A C++ hashing algorithm converts input data into a fixed-size string of characters, which is typically a hash code, to ensure quick data retrieval and integrity check.
#include <iostream>
#include <string>
#include <functional>
int main() {
std::string data = "Hello, World!";
std::hash<std::string> hash_fn;
size_t hash_value = hash_fn(data);
std::cout << "Hash value: " << hash_value << std::endl;
return 0;
}
What is Hashing?
Hashing is the process of transforming input data of any size into a fixed-size string of characters, which is typically a numerical value. This transformation is accomplished via a hash function, which outputs a hash code or hash value that uniquely represents the original data. Hashing plays a critical role in data structures like hash tables, cryptographic functions, and data integrity checks.
In programming, hashing is essential for ensuring efficient data retrieval and storage. It allows for constant-time complexity in searching and inserting elements compared to other data structures, such as arrays or linked lists. Real-world applications of hashing include password storage, data integrity verification, and quick data access in databases.
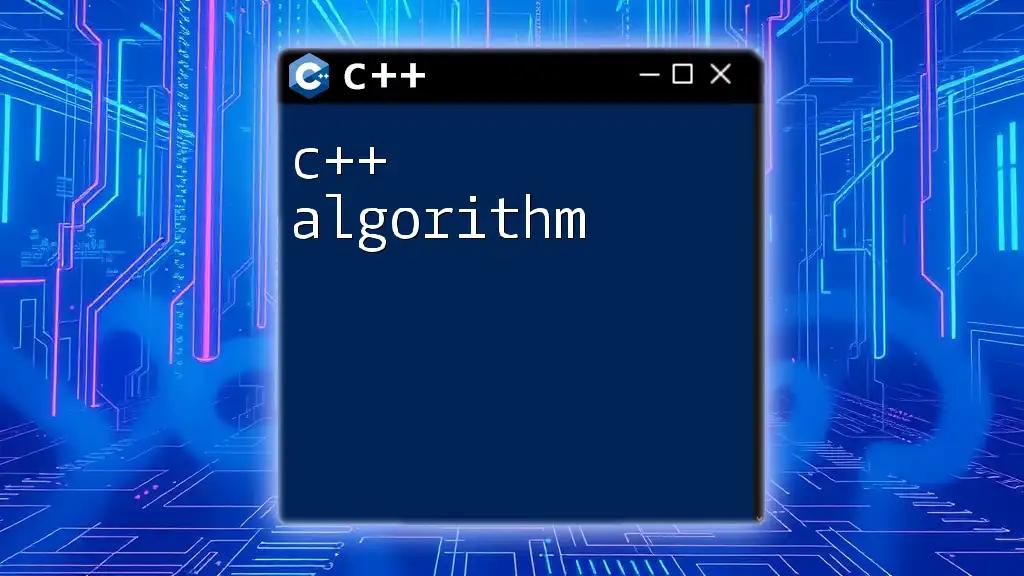
Overview of Hashing in C++
In C++, hashing provides essential functionalities that align well with the requirements of modern applications. Common use cases involve maintaining datasets, implementing cache mechanisms, and creating unique identifiers for objects.
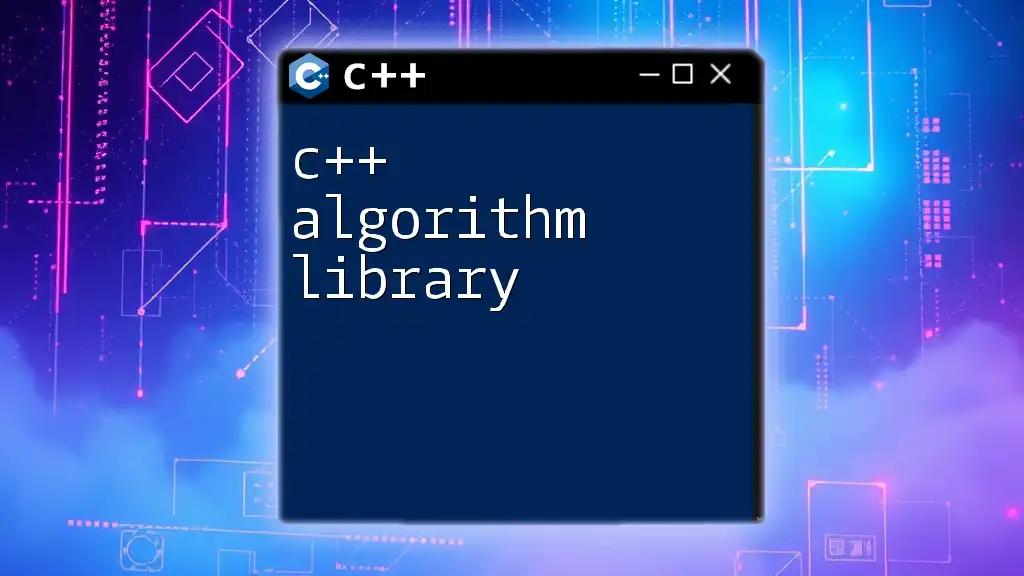
Types of Hashing Algorithms
Static vs. Dynamic Hashing
Static hashing refers to using a fixed-size hash table where the hash function does not change, regardless of the number of elements being stored. This approach can lead to issues like collisions when multiple keys hash to the same index, necessitating a collision resolution strategy.
