C++ freeware refers to freely available software tools and libraries that enhance C++ programming, enabling developers to create applications without licensing costs.
Here’s a simple example of a C++ program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Freeware?
Freeware refers to software that is available at no cost to the user, granting them the rights to use, copy, or distribute it without any monetary exchange. Unlike open-source software, where the source code is available for alteration, freeware often restricts modifications and redistribution of the original program. This category of software is crucial for developers, especially those starting their journey in programming, as it allows accessibility to powerful tools without financial constraints.
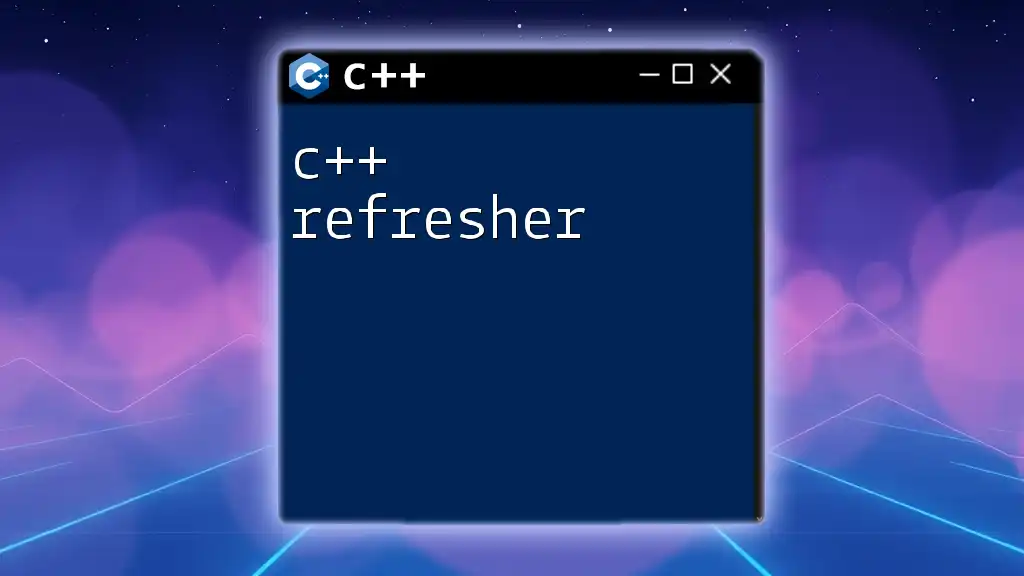
Why Choose C++ for Freeware Development?
C++ is a powerful, high-performance programming language that supports procedural, object-oriented, and generic programming. It is widely recognized for its versatility and efficiency, making it ideal for both system-level applications and large-scale software projects. Many well-known applications, such as Adobe applications and the Microsoft Office suite, are built using C++. With the availability of free resources and tools, you can utilize C++ without incurring any costs, making it a favorable choice for both budding and seasoned developers.
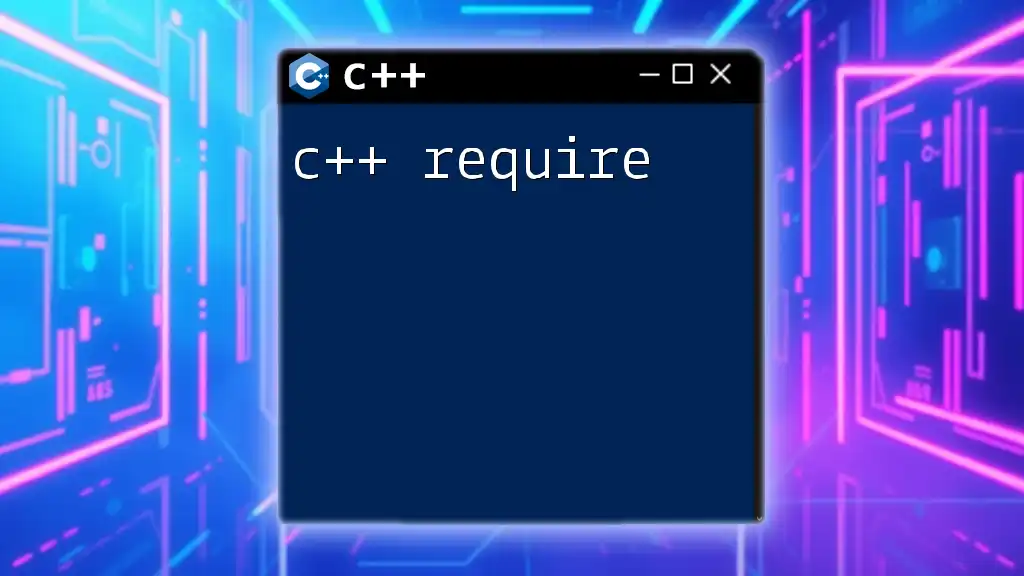
Free C++ Development Tools
Overview of Free C++ IDEs
An Integrated Development Environment (IDE) is essential for writing, testing, and debugging code efficiently. By providing a user-friendly interface and advanced features, an IDE simplifies the development process. Developers can benefit from syntax highlighting, code completion, debugging tools, and project management capabilities.
