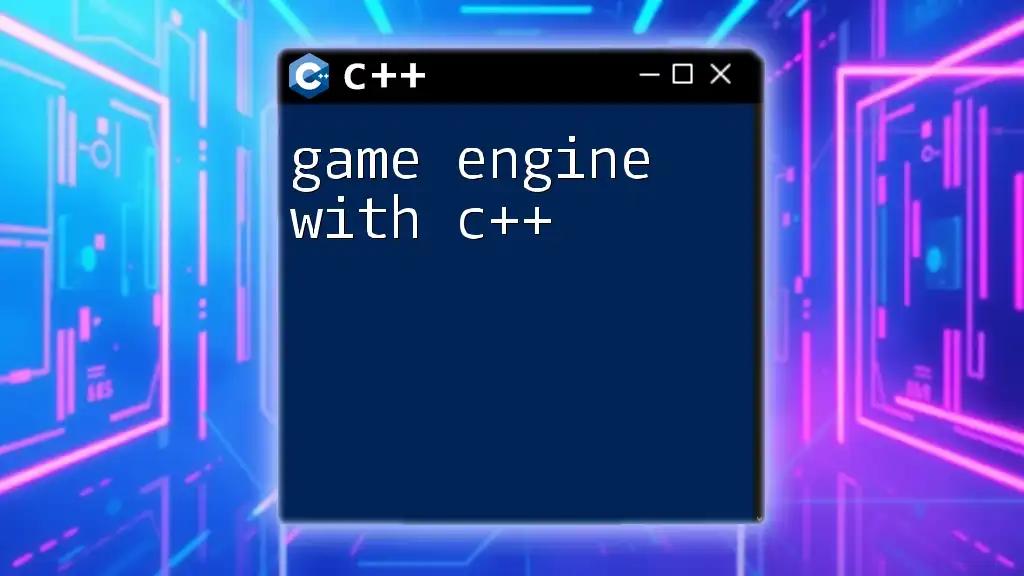
Real-World Applications of Machine Learning in C++
Computer Vision
Machine learning has become a cornerstone of computer vision. An example project might include image classification, where you implement a convolutional neural network (CNN) to classify images effectively.
Natural Language Processing
NLP focuses on enabling machines to understand human language. Here’s a simple sentiment analysis example that could be implemented in C++ using libraries mentioned previously, like Dlib.
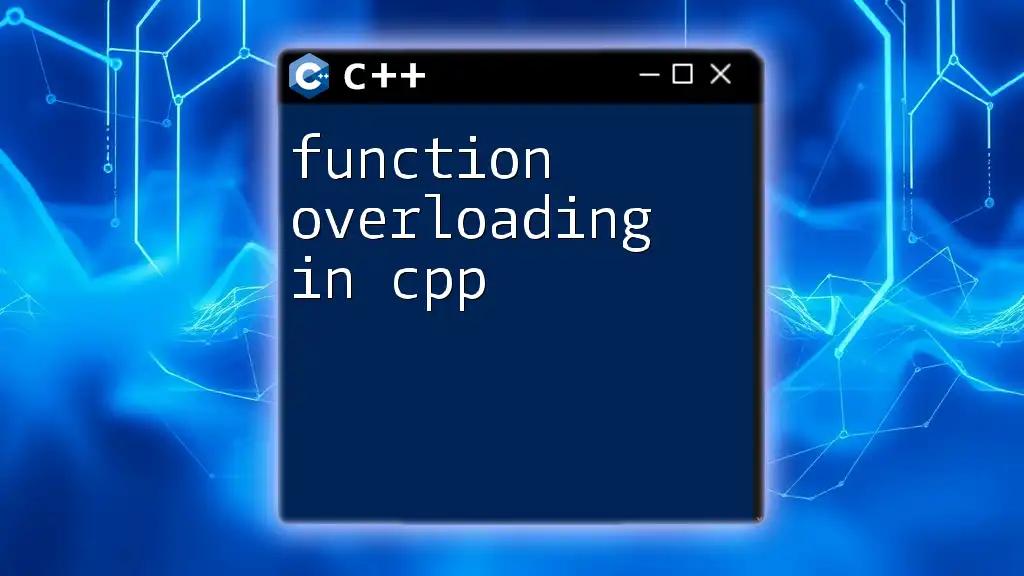
Conclusion
In summary, this guide has taken you through the essentials of hands-on machine learning with C++, from basic concepts to practical implementations. Using C++ provides the performance and control necessary for developing robust machine learning models.
Getting Started with Your Own Projects
I encourage you to take the knowledge you've gained and start your own machine learning projects. Start small, choose an interesting dataset, and apply the techniques you've learned.
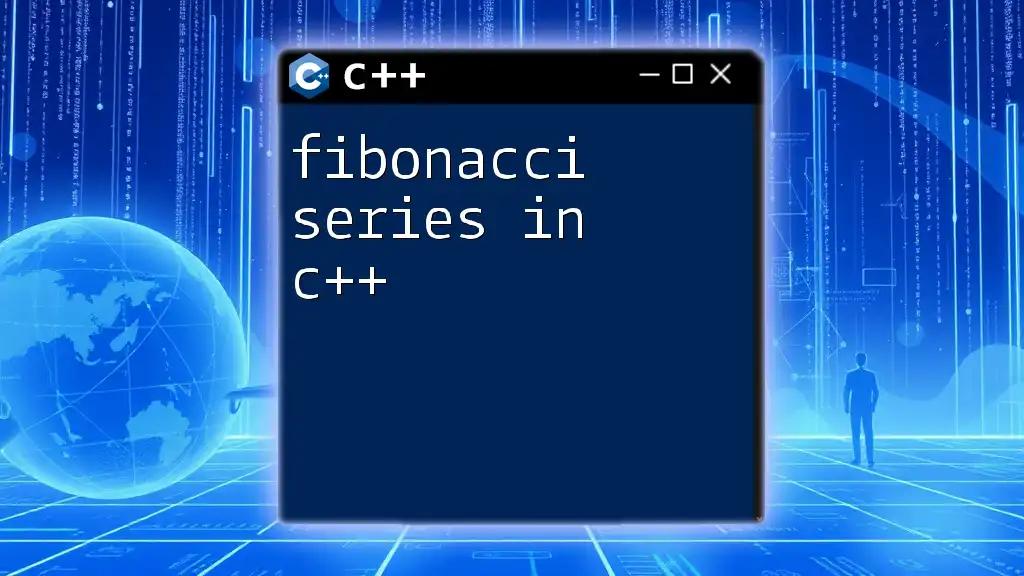
Additional Resources
For further exploration, consider delving into the following recommended reading and online courses to deepen your understanding and skill set in machine learning with C++. Engaging with communities—whether through forums, GitHub, or local meetups—can also provide invaluable support and encouragement.