A C++ game framework provides a structured foundation for developing games by offering essential tools, libraries, and functionalities to streamline the game development process.
Here's a simple example showcasing the initiation of a game loop using a C++ game framework:
#include <GameFramework.h>
int main() {
GameFramework game;
game.init("My Game", 800, 600); // Initialize the game with title and dimensions
while (game.isRunning()) {
game.handleEvents(); // Manage player input
game.update(); // Update game logic
game.render(); // Render the game scene
}
game.cleanup(); // Clean up resources
return 0;
}
Benefits of Using a C++ Game Framework
Performance and Efficiency
C++ is renowned for its ability to execute resource-intensive computations and manage hardware resources tightly. This is particularly advantageous in game development, where performance can be the difference between an immersive experience and a stuttered, frustrating one. A C++ game framework leverages the language’s speed, allowing developers to create games that can render high-quality graphics and support complex computations without lag. Real-world examples demonstrate that frameworks employing C++ can handle extensive game worlds and intricate physics simulations more efficiently than those built with higher-level languages.
Rapid Prototyping
One of the most compelling reasons to use a C++ game framework is the speed at which developers can prototype ideas. Frameworks provide pre-built functions and structures, which dramatically reduces the time required to move from concept to playable game. This is particularly valuable during iterative development when testing new features or gameplay mechanics. Instead of starting from scratch, developers can focus on refining ideas, knowing that the foundational elements of their game are pre-established.
Code Reusability
In game development, the ability to reuse code greatly enhances productivity. A well-structured C++ game framework facilitates modularity, allowing developers to build reusable components. This means that common functions—like rendering graphics or handling input—can be reused across multiple projects. As developers become familiar with the framework, they can quickly adapt existing code to new projects, streamlining the development process and minimizing errors.
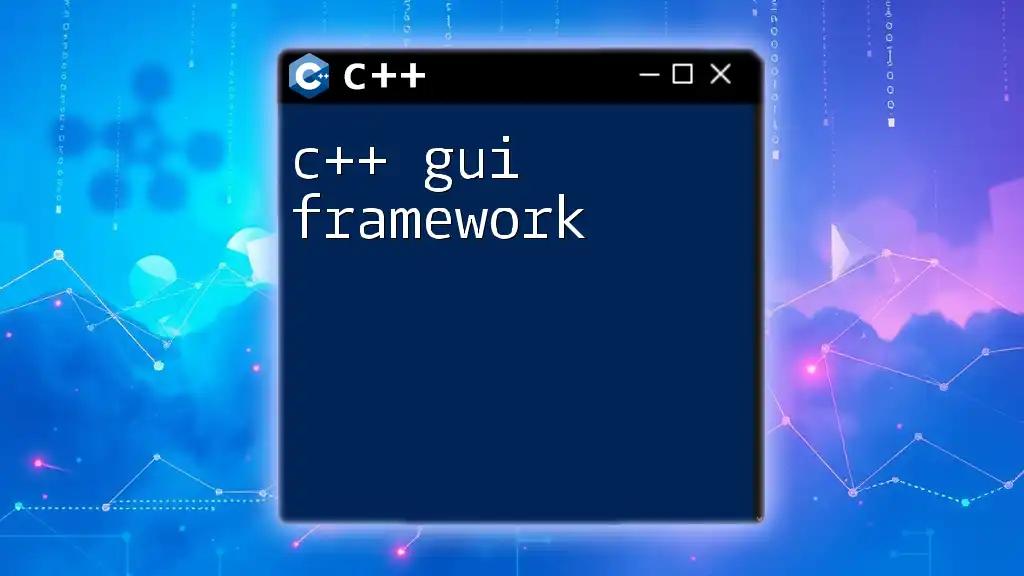
Key Features of C++ Game Frameworks
Graphics Rendering
Graphics rendering is at the heart of game development, and C++ frameworks excel in integrating with powerful rendering APIs like OpenGL and DirectX. Utilizing these APIs allows developers to produce stunning visuals and complex animations with relative ease.
For example, here’s a minimal OpenGL setup to create a simple window:
// Minimal OpenGL setup to create a window
#include <GL/glut.h>
void display() {
glClear(GL_COLOR_BUFFER_BIT);
// Drawing code here
glFlush();
}
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutCreateWindow("Simple Game");
glutDisplayFunc(display);
glutMainLoop();
return 0;
}
This code outlines the fundamental steps to initialize OpenGL and display a window.
Input Management
Handling user inputs efficiently is critical for engaging gameplay. C++ game frameworks offer built-in support for capturing input from various sources, such as keyboards and mice. Here’s a simple example of how to capture keyboard input using GLUT:
#include <iostream>
#include <GL/glut.h>
void keyboard(unsigned char key, int x, int y) {
std::cout << "Key pressed: " << key << std::endl;
}
int main(int argc, char** argv) {
// Setup code
glutKeyboardFunc(keyboard);
glutMainLoop();
return 0;
}
In this snippet, whenever a key is pressed, the program will output the corresponding character to the console.
