C++ game programming involves using the C++ language to create interactive games by leveraging its high performance and control over system resources.
#include <iostream>
int main() {
std::cout << "Welcome to C++ Game Programming!" << std::endl;
return 0;
}
Setting Up Your C++ Game Development Environment
Choosing the Right IDE
When embarking on your journey into C++ game programming, selecting the right IDE (Integrated Development Environment) is crucial. A good IDE enhances productivity with features like code completion, debugging tools, and integrated build systems. Some recommended IDEs for C++ game development include:
- Visual Studio: Widely used in the industry, especially for Windows-based games. It offers powerful debugging tools and is robust for large projects.
- Code::Blocks: A free and open-source option that is lightweight and easy to use, ideal for beginners.
- CLion: A cross-platform IDE by JetBrains that provides advanced code analysis and modern development tools.
Choosing an IDE should align with your needs and operating system preferences, ensuring that you can efficiently write and test your code.
Installing Necessary Libraries and Tools
For C++ game programming, leveraging libraries can significantly streamline game development. Here are some essential libraries:
- SFML (Simple and Fast Multimedia Library): Great for 2D games, providing functionalities for graphics, sound, and input.
- SDL (Simple DirectMedia Layer): Another option for 2D game development, widely used for developing games that run on multiple platforms.
- Unreal Engine: A more comprehensive framework for 3D game programming, known for its stunning graphics and powerful tools.
To install these libraries, follow their official documentation for setup instructions, ensuring that your development environment is ready for game creation.
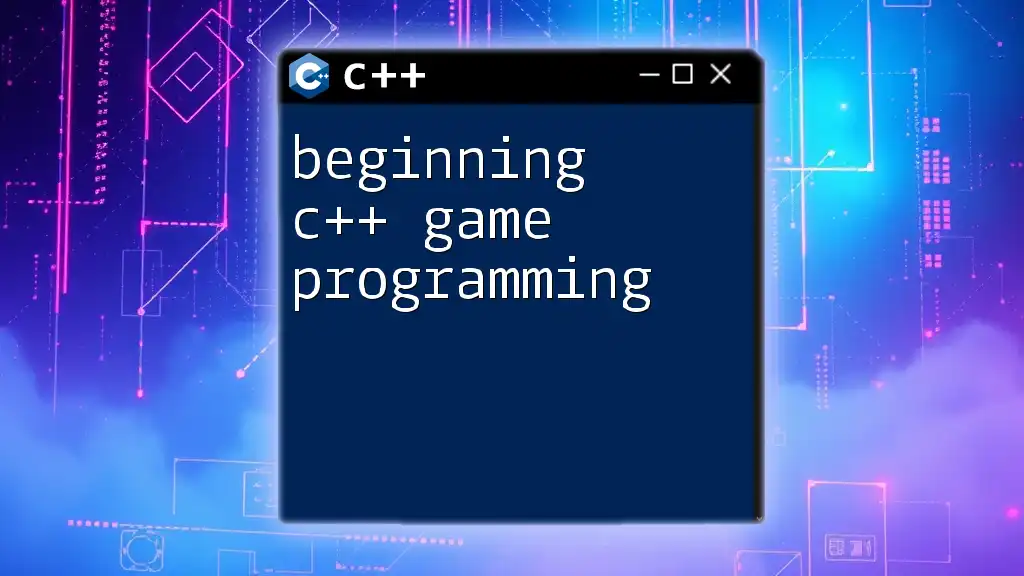
Understanding Game Development Concepts
Key Concepts in Game Development
Understanding fundamental concepts in C++ game programming is essential. Here are two core principles:
- Game Loop: This continuously running loop is responsible for managing the game state, updating object positions, handling user input, and rendering graphics on the screen.
- Events: Events are crucial in game programming for interaction, such as keyboard or mouse inputs that control player actions.
Game Design Patterns
Design patterns structure your game’s code effectively. A popular one is the Entity-Component-System (ECS) pattern, which separates the data (components) from the behaviors (systems) to facilitate better organization and flexibility.
