C++ builds on the foundations of C, adding object-oriented features and enhanced functionalities that enable programmers to write more structured and reusable code.
Here's a simple example illustrating the difference in handling a class in C++ compared to C:
#include <iostream>
class Rectangle {
public:
int width, height;
Rectangle(int w, int h) : width(w), height(h) {}
int area() {
return width * height;
}
};
int main() {
Rectangle rect(5, 10);
std::cout << "Area: " << rect.area() << std::endl;
return 0;
}
Fundamental Differences Between C and C++
Syntax and Structure
C and C++ share a similar syntax, but C++ introduces several enhancements that streamline coding practices. For instance, in C, a function is typically defined as follows:
int add(int a, int b) {
return a + b;
}
In contrast, C++ supports function overloading, allowing multiple functions with the same name but different parameters:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
Data Types and Variables
While both C and C++ share primitive types, C++ introduces several new data types. Among these are:
- std::string: A class for handling strings more effectively than character arrays in C.
- std::vector: A dynamic array that can resize automatically.
Understanding these extensions can drastically improve the efficiency of your code.
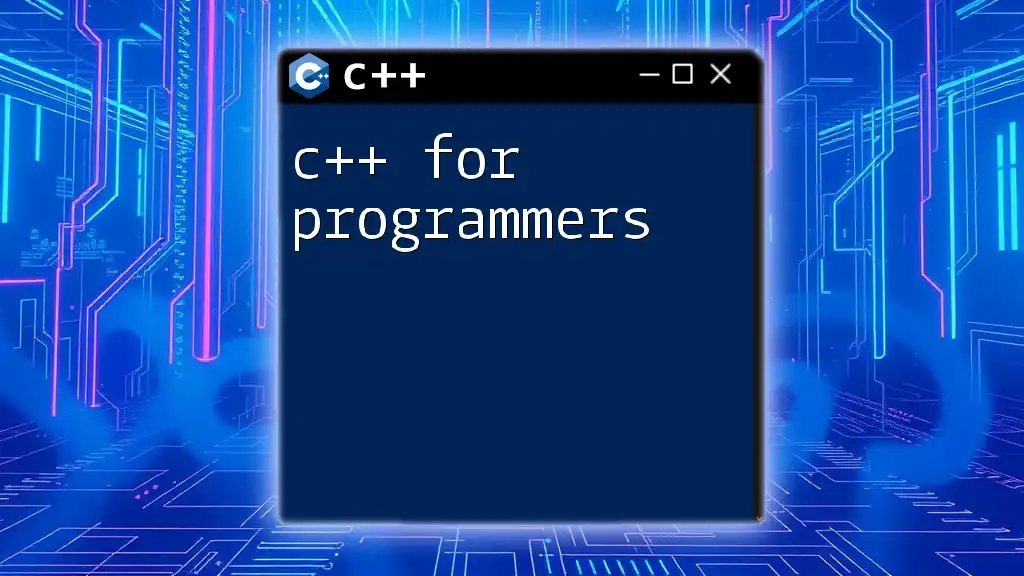
Object-Oriented Programming in C++
Introduction to Object-Oriented Concepts
Object-oriented programming (OOP) is a fundamental paradigm in C++. Whereas C is procedural, C++ allows developers to model real-world entities using classes and objects.
- Classes encapsulate data and behavior.
- Objects are instances of these classes.
Encapsulation, Inheritance, and Polymorphism
Three core concepts of OOP are encapsulation, inheritance, and polymorphism.
- Encapsulation involves bundling data and methods that operate on the data within one unit, e.g., a class.
class Car {
public:
void start() {
// Start the car
}
};
- Inheritance allows a new class to inherit properties of an existing class.
class Vehicle {
// Vehicle properties
};
class Car : public Vehicle {
// Additional Car properties
};
- Polymorphism enables objects to be treated as instances of their parent class, allowing for method overriding.
Creating Classes and Objects
Here’s how to define a simple class and instantiate an object in C++:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark(); // Outputs: Woof!