Collision Detection
Collision detection is vital for any interactive game mechanics. Many popular algorithms such as Axis-Aligned Bounding Box (AABB) and Circle Collision can be implemented using C++. A simple AABB collision detection function might look like this:
bool AABB_Collision(Box a, Box b) {
return (a.x < b.x + b.width &&
a.x + a.width > b.x &&
a.y < b.y + b.height &&
a.y + a.height > b.y);
}
This function checks for overlap between two rectangular boxes, which is essential for determining whether two game objects are interacting.
Audio Management
A comprehensive gaming experience isn’t complete without sound effects and music. C++ game frameworks often integrate seamlessly with audio libraries like FMOD and OpenAL, enabling developers to add sound capabilities easily. This involves setup for mixing sounds, adjusting volumes, and playing audio files in response to game events.
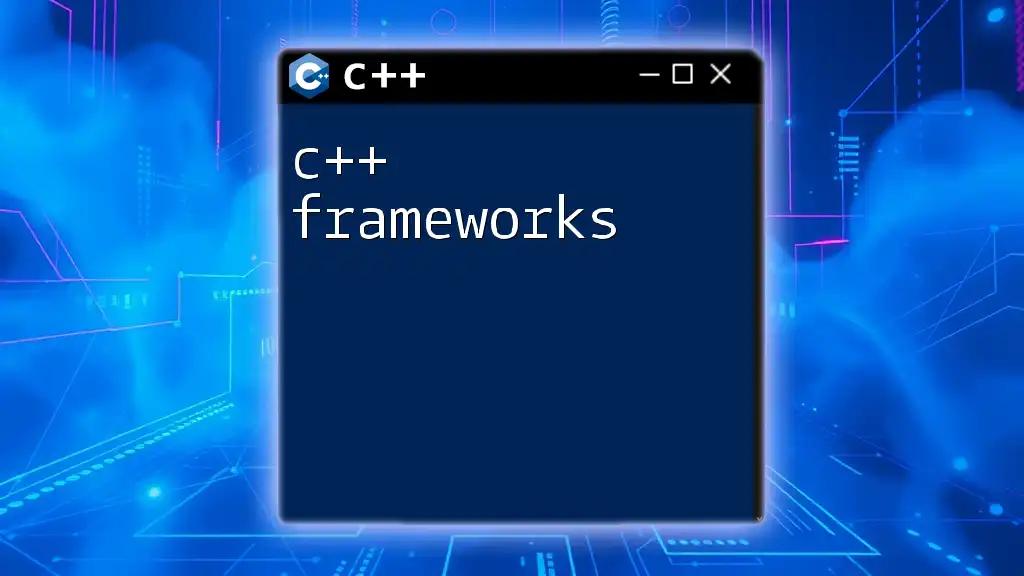
Popular C++ Game Frameworks
Unreal Engine
Unreal Engine is one of the most powerful game frameworks available today. Its robust capabilities, high-quality graphics rendering, and extensive support for 3D environments make it ideal for AAA titles. It offers blueprints for visual scripting, making the learning curve less steep for beginners while still giving advanced users access to C++ coding.
SFML (Simple and Fast Multimedia Library)
SFML is a popular framework known for its straightforward API, making it accessible for beginners. It provides tools for graphics, audio, and networking, allowing developers to create multimedia applications efficiently. Here's a basic example of setting up a simple window with SFML:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "SFML Works!");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
This code creates an SFML window that handles events until it is closed.
SDL (Simple DirectMedia Layer)
SDL is another robust framework that provides low-level access to audio, keyboard, mouse, and graphics. It’s widely used for multimedia applications and games. Below is a simple SDL application that opens a window:
#include <SDL2/SDL.h>
int main(int argc, char* argv[]) {
SDL_Init(SDL_INIT_VIDEO);
SDL_Window* window = SDL_CreateWindow("SDL Window", SDL_WINDOWPOS_CENTERED, SDL_WINDOWPOS_CENTERED, 800, 600, 0);
SDL_Delay(3000);
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
This piece of code initializes SDL, creates a window, and then waits for three seconds before closing it.

How to Choose the Right C++ Game Framework
Assessing Project Requirements
When selecting a C++ game framework, it is vital to consider the characteristics of your project. Is it a 2D platformer, a 3D shooter, or an open-world adventure? Understanding your game’s genre will help you choose a framework that best suits your needs.
Evaluating Community and Support
A strong community and well-maintained documentation can be invaluable. Look for frameworks with active forums and comprehensive guides, as this can significantly ease the learning curve and provide solutions to common issues.
Performance Considerations
Benchmarking is essential when deciding on a framework, especially for performance-oriented projects. Assess how well the framework handles complex calculations or large game worlds, as this will directly affect the player experience.

Getting Started with a C++ Game Framework
Setting Up Your Development Environment
An essential first step is to configure your development environment. Popular IDEs like Visual Studio, Code::Blocks, or CLion offer integrated support for C++ development. After installing your chosen framework, follow the provided installation guides to get started seamlessly.
