A thread-safe queue in C++ allows multiple threads to safely enqueue and dequeue items without risking data corruption, typically implemented using mutexes for synchronization.
Here’s a simple implementation using `std::queue` and `std::mutex`:
#include <queue>
#include <mutex>
#include <condition_variable>
template<typename T>
class ThreadSafeQueue {
public:
void enqueue(T value) {
std::lock_guard<std::mutex> lock(mutex_);
queue_.push(std::move(value));
cond_var_.notify_one();
}
bool dequeue(T& value) {
std::lock_guard<std::mutex> lock(mutex_);
if (queue_.empty()) return false;
value = std::move(queue_.front());
queue_.pop();
return true;
}
private:
std::queue<T> queue_;
mutable std::mutex mutex_;
std::condition_variable cond_var_;
};
What is a Thread Safe Queue?
A thread safe queue is a type of data structure that allows multiple threads to access and modify the queue concurrently without running into issues such as race conditions or data corruption. Thread safety is a crucial aspect of modern programming, particularly in scenarios where applications need to perform multiple tasks simultaneously, such as in multi-core processing systems.
The key characteristic of a thread-safe queue is its ability to ensure atomic operations, meaning that the operations on the queue are indivisible, preventing other threads from modifying the data during those operations. This feature is particularly beneficial in scenarios such as task scheduling, where producers generate tasks while consumers process them.
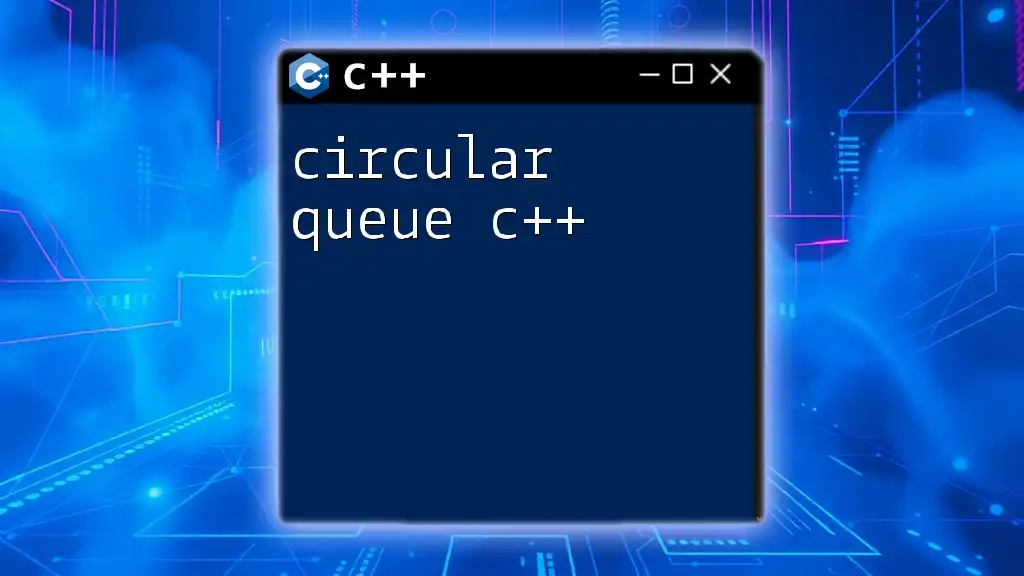
Why Use a Thread Safe Queue?
In today's programming landscape, concurrency is vital for performance, particularly for CPU-bound and I/O-bound applications. By utilizing a thread safe queue, developers can:
-
Avoid race conditions: In a multi-threaded environment, two or more threads might attempt to modify the same data simultaneously, leading to unpredictable outcomes. A thread safe queue mitigates this risk.
-
Enhance performance: A thread safe queue allows multiple threads to enqueue and dequeue items without waiting on each other excessively, thereby optimizing throughput.
-
Scale with ease: As applications grow, thread safe queues can handle increased load and user demands, making them indispensable in the architecture of most software systems.
