Running a C++ program typically involves compiling the source code into an executable file and then executing that file from the command line or terminal.
Here's a code snippet demonstrating the basic compilation and execution process:
g++ -o my_program my_program.cpp # Compile the C++ program
./my_program # Run the compiled executable
Understanding C++ Code
What is C++?
C++ is a general-purpose programming language created by Bjarne Stroustrup beginning in 1979 at Bell Labs. It is an extension of the C programming language and includes features that support object-oriented programming (OOP), such as classes and objects, which allow developers to create structured and reusable code. C++ stands out for its performance and efficiency, making it a popular choice for systems software, game development, and high-performance applications.
Structure of a C++ Program
Every C++ program is organized around a set of rules and components. Understanding these elements is essential for successfully running a C++ program.
A standard C++ program typically includes:
- Preprocessor Directives: Lines that begin with `#`, telling the compiler to include libraries or perform specific tasks before compilation.
- Main Function: The entry point of any C++ program is the `main()` function. This is where execution begins.
Here’s a simple C++ program that showcases these components:
#include <iostream>
int main() {
std::cout << "Hello, World!";
return 0;
}
In this example:
- `#include <iostream>` includes the Input-Output Stream library, which allows the program to handle input and output.
- `int main()` is the function signature indicating that the program is starting here.
- Inside the braces `{}`, `std::cout << "Hello, World!";` prints the text to the console. The `return 0;` indicates the program completed successfully.
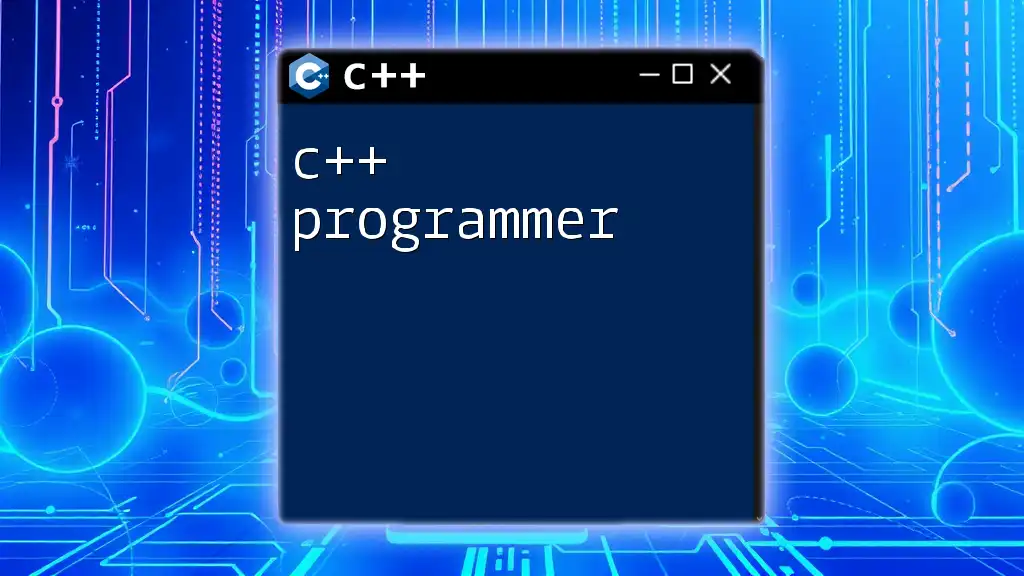
Setting Up Your Environment
Choosing a C++ Compiler
To successfully run C++ code, you need a compiler that interprets and translates the code into machine-readable format. There are several popular compilers:
- GCC (GNU Compiler Collection): Widely used and supports various operating systems. It's open-source and has robust support for C++ standards.
- Clang: Known for fast compilation and excellent error messages; favored within certain communities for its real-time code analysis.
- MSVC (Microsoft Visual C++): Integrated into the Visual Studio IDE, perfect for Windows-centric development.
When choosing a compiler, consider factors like your operating system, programming paradigm, and community support.
Installing a C++ Compiler
Installation can vary depending on the operating system:
-
For Windows: The simplest way to install GCC is through MinGW. Download and install it, making sure to add it to the system path for easy access from the command line.
-
For macOS: You can install LLVM via Homebrew. Run this command:
brew install llvm
Ensure that the installation path is added to your environment variables.
-
For Linux: Most distributions have GCC pre-installed. If not, use:
sudo apt install g++
Integrated Development Environments (IDEs)
Using an IDE can streamline the process of writing and running C++ programs. Popular options include:
- Code::Blocks: A free, open-source IDE with a user-friendly interface that supports multiple compilers.
