To program in C++, you need to write code using syntax that defines variables, functions, and control structures, as illustrated in the following example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++
What is C++?
C++ is a powerful and versatile programming language that was developed by Bjarne Stroustrup at Bell Labs in the early 1980s. It is an extension of the C programming language, adding object-oriented features, which has made it widely adopted for system/software development, game programming, and more. C++ combines the efficiency and control of C with features that support abstraction and encapsulation.
Why Learn C++?
Learning C++ is invaluable due to its vast applicability across several fields. Here are some reasons why you should consider diving into this language:
- Versatility: C++ is used in various domains, from game development (like using Unreal Engine) to systems programming and scientific applications.
- Career Opportunities: Due to its extensive use in performance-critical applications, organizations value developers with C++ skills. In today’s job market, proficiency in C++ can help you stand out and provide numerous career pathways.
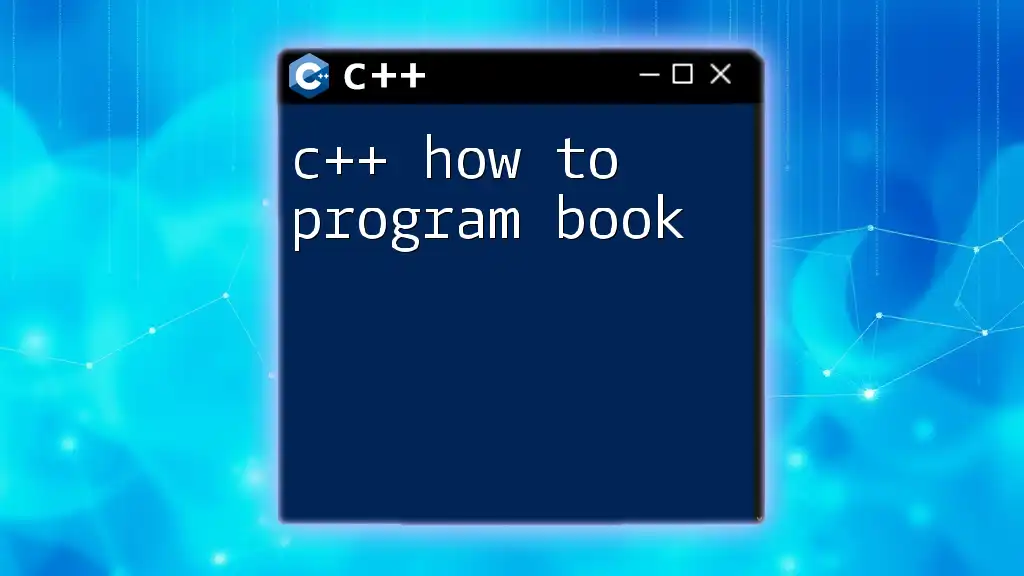
Getting Started with C++
Setting Up Your Development Environment
To effectively learn how to program C++, it's essential to set up your development environment properly.
-
Choose an Integrated Development Environment (IDE): Popular choices include Visual Studio, Code::Blocks, and Eclipse. Each of these IDEs offers features that simplify coding, debugging, and project management.
-
Installation Steps: Follow the installation guide for your chosen IDE. Most IDEs provide straightforward installation processes with helpful prompts to get you started.
-
Compiler: A reliable C++ compiler, such as GCC or Clang, is crucial for translating your C++ code into executable programs. Ensure that your IDE is set to use your selected compiler properly.
Writing Your First C++ Program
Now, let’s take a step towards coding by writing a simple C++ program.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This code snippet introduces you to several key components of a C++ program:
- #include <iostream>: This line includes the Input/Output stream library, which is necessary for using `std::cout` for output.
- int main(): The main function is where program execution begins.
- std::cout: This object is used to produce output to the console.
- return 0;: This signifies that the program has executed successfully.
