Meta programming in C++ is a programming technique that allows you to write code that manipulates code, typically at compile-time, enabling powerful abstractions and optimizations.
#include <iostream>
#include <type_traits>
template <typename T>
struct IsPointer {
static const bool value = false;
};
template <typename T>
struct IsPointer<T*> {
static const bool value = true;
};
int main() {
std::cout << "Is int a pointer? " << IsPointer<int>::value << std::endl; // Output: 0
std::cout << "Is int* a pointer? " << IsPointer<int*>::value << std::endl; // Output: 1
return 0;
}
What is Meta Programming?
Meta programming is a programming paradigm where programs can be treated as their own data. In the context of C++, meta programming allows for the writing of code that manipulates other code at compile-time or runtime. This results in increased efficiency and flexibility, allowing developers to create more dynamic and reusable components.
Understanding meta programming is crucial as C++ continues to evolve into a powerful tool for developers. By utilizing meta programming, programmers can significantly enhance the performance and maintainability of their code.
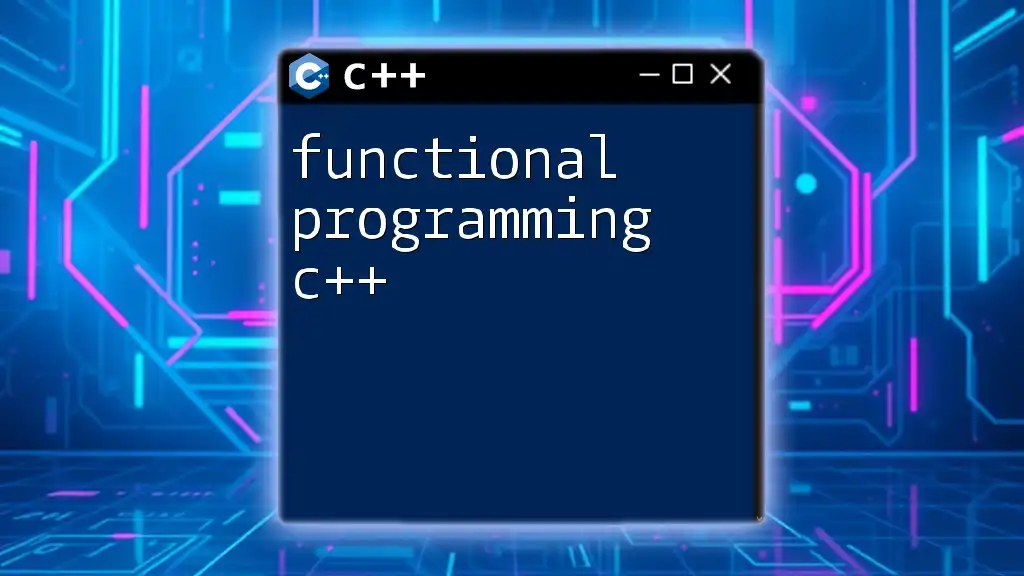
Why Use Meta Programming in C++?
Using meta programming in C++ offers several advantages:
- Performance Benefits: By performing computations at compile-time, meta programming reduces runtime overhead, leading to faster and more efficient applications.
- Code Reuse and Maintenance: Meta programming encourages a DRY (Don't Repeat Yourself) approach, allowing developers to create generic solutions that can be utilized across various applications.
- Enhanced Type Safety: Through compile-time checks and constraints, meta programming provides a higher degree of type safety, catching errors early in the development process.
