A circular queue in C++ is a linear data structure that uses a fixed-size array in a circular manner, allowing for efficient insertion and deletion of elements without the need for shifting.
Here’s a simple implementation of a circular queue in C++:
#include <iostream>
#define SIZE 5
class CircularQueue {
private:
int arr[SIZE];
int front, rear;
public:
CircularQueue() : front(-1), rear(-1) {}
bool isFull() {
return (front == (rear + 1) % SIZE);
}
bool isEmpty() {
return (front == -1);
}
void enqueue(int value) {
if (isFull()) {
std::cout << "Queue is full\n";
} else {
rear = (rear + 1) % SIZE;
arr[rear] = value;
if (front == -1) front = 0;
}
}
int dequeue() {
if (isEmpty()) {
std::cout << "Queue is empty\n";
return -1;
} else {
int value = arr[front];
if (front == rear) {
front = rear = -1; // Reset the queue after removing the last element
} else {
front = (front + 1) % SIZE;
}
return value;
}
}
void display() {
if (isEmpty()) {
std::cout << "Queue is empty\n";
return;
}
for (int i = front; i != rear; i = (i + 1) % SIZE) {
std::cout << arr[i] << " ";
}
std::cout << arr[rear] << "\n"; // Display the rear
}
};
int main() {
CircularQueue cq;
cq.enqueue(10);
cq.enqueue(20);
cq.enqueue(30);
cq.display();
cq.dequeue();
cq.display();
return 0;
}
Understanding Circular Queue in C++
Definition of Circular Queue
A circular queue is a linear data structure that operates in a circular manner. Unlike a conventional linear queue, where the last element is followed by `NULL`, allowing no further additions, a circular queue wraps around when it reaches the end of the buffer. This technique allows efficient use of space and eliminates the issue of the queue being full even when it has unused spaces at the beginning.
Use Cases for Circular Queue
Circular queues are particularly useful in scenarios where a fixed-size buffer is needed. Common applications include:
- CPU Scheduling: Circular queues serve as a basis for managing tasks and processes in operating systems, maintaining fairness and efficiency.
- Buffering: Used in scenarios like IO Buffers where the data is processed in a cyclic manner.
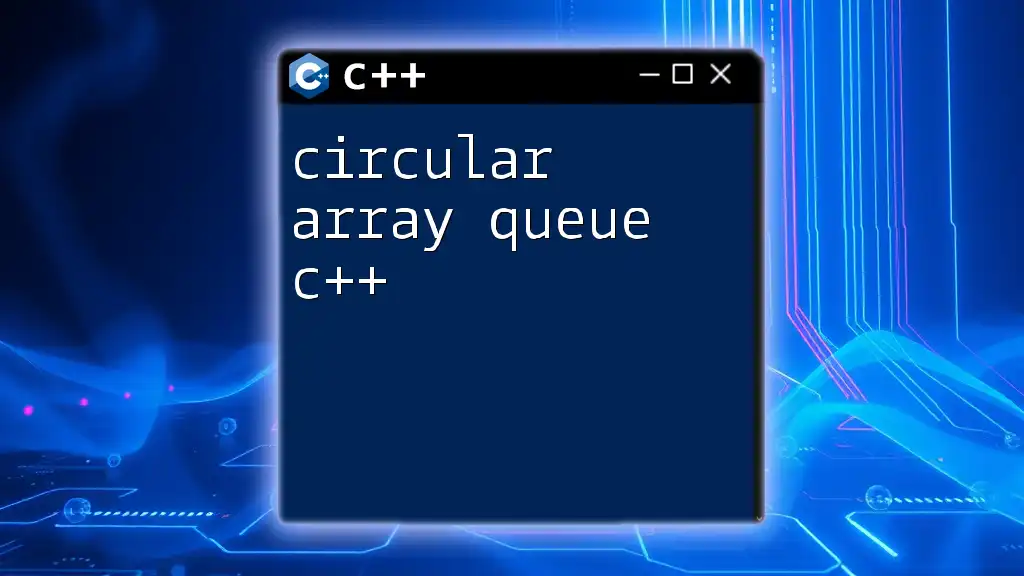
Basic Concepts of Circular Queue
Basic Terminology
To fully understand a circular queue implementation in C++, you'll want to familiarize yourself with a few key terms:
- Enqueue: The operation of adding an item to the rear of the queue.