- Visual Studio: A powerful environment for Windows development, featuring tools for debugging and performance analysis.
- CLion: A cross-platform IDE, preferred by many for its advanced features like refactoring and debugging tools.
Each IDE comes with built-in capabilities for running C++ code, which reduces the complexity typically associated with command-line operations.
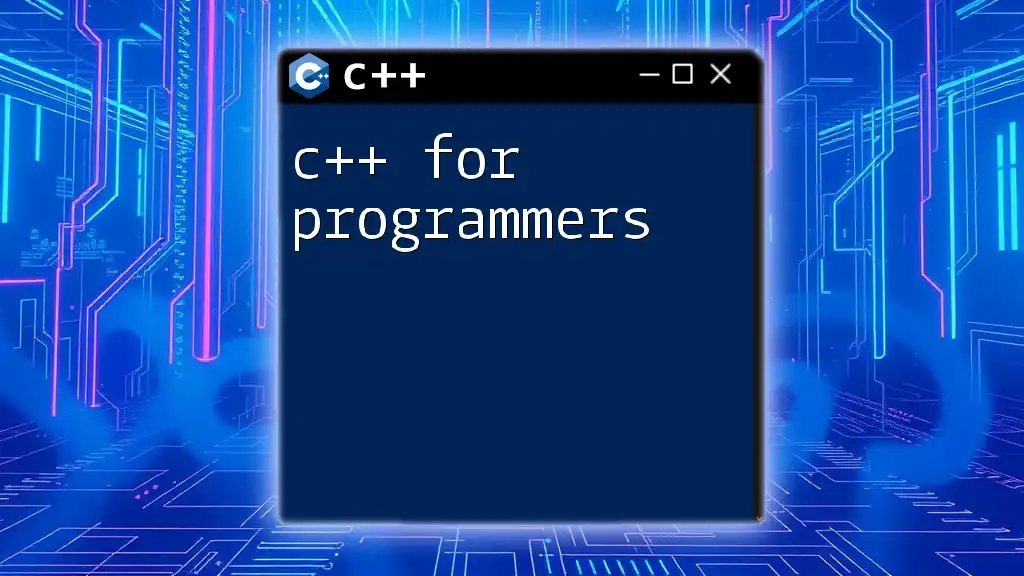
Running C++ Code
Running C++ Code Using Command Line
To run a C++ program via the command line, it's essential to understand a few key commands. These commands compile and execute your code. Here’s a straightforward workflow:
- Write your code in a file named `hello.cpp` (for instance).
- Open your terminal or command prompt.
- Navigate to the directory where your code is saved.
Run the following commands to compile and execute your program:
g++ hello.cpp -o hello
./hello
Here’s what these commands do:
- `g++ hello.cpp -o hello`: Compiles `hello.cpp` and creates an executable named `hello`.
- `./hello`: Executes the compiled program.
Running C++ Code in an IDE
When using an IDE, the process becomes simpler as most of the underlying commands are handled by the software itself. Here’s a quick overview of running a C++ program in popular IDEs:
-
Code::Blocks: After creating a new project and writing your code, click on the "Build" button to compile and then "Run" to execute.
-
Visual Studio: Set up a solution and add a C++ file. To run the program, simply click the green play button, which compiles and runs the code in a single step.
Using an IDE offers advantages like integrated debugging and real-time code errors, making it easier to develop and run C++ applications.
