A C++ GUI framework provides a set of libraries and tools to simplify the creation of graphical user interfaces, allowing developers to build interactive applications efficiently.
Here's a simple example using the Qt framework to create a basic window:
#include <QApplication>
#include <QWidget>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWidget window;
window.resize(320, 240);
window.setWindowTitle("Basic C++ GUI");
window.show();
return app.exec();
}
Understanding GUI Frameworks
What is a GUI Framework?
A GUI Framework is a collection of libraries and tools that facilitate the creation of graphical user interfaces for applications. Unlike general libraries, which provide specific functionalities (like mathematical operations or data manipulation), a GUI framework offers a comprehensive environment complete with structure, components, and event handling necessary to develop interactive applications.
The primary role of GUI frameworks is to abstract the complexities of directly interfacing with the underlying operating system's native GUI subsystem, enabling developers to create intuitive interfaces with reduced effort. They provide a set of components such as buttons, windows, text boxes, and more, allowing programmers to focus on functionality rather than the intricacies of GUI handling.
Benefits of Using C++ for GUI Development
C++ is renowned for its performance, making it an excellent choice for developing resource-intensive applications. When utilizing a C++ GUI framework, developers can create applications that execute quickly and efficiently. Another significant advantage of using C++ is its cross-platform capabilities; many C++ GUI frameworks allow for the development of applications that can run seamlessly on various operating systems with minimal changes to the codebase.
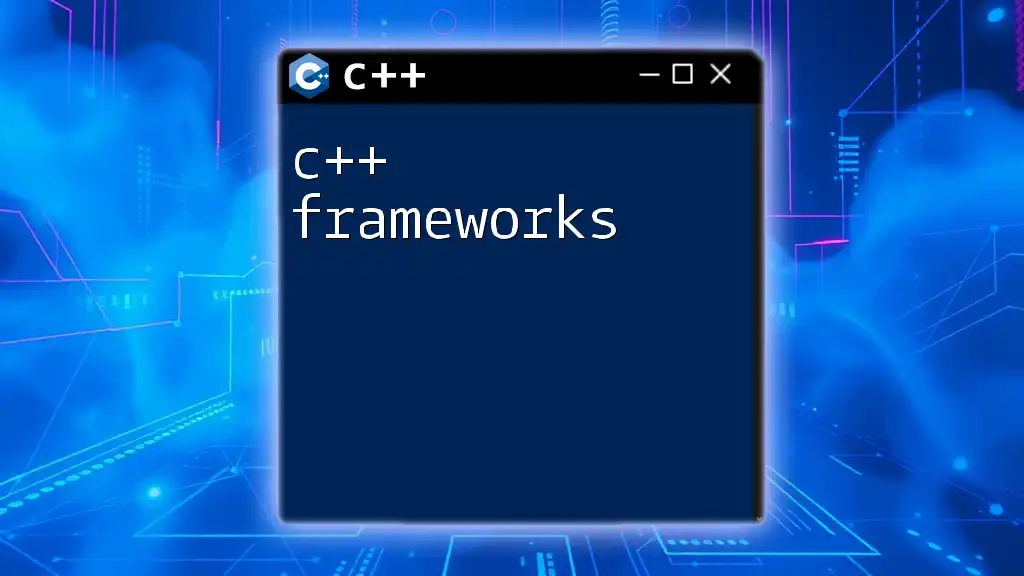
Popular C++ GUI Frameworks
Qt
Overview of Qt
Qt stands as one of the most widely used C++ GUI frameworks. Developed by the Qt Company, it provides a wide array of tools and components designed to simplify GUI development. Its key features include support for 2D and 3D graphics, integration with databases, and network handling.
Setting Up Qt Development Environment
To start developing with Qt, you must first install the Qt framework. This process typically involves:
- Downloading the Qt Installer from the official Qt website.
- Running the Installer and selecting the components you wish to include, such as `Qt Creator`, which is the development environment.
- Configuring your IDE, ensuring it recognizes the Qt installation.
Basic Qt Application Structure
Here’s a simple example of a Qt application that displays a button with the label "Hello, World!":
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, World!");
button.show();
return app.exec();
}
In this code snippet:
- `QApplication` manages the application’s control flow and main settings.
