A singly linked list in C++ is a data structure that consists of nodes where each node contains a value and a pointer to the next node, allowing dynamic memory allocation and efficient insertion and deletion.
Here's a simple code snippet demonstrating a singly linked list:
#include <iostream>
class Node {
public:
int data;
Node* next;
Node(int value) : data(value), next(nullptr) {}
};
class SinglyLinkedList {
public:
Node* head;
SinglyLinkedList() : head(nullptr) {}
void insert(int value) {
Node* newNode = new Node(value);
newNode->next = head;
head = newNode;
}
void display() {
Node* current = head;
while (current) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
};
int main() {
SinglyLinkedList list;
list.insert(10);
list.insert(20);
list.display(); // Output: 20 10
return 0;
}
Understanding Linked Lists
What is a Linked List?
A linked list is a linear data structure where elements are stored in nodes, each pointing to the next node in the sequence. Unlike arrays, linked lists do not have a fixed size; instead, they use dynamic memory allocation. This structure allows for flexible data management.
Why Use Linked Lists?
- Dynamic Memory Allocation: Linked lists can easily adjust to varying sizes by allocating memory as needed.
- Efficient Insertions and Deletions: Adding or removing elements doesn’t require shifting elements, which is a significant performance advantage over arrays.
- Use Cases: Linked lists are widely employed in real-world applications such as implementing queues, stacks, and adjacency lists for graphs.

Understanding Singly Linked Lists
Definition of Singly Linked List
A singly linked list is a type of linked list in which each node contains data and a pointer (or reference) to the next node. The last node points to `nullptr`, indicating the end of the list. This structure supports efficient traversal in one direction—from the head to the end.
Each node can be represented as follows:
class Node {
public:
int data;
Node* next;
Node(int val) : data(val), next(nullptr) {}
};
Visual Representation of Singly Linked Lists
Imagine a series of boxes (nodes), each containing data and an arrow pointing to the next box. This visual representation helps in understanding how data is organized in a singly linked list.

Basic Operations on Singly Linked Lists
Creating a Singly Linked List
To create a singly linked list, you need a head pointer initialized to `nullptr`, which will eventually point to the first node once it’s created.
Inserting Nodes
At the Beginning
Inserting a node at the beginning of the singly linked list involves creating a new node, pointing its `next` reference to the current head, and then updating the head to the new node.
void insertAtHead(Node*& head, int data) {
Node* newNode = new Node(data);
newNode->next = head;
head = newNode;
}
At the End
Inserting a node at the end requires traversing to the last node (i.e., the node whose `next` is `nullptr`) and updating its `next` reference to the new node.
void insertAtTail(Node*& head, int data) {
Node* newNode = new Node(data);
if (!head) {
head = newNode;
return;
}
Node* temp = head;
while (temp->next) {
temp = temp->next;
}
temp->next = newNode;
}
At a Specific Position
To insert a node at a specific position, you will traverse the list until you reach the desired index, adjusting the pointers accordingly. Care should be taken to check for valid indices to avoid segmentation faults.
Deleting Nodes
Deleting a Node by Value
To delete a node with a specific value, traverse the list while keeping track of the previous node. If you find the target value, adjust the pointers accordingly to bypass the node and delete it.
void deleteNode(Node*& head, int value) {
if (!head) return; // If the list is empty
if (head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* current = head;
while (current->next && current->next->data != value) {
current = current->next;
}
if (current->next) {
Node* temp = current->next;
current->next = current->next->next;
delete temp;
}
}
Deleting at the Beginning and End
- At the Beginning: Update the head to point to the second node and delete the first node.
- At the End: Traverse to the second-to-last node and update its `next` pointer to `nullptr`, then delete the last node.

Traversing a Singly Linked List
Basic Traversal Technique
To traverse a singly linked list, start from the head and follow the `next` pointers until you reach a node that points to `nullptr`. This is essential for accessing, displaying, or counting nodes within the list.
void printList(Node* head) {
Node* temp = head;
while (temp) {
std::cout << temp->data << " -> ";
temp = temp->next;
}
std::cout << "nullptr" << std::endl;
}
The above code will display the entire list, allowing you to visualize its contents.
Use Cases for Traversal
- Finding a Specific Value: By traversing, you can check if a node exists within the list.
- Counting the Number of Nodes: Traversal can lead to counting the total nodes, which may be useful for other operations.

