The Akuna Capital C++ coding challenge is designed to assess candidates' proficiency in C++ through practical coding exercises that emphasize efficiency and problem-solving skills.
Here's a sample C++ code snippet that demonstrates a simple function to calculate the factorial of a number:
#include <iostream>
int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
int main() {
int number = 5;
std::cout << "Factorial of " << number << " is " << factorial(number) << std::endl;
return 0;
}
Understanding the Coding Challenge
What is the Akuna Capital C++ Coding Challenge?
The Akuna Capital C++ coding challenge is designed to assess a candidate's proficiency in C++ programming, critical thinking, and problem-solving abilities. This challenge plays a crucial role in the recruitment process for candidates aspiring to work at Akuna Capital, a firm renowned for its cutting-edge technology and quantitative trading practices. It is typically administered online, enabling candidates to showcase their expertise in a timed environment that simulates real-world coding situations.
Key Objectives of the Challenge
The primary focus of the challenge lies in evaluating the following:
- Problem-solving skills: Candidates must tackle complex algorithms and data structure problems that require logical reasoning and analytical thinking.
- Knowledge of C++ syntax and concepts: A deep understanding of C++ is essential, including mastery of features such as pointers, references, and memory management.
- Algorithm design and efficiency: Candidates should demonstrate the ability to devise algorithms that are not only correct but also optimized for performance, reflecting real-world applications.
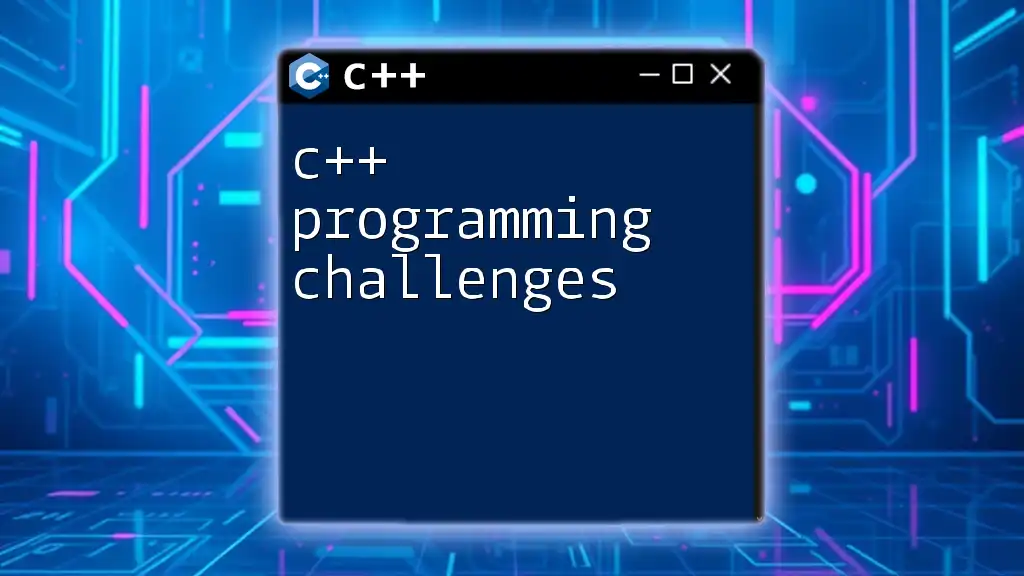
Preparation Steps
Essential C++ Topics to Master
To excel in the Akuna Capital C++ coding challenge, it is crucial to solidify your understanding of various fundamental C++ topics:
-
Data Structures: Be well-versed in essential data structures like arrays, linked lists, stacks, queues, trees (binary trees, AVL trees, etc.), and graphs. Understand their complexities in terms of time and space.
-
Object-Oriented Programming: Master core principles such as classes, inheritance, polymorphism, and encapsulation. Be prepared to apply these concepts in practical scenarios.
-
Templates and Standard Template Library (STL): Learn how to use STL effectively for efficient coding. Familiarity with template classes and functions will enhance your coding speed and capability.
Coding Best Practices
To produce high-quality code during the coding challenge, consider the following best practices:
-
Write clean and readable code: Prioritize clarity by using meaningful variable names and consistent formatting. This ensures that anyone reviewing your code can easily understand its intent.
-
Use effective debugging techniques and tools: Familiarize yourself with common debugging tools like `gdb` and memory checking tools like Valgrind to identify and resolve issues efficiently.
-
Emphasize commenting and documentation: While your code should be self-explanatory, well-placed comments help others (and your future self) to grasp the thought process behind your solutions easily.