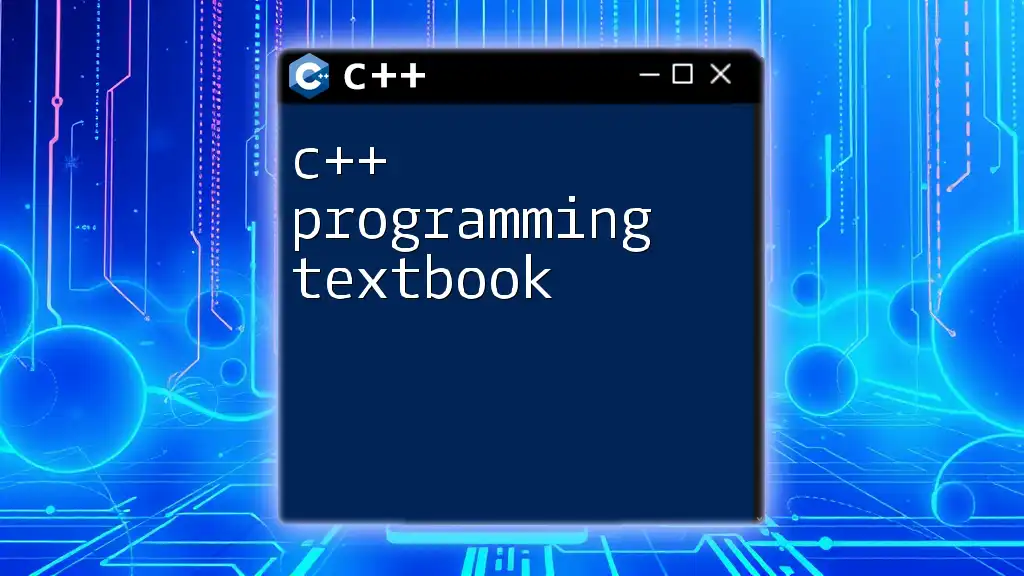
C++ Basics for Game Programming
Essential Syntax and Structures
Before diving into game development, familiarize yourself with C++ syntax and structures. Understanding variables, data types, and control structures helps lay the foundation.
Here is a simple example of a variable declaration and conditional statement:
int main() {
int playerScore = 0; // Player score variable
if (playerScore >= 100) {
// Player earns a bonus!
}
}
Object-Oriented Programming in C++
Object-oriented programming (OOP) is vital in C++ game programming. It allows you to model real-world objects as classes. A simple Player class can be represented as:
class Player {
public:
int health;
int score;
void takeDamage(int damage) {
health -= damage;
}
};
Building Your First Game
Conceptualizing Your Game
Start by brainstorming game ideas. Determine the genre (e.g., platformer, shooter) and think about what makes your game unique. Creating wireframes of levels or characters can also help visualize the gameplay.
Implementing Basic Game Mechanics
Creating a game loop is the backbone of your game. A simple structure can be like this:
while (gameIsRunning) {
processEvents();
update();
render();
}
This code continually processes user input, updates game states, and renders the graphics.
Creating Game Objects
Managing game entities is essential for gameplay. You can create a basic game object like this:
class GameObject {
public:
float x, y; // Position of the object