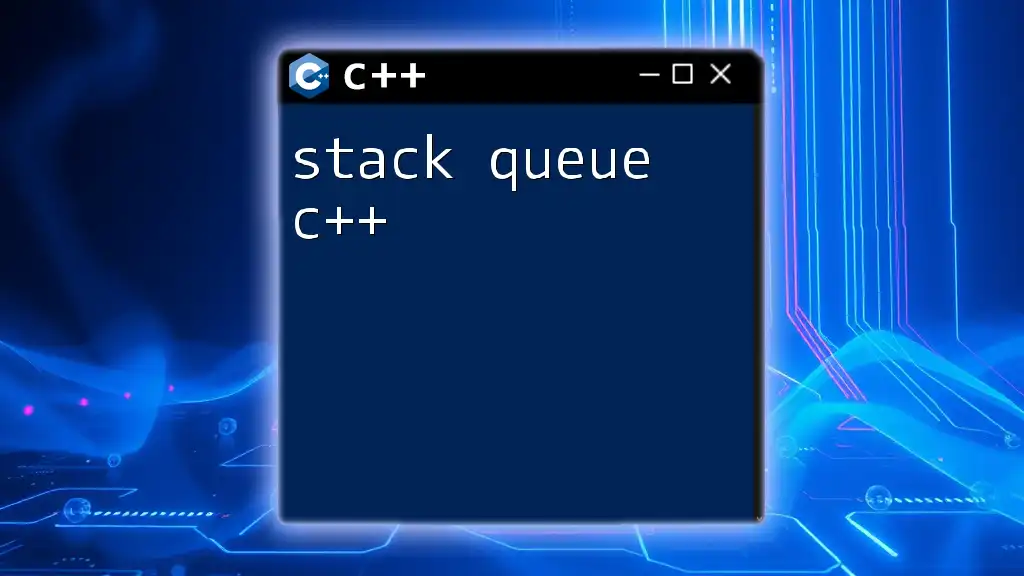
Overview of Multithreading in C++
To fully appreciate the implementation of a thread safe queue in C++, it is essential to understand the basics of multithreading in the language. C++ offers a robust thread library that provides developers with tools to create threads and manage their lifecycle effectively.
Multithreading helps programs perform operations concurrently, effectively utilizing system resources. However, with this power comes complexity, as developers must consider various concurrency issues. Some common problems faced in multithreading include:
-
Race conditions: Occur when multiple threads access shared data simultaneously, leading to incorrect results.
-
Deadlocks: A situation where two or more threads are waiting indefinitely for resources held by each other.
-
Starvation: When a thread cannot gain regular access to resources and is perpetually denied.
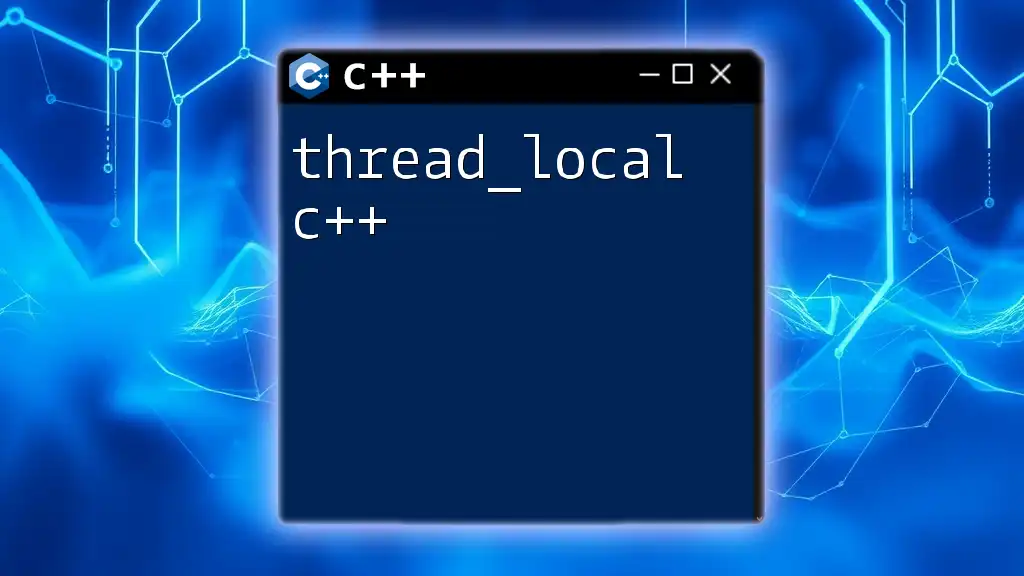
Implementing a Thread Safe Queue
Before diving into the logic behind a thread safe queue, it's crucial to understand what constitutes a standard queue. A queue operates based on the FIFO (First-In-First-Out) principle, meaning that the first item added to the queue will be the first one to be removed. The primary operations of a queue include enqueue (adding an element) and dequeue (removing an element).
Design Choices for Thread Safety
When designing a thread safe queue, several considerations must be made regarding how to achieve thread safety:
-
Mutex vs. Spinlocks: A mutex is a widely-used locking mechanism that allows only one thread to access a critical section of code at a time. Spinlocks can be more efficient in certain scenarios where thread contention is expected to be low.