Advanced Topics
Reversing a Singly Linked List
Reversing the order of nodes in a singly linked list is an interesting problem. You need to reassign the `next` pointers until the list is reversed.
void reverseList(Node*& head) {
Node* prev = nullptr;
Node* current = head;
Node* next = nullptr;
while (current) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
head = prev;
}
This algorithm efficiently reverses the list in a single traversal.
Detecting Loops in a Singly Linked List
Using Floyd’s Cycle Detection Algorithm, you can determine whether a loop exists in the list. It employs two pointers that traverse at different speeds. If they meet, a loop is present; if one reaches `nullptr`, the list is linear.
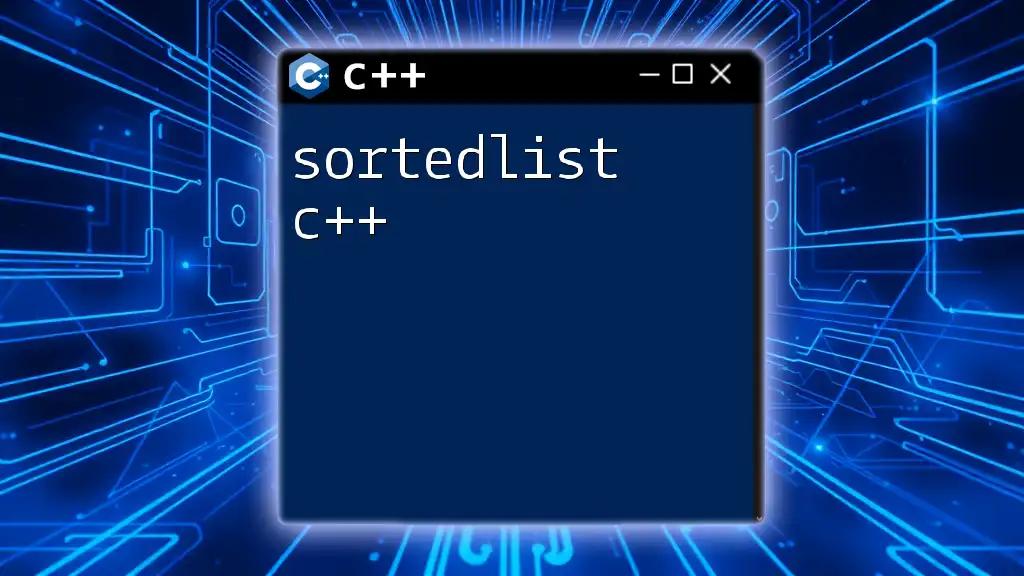
Conclusion
In summary, a singly linked list in C++ is a powerful data structure that presents numerous advantages for dynamic data handling and manipulation. Through its various operations like insertion, deletion, and traversal, it is clear that singly linked lists offer significant flexibility.
Understanding these concepts not only enhances your programming skills but lays a solid foundation for tackling more complex data structures. As you become proficient in using singly linked lists, consider exploring advanced topics such as doubly linked lists and balanced trees for further knowledge expansion.
![Understanding Unsigned Int in C++ [Quick Guide]](/_next/image?url=%2Fimages%2Fposts%2Fu%2Funsigned-int-cpp.webp&w=1080&q=75)
FAQs about Singly Linked Lists
What is the time complexity of operations in singly linked lists?
The time complexity for the main operations in singly linked lists includes:
- Insertion at the head: O(1)
- Insertion at the tail: O(n)
- Deletion by value: O(n)
- Traversal: O(n)
Can linked lists be circular?
Yes, linked lists can be circular, meaning the last node points back to one of the previous nodes instead of `nullptr`. This structure is useful for applications such as round-robin scheduling and maintaining a continuous sequence of items.