Here's a concise explanation along with a code snippet in markdown format:
When selecting a C++ programming book, it's essential to find one that emphasizes practical examples, allowing you to quickly grasp commands and implement them effectively.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Programming
What is C++?
C++ is a powerful programming language that was created as an extension of the C programming language. It combines the efficiency and flexibility of C with the object-oriented features that facilitate software design. Since its inception in the early 1980s, C++ has become a cornerstone in the world of software development, widely used across sectors such as game development, systems programming, and high-performance applications.
Key Features of C++
C++ boasts several key features that make it a formidable programming language:
-
Object-Oriented Programming (OOP): C++ supports concepts like encapsulation, inheritance, and polymorphism, allowing programmers to build reusable and maintainable code.
-
Standard Template Library (STL): STL provides a rich set of algorithms and data structures, enhancing productivity and performance in development.
-
Portability and Performance: C++ code can be compiled on various platforms, and its performance is often compared to lower-level languages like C, making it suitable for high-performance software.
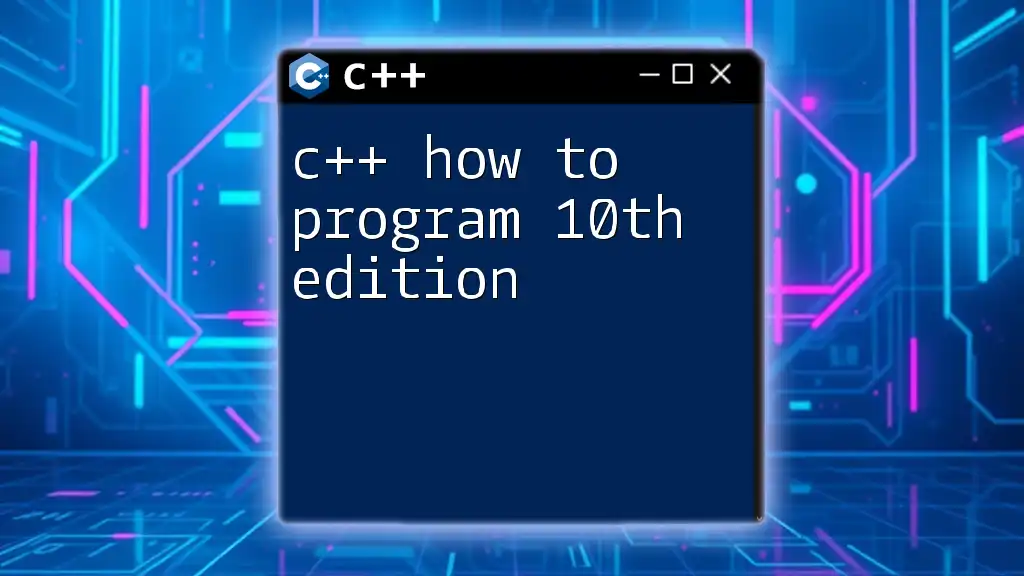
Types of C++ Programming Books
Beginner-Friendly Books
Beginner books focus on foundational knowledge and practical application. They typically cover crucial topics such as:
-
Fundamentals: Basic syntax, data types, and variable declaration.
-
Basic I/O: Methods for performing input and output operations, enabling users to interact with their programs.
Some recommended beginner books include:
-
"C++ Primer" by Lippman, Lajoie, and Moo: This book combines theory with practical exercises, guiding readers from basic concepts to more complex practices.
-
"Programming: Principles and Practice Using C++" by Bjarne Stroustrup: Written by the creator of C++, this book emphasizes programming as a discipline, making it ideal for novices looking to build their skills from the ground up.
Intermediate C++ Books
As learners progress, they encounter more complex topics that refine their skills. Intermediate books often cover:
-
Memory Management: Understanding pointers, dynamic memory allocation, and resource management is critical at this level.
-
OOP Concepts: Further exploration of classes, inheritance, polymorphism, and design patterns that optimize code reuse and structure.
Suggested books for intermediate learners include:
-
"Effective C++" by Scott Meyers: This book offers practical advice on how to use C++ effectively. It presents 55 specific ways to improve your programs and designs, focusing on common pitfalls.