-
Condition Variables: These are synchronization primitives that allow threads to wait for certain conditions (like the queue being non-empty) before proceeding, thus avoiding busy-waiting.
-
Performance and Scalability: The design must be optimized for how many threads will access the queue simultaneously and how they will behave under load.
Example: Simple Thread Safe Queue Implementation
To illustrate the concepts above, consider the following implementation of a simple thread safe queue:
#include <queue>
#include <mutex>
#include <condition_variable>
template<typename T>
class ThreadSafeQueue {
public:
void enqueue(T value);
void dequeue(T& value);
bool isEmpty() const;
private:
std::queue<T> queue_;
mutable std::mutex mutex_;
std::condition_variable cond_;
};
In this code snippet, we define a template class `ThreadSafeQueue`, which can hold elements of any data type. The class encapsulates the queue and its synchronization mechanisms.
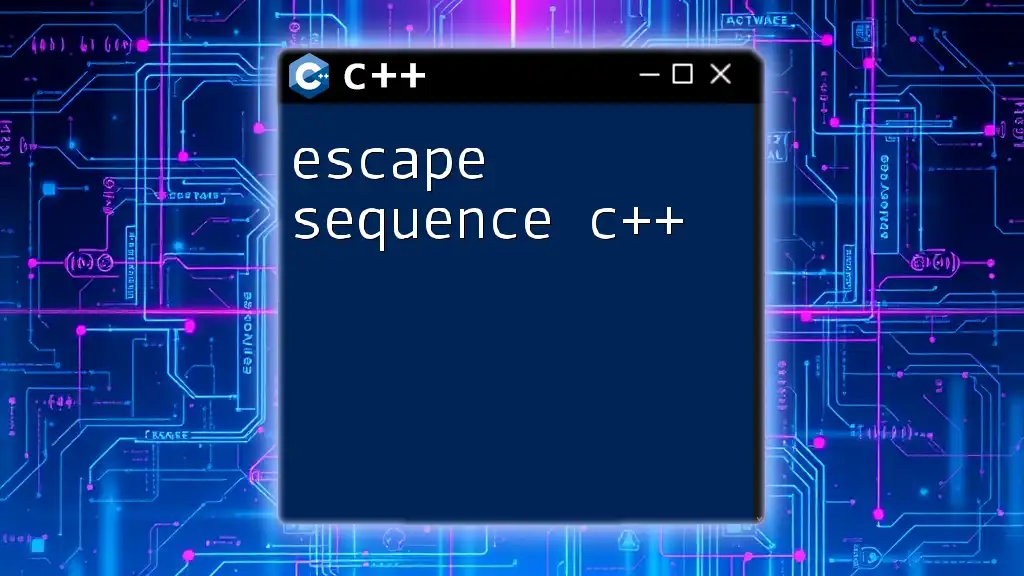
Enqueue and Dequeue Operations
The two main operations of a queue are enqueue (to add an item) and dequeue (to remove an item). Let’s detail the implementation of these methods.
Enqueue Operation
The enqueue function must be designed to allow multiple threads to add items safely. Here's how it’s done:
template<typename T>
void ThreadSafeQueue<T>::enqueue(T value) {
std::lock_guard<std::mutex> lock(mutex_);
queue_.push(std::move(value));
cond_.notify_one();
}
In this code, we use a `lock_guard` that automatically manages the mutex's lifecycle, ensuring the mutex is locked while the operation occurs. After adding the item to the queue, we notify at least one waiting thread (if any) that there is now an item in the queue.
Dequeue Operation
The dequeue function allows a thread to remove an item from the queue safely. Below is its implementation:
template<typename T>
void ThreadSafeQueue<T>::dequeue(T& value) {
std::unique_lock<std::mutex> lock(mutex_);
cond_.wait(lock, [this] { return !queue_.empty(); });
value = std::move(queue_.front());
queue_.pop();
}
Here we use a `unique_lock`, which is similar to `lock_guard`, but allows greater flexibility (like being able to unlock manually). We call `wait` on the condition variable, which blocks the thread until the queue is not empty, ensuring that we do not perform operations on an empty queue.
Checking if the Queue is Empty
It is also essential to implement a mechanism to check if the queue is empty. This can be done as follows:
template<typename T>
bool ThreadSafeQueue<T>::isEmpty() const {
std::lock_guard<std::mutex> lock(mutex_);
return queue_.empty();
}
This method requires a lock to ensure that the state of the queue does not change while it is being checked, guaranteeing accuracy.
