A circular linked list in C++ is a data structure where each node points to the next node, with the last node pointing back to the first, creating a circular loop.
Here’s a simple code snippet to illustrate a circular linked list:
#include <iostream>
class Node {
public:
int data;
Node* next;
Node(int value) : data(value), next(nullptr) {}
};
class CircularLinkedList {
public:
Node* head;
CircularLinkedList() : head(nullptr) {}
void append(int value) {
Node* newNode = new Node(value);
if (!head) {
head = newNode;
head->next = head; // Point to itself
} else {
Node* temp = head;
while (temp->next != head) {
temp = temp->next;
}
temp->next = newNode;
newNode->next = head; // Complete the circle
}
}
void display() {
if (head) {
Node* temp = head;
do {
std::cout << temp->data << " ";
temp = temp->next;
} while (temp != head);
std::cout << std::endl;
}
}
};
int main() {
CircularLinkedList cll;
cll.append(1);
cll.append(2);
cll.append(3);
cll.display(); // Output: 1 2 3
return 0;
}
What is a Circular Linked List?
A circular linked list is a data structure similar to a traditional linked list, but with a key difference: in a circular linked list, the last node points back to the first node, creating a circular pathway. This structural feature enables continuous traversal through the list without reaching a null pointer, which is common in standard linked lists. This unique property makes circular linked lists particularly useful in various applications, such as implementing circular buffers or scheduling processes.
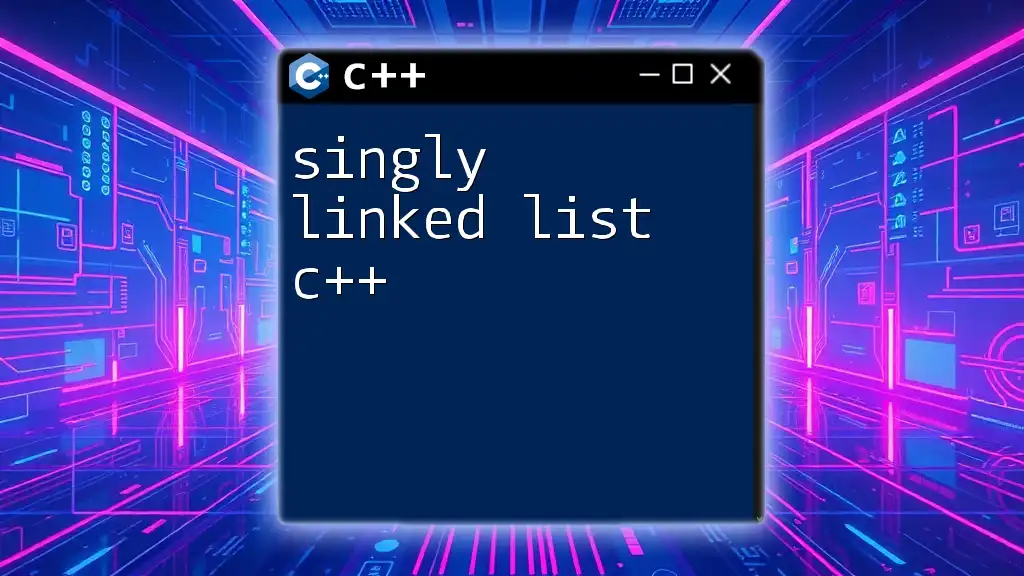
Importance of Circular Linked Lists
Circular linked lists offer several advantages over traditional linked lists:
- Memory Efficiency: Since nodes are not deallocated until they are explicitly removed, circular linked lists can effectively manage memory allocation.
- Efficient Traversal: The circular nature allows for looping through the list continuously, which is beneficial for applications like round-robin scheduling.
- Dynamic Size: Like traditional linked lists, circular linked lists can grow and shrink in size dynamically, accommodating varying amounts of data.