return 0;
}
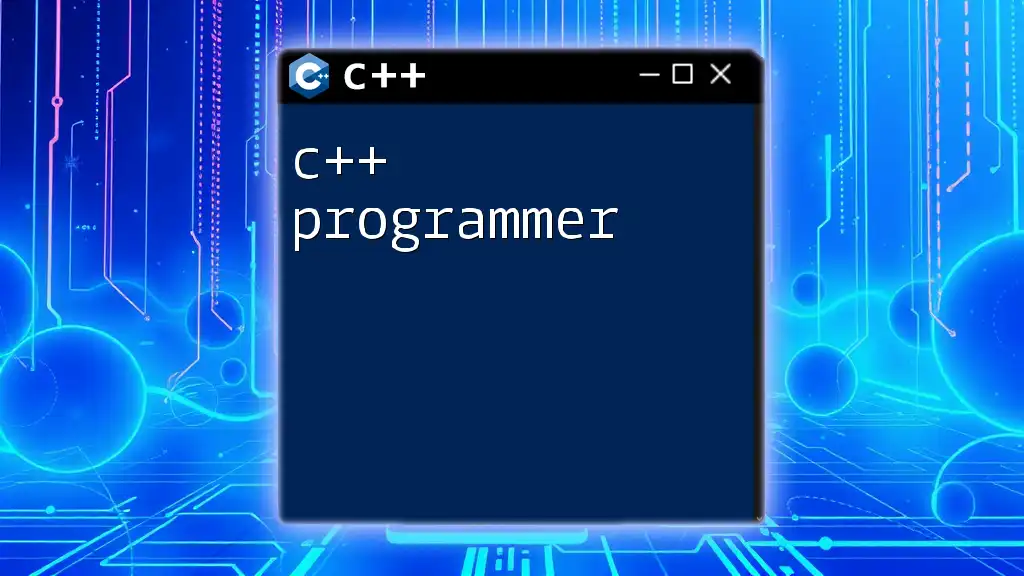
Memory Management in C++
Dynamic Memory
Memory management is one key area where C++ differs significantly from C. In C++, you can manage memory dynamically using new and delete:
int* p = new int; // Allocating memory
*p = 10;
// Use p
delete p; // Freeing memory
Constructors and Destructors
C++ employs constructors and destructors for resource management. Constructors automatically initialize an object, while destructors ensure resources are released:
class Example {
public:
Example() {
std::cout << "Constructor called!" << std::endl;
}
~Example() {
std::cout << "Destructor called!" << std::endl;
}
};
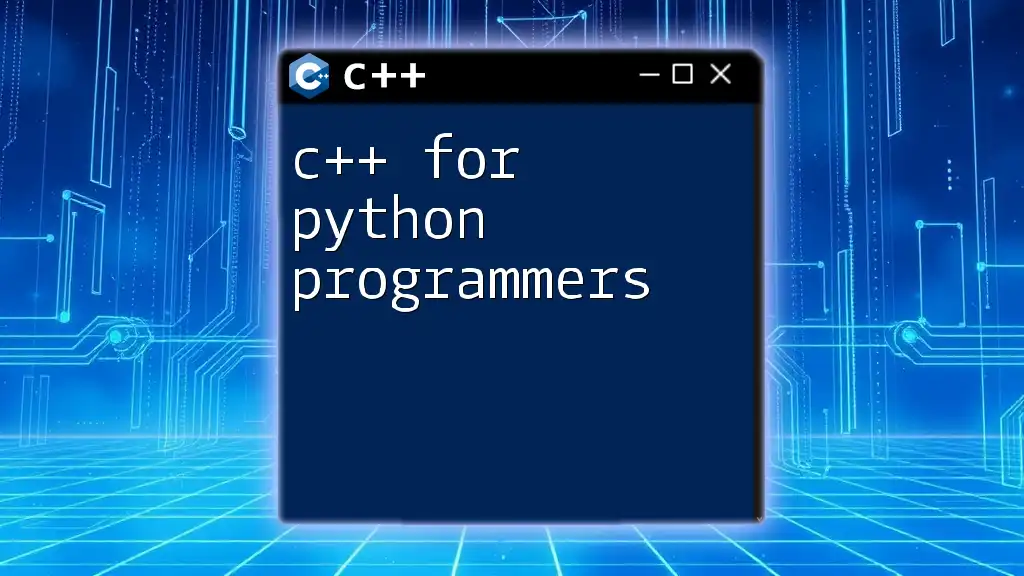
Advanced Features of C++
Function Overloading
Function overloading allows you to define multiple functions with the same name, differing in parameters. This can lead to more intuitive and cleaner code.
void display(int a) {
std::cout << "Integer: " << a << std::endl;
}
void display(double a) {
std::cout << "Double: " << a << std::endl;
}
Operator Overloading
Operator overloading allows you to redefine how operators work for user-defined types. For example, you can overload the `+` operator for a custom `Complex` class:
class Complex {
public:
float real, imag;
Complex operator+(const Complex& other) {
return Complex(real + other.real, imag + other.imag);
}
};
Standard Template Library (STL)
STL is an indispensable part of C++ that provides generic classes and functions. It includes data structures such as vectors, lists, and maps, which simplify coding processes.