-
"C++ Programming Language" by Bjarne Stroustrup: Providing a comprehensive view of C++, this book is perfect for those wanting to deepen their understanding through detailed explanations and examples.
Advanced C++ Books
For experienced programmers looking to specialize or master intricate topics, advanced books are invaluable. These works focus on areas such as:
-
Templates and their Uses: Mastery of generic programming can significantly increase the reusability of code in C++.
-
Concurrency: Understanding multi-threading, synchronization, and concurrent data structures is essential for high-performance applications.
Recommended advanced resources include:
-
"C++ Concurrency in Action" by Anthony Williams: This book provides essential insights into writing concurrent applications in C++ and offers practical examples to illustrate concepts.
-
"Modern C++ Design" by Andrei Alexandrescu: Focusing on advanced programming techniques, it explores design patterns that harness C++'s capabilities effectively.
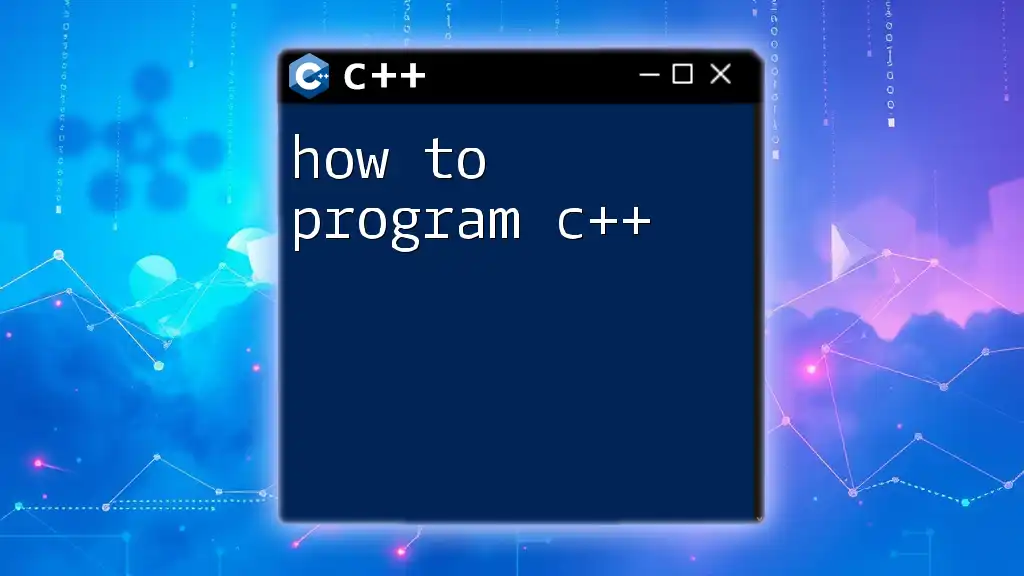
What to Look for in a C++ Programming Book
Clear and Concise Explanations
A good C++ programming book should offer clear and concise explanations that break down complex concepts into digestible portions. Books that excel in this aspect help prevent misunderstandings that can arise from technical jargon or overly complicated explanations. A well-structured narrative will guide learners progressively and logically through the subject matter.
Practical Examples and Exercises
It's vital for a programming book to contain numerous practical examples and exercises. This emphasis on practice ensures that readers can apply what they learn through active engagement, enabling them to solidify their grasp of the material. The best books provide exercises that challenge the reader to think critically and apply their knowledge in real-world scenarios.
Up-to-Date Content
In the rapidly evolving field of technology, staying current is essential. Look for books that reflect recent standards of C++ (like C++11, C++14, C++17, and C++20) which introduce modern features and best practices. Books that are frequently updated or have new editions cater to contemporary programming needs and methodologies.