void move(float dx, float dy) {
x += dx;
y += dy;
}
};
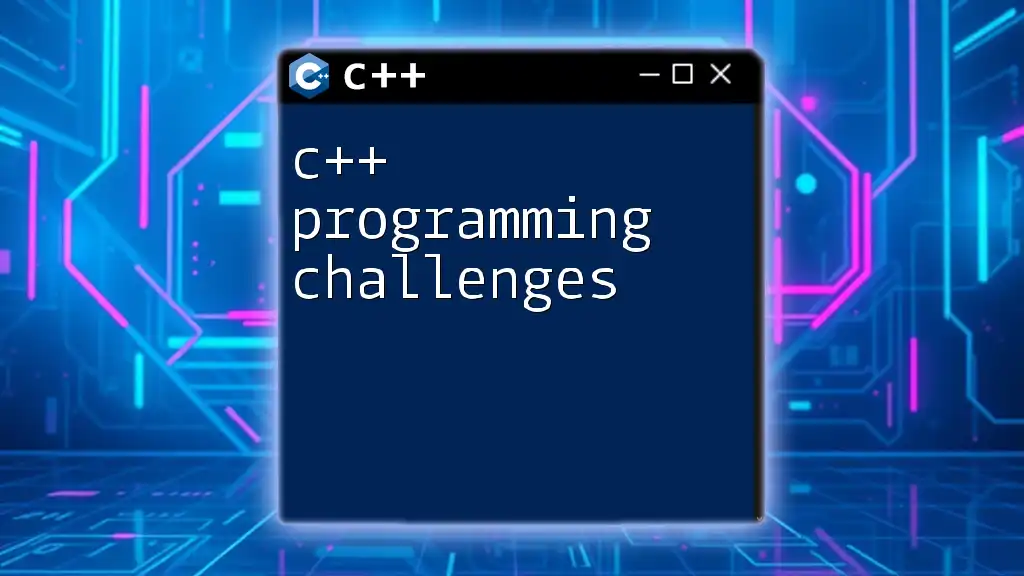
Handling Graphics and Sound
Rendering Graphics in C++
Graphics are vital for immersive gameplay. Using libraries like SFML makes rendering simple. Below is an example of creating a window and drawing a rectangle:
sf::RenderWindow window(sf::VideoMode(800, 600), "My Game");
sf::RectangleShape player(sf::Vector2f(50, 50));
player.setFillColor(sf::Color::Green);
while (window.isOpen()) {
window.clear();
window.draw(player);
window.display();
}
Implementing Sound Effects and Music
Incorporating sound adds depth to your game experience. Using SFML, you can easily integrate audio. Here’s how to load and play a sound effect:
sf::SoundBuffer buffer;
buffer.loadFromFile("sound.wav");
sf::Sound sound;
sound.setBuffer(buffer);
sound.play();
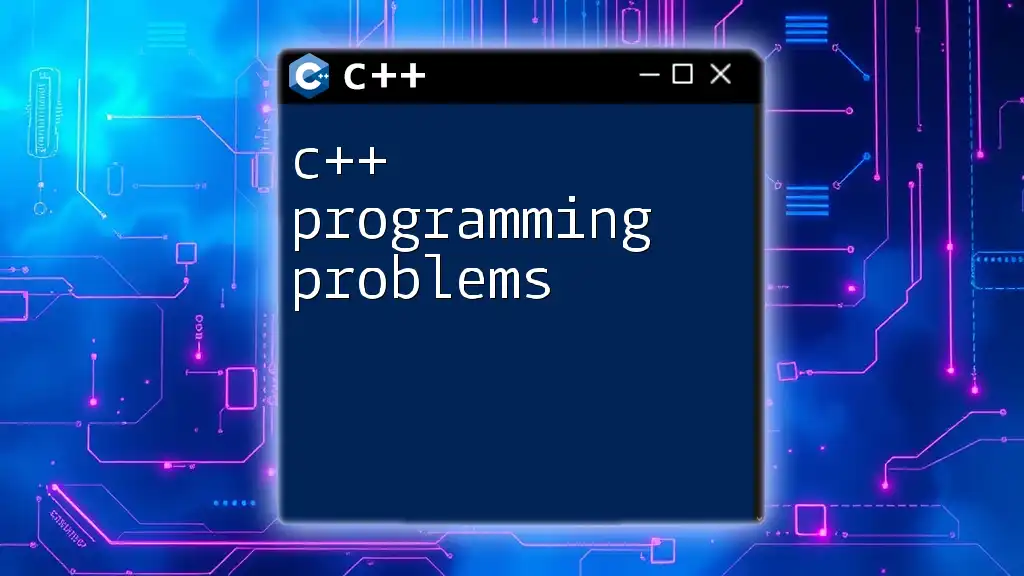
Managing Game States
Understanding Game States
Different game states (e.g., menu, playing, paused, game over) dictate the game’s flow and user experience. Knowing how to handle these states is crucial for smooth gameplay transitions.
Implementing State Management
You can implement game state management using an enum for clarity in your code:
enum class GameState { MENU, PLAYING, PAUSED, GAME_OVER };
GameState currentState = GameState::MENU;
void update() {
switch (currentState) {
case GameState::MENU:
// Handle menu updates
break;
case GameState::PLAYING:
// Handle gameplay updates
break;
// Additional cases...