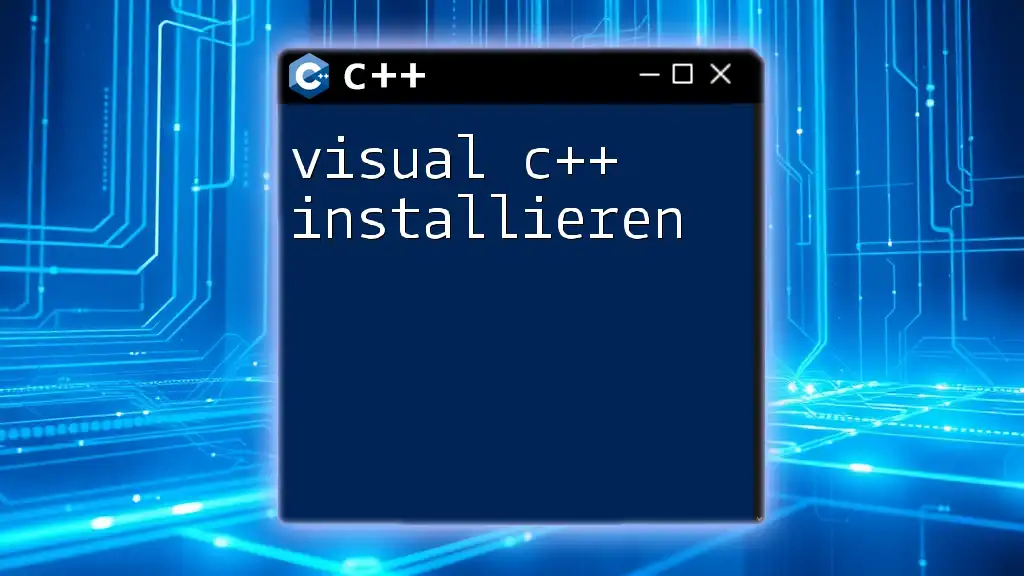
Example Problems from Akuna Capital C++ Coding Challenge
Problem Type: Algorithmic Challenges
Example Problem 1: Implementing Dijkstra’s Algorithm for Shortest Path
- Problem Statement: Given a graph represented by an adjacency list, find the shortest path from a source node to all other nodes. This problem tests your understanding of graph algorithms and your implementation skills in C++.
Here’s a code snippet to illustrate the solution using Dijkstra's Algorithm:
#include <iostream>
#include <vector>
#include <queue>
#include <limits>
using namespace std;
void dijkstra(int src, vector<vector<pair<int, int>>>& graph) {
vector<int> dist(graph.size(), numeric_limits<int>::max());
dist[src] = 0;
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq;
pq.push({0, src});
while (!pq.empty()) {
int node = pq.top().second;
pq.pop();
for (auto& edge : graph[node]) {
int neighbor = edge.first;
int weight = edge.second;
if (dist[node] + weight < dist[neighbor]) {
dist[neighbor] = dist[node] + weight;
pq.push({dist[neighbor], neighbor});
}
}
}
}
Explanation: This implementation initializes the distance to the source node as zero and all others as infinity. It uses a priority queue to repeatedly add the node with the smallest distance to the queue, updating the shortest distance to each neighbor whenever a shorter path is found.
Problem Type: Data Structure Implementation
Example Problem 2: Implementing a Custom Binary Search Tree (BST)
- Problem Statement: Create a binary search tree with insert and search functionalities. The challenge requires you to implement robust data structures and algorithms in C++.
Here’s a code snippet showing a basic illustration of a BST:
struct Node {
int value;
Node* left;
Node* right;
Node(int val) : value(val), left(nullptr), right(nullptr) {}
};
class BST {
public:
Node* root;
BST() : root(nullptr) {}
void insert(int value) {
root = insertRec(root, value);
}
Node* insertRec(Node* node, int value) {
if (node == nullptr) return new Node(value);
if (value < node->value)
node->left = insertRec(node->left, value);
else
node->right = insertRec(node->right, value);
return node;
}
bool search(int value) {
return searchRec(root, value);
}
bool searchRec(Node* node, int value) {
if (node == nullptr) return false;
if (node->value == value) return true;
return value < node->value ? searchRec(node->left, value) : searchRec(node->right, value);
}
};
Explanation: In this code, the `Node` structure represents each element in the BST, allowing insertion and searching through recursive helper methods. Candidates are expected to implement balanced search trees efficiently.
