In C++, the `fstream` library is used to read from files, allowing you to easily open a file, read its contents, and then close it after you are done.
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream inputFile("example.txt");
std::string line;
if (inputFile.is_open()) {
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
inputFile.close();
} else {
std::cerr << "Unable to open file" << std::endl;
}
return 0;
}
Understanding fstream
What is fstream?
`fstream` is an integral part of C++’s file handling capabilities. It represents a file stream class that allows developers to perform input and output operations on files using a single object. The functionality of `fstream` is encompassed in three primary classes: `ifstream`, `ofstream`, and `fstream`.
- `ifstream` is used for input operations, primarily reading from files.
- `ofstream` is used for output operations, primarily writing to files.
- `fstream` serves dual purposes, allowing both reading and writing.
This makes `fstream` a versatile choice for file operations when you need to perform both actions.
Why Use fstream?
Using `fstream` offers several advantages for file handling, including:
- Simplicity: It allows you to handle both reading and writing without maintaining separate objects.
- Flexibility: You can easily switch modes (read/write) without needing to close and reopen different streams.
- Performance: `fstream` can efficiently handle buffered I/O, which can speed up file operations in many scenarios.
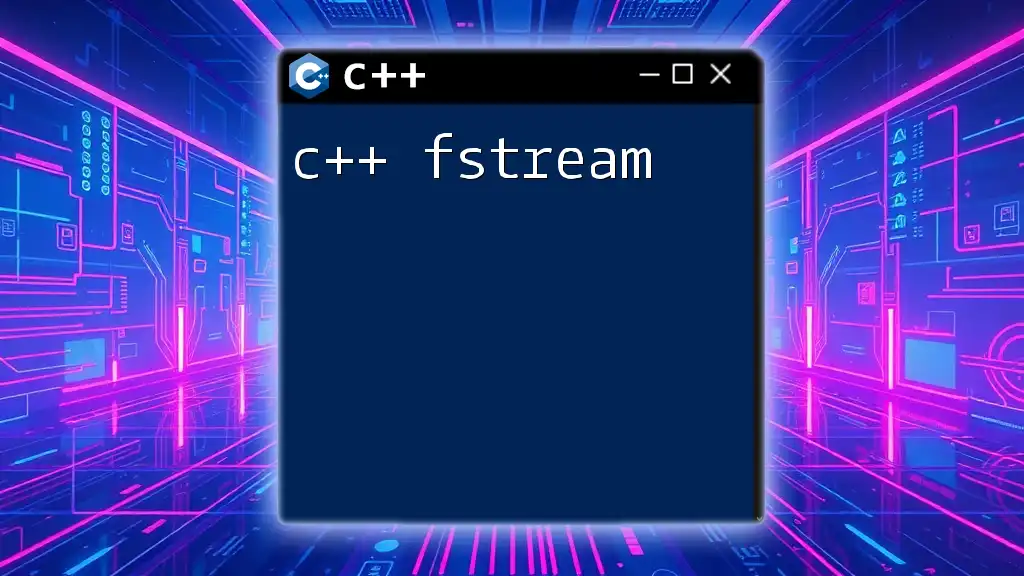
Setting Up Your Environment
Necessary Includes
To utilize `fstream`, certain header files must be included. The required headers are:
#include <iostream>
#include <fstream>
This allows access to standard input-output streams and file stream capabilities.
Compiling Your Code
When compiling C++ code that involves file handling, you often need to use a command like:
g++ -o program program.cpp
This command compiles the `program.cpp` file into an executable named `program`. Be sure to have your compiler installed and configured before running the command.