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
for(int num : numbers) {
std::cout << num << " ";
}
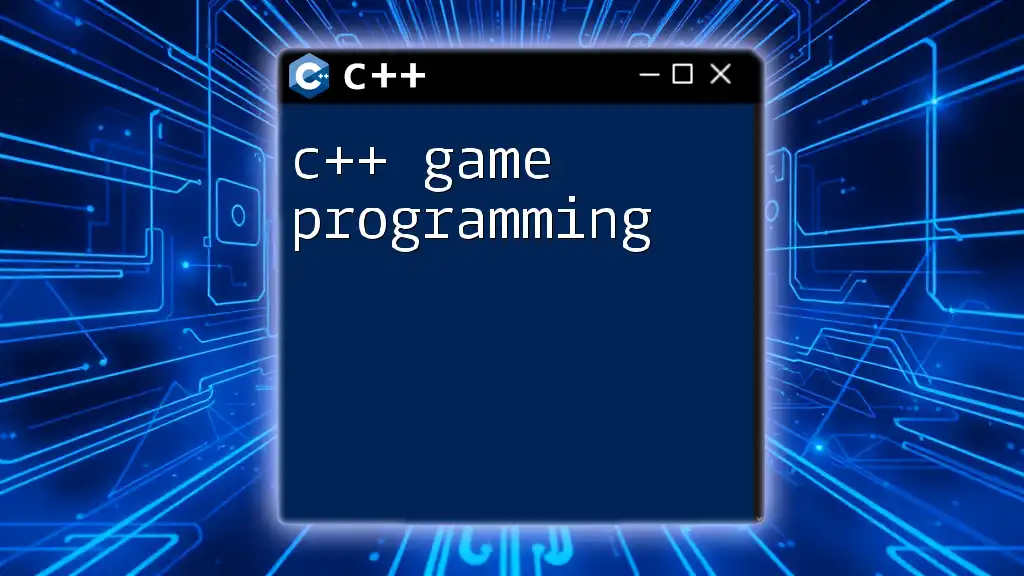
Error Handling in C++
Exceptions in C++
C++ introduces exception handling to manage errors gracefully. You can use `try`, `catch`, and `throw` to control error propagation.
try {
throw std::runtime_error("An error occurred");
} catch (std::runtime_error &e) {
std::cout << e.what() << std::endl; // Outputs the error message
}
Best Practices for Error Handling
- Always use exceptions for serious errors that are not expected.
- Avoid using exceptions for common control flow.
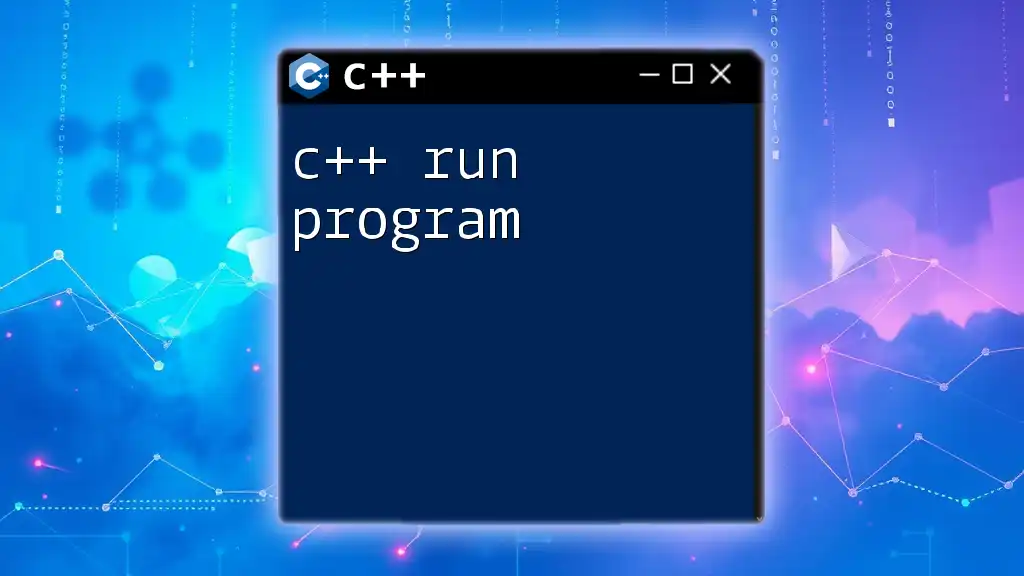
C++ and C Interoperability
Calling C Code from C++
C++ allows the inclusion of C libraries by using `extern "C"` to prevent name mangling:
extern "C" {
#include "cmylib.h"
}
Writing C++ Code that Works with C Compilers
To ensure your C++ code can be compiled by a C compiler:
- Use a subset of C++ that doesn't rely on C++-only features.