- Dequeue: The operation of removing an item from the front of the queue.
- Front and Rear Pointers: Indicators used to track the current positions of the first and last elements within the queue.
Circular Queue Properties
Circular queues utilize a wrap-around technique to handle the connections between the last and the first cells in the data structure. Key properties include:
- Capacity vs Size: Capacity refers to the total number of elements the queue can hold, while size refers to the current number of elements in the queue.
- Availability: By utilizing this circular mechanism, a queue can be effectively maintained even as elements are added or removed, ensuring that space isn't wasted.
Advantages of Circular Queue
The circular queue offers several advantages:
- Efficient Memory Usage: Users can readily enqueue and dequeue without leaving gaps, optimizing memory allocation.
- Reduction of Wasted Space: It resolves the problem of unused spaces that are common in linear queues, allowing the queue to maintain a consistent fill ratio.
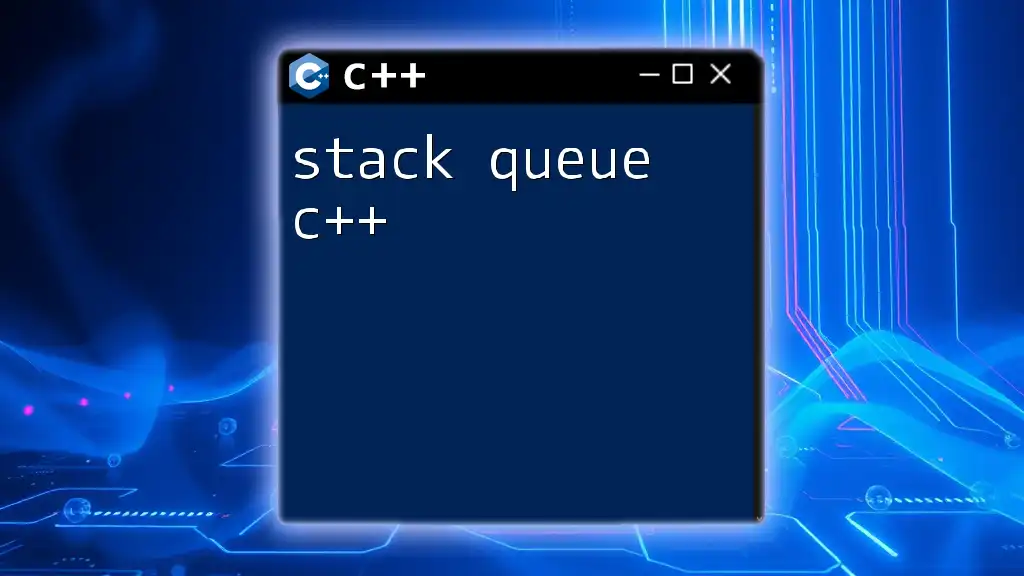
How to Implement Circular Queue in C++
Setting Up the Circular Queue Structure
To create a circular queue in C++, start by defining a class or struct that encompasses the essential components. Below is an example of how to set this up:
class CircularQueue {
private:
int* arr; // Pointer to the array holding the queue
int front; // Index of the front element
int rear; // Index of the rear element
int maxSize; // Maximum size of the queue
public:
