The Google C++ Testing Framework, also known as Google Test, is a popular open-source framework designed to simplify the process of writing and running C++ unit tests, enabling developers to ensure their code behaves as expected.
Here’s a simple example of how to use Google Test to create and run a basic test:
#include <gtest/gtest.h>
// Function to add two numbers
int add(int a, int b) {
return a + b;
}
// Test case for the add function
TEST(AddTest, HandlesPositiveInput) {
EXPECT_EQ(add(2, 3), 5);
EXPECT_EQ(add(-1, 1), 0);
}
int main(int argc, char **argv) {
::testing::InitGoogleTest(&argc, argv);
return RUN_ALL_TESTS();
}
What is Google C++ Testing Framework?
The Google C++ Testing Framework, commonly known as googletest, is a powerful and versatile library that simplifies the process of writing and executing tests for C++ code. It emphasizes the significance of unit testing in software development, allowing developers to catch bugs early, enhance code reliability, and improve maintainability.
What sets googletest apart from other testing frameworks is its rich feature set, which includes support for different types of assertions, test fixtures, and automatic test discovery. This robust functionality enables developers to write effective unit tests efficiently.
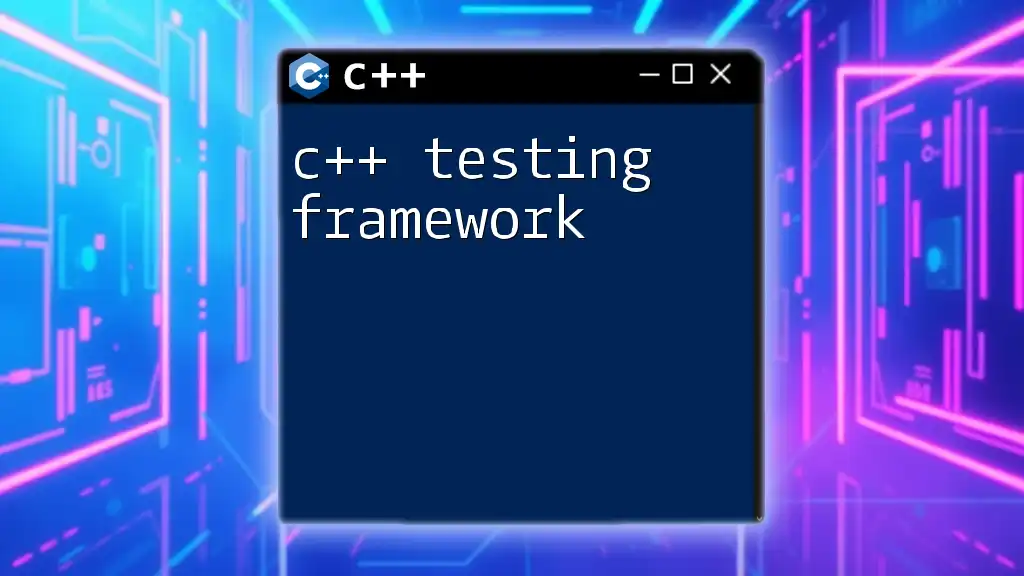
Getting Started with Googletest
Setting Up Your Environment
To get started with googletest, you need a few prerequisites:
- C++ Compiler: Ensure you have a modern C++ compiler installed (GCC, Clang, or MSVC).
- CMake: This tool will simplify the process of building your project.
Installation Instructions:
-
Clone the repository:
git clone https://github.com/google/googletest.git
-
Build and install googletest:
cd googletest mkdir build cd build cmake .. make sudo make install
Creating Your First Test Case
Once the setup is complete, it's time to create a simple test case. The basic structure of a test case in googletest is straightforward. For instance, consider the following example:
#include <gtest/gtest.h>
TEST(SimpleTest, PositiveAssertion) {
EXPECT_EQ(1, 1);
}
In this snippet, we include the necessary header file and define a test named `SimpleTest`. Inside the test, we're asserting that `1` equals `1` using `EXPECT_EQ()`. If this condition holds true, the test passes; otherwise, it fails.
