In C++, you can initialize an array to zero using either an initializer list or the `memset` function, as shown in the following example:
int arr[5] = {0}; // Initializes all elements to 0
// or
int arr[5];
memset(arr, 0, sizeof(arr)); // Sets all elements to 0
Understanding Arrays in C++
What is an Array?
An array in C++ is a collection of elements identified by index or key. Arrays allow you to store multiple values of the same type under a single variable name, enabling efficient data management and manipulation. The elements in an array are stored in contiguous memory locations, which allows C++ to access them quickly through indexing.
Types of Arrays
-
Single-dimensional arrays: These are the simplest form of arrays where elements are arranged in a linear fashion. For example, `int arr[5]` represents an array of five integers.
-
Multi-dimensional arrays: These arrays consist of more than one dimension, typically used to represent matrices or grids. For instance, `int arr[3][4]` creates a 3x4 matrix of integers.
Understanding the different types of arrays is crucial in determining how to initialize them properly.
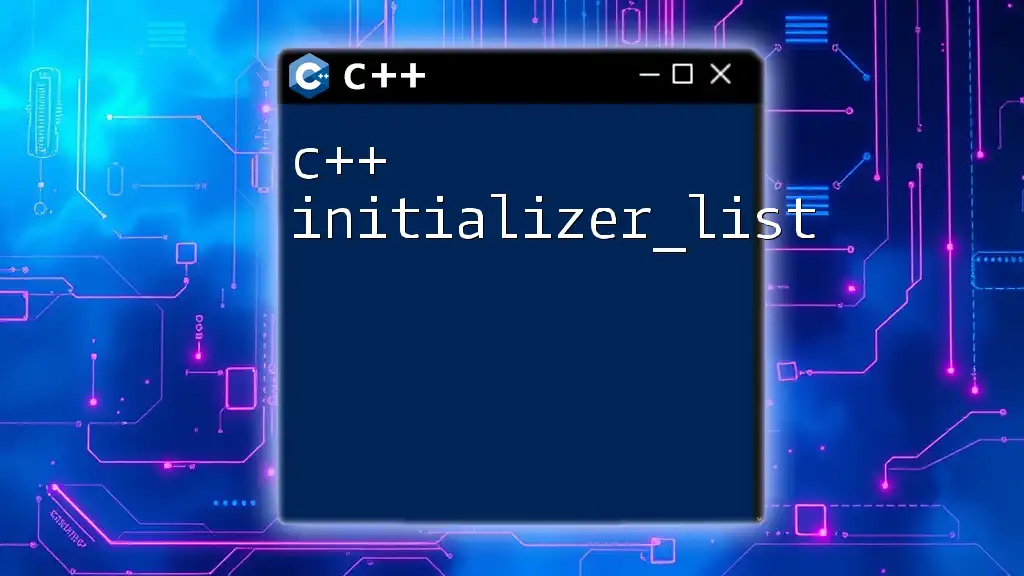
Why Initialize Arrays?
Importance of Initialization
Initialization is a critical step in programming, especially in C++, where failing to initialize can lead to undefined behavior. When arrays are declared but not initialized, they may contain garbage values left over in memory. This can lead to unpredictable behavior in your programs, making it essential to set array elements to known values—like zero—before use.
Consequences of Uninitialized Arrays
Using uninitialized arrays can introduce subtle, hard-to-track bugs. For example, consider a scenario where an array is expected to hold values representing scores but remains uninitialized. If any calculations are performed based on these garbage values, the outcome could be catastrophic. This underscores the necessity of properly initializing all variables, especially arrays.
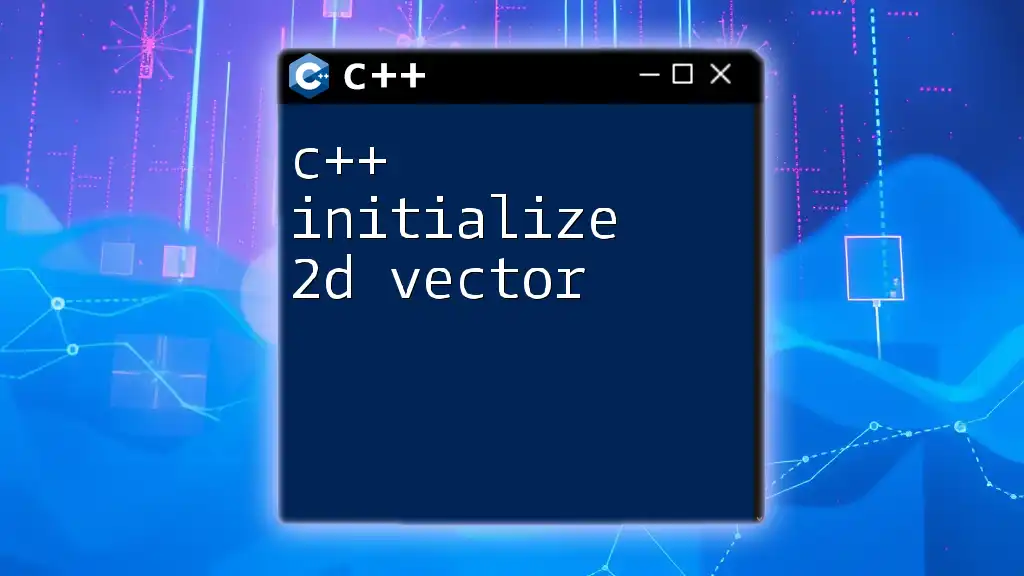
Methods to Initialize an Array to 0
Using Loop Constructs
One common way to initialize an array to 0 is by using a `for` loop. This method is straightforward and widely understood.