CircularQueue(int size); // Constructor
~CircularQueue(); // Destructor
bool enqueue(int value); // Function to add an element
int dequeue(); // Function to remove an element
bool isEmpty(); // Check if the queue is empty
bool isFull(); // Check if the queue is full
};
Constructor and Destructor Implementation
The constructor initializes the queue with a specified size, while the destructor cleans up allocated memory. Here’s how you might implement these methods:
CircularQueue::CircularQueue(int size) {
arr = new int[size];
maxSize = size;
front = -1;
rear = -1;
}
CircularQueue::~CircularQueue() {
delete[] arr; // Deallocate the memory when finished
}
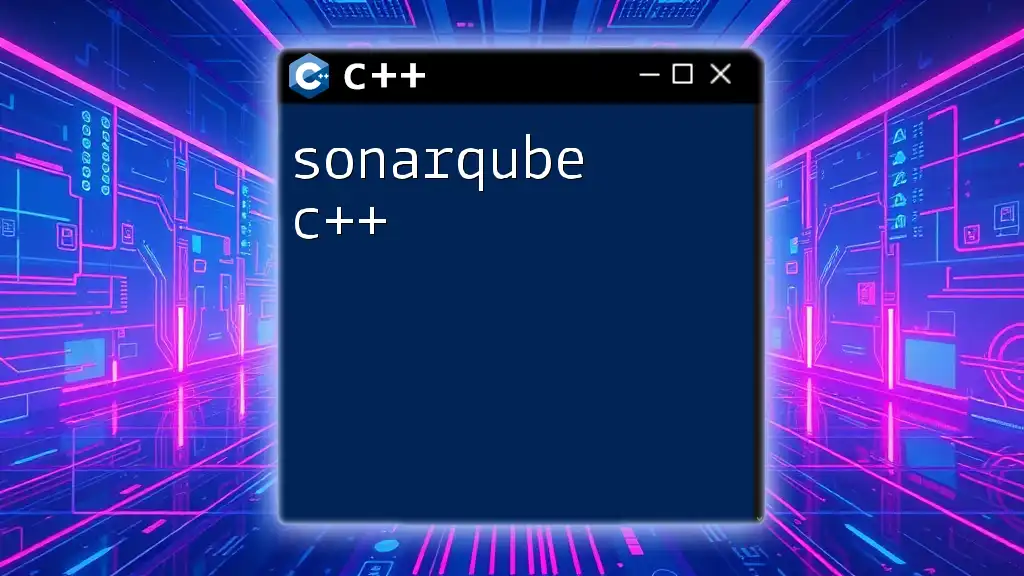
Enqueue Operation in Circular Queue
Function Logic for Enqueue
The `enqueue` function adds an element at the rear of the queue. Here’s a basic implementation:
bool CircularQueue::enqueue(int value) {
if (isFull()) {
return false; // Queue is full, cannot enqueue
}
if (front == -1) {
front = 0; // Set front to the starting index
}
rear = (rear + 1) % maxSize; // Circular increment
arr[rear] = value; // Add the element
return true; // Successful enqueue
}
Handling Overflow Conditions
Before adding an item, your code should check if the queue is full:
bool CircularQueue::isFull() {
return ((rear + 1) % maxSize == front);
}
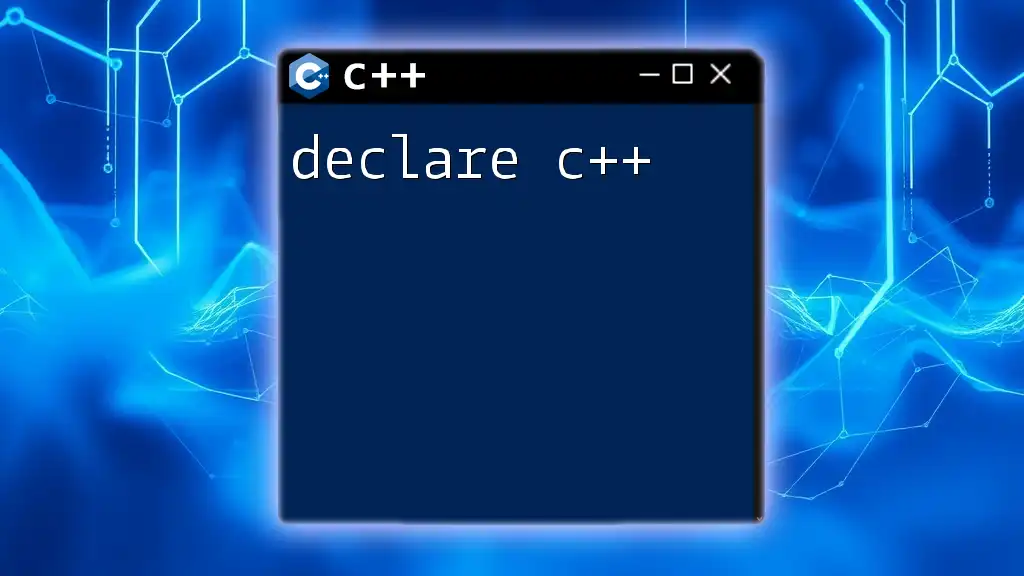
Dequeue Operation in Circular Queue
Function Logic for Dequeue
To remove an item from the front, you implement the `dequeue` function. Below is an example of how this may look:
