C++ software design refers to the systematic approach of structuring and organizing C++ code to enhance its efficiency, maintainability, and scalability, often utilizing principles such as encapsulation, inheritance, and polymorphism.
Here's a simple example illustrating the use of classes in C++:
class Shape {
public:
virtual void draw() = 0; // Pure virtual function
};
class Circle : public Shape {
public:
void draw() override {
// Implementation for drawing a circle
}
};
class Square : public Shape {
public:
void draw() override {
// Implementation for drawing a square
}
};
Principles of Software Design
Solid Principles in C++
Single Responsibility Principle (SRP)
The Single Responsibility Principle mandates that a class should have only one reason to change, meaning it should only have one job. In a C++ context, this drives us to create more focused, cohesive classes.
Example Code Snippet:
class User {
public:
void registerUser() { /* registration logic */ }
void notifyUser() { /* notification logic */ }
};
In the example above, the `User` class is trying to do two things: registration and notification. This violates SRP. Instead, we can refactor it into two separate classes—`UserRegistration` and `UserNotifier`—each dedicated to one task. This change not only adheres to SRP but also enhances maintainability.
Open/Closed Principle (OCP)
The Open/Closed Principle states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. In C++, this principle encourages building a flexible design that accommodates new features with minimal impact on existing code.
Example Code Snippet:
class Shape {
public:
virtual double area() = 0; // open for extension
};
class Circle : public Shape {
public:
Circle(double radius) : radius(radius) {}
double area() override { return 3.14 * radius * radius; }
private:
double radius;
};
By creating an abstract `Shape` class, we make it easy to add new shapes without changing existing code. This allows developers to extend the program by creating new subclasses without modifying the core logic.
Liskov Substitution Principle (LSP)
The Liskov Substitution Principle asserts that if S is a subtype of T, then objects of type T should be replaceable with objects of type S without altering the correctness of the program. In other words, derived classes must be substitutable for their base classes.
Example Code Snippet:
class Bird {
public:
virtual void fly() = 0;
};
class Sparrow : public Bird {
public:
void fly() override { /* flying logic */ }
};
class Ostrich : public Bird {
public:
void fly() override { throw std::logic_error("Ostrich can't fly!"); }
};
In this scenario, the `Ostrich` class breaks LSP because it cannot substitute in place of the `Bird` class without introducing errors. In a LSP-compliant design, we would perhaps reconsider our hierarchy or use interfaces that better encapsulate the capabilities of various bird types.
Design Patterns in C++
What are Design Patterns?
Design patterns are typical solutions to common problems in software design. They represent best practices and facilitate reuse of design ideas. Understanding and applying design patterns can significantly improve the structure and flexibility of your C++ software designs.
Creational Patterns
Factory Method
The Factory Method pattern defines an interface for creating an object but allows subclasses to alter the type of objects that will be created. This promotes loose coupling and adherence to the OCP.
Example Code Snippet:
class Shape {
public:
virtual double area() = 0; // interface for various shapes
};
class Circle : public Shape {
public:
double area() override { /* area calculation */ }
};
class ShapeFactory {
public:
static Shape* createShape(const std::string& type) {
if (type == "circle") return new Circle();
// Additional shape cases...
}
};
In this example, `ShapeFactory` encapsulates the creation logic, allowing new shapes to be added without altering the existing code base, adhering to the OCP.
Structural Patterns
Adapter Pattern
The Adapter Pattern allows incompatible interfaces to work together. It acts as a bridge between two incompatible interfaces.
Example Code Snippet:
class OldSystem {
public:
void doSomethingOld() { /* old implementation */ }
};
class Adapter {
OldSystem* oldSystem;
public:
Adapter(OldSystem* os) : oldSystem(os) {}
void doSomething() {
oldSystem->doSomethingOld();
}
};
Here, the `Adapter` makes the `OldSystem` instance compatible with a new interface, allowing it to be used seamlessly in a modern context.
Behavioral Patterns
Observer Pattern
The Observer Pattern is a behavioral pattern that establishes a one-to-many dependency between objects, allowing multiple observers to be notified of changes in the subject's state.