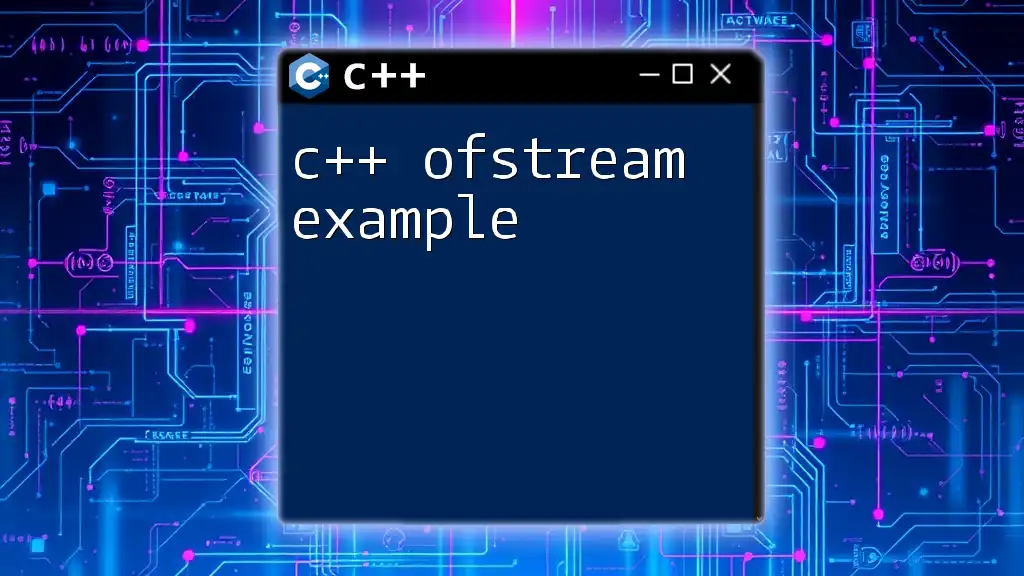
Opening a File with fstream
Syntax for Opening a File
To begin using `fstream`, you need to open a file, which can be accomplished using the `open()` method. The syntax follows:
std::fstream file;
file.open("example.txt", std::ios::in);
Here, `"example.txt"` is the name of the file you want to read, and `std::ios::in` indicates that the file is being opened for input operations.
Different Modes of Opening a File
Input Mode (`std::ios::in`) - This is used for reading from a file.
Output Mode (`std::ios::out`) - This allows writing data to a file. If the file already exists, it will be truncated.
Append Mode (`std::ios::app`) - This opens the file for writing, but data will be added at the end instead of overwriting existing content.
You can also combine these modes. For instance, if you want to read from and write to the same file, use:
file.open("example.txt", std::ios::in | std::ios::out);
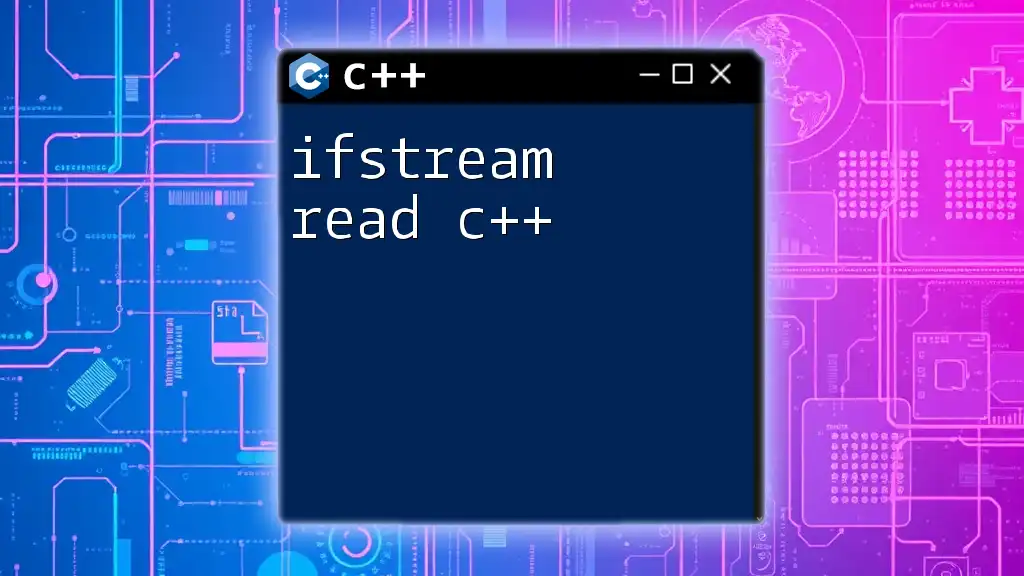
Reading from a File
Basic Reading Techniques
Using getline
To read an entire line from a file, the `getline()` function is your best bet. This function reads characters until it encounters a newline. Here's how to use it:
std::string line;
if (file.is_open()) {
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
}
In this code snippet, the program first checks if the file is successfully opened. If it is, it enters a loop where it reads each line and prints it.