int CircularQueue::dequeue() {
if (isEmpty()) {
return -1; // Queue is empty, cannot dequeue
}
int value = arr[front]; // Capture the front value
if (front == rear) {
front = rear = -1; // Reset if the queue becomes empty
} else {
front = (front + 1) % maxSize; // Circular increment
}
return value; // Return the dequeued value
}
Handling Underflow Conditions
Be sure to check for underflow situations where trying to dequeue from an empty queue could lead to errors:
bool CircularQueue::isEmpty() {
return (front == -1);
}
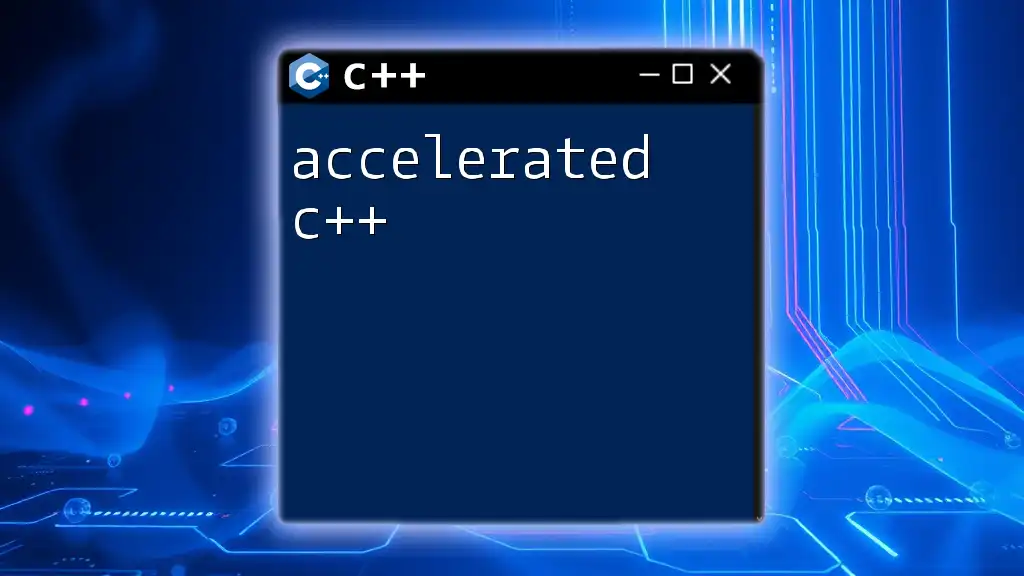
Circular Queue Operations Overview
Displaying the Queue
It’s beneficial to provide a method that displays the current state of the queue. Here’s an example of how you can implement this:
void CircularQueue::display() {
if (isEmpty()) {
std::cout << "Queue is empty\n";
return;
}
int i = front;
std::cout << "Circular Queue: ";
do {
std::cout << arr[i] << " ";
i = (i + 1) % maxSize;
} while (i != (rear + 1) % maxSize);
std::cout << "\n";
}
Quick Reference to Operations
Understanding the actions taken during enqueue and dequeue operations can be greatly aided with diagrams or flow charts, although these are not included here.
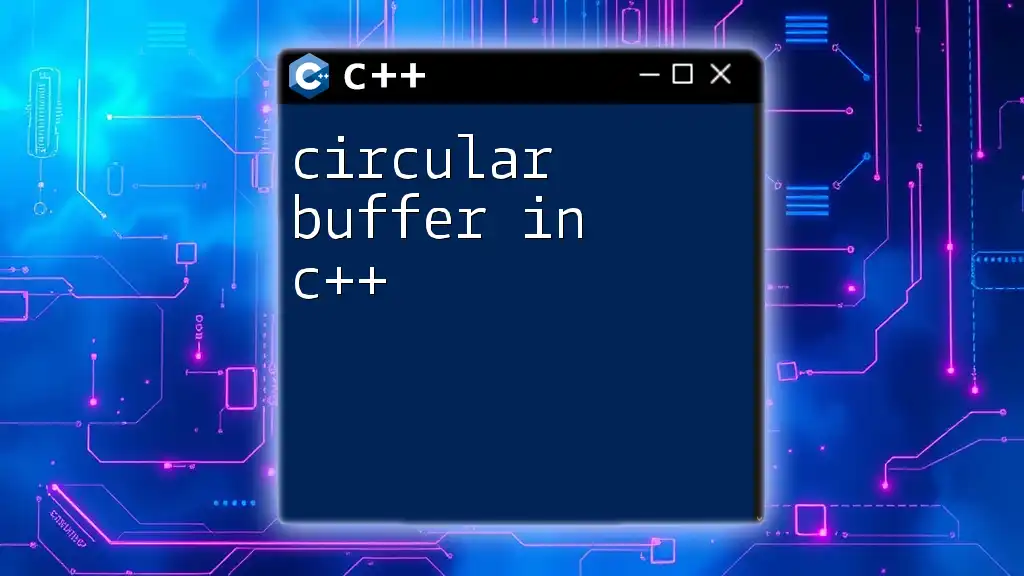
Comparison with Linear Queue
Key Differences Between Circular and Linear Queues
Circular queues differ from linear queues in essential ways:
- Memory Usage: A linear queue may waste space after several enqueue and dequeue operations, while a circular queue optimally utilizes available space.