Example Code Snippet:
class Observer {
public:
virtual void update() = 0;
};
class Subject {
std::vector<Observer*> observers;
public:
void attach(Observer* observer) {
observers.push_back(observer);
}
void notify() {
for (Observer* observer : observers) {
observer->update();
}
}
};
In this snippet, when the subject's state changes, it calls the `notify()` function, which updates all registered observers, thereby promoting loose coupling and enhanced system modularity.
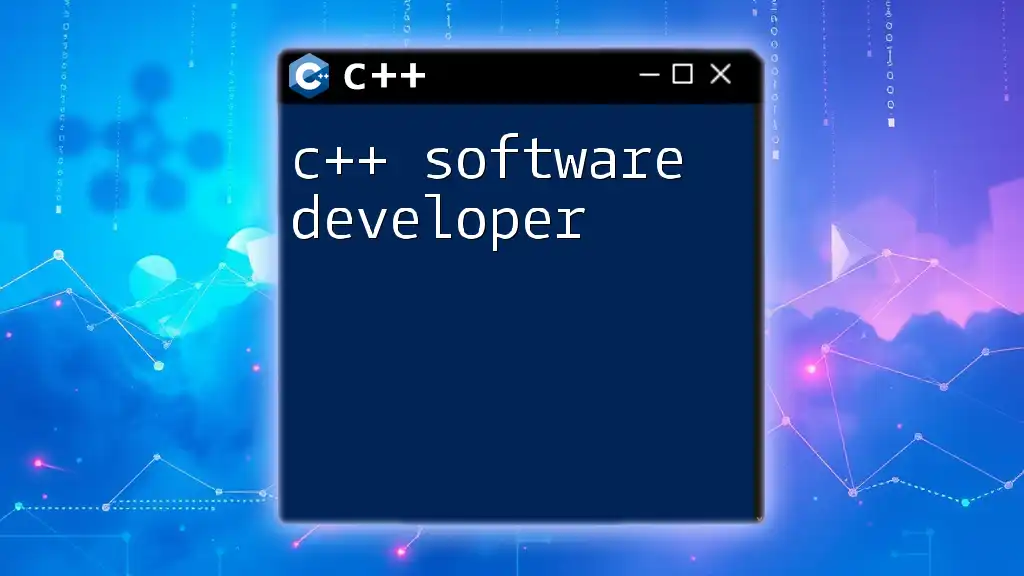
Best Practices in C++ Software Design
Code Modularity
Creating modular code has numerous benefits. Modularity improves readability, making it easier for teams to navigate and comprehend the codebase. Additionally, it enhances maintainability; isolated modules can be modified independently, reducing the risk of introducing bugs into the system.
Consistent Naming Conventions
Adhering to a consistent naming convention throughout your project is essential. It promotes readability and helps new developers understand your code faster. Good naming practices involve being descriptive yet concise. For instance, using names like `calculateTotal` instead of vague terms like `doStuff` provides clarity on what the function achieves.
Documentation and Comments
Robust documentation is the backbone of maintainable software. Good comments elucidate complex logic, clarify intent, and provide context. Aim to explain why certain decisions were made in addition to what the code does. This makes the development easier for current and future developers.
