In C++, an initializer list is a constructor feature that allows you to initialize member variables directly at the point of their declaration, leading to more concise and efficient code.
class Point {
public:
int x, y;
Point(int xVal, int yVal) : x(xVal), y(yVal) {} // using an initializer list
};
// Usage
Point p(10, 20);
What is an Initializer List?
An initializer list in C++ is a syntax used to initialize class member variables directly in the constructor's definition. This allows for a concise and clear way to set initial values for objects upon their creation.
Unlike typical constructor parameter passing, initializer lists provide an efficient way to initialize members before the constructor body executes. This is particularly beneficial when initializing `const` or reference members, which must be initialized using an initializer list since they cannot be assigned new values later on.
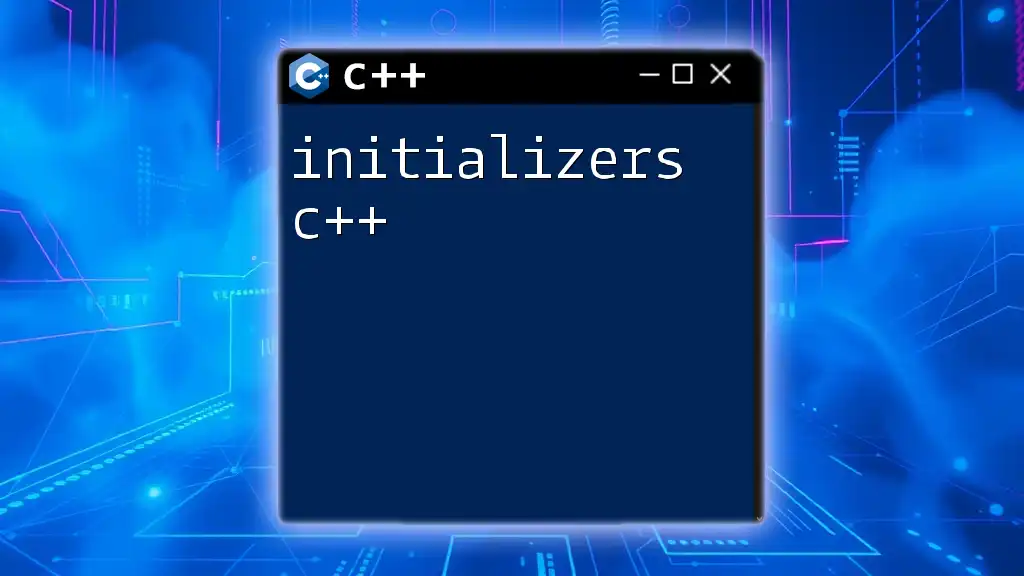
Why Use Initializer Lists?
Using initializer lists offers several critical advantages:
-
Performance Benefits: When you use an initializer list, objects are constructed directly rather than being default-constructed and then assigned values, which can lead to unnecessary overhead.
-
Initialization of const and Reference Members: Members declared as `const` or as references must be initialized upon their creation. An initializer list ensures that these members receive their values at the time of object construction.
-
Avoiding Unnecessary Default Construction: When using complex types, initializing an object with a default constructor followed by assignment can be inefficient. Initializer lists allow for direct initialization, leading to improved performance.
