In C++, the array subscript operator is denoted by square brackets `[]`, which allows access to elements in an array using their index.
int arr[] = {10, 20, 30, 40, 50};
int value = arr[2]; // value will be 30
Understanding Arrays in C++
What is an Array?
An array is a collection of items stored at contiguous memory locations. It is essential for storing multiple data items of the same type under a single variable name. Arrays are particularly useful when you want to manage large datasets without creating multiple variables manually.
Declaring and Initializing Arrays
To work with arrays, you must first declare them. The syntax for declaring an array typically includes specifying the data type, followed by the array name and its size. For example:
int numbers[5];
This line declares an array named `numbers` that can hold five integers.
Initialization can be done at the time of declaration or later. Here are two ways to initialize an array:
-
Static Initialization
int numbers[] = {1, 2, 3, 4, 5};
This line initializes the `numbers` array with five specific values.
-
Dynamic Initialization You can also assign values to an array after it has been declared:
int numbers[5]; for(int i = 0; i < 5; i++) { numbers[i] = i + 1; // Assigning values 1, 2, 3, 4, 5 }
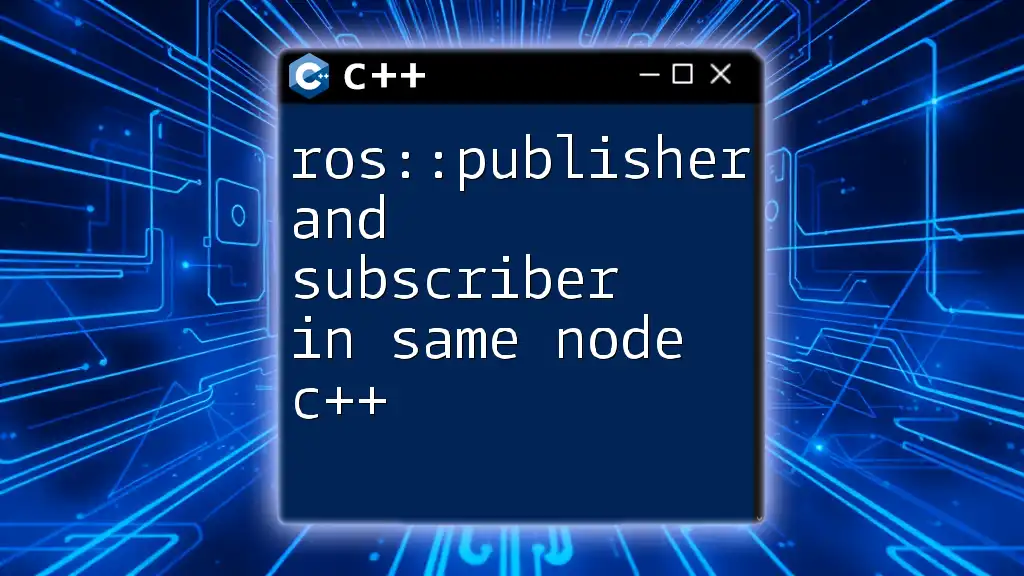
The Array Subscript Operator: An Overview
Definition and Syntax
The array subscript operator, represented by the `[]` symbol, allows access to individual elements of an array based on their index values. The general syntax is:
array_name[index]
For each access operation, you need to specify the index.
How Indexing Works
Zero-based Indexing
C++ uses zero-based indexing, which means that the first index in the array is `0`, the second is `1`, and so on. This can sometimes be confusing for beginners, but it’s a vital concept to grasp.