Example of Initialization with `for` Loop
int arr[5];
for(int i = 0; i < 5; i++) {
arr[i] = 0;
}
In this snippet, a loop iteratively assigns the value 0 to each element in the array. While this method is effective, it tends to be verbose and may introduce errors if not handled correctly.
Pros: Clear and explicit.
Cons: Verbose and prone to mistakes, especially with larger arrays.
Using `std::fill` from the `<algorithm>` Library
For those familiar with the Standard Library, `std::fill` offers a clean and efficient way to initialize arrays.
Example with `std::fill`
#include <algorithm>
int arr[5];
std::fill(arr, arr + 5, 0);
Here, `std::fill` takes three parameters: the beginning of the array, the end of the array, and the value to fill. This method is elegant and improves readability while maintaining efficiency.
Pros: More concise and less error-prone than loops.
Cons: Requires including the `<algorithm>` header.
Using C++11's Braced Initialization
C++11 introduced a new way to initialize arrays using the braced initializer syntax.
Example of Braced Initializer
int arr[5] = {};
In this case, the array is automatically initialized to 0. This method is highly efficient, concise, and eliminates the risk of introducing errors.
Pros: Very clean and efficient; automatically zeroes elements.
Cons: May be less familiar to those not used to C++11 features.
Using `std::array` (C++11 and Beyond)
Instead of traditional arrays, C++11 provides `std::array`, which offers enhanced functionality and safety.
Example of `std::array`
#include <array>
std::array<int, 5> arr = {};
In this approach, `std::array` behaves like a fixed-size array but comes with additional features like bounds checking, which can prevent out-of-bounds access. Furthermore, using `std::array` is a safer practice in modern C++.
Pros: Provides safety and additional functionality.
Cons: Requires the inclusion of the `<array>` header and is slightly less performant than raw arrays in specific contexts.
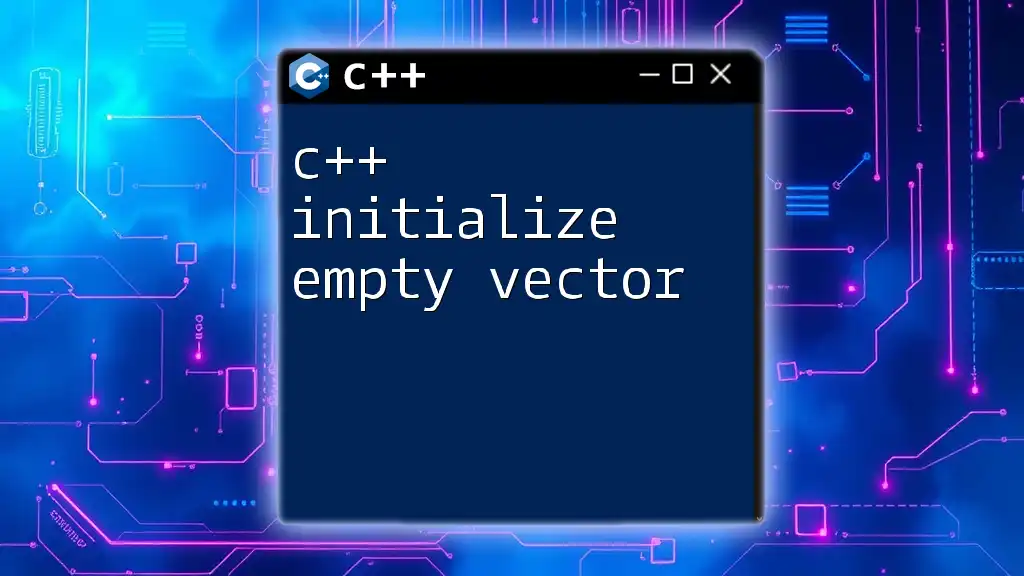
Comparing Methods of Initialization
Efficiency Analysis
While all methods effectively initialize an array to 0, performance can vary depending on context. For small arrays, the difference is negligible. However, as array size increases, `std::fill` and braced initialization typically offer better readability and potential performance benefits due to optimizations in library implementations.
