A C++ testing framework provides tools and libraries that facilitate the creation and execution of automated tests, ensuring code reliability and quality.
Here's an example of a simple test case using the Google Test framework:
#include <gtest/gtest.h>
int add(int a, int b) {
return a + b;
}
TEST(AdditionTest, HandlesPositiveInput) {
EXPECT_EQ(add(2, 3), 5);
EXPECT_EQ(add(10, 20), 30);
}
int main(int argc, char **argv) {
::testing::InitGoogleTest(&argc, argv);
return RUN_ALL_TESTS();
}
Understanding the Importance of Testing in C++
Why Testing is Essential
In software development, testing is crucial for several reasons. First and foremost, it helps identify defects and bugs early in the development process, reducing the cost of fixing them later. When issues arise in production due to untested code, they can lead to financial losses, damage to reputation, and decreased customer trust.
Unit testing, specifically, is a technique where individual components or functions of the software are tested. By validating small, isolated pieces of code, developers can ensure that each part behaves as expected before integrating it into larger systems. This proactive approach allows for more robust and reliable software.
Overview of Unit Testing
Unit tests are designed to validate the functionality of individual components of your codebase. The benefits of unit testing include:
- Immediate feedback: When changes are made to code, unit tests provide instant feedback to developers about the validity of their updates.
- Improved design: Writing tests forces developers to think about how their code is structured. It encourages modular design, ultimately leading to better-structured programs.
- Regression protection: Once a unit test is written, it can prevent future code changes from unintentionally breaking existing functionality.
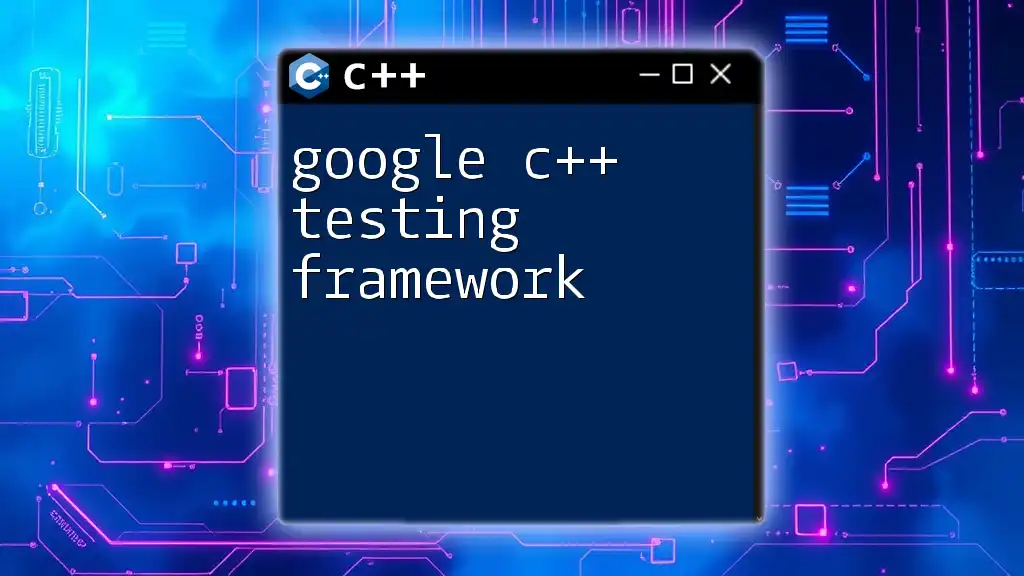
Choosing the Right C++ Test Framework
Introduction to C++ Test Frameworks
Choosing a C++ testing framework is essential for the success of your unit testing strategy. An effective framework helps streamline testing processes and can boost developer productivity. It can make it easier to write and maintain tests, view results, and integrate with build systems.
When evaluating frameworks, consider factors such as documentation, community support, and the specific features that meet your project's needs.
Popular C++ Unit Testing Frameworks
Several C++ testing frameworks are widely used in the industry. Some notable ones include:
- Google Test: Developed by Google, this is one of the most popular C++ testing frameworks. Known for its rich feature set and ease of use.