}
}
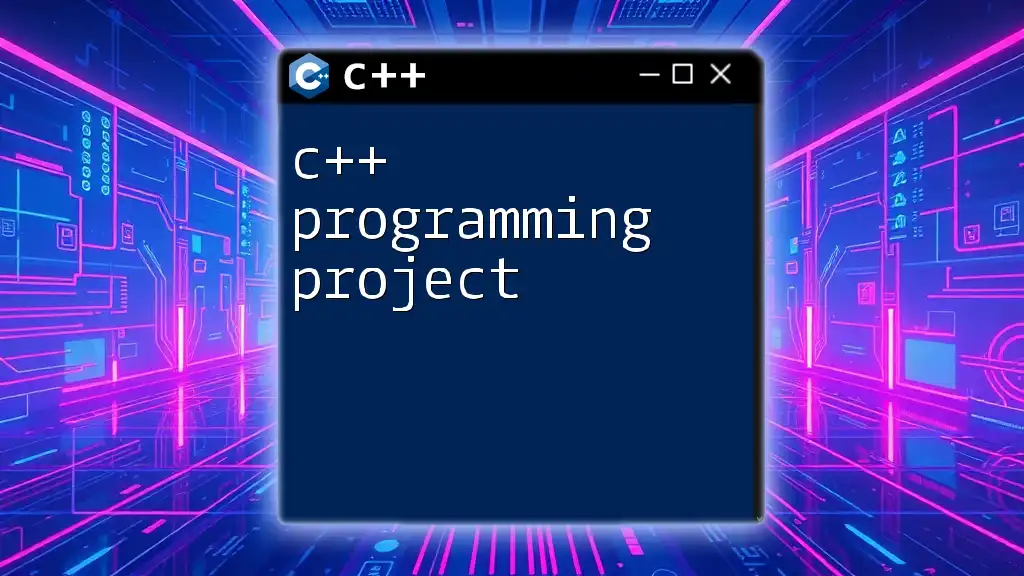
Debugging and Optimizing Your Game
Common Debugging Techniques
Debugging is an inevitable part of programming. Familiarize yourself with debugging tools integrated into your IDE, and consider print statements for tracking variable states to find bugs effectively.
Optimization Strategies
Optimizing game performance ensures a smooth player experience. Focus on identifying performance bottlenecks and refining your algorithms. Techniques such as efficient memory management and game object pooling (reusing objects instead of creating new ones) can greatly improve performance.
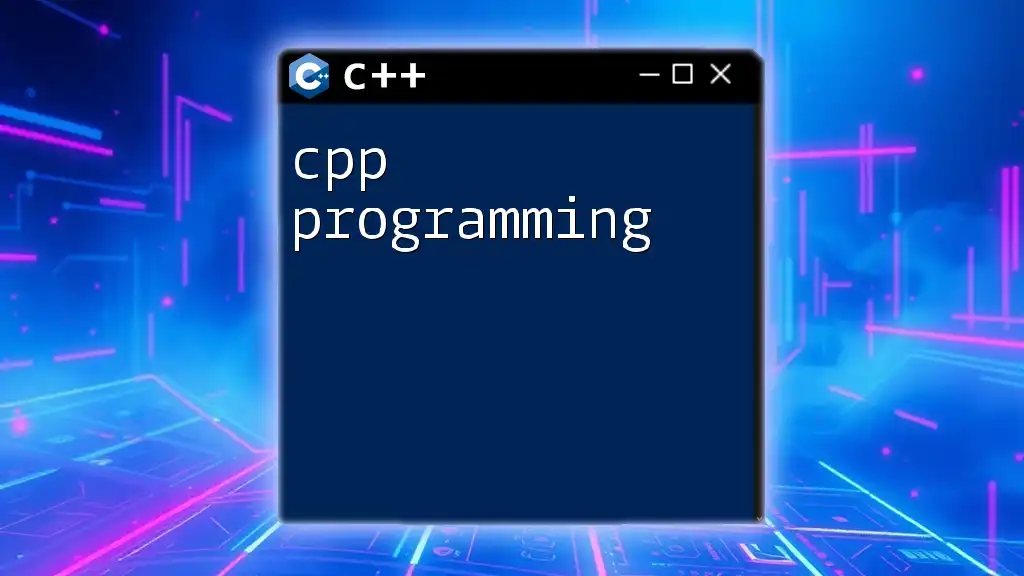
Conclusion
In summary, mastering C++ game programming requires understanding foundational concepts, choosing the right tools, and practicing coding techniques. As you progress, you can develop more complex games and explore the vast possibilities that C++ offers in game development.