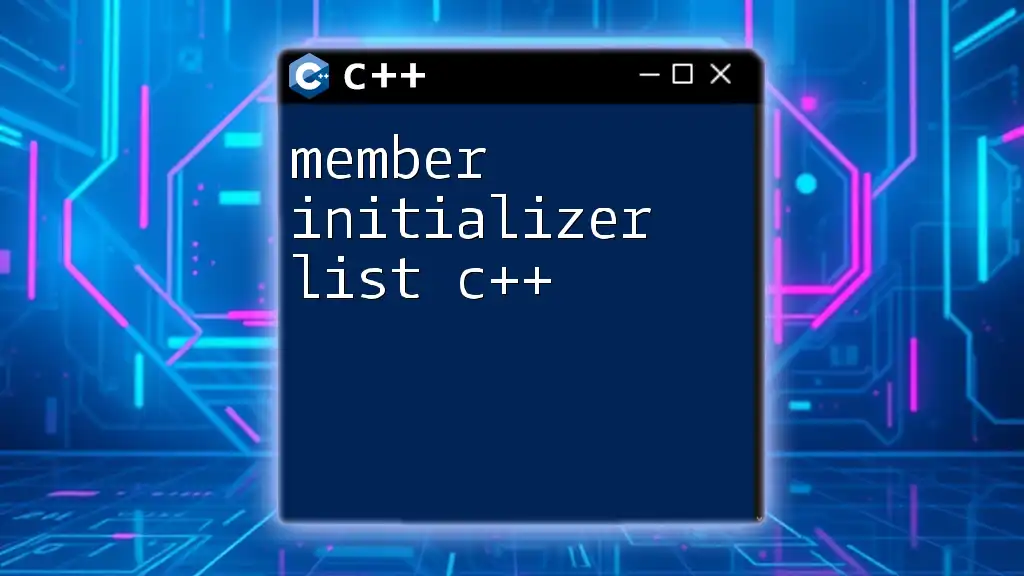
Syntax of Initializer Lists
Basic Structure
The general syntax for an initializer list is as follows:
ClassName(parameters) : member1(initializer1), member2(initializer2) {
// Constructor body
}
Here is a simple example demonstrating the syntax:
class MyClass {
public:
MyClass(int a, int b) : x(a), y(b) {}
private:
int x, y;
};
In this example, `MyClass` has two integer member variables, `x` and `y`, which are initialized directly in the constructor's initializer list.
Multiple Variables
Initializer lists can also be used to initialize multiple member variables simultaneously. Consider the example below:
class Point {
public:
Point(int x, int y) : x(x), y(y) {}
private:
int x, y;
};
This `Point` class initializes both `x` and `y` using the initializer list, providing a clean and efficient way of setting their values.
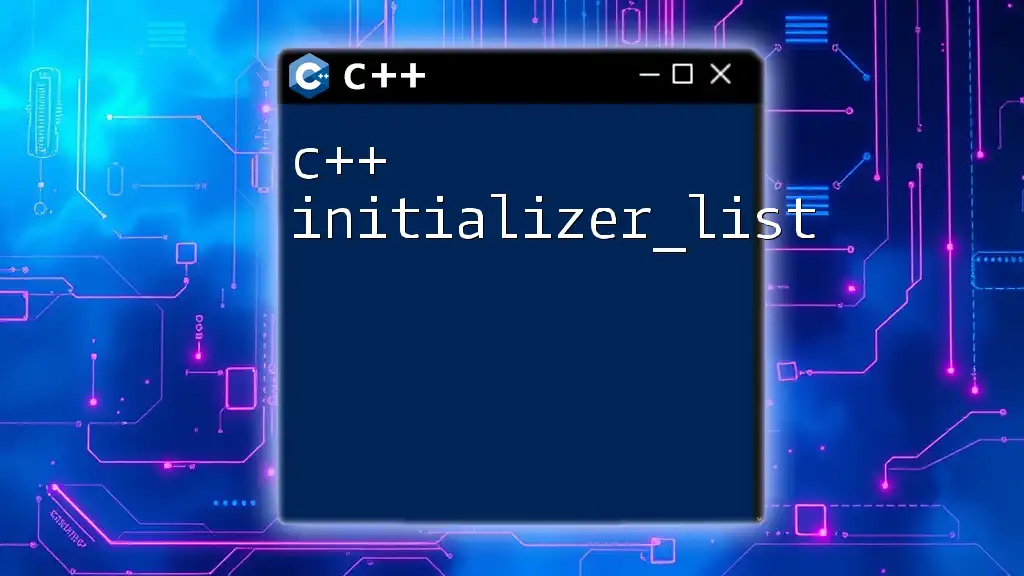
Understanding Constructor Initialization
How Initializer Lists Work with Constructors
The order of initialization in C++ is determined by the order of declaration in the class, not by the order in the initializer list. This is crucial understanding when designing classes to prevent unintentional bugs.
For instance:
class MyClass {
public:
MyClass(int a, int b) : y(b), x(a) {} // Order of initialization is x, then y
private:
int x;
int y;
};
Here, `x` is initialized before `y` due to its declaration order.