- Catch2: A single-header C++ testing framework that is easy to integrate into any project and has a clean syntax.
- Boost.Test: Part of the Boost Libraries, it provides a flexible framework suited for large applications.
Each of these frameworks has its own strengths, making them suitable for different projects and team preferences.
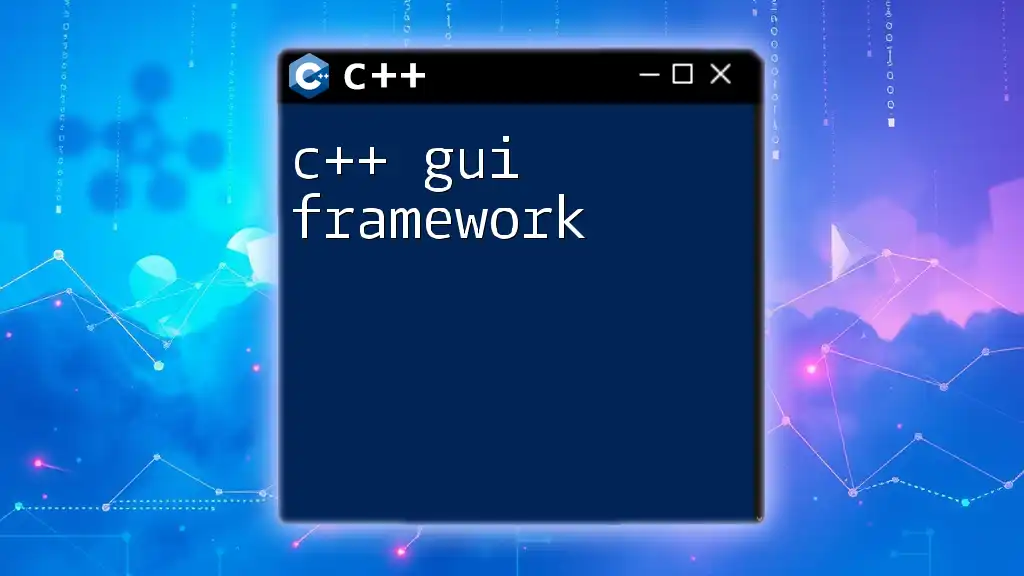
Exploring C++ Testing Framework Features
Key Features to Look For
When selecting a C++ test framework, consider features such as:
- Test fixture support: This allows you to set up common objects or states needed for multiple tests.
- Mocking capabilities: Essential for isolating tests by replacing dependencies with mocked objects.
- Suite management: The ability to organize tests into suites for better structure and execution control.
- Custom assertions: Enables developers to create more informative test outcomes that suit their application needs.
Installation and Setup
To illustrate the setup process, let’s look at installing Google Test. Here are the steps:
-
Clone the repository:
git clone https://github.com/google/googletest.git cd googletest
-
Build the library:
mkdir build cd build cmake .. make
-
Install the library (optional, depending on your needs):
sudo make install
This sets the stage for creating and executing your unit tests using Google Test.
