Hands-on C++ game animation programming involves creating dynamic and engaging visual experiences in games by manipulating object properties and movement within a game loop.
Here’s a simple code snippet illustrating a basic game loop that updates an object's position for animation:
#include <iostream>
#include <unistd.h> // For sleep function
struct Object {
float x, y; // Position coordinates
void update() {
x += 1.0f; // Move right
y += 0.5f; // Move down
}
void display() {
std::cout << "Object Position: (" << x << ", " << y << ")" << std::endl;
}
};
int main() {
Object obj = {0.0f, 0.0f};
for (int i = 0; i < 10; ++i) {
obj.update();
obj.display();
usleep(500000); // Sleep for half a second
}
return 0;
}
Setting Up Your Development Environment
Choosing the Right Tools
To dive into hands-on C++ game animation programming, the first step involves choosing the right development tools. Popular Integrated Development Environments (IDEs) like Visual Studio and Code::Blocks are highly recommended due to their robust features and support for C++.
For game development, utilizing an engine like Unreal Engine can streamline your workflow significantly, particularly if you aim to leverage the full power of C++. Another option is Unity, which allows you to integrate C++ plugins to enhance functionality.
Installing Required Libraries
To facilitate graphics and multimedia handling, installing essential libraries is vital.
-
SFML (Simple and Fast Multimedia Library) is versatile for 2D graphics, providing easy access to window management, graphics, and sound.
-
SDL (Simple DirectMedia Layer) is another option, particularly good for creating games that require high performance.
Ensure to follow the library installation guide relevant to your OS to set up correctly.
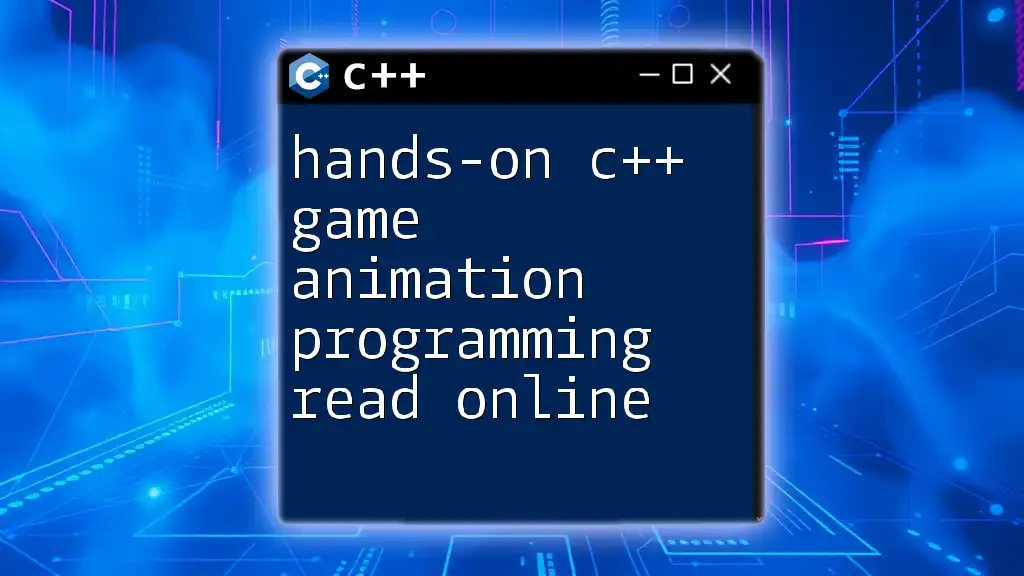
Understanding Game Animation Concepts
Basic Animation Principles
Animation in games relies on fundamental principles that dictate how characters and objects move. Keyframes represent important moments in an animation sequence, while frame rate is critical as it determines the smoothness of movement. Higher frame rates yield smoother animations, so understanding this relationship is crucial.
Additionally, interpolation techniques like linear and Bézier curves play a significant role in creating fluid transitions between keyframes.
Types of Animation in Games
Game animators typically work with 2D and 3D animation. Sprite animation is common in 2D games where characters or objects are drawn frame by frame, while skeletal animation is predominant in 3D, where a "skeleton" structure controls the animation of a model. Understanding these distinctions is pivotal as you embark on your animation journey.