Reading Word by Word
If you need to read word by word, you can utilize the input operator (`>>`) like so:
std::string word;
while (file >> word) {
std::cout << word << std::endl;
}
This method reads the next word from the file until it encounters whitespace. It’s useful for processing files where you are concerned about individual words rather than entire lines.
Error Handling
Error Handling is crucial. Always verify if a file was successfully opened before performing operations on it. Here’s how to check:
if (!file) {
std::cerr << "Error opening file!" << std::endl;
}
In addition, you should be aware of various flags that tell you the current state of the file:
- `file.eof()` checks for the end of the file.
- `file.fail()` checks for logical errors such as trying to read from a file that is not open.
- `file.bad()` checks for unreadable conditions, such as hardware failure or corruption.
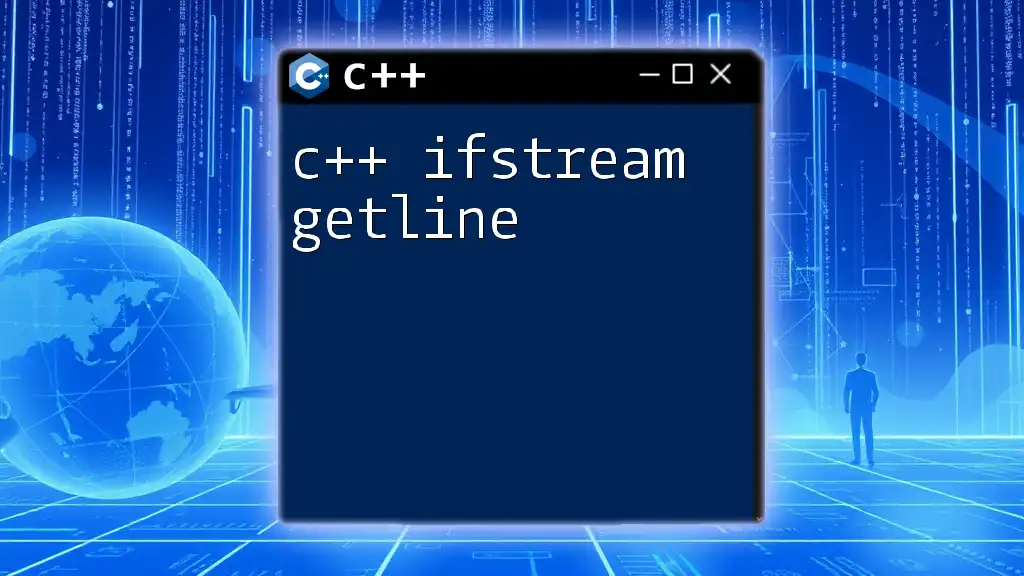
Closing a File
Why is it Important?
Closing a file is imperative to avoid memory leaks and to ensure that data is properly written and saved. When the file is closed, the system disassociates the file stream from the file, thereby releasing any resources associated with it.
How to Close a File
Closing a file in C++ is straightforward:
file.close();
It’s a good practice to always close files once you're done with them.