Creating Your First Game
Building your first game using a C++ game framework should be a structured process. Start with a simple game concept, such as a basic Pong or platformer. Use the framework’s built-in features to manage graphics, inputs, and sound to create a prototype and refine your gameplay mechanics.
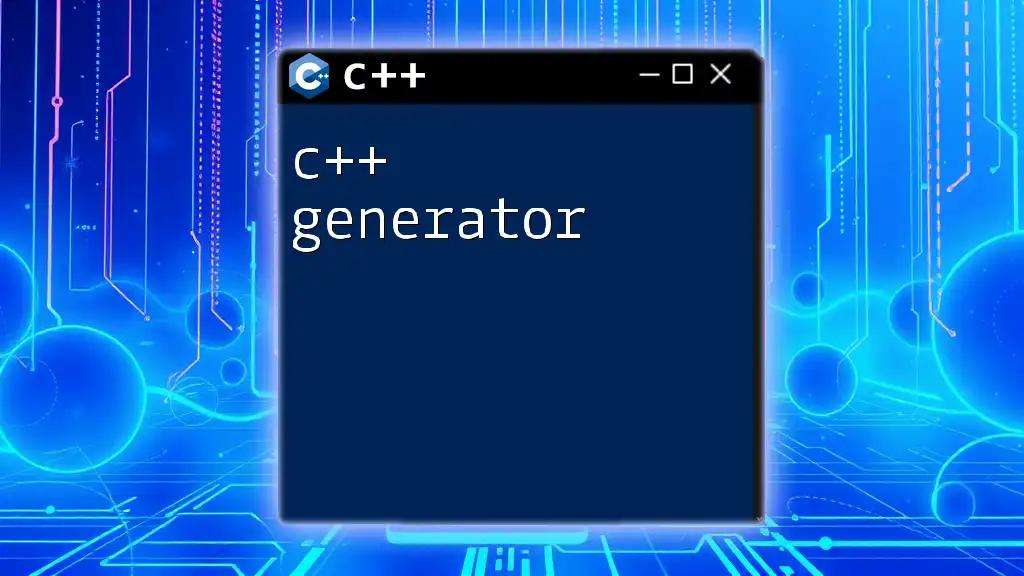
Common Challenges and Solutions
Debugging and Optimization
Debugging is an unavoidable part of game development. Familiarize yourself with debugging tools available in your IDE and frameworks. C++ also allows for performance profiling using dedicated tools to identify and address any bottlenecks.
Integration Issues
Integration of various libraries for graphics, sound, and input management can sometimes pose challenges. Read the documentation thoroughly, and don't hesitate to reach out to community forums for assistance with any integration problems you encounter.

Conclusion
A C++ game framework is a potent tool for developers looking to create engaging and efficient games. By utilizing frameworks like Unreal Engine, SFML, and SDL, you can benefit from rapid prototyping, performance optimization, and reusable code components. As you embark on your game development journey, remember to leverage the frameworks’ capabilities to streamline your workflow, overcome common challenges, and finally bring your game ideas to life.
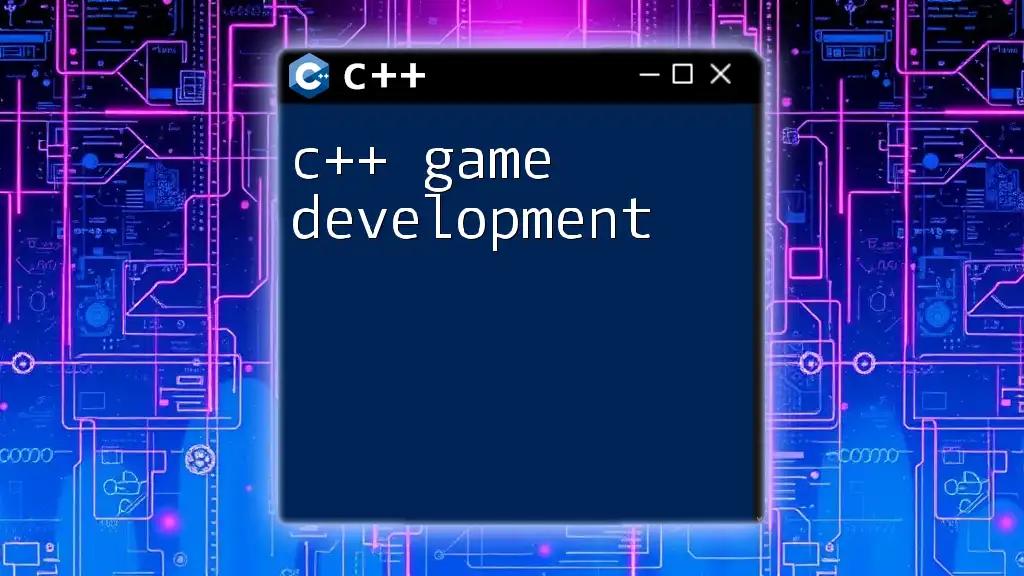
Additional Resources
To enhance your learning experience, consider exploring recommended books, online courses, and community forums dedicated to C++ game development. By engaging with these resources, you will continually expand your knowledge and skills in this exciting field.