- Operation Efficiency: Circling back to the first element upon reaching the last ensures that all operations can continue seamlessly without the need for reallocation.
When to Use Circular Queue Over Linear Queue
It's advisable to choose circular queues over linear ones in situations requiring high-frequency enqueue and dequeue operations. Scenarios like task scheduling, resource management, and network buffering can greatly benefit from the circular nature.
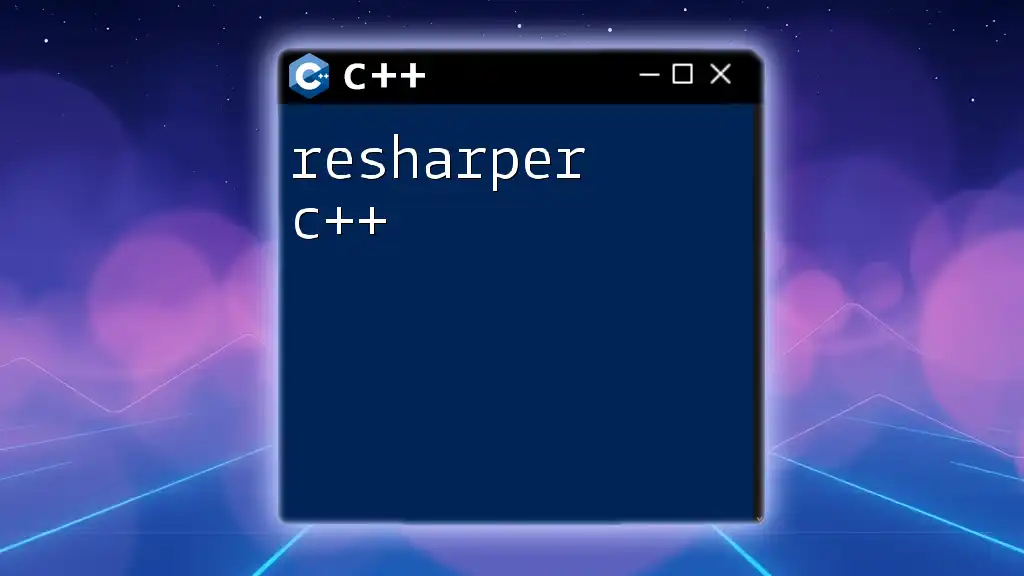
Common Problems and Solutions
Debugging Circular Queue Implementation
Potential issues can arise in circular queue implementations, such as:
- Incorrect index management leading to overflows or underflows.
- Ensure that the logic for incrementing `front` and `rear` pointers is wrapped within the bounds of the circular array.
Complexity Analysis
When considering performance, the time complexities for enqueue and dequeue operations in circular queues are O(1), meaning that operations can be executed in constant time. Space complexity is O(n), where n is the maximum size of the queue.
