Kruskal's algorithm is a popular greedy algorithm used to find the minimum spanning tree of a graph by sorting all edges and adding them one by one to the tree, ensuring no cycles are formed.
Here’s a simple C++ implementation of Kruskal's algorithm:
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
struct Edge {
int src, dest, weight;
};
class DisjointSet {
public:
vector<int> parent, rank;
DisjointSet(int n) {
parent.resize(n);
rank.resize(n, 0);
for (int i = 0; i < n; i++) parent[i] = i;
}
int find(int u) {
if (u != parent[u]) parent[u] = find(parent[u]);
return parent[u];
}
void unionSet(int u, int v) {
int pu = find(u), pv = find(v);
if (pu != pv) {
if (rank[pu] < rank[pv]) swap(pu, pv);
parent[pv] = pu;
if (rank[pu] == rank[pv]) rank[pu]++;
}
}
};
bool compareEdge(Edge a, Edge b) {
return a.weight < b.weight;
}
void kruskal(int V, vector<Edge>& edges) {
sort(edges.begin(), edges.end(), compareEdge);
DisjointSet ds(V);
vector<Edge> result;
for (auto& edge : edges) {
int u = edge.src;
int v = edge.dest;
if (ds.find(u) != ds.find(v)) {
ds.unionSet(u, v);
result.push_back(edge);
}
}
for (auto& edge : result) {
cout << edge.src << " -- " << edge.dest << " == " << edge.weight << endl;
}
}
int main() {
int V = 4;
vector<Edge> edges = { {0, 1, 10}, {0, 2, 6}, {0, 3, 5}, {1, 3, 15}, {2, 3, 4} };
kruskal(V, edges);
return 0;
}
What is Kruskal's Algorithm?
Kruskal's Algorithm is a fundamental algorithm in graph theory used to find the Minimum Spanning Tree (MST) of a connected, undirected graph. The MST is a subset of the graph's edges that connects all vertices without forming any cycles and with the minimal possible total edge weight. This algorithm is particularly useful when dealing with sparse graphs, where it can efficiently minimize the total weight.
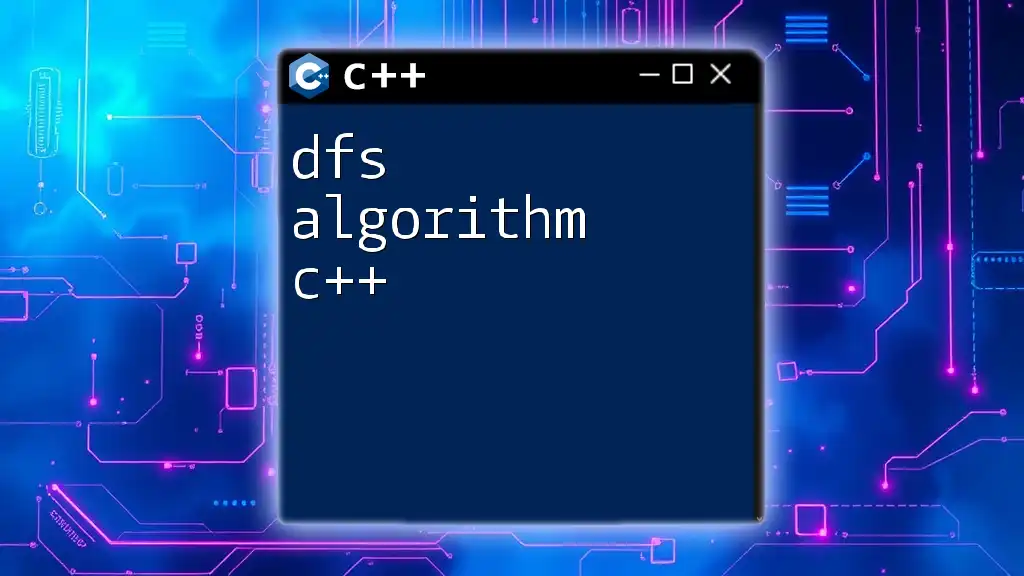
When to Use Kruskal's Algorithm?
