In C++, a `struct` is a user-defined data type that allows you to group related variables together, and you can easily pass it as an argument to functions for processing.
Here's a simple code snippet demonstrating how to define a `struct` and use it in a function:
#include <iostream>
using namespace std;
struct Point {
int x;
int y;
};
void printPoint(Point p) {
cout << "Point(" << p.x << ", " << p.y << ")" << endl;
}
int main() {
Point pt = {10, 20};
printPoint(pt);
return 0;
}
Understanding Structs in C++
A struct in C++ is a user-defined data type that allows the grouping of variables under a single name. This grouping is essential for organizing complex data, allowing for easier management and manipulation within your programs.
Structs are particularly important because they provide a way to model real-world entities more naturally. For example, if you’re creating a program for handling geometric shapes, you could define a struct to represent a `Circle` with properties like `radius` and `center`.
Definition of a Struct
In C++, the syntax for declaring a struct is straightforward. You use the `struct` keyword followed by the name of the structure, followed by the variable members you want to include.
Example: Basic Struct Definition
struct Point {
int x; // X-coordinate
int y; // Y-coordinate
};
Initializing Structs
Once you define a struct, you can easily create instances of it and initialize its members. You can either set the values at declaration or assign them later.
Example: Initialization of Struct
Point p1 = {10, 20}; // Initializing a Point struct
Accessing Struct Members
Accessing members of a struct is done using the dot operator (`.`). This operator allows you to refer to individual members of the struct, facilitating the extraction and manipulation of data.
Example: Accessing Members
std::cout << "X: " << p1.x << ", Y: " << p1.y << std::endl; // Output: X: 10, Y: 20
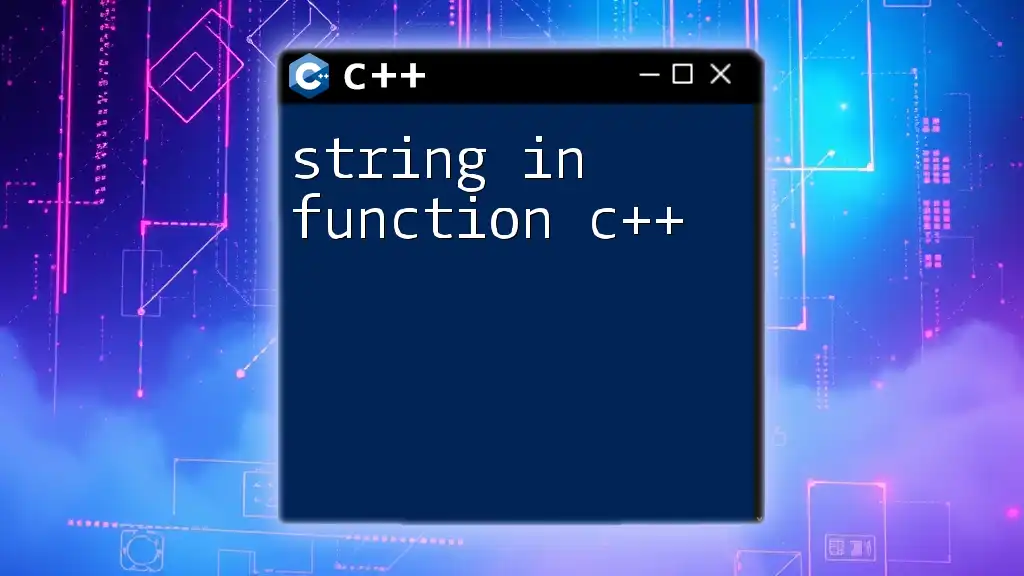
Using Structs in Functions
Structs can greatly enhance the capability of functions in C++. They not only provide a clean way to pass multiple related data items but also make your code more expressive.
Passing Structs to Functions by Value
When you pass a struct to a function by value, a copy of the entire struct is made. This means changes made to the struct inside the function do not affect the original struct.
Example: Pass by Value
void printPoint(Point p) {
std::cout << "Point: (" << p.x << ", " << p.y << ")" << std::endl;
}
In this example, calling `printPoint(p1)` would print the coordinates of `p1` without modifying it.
Passing Structs to Functions by Reference
You can also pass structs by reference using the `&` symbol. This allows your function to modify the original struct since it operates on its memory address rather than a copy.
Example: Pass by Reference
void movePoint(Point& p, int dx, int dy) {
p.x += dx; // Modifies the original struct
p.y += dy;
}
Calling `movePoint(p1, 5, 3)` would change the coordinates of `p1` directly.