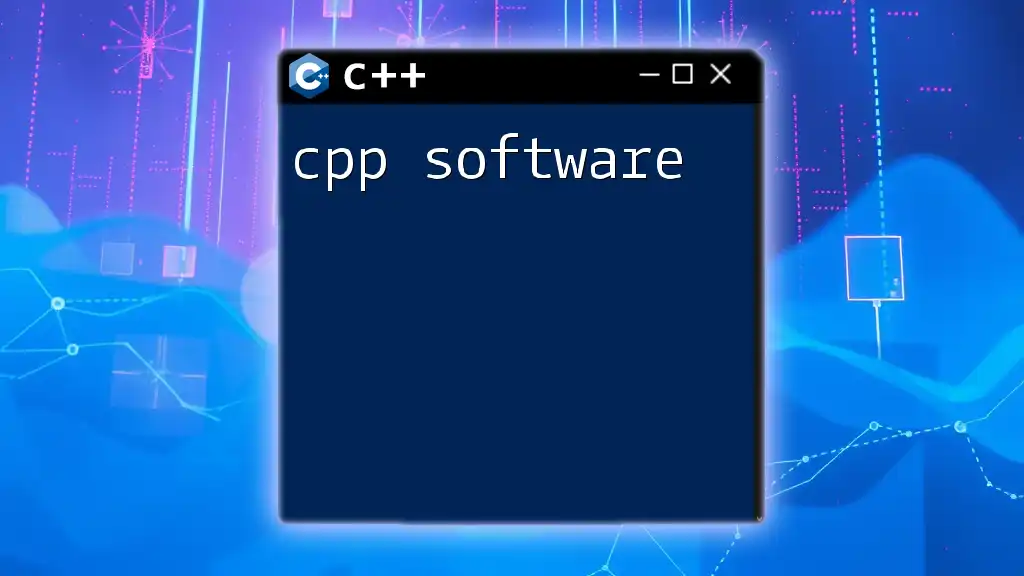
Testing and Validation in C++ Software Design
Unit Testing
Unit testing is an essential part of ensuring your system correctness. It involves testing individual components of your system to verify they are functioning as intended. This process makes it easier to identify issues early in the development cycle.
Testing Frameworks
There are several testing frameworks available for C++, such as Google Test and Catch2, which facilitate the process of writing and organizing tests. These frameworks provide substantial infrastructure to streamline the unit testing process, making it less tedious and more efficient.
Code Reviews
Code reviews enhance code quality and foster collaboration among team members. They encourage knowledge sharing and ensure adherence to the coding standards established by the team or organization. A thorough review process helps catch bugs and design flaws before they become entrenched in the codebase.
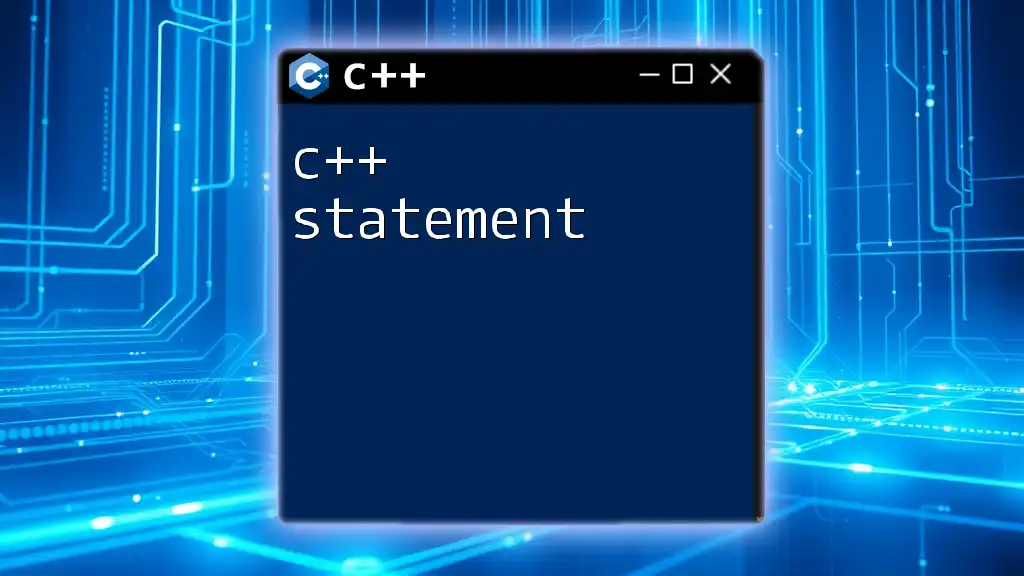
Conclusion
C++ software design encompasses a range of principles, design patterns, and best practices aimed at producing robust, maintainable code. By adhering to the SOLID principles, utilizing design patterns, creating modular code, and implementing rigorous testing, you set the stage for successful software development. Engaging with these concepts will not only enhance your skills but also fortify your projects, paving the way for future innovations. Explore further resources, enroll in courses, and immerse yourself in the vibrant C++ community to continue your journey in mastering C++ software design.