You would typically opt for Kruskal's Algorithm in scenarios where you need to find the MST quickly with a focus on edge weights. It is an excellent choice when you have a large number of edges but relatively fewer vertices. This algorithm is more efficient than others like Prim's in cases of sparse graphs.
Comparison with Prim's Algorithm:
While both algorithms ultimately find the MST, Prim's approaches the problem by expanding from a starting vertex, whereas Kruskal’s focuses on edges. If you find it necessary to work with edge lists rather than adjacency matrices, Kruskal's is often the preferred choice.
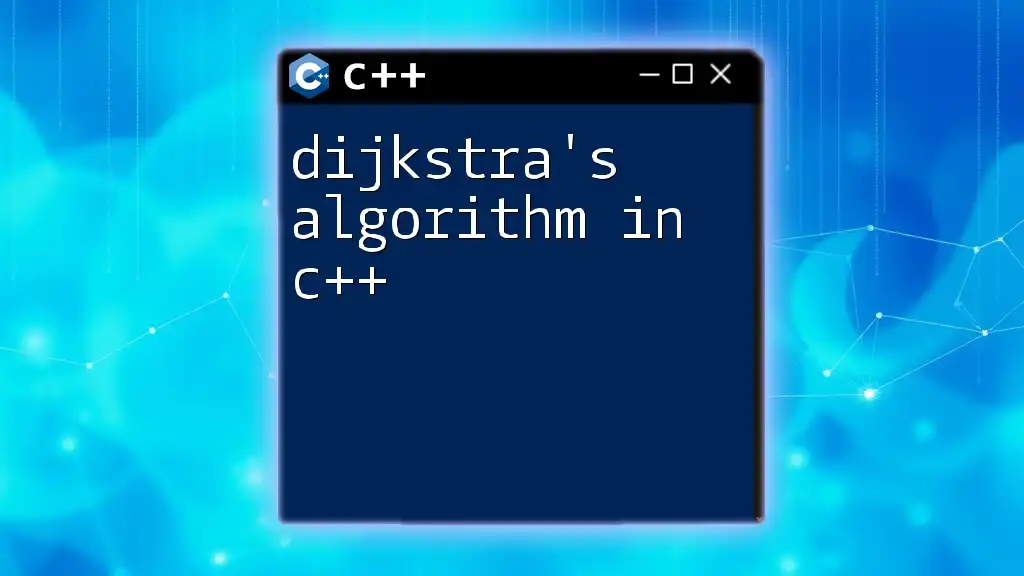
Understanding the Basics
Graphs and Trees
To appreciate Kruskal's Algorithm fully, it’s essential to understand some basic concepts:
- Graphs consist of vertices (or nodes) connected by edges. They can be directed or undirected and may contain weights assigned to each edge.
- Trees are a special type of graph that is connected and acyclic. The MST is a tree that connects all vertices using the least total weight.
Components of Kruskal's Algorithm
Edge List
In Kruskal’s approach, the graph is typically represented as an edge list, which is a collection of edges defined by their endpoints and weights. This representation simplifies the process of sorting edges by weight, which is the first step in the algorithm.
Disjoint Set Data Structure
A critical component of Kruskal’s Algorithm is the Disjoint Set (or Union-Find) data structure. This structure keeps track of which vertices are connected and helps ensure that no cycles form during edge selection. The efficiency of the disjoint set is significantly enhanced by techniques like path compression and union by rank.