- `QPushButton` is a GUI component representing a clickable button. The `show()` function makes it visible to the user.
wxWidgets
Overview of wxWidgets
wxWidgets is another powerful GUI framework that enables developers to create native-looking applications for various platforms. Its flexibility and ease of use make it a popular choice among C++ developers. wxWidgets offers a comprehensive set of widgets and tools for creating sophisticated user interfaces.
Setting Up wxWidgets Development Environment
To begin utilizing wxWidgets, follow these steps:
- Download wxWidgets from the official wxWidgets website.
- Compile the library according to the platform's requirements.
- Integrate wxWidgets with your chosen IDE, such as Code::Blocks, by linking the necessary library files.
Basic wxWidgets Application Structure
Here’s an example showing how to create a simple wxWidgets application:
#include <wx/wx.h>
class MyApp : public wxApp {
public:
virtual bool OnInit();
};
class MyFrame : public wxFrame {
public:
MyFrame() : wxFrame(NULL, wxID_ANY, "Hello, World!") {}
};
wxIMPLEMENT_APP(MyApp);
bool MyApp::OnInit() {
MyFrame *frame = new MyFrame();
frame->Show(true);
return true;
}
In this example:
- The `MyApp` class inherits from `wxApp`, which serves as the application’s entry point.
- `MyFrame` is a frame window that will be displayed. The constructor sets its title to "Hello, World!".
GTKmm
Overview of GTKmm
GTKmm is the official C++ interface for the popular GTK graphical user interface library used mainly on Linux. It provides a rich set of features, including support for various UI components and advanced drawing capabilities.
Setting Up GTKmm Development Environment
To set up GTKmm, you should:
- Install GTK+ development package for your operating system (on Linux, this can usually be done via package managers).
- Install GTKmm package that corresponds with the installed GTK+.
- Configure your IDE to recognize GTKmm libraries.
Basic GTKmm Application Structure
Here is an elementary example of a GTKmm application:
#include <gtkmm.h>
class MyWindow : public Gtk::Window {
public:
MyWindow();
};
MyWindow::MyWindow() {
set_title("Hello, World!");
set_default_size(200, 200);
}
int main(int argc, char *argv[]) {
auto app = Gtk::Application::create(argc, argv, "org.gtkmm.example");
MyWindow window;
return app->run(window);
}
In this structure:
- `MyWindow` inherits from `Gtk::Window` which serves as the main window of the application.
- The `set_title()` function defines the window title, while `set_default_size()` specifies its dimensions.
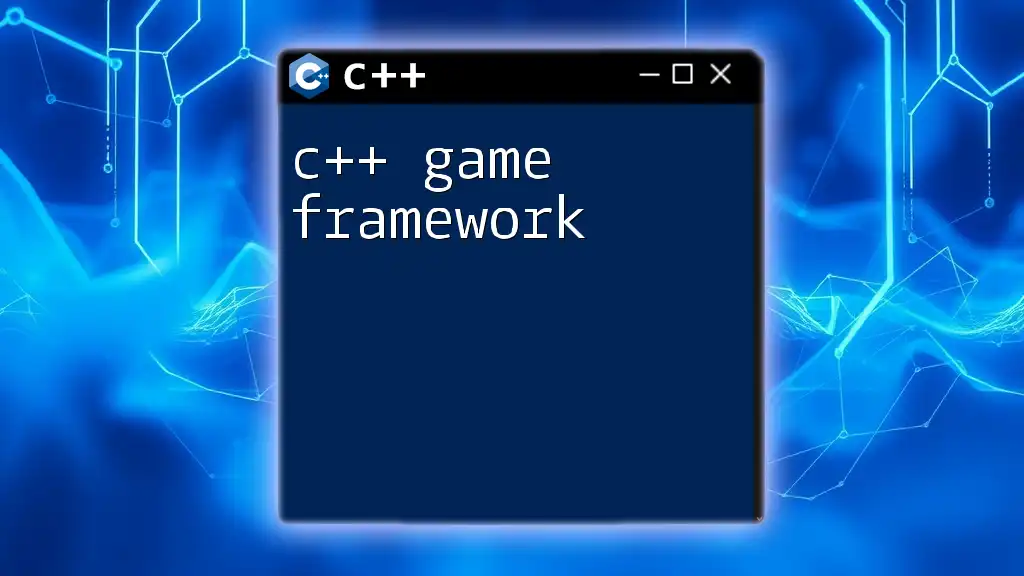
Choosing the Right C++ GUI Framework for Your Needs
Factors to Consider
When selecting a C++ GUI framework, it's crucial to consider several factors:
- Complexity of the Project: Evaluate the complexity of the application to determine if a lightweight library or a comprehensive framework is needed.