Code Readability and Maintenance
Readability is a crucial factor when selecting an initialization method. Opting for `std::fill` or braced initialization can make your code cleaner and easier to understand. Aim to pick a method that not only works efficiently but also minimizes the cognitive load for other programmers reading your code.
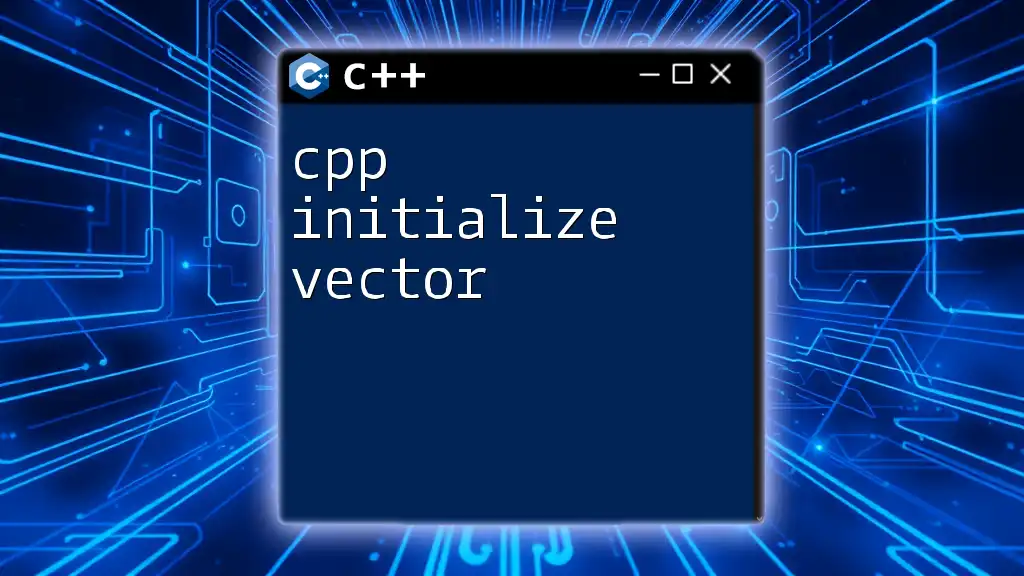
Common Mistakes to Avoid
-
Attempting to initialize dynamically allocated arrays: Remember that traditional initialization methods won't work directly on dynamically allocated arrays. Always use `new` and manually set values in those cases.
-
Forgetting to include necessary headers: Missed headers like `<algorithm>` or `<array>` can lead to compilation errors. Always check your includes when using standard library features.
-
Misunderstanding array sizes: Ensure that you always account for the correct size when initializing arrays to avoid overflow or unintentional garbage values.
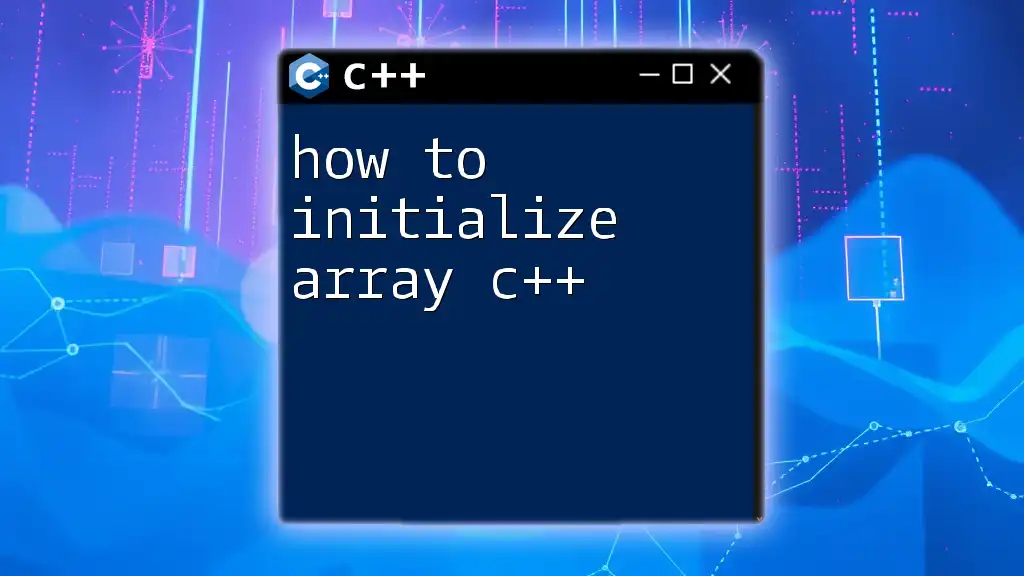
Conclusion
Effectively initializing an array to 0 using the various methods discussed is a fundamental programming skill in C++. From simple loops to modern techniques like `std::array`, each approach offers unique benefits. Choose the initialization style that suits your coding standards and project requirements, and always remember the importance of eliminating uninitialized values from your code to produce reliable software.