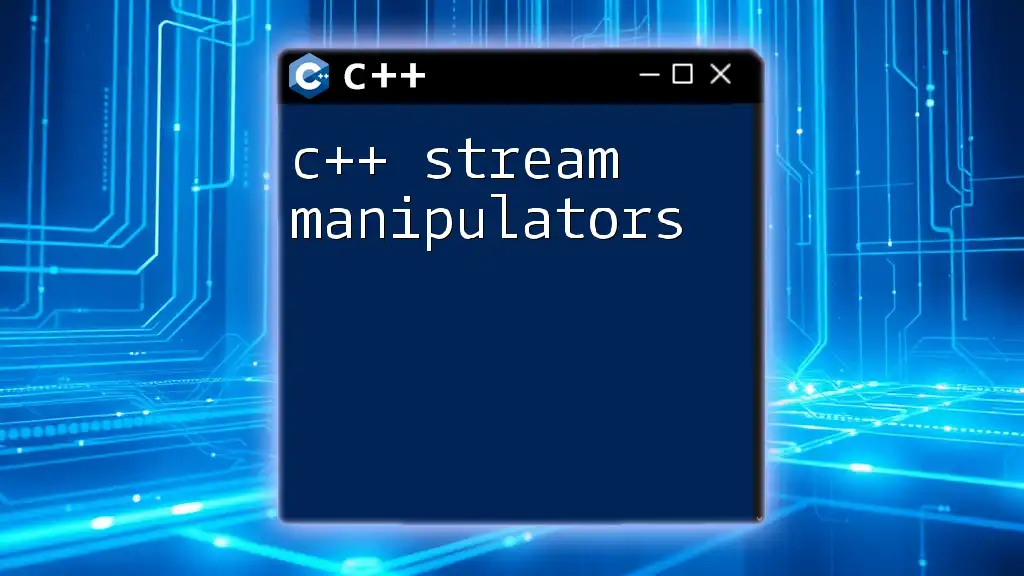
Real-World Applications
Reading Configuration Files
In many applications, configuration settings are stored in external files. Using `fstream`, you can readily read user-defined settings into your program. This allows for customizability and flexibility in how your application operates.
CSV File Reading
Reading and parsing CSV files can easily be accomplished with `fstream`. Here’s a simple read example for a CSV:
std::string line;
while (std::getline(file, line)) {
std::stringstream ss(line);
std::string item;
while (std::getline(ss, item, ',')) {
std::cout << item << std::endl; // Process each field
}
}
This snippet reads a line, then splits the line into items based on the comma delimiter, making it easier to handle structured data.
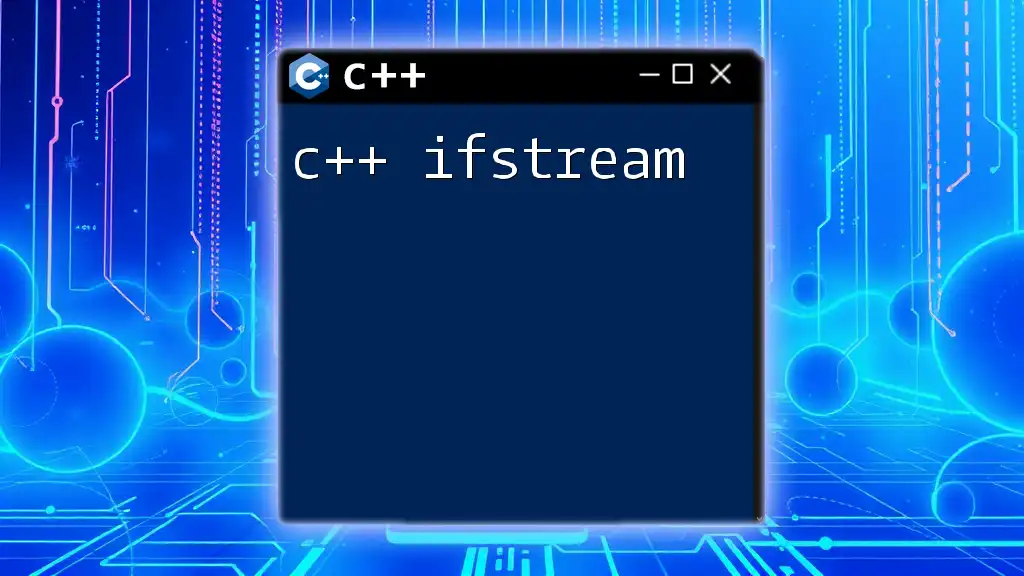
Best Practices
File Handling Best Practices
- Always check if the file is open. It’s simple but crucial to avoid runtime errors.