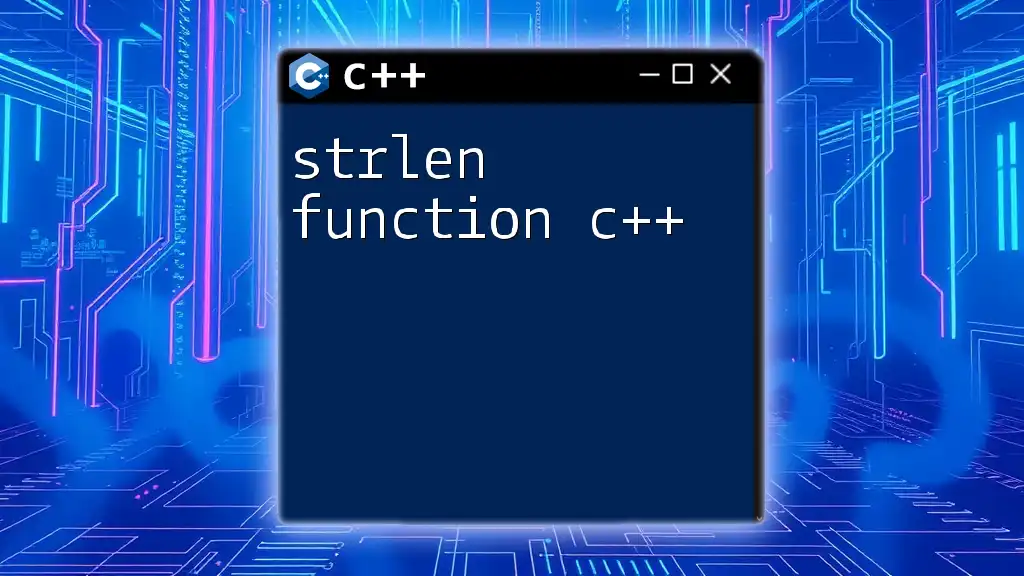
Returning Structs from Functions
C++ allows functions to return structs, which can help encapsulate complex data manipulations neatly within a single return value.
Understanding Function Return Types
Returning a struct can simplify function signatures, making it clear that you are returning a set of related data.
Example: Function Returning a Struct
Point createPoint(int x, int y) {
Point p; // Declare a Point
p.x = x; // Set the x-coordinate
p.y = y; // Set the y-coordinate
return p; // Return the Point
}
By calling `Point newPoint = createPoint(10, 20);`, you will have a `Point` instance initialized with the specified coordinates.
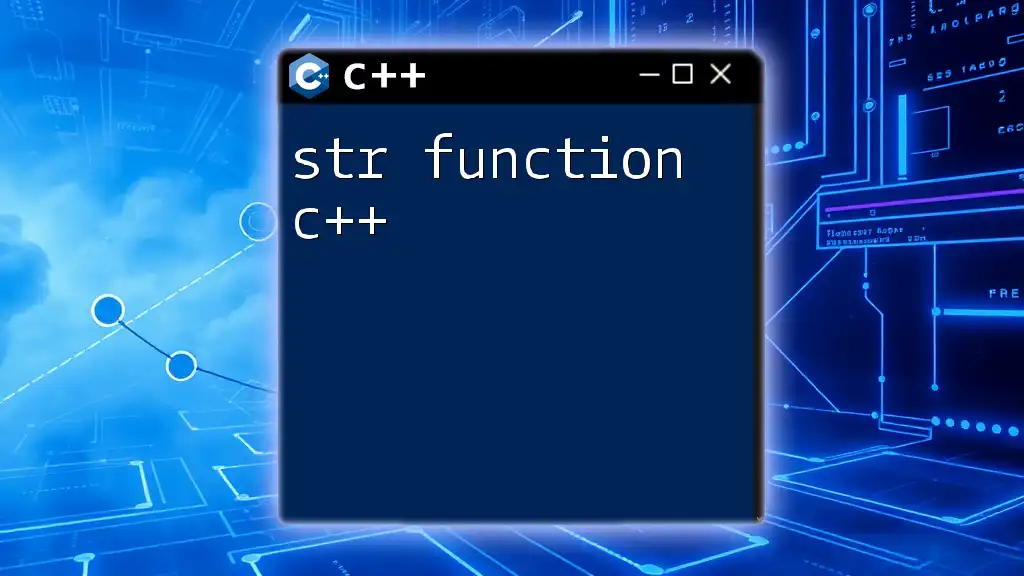
Structs in Function Overloading
C++ allows you to overload functions based on the parameters passed. This means you can have multiple functions with the same name but different parameters, including structs.
Example: Function Overloading with Structs
void display(Point p) {
std::cout << "Displaying Point" << std::endl;
}
void display(int x, int y) {
std::cout << "Displaying Coordinates: " << x << ", " << y << std::endl;
}
In this scenario, both `display` functions are valid, depending on the arguments passed.
