The `fstream` library in C++ allows you to create, read, and write files, and here's a simple example of how to write text to a file using `fstream`:
#include <fstream>
int main() {
std::ofstream file("example.txt");
file << "Hello, World!";
file.close();
return 0;
}
Understanding `fstream`
What is `fstream`?
In C++, `fstream` is a powerful library that allows for both input and output operations on files. It combines the capabilities of `ifstream` (for reading) and `ofstream` (for writing) into a single class that can handle both. To use `fstream`, make sure to include its header file in your code:
#include <fstream>
This will allow you to access the functionalities necessary to read from and write to files seamlessly.
Types of File Streams in C++
C++ provides three main types of file streams:
- Input File Streams (`ifstream`): Used for reading data from files.
- Output File Streams (`ofstream`): Used for writing data to files.
- Bidirectional Streams (`fstream`): Allows both reading from and writing to files.
Understanding these types will help in selecting the most appropriate one based on your needs.
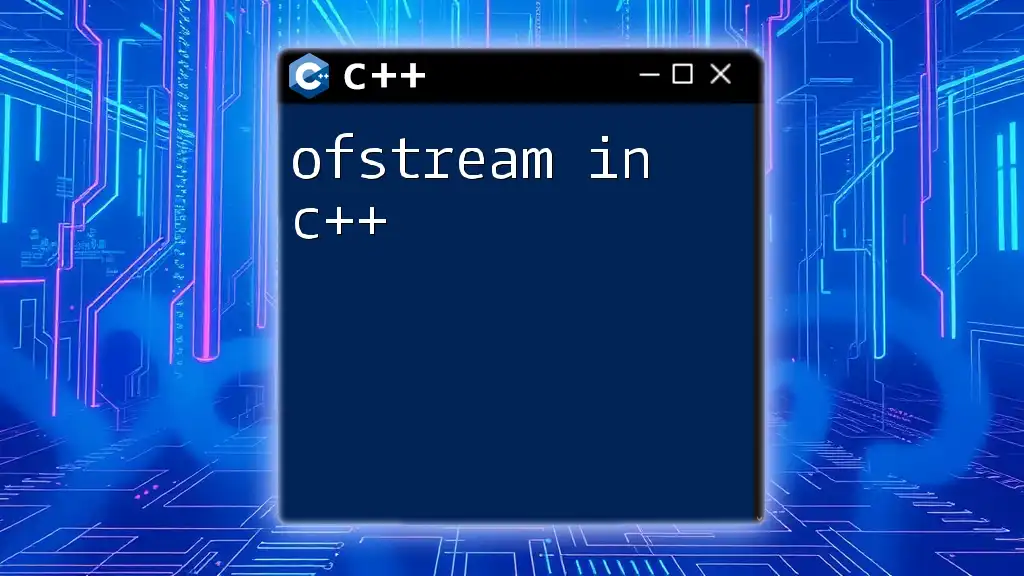
Using `ofstream` to Write to Files
Opening a File for Writing
To begin writing to a file, you need to create an instance of the `ofstream` class. This is done by specifying the filename:
std::ofstream outFile("example.txt");
It is crucial to check if the file has been opened successfully. If the file cannot be opened, attempting to write to it would lead to errors. Therefore, always use an if-statement to verify:
if (outFile.is_open()) {
// Proceed with writing
} else {
std::cerr << "Could not open the file!" << std::endl;
}
Writing Data to Files
Writing Strings
To write strings to a file, you can utilize the stream insertion operator `<<`. This is both intuitive and convenient:
outFile << "Hello, World!" << std::endl;
The use of `std::endl` not only inserts a newline character but also flushes the output buffer, ensuring that all written data is immediately saved to the file.
Writing Variables
When you need to write variable content to a file, it can be done using a similar approach:
int number = 42;
outFile << "The answer is: " << number << std::endl;
Here, the variable `number` is dynamically included in the output alongside a descriptive string.
Writing Multiple Lines
If writing multiple lines is your goal, a loop can be very effective:
for (int i = 0; i < 5; ++i) {
outFile << "Line " << i + 1 << std::endl;
}
This example demonstrates how to generate output dynamically, which is especially useful for bulk data entries.