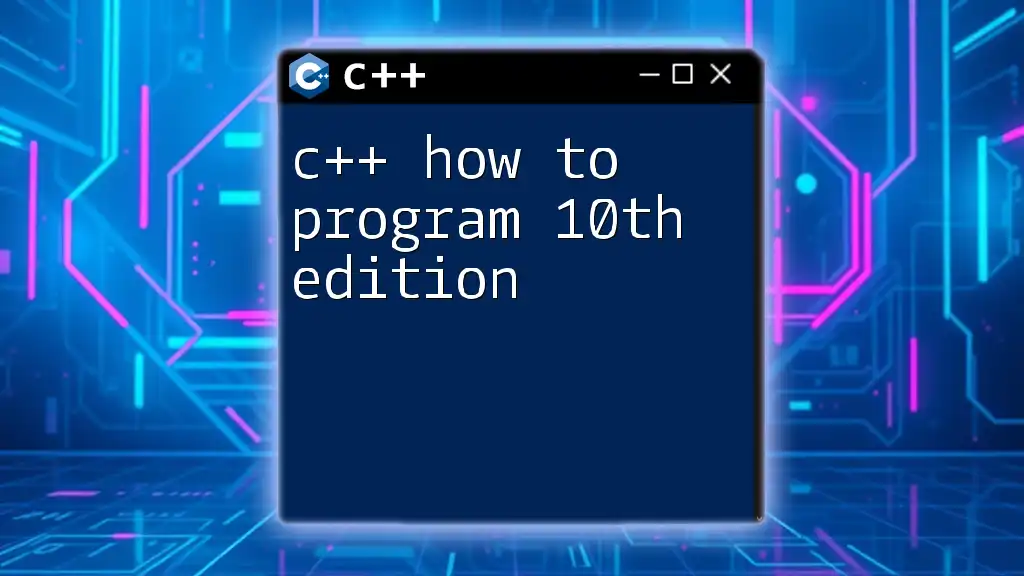
Essential C++ Concepts
Variables and Data Types
In C++, variables are essential for storing data. Each variable must have a data type, which defines the type of data it can store. Common data types include:
- int (for integers)
- float (for floating-point numbers)
- char (for single characters)
- string (for text)
Here's an example:
int age = 25;
float height = 5.9;
char initial = 'A';
std::string name = "Alice";
Operators in C++
C++ provides several types of operators that allow you to perform operations on variables.
- Arithmetic Operators: Used for basic mathematical operations (`+`, `-`, `*`, `/`).
- Comparison Operators: Useful to compare values (`==`, `!=`, `<`, `>`).
- Logical Operators: Used to perform logical operations (`&&`, `||`, `!`).
Example using a comparison operator:
int a = 10;
int b = 20;
if (a < b) {
std::cout << "A is less than B" << std::endl;
}
Control Structures
Conditional Statements
Conditional statements in C++ help branch the logic in your programs. The `if`, `else if`, and `else` statements are the most commonly used.
Consider the example:
if (age >= 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
Here, the program checks if a person’s age qualifies them as an adult.
Loops
Loops are fundamental in programming to repeat operations. C++ supports several types of loops:
- For loop: Ideal when the number of iterations is known.
- While loop: Best when iteration depends on a condition.
- Do-while loop: Similar to while, but it guarantees at least one execution.