- Use exceptions for error handling. Consider wrapping file operations in try-catch blocks for graceful error management.
- Close files in a timely manner. Do this to free up resources and maintain system stability.
Performance Considerations
Consider using buffered versus unbuffered I/O based on the size and frequency of file access in your application. Buffered I/O can significantly enhance performance for larger files, while unbuffered may be necessary for real-time processing scenarios.
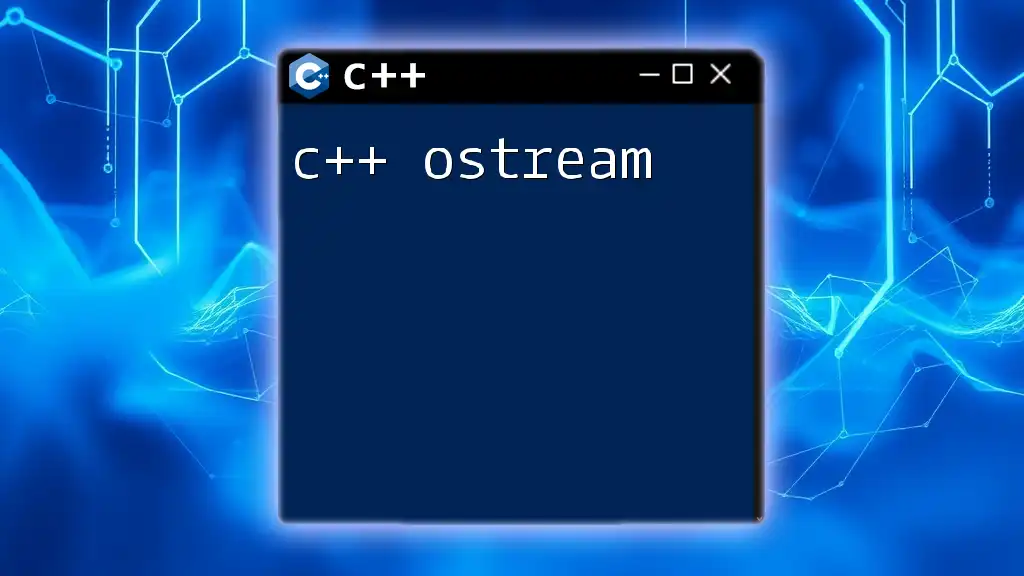
Conclusion
From basic file operations to reading complex data structures like CSV files, the `c++ fstream read` capability unlocks a world of possibilities in file handling. Whether you're developing a small application or a robust system, mastering these techniques will empower you to effectively manipulate file data in your C++ projects.