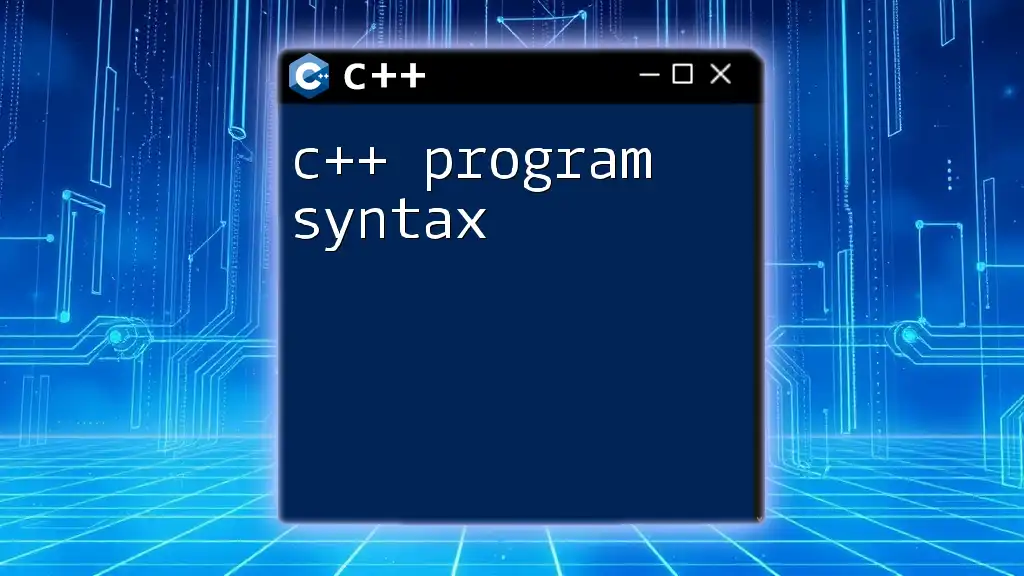
Troubleshooting Common Issues
Compilation Errors
Occasionally, your code may fail to compile due to syntax issues. These are known as compilation errors. Here are steps to identify and fix them:
- Syntax Errors: Commonly occur when a semicolon or bracket is missing.
Example of a syntax error:
int main() {
std::cout << "Missing Semicolon" // This will cause an error
return 0;
}
To fix it, simply add a semicolon:
std::cout << "Missing Semicolon"; // Corrected version
Runtime Errors
Runtime errors arise while the program is executing, often due to logical errors or unexpected inputs. To effectively troubleshoot runtime issues:
- Use debugging tools integrated into your IDE or compile with debugging options enabled (for example, using `-g` with `g++`).
- Carefully read the error messages; they often indicate where the problem lies.
- Test edge cases to ensure your program handles unexpected or extreme input.
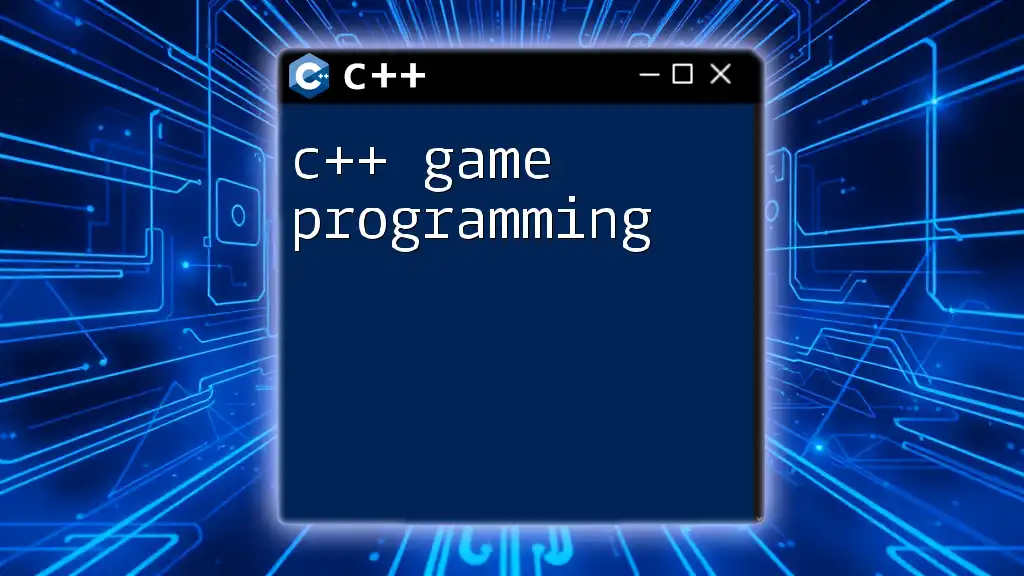
Best Practices for Running C++ Code
Writing Clean Code
Creating clean, readable code is crucial, especially in collaborative environments. This includes:
- Using meaningful variable names that describe their purpose.
- Structuring your code with consistent indentation and spacing.
- Implementing logical spacing between functional blocks for better readability.
Using Comments and Documentation
Comments in your code explain the logic and purpose behind specific code blocks. They enhance collaboration and make the codebase maintainable. Use them effectively:
// This function adds two integers and returns the result
int add(int a, int b) {
return a + b;
}
Regular Testing
Testing your code as you write enhances quality and reduces bugs. Employ testing frameworks like Google Test for more robust testing strategies, ensuring both individual components and overall functionality perform as expected.