Example of a for loop:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
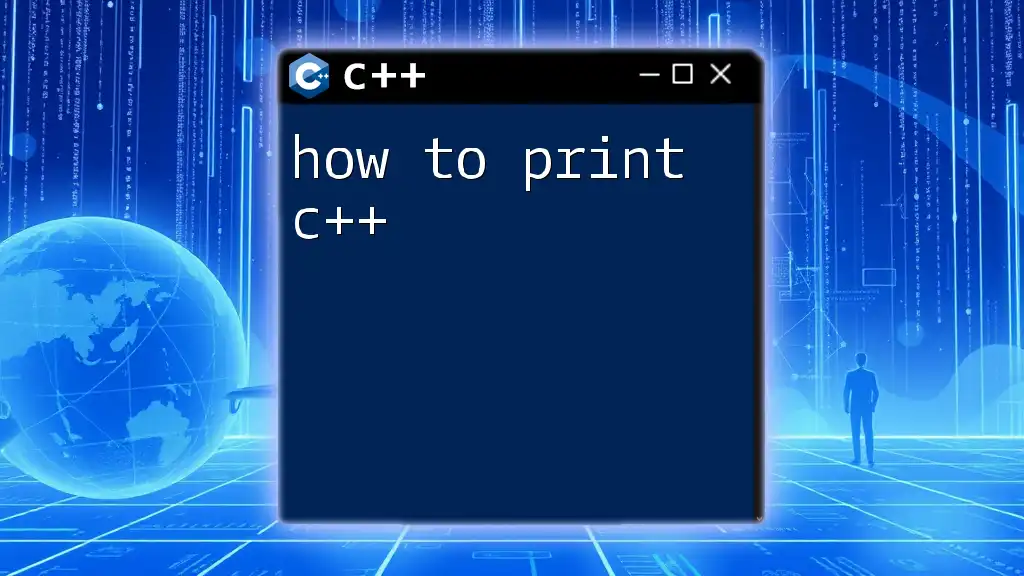
Functions in C++
Defining and Calling Functions
Functions are blocks of code designed to perform a specific task. Their use promotes code reusability and clarity.
Here's a simple function:
void greet() {
std::cout << "Hello!" << std::endl;
}
int main() {
greet();
return 0;
}
The `greet` function can be called anytime in `main`, demonstrating modularity.
Function Overloading
C++ allows you to define multiple functions with the same name but different parameters, a feature known as function overloading.
Example:
void display(int number) {
std::cout << "Number: " << number << std::endl;
}
void display(double number) {
std::cout << "Double: " << number << std::endl;
}
In this scenario, two `display` functions serve different data types.
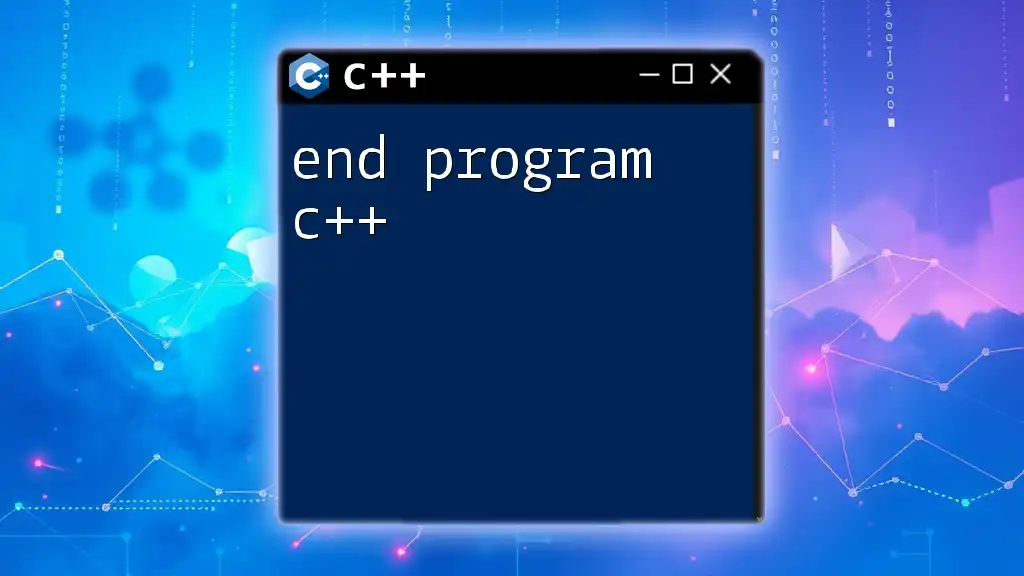
Object-Oriented Programming in C++
Principles of OOP
Object-Oriented Programming (OOP) is a paradigm that focuses on using objects to model real-world concepts, emphasizing four main principles:
- Encapsulation: Bundling data and methods that operate on that data.