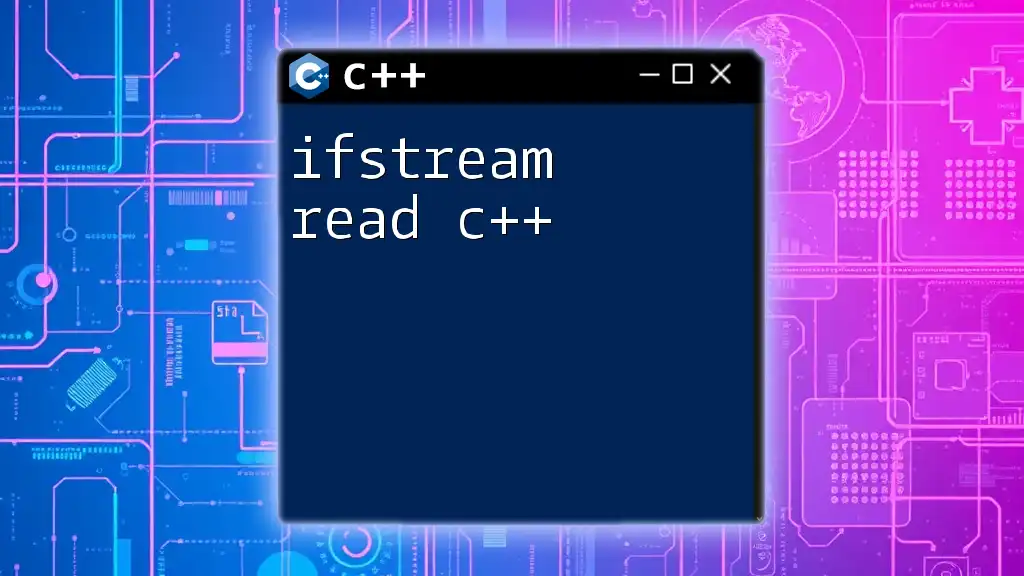
Closing a File
Importance of Closing the File
It is essential to close the file after completing your write operations to ensure that all data is saved and resources are freed. Failing to do this might result in lost data or corrupt files.
Using `close()`
To close a file, simply call the `close()` method on your `ofstream` object:
outFile.close();
After closing, you can safely assume that all operations are finalized. While it is not strictly necessary to check if closure was successful, it is a good habit to maintain data integrity.
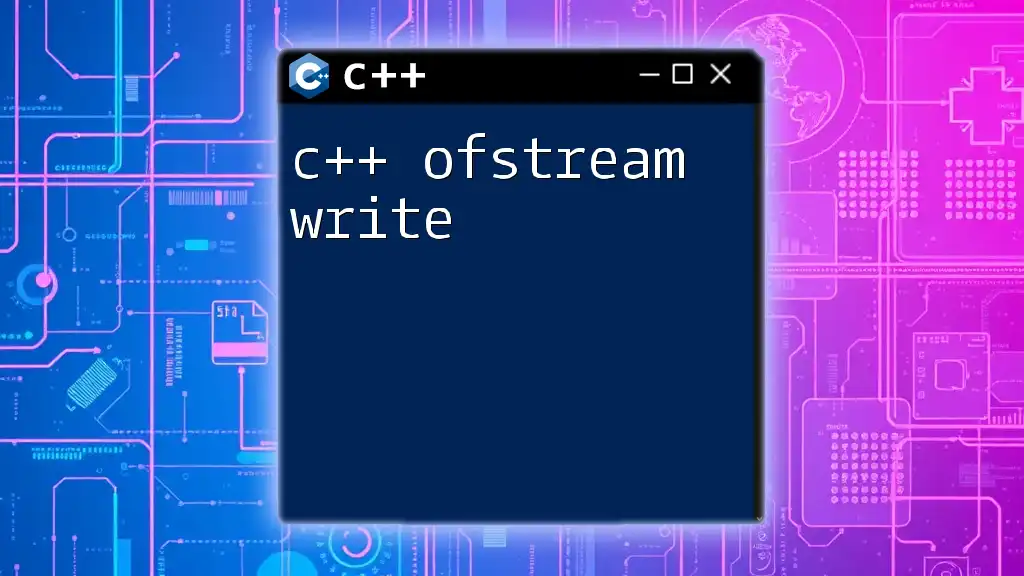
Error Handling
Checking for Errors
When conducting file operations, it is prudent to account for potential errors. The `fail()` method can be employed to determine if the file operations encountered any issues:
if (outFile.fail()) {
std::cerr << "Error writing to file!" << std::endl;
}
This enables you to capture and handle errors more gracefully, enhancing the user experience.
Exception Handling
For a more robust error handling strategy, consider using `try-catch` blocks. This allows you to manage exceptions that may arise during file operations:
try {
outFile.open("example.txt");
// Write operations
} catch (const std::ios_base::failure& e) {
std::cerr << "File operation failed: " << e.what() << std::endl;
}
This technique provides a structured approach to error management, ensuring your program can respond effectively to issues without crashing.