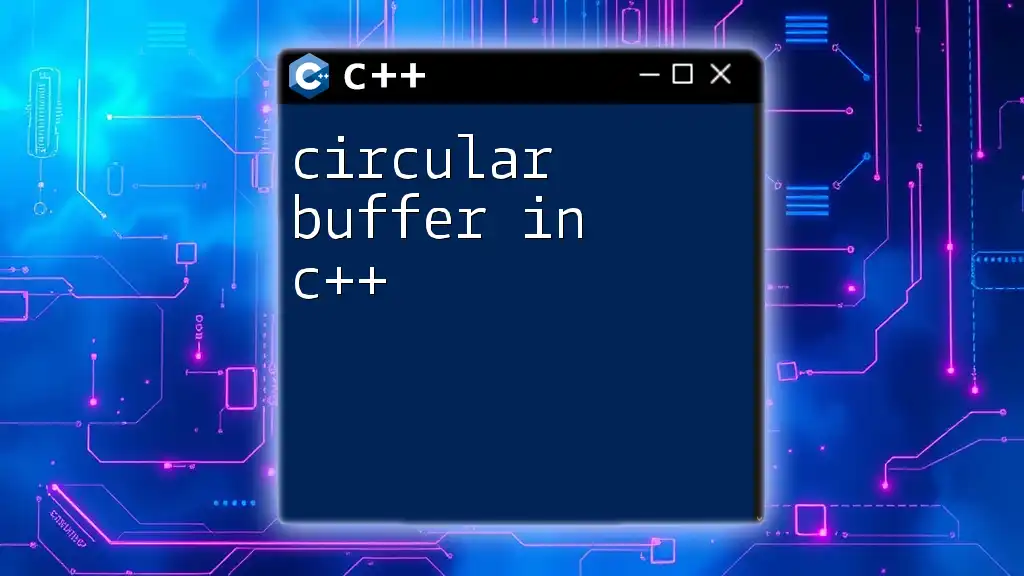
Basic Structure of a Circular Linked List
Node Structure
Each node in a circular linked list comprises two key components: the data it holds and a pointer to the next node. Here's the definition of a node:
struct Node {
int data;
Node* next;
};
Circular Link Explanation
In a circular linked list, the last node does not point to `nullptr` as it would in a traditional linked list. Instead, it points back to the head of the list. This connection forms a loop, allowing traversal to continue indefinitely. Visual representations can help clarify this structure.
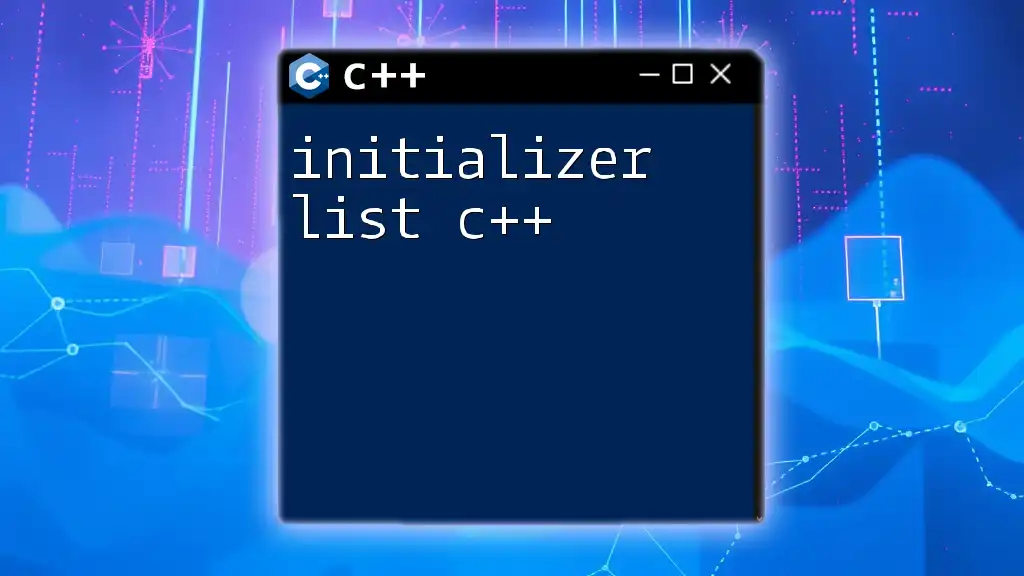
How to Create a Circular Linked List
Creating Nodes
To start, you’ll need a method to create nodes. The following code snippet illustrates how to create a new node:
Node* createNode(int value) {
Node* newNode = new Node;
newNode->data = value;
newNode->next = nullptr; // Initial next pointer will be null
return newNode;
}
Inserting Elements
In circular linked lists, we can insert new elements either at the beginning or the end.