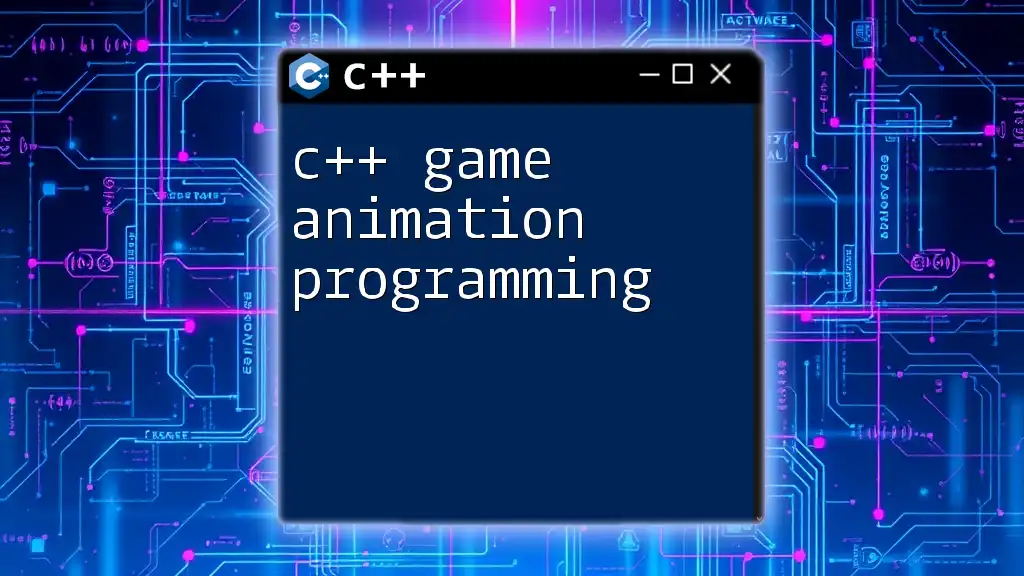
Creating a Simple 2D Animation
Setup Your First Game Window
The first hands-on aspect of C++ game animation programming involves creating a basic game window. Using SFML, you can set up a simple window like this:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "Simple Animation");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
// Draw things
window.display();
}
return 0;
}
The code above initializes a window where your animation will take place, setting the stage for a simple animation loop.
Loading and Displaying a Sprite
Next, loading and displaying a sprite serves as another fundamental step. Here’s how you can load a texture and display a sprite:
sf::Texture texture;
if (!texture.loadFromFile("sprite.png")) {
// Handle error
}
sf::Sprite sprite(texture);
window.draw(sprite);
In this code snippet, the texture is loaded from a file while the sprite utilizes the loaded texture for rendering. This is crucial for bringing animated characters and objects to life.
Animating the Sprite
With the sprite in place, you can animate it by updating its position. For instance, to move the sprite continuously across the screen, integrate the following code in your main loop:
sprite.move(1, 0); // Move right
This command shifts the sprite to the right along the x-axis, illustrating a basic animation effect.
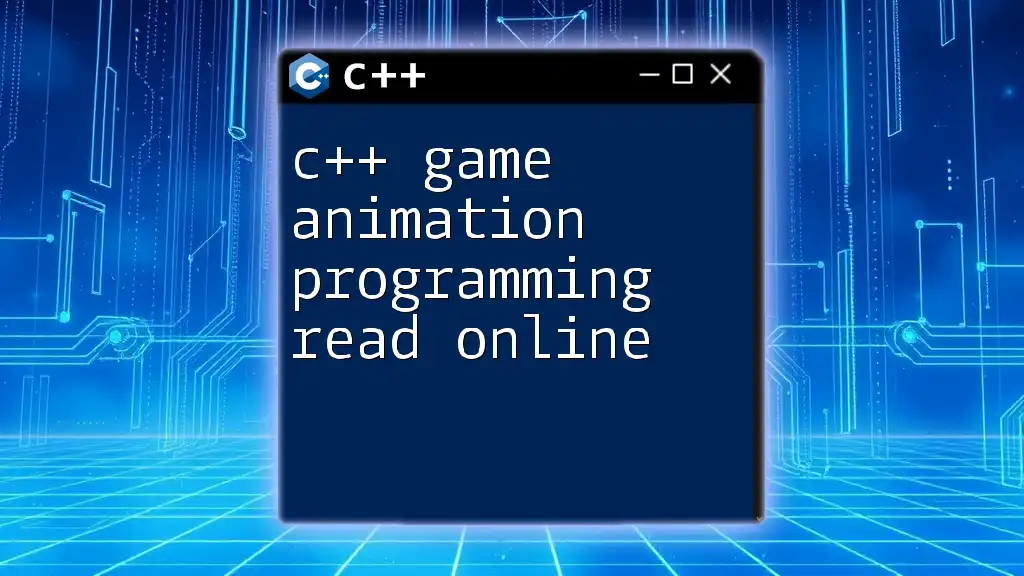
Implementing Frame-Based Animation
Creating Animation Frames
To create a more complex animation, you will need multiple frames for a sprite. Organizing these frames in a sprite sheet ensures efficient memory usage and performance.