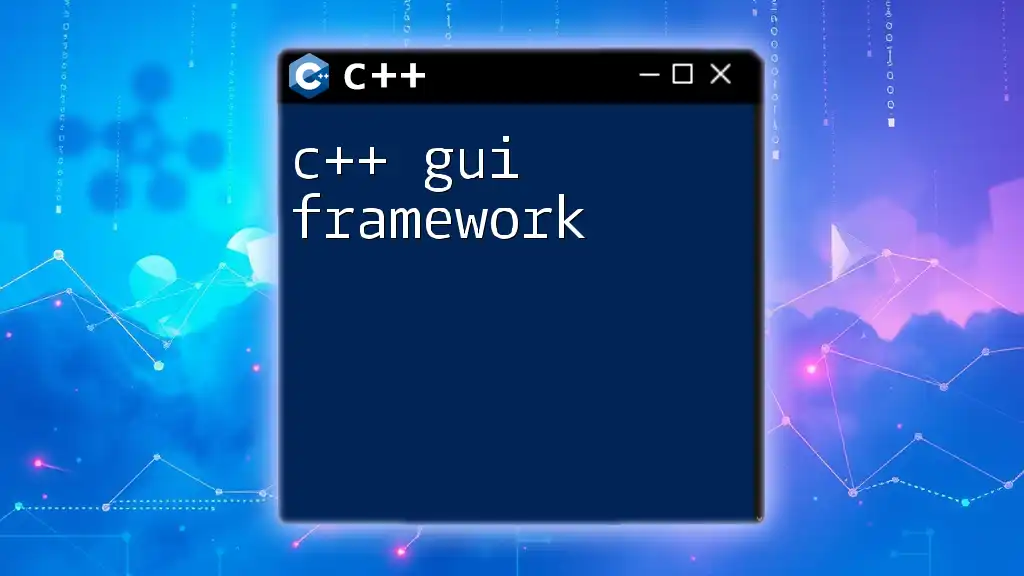
Writing Effective Tests
Test Fixtures
Test fixtures are an essential feature of googletest, allowing you to define common setup and teardown behaviors for a group of tests. This is particularly useful for avoiding code duplication.
Example of Using Test Fixtures:
class MyTest : public ::testing::Test {
protected:
void SetUp() override {
// Code that will be called before each test
}
void TearDown() override {
// Code that will be called after each test
}
};
TEST_F(MyTest, TestOne) {
// Test implementation here
}
In this example, the `MyTest` class inherits from `::testing::Test`, enabling the use of the `SetUp` and `TearDown` methods to prepare and clean up resources needed for the tests.
Assertions in Googletest
Assertions are crucial for determining the outcomes of tests. Googletest provides various assertion macros such as:
- `EXPECT_EQ()`: Checks for equality.
- `EXPECT_NE()`: Checks for inequality.
- `EXPECT_LT()`: Checks if one value is less than another.
Code Examples:
EXPECT_EQ(2 + 2, 4); // Asserts that the sum is correct
EXPECT_NE(3, 4); // Asserts that the numbers are not equal
EXPECT_LT(3, 5); // Asserts that 3 is less than 5
These assertions provide clear feedback during testing, helping you quickly identify incorrect logic in your code.
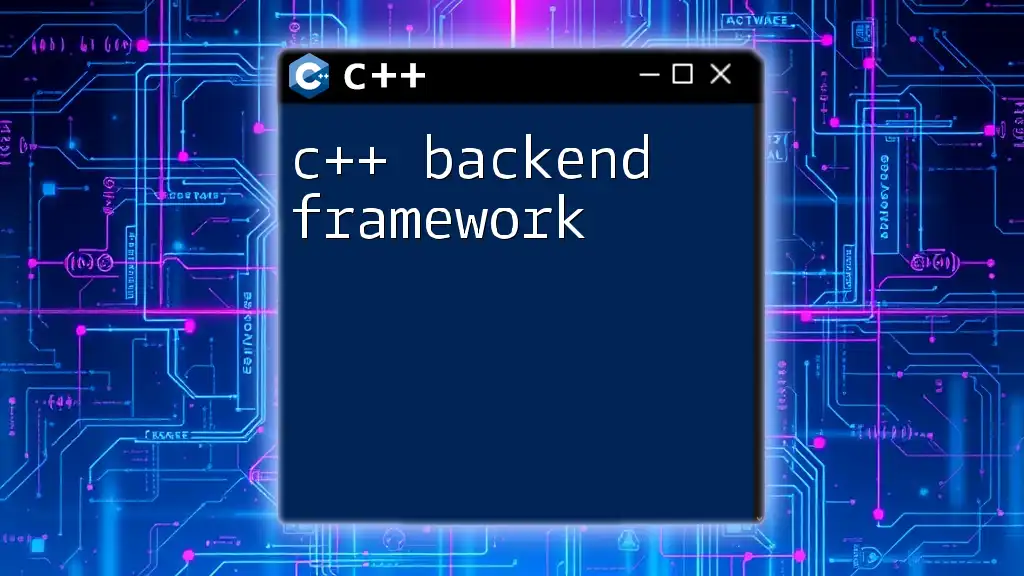
Advanced Features of Googletest
Parameterized Tests
Parameterized tests allow you to run the same test logic on different sets of input data. This reduces the amount of repetitive code and increases coverage.