- Cross-Platform Requirements: If you plan to deploy on multiple operating systems, choose a framework that supports cross-platform development.
- Community Support and Documentation: Good documentation and community support can significantly enhance the development experience and help solve issues quickly.
Comparison of Popular Frameworks
Each framework has its unique strengths and weaknesses. A brief side-by-side comparison could look like this:
- Qt: Excellent documentation and wide community support, suitable for complex applications.
- wxWidgets: Provides a native look and feel with sufficient documentation; consider it for cross-platform needs.
- GTKmm: Best for applications primarily targeting Linux, but also works on Windows and macOS.
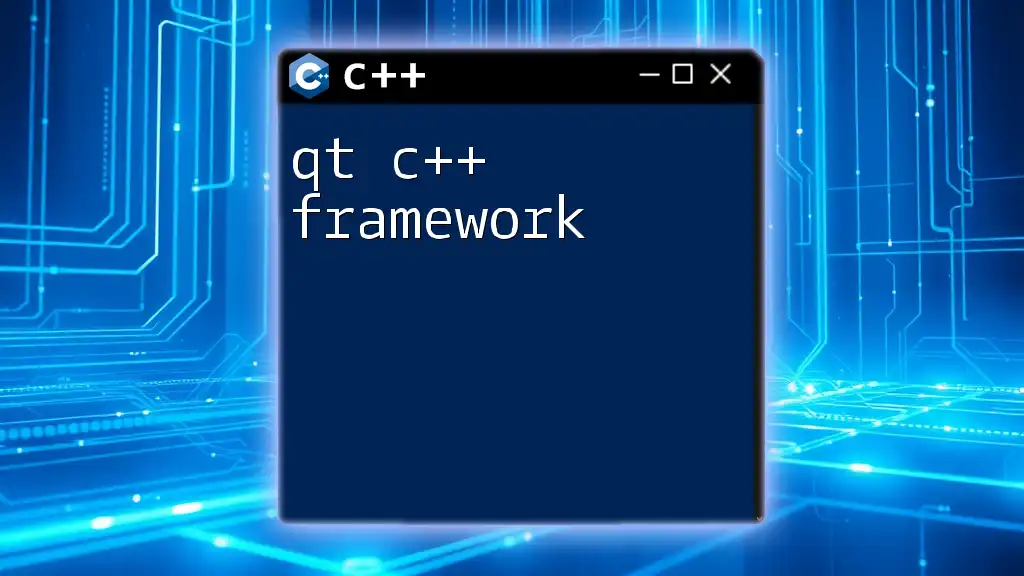
Advanced Topics
Integrating C++ GUIs with Databases
Integrating databases into your C++ GUI can vastly enhance application functionality. A common choice is SQLite due to its lightweight nature and ease of use. Below is a brief outline of integrating SQLite with a Qt application:
- Include the SQLite header in your Qt project.
- Open a connection to the database using `QSqlDatabase`.
- Query data using `QSqlQuery` and display results in GUI components.
Custom Widgets and Designs
Creating custom widgets can elevate the user experience. In Qt, custom widgets are created by subclassing existing components and overriding key functions such as `paintEvent()` for custom drawing. This allows fine-tuning of appearance and behavior tailored to specific needs.