At the Beginning
To insert a node at the beginning, we need to update the head pointer and maintain the connection with the last node:
void insertAtBeginning(Node*& head, int value) {
Node* newNode = createNode(value);
if (!head) {
head = newNode;
newNode->next = head; // Last node points to head itself
} else {
Node* temp = head;
while (temp->next != head) { // Traverse to find last node
temp = temp->next;
}
temp->next = newNode; // Insert new node at the beginning
newNode->next = head; // New node points to old head
head = newNode; // Update head to new node
}
}
At the End
To insert a node at the end of the list, follow this approach:
void insertAtEnd(Node*& head, int value) {
Node* newNode = createNode(value);
if (!head) {
head = newNode;
newNode->next = head; // Last node points to head
} else {
Node* temp = head;
while (temp->next != head) { // Find the last node
temp = temp->next;
}
temp->next = newNode; // Link last node to new node
newNode->next = head; // New node points to head
}
}
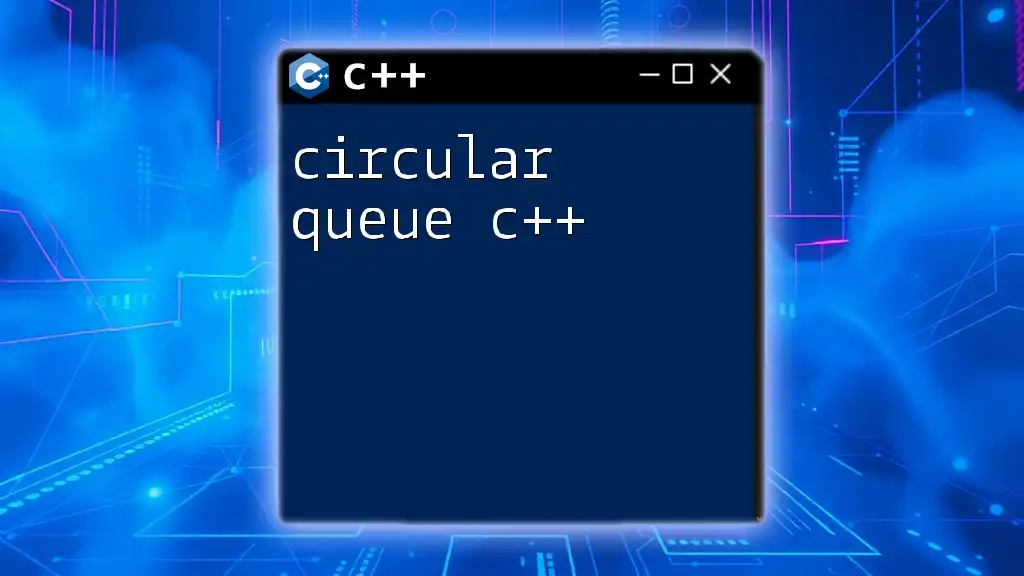
Displaying Elements in a Circular Linked List
To traverse and display the elements in a circular linked list, you can implement the following method:
void display(Node* head) {
if (!head) return; // Check for empty list
Node* temp = head;
do {
std::cout << temp->data << " "; // Display current node data
temp = temp->next; // Move to next node
} while (temp != head); // Stop when back to head
std::cout << std::endl;
}
This function initiates traversal from the head and continues until it loops back to the starting point.
