The "no matching function call" error in C++ occurs when the compiler cannot find a function that matches the arguments you provided; this typically happens due to incorrect parameter types, numbers, or lack of the required function overload.
Here's a code snippet illustrating this error:
#include <iostream>
void display(int a) {
std::cout << "Integer: " << a << std::endl;
}
int main() {
display(5); // Valid call
display(3.14); // Error: no matching function call
return 0;
}
What is the "No Matching Function Call" Error?
The "no matching function call" error in C++ signifies that the compiler cannot find a function that matches the specified name and argument types provided in a function call. This message is critical because it can highlight issues related to function signatures, argument types, or even the absence of a function definition altogether.
Importance of Correct Function Calls
Correct function calls are paramount in C++. Each function has a defined signature, which includes its name, number of parameters, and the types of those parameters. If any aspect mismatches, the compiler throws the no matching function call c++ error. This type safety is vital in C++ to prevent runtime errors and ensure that function behavior aligns with expectations.
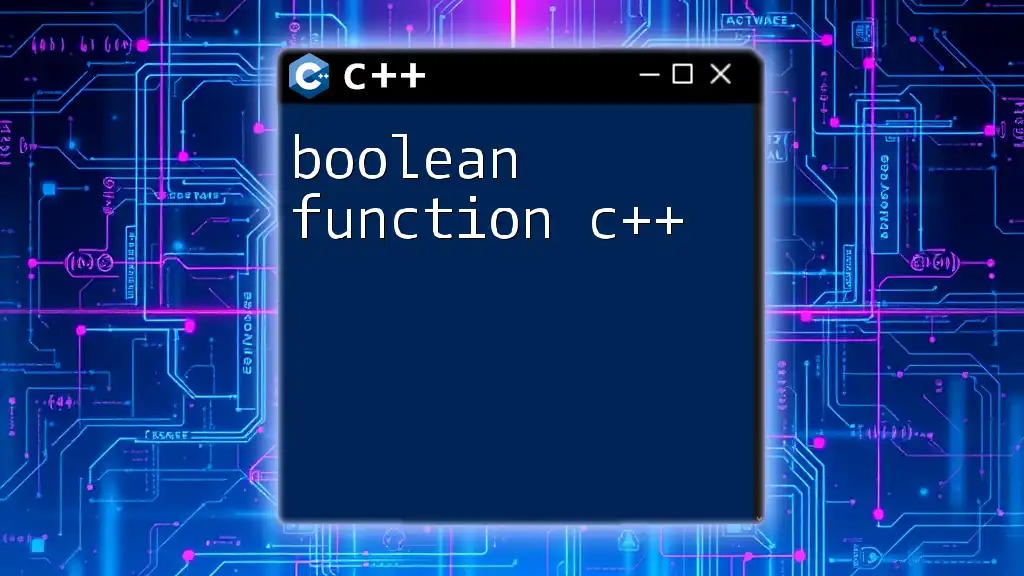
Common Scenarios Leading to "No Matching Function Call" Error
Function Overloading Issues
Function overloading allows multiple functions to have the same name with different parameter lists. However, if a call does not uniquely match any of the overloaded function signatures, the compiler cannot resolve it, leading to an error.
For example:
void func(int a);
void func(double b);
func("text"); // Will trigger the error
In this case, the string literal "text" does not match any of the function signatures, causing a mismatch. This error emphasizes the need for ensuring that argument types correspond to existing overloads.
Mismatched Argument Types
Type matching is essential in C++, as functions expect parameters of specific types. If a function is called with arguments of types that do not match the expected types, the no matching function call error appears.
Consider the following:
void add(int x, double y);
add(5, "10"); // Causes the error
The second argument is a string, which does not correspond to the expected `double` type. The compiler flags this as an issue, reminding programmers to check the types of the arguments supplied.
Incomplete Function Implementations
Incomplete implementations occur when a function is declared but remains undefined. If you attempt to call such a function, the compiler will raise a no matching function call c++ error, as there is no defined action accompanying the call.
Example:
void printValue(int val); // Declaration without definition
printValue(10); // No matching function call error
Here, the function `printValue` lacks a definition, leading to ambiguity about what to execute.