In contrast, dynamic hashing allows the hash table to grow or shrink in size according to the number of elements. This adaptability minimizes collisions and maintains efficiency as the dataset scales.
Popular Hashing Algorithms
-
MD5: Originally crafted for checksums and file integrity checks, MD5 generates a 128-bit hash value. However, its vulnerabilities make it unsuitable for cryptographic applications.
-
SHA-1: Producing a 160-bit hash, SHA-1 was used widely in security protocols but has since been noted for its weaknesses, making it less favorable.
-
SHA-256: Part of the SHA-2 family, SHA-256 generates a 256-bit hash. Its strength over SHA-1 and MD5 makes it standard in applications requiring strong cryptography.

Implementing Hashing in C++
Basic C++ Hash Functions
Understanding hash functions is fundamental to implementing hashing mechanisms effectively. A hash function must be deterministic, meaning the same input will always produce the same output. Additionally, it should minimize collisions—where different inputs produce the same hash value.
Here’s an example of a simple hash function that maps an integer key to a range of 0-9:
int simpleHash(int key) {
return key % 10; // Example: hash function to produce 0-9
}
Standard Libraries for Hashing
The C++ Standard Template Library (STL) provides built-in hash functions that simplify the implementation of hashing in your programs. The provided functions are optimized and tested, ensuring reliable performance.
For example, you can use the `std::hash` library to generate hash values for strings:
#include <iostream>
#include <string>
#include <functional>
int main() {
std::string key = "example";
std::size_t hashValue = std::hash<std::string>{}(key);
std::cout << "Hash value of \"" << key << "\": " << hashValue << std::endl;
return 0;
}
Custom Hash Functions
In certain situations, pre-defined hash functions may not meet application needs. This is when creating custom hash functions becomes beneficial. Custom hash functions provide more control over collision management and can optimize performance for specific datasets.
However, designing a good hash function requires careful consideration to maintain a uniform distribution of hash values and minimize collisions.
Here's an example of a simple custom hash function for strings:
unsigned long customHash(const std::string &key) {
unsigned long hash = 5381;
for (char c : key) {
hash = ((hash << 5) + hash) + c; // hash * 33 + c
}
return hash;
}

Hash Table Implementation in C++
What is a Hash Table?
A hash table is a data structure that implements an associative array, allowing you to associate keys with values using a hash function. It offers efficient performance for key operations like insertion, deletion, and searching, typically operating in O(1) time complexity.
Building a Hash Table from Scratch
Creating a hash table from scratch involves defining a structure that will contain the array of linked lists or an array for storing the elements. Below is a snippet of a simple hash table implementation:
#include <iostream>
#include <vector>
#include <list>
#include <utility> // For std::pair
class HashTable {
private:
std::vector<std::list<std::pair<int, std::string>>> table;
int size;
public:
HashTable(int s) : size(s) {
table.resize(size);
}
void insert(int key, const std::string &value) {
int hashIndex = key % size;
table[hashIndex].push_back({key, value});
}
std::string search(int key) {
int hashIndex = key % size;
for (auto &pair : table[hashIndex]) {
if (pair.first == key) {
return pair.second; // Return the found value
}
}
return "Not found"; // If the key does not exist
}
void remove(int key) {
int hashIndex = key % size;
table[hashIndex].remove_if([key](const std::pair<int, std::string>& pair) {
return pair.first == key;
});
}
};
Handling Collisions
Collisions are an inevitable challenge when hashing. A collision occurs when two distinct inputs produce the same hash value.
Collision Resolution Techniques
-
Chaining: In chaining, each array index points to a list (or another structure) that holds all elements that hash to that index. This method dynamically links items, allowing for easy scalability.
-
Open Addressing: This strategy involves finding another open slot in the array to store the colliding item. Techniques like linear probing (checking the next available index sequentially) or quadratic probing (using a quadratic function to find open slots) are common approaches.