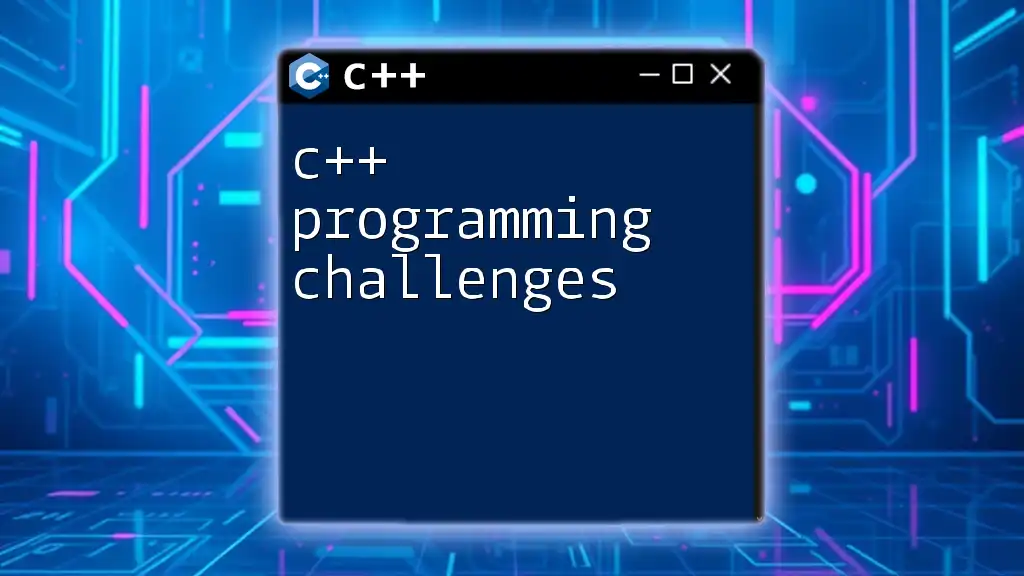
Understanding C++ Metaprogramming
Defining C++ Metaprogramming
C++ metaprogramming is a technique that enables developers to leverage the power of C++ templates and compile-time computations. This allows for the creation of algorithms that run during compilation rather than at runtime.
Core Concepts in C++ Metaprogramming
Templates Overview:
Templates are the cornerstone of meta programming in C++. Both function templates and class templates allow developers to write generic and reusable code. When properly utilized, templates can adapt to various data types while ensuring type safety.
Type Traits:
Type traits are a collection of templates that help manipulate types at compile-time. They can provide information about types, enabling developers to distinguish between different types without incurring performance penalties.
For example, common type traits include `std::is_integral`, which checks if a type is an integer:
#include <type_traits>
static_assert(std::is_integral<int>::value, "int should be integral type");
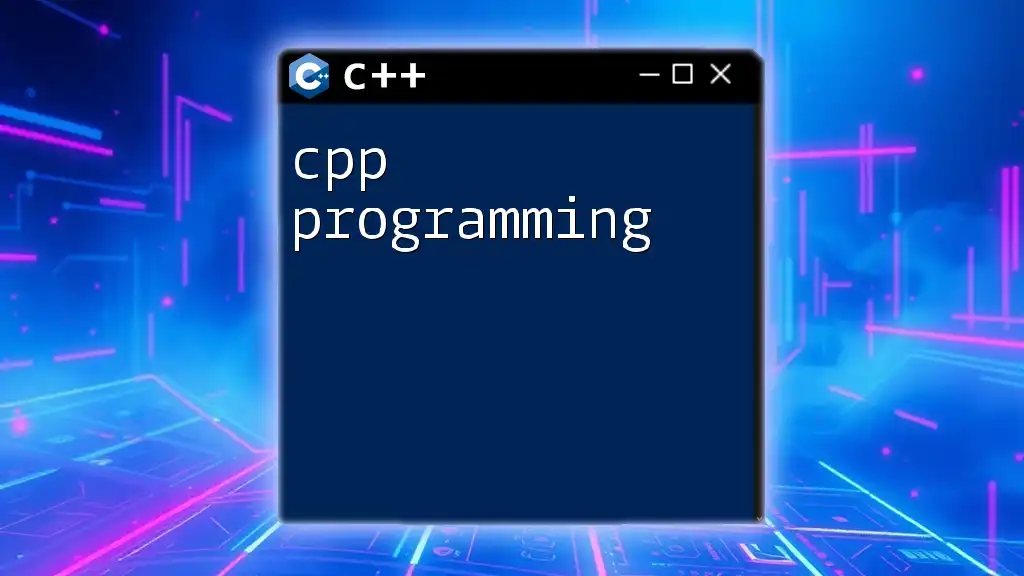
Template Metaprogramming with C++
What is Template Metaprogramming?
Template metaprogramming refers to the use of C++ templates to perform computations at compile-time. Unlike conventional programming, where calculations are executed at runtime, template metaprogramming shifts this burden to the compilation phase, allowing developers to optimize their code for performance.
Core Principles of Template Metaprogramming
Recursive Templates:
Recursion is a powerful tool in template metaprogramming. Templates can call themselves to perform repetitive tasks, creating compilations that leverage the recursive structure of the templates.
For instance, a recursive template for calculating the factorial of a number can look like this:
template<int N>
struct Factorial {
static const int value = N * Factorial<N - 1>::value;
};
template<>
struct Factorial<0> {
static const int value = 1;
};
In this example, `Factorial<5>::value` will evaluate to `120` at compile-time.