Template Function Complications
Templates in C++ provide a powerful means of type abstraction, but they can introduce errors if not appropriately specialized. If a template function is called with a type that lacks a matching specialization, a compilation error arises.
For instance:
template <typename T>
void process(T val);
process(5.5); // Assuming no specialization exists
Without a specialization that handles the `double` or its relevant operations, the compiler cannot find a matching function, leading to an error.
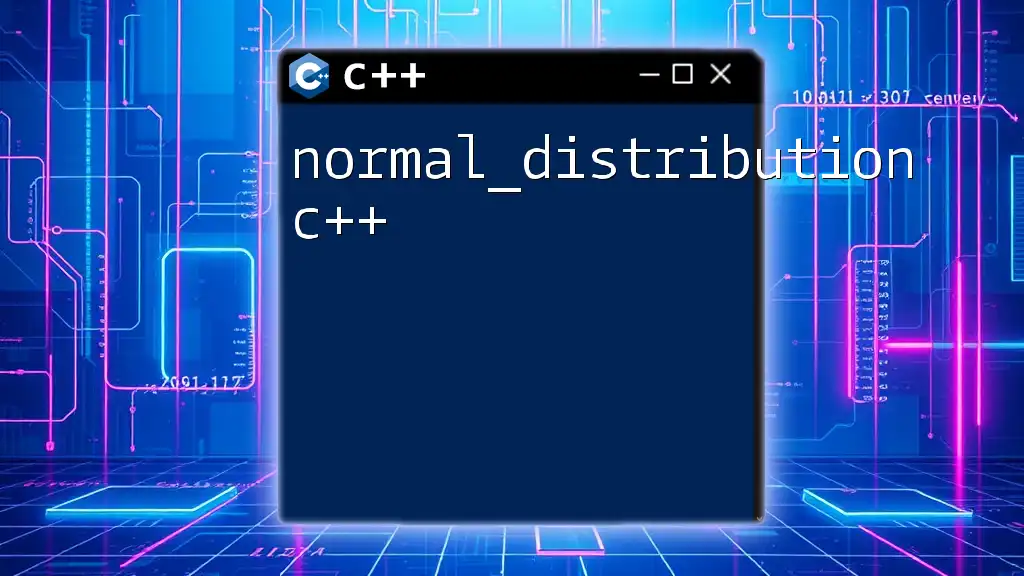
Understanding Compiler Errors
Deciphering Compiler Messages
When encountering a no matching function call c++ error, understanding the compiler's error message can help isolate the issue. The compiler may indicate the specific function being called and the expected versus provided types. By carefully analyzing the message, developers can trace the problem's source effectively.
Using Compiler Flags for Debugging
C++ compilers offer various flags that enhance error reporting and debugging capabilities. For example, using the `-Wall` flag in GCC enables warnings for most potential issues, which may lead to the identification of mismatches before they become problematic.
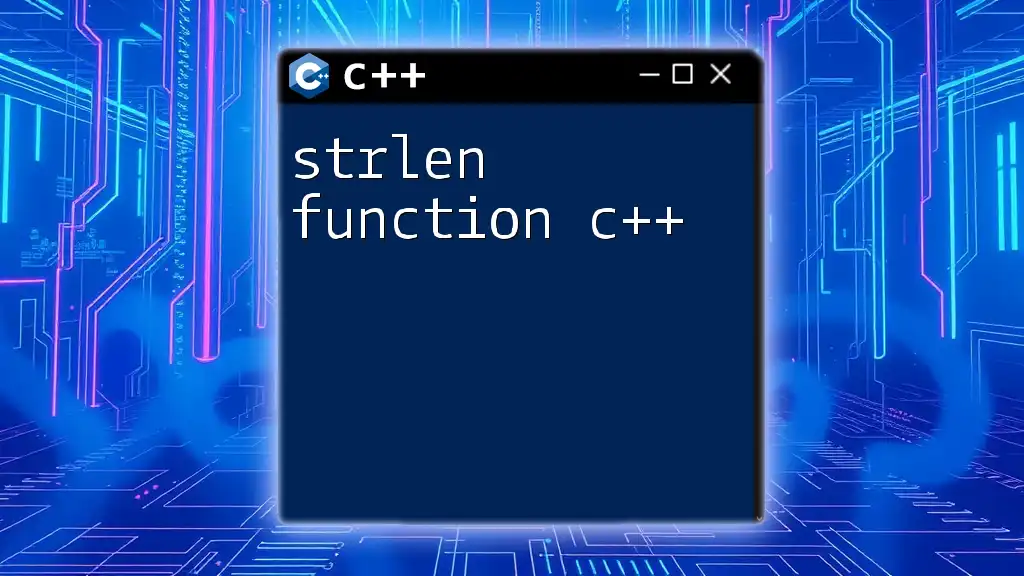
Best Practices to Avoid "No Matching Function Call" Error
Clear Function Signatures
Defining clear and precise function signatures removes ambiguity and helps prevent function call errors. Ensure that function names accurately describe their purpose, and use meaningful parameter names reflecting their intended data types.