Top Free C++ IDEs
Code::Blocks
Features of Code::Blocks
Code::Blocks is a free and open-source IDE that is both flexible and feature-rich. It supports multiple compilers, including GCC and MSVC. Due to its customizable interface, developers can easily modify layouts and tools to fit their needs.
Installation and Setup
To install Code::Blocks, you can download the appropriate version from the [Code::Blocks website](http://www.codeblocks.org/downloads/26). Follow the installation wizard. Once installed, configure the compiler by going to Settings > Compiler and make sure to select the correct compiler you have on your system.
Example Code Snippet
#include <iostream>
using namespace std;
int main() {
cout << "Hello, Code::Blocks!" << endl;
return 0;
}
This simple code snippet demonstrates outputting text to the console, offering newcomers a quick way to test their IDE setup.
Dev-C++
Features of Dev-C++
Dev-C++ is another free IDE that is lightweight and offers essential features such as an integrated debugger and support for multiple compilers. It is particularly suitable for students and beginners.
How to Get Started with Dev-C++
You can download Dev-C++ from [Bloodshed's Dev-C++ page](http://www.bloodshed.net/devcpp.html). The installation process is straightforward. Post installation, you can start a new project using File > New > Project.
Example Code Snippet
#include <iostream>
using namespace std;
int main() {
cout << "Welcome to Dev-C++!" << endl;
return 0;
}
Running this snippet enables users to familiarize themselves with coding and testing functionality in the environment.
Visual Studio Community
Overview of Visual Studio Community
Visual Studio Community is a free IDE offered by Microsoft, designed specifically for individual developers and small teams. It comes with an extensive feature set, including support for C++ and a variety of extensions available through its marketplace.
Setting Up Visual Studio for C++
To configure Visual Studio for C++ development, visit the [Visual Studio download page](https://visualstudio.microsoft.com/free-developer-offers/), and choose the appropriate installer. During installation, ensure you select the "Desktop development with C++" workload to set up the necessary tools.
Example Code Snippet
#include <iostream>
using namespace std;
int main() {
cout << "Hello from Visual Studio!" << endl;
return 0;
}
This snippet will allow you to see the IDE in action while testing simple C++ commands.