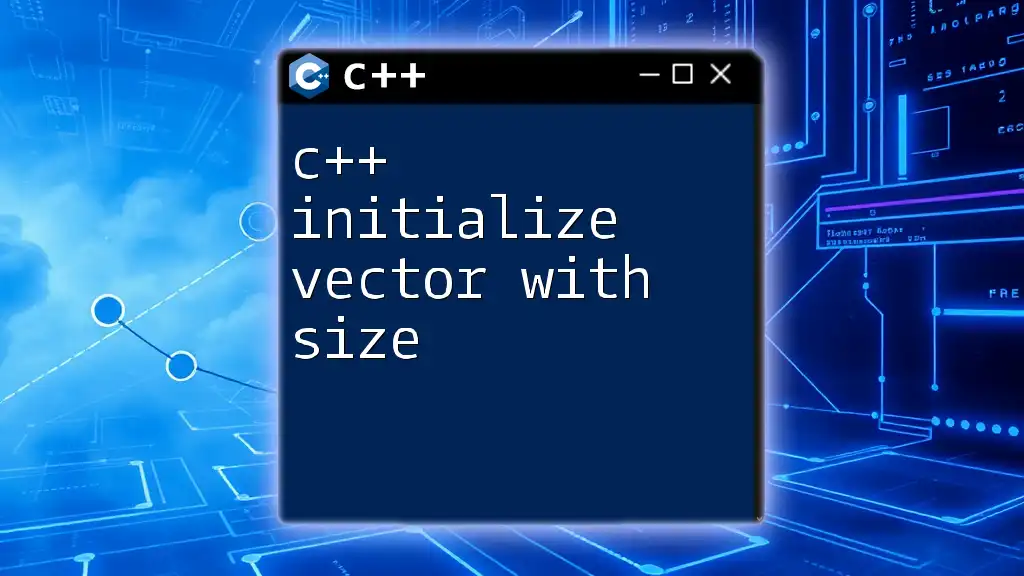
Additional Resources
To further enhance your understanding of C++ and array initialization, consider exploring the following:
- Official C++ documentation for detailed insights.
- Recommended programming books and online courses.
- Community forums and platforms dedicated to C++ programming for practical experience and peer feedback.
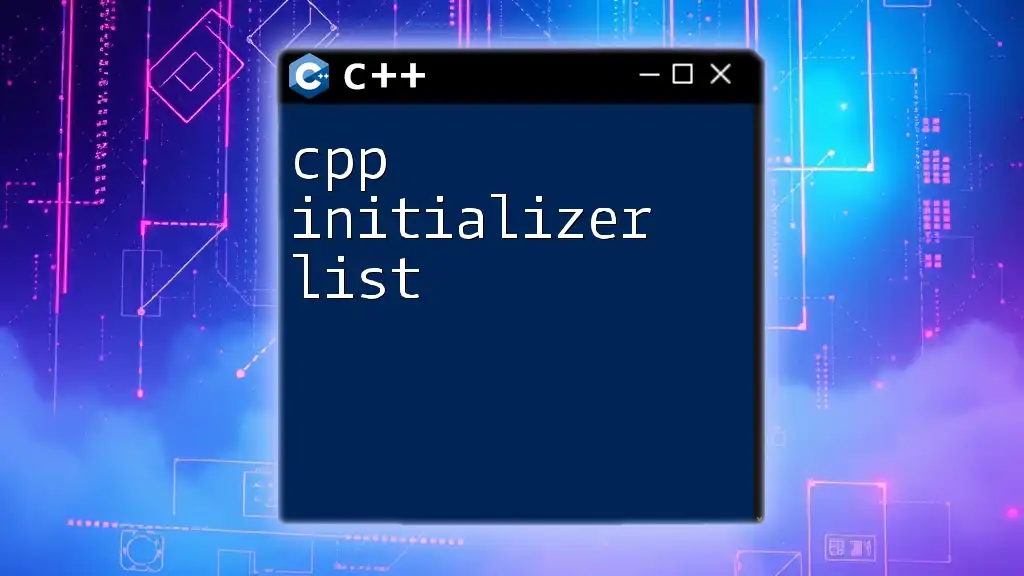
Call to Action
Now that you are equipped with knowledge on how to c++ initialize array to 0, why not take a moment to share your own experiences or ask any questions in the comments section? Don’t forget to subscribe for more insightful tips and tricks related to C++ programming!