Example:
class ParamTest : public ::testing::TestWithParam<int> {};
TEST_P(ParamTest, Example) {
EXPECT_GT(GetParam(), 10);
}
INSTANTIATE_TEST_SUITE_P(MyTests, ParamTest, ::testing::Values(1, 15, 20));
In the above code, the `ParamTest` class can be executed with multiple values, allowing you to easily validate different scenarios.
Mock Objects
Mock objects are invaluable when you need to simulate behavior and interaction with complex dependencies. They allow you to isolate the unit of work and test it independently.
Example:
class MockFoo {
public:
MOCK_METHOD(void, Bar, (int));
};
TEST(MockTest, MockMethod) {
MockFoo mock;
EXPECT_CALL(mock, Bar(5));
mock.Bar(5); // This call should match the expectation
}
In this example, `MockFoo` defines a mocked method called `Bar`, which we can configure expectations for. This flexibility is crucial for testing in environments that require dependencies.
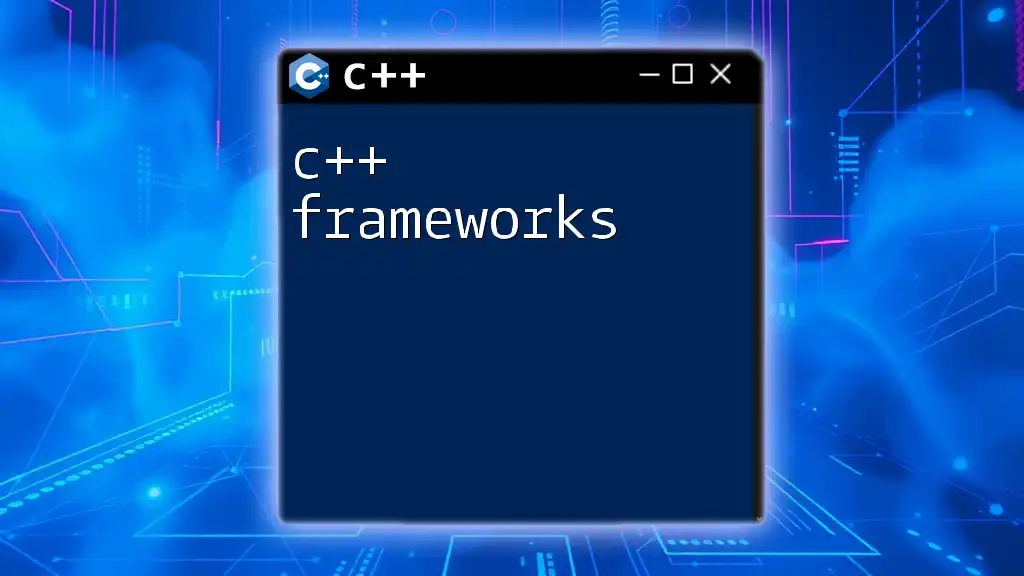
Organizing Your Tests
Directory Structure
Maintaining an organized directory structure for your tests is vital for a clean codebase. A recommended layout could include:
- /src: Source files
- /include: Header files
- /tests: Unit tests pertaining to your codebase
This well-organized structure helps in navigating and maintaining your tests efficiently.
Running Tests
Running tests in googletest can be done through a command line or integrated into your preferred development environment. You can compile your source and test files into an executable that runs your tests, providing an easy way to validate your code continuously.
Additionally, consider using tools to generate test coverage reports, which assist in identifying untested parts of your code.