For example, if you have an array defined as follows:
int arr[5] = {10, 20, 30, 40, 50};
To access the first element, you would use `arr[0]`, which would output:
cout << arr[0]; // Output: 10
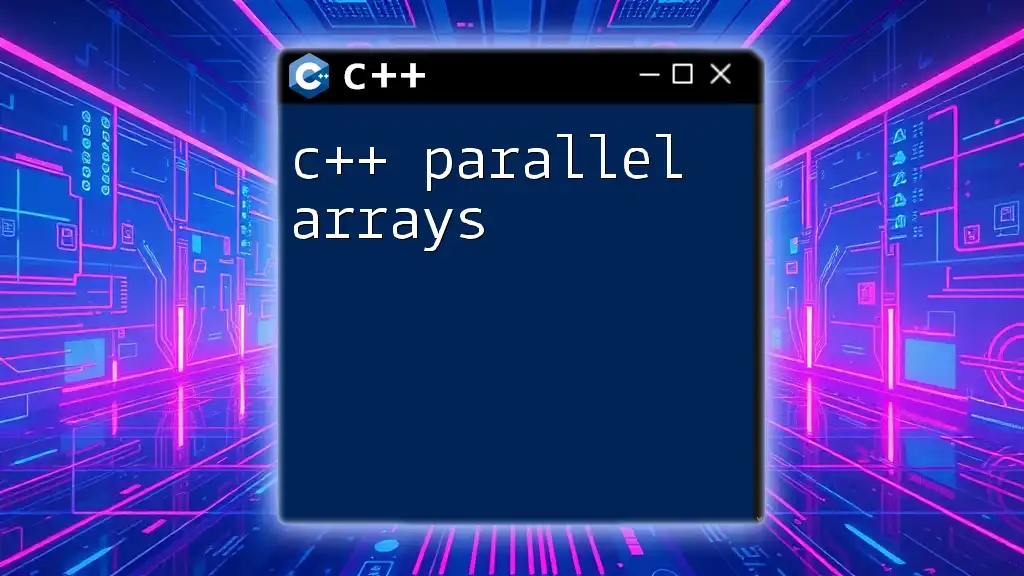
Accessing and Modifying Array Elements
Accessing Elements
To access individual elements within an array, utilize the array subscript operator. Continuing with our previous example, you can access other elements like this:
cout << arr[3]; // Output: 40
This shows that `arr[3]` refers to the fourth element in the array.
Modifying Elements
You can change the value of an array element using the subscript operator:
arr[2] = 100; // Now arr[2] is 100
This will modify the third element of `arr`, changing its original value of `30` to `100`.
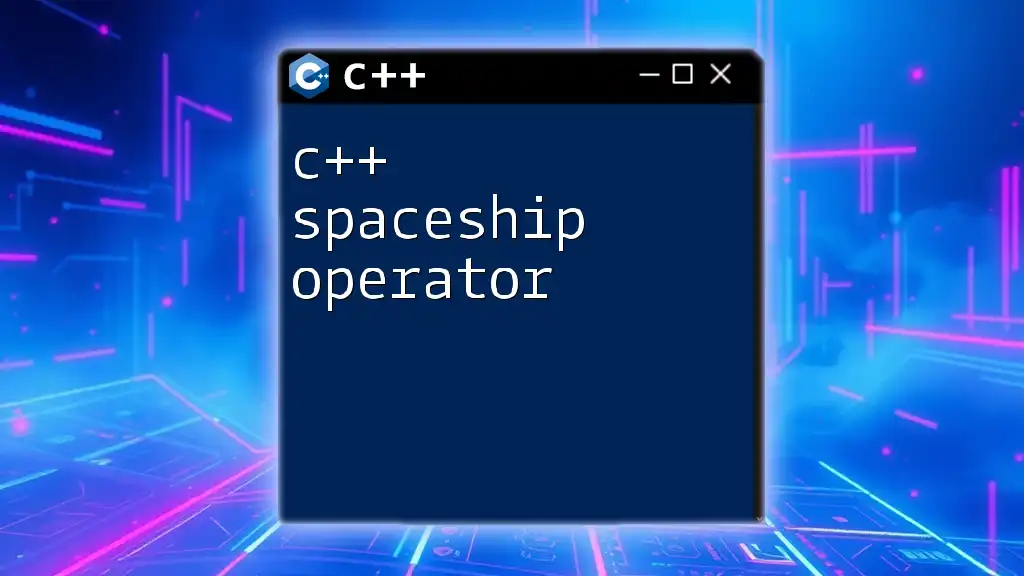
Array Subscript Operator in Different Scenarios
Single-Dimensional Arrays
The array subscript operator is incredibly handy. For instance, you might want to display all the values in an array:
for (int i = 0; i < 5; i++) {
cout << arr[i] << " "; // Output: 10 20 100 40 50
}
This loop iterates over each element in `arr` and prints it.
Multi-Dimensional Arrays
Understanding Multi-Dimensional Indexing
Multi-dimensional arrays can be visualized as arrays of arrays. This allows for more complex data storage, such as matrices. Here's how to declare a two-dimensional array:
int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}};
Accessing Multi-Dimensional Elements
Accessing elements in a multi-dimensional array also utilizes the subscript operator, but it requires multiple indices:
cout << matrix[1][2]; // Output: 6
This line accesses the element in the second row, third column of the `matrix` array.