Switching Between Frames
To enable switching between frames for your sprite animation, consider the following logic:
int currentFrame = 0;
// Assuming 4 frames
sprite.setTextureRect(sf::IntRect(currentFrame * spriteWidth, 0, spriteWidth, spriteHeight));
currentFrame = (currentFrame + 1) % 4; // Loop through frames
Here, the texture rectangle for the sprite is updated based on the `currentFrame` variable, allowing you to loop through multiple frames efficiently, creating the illusion of movement.
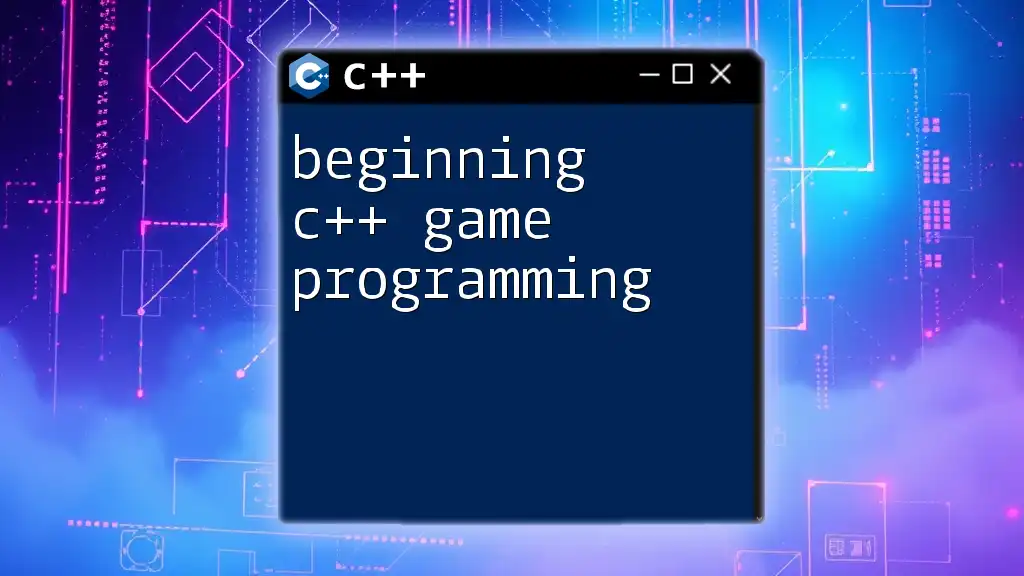
Advanced Animation Techniques
Skeletal Animation
To elevate your animation skills, skeletal animation introduces the concept of using a rig to control movements. Engines like Assimp and Cocos2d-x support skeletal animations, allowing for more flexible and realistic motion than traditional frame-by-frame techniques.
Tweening and Interpolation
Another advanced method is using tweening, which smoothly transitions properties over time. For example:
float currentValue = 0.0f;
float targetValue = 100.0f;
currentValue += (targetValue - currentValue) * 0.1f; // Basic easing
This code snippet demonstrates a basic easing function that allows for gradual movement towards a target value, contributing to a polished animation effect.