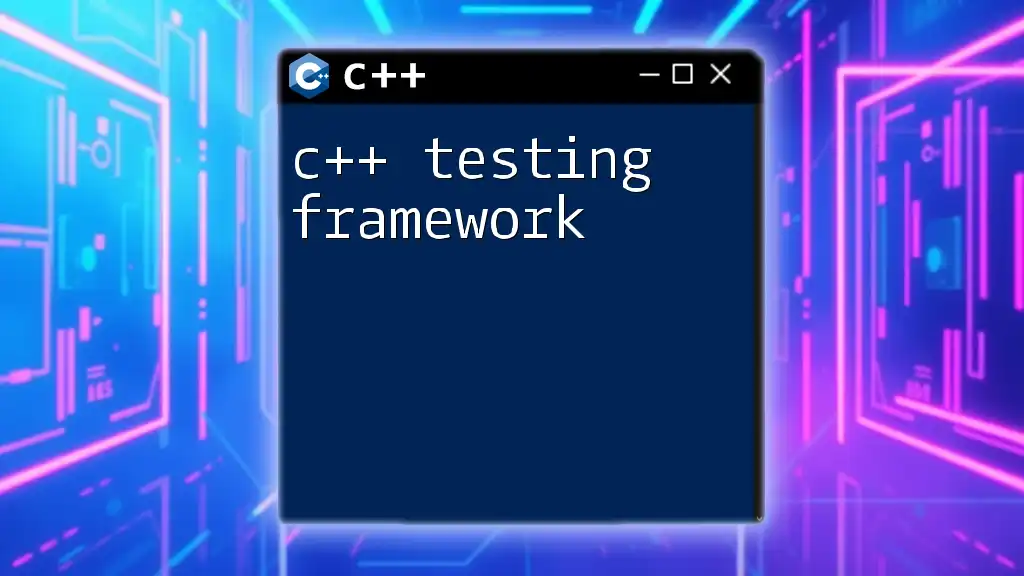
Conclusion
In summary, selecting the right C++ GUI framework is a critical step that can dictate your project’s success. Whether you opt for Qt, wxWidgets, or GTKmm, understanding the tools, their structures, and capabilities will pave the way for creating robust applications.
Continually experimenting with different frameworks will allow you to discover their strengths, weaknesses, and how they best suit your development objectives. With the right framework in hand, you can produce a seamless user experience paired with the robust performance expected from C++ applications.
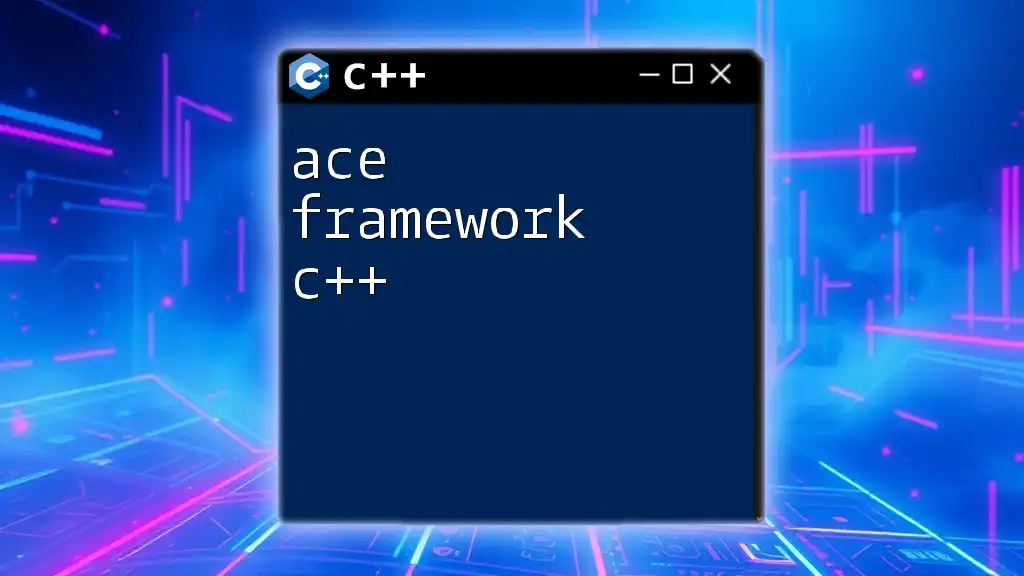
Call to Action
Join our community for more tutorials, insights, and tips on C++ GUI frameworks. Share your experiences and inquiries in the comments section—let's learn together!