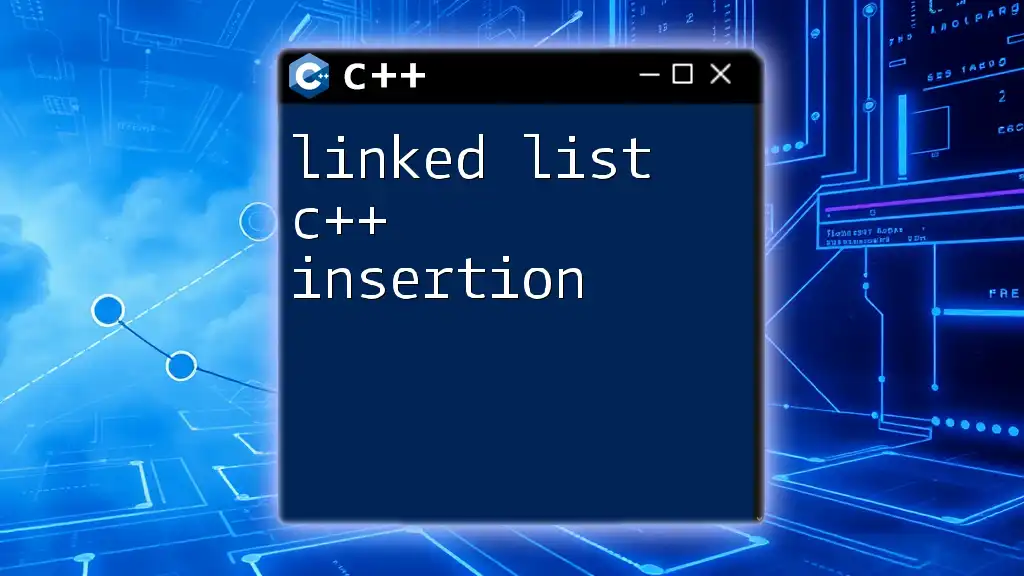
Deleting Nodes in a Circular Linked List
Deleting a Node (By Key)
Deleting a specific node involves searching for it by its value and adjusting the pointers accordingly:
void deleteNode(Node*& head, int key) {
if (!head) return; // Empty list check
Node* temp = head;
Node* prev = nullptr;
// If head node itself holds the key
if (temp != nullptr && temp->data == key) {
prev = head;
while (prev->next != head) prev = prev->next;
if (temp->next == head) { // Only one node
delete temp; // Deallocate memory
head = nullptr; // List becomes empty
return;
}
prev->next = temp->next; // Adjust last node's pointer
head = temp->next; // Update head
delete temp; // Deallocate memory
return;
}
// Searching for the key to be deleted
while (temp->next != head && temp->data != key) {
prev = temp;
temp = temp->next; // Move to next node
}
// If key was not present in linked list
if (temp->data != key) return;
prev->next = temp->next; // Bypass the node to be deleted
delete temp; // Deallocate memory
}
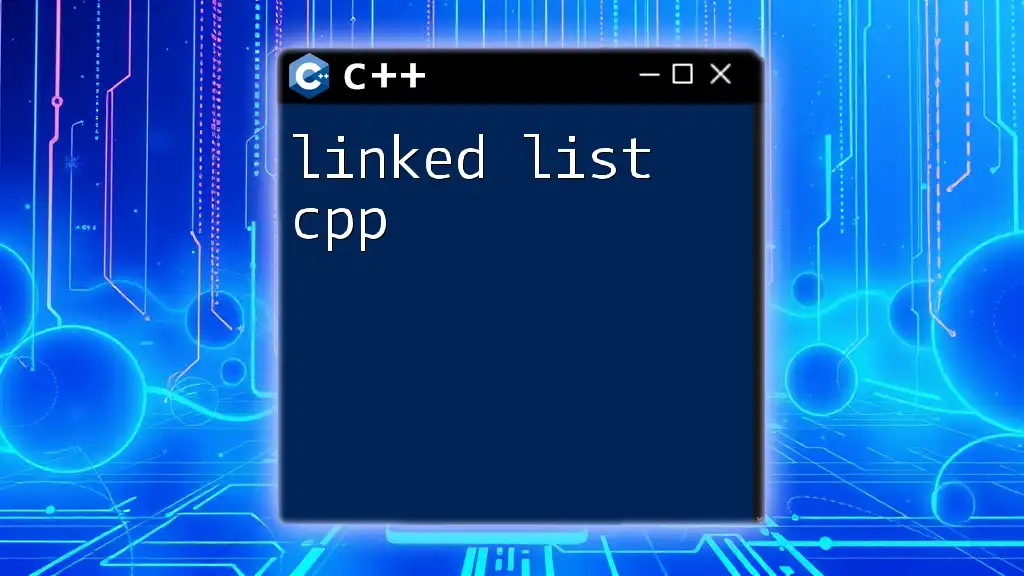
Advanced Operations on Circular Linked Lists
Reverse a Circular Linked List
Reversing a circular linked list can be implemented by rearranging the next pointers of the nodes. This can be crucial for certain applications and algorithms:
void reverse(Node*& head) {