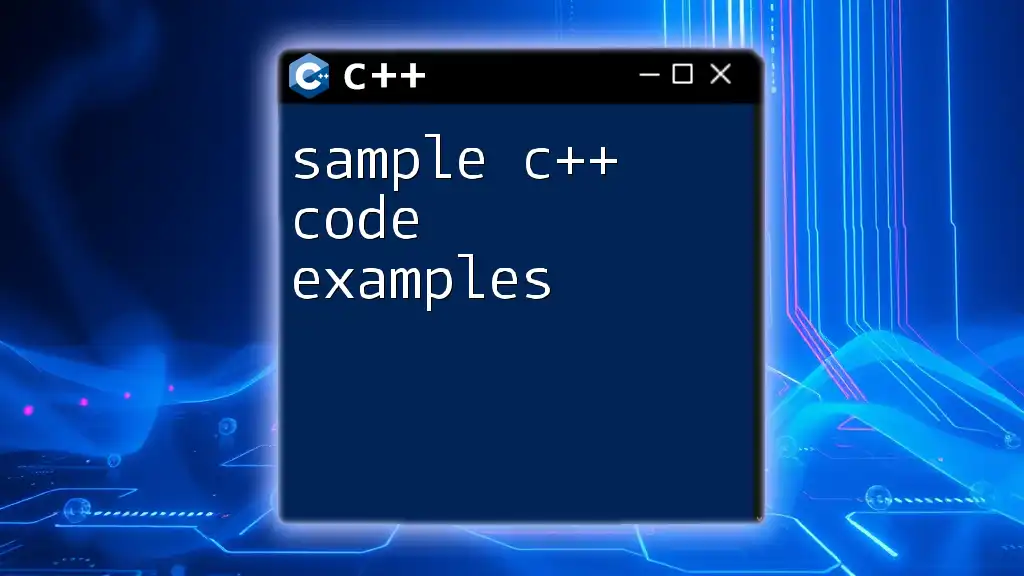
Resources for Further Study
Recommended C++ Books and Online Courses
To enhance your C++ skills, consider reading influential books such as “Effective C++” by Scott Meyers, which offers practical advice for writing high-quality C++ code. Online courses offered by platforms like Coursera and Udacity provide structured learning pathways from beginner to advanced levels, especially focused on C++ applications in finance and algorithms.
Coding Practice Platforms
Engage with platforms like LeetCode, HackerRank, and CodeSignal. These resources offer a multitude of coding challenges similar to the Akuna Capital C++ coding challenge, providing ample opportunities to practice and improve your coding skills in a timed environment.
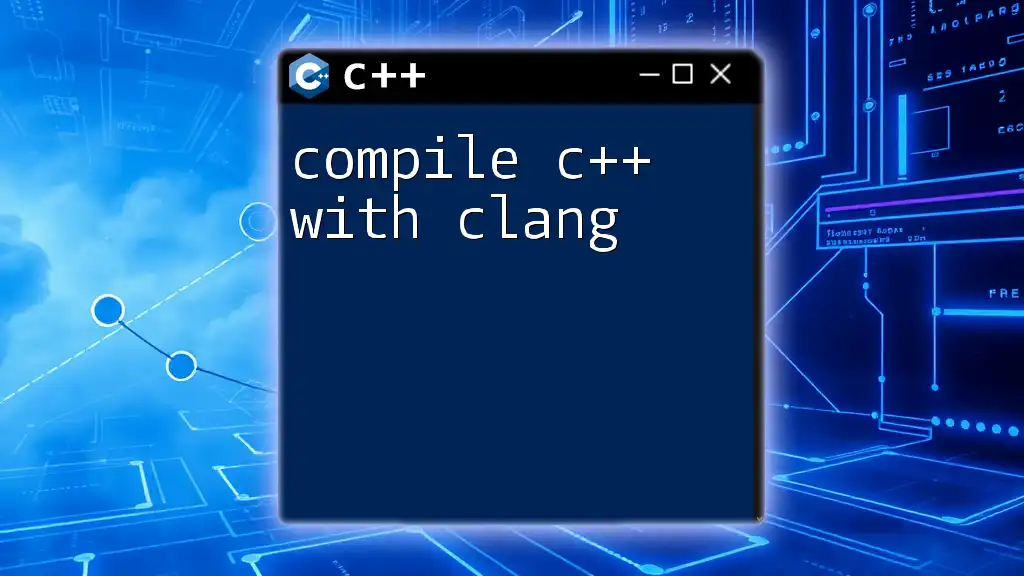
Conclusion
Preparing for the Akuna Capital C++ coding challenge involves a combination of mastering essential C++ concepts, practicing code implementation, and familiarizing yourself with best coding practices. The key to success is to apply your knowledge in solving a variety of problems and consistently engaging with the coding community for support and resources.