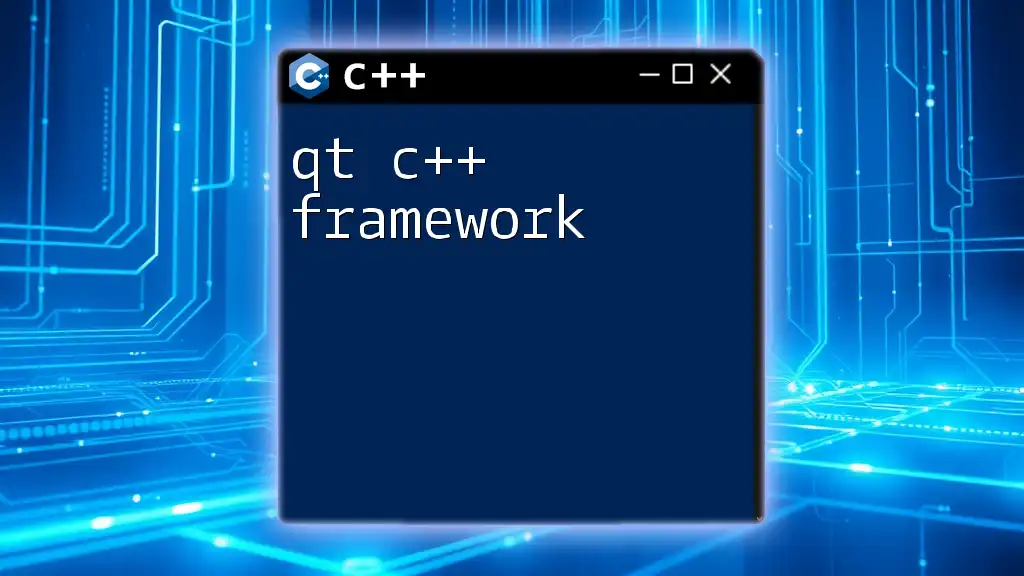
Best Practices for Testing in C++
Continuous Integration
Integrating testing into a Continuous Integration (CI) pipeline is crucial for modern software practices. CI tools such as Travis CI, CircleCI, or GitHub Actions can automate the testing process, ensuring that tests are consistently run every time changes are made to the codebase.
Writing Maintainable Tests
To ensure your tests remain maintainable:
- Write clear and descriptive names for your tests.
- Keep your tests independent—no test should rely on another.
- Regularly refactor your test code alongside production code to keep it clean and understandable.
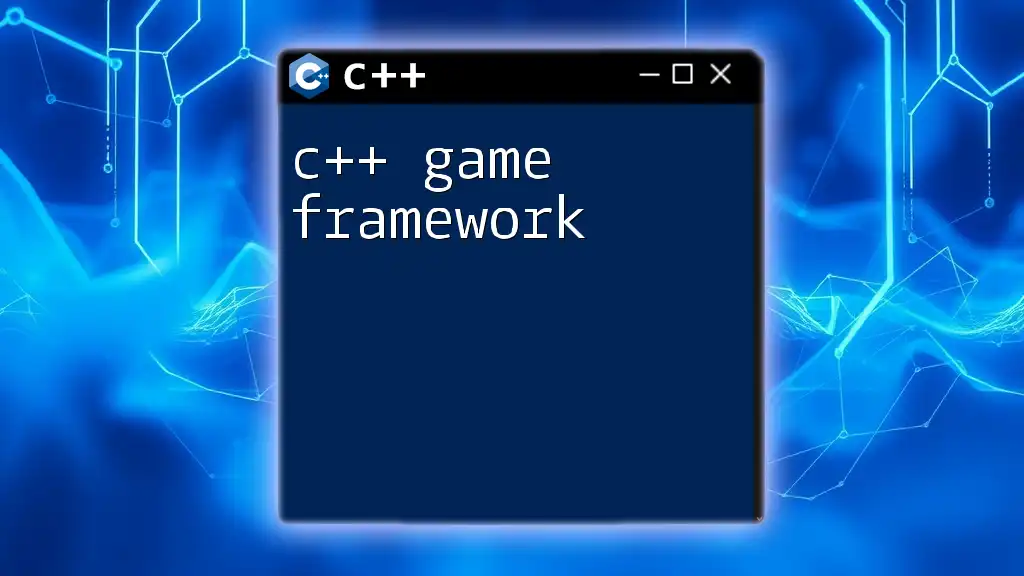
Common Pitfalls
When using the Google C++ Testing Framework, developers often encounter pitfalls like:
- Neglecting Edge Cases: Make sure to cover edge cases as they frequently lead to bugs.
- Complex Assertions: Keep assertions straightforward; complex logic can obscure what a test is meant to validate.
- Ignoring Compiler Warnings: Always pay attention to warnings, as these can lead to issues in tests.
Troubleshooting failed tests typically involves understanding the output that googletest provides. Digging into the lines of code where failures happen can illuminate potential fixes.