- Follow conventions that ensure compatibility.
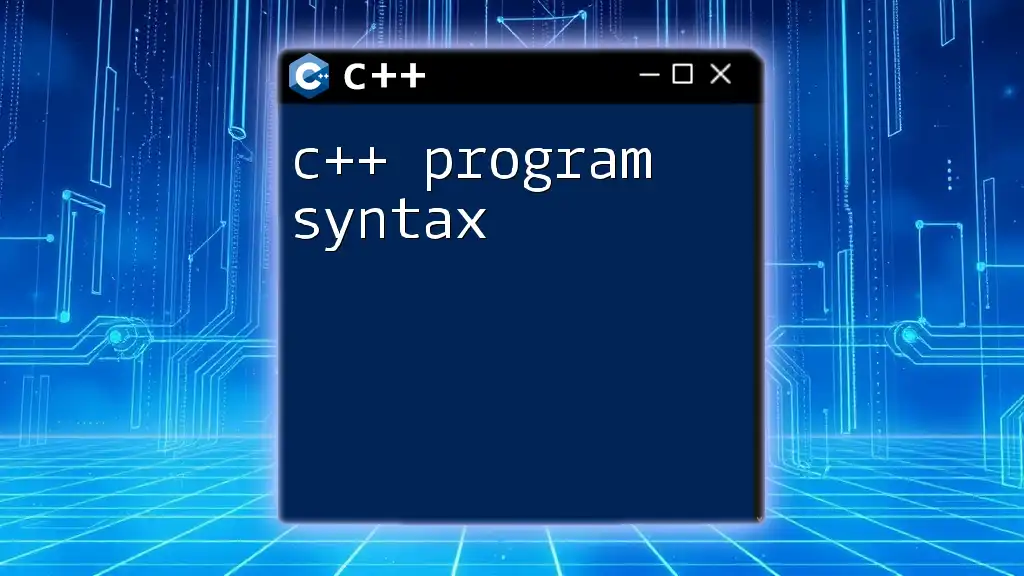
Best Practices for Writing C++ Code
Code Readability and Maintenance
Clean, readable code is crucial for maintaining software:
- Use meaningful variable names.
- Keep formatting consistent and add comments when necessary.
Performance Considerations
When coding in C++, consider the following:
- Avoid unnecessary copies of objects by using references.
- Utilize move semantics when appropriate to enhance performance.
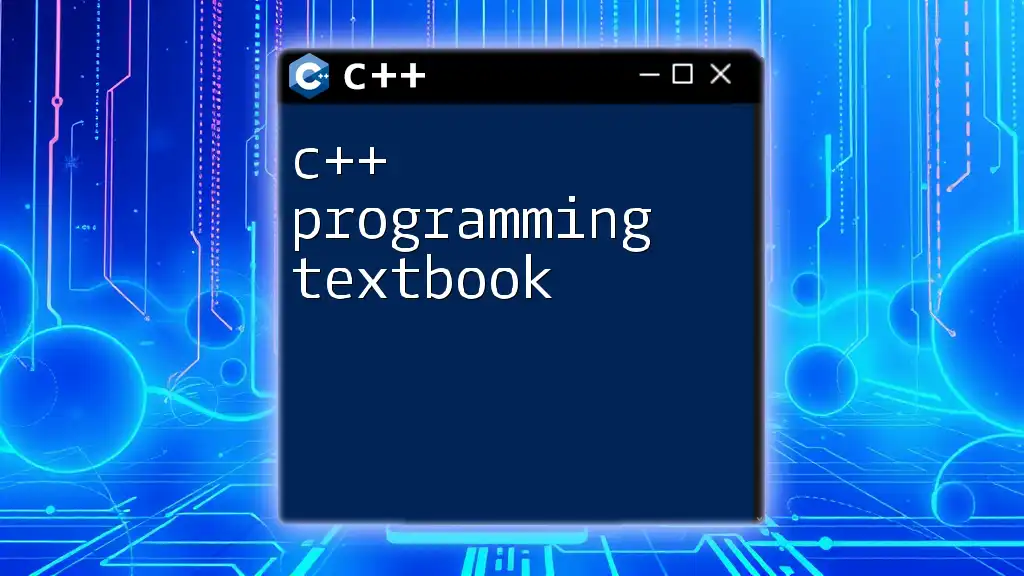
Conclusion
Transitioning from C to C++ can significantly enhance your programming skill set. C++ for C programmers opens up a wealth of features and capabilities that can lead to improved code organization, better resource management, and a more intuitive way to model real-world entities. Embrace the power of OOP, take advantage of STL, and employ good coding practices to become a proficient C++ developer.