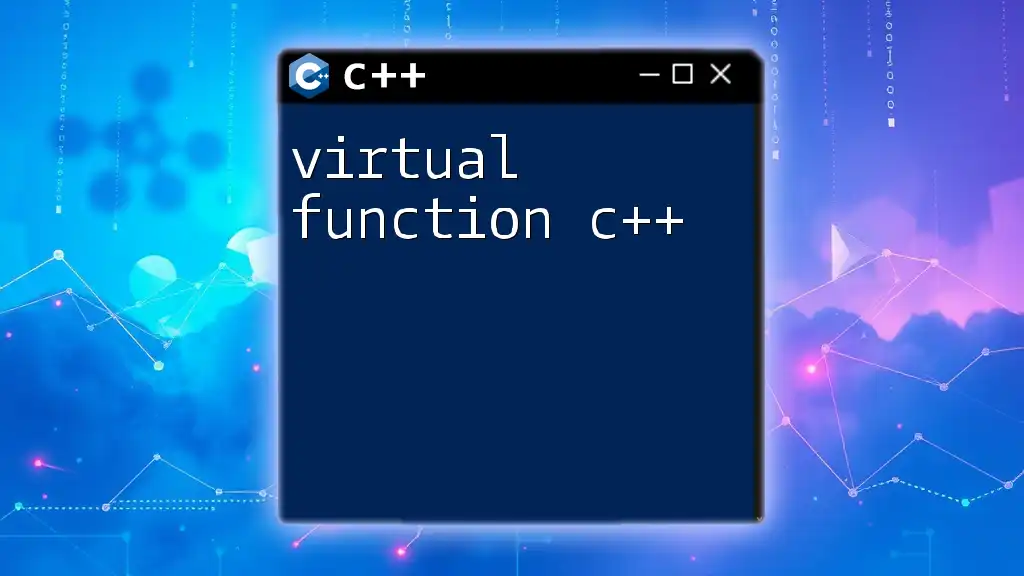
Real-World Applications of Structs in Functions
Structs serve as effective data containers in various applications, encapsulating related properties.
In game development, for instance, a `Player` struct can represent characteristics such as health, position, and score, neatly packaged together, enabling functions that manipulate player data to interact with a single structured entity.
In data processing applications, structs can represent records, such as `Employee`, allowing for organized handling of related fields like `name`, `age`, and `salary` within a function that processes employee information.
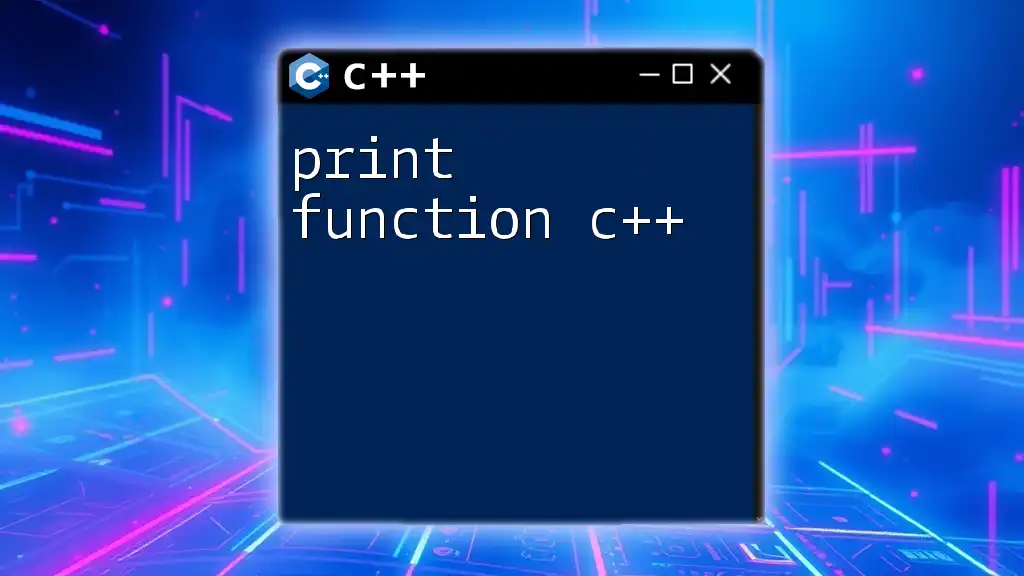
Best Practices when Using Structs in Functions
Understanding when to use structs versus classes is paramount. Use structs when you want to group simple data types without additional functionality, while classes are better for encapsulated data with methods.