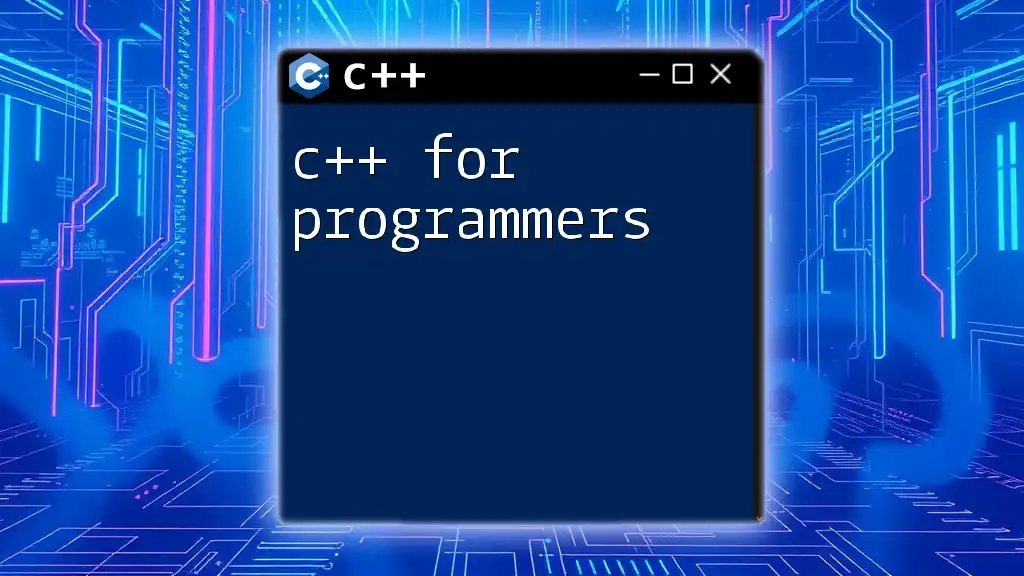
Recommended C++ Programming Books for Different Levels
Top Beginner Books
-
"C++ Primer" by Lippman, Lajoie, and Moo: Comprehensive and structured, it supports learners through a series of challenges and reinforces concepts with effective explanations.
-
"Programming: Principles and Practice Using C++" by Bjarne Stroustrup: Its approach to introducing programming through projects and conceptual topics makes it an excellent start for new programmers.
Top Intermediate Books
-
"Effective C++" by Scott Meyers: This resource is indispensable for those wishing to deepen their knowledge of C++ and write cleaner, more efficient code.
-
"C++ Programming Language" by Bjarne Stroustrup: As a definitive guide to C++, it provides deliberate detail and thorough explanations for intermediate learners.
Top Advanced Books
-
"C++ Concurrency in Action" by Anthony Williams: Readers gain robust technical knowledge that they can apply directly in programming for multi-threaded applications.
-
"Modern C++ Design" by Andrei Alexandrescu: It encourages a deeper understanding of design patterns and concepts that enhance performance and maintainability.
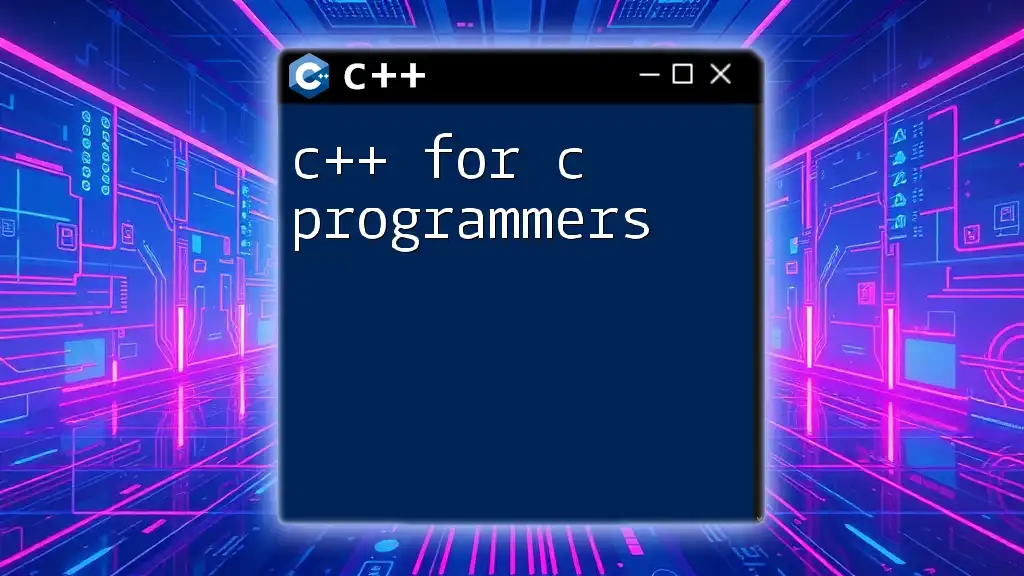
How to Study From C++ Programming Books Effectively
Creating a Study Plan
Establishing a structured study plan is crucial for effective learning. Define clear objectives, outline a timetable, and allocate specific time blocks for reading, exercises, and practical implementations. This approach fosters disciplined learning and clear progress tracking, allowing you to reach your C++ learning goals systematically.