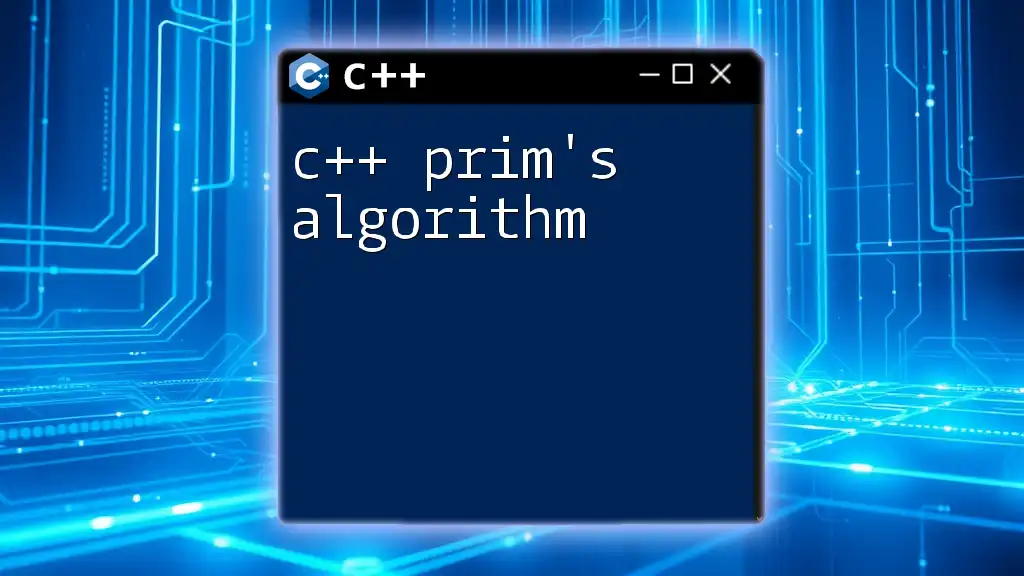
Step-by-Step Breakdown of Kruskal's Algorithm
Initial Setup
Before applying Kruskal's Algorithm, you will need to represent the graph using an edge list. Each edge should include its two endpoints and its associated weight. The next vital step involves sorting all edges based on their weights in ascending order.
The Algorithm Process
1. Sort All Edges
Sorting the edges is essential because it allows the algorithm to consider the least expensive edges first. The edges will be compared, and the one with the lowest weight will be selected and added to the MST.
Here’s a code snippet illustrating how to sort edges in C++:
std::sort(edges.begin(), edges.end(), [](Edge a, Edge b) {
return a.weight < b.weight;
});
2. Iterating Through Edges
After sorting, the algorithm iterates through the edges and evaluates whether adding each edge to the MST would form a cycle. This is determined using the disjoint set structure.
3. Forming the Minimum Spanning Tree
For an edge to be included in the MST, it must connect two vertices that are not already connected. In this way, the algorithm utilizes the disjoint set to check connectivity and implements union operations to link sets without forming cycles.
Example Walkthrough
To provide a better understanding, consider the following sample graph represented by an edge list of weighted edges:
- (A, B, 1)
- (B, C, 3)
- (A, C, 2)
- (C, D, 4)
Implementing the Algorithm
Here is how you would implement Kruskal’s Algorithm in C++:
#include <iostream>
#include <vector>
#include <algorithm>
struct Edge {
char u, v;
int weight;
};
class DisjointSet {
public:
DisjointSet(int n) {
// Initialization of parent and rank arrays
}
int find(char u) {
// Path compression implementation
}
void unionSets(char u, char v) {
// Union by rank implementation
}
};
// Kruskal's Algorithm implementation
void kruskal(std::vector<Edge> &edges, int numberOfVertices) {
std::sort(edges.begin(), edges.end(), [](Edge a, Edge b) {
return a.weight < b.weight;
});
DisjointSet ds(numberOfVertices);
std::vector<Edge> mst;
for (Edge &edge : edges) {
char u = edge.u;
char v = edge.v;
if (ds.find(u) != ds.find(v)) {
mst.push_back(edge);
ds.unionSets(u, v);
}
}
// Print the result
for (const Edge &edge : mst) {
std::cout << edge.u << " -- " << edge.v << " == " << edge.weight << "\n";
}
}
Result Explanation
Once you execute the algorithm, the result will include the edges of the Minimum Spanning Tree, accompanied by the respective weights. For example, the result might yield:
A -- B == 1
A -- C == 2
C -- D == 4
This output means that these edges form the MST, connecting all vertices at the lowest possible cost. Kruskal’s efficiency lies in its linear processing time concerning the number of edges.