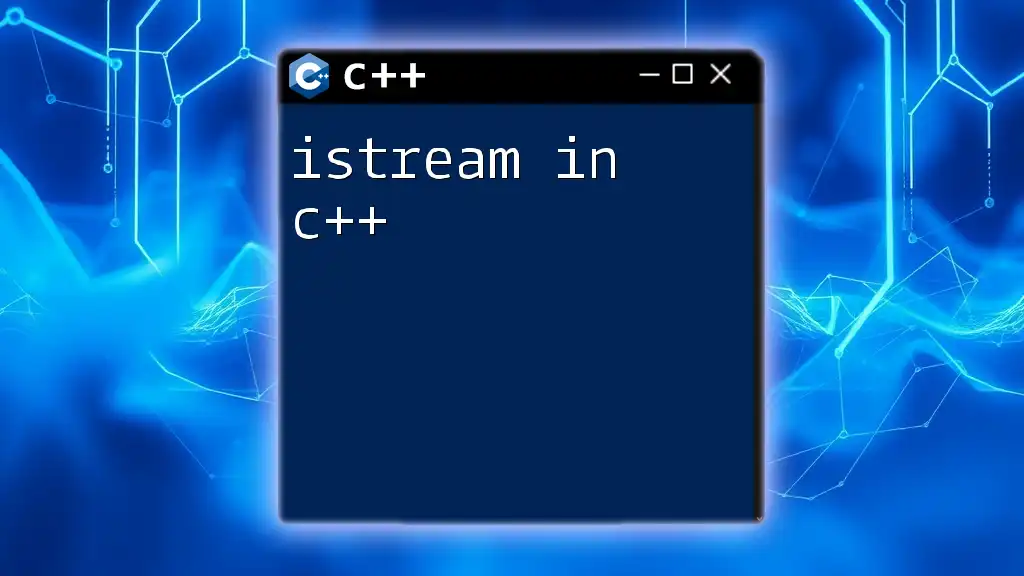
Best Practices with `fstream`
Choosing the Right Mode
When opening a file, it’s important to choose the correct mode based on your requirements. For example, appending data to an existing file instead of overwriting can be achieved by using `ios::app`:
std::ofstream outFile("example.txt", std::ios::app);
Understanding these modes helps in avoiding unintentional data loss.
Keeping Files Organized
File naming conventions and directory management play critical roles in file handling. Using descriptive names along with structured directories can prevent confusion, particularly in larger projects or applications.
Utilizing RAII for Resource Management
Resource Acquisition Is Initialization (RAII) is a programming idiom that ensures resource management is handled properly. By creating the `ofstream` object in a local scope, the file is automatically closed when the object goes out of scope:
{
std::ofstream outFile("example.txt");
// Write operations
} // Automatically closed when scope ends
This automatically takes care of cleanup, promoting safer code practices.
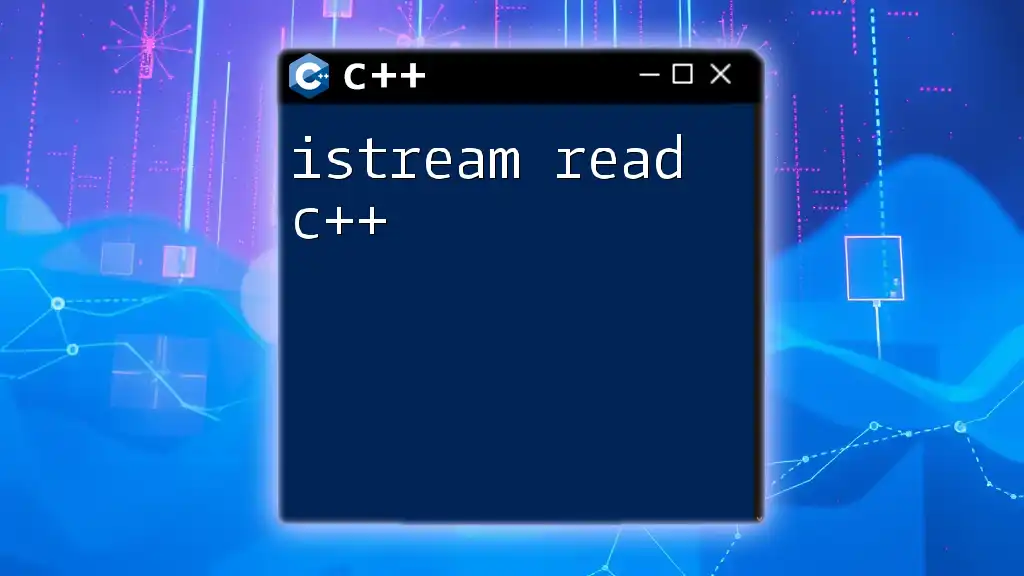
Sample Project: Writing a Simple Contact Book
Project Overview
To put the concepts learned into practice, let’s build a simple contact book application that allows users to add contacts, each consisting of a name and phone number.