Supplementing Your Learning
Books are incredibly beneficial, but supplementing your study with online resources can enrich your understanding. Websites featuring interactive tutorials, video lectures, and coding challenges are excellent for solidifying concepts learned from books. Online platforms like Codecademy and Coursera offer courses tailored to different levels of C++ expertise.
Joining Coding Communities
Engaging with fellow learners and industry professionals through coding communities can provide additional support and motivation. Platforms like Stack Overflow and GitHub allow you to ask questions, share ideas, and collaborate on projects, enabling a deeper exchange of knowledge.
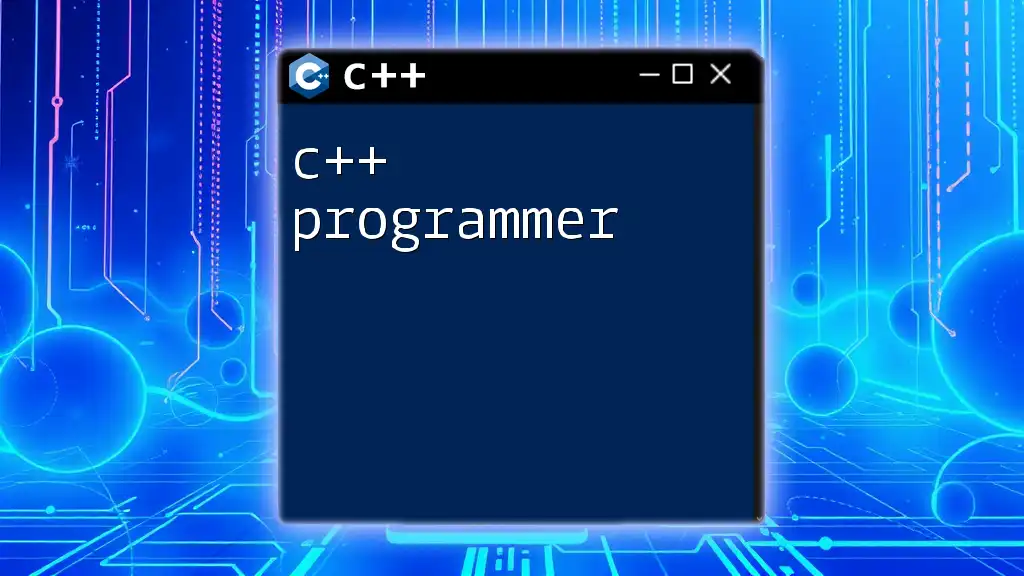
Common Mistakes to Avoid When Learning C++
Skipping the Basics
One of the most significant mistakes learners can make is skipping fundamental concepts. Mastering the basics, such as variable types, control flow, and functions, builds a strong foundation necessary for understanding advanced topics. Neglecting this groundwork often leads to difficulties in grasping more intricate concepts later on.
Not Practicing Enough
Failing to practice consistently can hinder your growth as a C++ programmer. The adage “learning by doing” is crucial in programming; without practical exercises and real projects, concepts may remain abstract and vague. Challenge yourself regularly with coding exercises and projects that can reinforce your learning.
Relying Solely on Books
While books are an excellent resource, relying exclusively on them is another common pitfall. Balance theoretical knowledge with practical implementation by engaging in online coding challenges, project work, or contributing to open-source projects. This hands-on experience is vital for applying what you've learned and improving your skills.