Consistent Data Types
Promoting consistency in data types within function parameters is crucial. When designing functions, stick to a limited set of data types and avoid implicit type conversions. Explicitly converting incompatible types before passing them to functions mitigates the potential for mismatch errors.
Testing and Validation
Incorporating unit tests as part of the development process allows for early identification of such errors. Utilize testing frameworks like Google Test or Catch2 to systematically validate functions. Effective testing not only delineates function behavior but also strengthens overall code quality.
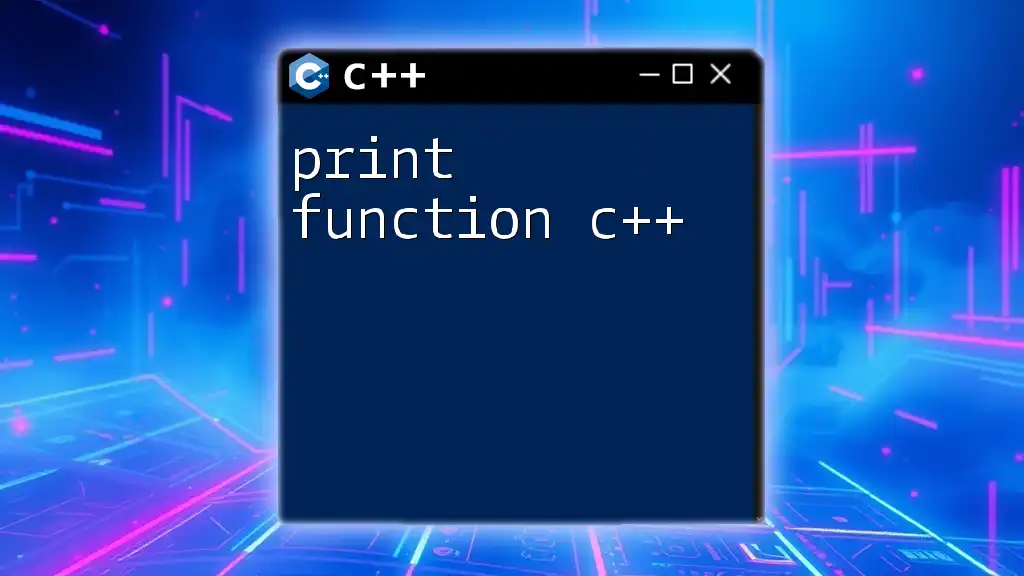
Case Studies and Solutions
Real-World Example 1: Resolving the Error
Imagine a situation where the function `compute` is designed to handle two integers but is inadvertently called with a float:
void compute(int a, int b);
compute(5.0, 10.0); // Intent is to call with integers but passes floats
To fix this, ensure type consistency in the function call or modify the function to accept floating-point types explicitly.
Real-World Example 2: Successful Function Overloads
Let's check a working scenario with overloaded functions:
void display(int x);
void display(double y);
display(5); // Calls display(int)
display(5.5); // Calls display(double)
These overloads work effectively because the calls clearly correspond to their respective signatures, showing how to manage multiple function definitions with the same name.