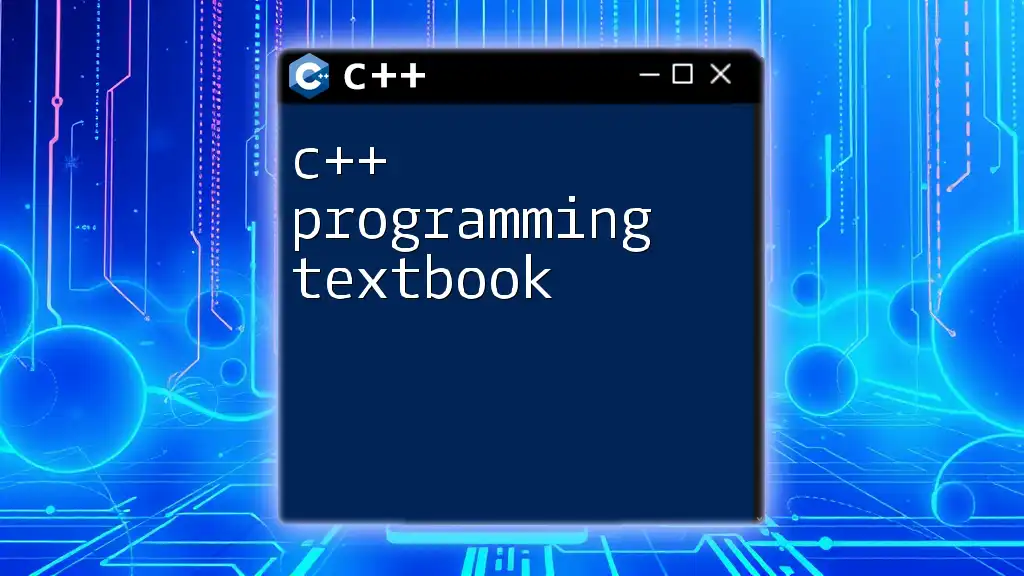
Conclusion
In this guide, we explored how to effectively run a C++ program, including compiler selection, environment setup, and best practices for debugging. Running C++ code can seem intimidating at first, but gaining experience and practicing consistently will improve your skills. We're excited to share more insights and strategies through our courses and community, encouraging you to further hone your programming abilities!
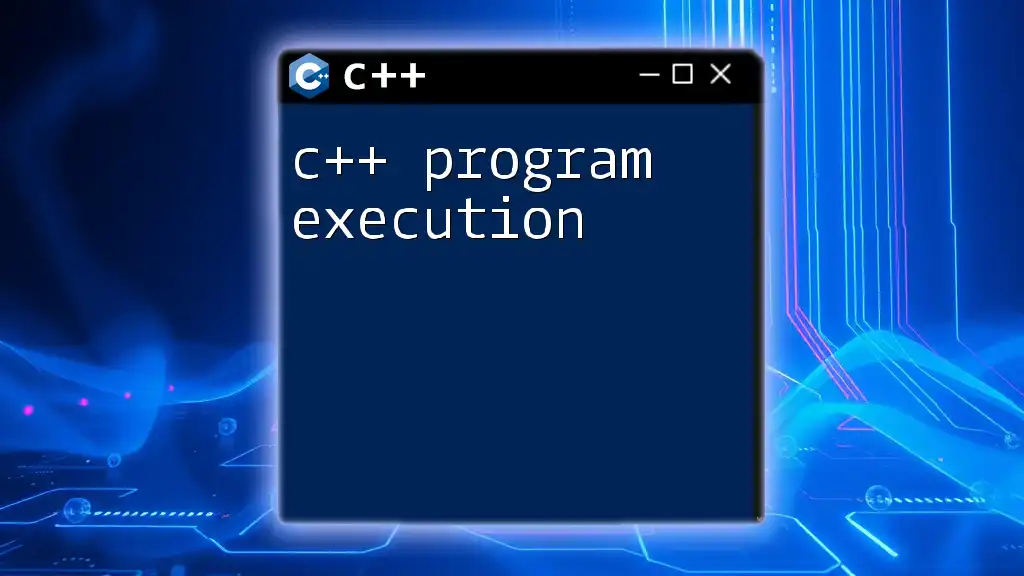
Additional Resources
For deeper knowledge and tools, seek out C++ documentation, recommended reading materials, and online video tutorials to augment your learning experience. These resources can provide valuable insights and help you deepen your understanding of the C++ programming language.