Node* prev = nullptr, *current = head, *next = nullptr;
do {
next = current->next; // Store the next node
current->next = prev; // Reverse the link
prev = current; // Move prev forward
current = next; // Move current forward
} while (current != head); // Continue until back to head
head->next = prev; // Connect last node to new head
head = prev; // Update head to the new beginning
}
Finding the Length
Determining the length of a circular linked list involves counting the nodes till we return to the head:
int length(Node* head) {
if (!head) return 0; // Empty list check
int count = 0;
Node* temp = head;
do {
count++; // Count each node
temp = temp->next; // Move to next node
} while (temp != head); // Stop when back to head
return count; // Return count
}
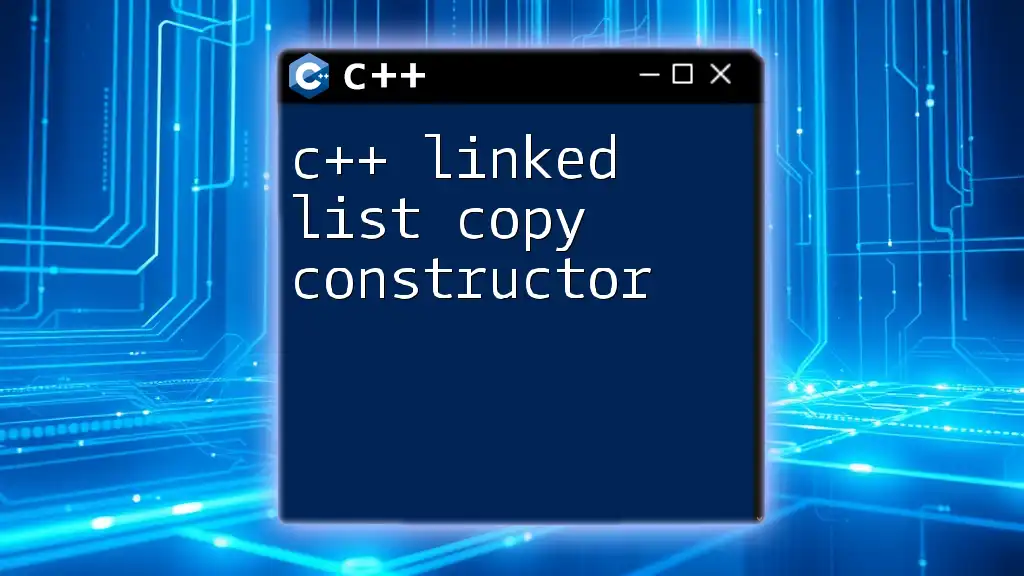
Common Mistakes and Best Practices
Memory Management
One of the biggest risks with circular linked lists is memory leaks. Always ensure that whenever nodes are deleted, you properly deallocate memory to avoid leaks. Use tools like Valgrind to help identify memory-related issues.
Edge Cases
Pay close attention to edge cases, such as operations on an empty list or a list containing a single node. For instance, ensure that insertions and deletions are handled correctly under these conditions to maintain the integrity of the circular links.