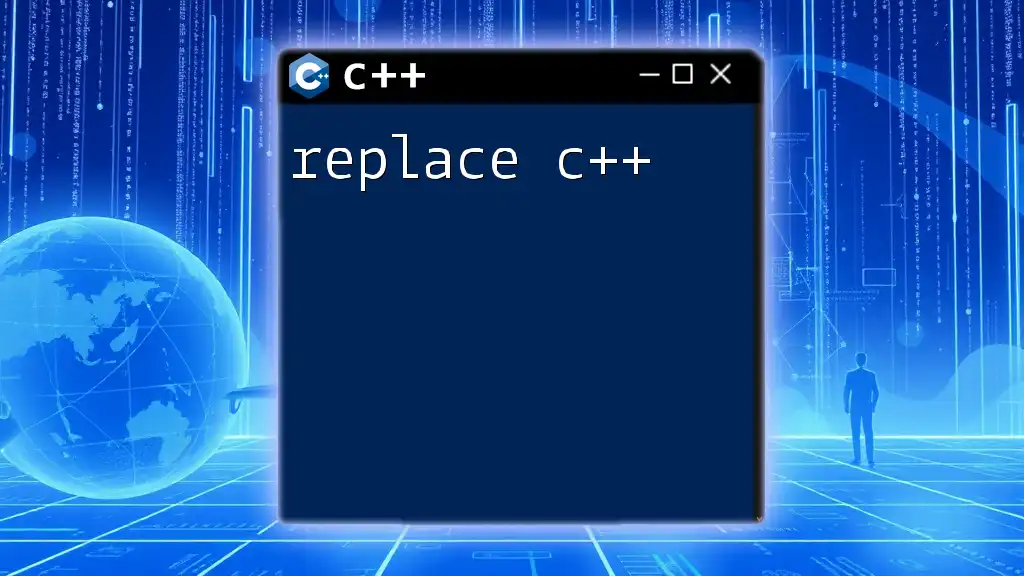
Conclusion
Circular queues provide a robust and efficient solution for managing data in a constrained memory environment, allowing for dynamic queuing and dequeuing without wasted space. As you continue to work with circular queues in C++, it's encouraged to practice implementing different scenarios and experimenting with variations to solidify your understanding.
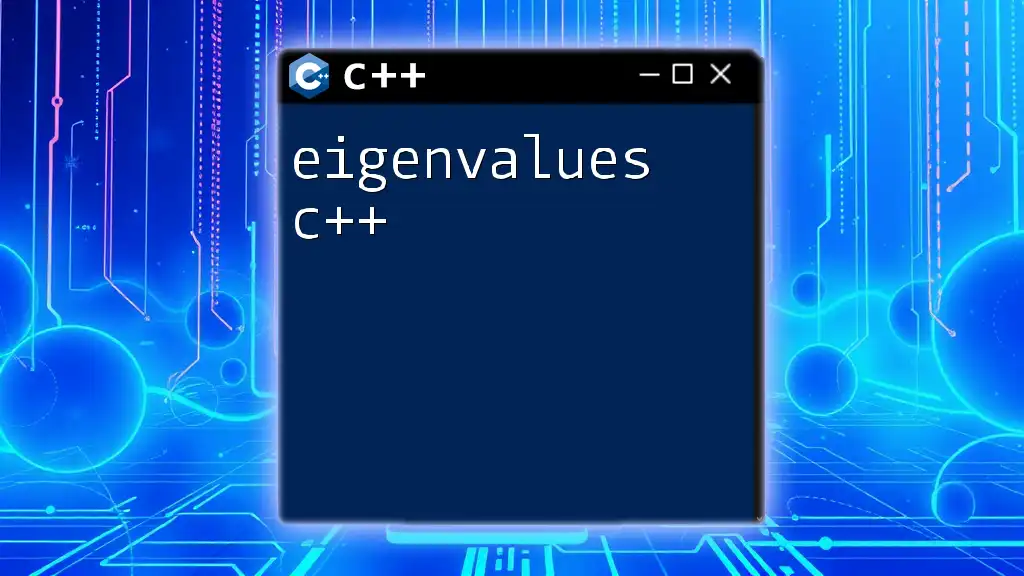
Additional Resources
Recommended Readings and References
Further reading on circular queues, including books, articles, and online tutorials, can significantly help enhance your grasp of the topic. Exploring community forums such as Stack Overflow or dedicated programming websites will also provide you with valuable insights and problem-solving examples.
Sample Projects to Enhance Skills
To deepen your proficiency with circular queues, consider creating small projects that incorporate enqueue and dequeue operations, such as a simple task manager or a print job scheduler. These projects can reinforce your understanding and show the practical application of circular queue data structures.