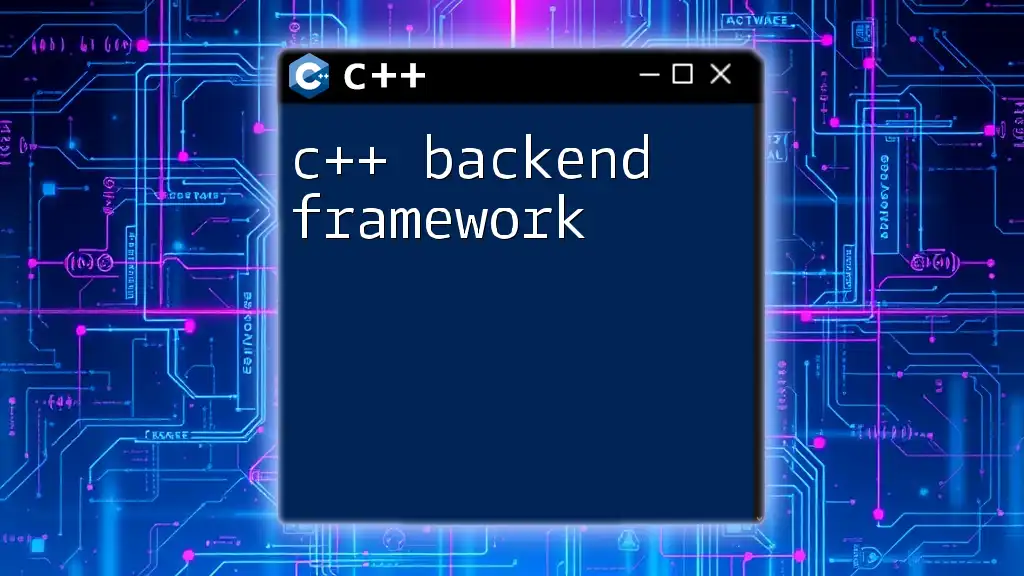
Getting Started with C++ Unit Testing Frameworks
Writing Your First Unit Test
Now that your testing framework is set up, let’s write a simple unit test. The syntax for writing tests is generally the same across frameworks. Here, we’ll use Google Test:
#include <gtest/gtest.h>
// Function to be tested
int Add(int a, int b) {
return a + b;
}
// Test case
TEST(AddTest, BasicAssertions) {
EXPECT_EQ(Add(1, 2), 3);
EXPECT_EQ(Add(-1, 1), 0);
}
In this example, we define a function `Add`, then create a test case for it. The assertions `EXPECT_EQ` check that the expected results match the function's output. If they do not, the test fails, clearly indicating that there is an issue.
Running Your Tests
Once you have written your tests, running them is straightforward. Compile the test file along with the testing framework’s library. For example, if you have named your test file `test_add.cpp`, you can compile and run it as follows:
g++ -o test_add test_add.cpp -lgtest -lpthread
./test_add
When you run the compiled program, Google Test will execute all your test cases and provide a summary of the results.
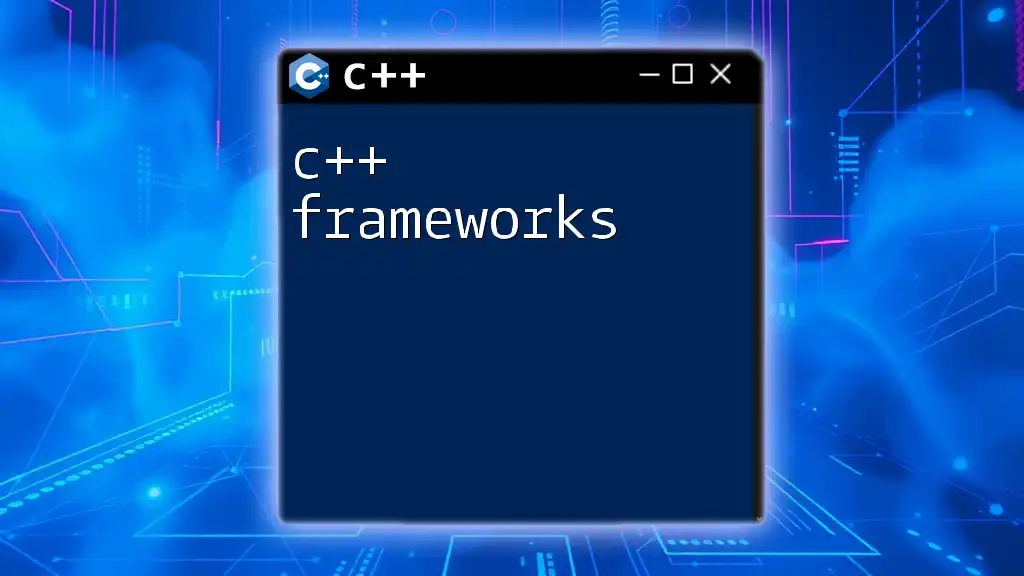
Advanced Testing Techniques
Test Fixtures
Test fixtures are an excellent way to organize tests by creating a fixed baseline within a class from which tests can be drawn. This concept is particularly useful when multiple tests share common setup and teardown processes.