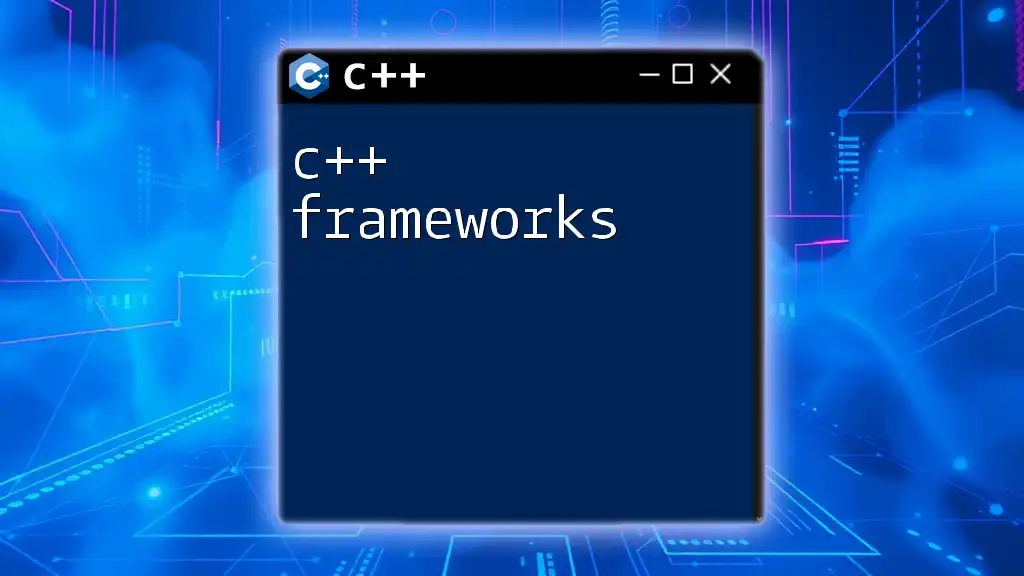
Free C++ Compilers
Importance of Using a Good Compiler
A compiler translates high-level code into machine language, enabling executable files to run on the target machine. A quality compiler not only improves performance but also assists in identifying errors during the coding and testing phases.
Recommended Free C++ Compilers
GCC (GNU Compiler Collection)
What is GCC?
GCC is a well-known, widely-used set of compilers that supports various programming languages, including C++. Its cross-platform functionality enables developers to compile programs on different operating systems.
Installation Instructions
To install GCC, users can follow platform-specific guides:
- Windows: Use [MinGW](http://www.mingw.org/) for a simple installation.
- Linux: Use package managers like `apt` or `yum` to install it:
sudo apt install build-essential
- macOS: Install Xcode which includes GCC.
Example Command
g++ -o hello hello.cpp
This command compiles `hello.cpp` into an executable named `hello`.
Clang
Overview of Clang
Clang is a compiler front end for the C family of programming languages developed as a part of the LLVM project. It is known for its fast compilation and modern code analysis features.
Installation and Usage
You can install Clang using platform-specific methods:
- Windows: Download it from the official [LLVM website](https://llvm.org/).
- Linux: You can install it via:
sudo apt install clang
- macOS: Clang comes pre-installed with Xcode.
Example Command
clang++ -o hello hello.cpp
This command will compile the `hello.cpp` file into an executable using Clang.
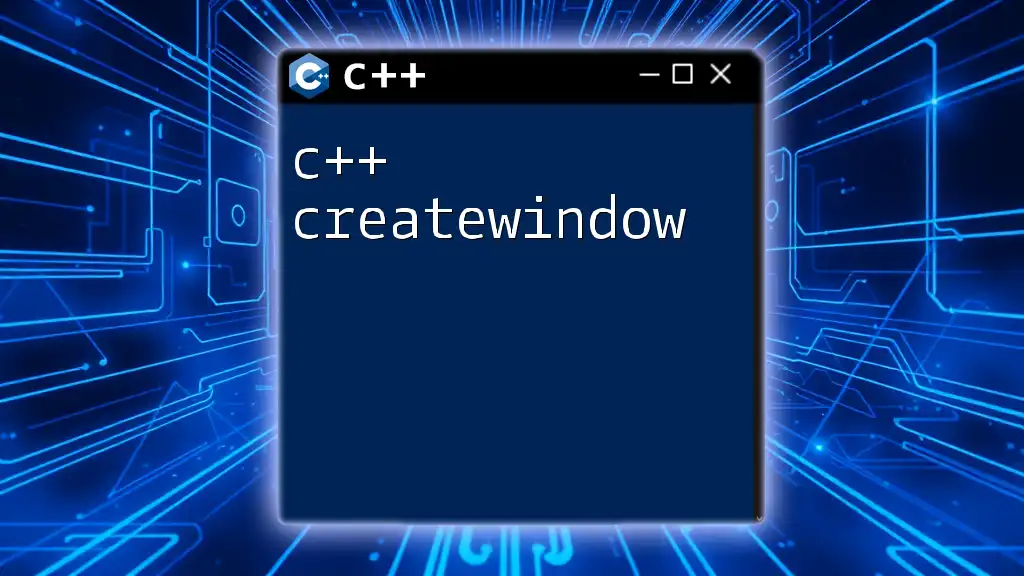
Free C++ Libraries and Frameworks
Advantages of Using Libraries
Using libraries in C++ allows developers to leverage pre-written, optimized code that can save time and increase productivity. Libraries can handle complex tasks, such as graphics rendering or network communication, freeing developers to focus on the unique aspects of their application.