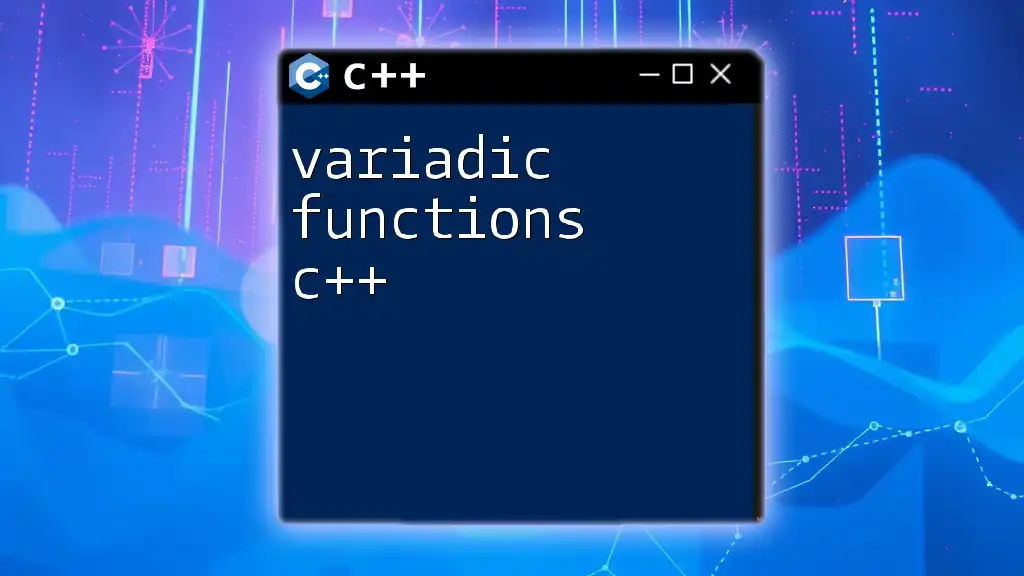
Conclusion
To recap, understanding the no matching function call c++ error is vital for effective C++ programming. This error commonly arises from function overloading issues, mismatched argument types, incomplete implementations, and complications with templates. By employing best practices such as clear function signatures, maintaining consistent data types, and engaging in thorough testing, developers can significantly reduce the chances of encountering this error.
Encouragement to Practice
Engage with practical coding exercises that challenge your understanding of function calls in C++. Regular practice not only solidifies concepts but also enhances your problem-solving skills as you encounter and solve the no matching function call error.
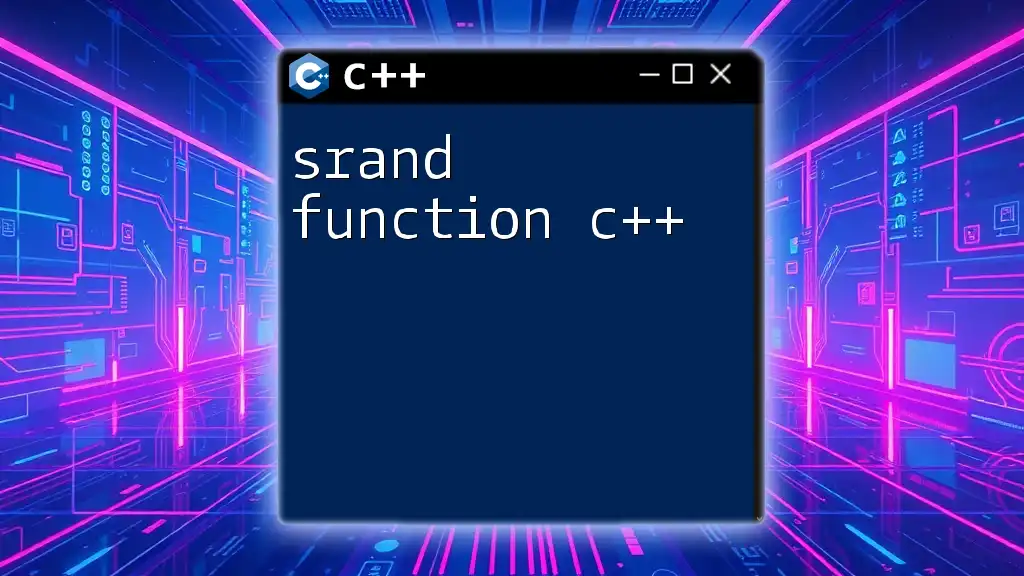
References for Further Learning
For those looking to deepen their understanding of C++, explore the official C++ documentation, recommended online courses, and community forums dedicated to C++ development. These resources will provide further insights and support as you navigate your programming journey.