Template Specialization:
Template specialization allows developers to customize the behavior of templates for specific types or values. This technique is essential, as it enables you to provide tailored implementations when necessary.
Consider the example below, which demonstrates specialization for an integer type:
template<typename T>
struct PrintType;
template<>
struct PrintType<int> {
static void print() { std::cout << "This is an int." << std::endl; }
};
When `PrintType<int>::print()` is called, it produces the output "This is an int."
Advanced Techniques in Template Metaprogramming
SFINAE (Substitution Failure Is Not An Error):
SFINAE is a principle in C++ where the compiler does not generate errors when substituting invalid template arguments; rather, it simply ignores those substitutions. This crucial property allows developers to create more flexible and adaptive templates.
Here’s a practical implementation demonstrating SFINAE:
#include <iostream>
#include <type_traits>
template<typename T>
typename std::enable_if<std::is_integral<T>::value, void>::type
process(T value) {
std::cout << "Processing integral type: " << value << std::endl;
}
When calling `process(5)`, it will execute successfully, whereas passing a non-integral type (like `std::string`) will result in a substitution failure, allowing for better flexibility in templates.
Variadic Templates:
Variadic templates allow developers to define templates that can accept an arbitrary number of template parameters. This powerful feature can simplify code and enable the creation of highly flexible functionality.
Here's how variadic templates can be utilized:
#include <iostream>
template<typename... Args>
void print(Args... args) {
(std::cout << ... << args) << '\n';
}
In this example, `print(1, 2.5, "Hello")` can be called, and the output would be "12.5Hello," demonstrating how multiple types can be handled seamlessly.
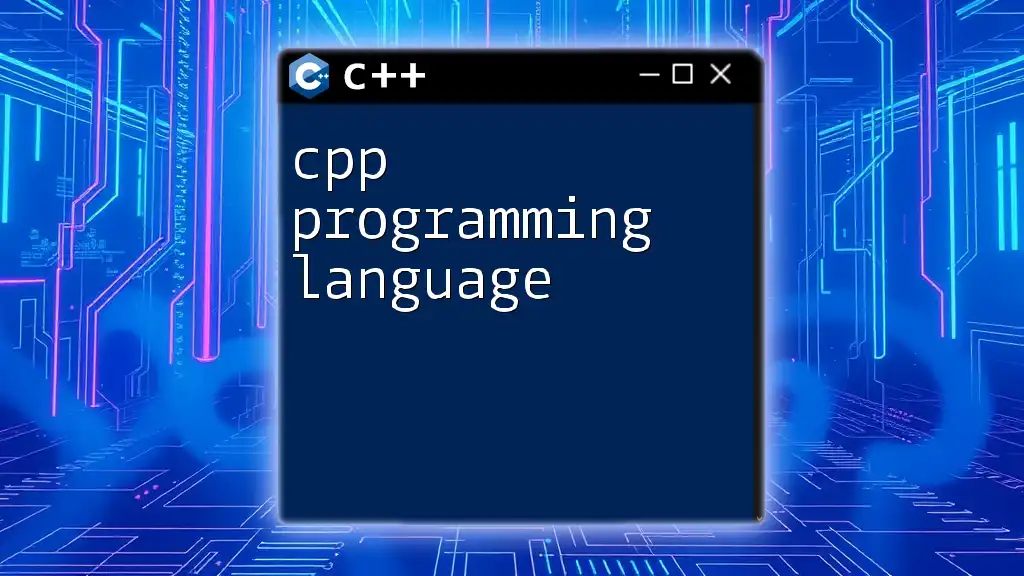
Practical Applications of C++ Metaprogramming
Compile-Time Computation
One of the most significant advantages of C++ meta programming is the ability to perform computations at compile-time. This capability can lead to more optimized code.
An example can be seen in calculating Fibonacci numbers:
template<int N>
struct Fibonacci {
static const int value = Fibonacci<N - 1>::value + Fibonacci<N - 2>::value;
};
template<>
struct Fibonacci<0> { static const int value = 0; };
template<>
struct Fibonacci<1> { static const int value = 1; };
Here, `Fibonacci<5>::value` calculates the fifth Fibonacci number during compilation, resulting in the computation `5`.
Building Type-Safe Container Classes
Meta programming can also be leveraged to create type-safe collection classes. For instance, a generic container class can be designed to accept only specific data types, ensuring that runtime type errors are minimized.