Default Member Initializers
With C++11 and later, default member initializers can be used to provide default values during declaration. This allows for specifying initial values without needing to do it in every constructor.
class Rectangle {
public:
Rectangle(int w, int h) : width(w), height(h) {}
private:
int width = 0; // Default member initializer
int height = 0;
};
In this case, `width` and `height` will receive values based on the constructor but will default to `0` if the constructor arguments are not provided.
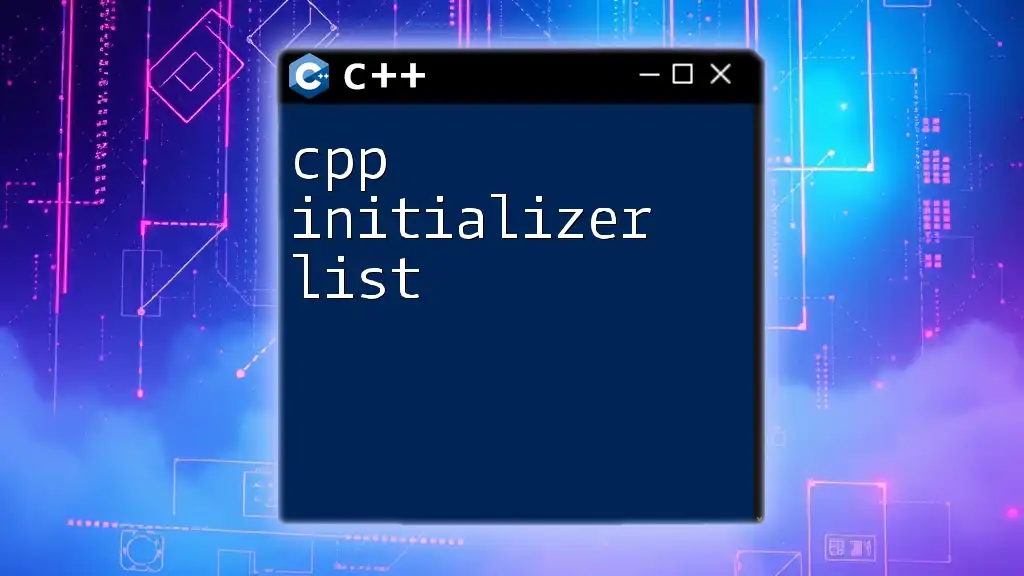
Advanced Concepts with Initializer Lists
Using Initializer Lists with Inheritance
Initializer lists are also useful in the context of inheritance. Base class constructors must be called using an initializer list in derived class constructors.
class Base {
public:
Base(int a) { /* initialization code */ }
};
class Derived : public Base {
public:
Derived(int a, int b) : Base(a), y(b) {}
private:
int y;
};
This structure shows how the `Derived` class constructor explicitly initializes its base class `Base` using an initializer list.
Initializing Arrays and Containers
Initializer lists can also be utilized in scenarios involving array initialization and STL containers, such as `std::vector`. Here’s an example:
class ArrayHolder {
public:
ArrayHolder(std::initializer_list<int> list) : data(list) {}
private:
std::vector<int> data;
};
In this code snippet, the constructor takes an initializer list, facilitating the efficient initialization of the `data` member.
Initialization of Const and Reference Members
When dealing with `const` and reference member variables, it's essential to initialize them through an initializer list, as they cannot be assigned values later.
class ConstRefHolder {
public:
ConstRefHolder(const int& val) : ref(val), constVal(val) {}
private:
const int& ref;
const int constVal;
};
In this example, the `ConstRefHolder` class demonstrates how both a constant reference and a constant value must be initialized using an initializer list to adhere to C++ constraints.