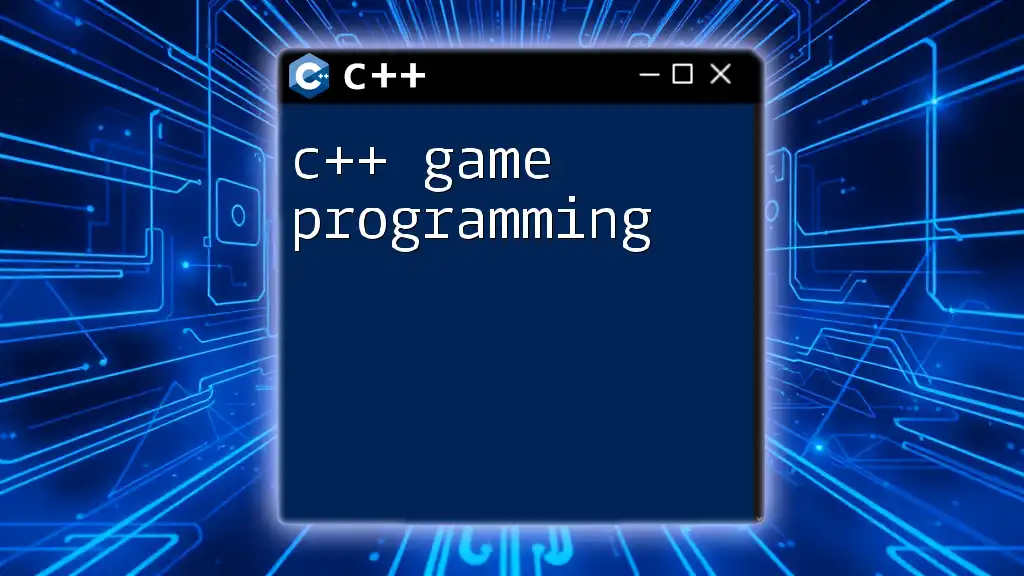
Performance Optimization
Profiling Your Animation
To ensure your animations run smoothly, it's essential to profile your application. Tools for profiling C++ applications like Valgrind or built-in profilers within IDEs can help identify bottlenecks in your animation code.
Using Efficient Rendering Techniques
Utilizing batch rendering can significantly boost performance by minimizing the number of draw calls. This approach is essential when rendering numerous objects on screen simultaneously, ensuring your game remains fast and responsive.
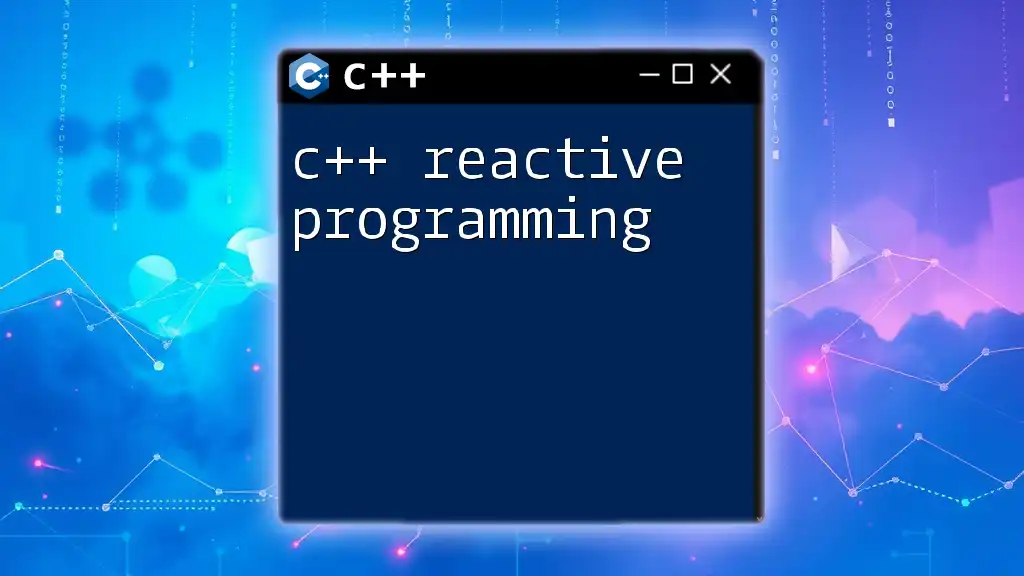
Debugging Common Animation Issues
Identifying and Fixing Bugs
As you work through hands-on C++ game animation programming, be prepared to encounter common bugs such as jittering animations or delays. Understanding how to debug these issues is crucial. Use tools like GDB and the Visual Studio Debugger to step through your code and identify the source of the problems.