Notable Free C++ Libraries
Boost
What is Boost?
Boost is a collection of high-quality C++ libraries that extend the functionality of C++. The libraries cover various domains, from smart pointers to multi-threading and regular expressions.
Example Usage
To include Boost in your project, you need to link it correctly during compilation. Here’s a simple example demonstrating string manipulation:
#include <boost/algorithm/string.hpp>
Qt
Introduction to the Qt Framework
Qt is a powerful, cross-platform framework used for developing GUI applications. It offers a comprehensive set of libraries and tools to create both simple and complex applications quickly.
Installation Process
You can download Qt from the [Qt official website](https://www.qt.io/download). Choose the open-source version and follow the instructions for installation.
Example Code Snippet
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, Qt!");
button.show();
return app.exec();
}
This snippet sets up a basic Qt application with a single button.
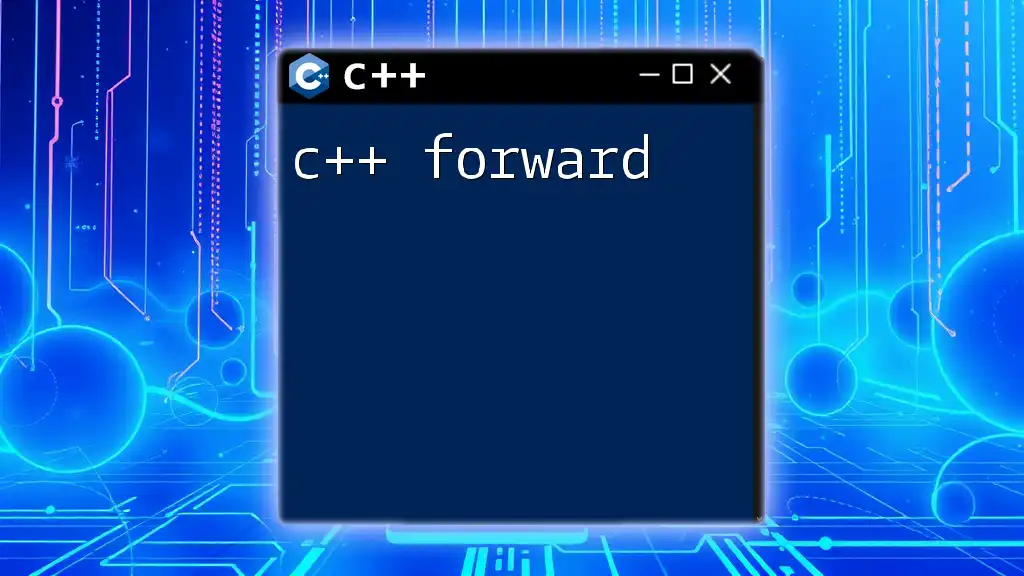
Recap of Free C++ Resources
In this guide, we have covered a variety of C++ freeware, including some of the best free IDEs like Code::Blocks, Dev-C++, and Visual Studio Community. We also discussed valuable free compilers such as GCC and Clang, and we introduced the utility of libraries like Boost and Qt, essential for developing feature-rich applications.