Here’s a quick example of using a test fixture with Google Test:
class MyTest : public ::testing::Test {
protected:
void SetUp() override {
// Code to run before each test
// e.g., initialize a resource or structure
}
void TearDown() override {
// Code to run after each test
// e.g., clean up resources
}
};
In this example, any tests derived from `MyTest` will automatically call the `SetUp` before each individual test and `TearDown` after execution.
Mocking in Unit Tests
Mocking reduces dependencies in your tests by "faking" external interactions. This is particularly helpful when a function calls functions from other classes or external libraries.
With Google's Mocking framework, you can create mock objects to test your code with controlled behaviors. Here’s a simple example of a mock test involving a database class:
#include <gmock/gmock.h>
// Mock Database class
class MockDatabase {
public:
MOCK_METHOD(void, Connect, ());
};
// Test case using the mock
TEST(DatabaseTest, ConnectTest) {
MockDatabase mockDb;
EXPECT_CALL(mockDb, Connect());
// Invoke the Connect method
mockDb.Connect();
}
In this case, the `EXPECT_CALL` sets an expectation that the `Connect` method will be called, and the test will pass if this expectation is met.
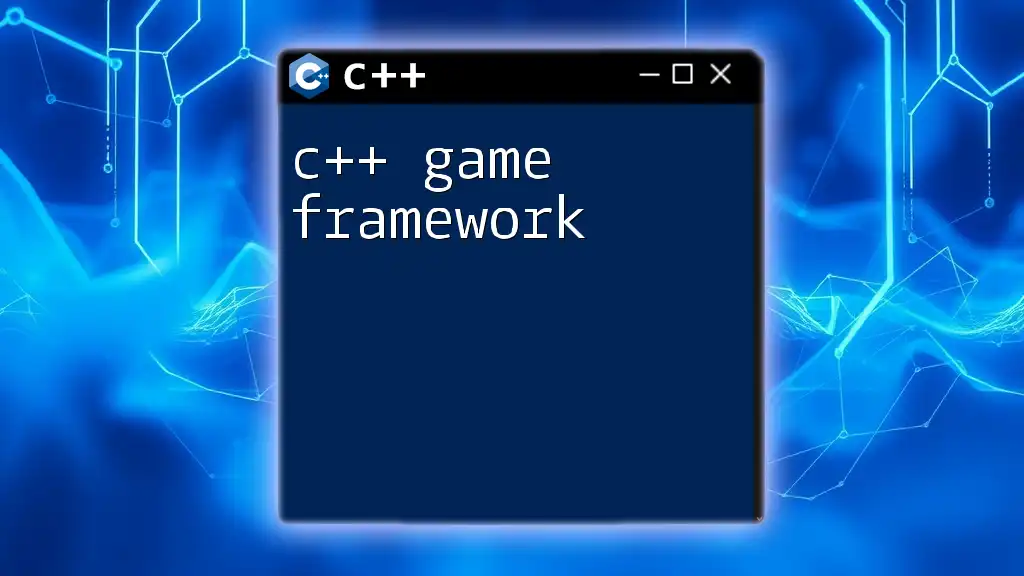
Best Practices for C++ Unit Testing
Writing Effective Tests
Effective unit tests should be:
- Independent: Each test should be able to run in isolation without dependencies on other tests.
- Readable: Clear and descriptive names help understand what the test is checking without diving into implementation details.
- Fast: Unit tests need to run quickly to maintain developer productivity.
The concept of "Arrange, Act, Assert" is a helpful pattern for structuring tests. Arrange your setup, Act on the code being tested, and Assert the outcome to ensure accuracy.