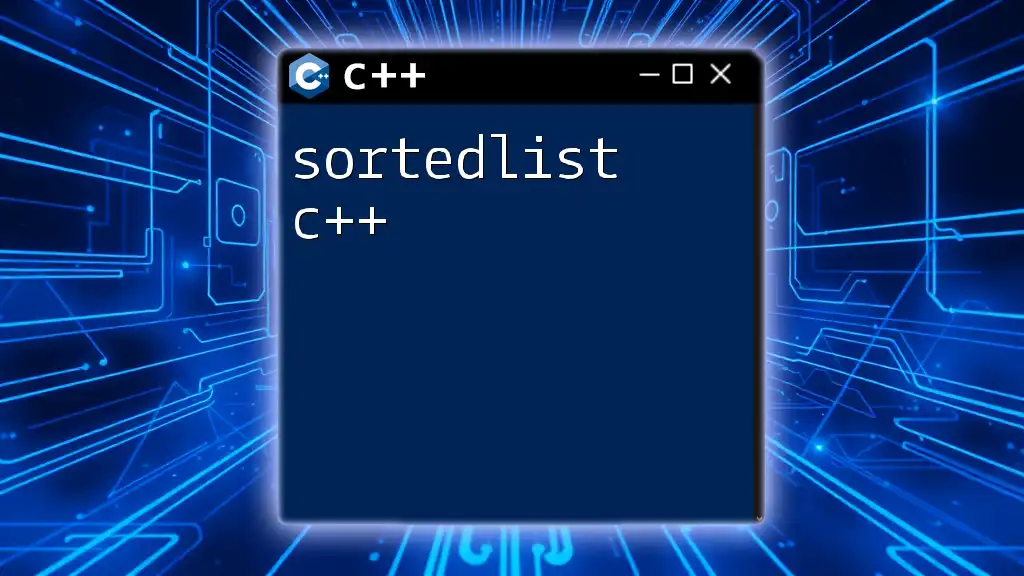
Conclusion
Circular linked lists are powerful data structures that provide numerous advantages in specific applications. Understanding how to implement, traverse, and manipulate them is crucial for any developer working with linked data structures. Being mindful of the complexities and best practices associated with circular linked lists will help you harness their full potential in your coding endeavors.
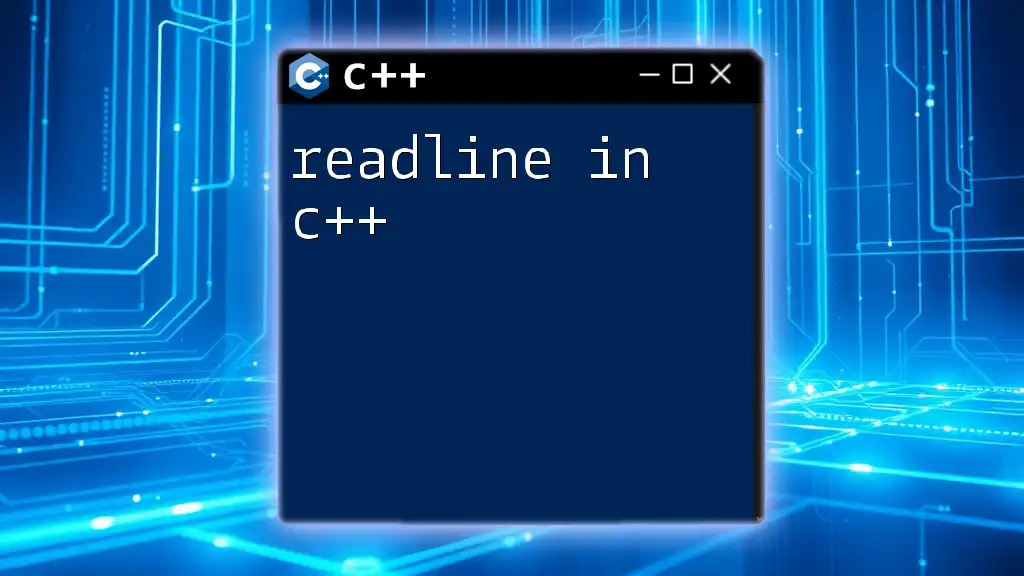
Further Reading and Resources
For enhanced comprehension, consider exploring more advanced topics, such as doubly circular linked lists or data structures that incorporate circular linked lists, like queues and hash tables.