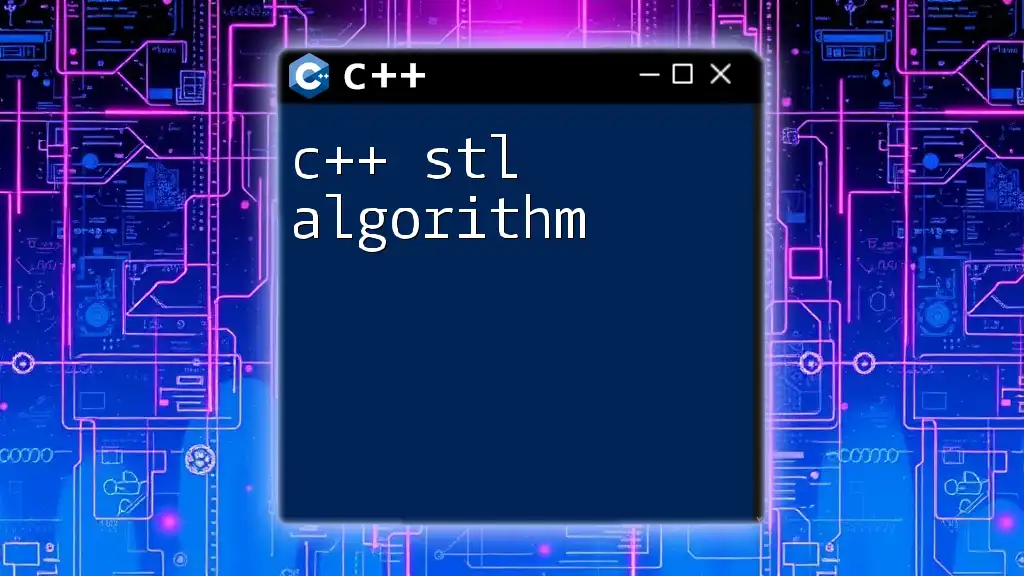
Edge Cases and Considerations
Handling Duplicates
In certain cases, the edge list may contain duplicate edges. To handle such scenarios, ensure that you only incorporate unique edges into your algorithm, which can be achieved through data structures that inherently avoid redundancy.
Performance Analysis
The time complexity of Kruskal's Algorithm is O(E log E), primarily driven by the edge sorting step, where E represents the number of edges. Its space complexity is O(V) due to the storage of the disjoint set.
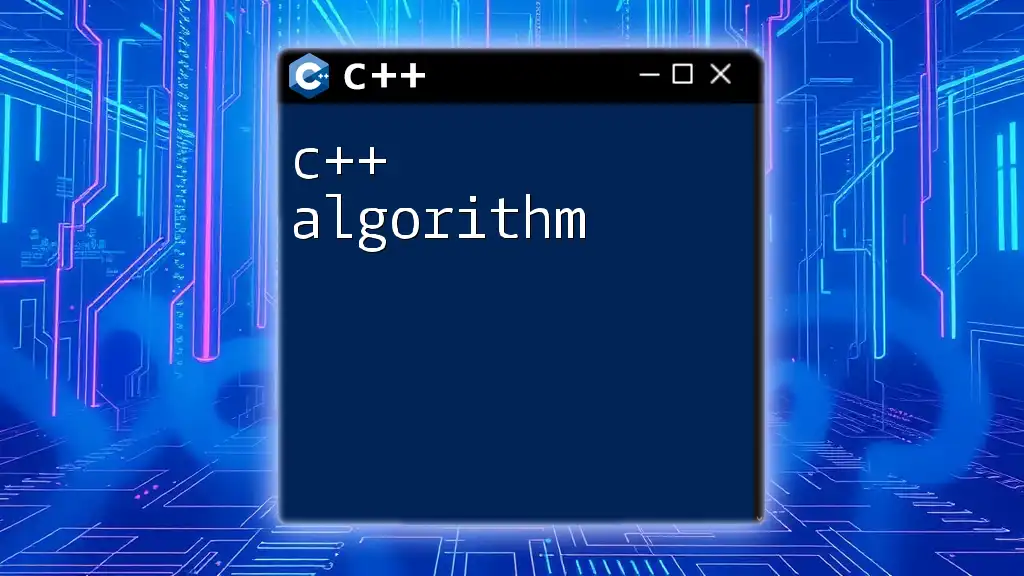
Practical Applications of Kruskal's Algorithm
Networking
Kruskal's Algorithm finds widespread use in designing networks where minimal connection costs must be confirmed. It’s often applied in telecommunications and computer networking to optimize routes between nodes while minimizing costs.