Maintaining and Organizing Tests
Maintaining and organizing tests is pivotal for scalability and collaboration. Consider the following strategies:
- Group tests by functionality or module.
- Use naming conventions for test suites and cases that reflect their purpose.
- Regularly review and refactor tests to eliminate redundancy.
Documentation on what each test does will also foster better collaboration in team environments.
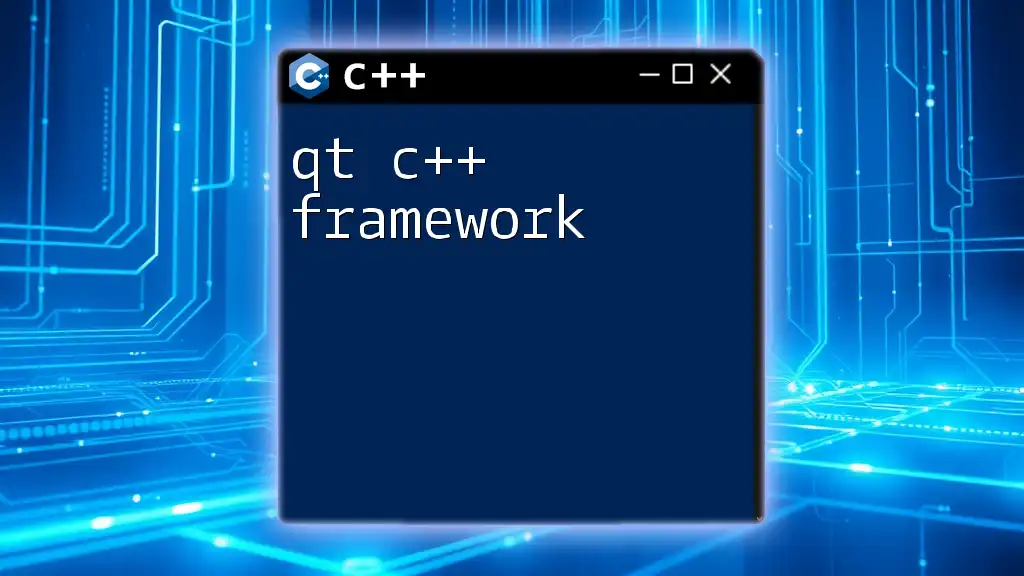
Integrating C++ Testing Framework With CI/CD
Continuous Integration and Testing
Integrating your tests into a Continuous Integration (CI) pipeline adds significant value by ensuring that your tests run automatically with each code change. This practice helps catch issues early and enhances the reliability of your codebase.
Choosing the right tools, like Jenkins or GitHub Actions, can facilitate this integration, allowing automated builds and test executions whenever changes are pushed to your repository.
Automating Unit Tests
Setting up automated tests in your CI/CD pipeline is crucial to maintaining code quality. Here’s an example of a simple CI configuration using GitHub Actions:
name: C++ CI
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up C++ environment
run: sudo apt-get install g++ cmake
- name: Build and run tests
run: |
mkdir build
cd build
cmake ..
make
./your_test_executable
This configuration checks out your code, sets up the build environment, builds your code and runs the tests each time you push changes.