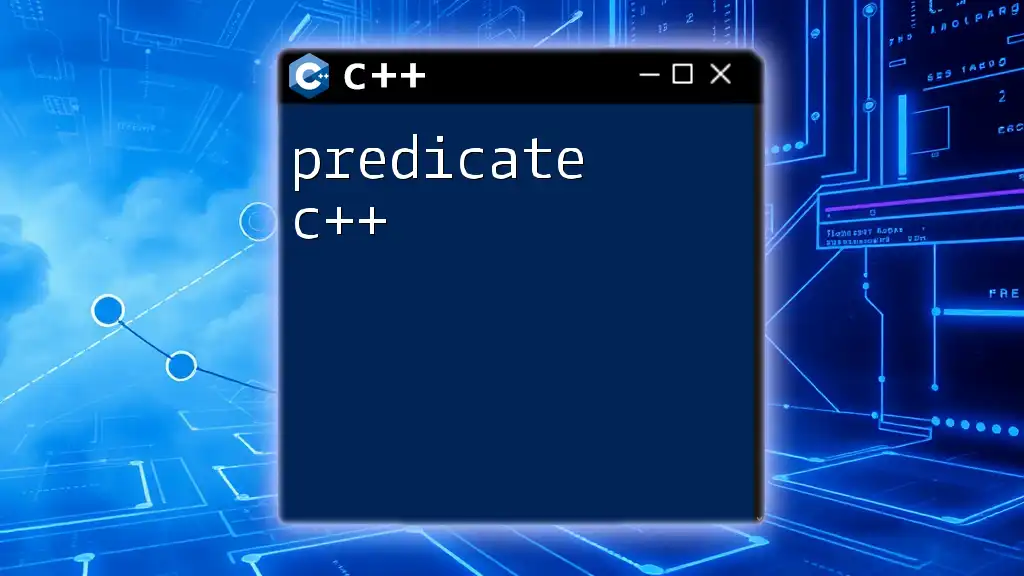
Best Practices for Using Thread Safe Queues
Avoiding Common Pitfalls
When working with thread safe queues, developers must be cautious of overusing locks, which can lead to performance bottlenecks and blocked threads. Striking an appropriate balance in lock granularity is essential.
Balancing Performance and Safety
Design choices must reflect the expected load on the queue. Using more efficient synchronization mechanisms or reducing lock duration can drastically impact the performance of the queue in real-world scenarios.
Testing and Debugging
Robust testing of a thread safe queue implementation is crucial. Developers should consider writing tests that simulate high levels of concurrency to ensure the implementation holds up under stress. Additionally, debugging tools such as thread sanitizer can help detect concurrency issues during the development process.
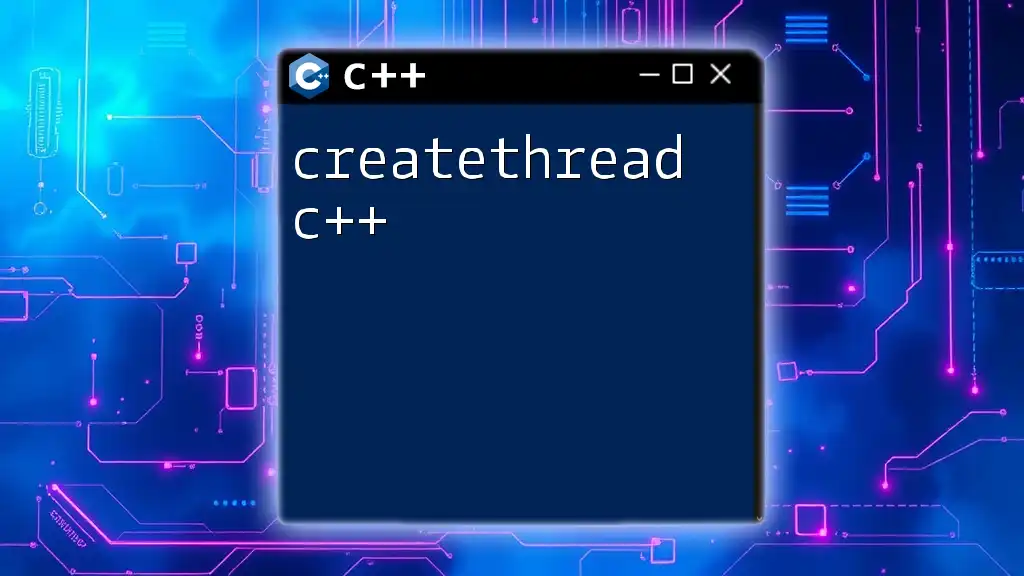
Conclusion
A thread safe queue in C++ is an indispensable tool in concurrent programming. It provides a means to manage shared resources effectively while minimizing the risks associated with multi-threaded access. By understanding the fundamental design principles and best practices of thread safe queues, developers can enhance the reliability and performance of their applications.