Geographic Information Systems (GIS)
In GIS, Kruskal’s Algorithm is applied in mapping and analyzing spatial relationships among points. It helps in minimizing transport paths and resource allocation, making it an invaluable algorithm in various geographic applications.
Other Practical Scenarios
Kruskal's Algorithm also has utility in clustering in data science, constructing decision trees, and other fields that require optimization of pathways, networks, or connections.
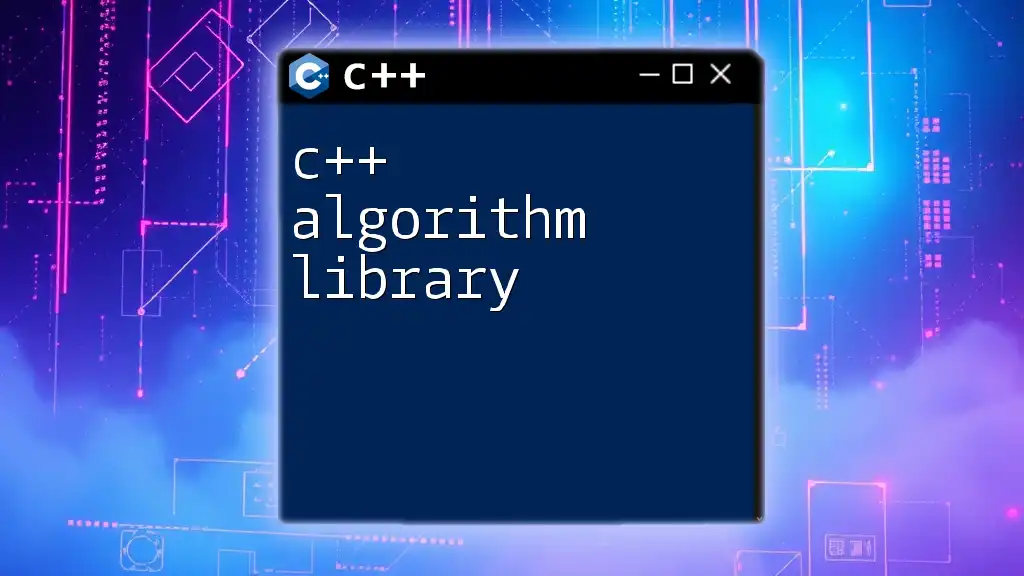
Conclusion
In summary, Kruskal's Algorithm serves a critical function in graph theory by providing an effective means of identifying the Minimum Spanning Tree of a graph. Through its efficient use of sorting, iteration, and union-find techniques, it offers a robust approach to solving spanning tree problems in various real-world applications.