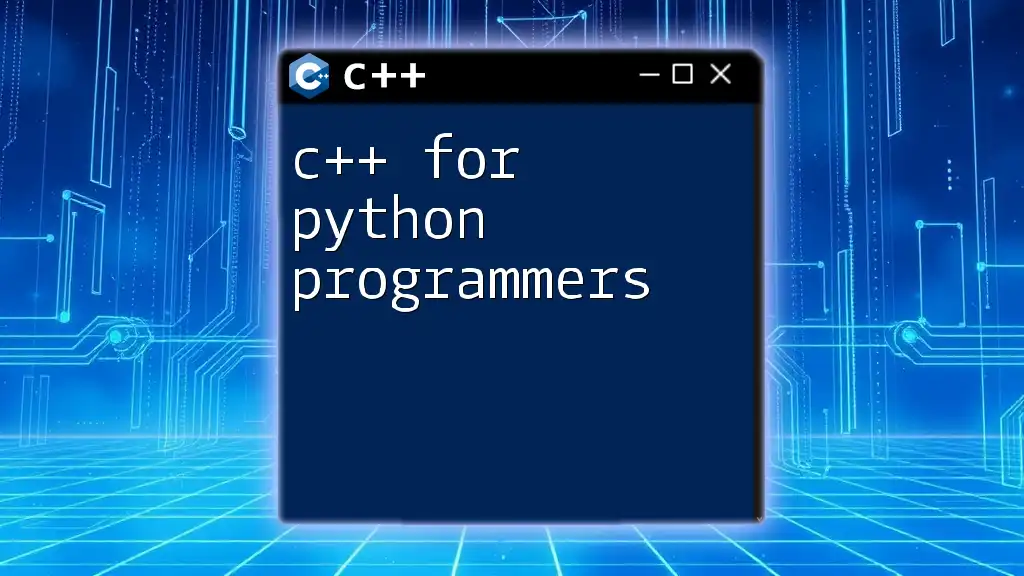
Final Thoughts and Further Learning
Embrace online resources and communities for continued learning. Courses, tutorials, and forums like GameDev.net and Stack Overflow provide avenues for deeper exploration and community support.
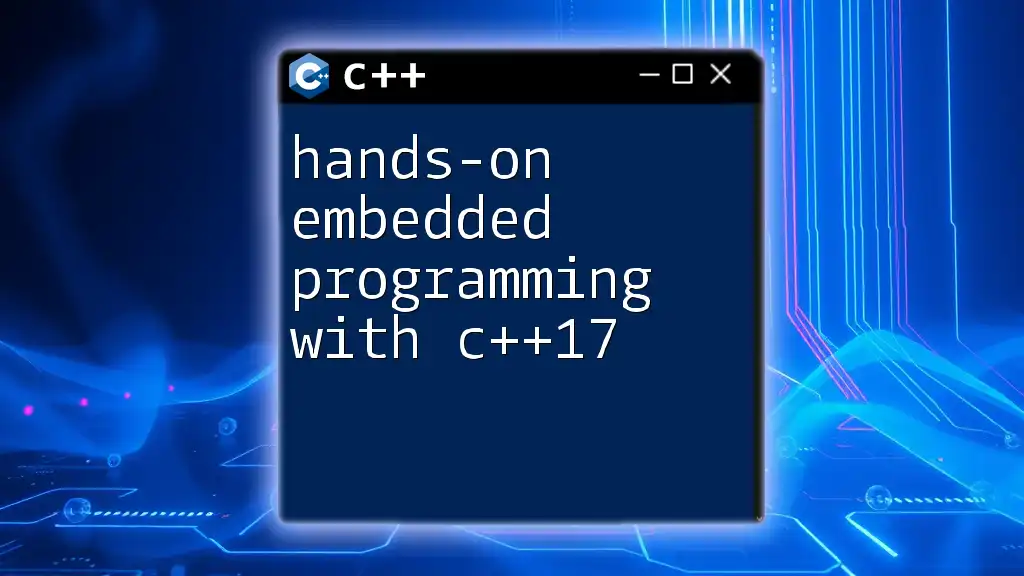
Conclusion
In this guide on hands-on C++ game animation programming, we've covered fundamental concepts, practical implementations, and advanced techniques essential for creating engaging animations in games. Practice consistently, and don’t hesitate to experiment to refine your skills further.