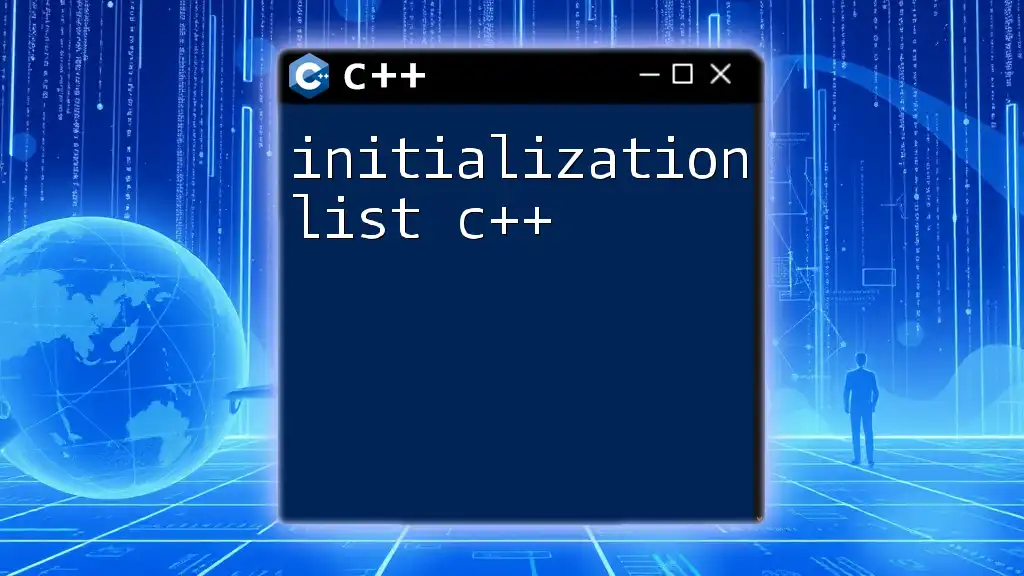
Common Pitfalls and Best Practices
Mistakes to Avoid with Initializer Lists
While using initializer lists is beneficial, several common pitfalls can arise:
-
Incorrect Initialization Order: Forgetting that initializations occur in the order of declaration can lead to using uninitialized data.
-
Improper Handling of References: Failing to initialize reference members can cause compilation errors, as references cannot exist without being initialized.
Best Practices for Efficient Use
To leverage initializer lists effectively, consider the following best practices:
-
Always prefer initializer lists for initializing member variables, especially complex data types.
-
Use default member initializers where applicable, as they reduce duplication across constructors.
-
Clearly document the expected order of parameters in your constructors to avoid confusion about member initialization.
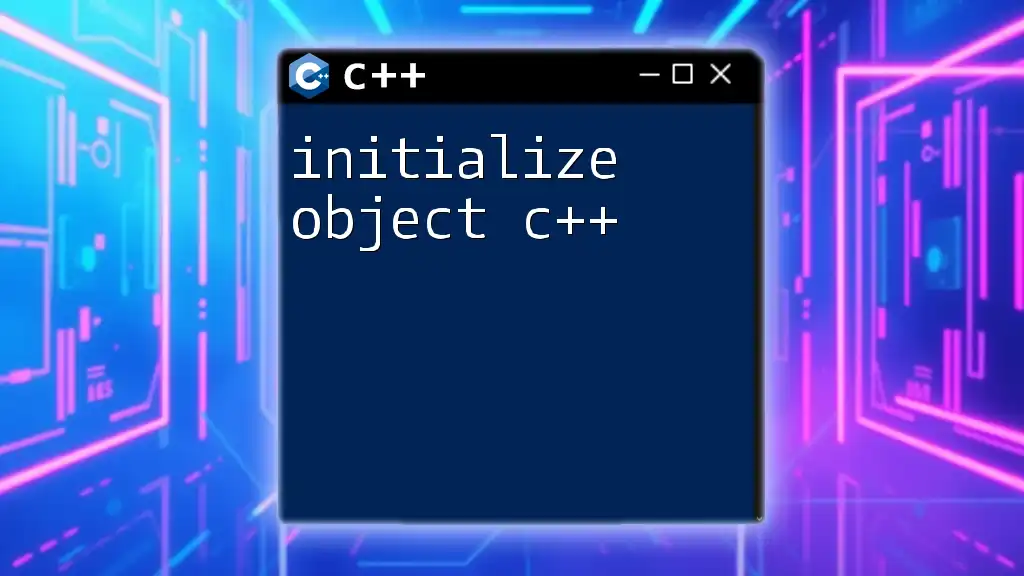
Conclusion
In summary, initializer lists in C++ provide a powerful and efficient mechanism for initializing class members. They eliminate unnecessary overhead, enforce necessary constraints for `const` and reference members, and ensure clear initialization practices.
To become adept at using initializer lists, it's encouraged to incorporate them into your own C++ classes frequently, experimenting with their syntactic advantages and performance benefits.