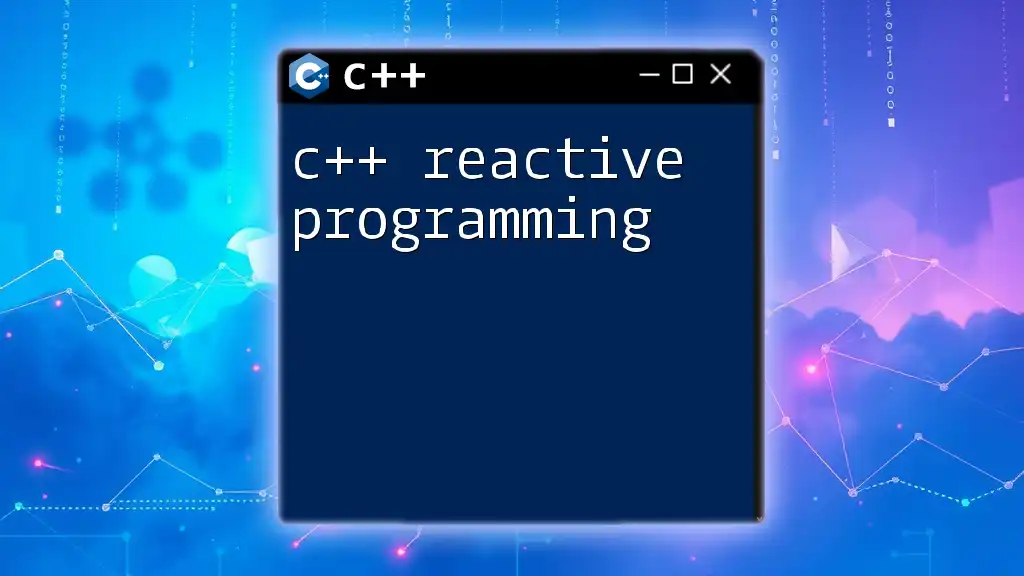
Resources for Further Learning
To deepen your knowledge, consider exploring books, online courses, and forums dedicated to C++ and game development. Engaging with the community can provide valuable insights and support.
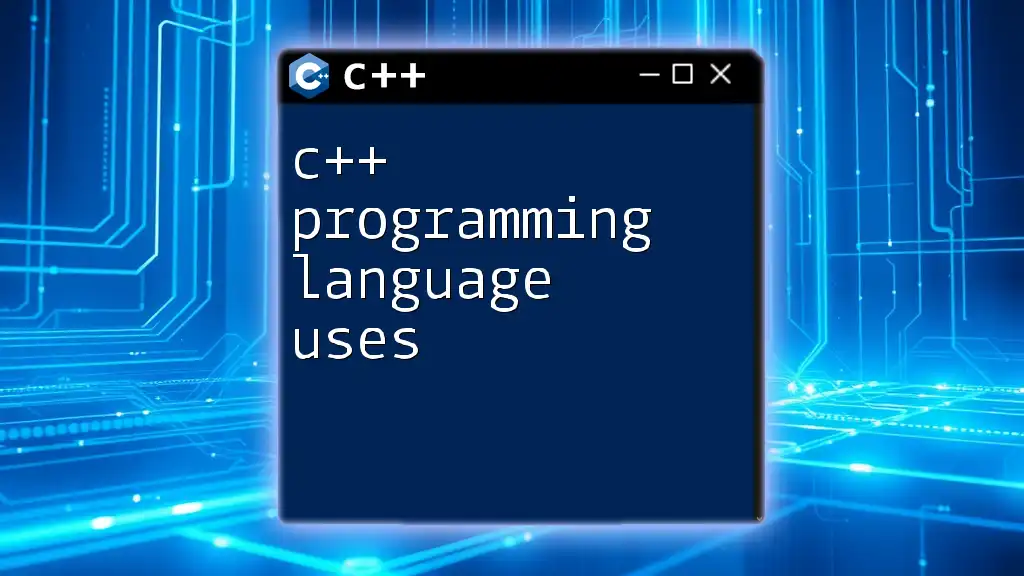
Call-to-Action
Join our company to enhance your skills in C++ commands and game programming. Our structured learning approach ensures you build a solid foundation and advance your knowledge efficiently.