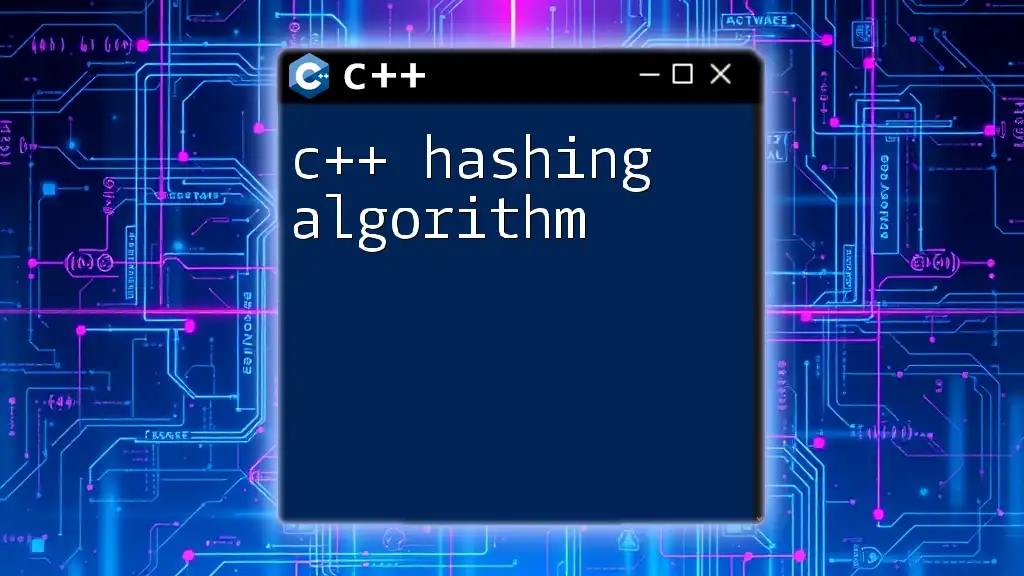
Additional Resources
For those interested in deepening their understanding of Kruskal’s Algorithm and its related concepts, consider exploring academic papers on graph theory, engaging in dedicated online courses, and participating in communities poised to expand knowledge in algorithmic strategies.
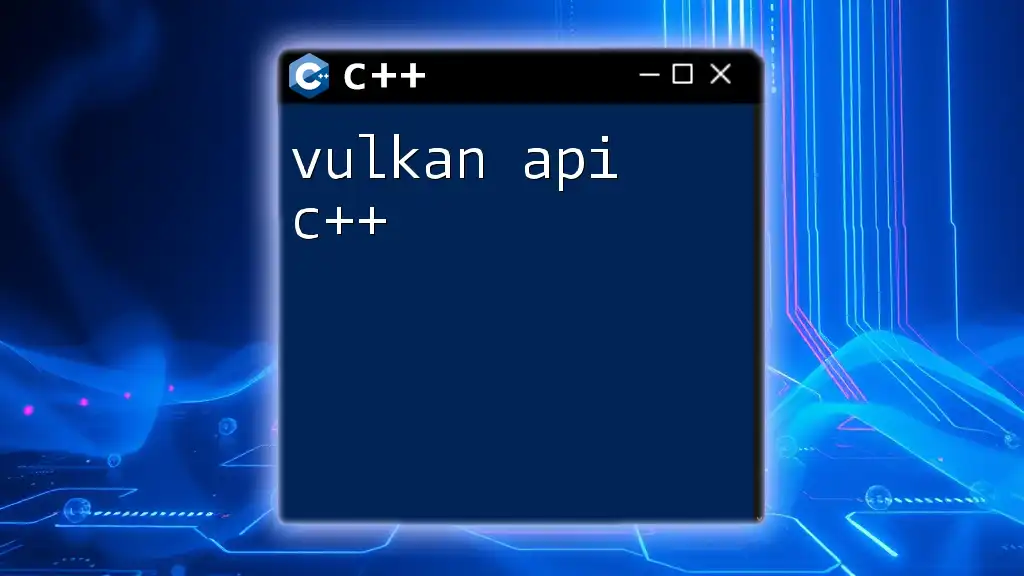
Call to Action
As you dive into implementing Kruskal's Algorithm in your projects, don't hesitate to share your experiences and challenges. For more quick and concise C++ command tutorials, feel free to explore the resources provided by our company to support your programming journey.