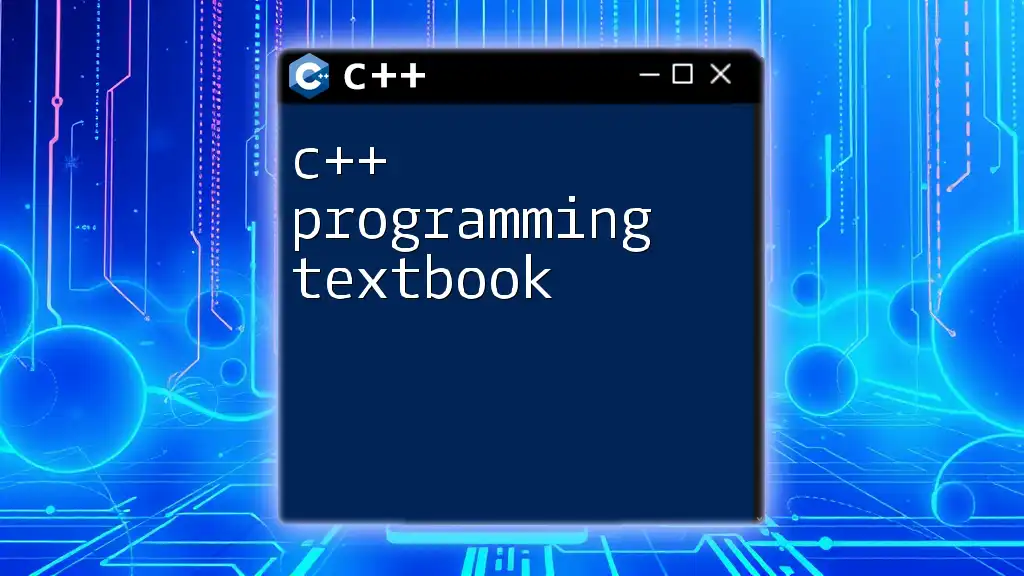
The Future of Meta Programming in C++
As C++ continues to evolve, the future of meta programming in C++ looks promising. New standards like C++20 and the potential for further enhancements will undoubtedly create more opportunities for using meta programming to write cleaner, faster, and more efficient code.
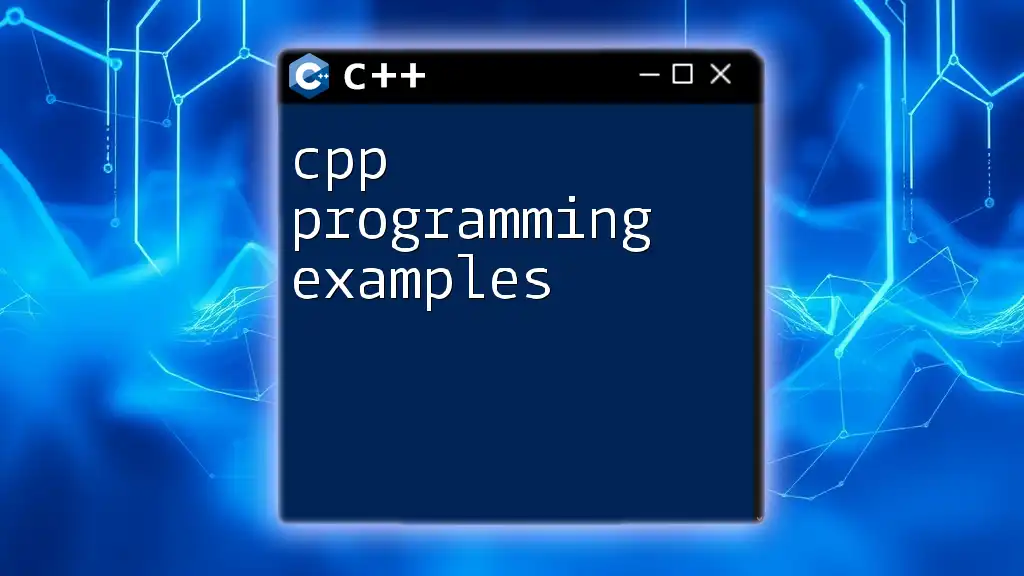
Final Thoughts on Learning Metaprogramming in C++
Learning meta programming in C++ requires practice and experimentation. The ability to manipulate types, perform computations at compile-time, and create more robust code can dramatically improve overall software quality.