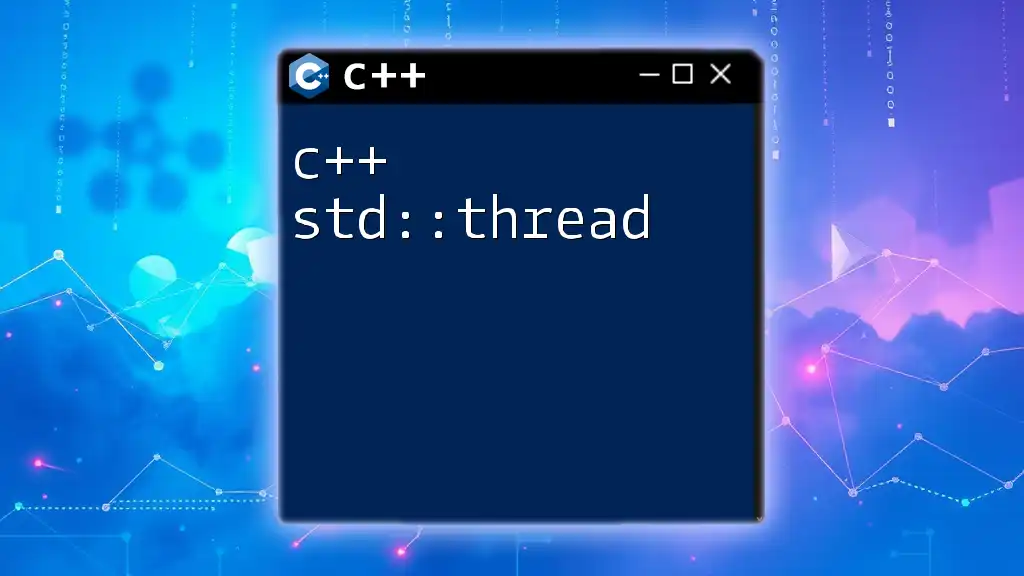
Additional Resources
For further learning, consider delving into recommended books and online resources such as free coding platforms or tutorials that provide deeper insights into C++ file handling, particularly focusing on the `fstream` class.
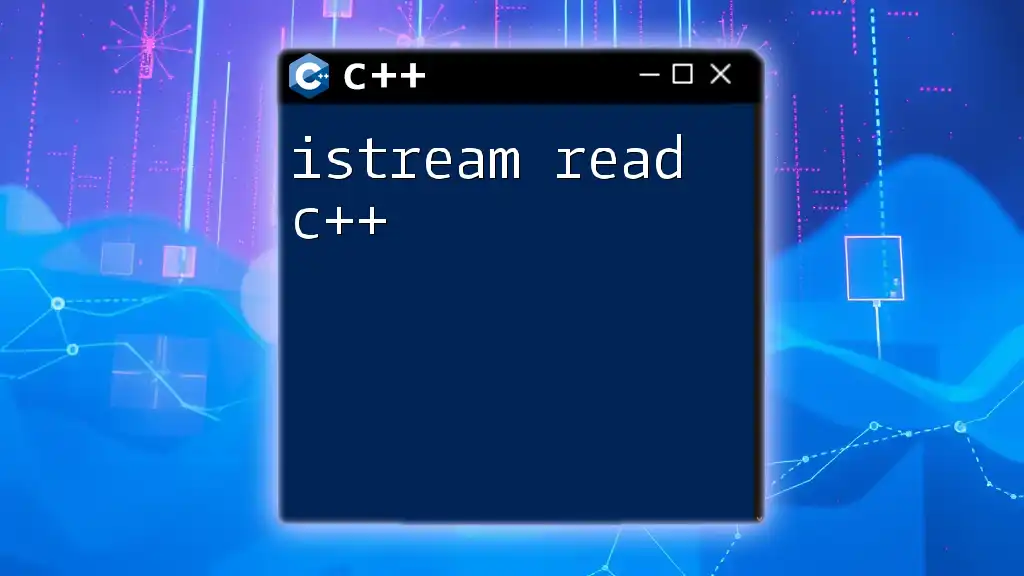
FAQs
Common Questions about fstream Reading
-
What happens if I try to read from a file that does not exist? The program will fail silently unless you’ve implemented error handling measures to check if the file opened correctly.
-
How do I read binary files using fstream? To read binary files, you need to open the file in binary mode using `std::ios::binary`. This allows you to read and write raw bytes directly.
-
Can I read from multiple files simultaneously? Yes, you can create multiple `fstream` objects, each associated with a different file, and manage them independently in your program.