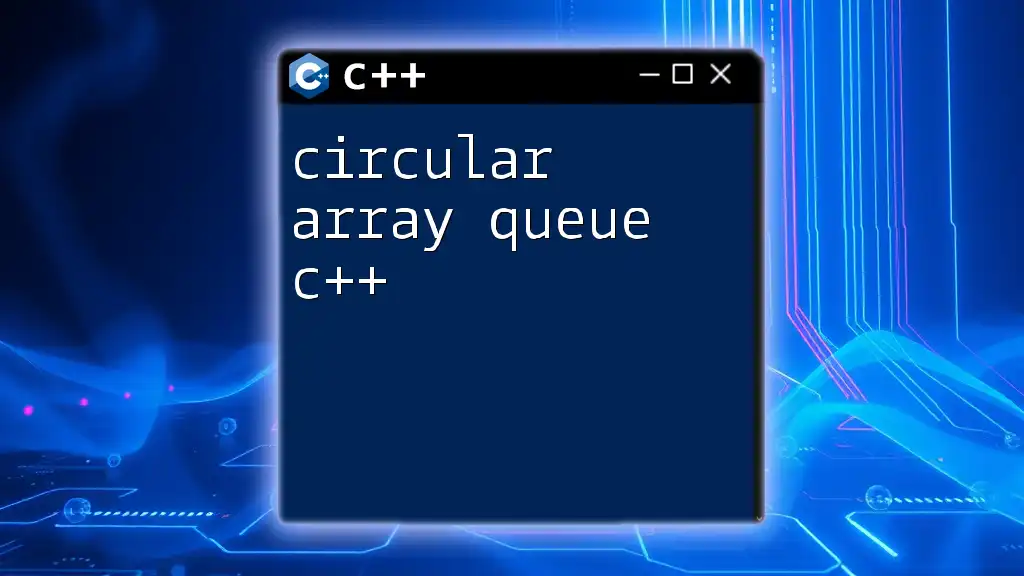
Further Reading and Resources
For those looking to deepen their knowledge of thread safe data structures and concurrent programming in C++, consider exploring online courses, literature focused on C++ standards, and engaging with community forums. Practical experience and real-world examples are invaluable in mastering this skill set.
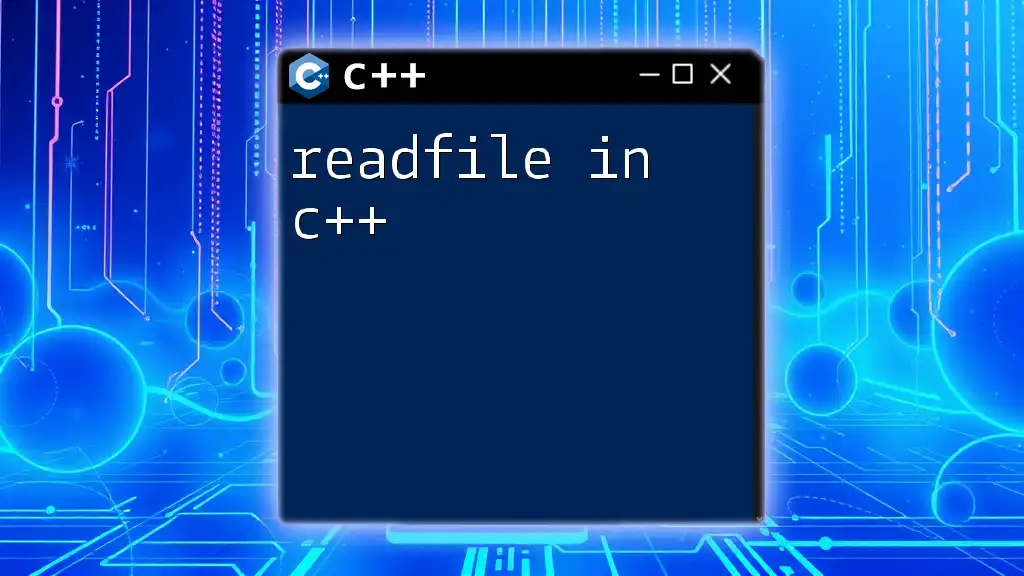
Call to Action
Join our growing community of programmers keen on mastering C++ concepts. Sign up for our newsletter to stay updated on upcoming courses and fresh content tailored to enhance your programming journey!