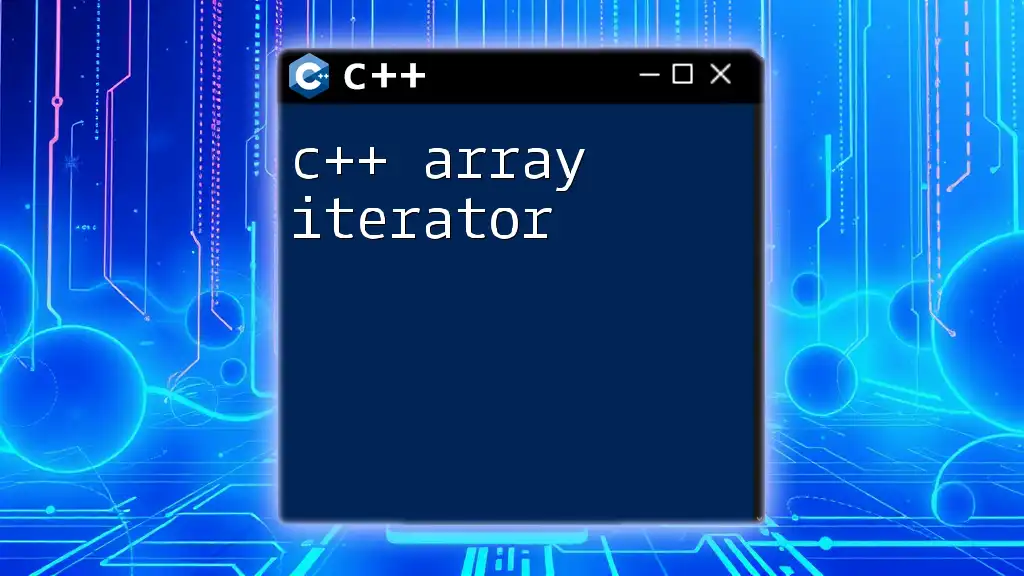
Advanced Uses of the Array Subscript Operator
Pointer Arithmetic and Arrays
One of the interesting aspects of arrays in C++ is their relationship with pointers. The name of an array acts as a pointer to its first element. You can leverage this with the subscript operator as follows:
int* ptr = arr;
cout << ptr[1]; // Output: 20
Here, `ptr[1]` accesses the second element of the original array `arr`.
Common Pitfalls to Avoid
While using the array subscript operator, it's crucial to be aware of out-of-bounds errors. Accessing an index outside the defined range can lead to undefined behavior and potential program crashes. Always ensure your index is within the limits you set when declaring your array.
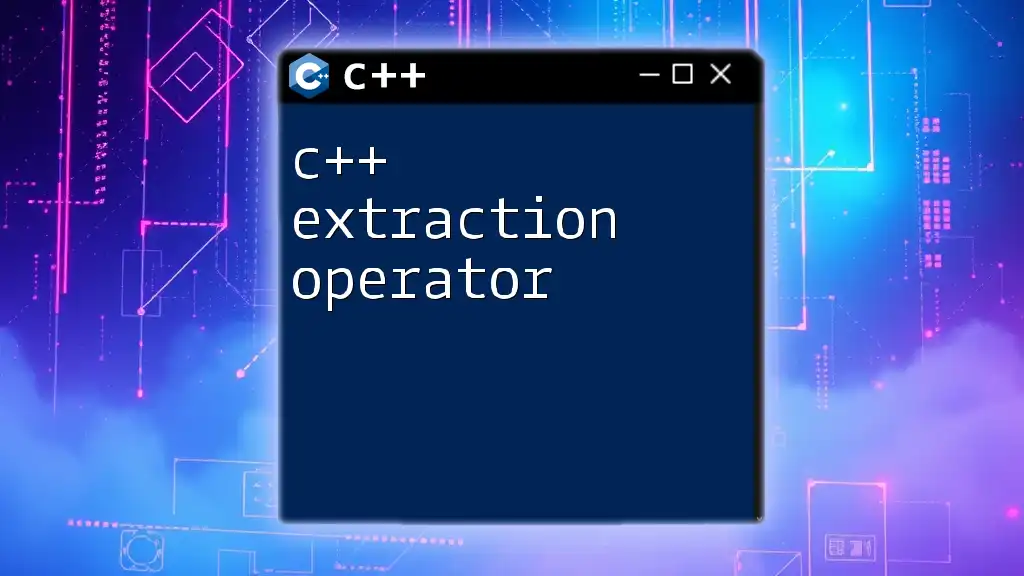
Conclusion
In C++, the array subscript operator is an essential tool for accessing and manipulating array elements conveniently. Understanding its significance along with the proper use of indices allows for powerful data management skills. By practicing these techniques, you will gain confidence in programming with arrays, facilitating more complex and efficient code. Remember to apply safe coding practices when working with arrays to avoid common pitfalls and optimize your programs.