- Inheritance: Allowing one class to inherit properties from another.
- Polymorphism: The ability to treat objects of different classes through a common interface.
- Abstraction: Hiding complex implementation details and showing only essential features.
Creating Classes and Objects
In C++, classes serve as blueprints for creating objects.
Here’s how you can define a class:
class Car {
public:
std::string brand;
void honk() {
std::cout << "Beep! Beep!" << std::endl;
}
};
int main() {
Car myCar;
myCar.brand = "Toyota";
myCar.honk();
return 0;
}
In this example, the `Car` class has a public variable `brand` and a method `honk`. An instance of the class, `myCar`, is created and used.
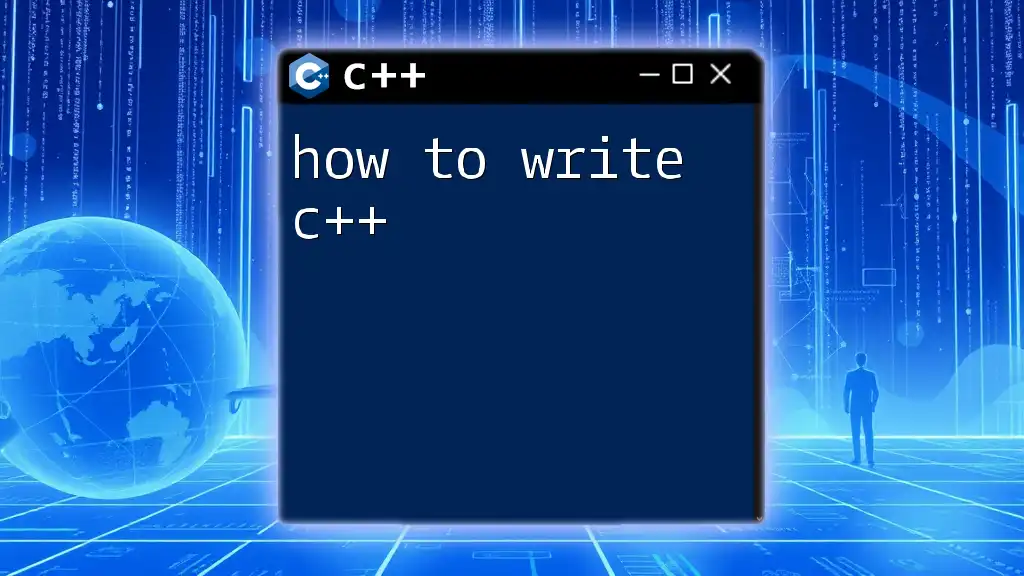
Working with C++ Libraries
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful library in C++ that provides generic classes and functions. It includes components like vectors, lists, and algorithms, which greatly simplify complex tasks.
For instance, using `vector` from STL:
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << std::endl;
}
}
STL can significantly optimize the efficiency of your code by providing well-tested algorithms and data structures.