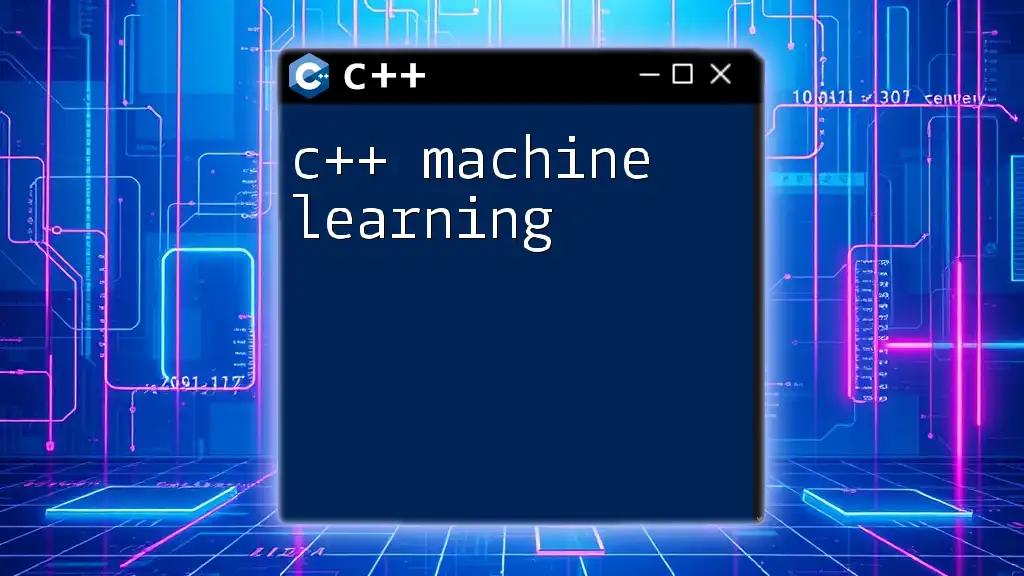
Performance Considerations
Time Complexity of Hash Functions
The performance of a hash table is largely influenced by the hash function used and the load factor (the number of elements divided by the number of buckets). An efficient hash function generally ensures that operations maintain average-case time complexities of O(1) for insertion, deletion, and lookup.
Common Pitfalls in Hashing
Designing an effective hash function requires avoiding common pitfalls. Poor hashing can lead to uneven distribution of hash codes, resulting in clustering and degraded performance. Effective hashing should distribute inputs uniformly across the available buckets.

Conclusion
In summary, C++ hashing algorithms are crucial for achieving efficient data access and manipulation. Understanding the principles of hashing, different types of hash functions, and how to implement them in hash tables are foundational skills for any C++ programmer. As you explore more complex data structures and applications, the significance of hashing will only increase in relevance.
Additional Resources
For further learning, consider delving into textbooks, online courses, and forums focused on C++ development. Websites like Stack Overflow and GitHub repositories are instrumental for software development communities and can provide practical insights into best practices in hashing.
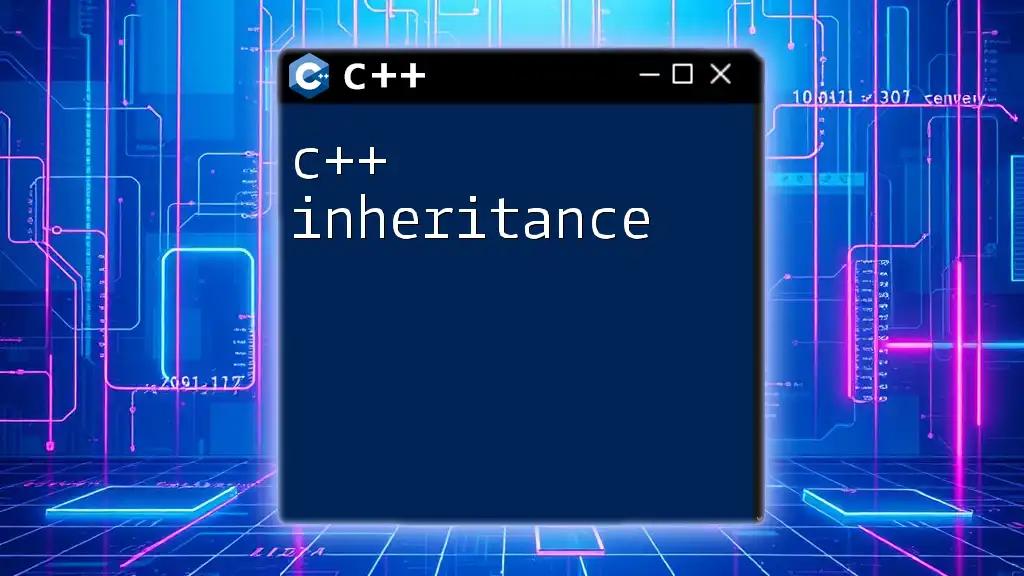
Call to Action
Dive into your projects and start implementing various hashing algorithms! Join the C++ community to continue learning about hashing and its applications or consider enrolling in courses that delve deeper into C++ programming techniques and algorithms.