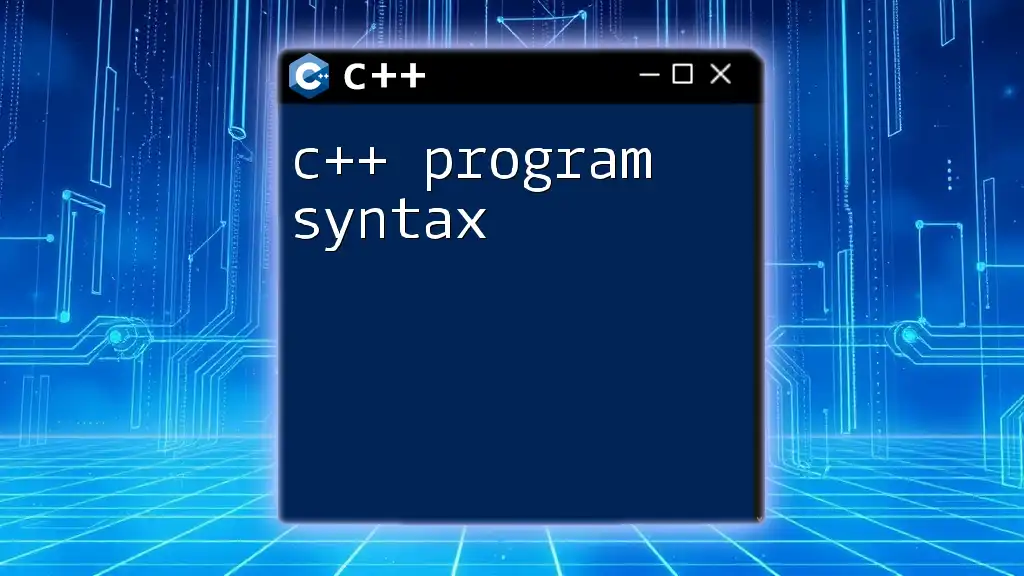
Conclusion
Choosing the right C++ programming book is an essential step in your journey to becoming a proficient programmer. Remember that learning to program is a process that requires patience and practice. Explore various resources, engage with the community, and continuously challenge yourself. With diligence and the right materials, you'll successfully build your skill set and confidently tackle complex programming tasks.
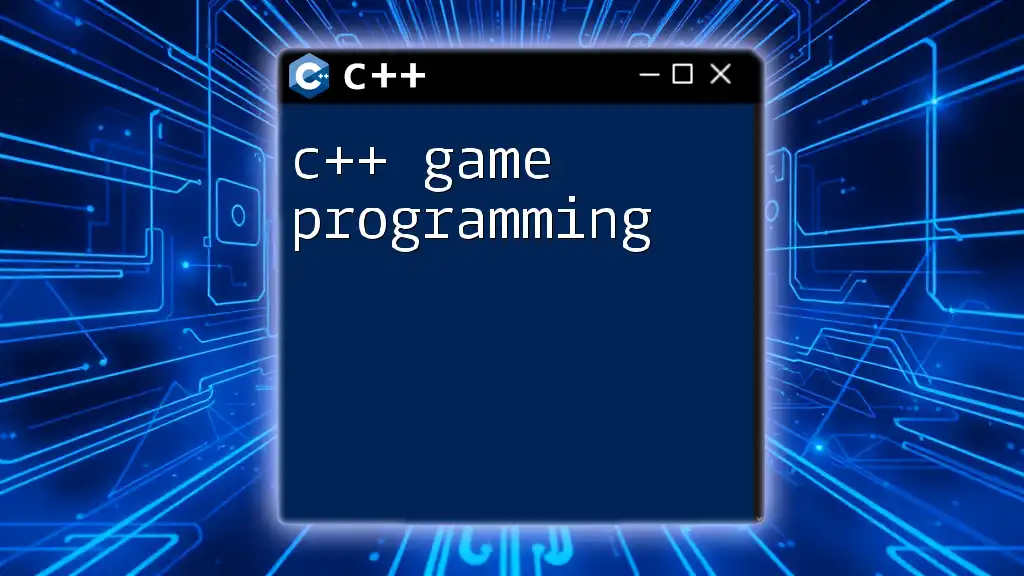
Additional Resources
To further enhance your learning experience, consider exploring free online C++ tutorials, instructional videos, and active coding forums. These resources can complement your book studies and provide ongoing support as you navigate your programming journey.