Code Structure
The following code snippet illustrates how to append new contacts to a file named `contacts.txt`:
#include <iostream>
#include <fstream>
#include <string>
void addContact(const std::string& name, const std::string& phone) {
std::ofstream outFile("contacts.txt", std::ios::app);
if (outFile.is_open()) {
outFile << "Name: " << name << ", Phone: " << phone << std::endl;
outFile.close();
} else {
std::cerr << "Error opening file!" << std::endl;
}
}
In this example, the `addContact` function creates or appends to the file, ensuring that all existing data remains intact.
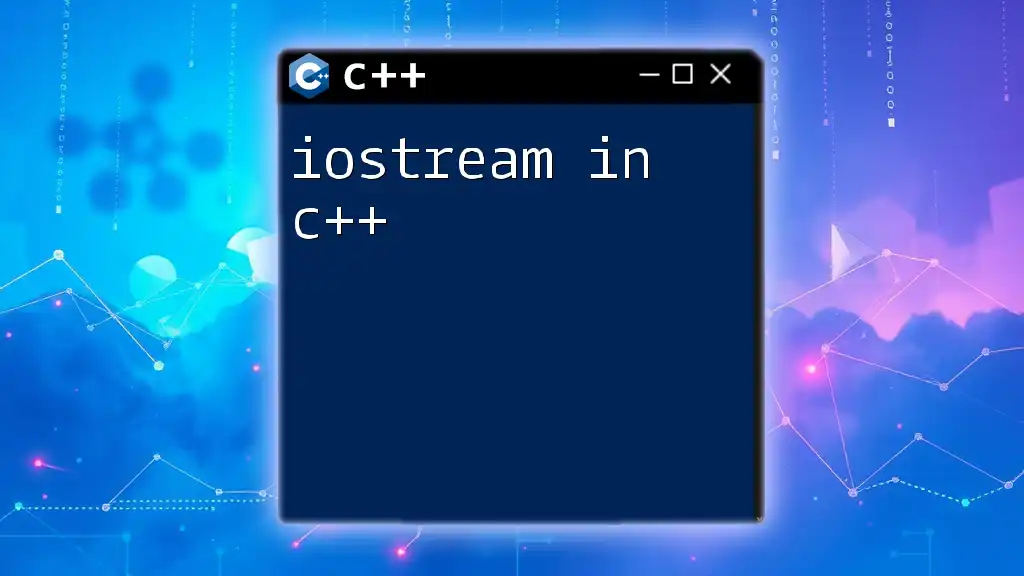
Conclusion
In summary, understanding fstream write in C++ is invaluable when it comes to file handling. With `ofstream`, you can efficiently create and manage file outputs while ensuring data integrity through proper error handling and file management practices.
As you continue your C++ journey, take the time to experiment with the techniques discussed in this guide, and don’t hesitate to reach out for support if you encounter challenges along the way. Embrace the learning process, and happy coding!
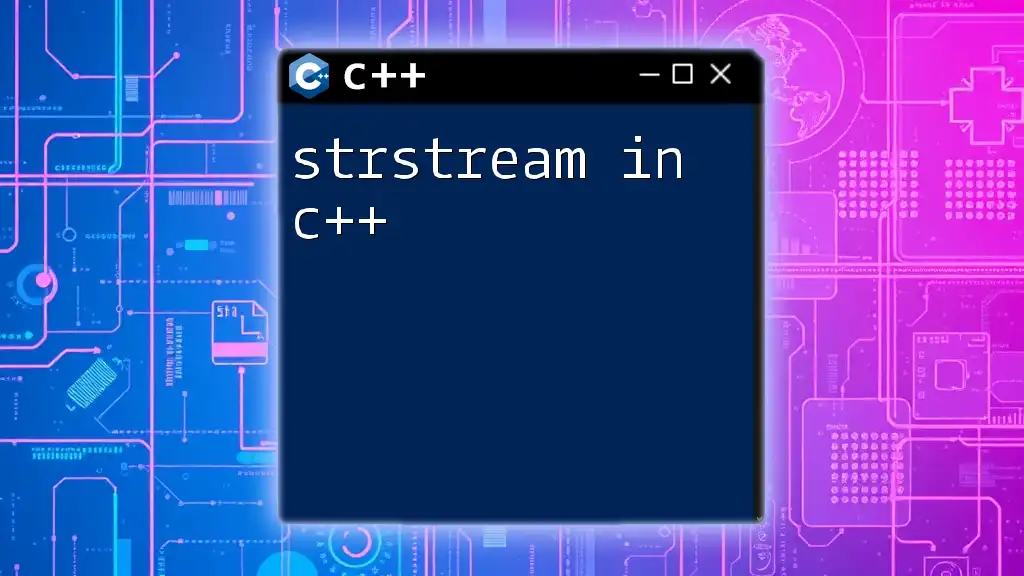
Additional Resources
For further reading and exploration, consider delving into books focused on C++ programming, online tutorials, and courses that dive deeper into file handling and other advanced topics. Enrich your knowledge and skills to become proficient in utilizing `fstream` and beyond!