Performance Considerations: Always consider the overhead of copying large structs. Use references when passing structs to avoid unnecessary memory use.
Clarity and Maintainability: Keep your code clear and maintainable. Structs can enhance readability by consolidating related information, which can be crucial for others reading your code.
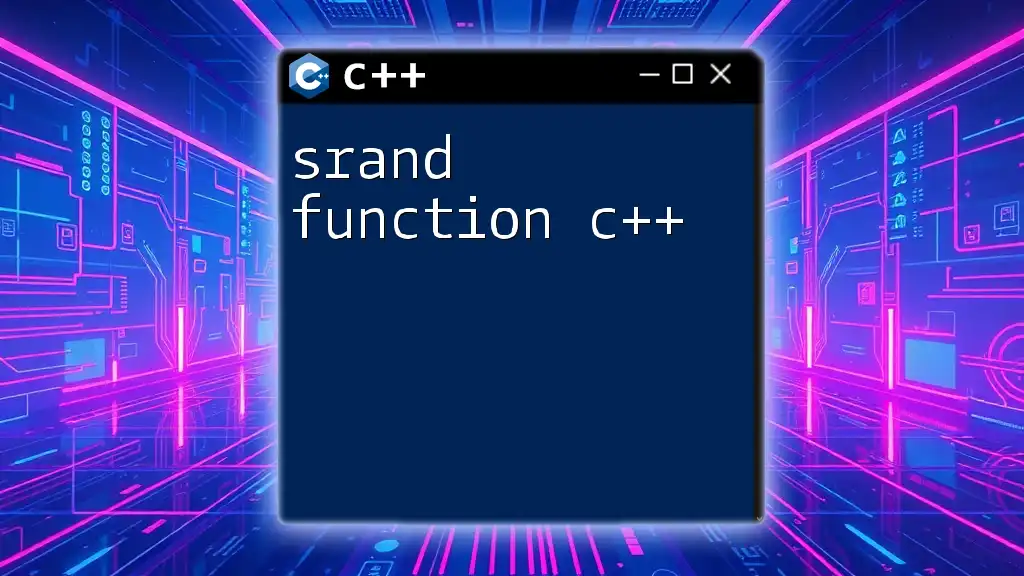
Conclusion
Structs in functions provide a robust structure for handling related data efficiently in C++. They not only simplify the process of passing complex data but also enhance the clarity and maintainability of your code.
As you practice and implement these concepts in your coding projects, you will see the advantages of incorporating struct in function c++. Enjoy exploring more ways to harness the power of C++ structures!
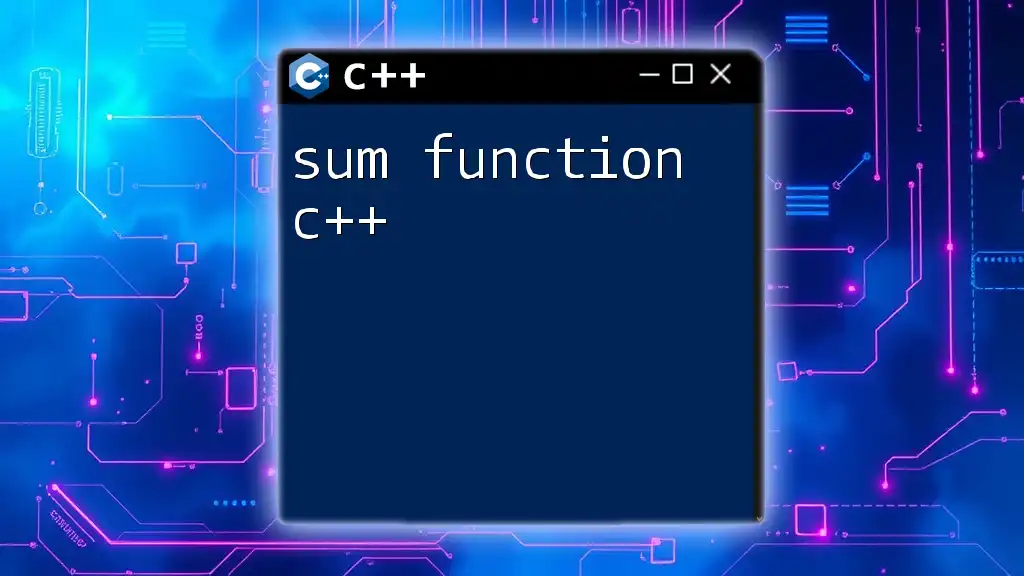
Additional Resources
To deepen your understanding, consult the official C++ documentation, and consider following educational tutorials specifically focused on C++ structures and functions for a more profound learning experience. Recommended books on the subject can also offer comprehensive insights into mastering the language.