Continuous learning and exploration of this powerful feature in C++ will yield significant benefits in both productivity and performance.
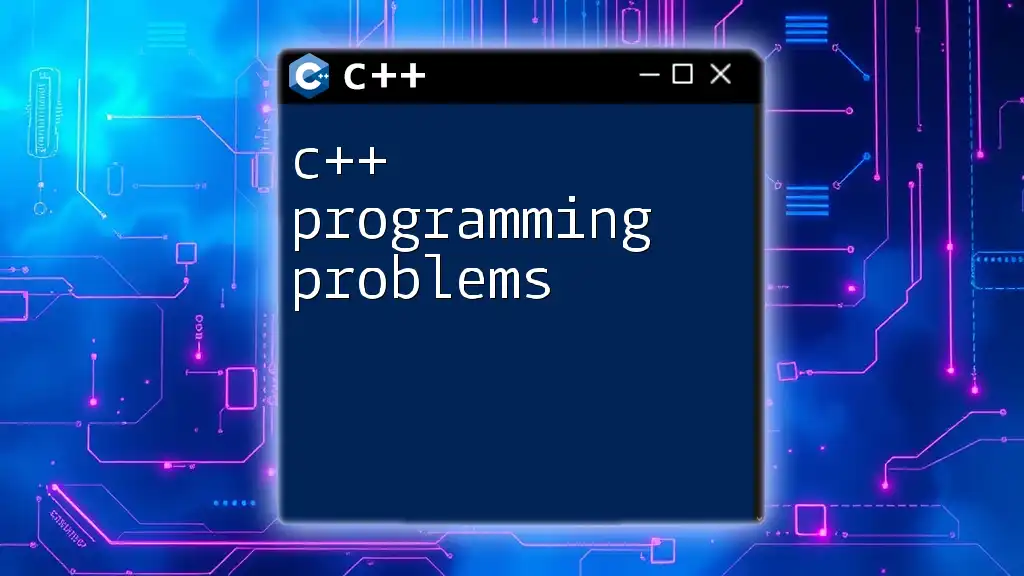
Learn More About C++ Metaprogramming
Embark on your journey to mastering C++ metaprogramming. Engage with interactive courses, workshops, and hands-on tutorials to refine your skills and expand your understanding of this advanced C++ feature.
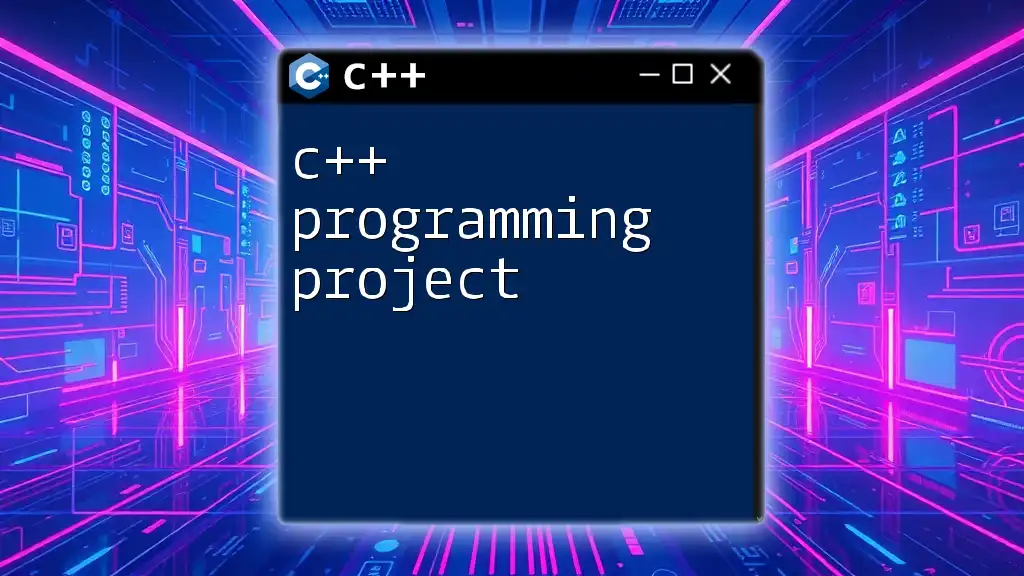
Recommended Books and Online Resources
Explore literature and websites dedicated to C++ meta programming to deepen your insights and encourage further practice. Make use of these resources to stay updated with the latest trends and techniques in meta programming.