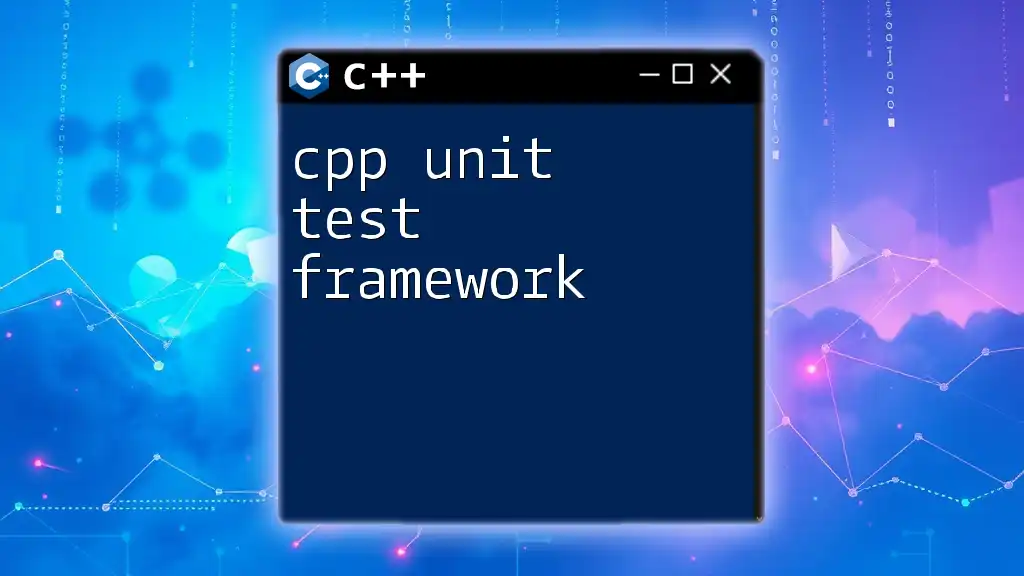
Conclusion
The Google C++ Testing Framework is an essential tool for developers seeking to implement effective unit testing in their C++ projects. Its flexibility, rich set of features, and straightforward syntax make it a go-to choice for ensuring code reliability. Embracing a testing culture in your projects encourages higher software quality and fosters improved maintainability.
By harnessing the power of googletest, you can significantly enhance your development workflow. As you continue to explore this framework, remember to subscribe for more tips and resources on C++ programming and testing!
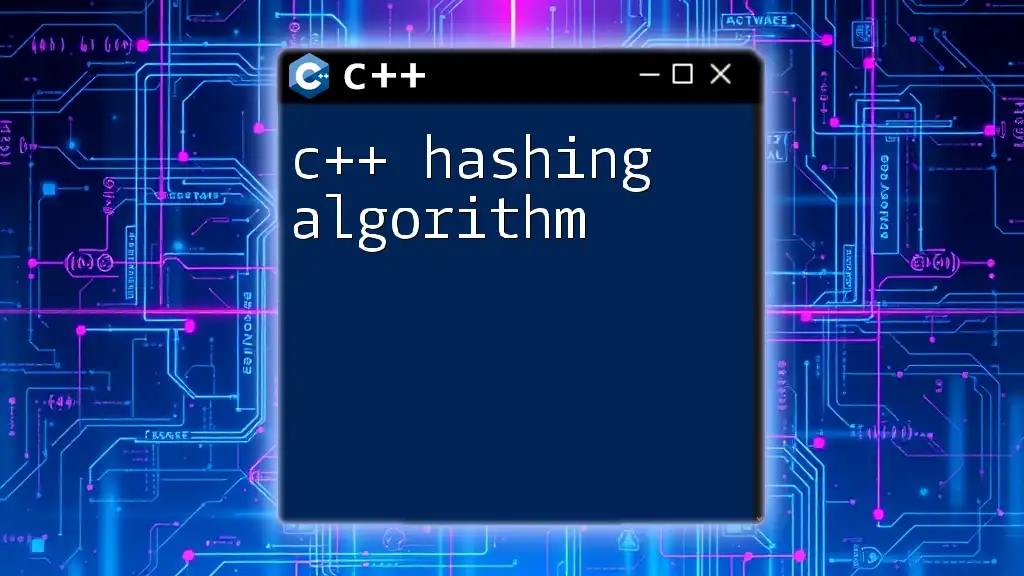
Additional Resources
For further learning, consider looking into the official googletest documentation. You can also explore recommended readings and tutorials, as well as engaging in community forums to get support and share knowledge about best practices with googletest.