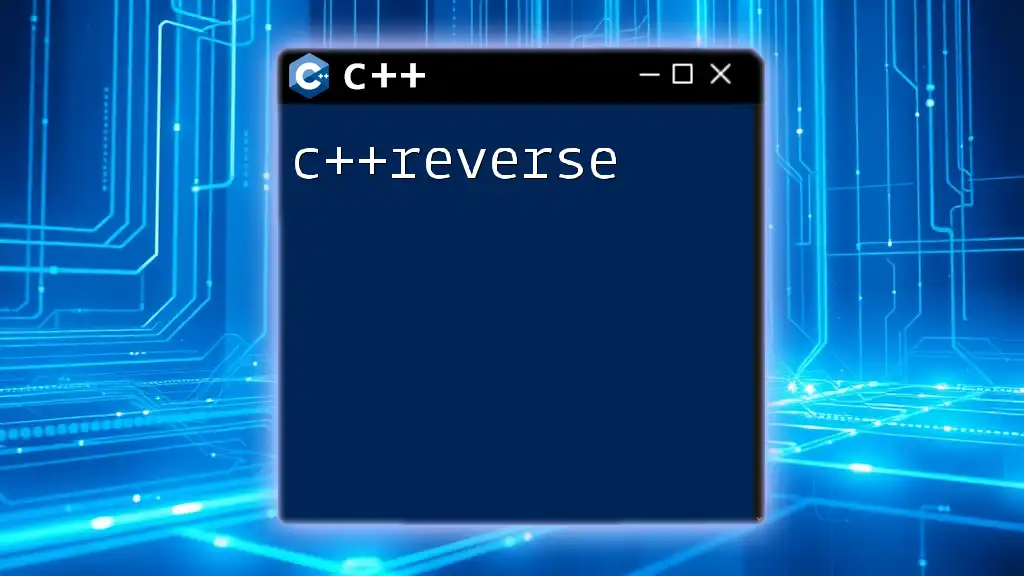
Encouragement to Explore
Exploring these free resources can significantly enhance your C++ programming skills. Whether you are a beginner or a seasoned developer, the tools and libraries discussed in this article offer a pathway to success. Don't hesitate to dive into coding, creating your projects, and experimenting with different libraries!
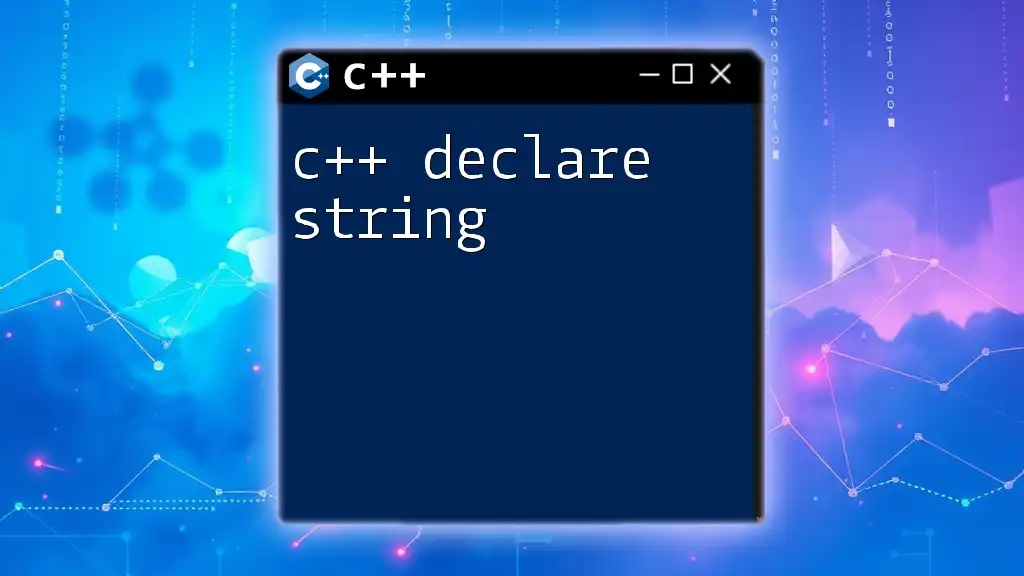
Additional Resources
- Links to Downloads: Directly access the respective websites to download the free IDEs, compilers, and libraries mentioned.
- Recommended Tutorials and Book Resources: Consider exploring online tutorials, courses, and books dedicated to C++ to further bolster your knowledge and skills.