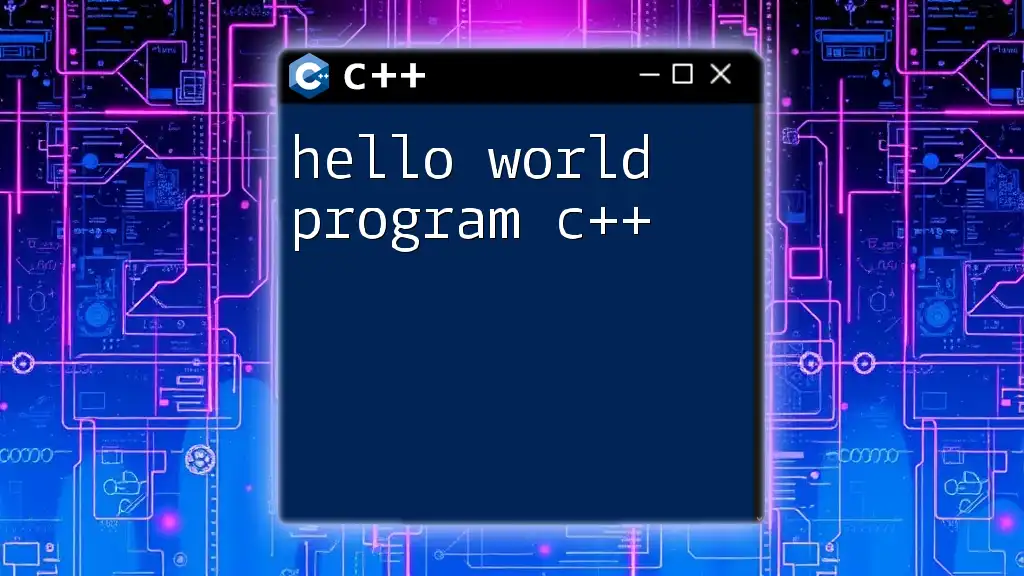
Debugging and Best Practices
Common Debugging Techniques
Debugging is an essential skill. Here are some techniques to effectively diagnose issues in your code:
- Print Statements: Use `std::cout` to display variable values.
- Debuggers: Use tools that let you step through code line by line, watch variables, and set breakpoints.
Best Practices in C++ Programming
Keep your code clean and maintainable with these best practices:
- Code Readability: Use meaningful variable names, indentation, and spacing to enhance readability.
- Comments: Include comments judiciously to explain complex logic.
- Consistent Naming Conventions: Choose a naming convention (camelCase, snake_case, etc.) and stick to it throughout your code.
- Error Handling Techniques: Use exceptions and checks to gracefully handle errors.
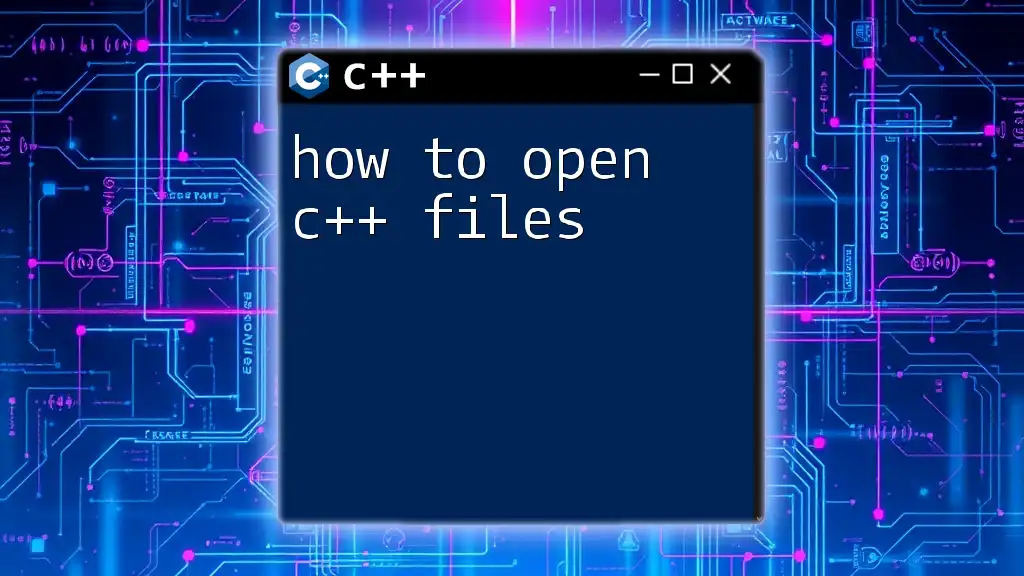
Conclusion
In this guide, we've covered the fundamentals of how to program C++ — from understanding its basic structure to implementing essential programming techniques. Mastery of C++ opens doors to a range of opportunities in modern technology fields. Keep practicing and exploring further resources to continue enhancing your skills in this powerful programming language.