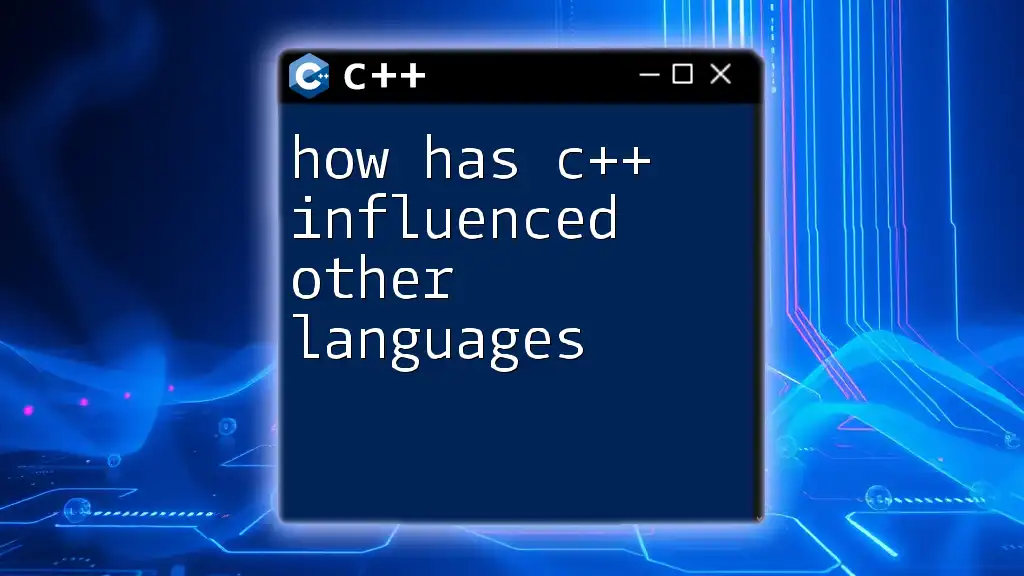
Frequently Asked Questions (FAQs)
What is the average duration of the Akuna Capital C++ coding challenge?
Typically, the challenge lasts between one to two hours, allowing candidates enough time to tackle multiple problems.
What resources are allowed during the coding challenge?
Candidates are generally permitted to use online documentation and references, but not other external help like forums or collaboration with others.
How can I find similar coding challenges to practice for this challenge?
The aforementioned coding practice platforms offer a wide range of problems akin to the type you'll encounter in the Akuna Capital C++ coding challenge.
What should I do if I get stuck during a problem?
If you find yourself struggling, try to break down the problem into smaller parts or look at similar problems you’ve solved. If time permits, don't hesitate to move on and return later with a fresh perspective.
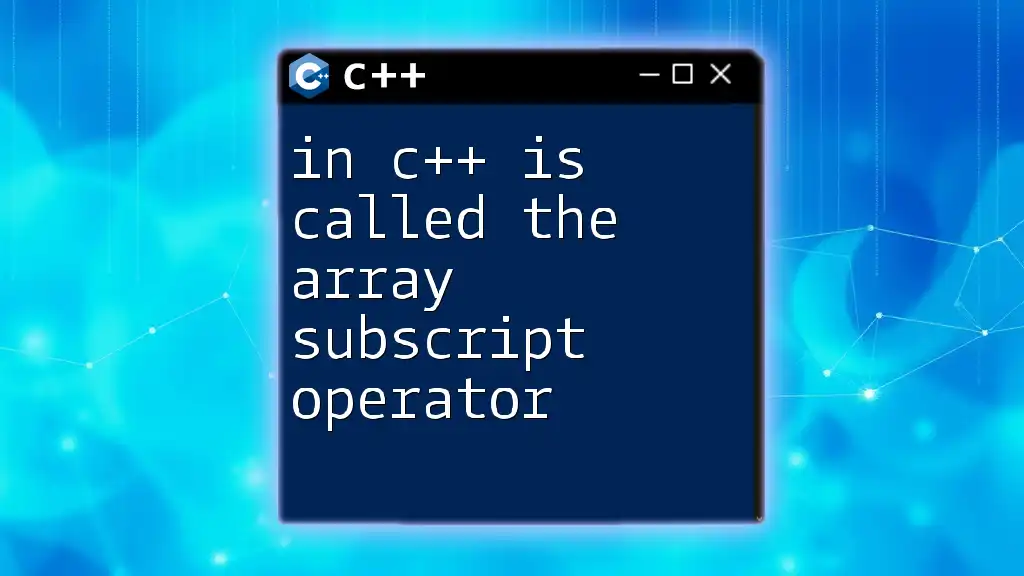
Final Thoughts
The landscape of coding challenges is ever-evolving, making it essential for candidates to keep learning and adapting. Embrace the challenges presented and use every opportunity to refine your C++ skills, especially as they pertain to applications in finance and quantitative analysis.