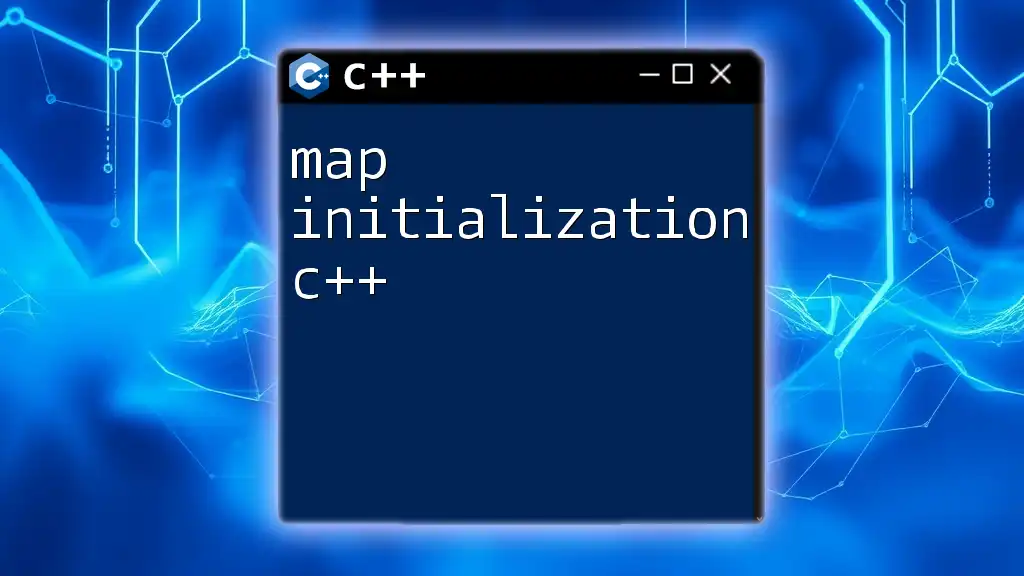
Additional Resources
For further study on initializer lists and related C++ features, refer to C++ official documentation and consider exploring advanced C++ programming textbooks and online courses tailored to enhance your understanding.
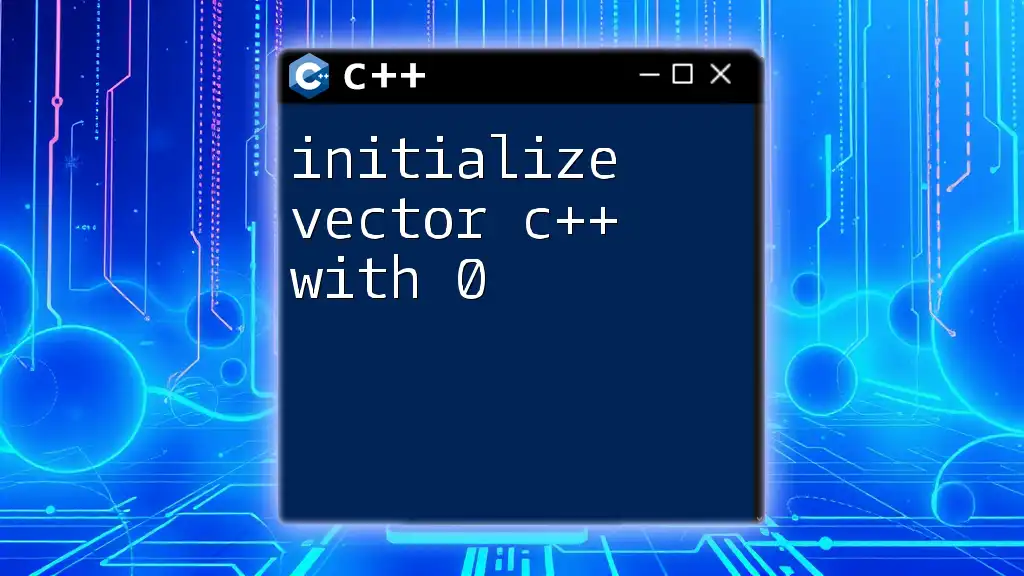
Call to Action
Feel free to share your experiences or questions about using initializer lists in your own projects. Engaging with the community will undoubtedly deepen your understanding of this fundamental C++ feature!