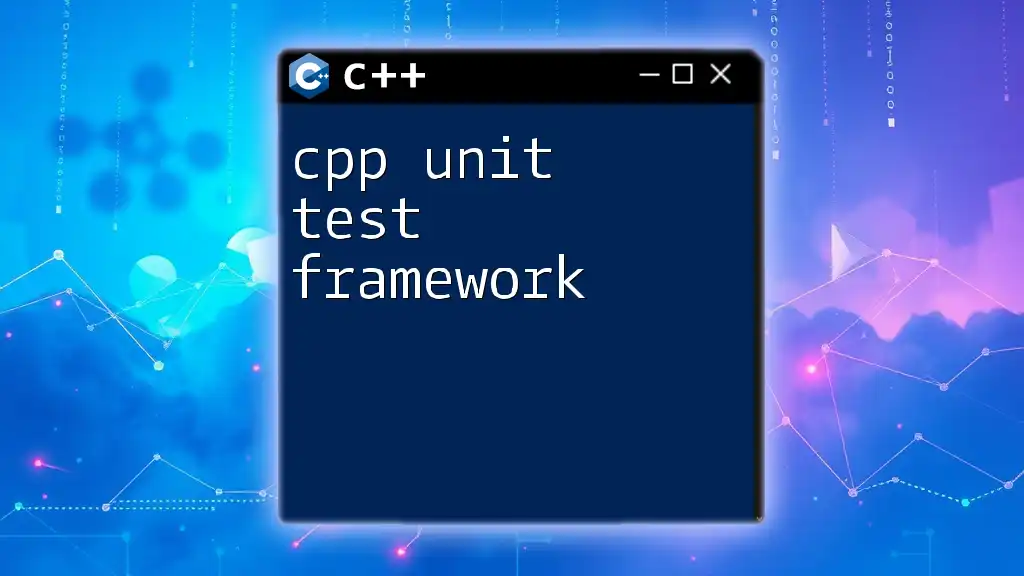
Conclusion
In summary, C++ testing frameworks play a vital role in ensuring code quality and reliability in software development. By adopting practices such as unit testing, using an appropriate testing framework, and integrating testing into CI/CD workflows, developers can significantly boost their productivity and maintain higher project standards.
Take the time to choose a framework that aligns with your project's needs, write effective tests, and leverage automation in your development process. In doing so, you'll foster a more resilient codebase that stands the test of time.
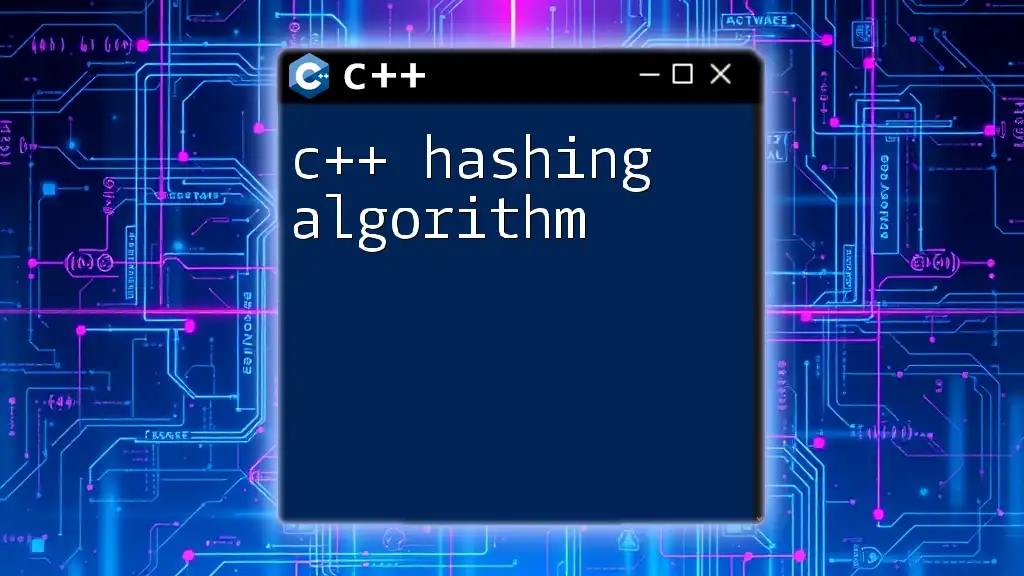
Further Resources
To deepen your understanding of C++ testing frameworks and practices, consider exploring the following resources:
- Books on unit testing, such as "Test-Driven Development for Embedded C++" or "Growing Object-Oriented Software, Guided by Tests."
- Online courses that focus on C++ development and testing methodologies.
- Relevant documentation and community forums such as Stack Overflow, GitHub Discussions, and the Google Test official documentation.
Connecting with other developers can provide insights, alternative approaches, and enhance your understanding of effective unit testing in C++.